Mastering Go Language Interview Prep: Essential Tips and Strategies for Success
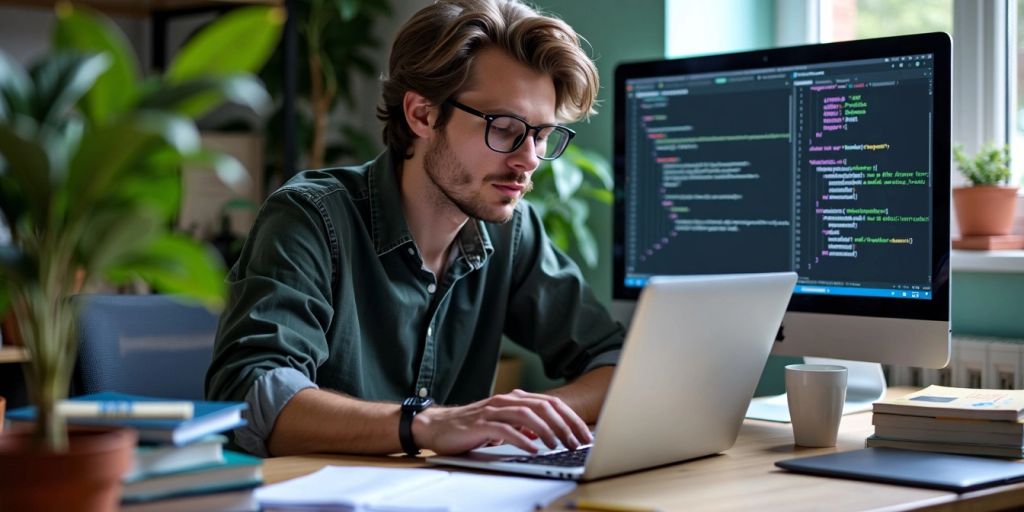
Preparing for interviews in Go language can be challenging but rewarding. This guide offers essential tips and strategies to help you succeed in your Go interviews. From understanding the interview process to mastering key concepts, this article will equip you with the knowledge you need to shine during your interviews.
Key Takeaways
- Know the different types of interviews you might face and how to prepare for each.
- Practice explaining your thought process clearly and listen carefully to the interviewer.
- Stay calm and confident by using relaxation techniques and positive body language.
- Keep your coding skills sharp by practicing regularly and learning from online resources.
- Avoid common mistakes like not practicing enough and failing to communicate well.
Understanding the Go Language Interview Process
Types of Interviews You May Encounter
When preparing for a Go language interview, you might face several types of interviews:
- Technical Interviews: Focus on coding skills and problem-solving.
- Behavioral Interviews: Assess your soft skills and cultural fit.
- System Design Interviews: Evaluate your ability to design scalable systems.
What Interviewers Are Looking For
Interviewers typically seek candidates who can demonstrate:
- Strong coding skills in Go.
- A solid understanding of concurrency and goroutines.
- The ability to explain your thought process clearly.
Having a comprehensive list of Golang interview questions can help you effectively assess candidates’ skills and knowledge.
Common Pitfalls to Avoid
To succeed in your Go interviews, be mindful of these common mistakes:
- Not practicing enough: Regular coding practice is essential.
- Failing to communicate: Always explain your thought process.
- Ignoring edge cases: Consider all possible scenarios in your solutions.
Remember, interviews are not just about getting the right answer; they are also about showing your problem-solving approach and communication skills.
Essential Go Language Concepts to Master
Core Syntax and Semantics
Understanding the core syntax of Go is crucial for any developer. Here are some key points to remember:
- Go is a statically typed language, meaning types are checked at compile time.
- Variable declaration can be done using the
var
keyword or shorthand:=
. - The zero value is automatically assigned to variables that are declared but not initialized.
Understanding Goroutines and Concurrency
Goroutines are lightweight threads that allow you to run multiple tasks at once. This is important for efficient programming. Here’s how to use them:
- Start a goroutine with the
go
keyword before a function call. - Use channels to communicate between goroutines safely.
- The
select
statement can manage multiple channel operations effectively.
Error Handling in Go
Error handling in Go is straightforward but essential. Here are some strategies:
- Always check for errors returned by functions.
- Use the
defer
statement to ensure cleanup actions are performed. - Consider creating custom error types for better clarity.
Mastering these concepts will help you tackle top Golang interview questions you must be prepared for.
By focusing on these essential concepts, you will build a strong foundation for your Go programming skills and be better prepared for interviews.
Data Structures and Algorithms in Go
Key Data Structures to Know
Understanding basic data structures is crucial for any Go programmer. Here are some essential ones:
- Array: A fixed-size collection of elements.
- Slice: A flexible, dynamic array that can grow and shrink.
- Map: A collection of key-value pairs for efficient data retrieval.
Data Structure | Description | Flexibility |
---|---|---|
Array | Fixed size | Low |
Slice | Dynamic size | High |
Map | Key-value pairs | High |
Common Algorithmic Patterns
When working with data structures, you will often encounter common patterns:
- Searching: Finding an element in a data structure.
- Sorting: Arranging elements in a specific order.
- Traversal: Visiting each element in a data structure.
Mastering these patterns will help you solve problems more efficiently. They are the foundation of many algorithms.
Time and Space Complexity Analysis
Understanding the efficiency of your algorithms is key. Here are some points to consider:
- Time Complexity: How the runtime of an algorithm increases with input size.
- Space Complexity: How the memory usage of an algorithm increases with input size.
- Big O Notation: A way to express time and space complexity.
In Go, knowing how to analyze these complexities will help you write better code and prepare for interviews. Advanced data structures can also play a significant role in optimizing your solutions, so be sure to explore them as well!
Effective Problem-Solving Techniques
Breaking Down the Problem
When faced with a coding challenge, it’s essential to break it down into smaller parts. This makes it easier to tackle each section step by step. Here’s how you can do it:
- Understand the problem: Read the question carefully and make sure you know what is being asked.
- Identify inputs and outputs: Determine what data you will receive and what you need to return.
- Create a plan: Outline the steps you will take to solve the problem before jumping into coding.
Writing Efficient Code
Writing code that is not only correct but also efficient is crucial. Here are some tips:
- Use built-in functions: They are often optimized and can save you time.
- Avoid unnecessary loops: Try to minimize the number of iterations in your code.
- Optimize data structures: Choose the right data structure for the task to improve performance.
Testing and Debugging Your Solutions
Testing your code is just as important as writing it. Here’s how to ensure your solution works:
- Write test cases: Create different scenarios to test your code thoroughly.
- Use debugging tools: Familiarize yourself with tools that can help you find errors quickly.
- Review your code: Take a moment to look over your code for any mistakes before submitting it.
Remember, practicing problem-solving is key to improving your skills. Regularly challenge yourself with new problems to build confidence and expertise.
By mastering these techniques, you can enhance your problem-solving abilities and perform better in interviews. Practice makes perfect, so keep solving problems and refining your approach!
Leveraging Online Resources for Practice
Top Platforms for Coding Practice
To prepare effectively for Go language interviews, utilizing online platforms can be incredibly beneficial. Here are some of the best resources:
- LeetCode: A popular site for coding challenges that helps you practice various algorithms and data structures.
- HackerRank: Offers a wide range of coding problems and competitions to sharpen your skills.
- Codewars: Focuses on improving your coding skills through fun challenges and community engagement.
Recommended Courses and Tutorials
Learning through structured courses can enhance your understanding of Go. Consider these options:
- Udemy: Features numerous courses on Go programming, covering everything from basics to advanced topics.
- Coursera: Offers courses from universities that delve into Go and its applications.
- Pluralsight: Provides in-depth tutorials on Go, including best practices and real-world applications.
Utilizing Mock Interviews
Mock interviews are a great way to simulate the real interview experience. Here’s how to make the most of them:
- Practice with Peers: Pair up with friends or colleagues to conduct mock interviews.
- Use Platforms: Websites like Pramp allow you to practice live coding interviews for free.
- Record Yourself: Reviewing your performance can help identify areas for improvement.
Practice consistently to build confidence and improve your skills.
By leveraging these online resources, you can enhance your preparation and increase your chances of success in Go language interviews. Remember, the more you practice, the more comfortable you will become with the material!
Communication Skills for Technical Interviews
Explaining Your Thought Process
In technical interviews, clearly explaining your thought process is essential. Practice articulating your ideas as you work through problems. This helps interviewers see how you think and solve issues. Here are some tips:
- Break down complex problems into smaller parts.
- Explain each step clearly.
- Use simple language to describe your approach.
Active Listening Techniques
Active listening is a key part of good communication. It shows you are engaged and interested. Here are ways to improve your active listening:
- Pay close attention to the interviewer’s questions.
- Nod and give brief verbal acknowledgments.
- If something is unclear, ask for clarification.
Effective Team Communication
In many interviews, you may discuss your teamwork experiences. Highlight how you communicated effectively in past projects. Consider these points:
- Share examples of how you contributed to team success.
- Discuss how open communication helped solve problems.
- Mention any tools or methods you used to keep everyone informed.
Remember, staying calm and communicating well can help you navigate through the interview with ease.
Stress Management During Interviews
Mindfulness and Relaxation Techniques
Managing stress during interviews is crucial. Practicing mindfulness can help you stay calm. Here are some techniques:
- Deep Breathing: Take slow, deep breaths to relax your mind and body.
- Visualization: Picture yourself succeeding in the interview.
- Positive Affirmations: Repeat encouraging phrases to boost your confidence.
Positive Body Language
Your body language can say a lot about your confidence. Here are some tips to maintain a positive presence:
- Stand Tall: Good posture can make you feel more confident.
- Make Eye Contact: This shows you are engaged and interested.
- Smile: A genuine smile can create a friendly atmosphere.
Preparing for Difficult Questions
Difficult questions can be stressful, but preparation can ease your anxiety. Here’s how:
- Anticipate Questions: Think about common tough questions and prepare your answers.
- Practice Responses: Rehearse your answers with a friend or in front of a mirror.
- Stay Calm: If you get a tough question, take a moment to think before answering.
Remember, planning is an important tool in handling stress for me. Drawing up detailed plans for projects and even my daily work helps me to get ahead of stressful situations.
By using these techniques, you can manage stress effectively and perform your best during interviews.
Advanced Go Language Topics
Memory Management in Go
In Go, memory management is handled automatically through a process called garbage collection. This means that the Go runtime takes care of allocating and freeing memory, which helps prevent memory leaks. Here are some key points to remember:
- Go uses a garbage collector to manage memory.
- You can tune the garbage collector’s frequency using the
GOGC
environment variable. - Understanding how to minimize memory allocation can improve performance.
Using Go for Web Development
Go is a great choice for web development due to its speed and efficiency. Here are some advantages:
- Built-in support for HTTP servers and clients.
- A rich standard library that simplifies tasks like routing and middleware.
- Strong concurrency support, allowing for handling multiple requests simultaneously.
Advanced Concurrency Patterns
Concurrency in Go is powerful, but it can be tricky. Here are some advanced patterns to consider:
- Worker Pools: Limit the number of goroutines to manage resources better.
- Fan-Out, Fan-In: Distribute tasks across multiple goroutines and then consolidate results.
- Context Package: Use it to manage cancellation and timeouts in concurrent operations.
Understanding these advanced topics can set you apart in a Go interview. Mastering concurrency is essential for writing efficient Go applications.
In summary, mastering these advanced Go topics will not only help you in interviews but also in real-world applications. Be prepared to tackle tricky Golang interview questions that focus on these areas, especially regarding concurrency and memory management.
Post-Interview Strategies
Following Up with Interviewers
After your interview, it’s important to follow up with your interviewers. A simple thank-you email can go a long way. Here are some tips:
- Send your email within 24 hours.
- Mention specific topics discussed during the interview.
- Express your enthusiasm for the position.
Reflecting on Your Performance
Take some time to think about how the interview went. Reflecting can help you improve for next time. Consider:
- What questions were challenging?
- How did you communicate your thoughts?
- What could you have done differently?
Continuing Your Learning Journey
Regardless of the outcome, keep learning. This is key to growth in your career. Here are some ways to continue:
- Enroll in online courses to strengthen your skills.
- Work on personal projects to apply what you’ve learned.
- Join coding communities to stay engaged.
Remember, every interview is a chance to learn and grow. Staying positive will help you in your next opportunity!
Building a Strong Portfolio
Creating a strong portfolio is essential for showcasing your skills and projects. A well-organized portfolio can set you apart from other candidates. Here are some key elements to consider:
Showcasing Your Projects
- Include a variety of projects that demonstrate your skills.
- Highlight projects that are relevant to the job you’re applying for.
- Use clear descriptions to explain your role and the technologies used.
Writing a Technical Blog
- Share your knowledge and experiences through blog posts.
- Write about challenges you faced and how you solved them.
- This not only shows your expertise but also your ability to communicate effectively.
Contributing to Open Source
- Get involved in open-source projects to gain real-world experience.
- This helps you learn from others and improve your coding skills.
- It also shows potential employers your commitment to the tech community.
Remember, a portfolio is not just a collection of work; it’s a reflection of your journey and growth as a developer.
By focusing on these areas, you can create a portfolio that effectively showcases your abilities and makes a strong impression on interviewers.
Portfolio Element | Importance |
---|---|
Variety of Projects | Demonstrates diverse skills |
Technical Blog | Shows communication skills |
Open Source Contributions | Reflects commitment and teamwork |
Understanding the Importance of System Design
System design is a crucial part of technical interviews, especially for roles in software development. Understanding system design helps you create scalable and efficient applications. Here are some key points to consider:
Basic Principles of System Design
- Scalability: Ensure your system can handle increased loads.
- Reliability: Design systems that are dependable and can recover from failures.
- Maintainability: Create systems that are easy to update and manage.
Common System Design Questions
- How would you design a URL shortening service?
- What architecture would you use for a chat application?
- How would you build a recommendation system?
Resources for Learning System Design
- Books on system architecture
- Online courses focusing on system design
- Practice with mock interviews to refine your skills
System design interviews are a critical component of the hiring process for many technology companies, especially those that develop large-scale and highly complex systems. Understanding these concepts can significantly improve your chances of success in interviews.
Behavioral Interview Preparation
Common Behavioral Questions
When preparing for behavioral interviews, it’s important to anticipate the types of questions you might face. Here are some common ones:
- Tell me about yourself.
- What is your biggest strength and area of growth?
- Why are you interested in this position?
Practice answering these questions clearly and confidently.
Structuring Your Responses
Using the STAR method can help you structure your answers effectively:
- Situation: Describe the context within which you performed a task or faced a challenge.
- Task: Explain the actual task or challenge that was involved.
- Action: Detail the specific actions you took to address the task or challenge.
- Result: Share the outcomes of your actions, including what you learned.
Demonstrating Soft Skills
Soft skills are just as important as technical skills. Here are some key soft skills to highlight:
- Communication: Clearly explain your thought process.
- Teamwork: Show how you collaborate with others.
- Adaptability: Discuss how you handle change and challenges.
Remember, your interviewers are human! Finding a point of connection can make a big difference in how you are perceived.
Key Takeaways
- Practice makes perfect. Regularly rehearse your answers to common questions.
- Be genuine. Authenticity can help you stand out.
- Stay calm. Managing stress will help you communicate better during the interview.
By preparing thoroughly and practicing your responses, you can approach behavioral interviews with confidence and clarity.
Preparing for a behavioral interview can be a game-changer in landing your dream job. It’s essential to practice common questions and reflect on your experiences. Don’t wait any longer—visit our website to start your journey toward mastering coding interviews today!
Final Thoughts on Go Language Interview Preparation
In conclusion, preparing for a Go language interview can be challenging, but with the right approach, you can succeed. Focus on understanding the key concepts and practicing coding problems regularly. Use resources like LeetCode to get familiar with common questions. Remember to communicate your thought process clearly during the interview. Mock interviews can help you feel more comfortable and ready. Lastly, stay positive and keep practicing, as this will boost your confidence and skills. With dedication and effort, you can ace your Go language interview!
Frequently Asked Questions
What is the Go language interview process like?
The Go language interview process usually includes coding challenges, problem-solving questions, and discussions about your previous work. You may face online tests, phone interviews, and in-person interviews.
How can I improve my coding skills for Go interviews?
To boost your coding skills, practice regularly on platforms like LeetCode or HackerRank. Focus on understanding algorithms and data structures in Go.
What should I do to prepare for a technical interview?
Prepare by practicing coding problems, studying common algorithms, and reviewing your past projects. Mock interviews can also help you get comfortable.
How can I communicate better during interviews?
Practice explaining your thought process out loud while coding. Listen carefully to the interviewer’s questions and respond clearly.
What are some common mistakes to avoid in interviews?
Avoid rushing into coding without planning. Don’t forget to practice your communication skills, and be aware of your body language.
How can I manage stress during an interview?
Try deep breathing exercises and maintain a positive attitude. Prepare for tough questions so you feel more confident.
What resources can I use to practice Go coding?
You can use online platforms like AlgoCademy, LeetCode, and HackerRank for coding practice. There are also many courses available for structured learning.
What should I do after the interview?
After the interview, follow up with a thank-you note to the interviewer. Reflect on your performance and identify areas for improvement.