Understanding Request-Response Cycle Forms: A Comprehensive Guide
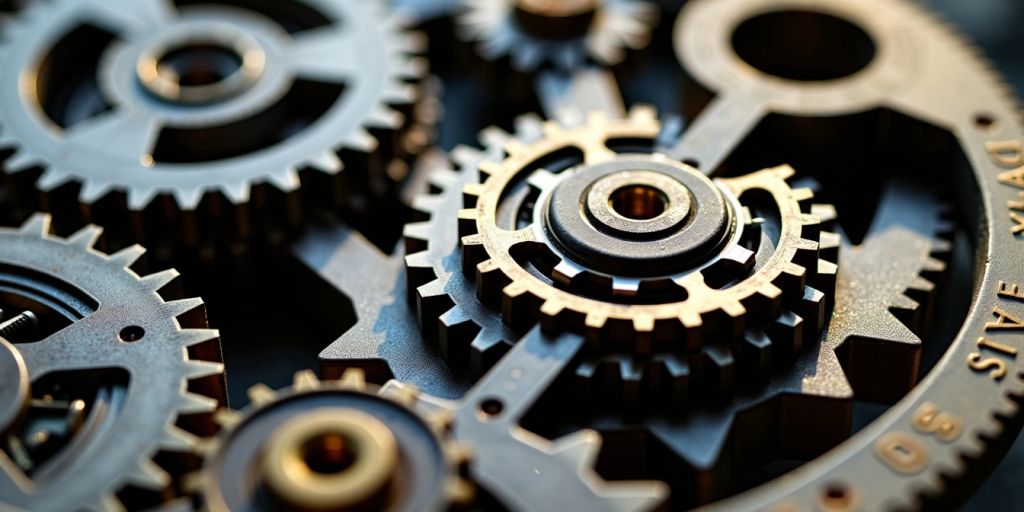
The request-response cycle is a fundamental concept in web development. It describes how a client, like a web browser, interacts with a server to request information and receive a response. Understanding this cycle is crucial for building effective web applications, as it helps developers manage data flow and enhance user experience. This guide will break down the various components of the request-response cycle, including how it works in different frameworks like Flask, Node.js, Express.js, and Nest.js.
Key Takeaways
- The request-response cycle is how clients and servers communicate over the web.
- Different types of requests (like GET and POST) serve different purposes.
- Frameworks like Flask and Express.js simplify handling requests and responses.
- Middleware plays a key role in processing requests and responses effectively.
- Understanding error handling is important for improving user experience.
Introduction to Request-Response Cycle Forms
Definition and Importance
The request-response cycle is a fundamental concept in web development. It describes how a client, like a web browser, communicates with a server. When a client wants something, it sends a request, and the server responds with the requested information. This cycle is crucial because it allows users to interact with web applications seamlessly.
Basic Components
The request-response cycle consists of several key components:
- Client: The device or application making the request.
- Request: The message sent by the client, which includes the method (like GET or POST) and the URL.
- Server: The system that processes the request and sends back a response.
- Response: The data sent back from the server to the client, which includes the status code and the requested information.
Component | Description |
---|---|
Client | The user’s device or application |
Request | The message sent to the server |
Server | The system that processes the request |
Response | The data sent back to the client |
Real-World Examples
In everyday life, we encounter the request-response cycle frequently. Here are a few examples:
- Web Browsing: When you type a URL in your browser, it sends a request to the server hosting that website.
- Online Shopping: When you add an item to your cart, your browser sends a request to update your shopping cart on the server.
- Social Media: When you post a photo, your app sends a request to the server to store that photo and update your profile.
Understanding the request-response cycle is essential for anyone looking to build effective web applications. It helps in creating a smooth user experience and ensures that data flows correctly between clients and servers.
Understanding Client Requests
Types of Client Requests
Client requests are how users interact with servers. Here are the main types:
- GET: Used to retrieve data from the server.
- POST: Sends data to the server, often used for submitting forms.
- PUT: Updates existing data on the server.
- DELETE: Removes data from the server.
Request Headers and Body
When a client sends a request, it includes important information:
- Headers: Provide metadata about the request, like content type and authorization.
- Body: Contains the actual data being sent, especially in POST requests.
Common Request Methods
Understanding the different methods is crucial for effective communication:
- GET: Fetches data without changing anything.
- POST: Sends new data to the server.
- PUT: Updates existing data.
- DELETE: Removes data from the server.
The request-response cycle is essential for web communication. Before a client and server can exchange an HTTP request/response pair, they must establish a TCP connection, a process which requires several round-trips.
This cycle is what powers HTTP and makes the web tick!
Server-Side Processing
Routing Requests
Routing is a key part of how servers handle incoming requests. It directs each request to the right function based on the URL. Here’s how it works:
- Receive the Request: The server gets the request from the client.
- Match the URL: The server checks the URL against its list of routes.
- Execute the Function: Once a match is found, the server runs the corresponding function to process the request.
Middleware Functions
Middleware functions are like helpers that sit between the request and response. They can do various tasks, such as:
- Logging: Keep track of requests for debugging.
- Authentication: Check if a user is allowed to access certain data.
- Data Parsing: Convert incoming data into a usable format.
Error Handling
Errors can happen at any time during processing. Here’s how to manage them:
- Catch Errors: Use try-catch blocks to catch errors in your code.
- Send Error Responses: If an error occurs, send a clear message back to the client.
- Log Errors: Keep a record of errors for future reference.
Understanding how to handle errors is crucial for creating reliable applications. Good error handling can improve user experience and make debugging easier.
In summary, server-side processing involves routing requests, using middleware for various tasks, and handling errors effectively. This ensures that the server can respond correctly to client requests, making the web work smoothly. The core concepts of this process are essential for any web application.
Response Creation and Handling
Generating Responses
Creating responses is a key part of the request-response cycle. When a server receives a request, it processes the information and generates a response. This response can be in various formats, such as HTML, JSON, or plain text. The type of response depends on the request and the server’s logic. Here are some common types of responses:
- HTML: Used for web pages.
- JSON: Commonly used for APIs.
- Plain Text: Simple text responses.
Response Headers and Body
The response consists of two main parts: headers and body. The headers provide metadata about the response, while the body contains the actual content. Here’s a simple breakdown:
Component | Description |
---|---|
Headers | Information about the response (e.g., content type, length) |
Body | The main content being sent back to the client |
Status Codes
Status codes are crucial for indicating the result of a request. They help clients understand what happened. Here are some common status codes:
- 200 OK: The request was successful.
- 404 Not Found: The requested resource was not found.
- 500 Internal Server Error: There was a problem on the server side.
Understanding how to create and handle responses is essential for building effective web applications. It ensures that users receive the correct information and enhances their experience.
In summary, the response creation and handling process is vital in the request-response cycle. By generating appropriate responses, setting the right headers, and using status codes effectively, developers can create robust applications that communicate well with users.
Request-Response Cycle in Flask
Overview of Flask
Flask is a lightweight web framework for Python. It helps developers create web applications quickly and easily. Flask is popular because it is simple to use and flexible enough for complex projects.
Handling Requests in Flask
When a user wants something from a Flask app, they send a request. This request can be for a webpage, data, or to submit a form. Here’s how Flask handles it:
- Client Request: The user’s browser sends a request to the server.
- Server Processing: Flask receives the request and figures out what to do with it. It uses routing to send the request to the right function.
- Response Creation: After processing, Flask creates a response to send back to the user.
- Client Receives Response: The server sends the response back, and the browser shows it to the user.
Creating Responses in Flask
Flask can create different types of responses:
- HTML pages
- JSON data
- Redirects
The response is wrapped in a special object that helps manage things like headers and status codes. This makes it easier to control what the user sees.
Understanding how Flask processes requests and creates responses is key to building effective web applications.
Key Points
- Flask uses routing to direct requests to the correct function.
- The request object holds all the information sent by the client, like form data and headers.
- The response object is what Flask sends back to the client, containing the data the user requested.
Conclusion
In summary, the request-response cycle in Flask is essential for web development. By understanding how requests are handled and responses are created, developers can build better applications. Flask’s simplicity and flexibility make it a great choice for many projects.
Highlight
In unit testing in Python with Flask and pytest, we’ll use the client fixture provided by pytest to make requests to the application and then assert the responses, checking for correct HTTP status codes.
Request-Response Cycle in Node.js
Node.js Basics
The request-response cycle is essential for web communication. It’s how your browser or app asks for information and how the server replies. In Node.js, this cycle is handled using a non-blocking, event-driven approach, making it fast and efficient even when many requests come in at once.
Handling Requests in Node.js
Here’s a simple example of how to create a server in Node.js:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, World!\n');
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
In this code:
- The server listens for requests on port 3000.
- It sends back "Hello, World!" when a request is made.
Creating Responses in Node.js
When a request is received, the server processes it and sends back a response. Here are some common request types:
- GET: Fetching data.
- POST: Sending data to the server.
- PUT: Updating existing data.
- DELETE: Removing data.
For example:
const server = http.createServer((req, res) => {
if (req.method === 'GET') {
res.end('You made a GET request');
} else if (req.method === 'POST') {
res.end('You made a POST request');
}
});
Summary
In summary, the request-response cycle is the backbone of how the web works. Understanding this model is crucial for building web applications. Experimenting with Node.js can help you see how this cycle operates in practice.
The request-response model is the backbone of how the web works. It’s the process that happens when you visit a website or call an API, with your browser asking for something and the server replying.
Request-Response Cycle in Express.js
Introduction to Express.js
Express.js is a popular web framework for Node.js that simplifies the process of building web applications. It allows developers to create robust APIs and handle requests and responses efficiently. Express makes it easier to manage the request-response cycle.
Middleware in Express.js
Middleware functions are essential in Express. They act as a bridge between the request and response. Here are some key points about middleware:
- Middleware can modify the request or response objects.
- It can perform tasks like logging, authentication, and error handling.
- If the current middleware function does not end the request-response cycle, it must call
next()
to pass control to the next middleware function.
Routing and Responses in Express.js
Routing in Express is straightforward. You define routes to handle different HTTP methods. Here’s a simple example:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
In this example, when a GET request is made to the root URL, the server responds with "Hello, World!". This shows how Express handles routing and responses seamlessly.
Conclusion
The request-response cycle in Express.js is a vital concept for web development. Understanding how middleware works and how to define routes will help you build efficient applications.
Mastering the request-response cycle is key to becoming a proficient Express developer!
Request-Response Cycle in Nest.js
Overview of Nest.js
Nest.js is a powerful framework for building server-side applications. It uses TypeScript and is built on top of Node.js. Understanding how it processes requests is essential for creating efficient applications.
Handling Requests in Nest.js
In Nest.js, the request-response cycle involves several key components:
- Middleware: These are functions that run before the request reaches the route handler. They can modify the request and response objects or end the request-response cycle. Middleware is crucial for optimizing middleware and interceptors in Nest.js.
- Guards: These are used to protect routes by checking if a request should be processed based on certain conditions, like user authentication.
- Interceptors: They allow you to add extra logic before and after the request handling, giving you control over the response.
- Pipes: These are used for data transformation and validation, ensuring that incoming data is in the correct format.
- Controllers: They handle the business logic after the request has passed through middleware, guards, and interceptors.
- Exception Filters: These catch errors that occur during the request processing and format the error responses appropriately.
- Response: Finally, the server sends a response back to the client after processing the request.
Creating Responses in Nest.js
Responses in Nest.js can be customized based on the needs of your application. You can send different types of responses, such as JSON or HTML, depending on the request type. Here’s a simple example:
@Get()
getHello(): string {
return 'Hello World!';
}
This code snippet shows how to create a simple GET endpoint that returns a string response.
The request-response cycle in Nest.js is designed to be efficient and secure, allowing developers to build robust applications.
Understanding these components helps in creating applications that are not only functional but also maintainable and scalable.
Advanced Concepts in Request-Response Cycle
Asynchronous Processing
Asynchronous processing allows a server to handle multiple requests at the same time without waiting for each one to finish. This is important for improving the overall performance of web applications. Here are some key points:
- Non-blocking I/O: This allows the server to continue processing other requests while waiting for data.
- Callbacks and Promises: These are used to handle operations that take time, like database queries.
- Event Loop: This is a core part of JavaScript that helps manage asynchronous operations.
Security Considerations
When dealing with requests and responses, security is crucial. Here are some common practices:
- Input Validation: Always check and sanitize user inputs to prevent attacks.
- Authentication: Ensure that users are who they say they are before processing their requests.
- HTTPS: Use secure connections to protect data in transit.
Performance Optimization
Optimizing the request-response cycle can lead to faster applications. Consider these strategies:
- Caching: Store frequently accessed data to reduce load times.
- Load Balancing: Distribute incoming requests across multiple servers.
- Minimizing Payloads: Reduce the size of data sent in requests and responses.
Understanding these advanced concepts can greatly enhance the efficiency and security of your web applications. A request-reply exchange consists of a request message, and an eventual reply message. In the simple request-reply pattern, there’s one reply for each request.
By mastering these advanced topics, developers can create more robust and efficient web applications that handle user requests effectively.
Common Challenges and Solutions
Debugging Issues
Debugging can be tricky in the request-response cycle. Here are some common problems:
- Network Errors: These can occur if the server is down or unreachable.
- Incorrect Endpoints: Make sure the URL is correct.
- Data Format Issues: Ensure the data sent matches what the server expects.
Tip: Use tools like Postman to test your requests and responses.
Handling Large Data
When dealing with large amounts of data, performance can suffer. Here are some strategies:
- Pagination: Break data into smaller chunks.
- Compression: Use gzip or similar methods to reduce data size.
- Streaming: Send data in smaller pieces instead of all at once.
Ensuring Scalability
As your application grows, it’s important to keep it scalable. Consider these points:
- Load Balancing: Distribute traffic across multiple servers.
- Caching: Store frequently accessed data to speed up responses.
- Microservices: Break your application into smaller, manageable services.
Remember: Identifying website performance bottlenecks is crucial for maintaining a smooth user experience. Simplifying the performance troubleshooting process can help unlock the potential of your application.
Best Practices for Request-Response Cycle Forms
Efficient Routing
- Organize your routes logically to improve readability and maintenance.
- Use route parameters to handle dynamic segments in URLs.
- Implement nested routes for better structure in complex applications.
Effective Error Handling
- Always return meaningful error messages to help users understand issues.
- Use try-catch blocks to manage exceptions gracefully.
- Log errors for future debugging and analysis.
Optimizing Performance
- Minimize the size of response payloads to speed up data transfer.
- Use caching strategies to reduce server load and improve response times.
- Implement rate limiting to prevent abuse and ensure fair usage.
Following these best practices can significantly enhance the performance and security of your web applications. Always prioritize security by validating inputs and defining allowable methods for each API endpoint. This helps prevent misuse and ensures a smoother user experience.
Best Practice | Description |
---|---|
Efficient Routing | Organize routes logically for better maintenance. |
Effective Error Handling | Return meaningful messages and log errors for debugging. |
Optimizing Performance | Minimize payload size and implement caching strategies. |
When it comes to creating forms for the request-response cycle, following best practices is key. Make sure your forms are clear and easy to fill out. This will help users provide the right information quickly. If you want to learn more about coding and improve your skills, visit our website and start coding for free today!
Conclusion
In summary, grasping the request-response cycle is key to building strong web applications. This cycle shows how a client, like a web browser, asks for information and how the server responds. By managing requests and responses well, developers can create applications that are not only effective but also user-friendly. Flask, with its easy-to-use features, is a great choice for anyone looking to dive into web development. Whether you’re making a simple site or a more complex application, understanding this cycle will help you succeed.
Frequently Asked Questions
What is the request-response cycle?
The request-response cycle is a process that happens when a client, like a web browser, asks a server for information and the server sends back a response.
Why is the request-response cycle important?
It’s important because it helps web applications communicate with users. Understanding this cycle allows developers to build better websites.
What are some common types of client requests?
Common types include GET, which asks for data, and POST, which sends data to the server.
What role do headers play in requests?
Headers give extra information about the request, like what type of data is being sent or how the client wants to receive a response.
How does a server process a request?
The server receives the request, figures out what the client wants, runs any necessary code, and then prepares a response.
What is a response object?
A response object is what the server sends back to the client. It includes the data requested and other important details.
What is middleware in web development?
Middleware is code that runs between the request and response. It can modify requests, handle errors, or perform tasks like logging.
How can I optimize the request-response cycle?
You can optimize it by using efficient routing, handling errors effectively, and minimizing the amount of data sent back and forth.