Unlocking the Secrets of Data Structures and Algorithms PDF: A Comprehensive Guide for Beginners
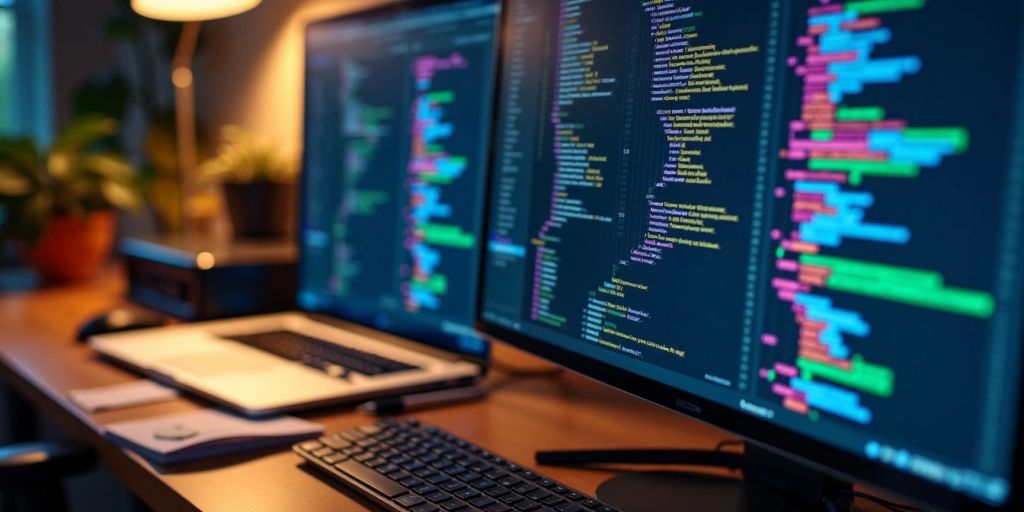
Data structures and algorithms are the backbone of computer science. They help us organize and manage data efficiently while solving problems effectively. This guide is designed for beginners to understand these concepts better and apply them in real-world scenarios. By mastering these fundamentals, you can enhance your coding skills and prepare for technical interviews with confidence.
Key Takeaways
- Data structures help organize information in a way that computers can use efficiently.
- Algorithms are step-by-step procedures for solving problems or completing tasks.
- Understanding these concepts is crucial for coding interviews and real-world applications.
- Practice is key to mastering data structures and algorithms, so use coding platforms and tutorials.
- Stay curious and seek help when you encounter challenges in your learning journey.
Understanding the Basics of Data Structures and Algorithms
What Are Data Structures?
Data structures are ways to organize and store data so that we can use it efficiently. They help us manage large amounts of information in a way that makes it easy to access and modify. Here are some common types of data structures:
- Arrays: A collection of items stored at contiguous memory locations.
- Linked Lists: A series of connected nodes, where each node contains data and a reference to the next node.
- Stacks: A collection of elements that follows the Last In First Out (LIFO) principle.
Introduction to Algorithms
An algorithm is a step-by-step procedure for solving a problem. It’s like a recipe that tells you how to get from one point to another. Algorithms can be simple or complex, depending on the problem they are trying to solve. Here are some key points about algorithms:
- Efficiency: How fast an algorithm runs.
- Correctness: Whether the algorithm gives the right answer.
- Clarity: How easy it is to understand the algorithm.
Importance of Learning Data Structures and Algorithms
Learning data structures and algorithms (DSA) is crucial for anyone interested in computer science. They are fundamental in computer science that help us to organize and process data efficiently. Here are a few reasons why:
- Problem Solving: They provide tools to tackle complex problems.
- Job Opportunities: Many tech companies look for candidates with strong DSA skills.
- Foundation for Advanced Topics: Understanding DSA is essential for learning more advanced concepts in computer science.
Mastering data structures and algorithms can open doors to many opportunities in the tech world.
By grasping these basics, you set a strong foundation for further exploration in the field of computer science.
Types of Data Structures
Data structures are ways to organize and store data so that we can use it efficiently. They can be divided into different types based on their characteristics and how they manage data. Here are the main types:
Linear Data Structures
Linear data structures are organized in a sequential manner. Each element is connected to its previous and next element. Common examples include:
- Arrays: A collection of items stored at contiguous memory locations.
- Linked Lists: A series of connected nodes, where each node contains data and a reference to the next node.
- Stacks: A collection of elements that follows the Last In First Out (LIFO) principle.
- Queues: A collection that follows the First In First Out (FIFO) principle.
Non-linear Data Structures
Non-linear data structures do not store data in a sequential manner. Instead, they allow for more complex relationships between elements. Examples include:
- Trees: A hierarchical structure with a root value and subtrees of children.
- Graphs: A collection of nodes connected by edges, which can represent various relationships.
- Heaps: A special tree-based structure that satisfies the heap property.
Abstract Data Types
Abstract data types (ADTs) are theoretical concepts that define data types by their behavior from the point of view of a user. They include:
- Lists: A collection of ordered elements.
- Sets: A collection of unique elements.
- Maps: A collection of key-value pairs.
Understanding the different types of data structures is crucial for selecting the right one for your programming needs. Each type has its own strengths and weaknesses, making them suitable for various applications.
Common Algorithms and Their Applications
Sorting Algorithms
Sorting algorithms are essential for organizing data. They help in arranging items in a specific order, such as ascending or descending. Here are some common sorting algorithms:
- Bubble Sort: A simple method that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
- Quick Sort: A more efficient algorithm that divides the list into smaller parts and sorts them independently.
- Merge Sort: This algorithm divides the list into halves, sorts each half, and then merges them back together.
Algorithm | Time Complexity | Space Complexity |
---|---|---|
Bubble Sort | O(n²) | O(1) |
Quick Sort | O(n log n) | O(log n) |
Merge Sort | O(n log n) | O(n) |
Searching Algorithms
Searching algorithms are used to find specific data within a structure. Here are a few popular ones:
- Linear Search: This method checks each element one by one until it finds the target.
- Binary Search: This efficient method works on sorted lists by repeatedly dividing the search interval in half.
- Depth-First Search (DFS): A technique used mainly in graph algorithms that explores as far as possible along each branch before backtracking.
Graph Algorithms
Graph algorithms are crucial for solving problems related to networks. They help in finding the shortest path, detecting cycles, and more. Some key graph algorithms include:
- Dijkstra’s Algorithm: Used to find the shortest path from a starting node to all other nodes in a weighted graph.
- A Search Algorithm*: An extension of Dijkstra’s that uses heuristics to improve efficiency.
- Kruskal’s Algorithm: A method for finding the minimum spanning tree of a graph.
Understanding these algorithms is vital for tackling complex problems in programming. They form the backbone of many applications, from simple tasks to advanced systems.
In summary, mastering these algorithms can greatly enhance your problem-solving skills and prepare you for challenges in coding interviews and real-world applications. Graph algorithms and dynamic programming are particularly important for competitive programming and complex problem-solving.
How to Approach Problem-Solving with Algorithms
Understanding the Problem
To solve a problem effectively, you first need to understand what the problem is asking. This means breaking it down into smaller parts. Here are some steps to help you:
- Read the problem carefully.
- Identify the input and output.
- Think about examples to clarify your understanding.
Choosing the Right Algorithm
Once you understand the problem, the next step is to choose the right algorithm. Different problems require different approaches. Here are some common strategies:
- Brute Force: Trying all possible solutions.
- Divide and Conquer: This method involves breaking down a complex problem into smaller, more manageable parts.
- Dynamic Programming: This is useful for problems that can be broken down into overlapping subproblems.
Optimizing Your Solution
After you have a solution, it’s important to make it better. Here are some tips for optimization:
- Look for ways to reduce time complexity.
- Minimize space usage if possible.
- Test your solution with different inputs to ensure it works efficiently.
Remember, problem-solving is a skill that improves with practice. The more problems you tackle, the better you will become!
Learning Resources for Data Structures and Algorithms
Books and PDFs
When starting your journey in data structures and algorithms, books and PDFs are great resources. Here are some popular options:
- Introduction to Algorithms by Cormen, Leiserson, Rivest, and Stein
- Data Structures and Algorithms Made Easy by Narasimha Karumanchi
- Grokking Algorithms by Aditya Bhargava
Online Courses and Tutorials
Online courses can provide a structured way to learn. Consider these platforms:
- Coursera
- edX
- Udacity
Interactive Coding Platforms
Practicing coding is essential. Here are some platforms where you can practice:
- LeetCode
- HackerRank
- CodeSignal
Learning data structures and algorithms is a step-by-step process. Start with the basics and gradually move to more complex topics.
Resource Type | Examples |
---|---|
Books and PDFs | Introduction to Algorithms, Data Structures and Algorithms Made Easy |
Online Courses | Coursera, edX, Udacity |
Interactive Platforms | LeetCode, HackerRank, CodeSignal |
Practical Applications of Data Structures and Algorithms
Real-World Examples
Data structures and algorithms are not just theoretical concepts; they have real-life applications that impact our daily lives. Here are some examples:
- Spam email detection: Algorithms help identify unwanted emails.
- Plagiarism detection: They check if content is copied from other sources.
- Search engines: Data structures organize information for quick retrieval.
Industry Use Cases
Different industries utilize data structures and algorithms to solve problems efficiently. Some common use cases include:
- Finance: Algorithms analyze market trends and make predictions.
- Healthcare: Data structures manage patient records and treatment plans.
- E-commerce: Algorithms recommend products based on user behavior.
Coding Interview Preparation
Understanding data structures and algorithms is crucial for coding interviews. Here are some tips to prepare:
- Practice coding problems regularly.
- Study common algorithms and their applications.
- Mock interviews can help simulate real interview conditions.
Mastering data structures and algorithms can open doors to many opportunities in tech. They are the backbone of software development and problem-solving in various fields.
Challenges and Pitfalls in Learning Data Structures and Algorithms
Common Mistakes to Avoid
Learning data structures and algorithms can be tricky. Here are some common mistakes to watch out for:
- Ignoring the Basics: Skipping foundational concepts can lead to confusion later.
- Overcomplicating Solutions: Sometimes, the simplest solution is the best one.
- Not Practicing Enough: Regular practice is essential to mastering these topics.
Overcoming Learning Obstacles
If you find yourself struggling, consider these strategies:
- Break Down Problems: Tackle complex problems in smaller parts.
- Use Visual Aids: Diagrams and charts can help clarify concepts.
- Join Study Groups: Collaborating with peers can enhance understanding.
Staying Motivated
Staying engaged while learning can be challenging. Here are some tips to keep your motivation high:
- Set small, achievable goals.
- Reward yourself for completing tasks.
- Remind yourself of the benefits of mastering algorithms and data structures.
Mastering algorithms and data structures is key to solving complex problems. Issues such as poor design, inappropriate data structure selection, and implementation problems can hinder learning.
By being aware of these challenges and actively working to overcome them, you can make your learning journey smoother and more enjoyable.
Advanced Topics in Data Structures and Algorithms
Dynamic Programming
Dynamic programming is a method used to solve complex problems by breaking them down into simpler subproblems. It is particularly useful for optimization problems. Key concepts include:
- Overlapping Subproblems: Problems that can be broken down into smaller, similar problems.
- Optimal Substructure: The optimal solution to a problem can be constructed from optimal solutions of its subproblems.
Greedy Algorithms
Greedy algorithms make the best choice at each step, hoping to find the global optimum. They are often easier to implement and can be very efficient. Here are some common characteristics:
- Locally Optimal Choice: At each step, the best option is chosen.
- No Backtracking: Once a choice is made, it is not reconsidered.
- Fast Execution: Greedy algorithms usually run in linear time.
Complexity Analysis
Understanding how algorithms perform is crucial. Complexity analysis helps us evaluate the efficiency of algorithms. Here’s a simple breakdown:
- Time Complexity: How the runtime of an algorithm increases with the size of the input.
- Space Complexity: How the memory usage of an algorithm changes with the input size.
In the world of programming, mastering these advanced topics can significantly enhance your problem-solving skills.
Summary Table of Key Concepts
Topic | Key Features |
---|---|
Dynamic Programming | Overlapping subproblems, Optimal substructure |
Greedy Algorithms | Locally optimal choice, No backtracking |
Complexity Analysis | Time and space complexity |
Tools and Software for Practicing Algorithms
Coding Practice Platforms
There are many platforms where you can practice coding and algorithms. Here are some popular ones:
- LeetCode: Great for preparing for coding interviews.
- HackerRank: Offers challenges in various domains.
- CodeSignal: Focuses on skill assessments and competitions.
Algorithm Visualization Tools
Understanding algorithms can be easier with visualization tools. These tools help you see how algorithms work step by step:
- VisuAlgo: Visualizes data structures and algorithms.
- Algorithm Visualizer: Interactive tool for various algorithms.
- Pythontutor: Helps visualize Python code execution.
Integrated Development Environments (IDEs)
Using an IDE can make coding more efficient. Here are some popular IDEs:
- Visual Studio Code: Lightweight and customizable.
- PyCharm: Great for Python developers.
- Eclipse: Good for Java programming.
Practicing algorithms regularly is key to mastering them. Consistency helps you understand and remember concepts better.
By using these tools, you can enhance your skills and become more confident in solving algorithmic problems. Get ahead in AI with these resources!
Tips for Mastering Data Structures and Algorithms
Consistent Practice
- Set a regular schedule for studying. This helps you stay on track and make steady progress.
- Try to solve at least one problem every day. This builds your skills over time.
- Use different platforms to practice, like LeetCode or HackerRank.
Understanding Core Concepts
- Focus on understanding patterns in problems. This will help you recognize similar problems in the future.
- Break down complex topics into smaller parts. This makes them easier to understand.
- Discuss concepts with friends or study groups. Teaching others can help reinforce your own understanding.
Seeking Help and Feedback
- Don’t hesitate to ask for help when you’re stuck. Online forums can be very useful.
- Review your solutions and seek feedback. This can help you find better ways to solve problems.
- Keep a journal of your learning. Write down what you learn and any mistakes you make.
Remember, mastering data structures and algorithms takes time and effort. Stay motivated and keep pushing yourself!
The Future of Data Structures and Algorithms
Emerging Trends
- Increased Use of AI: Data structures and algorithms are becoming crucial in developing AI applications.
- Real-time Data Processing: As data grows, the need for efficient algorithms to process it in real-time is rising.
- Cloud Computing: More algorithms are being designed to work efficiently in cloud environments.
Impact of AI and Machine Learning
- Automation: Algorithms are being used to automate tasks, making processes faster and more efficient.
- Data Analysis: Machine learning relies heavily on data structures to analyze large datasets.
- Personalization: Algorithms help in creating personalized experiences for users based on their data.
Future Learning Pathways
- Online Courses: Many platforms offer courses focused on the latest trends in data structures and algorithms.
- Workshops and Bootcamps: Short, intensive programs can help you learn quickly.
- Community Engagement: Joining forums and groups can provide support and resources.
The future of data structures and algorithms is bright, with endless possibilities for innovation and improvement.
As we look ahead, the world of data structures and algorithms is evolving rapidly. With new technologies and methods emerging, it’s crucial to stay updated. If you’re eager to enhance your coding skills and prepare for interviews, visit our website today! Start your journey towards mastering coding with us!
Final Thoughts
In conclusion, understanding data structures and algorithms is essential for anyone looking to excel in programming. This guide has provided you with a solid foundation to start your journey. Remember, practice is key! Use the resources available, like AlgoCademy, to sharpen your skills and prepare for coding interviews. With time and effort, you can unlock the secrets of coding and become a proficient programmer.
Frequently Asked Questions
What are data structures?
Data structures are ways to organize and store information on a computer. They help us manage data efficiently.
Why should I learn about algorithms?
Learning algorithms is important because they show you how to solve problems step by step. They help in making programs run faster and better.
What types of data structures are there?
There are many types of data structures, but the main ones are linear structures like arrays and lists, and non-linear structures like trees and graphs.
How do I choose the right algorithm for a problem?
To pick the right algorithm, first understand the problem clearly. Then, think about what the algorithm needs to do and how it can do it best.
What resources can I use to learn about data structures and algorithms?
You can find books, online courses, and interactive platforms that offer coding challenges to help you learn.
How can data structures and algorithms be used in real life?
They are used in many areas like software development, games, and even in apps that you use every day.
What are common mistakes when learning data structures?
Many beginners rush through the material or skip practicing. It’s important to take your time and practice regularly.
What’s the future of learning data structures and algorithms?
As technology grows, understanding data structures and algorithms will be even more important, especially with new fields like AI and machine learning.