Unlocking the Power of React Suspense: A Guide to Enhanced Performance in Your Applications
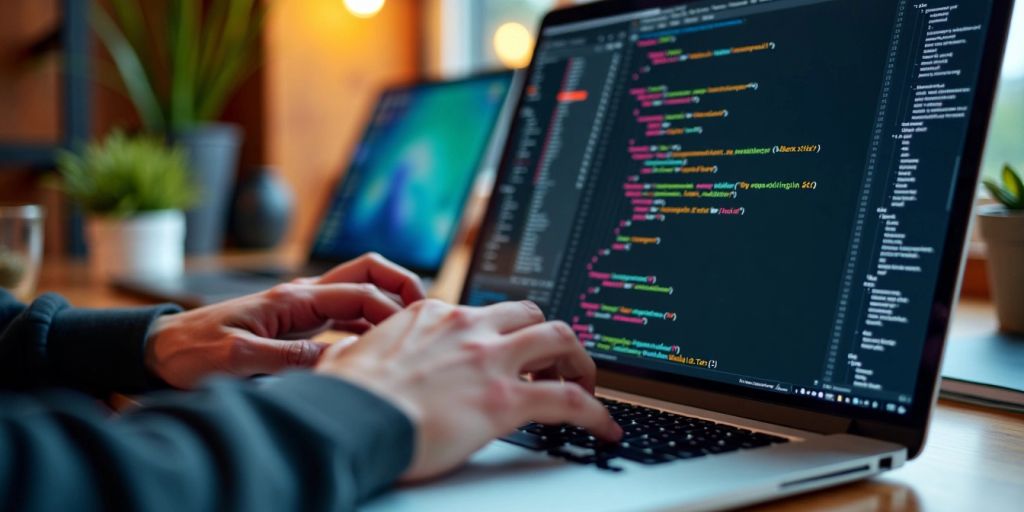
React Suspense is a game-changing feature that helps developers create smoother and faster applications. By allowing components to pause while waiting for data or resources, it enhances user experience and makes code easier to manage. This guide will explore how to effectively use React Suspense in your projects, highlighting its benefits and best practices.
Key Takeaways
- React Suspense allows components to pause rendering while waiting for data, improving user experience.
- It simplifies code by managing loading states automatically, making it easier to maintain.
- Using Suspense can lead to better performance by preventing unnecessary re-renders.
- Lazy loading components with Suspense helps reduce initial load times in large applications.
- React Suspense also supports server-side rendering, enhancing performance for dynamic web apps.
Understanding React Suspense
What is React Suspense?
React Suspense is a powerful feature in React that allows components to pause rendering while they wait for asynchronous tasks, like fetching data or loading resources. This makes it easier to manage loading states and improves the overall user experience.
Key Features of React Suspense
- Asynchronous Rendering: Components can wait for data before rendering.
- Fallback UI: You can show a loading indicator while waiting for data.
- Lazy Loading: Components can be loaded only when needed, reducing initial load time.
Benefits of Using React Suspense
Using React Suspense can lead to several advantages:
- Simplified Code: It reduces the complexity of managing loading states manually.
- Better User Experience: Users see a loading message instead of a blank screen, which enhances perceived performance.
- Performance Optimization: Components only render when the necessary data is ready, avoiding unnecessary re-renders.
By using React Suspense, developers can create applications with smoother user experiences. Suspense helps manage loading states and prevents the app from rendering incomplete UI.
In summary, React Suspense is a game-changer for handling asynchronous rendering in React applications, making it easier to build responsive and user-friendly interfaces. Unlocking the power of React Suspense can lead to cleaner code and a better experience for users.
Getting Started with React Suspense
Installing React Suspense
To begin using React Suspense, you need to install the necessary package. Here’s how you can do it:
- Using npm:
cd react-suspense npm install @suspensive/react
- Using yarn:
cd react-suspense yarn add @suspensive/react
After installation, you can import Suspense into your components like this:
import { Suspense } from 'react';
Basic Usage of React Suspense
Once you have Suspense imported, you can use it to manage loading states in your components. Here’s a simple example:
function MyComponent() {
return (
<Suspense fallback={<div>Loading...</div>}>
<OtherComponent />
</Suspense>
);
}
In this example, Suspense will show a loading message while OtherComponent
is being fetched.
Setting Up Your Development Environment
To set up your development environment for React Suspense, follow these steps:
- Clone the example project:
git clone https://github.com/nvkhuy/examples.git cd react-suspense
- Checkout the branch:
git checkout -b react-suspense-separate-error
- Install dependencies:
npm install
- Run the application:
npm run dev
- Open your browser at
http://localhost:3000
to see the application in action.
Setting up your environment correctly is crucial for a smooth development experience.
By following these steps, you can easily get started with React Suspense and begin enhancing your applications with better performance and user experience. Remember, learning Suspense by building a suspense-enabled library will help solidify your understanding!
Implementing React Suspense in Your Application
Using Suspense for Data Fetching
React Suspense allows you to simplify data fetching in your applications. By wrapping your components in a Suspense
component, you can pause rendering until the data is ready. Here’s how to do it:
- Wrap your component: Use the
Suspense
component to wrap the part of your app that needs data. - Provide a fallback UI: Specify a loading indicator to show while waiting for data.
- Use lazy loading: Load components only when needed to improve performance.
Lazy Loading Components with Suspense
Lazy loading helps in reducing the initial load time of your application. Here’s a simple example:
import React, { Suspense, lazy } from 'react';
const MyComponent = lazy(() => import('./MyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<MyComponent />
</Suspense>
);
}
In this example, MyComponent
is loaded only when it’s needed, which can lead to faster load times.
Error Handling with Suspense and Error Boundaries
React Suspense can handle errors gracefully using the ErrorBoundary
. You simply need to wrap your Suspense component in an error boundary to catch any errors that occur during data fetching. This ensures that your application remains stable even when something goes wrong.
Using error boundaries helps maintain a smooth user experience, even when errors occur.
Summary
Implementing React Suspense can greatly enhance your application’s performance and user experience. By using it for data fetching, lazy loading components, and handling errors, you can create a more responsive and efficient application. Remember to keep your components modular and maintainable for the best results!
Advanced Techniques with React Suspense
Code Splitting with Suspense
React Suspense allows for efficient data fetching patterns by enabling code splitting. This means you can load parts of your application only when needed, which can significantly improve performance. Here’s how you can implement it:
- Dynamic Imports: Use
React.lazy
to load components only when they are required. - Suspense Fallbacks: Provide a loading indicator while the component is being fetched.
- Granular Control: Split code within a single component for better optimization.
For example:
const Details = lazy(() => import('./Details'));
This allows the Details
component to load only when necessary, reducing the initial load time.
Optimizing Performance with Suspense
To get the most out of React Suspense, consider these strategies:
- Limit Suspense Boundaries: Use them only where necessary to avoid performance hits.
- Combine with Memoization: This helps prevent unnecessary re-renders.
- User Experience Focus: Always provide clear feedback during loading states to keep users informed.
Combining Suspense with Concurrent Rendering
React’s concurrent rendering works hand-in-hand with Suspense to enhance performance. This allows your app to prioritize rendering tasks, ensuring a smoother user experience. Here’s how:
- Prioritize UI Updates: React can pause rendering to focus on more important updates.
- Seamless Loading States: Users can interact with parts of the app while others are still loading.
- Improved Responsiveness: The app feels faster and more responsive, even during heavy data fetching.
By leveraging these advanced techniques, you can create applications that are not only faster but also provide a better user experience. React Suspense is a game-changer for managing asynchronous operations.
Suspense for Server-Side Rendering (SSR)
Introduction to Suspense SSR
Server-Side Rendering (SSR) is a method where the server creates the HTML content of a webpage and sends it to the browser. This technique improves loading times and user experience. With the introduction of Suspense in React 18, developers can enhance SSR by allowing parts of the page to load independently.
Benefits of Suspense SSR
Using Suspense with SSR offers several advantages:
- Faster Initial Load: The server can send HTML to the client without waiting for all JavaScript to load.
- Selective Hydration: Only the necessary parts of the page are hydrated, which speeds up interactivity.
- Improved User Experience: Users see content faster, reducing frustration from long loading times.
Implementing Suspense SSR in Your Application
To effectively use Suspense for SSR, follow these steps:
- Wrap Components: Use the Suspense component to wrap parts of your application that can load independently.
- Set Fallbacks: Provide fallback content, like loading spinners, while the main content is being fetched.
- Optimize Data Fetching: Ensure that data fetching is efficient to minimize delays in rendering.
By leveraging Suspense for SSR, developers can create applications that are not only faster but also more responsive to user interactions.
Drawbacks of Suspense SSR
While there are many benefits, there are also challenges:
- Increased Data Download: Users may need to download more data as applications grow.
- Hydration Issues: All components must be hydrated, which can slow down performance.
- Client-Side Processing: Heavy processing on the client can lead to performance issues, especially on less powerful devices.
In summary, using Suspense for SSR can significantly enhance the performance of React applications, but developers must be aware of the potential drawbacks to optimize their implementations effectively.
Common Pitfalls and How to Avoid Them
Excessive Suspense Boundaries
Using too many Suspense boundaries can slow down your application. Wrap components in Suspense only when they need to load data asynchronously. This helps keep your app running smoothly.
Improper Error Handling
Relying only on Suspense for catching errors is not enough. You should also use Error Boundaries to handle errors that happen during data fetching. This way, you can show users a friendly message instead of a broken page.
Unnecessary Re-renders
Avoid making your components re-render more than they need to. Here are some tips to help:
- Use memoization to keep components from re-rendering unnecessarily.
- Check your dependencies in hooks to ensure they are correct.
- Keep your state management simple to avoid extra renders.
Remember, a smooth user experience is key. Always provide clear feedback during loading states to keep users informed.
By being aware of these common pitfalls, you can enhance the performance of your React applications and create a better experience for your users.
Real-World Examples of React Suspense
Example 1: Data Fetching with Suspense
In this example, we will see how React Suspense simplifies the process of fetching data. Imagine you have a component that needs to display a list of countries. Without Suspense, you would have to manage loading states manually. Here’s how it looks:
- Before Suspense:
- With Suspense:
Example 2: Lazy Loading Components
Lazy loading is another powerful feature of Suspense. For instance, you can load a component only when it’s needed:
- Use
React.lazy
to define a component that will be loaded on demand. - Wrap it in a
Suspense
component to show a loading indicator while it’s being fetched.
This approach helps in reducing the initial load time of your application.
Example 3: Error Handling with Suspense
Error handling is crucial in any application. With Suspense, you can combine it with Error Boundaries:
- Use
ErrorBoundary
to catch errors during data fetching. - This allows you to display a fallback UI when something goes wrong, improving user experience.
By using React Suspense, developers can create applications with smoother user experiences. Suspense helps manage loading states and prevents the app from rendering incomplete UI.
Summary
React Suspense provides a cleaner way to handle asynchronous operations in your applications. By using it for data fetching, lazy loading, and error handling, you can enhance the performance and user experience of your React applications. Understanding the Suspense component is key to unlocking its full potential.
Best Practices for Using React Suspense
When to Use React Suspense
- Use Suspense when you have components that rely on asynchronous data fetching.
- Avoid wrapping components that do not require data loading.
- Use it for lazy loading components to enhance performance.
Optimizing User Experience
- Provide a clear fallback UI to inform users that data is loading.
- Use loading indicators that are visually appealing and informative.
- Ensure that the fallback UI is consistent across your application.
Maintaining Clean and Modular Code
- Keep your components focused on a single responsibility.
- Use React.lazy for lazy loading components to keep your code organized.
- Avoid excessive nesting of Suspense components to prevent performance issues.
By following these best practices, you can create applications that are not only efficient but also provide a better user experience. React best practices help you write cleaner and more maintainable code, making your applications easier to manage and scale.
Best Practice | Description |
---|---|
Use Suspense for Async Operations | Wrap components that fetch data asynchronously. |
Provide Clear Feedback | Use informative loading indicators in your fallback UI. |
Keep Code Modular | Use lazy loading and maintain single responsibility in components. |
Future of React Suspense
Upcoming Features in React Suspense
The future of React Suspense looks promising with several upcoming features that aim to enhance its capabilities. Some of these features include:
- Improved Server-Side Rendering (SSR): React Suspense will allow for more efficient data fetching on the server, making it easier to render components with the necessary data already available.
- Enhanced Concurrent Features: With the introduction of new concurrent rendering techniques, Suspense will better manage loading states, ensuring smoother transitions and improved user experiences.
- Integration with the ‘use’ API: The new ‘use’ API in React 19 is set to revolutionize how we handle asynchronous operations in our components. This will simplify data fetching and state management, making it easier to implement Suspense in various scenarios.
Community Contributions and Plugins
The React community is actively contributing to the evolution of Suspense. Some notable contributions include:
- Custom Hooks: Developers are creating custom hooks that leverage Suspense for specific use cases, enhancing its functionality.
- Plugins: Various plugins are being developed to extend the capabilities of Suspense, making it easier to integrate with other libraries and frameworks.
- Documentation and Tutorials: The community is producing a wealth of resources to help developers understand and implement Suspense effectively.
How React Suspense is Evolving
React Suspense is evolving to meet the needs of modern web applications. Key areas of evolution include:
- Focus on Performance: Continuous improvements are being made to optimize performance, ensuring that applications remain responsive even during heavy data fetching.
- User Experience Enhancements: Future updates will prioritize user experience, providing better feedback during loading states and reducing the chances of abrupt content changes.
- Broader Adoption: As more developers recognize the benefits of Suspense, its adoption is expected to grow, leading to a more standardized approach to handling asynchronous operations in React applications.
The evolution of React Suspense is not just about new features; it’s about creating a better experience for developers and users alike. By simplifying complex tasks, React Suspense empowers developers to build faster and more efficient applications.
Comparing React Suspense with Other Solutions
React Suspense vs. Traditional Data Fetching
React Suspense changes how we handle data fetching in React applications. Here’s how it compares to traditional methods:
- Simplified Code: With Suspense, you don’t need to manage loading states manually. This leads to cleaner and more maintainable code.
- User Experience: Users see a loading indicator while data is being fetched, which improves their experience compared to a blank screen.
- Performance: Suspense ensures components only render when the required data is available, reducing unnecessary re-renders.
React Suspense vs. React Query
React Query is another popular solution for data fetching. Here’s a comparison:
Feature | React Suspense | React Query |
---|---|---|
Data Fetching | Built-in with Suspense | Requires setup |
Caching | Limited caching capabilities | Advanced caching options |
Loading States | Managed by Suspense | Manual management |
React Suspense vs. Redux
Redux is often used for state management. Here’s how it stacks up against Suspense:
- State Management: Redux is more complex and requires more boilerplate code compared to Suspense.
- Asynchronous Handling: Suspense simplifies async operations, while Redux needs middleware like Redux Thunk or Saga.
- Performance: By using Suspense, you can avoid unnecessary re-renders, which can be a challenge with Redux.
In summary, React Suspense provides a more streamlined approach to handling asynchronous data fetching, leading to better performance and user experience.
Troubleshooting React Suspense Issues
Common Errors and Fixes
When working with React Suspense, you might encounter some common issues. Here are a few errors and how to fix them:
- Loading State Not Displaying: Ensure that your fallback UI is correctly set up within the Suspense component.
- Data Not Fetching: Check if the data fetching function is correctly implemented and returns a promise.
- Error Boundaries Not Catching Errors: Make sure you are using Error Boundaries properly to catch errors during data fetching.
Debugging Suspense in Development
Debugging can be tricky, but here are some tips to help you:
- Use console logs to track the loading state and data fetching process.
- Check the network tab in your browser’s developer tools to see if requests are being made.
- Ensure that your components are wrapped in Suspense correctly.
Community Resources for Help
If you run into issues, consider these resources:
- React Documentation: The official React docs provide detailed explanations and examples.
- Stack Overflow: A great place to ask questions and find answers from the community.
- GitHub Issues: Check the React repository for reported issues and solutions.
Remember: React Suspense is a powerful tool, but it requires careful implementation to avoid common pitfalls. By following best practices and utilizing community resources, you can effectively troubleshoot issues and enhance your application’s performance.
Highlighted Points
- Excessive Suspense Boundaries: Avoid wrapping too many components in Suspense, as it can lead to performance issues.
- Improper Error Handling: Always use Error Boundaries alongside Suspense to manage errors effectively.
- Unnecessary Re-renders: Optimize your components to prevent unnecessary re-renders within Suspense boundaries.
If you’re facing problems with React Suspense, don’t worry! Many developers encounter similar issues. To get the best tips and solutions, visit our website. We have resources that can help you troubleshoot effectively and improve your coding skills. Start your journey today!
Conclusion
In summary, React Suspense is a game-changer for building better applications. It helps manage loading states smoothly, making sure users don’t see a blank screen while waiting for data. By using Suspense, developers can write cleaner code and improve the overall experience for users. As you explore React, consider using Suspense to enhance your projects. With its ability to handle asynchronous tasks effectively, you can create applications that are not only faster but also more enjoyable to use.
Frequently Asked Questions
What is React Suspense and why is it important?
React Suspense is a feature that helps manage loading states in your app while waiting for data or components to load. It makes your app smoother and more user-friendly.
How do I install React Suspense?
You can install React Suspense by using npm or yarn. Just run `npm install @suspensive/react` or `yarn add @suspensive/react` in your project.
Can I use React Suspense for lazy loading components?
Yes! React Suspense is great for lazy loading components. It allows you to load components only when they are needed, which can speed up your app.
What are some common mistakes when using React Suspense?
Some common mistakes include using too many Suspense boundaries, not handling errors properly, and causing unnecessary re-renders.
How does React Suspense improve user experience?
React Suspense shows a loading indicator while data is being fetched, so users don’t see a blank screen. This keeps them informed and improves their experience.
What is the difference between React Suspense and traditional data fetching?
Traditional data fetching often leads to complex code and loading states. React Suspense simplifies this by managing loading states automatically.
Is React Suspense compatible with server-side rendering?
Yes, React Suspense works with server-side rendering (SSR). It helps improve the performance of SSR by allowing parts of the page to load more efficiently.
Where can I find more resources to learn about React Suspense?
You can find more resources in the React documentation, coding blogs, and tutorials that focus on modern React features.