Unlocking the Power of GraphQL API: A Comprehensive Guide for Developers
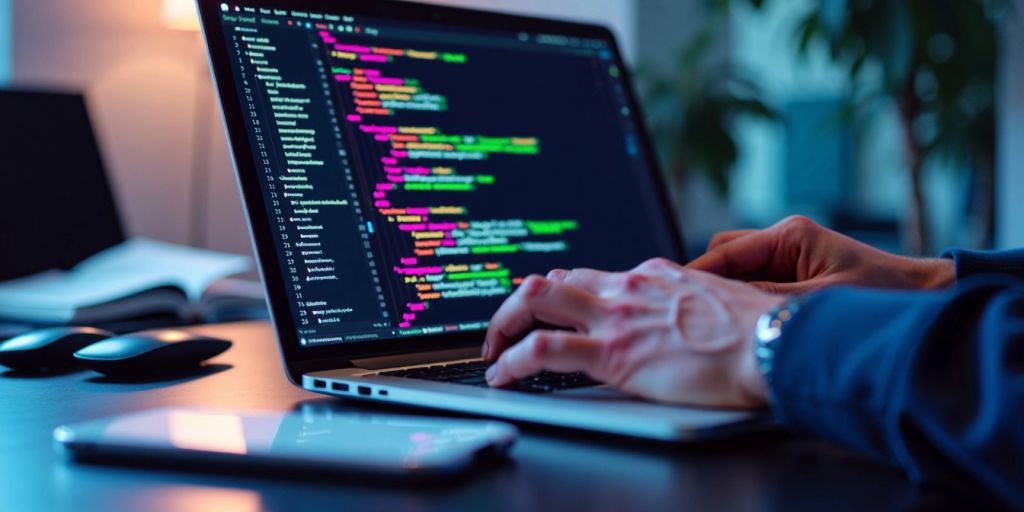
GraphQL is changing the way developers access and manage data. Unlike traditional APIs, GraphQL allows for more control over the data being retrieved, making it easier and faster to get exactly what you need. This guide will explore the key features of GraphQL, how to set it up, and best practices for using it effectively in your projects.
Key Takeaways
- GraphQL allows clients to choose exactly what data they need, reducing unnecessary data transfers.
- It uses a single endpoint for all requests, making it simpler than traditional APIs that require multiple endpoints.
- GraphQL supports real-time updates through subscriptions, enhancing user experience in applications.
- The schema-driven approach helps maintain clear data structures and relationships, aiding in development.
- GraphQL is flexible and can be integrated with various frontend frameworks, making it a versatile choice for developers.
Understanding the Basics of GraphQL API
What is GraphQL API?
GraphQL is a query language for your API and a server-side runtime for executing queries using a type system you define for your data. It allows clients to request exactly what they need, which helps avoid problems like over-fetching or under-fetching data.
Key Concepts of GraphQL
Here are some important concepts to understand:
- Schema: This defines the types of data available in your API.
- Query: This is how you request data from the server.
- Mutation: This is used to change data on the server.
- Subscription: This allows clients to get real-time updates from the server.
GraphQL vs REST APIs
GraphQL and REST APIs are different in several ways:
Feature | GraphQL | REST |
---|---|---|
Data Fetching | Client-driven, flexible queries | Predefined endpoints, fixed data structures |
Network Efficiency | Reduces over-fetching and under-fetching | Potential for multiple requests, data redundancy |
API Structure | Single endpoint, schema-driven | Multiple endpoints, resource-based |
Real-Time Updates | Supports subscriptions for real-time data | Limited to polling or websockets |
In summary, GraphQL provides a more efficient way to interact with APIs by allowing clients to specify exactly what data they need. This flexibility can lead to better performance and a smoother development experience.
Setting Up Your First GraphQL API
Installing GraphQL
To start using GraphQL, you need to install it in your project. Here’s how you can do it:
- Create a new project using Node.js.
- Install the necessary packages by running:
npm install graphql express express-graphql
- Set up your server to handle GraphQL requests.
Creating a Basic Schema
A schema defines the structure of your GraphQL API. Here’s a simple example:
const { buildSchema } = require('graphql');
const schema = buildSchema(`
type Query {
hello: String
}
`);
This schema has a single query called hello
that returns a string.
Running Your First Query
Once your schema is set up, you can run your first query. Here’s how:
- Set up an Express server to handle requests:
const express = require('express'); const { graphqlHTTP } = require('express-graphql'); const app = express(); app.use('/graphql', graphqlHTTP({ schema: schema, rootValue: { hello: () => 'Hello, world!' }, graphiql: true, })); app.listen(4000, () => console.log('Server running on http://localhost:4000/graphql'));
- Open your browser and go to
http://localhost:4000/graphql
. - Run the query:
{ hello }
You should see a response with the message "Hello, world!".
Setting up your first GraphQL API is a straightforward process that opens up many possibilities for your applications. With GraphQL, you can create flexible and efficient APIs that meet your needs.
Advanced GraphQL Queries and Mutations
Nested Queries
In GraphQL, queries and mutations allow you to fetch and modify data efficiently. One of the powerful features of GraphQL is the ability to perform nested queries. This means you can retrieve related data in a single request. For example, if you want to get a list of users along with their posts, you can structure your query like this:
query {
users {
id
name
posts {
title
content
}
}
}
This query fetches users and their associated posts in one go, reducing the number of requests needed.
Using Variables in Queries
Variables in GraphQL make your queries more dynamic. Instead of hardcoding values, you can use variables to pass in data. This is especially useful for filtering results. Here’s how you can use variables:
query GetUser($userId: ID!) {
user(id: $userId) {
name
email
}
}
When you execute this query, you can provide the userId
variable, allowing for flexible data retrieval.
Handling Mutations
Mutations are used to change data on the server. They can create, update, or delete records. Here’s a simple example of a mutation to create a new user:
mutation CreateUser($name: String!, $email: String!) {
createUser(name: $name, email: $email) {
id
name
}
}
When you call this mutation, you can pass the name
and email
variables to create a new user. This structured approach helps maintain clarity and consistency in your API operations.
In GraphQL, queries can traverse related objects and their fields, letting clients fetch lots of related data in one request, instead of making several roundtrips.
Summary
- Nested Queries allow fetching related data in one request.
- Variables make queries dynamic and flexible.
- Mutations handle data changes, ensuring clarity in operations.
By mastering these advanced features, you can unlock the full potential of GraphQL in your applications.
Optimizing GraphQL Performance
Reducing Query Complexity
To enhance performance, it’s crucial to reduce query complexity. Here are some strategies:
- Fetch only necessary data: Limit the fields you request to just what your components need.
- Avoid deep nesting: Keep your queries flat to minimize server load.
- Use fragments: Reuse common fields across queries to maintain clarity and reduce repetition.
Batching and Caching
Implementing effective caching strategies can significantly improve performance. Consider these options:
- Cache-and-Network: Fetch data from the cache first, then update it with a network request.
- Cache-First: Use cached data whenever possible, only hitting the network if the data isn’t available.
- Network-Only: Directly fetch data from the server, bypassing the cache.
Caching Strategy | Description |
---|---|
Cache-and-Network | Fetch from cache and update with network data. |
Cache-First | Use cached data first, then network if needed. |
Network-Only | Always fetch data from the server. |
Using Aliases and Fragments
Utilizing aliases and fragments can streamline your queries:
- Aliases: Rename fields in your queries to avoid conflicts and improve readability.
- Fragments: Define common fields once and reuse them, making your queries cleaner and easier to manage.
By optimizing your GraphQL queries, you can significantly enhance performance and user experience. Focus on fetching only the data you need to keep your application running smoothly.
Integrating GraphQL with Frontend Frameworks
GraphQL with React
Integrating GraphQL with React is a popular choice among developers. One of the most straightforward ways to integrate GraphQL is by building a GraphQL layer on top of your REST API. In this approach, you’ll use Apollo Server to manage your data fetching. Here are some key steps to follow:
- Set up Apollo Client in your React application.
- Create a GraphQL schema that defines your data types.
- Use hooks like
useQuery
to fetch data in your components.
GraphQL with Vue.js
Vue.js also works well with GraphQL. You can use Apollo Client with Vue to manage your data. Here’s how:
- Install the Apollo Client and Vue Apollo.
- Set up your Apollo Provider in your main Vue instance.
- Use the
apollo
option in your components to fetch data.
GraphQL with Angular
For Angular developers, integrating GraphQL is seamless with Apollo Angular. Follow these steps:
- Install Apollo Angular and GraphQL dependencies.
- Set up Apollo Client in your Angular module.
- Use Apollo services in your components to execute queries and mutations.
Integrating GraphQL with frontend frameworks allows for efficient data management and enhances user experience. By leveraging the strengths of each framework, developers can create powerful applications that respond quickly to user interactions.
Real-Time Data with GraphQL Subscriptions
Setting Up Subscriptions
GraphQL has a special type of operation called subscription that allows you to add real-time features to your applications. To set up subscriptions, you need to establish a WebSocket connection. Here’s a simple way to do it:
- Install Apollo Client: Make sure you have Apollo Client installed in your project.
- Create WebSocket Link: Use the WebSocketLink to connect to your GraphQL server.
- Use the Subscription Hook: In your component, use the
useSubscription
hook to listen for updates.
Handling Real-Time Updates
When you set up subscriptions, your application can receive updates as they happen. This is great for applications that need to show live data, like chat apps or dashboards. Here’s how you can handle real-time updates:
- Listen for Changes: Your app will automatically receive new data when it changes on the server.
- Update the UI: Use the data received to update your user interface instantly.
- Manage Loading States: Show loading indicators while waiting for data.
Best Practices for Subscriptions
To make the most of GraphQL subscriptions, consider these best practices:
- Limit Data: Only subscribe to the data you really need to avoid unnecessary load.
- Handle Errors: Make sure to manage any errors that occur during the subscription.
- Clean Up: Unsubscribe when the component unmounts to prevent memory leaks.
Subscriptions are a powerful way to keep your application data fresh and responsive. They allow you to create a more engaging user experience by providing instant updates without needing to refresh the page.
By following these steps, you can effectively implement real-time data handling in your applications using GraphQL subscriptions. This will enhance user interaction and keep your data up-to-date seamlessly.
Security Best Practices for GraphQL API
Authentication and Authorization
To keep your GraphQL API safe, it’s crucial to implement strong authentication and authorization methods. Here are some key points to consider:
- Use tokens (like JWT) for user authentication.
- Ensure that users can only access data they are allowed to see.
- Regularly review user permissions to maintain security.
Rate Limiting
Rate limiting helps prevent abuse of your API. By controlling how many requests a user can make in a given time, you can protect your server from overload. Here are some strategies:
- Set a maximum number of requests per minute.
- Use a timeout for excessive requests.
- Throttle queries based on server response time.
Preventing Over-Querying
GraphQL allows clients to request a lot of data, which can lead to performance issues. To avoid this, consider the following:
- Set a maximum depth for queries to limit how complex they can be.
- Analyze query complexity to reject overly complicated requests.
- Regularly audit your schemas and query patterns for vulnerabilities.
By following these best practices, you can significantly enhance the security of your GraphQL API and protect your application from common threats.
Testing and Debugging GraphQL APIs
Writing Test Cases
Testing your GraphQL API is crucial to ensure it behaves as expected. Here are some steps to follow:
- Define your test cases based on the queries and mutations you want to validate.
- Use tools like Jest or Mocha to write your tests.
- Ensure you cover both successful responses and error scenarios.
Using GraphQL Playground
GraphQL Playground is a powerful tool for testing your API. It allows you to:
- Execute queries and mutations directly.
- View the schema and documentation.
- Test different inputs easily.
Common Debugging Techniques
Debugging is essential for fixing issues in your GraphQL API. Here are some common techniques:
- Log requests and responses to understand what’s happening.
- Use breakpoints in your code to pause execution and inspect variables.
- Implement error handling to catch and manage errors gracefully.
Effective testing and debugging can significantly improve the reliability of your GraphQL API.
Debugging Tools and Techniques
Utilize various tools to streamline your debugging process:
- Postman: Great for testing API endpoints.
- Chrome DevTools: Useful for inspecting network requests.
- Requestly: This tool helps you intercept and modify APIs on the fly using HTTP rules, making it easier to debug and mock GraphQL APIs.
By following these practices, you can ensure your GraphQL API is robust and performs well under various conditions.
GraphQL API in Production
Monitoring and Logging
To ensure your GraphQL API runs smoothly in production, it’s essential to implement effective monitoring and logging. Here are some key points to consider:
- Track performance metrics: Monitor response times, error rates, and request counts.
- Log errors: Capture detailed error logs to help diagnose issues quickly.
- Use analytics tools: Tools like Grafana or Prometheus can provide insights into your API’s performance.
Handling Errors Gracefully
When errors occur, how you handle them can greatly affect user experience. Here are some strategies:
- Return meaningful error messages: Provide clear and helpful messages to users.
- Use error codes: Implement standardized error codes to categorize issues.
- Fallback mechanisms: Have backup plans in case of failures, such as serving cached data.
Scaling Your GraphQL API
As your application grows, scaling your API becomes crucial. Consider these approaches:
- Load balancing: Distribute incoming requests across multiple servers.
- Horizontal scaling: Add more servers to handle increased traffic.
- Optimize queries: Ensure that your queries are efficient to reduce server load.
In production, it’s vital to prioritize both performance and user experience.
By following these practices, you can ensure that your GraphQL API remains robust and responsive, even under heavy load. Remember, monitoring, error handling, and scaling are key components of a successful production environment.
Case Studies and Real-World Applications
GraphQL in E-commerce
In the e-commerce sector, GraphQL is transforming how businesses manage their data. By using GraphQL, companies can streamline their operations and enhance customer experiences. Here are some key benefits:
- Efficient Data Retrieval: GraphQL allows clients to request only the data they need, reducing unnecessary data transfer.
- Unified Data Access: It acts as a single point of access for various data sources, simplifying integration.
- Improved Performance: Faster load times lead to better user satisfaction.
GraphQL for Mobile Apps
Mobile applications often face challenges with limited resources. GraphQL helps by:
- Minimizing data transfer, which is crucial for mobile devices.
- Allowing developers to fetch only the necessary data, optimizing performance.
- Enhancing battery life by reducing network requests.
GraphQL in Microservices Architecture
In a microservices setup, GraphQL serves as a single pane of glass into your entire organization. It enables:
- Simplified Data Access: Clients can fetch data from multiple services in one request.
- Reduced Network Latency: Fewer API calls mean faster responses.
- Better Developer Experience: Developers can focus on building features rather than managing multiple APIs.
GraphQL is not just a technology; it’s a way to rethink how we interact with data across various platforms and services.
By leveraging GraphQL, organizations can unlock new possibilities and drive innovation in their applications.
Future Trends in GraphQL API Development
Federation and Schema Stitching
The future of GraphQL is likely to see federation and schema stitching becoming more common. These techniques allow developers to combine multiple GraphQL services into a single API. This means that teams can work independently on different parts of an application without stepping on each other’s toes.
GraphQL and Serverless
Another trend is the integration of GraphQL with serverless architectures. This approach allows developers to build applications without managing servers, making it easier to scale and deploy. Serverless functions can respond to GraphQL queries, providing a flexible and cost-effective solution for modern applications.
Evolving GraphQL Standards
As GraphQL continues to grow, we can expect evolving standards that improve its functionality and usability. This includes better tools for caching, security, and performance optimization. Developers will benefit from a more mature ecosystem that supports their needs.
Embracing these trends will help developers unlock the full potential of GraphQL, making it a powerful tool for building modern applications.
Summary of Future Trends
Trend | Description |
---|---|
Federation and Stitching | Combining multiple GraphQL services into one API. |
Serverless Integration | Using serverless functions to handle GraphQL queries. |
Evolving Standards | Improved tools and practices for better performance and security. |
Conclusion
In conclusion, the future of GraphQL API development looks promising. By keeping an eye on these trends, developers can stay ahead of the curve and create more efficient and scalable applications. The evolution of API development will likely involve a combination of both approaches, with developers choosing the best tool for their specific needs.
As we look ahead, GraphQL API development is set to evolve in exciting ways. With the rise of real-time data and improved performance, developers will need to adapt to these changes. If you’re eager to stay ahead in this fast-paced field, visit our website to explore resources that can help you master these trends and enhance your skills!
Conclusion
In summary, GraphQL API changes how we work with data, making it easier and faster to get what we need. By using GraphQL, developers can cut down on unnecessary data requests and improve the speed of their applications. This tool is great for many types of projects, whether you’re building apps for phones, managing content, or working with Internet of Things (IoT) devices. Embracing GraphQL can help you create better user experiences and make your applications more powerful. So, if you want to improve your coding skills and build amazing apps, start using GraphQL today!
Frequently Asked Questions
What is GraphQL API?
GraphQL API is a way to fetch data that lets you ask for exactly what you need. Unlike other methods, it allows you to get related data in one go.
How does GraphQL compare to REST APIs?
GraphQL uses a single endpoint to get all the data you need, while REST APIs often require multiple endpoints. This makes GraphQL more efficient.
What are the main advantages of using GraphQL?
Some benefits of GraphQL include flexible data fetching, reduced network requests, and a clear structure of data.
Can I use GraphQL for real-time data?
Yes! GraphQL supports subscriptions, which allow you to get real-time updates when data changes.
How do I set up a GraphQL API?
To set up GraphQL, you need to install the necessary tools, create a schema that defines your data structure, and run your first query.
What is a GraphQL schema?
A schema in GraphQL defines the types of data you can request and how they relate to each other. It acts like a contract between the client and server.
What are mutations in GraphQL?
Mutations are how you change data on the server with GraphQL. They allow you to add, update, or delete data.
How can I test my GraphQL API?
You can test your GraphQL API using tools like GraphQL Playground, which lets you write and run queries to see how your API responds.