Unlocking the Power of CUDA Programming: A Comprehensive Guide for Beginners
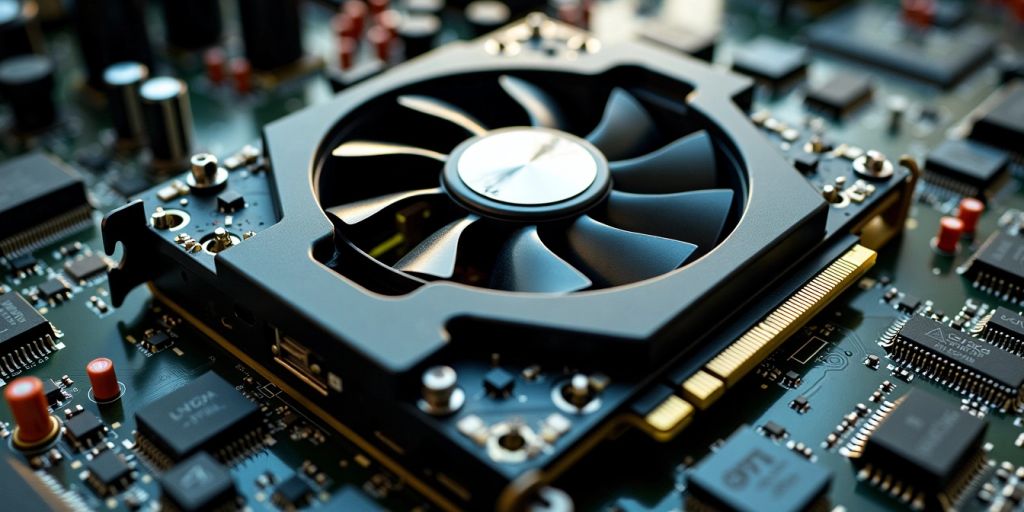
CUDA programming lets you use the power of NVIDIA graphics cards to speed up computing tasks. This guide is designed to help beginners understand the basics of CUDA, set up their development environment, and start writing their first CUDA programs. You’ll learn about key concepts, performance tips, and real-world applications, making it easier for you to dive into the world of parallel computing.
Key Takeaways
- CUDA is a tool that helps programmers use NVIDIA GPUs for fast computing.
- Setting up your CUDA environment is essential for writing and running CUDA programs.
- Understanding threads, blocks, and grids is key to effective CUDA programming.
- Optimizing memory usage and thread management can significantly boost performance.
- There are many real-world uses for CUDA, including in science, AI, and graphics.
Understanding the Basics of CUDA Programming
What is CUDA?
CUDA, which stands for Compute Unified Device Architecture, is a programming model created by NVIDIA. It allows developers to use NVIDIA GPUs to speed up general-purpose computing tasks. This means you can run many calculations at once, making your programs faster and more efficient.
The Evolution of CUDA
CUDA has come a long way since its introduction. Here are some key milestones in its development:
- 2006: CUDA was first released, enabling developers to write programs that run on GPUs.
- 2010: The introduction of CUDA 3.0 brought support for dynamic parallelism, allowing kernels to launch other kernels.
- 2020: CUDA 11 was released, enhancing performance and adding new features for machine learning and AI applications.
Key Concepts in CUDA Programming
To get started with CUDA, it’s important to understand some basic concepts:
- Threads: The smallest unit of execution in CUDA. Each thread can run a part of your program.
- Blocks: A group of threads that can cooperate and share data.
- Grids: A collection of blocks that work together to solve a problem.
Concept | Description |
---|---|
Threads | Smallest unit of execution |
Blocks | Groups of threads that can share data |
Grids | Collections of blocks working on a problem |
Understanding these concepts is crucial for writing efficient CUDA programs. CUDA programming with C++ is tailored for both beginners and experienced developers, covering fundamental concepts and advanced techniques.
Setting Up Your CUDA Development Environment
Installing the CUDA Toolkit
To start programming with CUDA, you first need to install the CUDA Toolkit. This toolkit includes all the necessary tools and libraries for CUDA development. Here’s how to do it:
- Visit the NVIDIA website.
- Choose your operating system (Windows, Linux, or macOS).
- Follow the cuda installation guide for linux to ensure proper setup.
Configuring Your IDE for CUDA
After installing the toolkit, you need to set up your Integrated Development Environment (IDE) to work with CUDA. Here are the steps:
- Open your IDE settings.
- Add the CUDA Toolkit path to your project settings.
- Ensure that your IDE recognizes CUDA files (.cu).
Verifying Your Installation
Once everything is set up, it’s important to verify that your installation is working correctly. You can do this by:
- Running a sample CUDA program that comes with the toolkit.
- Checking the CUDA compiler version using the command
nvcc --version
. - Ensuring that your IDE can compile and run CUDA code without errors.
Setting up your CUDA environment correctly is crucial for a smooth development experience. Make sure to follow each step carefully to avoid common issues.
CUDA Programming Model and Architecture
Threads, Blocks, and Grids
In CUDA, the basic unit of execution is a thread. Threads are organized into blocks, and blocks are grouped into grids. This structure allows for efficient parallel processing. Here’s a simple breakdown:
- Threads: The smallest unit of execution.
- Blocks: A collection of threads that can communicate with each other.
- Grids: A collection of blocks that execute a kernel.
This hierarchy helps in managing resources and optimizing performance.
Memory Hierarchy in CUDA
CUDA has a unique memory structure that is crucial for performance. Here’s a quick overview:
Memory Type | Description | Scope |
---|---|---|
Global Memory | Accessible by all threads, but slow | Device-wide |
Shared Memory | Fast, shared among threads in a block | Block-wide |
Local Memory | Private to each thread, used for variables | Thread-specific |
Constant Memory | Read-only, fast access for all threads | Device-wide |
Texture Memory | Optimized for 2D spatial locality | Device-wide |
Understanding this hierarchy is key to writing efficient CUDA programs.
Execution Model
The execution model in CUDA is designed for parallelism. When a kernel is launched, it runs on the GPU, and multiple threads execute simultaneously. Here are some important points:
- Kernel Launch: A kernel is a function that runs on the GPU.
- Synchronization: Threads within a block can synchronize, but blocks cannot.
- Scalability: The model allows for scaling up to thousands of threads, making it suitable for large computations.
The CUDA programming model enables developers to leverage the power of GPUs for high-performance computing tasks, making it a vital tool in modern programming.
By understanding these concepts, you can start to unlock the full potential of CUDA programming and create efficient applications that utilize the power of NVIDIA GPUs effectively.
Writing Your First CUDA Program
Hello World in CUDA
To get started with CUDA, the first program you’ll write is often a simple "Hello World". This program will help you understand the basic structure of a CUDA application. Here’s a simple example:
#include <stdio.h>
__global__ void helloWorld() {
printf("Hello, World from CUDA!\n");
}
int main() {
helloWorld<<<1, 1>>>();
cudaDeviceSynchronize();
return 0;
}
This code demonstrates how to launch a kernel. The <<<1, 1>>>
indicates that you are launching one block with one thread.
Compiling and Running CUDA Programs
To compile and run your CUDA program, follow these steps:
- Open your terminal or command prompt.
- Navigate to the directory where your CUDA file is saved.
- Compile the program using the following command:
nvcc -o hello hello.cu
- Run the program with:
./hello
Debugging Tips for Beginners
Debugging can be tricky when you start with CUDA. Here are some tips to help you:
- Use
cudaMemcpy
to check data transfer between host and device. - Check for errors after CUDA API calls using
cudaGetLastError()
. - Use debugging tools like
cuda-gdb
for more complex issues.
Remember, every expert was once a beginner. Don’t hesitate to experiment and learn from your mistakes!
Optimizing CUDA Performance
Memory Optimization Techniques
To get the most out of your CUDA programs, boosting CUDA efficiency is crucial. Here are some techniques to optimize memory usage:
- Use Shared Memory: This is faster than global memory and can significantly speed up your program.
- Minimize Memory Transfers: Try to reduce the amount of data sent between the CPU and GPU.
- Coalesce Memory Access: Ensure that threads access memory in a way that minimizes delays.
Efficient Thread Management
Managing threads effectively can lead to better performance. Consider these strategies:
- Choose the Right Number of Threads: Too many threads can cause overhead, while too few can underutilize the GPU.
- Use Thread Blocks Wisely: Organize threads into blocks that fit well with the GPU architecture.
- Avoid Divergence: Keep threads in a block executing the same instruction to prevent delays.
Using CUDA Profiling Tools
Profiling tools can help you identify bottlenecks in your code. Here are some useful tools:
- NVIDIA Visual Profiler: This tool provides a visual representation of your program’s performance.
- Nsight Compute: A command-line tool that gives detailed performance metrics.
- CUDA-GDB: A debugger for CUDA applications that helps find issues in your code.
Optimizing your CUDA programs can lead to significant performance gains, making your applications faster and more efficient.
By applying these techniques, you can unlock the full potential of your CUDA applications and ensure they run smoothly on NVIDIA GPUs.
Advanced CUDA Programming Techniques
Dynamic Parallelism
Dynamic Parallelism is a powerful feature in CUDA that allows a kernel to create new threads directly from the GPU. This means that a kernel can launch other kernels without needing the CPU. This reduces the time spent transferring data between the CPU and GPU. Here are some key points about Dynamic Parallelism:
- Adaptive Workload Handling: Threads can make decisions in real-time based on the data they process.
- Reduced CPU-GPU Overhead: It minimizes unnecessary data transfers, enhancing performance.
- Greater Flexibility: It simplifies code design for complex tasks.
Stream and Event Management
Streams and events are essential for managing multiple tasks in CUDA. They help in executing tasks concurrently, which can significantly improve performance. Here’s how to effectively use them:
- Create Streams: Use streams to manage different tasks that can run simultaneously.
- Use Events: Events help in synchronizing tasks and measuring execution time.
- Optimize Memory Transfers: Ensure that memory transfers do not block the execution of kernels.
Unified Memory
Unified Memory simplifies memory management in CUDA by allowing the CPU and GPU to share data seamlessly. This means you don’t have to manually copy data between the two. Here are some benefits:
- Simplified Programming: Developers can focus on algorithms rather than memory management.
- Automatic Data Migration: The system automatically moves data between CPU and GPU as needed.
- Improved Performance: It can lead to better performance in applications with complex memory access patterns.
Using advanced strategies for high-performance GPU programming with NVIDIA CUDA can significantly enhance your applications. By leveraging features like Dynamic Parallelism, Stream Management, and Unified Memory, you can unlock the full potential of your GPU.
Common Pitfalls and How to Avoid Them
Debugging Common Errors
When working with CUDA, you might run into various errors. Here are some common ones:
- Outdated or incompatible drivers: Ensure your NVIDIA graphics drivers are up to date to avoid issues with CUDA.
- Memory access violations: Always check your memory allocations and ensure you’re not accessing out-of-bounds.
- Kernel launch failures: Verify that your kernel configurations (like grid and block sizes) are correct.
Performance Bottlenecks
To keep your CUDA programs running smoothly, watch out for these bottlenecks:
- Memory transfer times: Minimize data transfers between the CPU and GPU.
- Thread divergence: Try to keep threads in a warp executing the same instruction.
- Uncoalesced memory accesses: Ensure that your memory accesses are coalesced for better performance.
Best Practices for Stable Code
To write stable and efficient CUDA code, follow these tips:
- Use error checking after CUDA API calls to catch issues early.
- Optimize memory usage by using shared memory wisely.
- Regularly profile your code to identify and fix performance issues.
Remember, avoiding common pitfalls can save you a lot of time and frustration in your CUDA programming journey!
Real-World Applications of CUDA Programming
CUDA programming has transformed various fields by enabling faster computations and more efficient processing. Here are some key areas where CUDA is making a significant impact:
Scientific Computing
- High-Performance Simulations: CUDA allows scientists to run complex simulations much faster than traditional methods.
- Data Analysis: Large datasets can be processed quickly, making it easier to derive insights.
- Modeling: Researchers can create detailed models of physical systems, such as climate models or molecular dynamics.
Machine Learning and AI
- Deep Learning: CUDA is widely used in training deep neural networks, significantly speeding up the process.
- Real-Time Inference: It enables quick predictions from trained models, which is crucial for applications like image recognition.
- Data Preprocessing: Large datasets can be prepared and processed efficiently, improving the overall workflow.
Graphics and Visualization
- Rendering: CUDA enhances the speed of rendering graphics, making it essential for video games and simulations.
- Image Processing: Tasks like filtering and transformations can be done much faster.
- 3D Visualization: Complex 3D models can be visualized in real-time, aiding in design and analysis.
In summary, CUDA programming is a powerful tool that opens up new possibilities in various fields. It allows developers to harness the full potential of GPUs, leading to faster and more efficient applications.
Application Area | Key Benefits |
---|---|
Scientific Computing | Faster simulations and data analysis |
Machine Learning and AI | Speedy training and inference |
Graphics and Visualization | Enhanced rendering and processing |
Resources for Further Learning
Official Documentation and Guides
- NVIDIA CUDA Toolkit Documentation: This is the main resource for understanding CUDA. It provides detailed information about the CUDA Toolkit, which is a software package that includes everything you need to develop CUDA applications.
- CUDA C Programming Guide: A comprehensive guide that covers the programming model, architecture, and best practices.
- CUDA Samples: A collection of sample projects that demonstrate various CUDA features and techniques.
Online Courses and Tutorials
- Coursera: Offers courses on CUDA programming, often in partnership with universities.
- Udacity: Provides a nanodegree program focused on parallel programming with CUDA.
- YouTube: Many channels offer free tutorials and walkthroughs on CUDA programming.
Community Forums and Support
- NVIDIA Developer Forums: A great place to ask questions and share knowledge with other CUDA developers.
- Stack Overflow: Search for CUDA-related questions or ask your own to get help from the community.
- Reddit: Subreddits like r/CUDA can be useful for discussions and resources.
Learning CUDA can be challenging, but with the right resources, you can master it and unlock its full potential!
Future Trends in CUDA Programming
Emerging Technologies
The world of CUDA programming is rapidly evolving. Here are some key trends to watch:
- Increased use of AI: CUDA is becoming essential in developing AI applications, allowing for faster processing and better performance.
- Integration with Quantum Computing: As quantum computing grows, CUDA may adapt to leverage its capabilities.
- Enhanced Libraries: New CUDA libraries are expanding accelerated computing into new areas, delivering order-of-magnitude speedups and reducing energy consumption.
CUDA in Cloud Computing
Cloud computing is changing how we use CUDA. Here’s how:
- Scalability: Developers can easily scale their applications in the cloud.
- Cost Efficiency: Using cloud resources can lower costs for running CUDA applications.
- Accessibility: More developers can access powerful GPUs without needing expensive hardware.
The Role of AI in CUDA Development
AI is playing a significant role in shaping CUDA programming. Key points include:
- Automated Optimization: AI can help optimize CUDA code for better performance.
- Smart Resource Management: AI can manage GPU resources more effectively, improving efficiency.
- Predictive Analysis: AI can analyze workloads and predict performance bottlenecks before they occur.
The future of CUDA programming is bright, with innovations that promise to enhance performance and accessibility for developers everywhere.
As we look ahead, CUDA programming is set to evolve in exciting ways. With advancements in hardware and software, developers will have more tools at their disposal to create faster and more efficient applications. If you’re eager to stay ahead in this field, visit our website to start your coding journey today!
Conclusion
In conclusion, CUDA programming opens up a world of possibilities for anyone looking to speed up their computing tasks. By learning the basics of CUDA, you can tap into the power of NVIDIA GPUs, making your programs run faster and more efficiently. Remember, practice is key! Start with simple projects and gradually take on more complex challenges. With time and effort, you’ll become skilled in CUDA programming. So, dive in, explore, and unlock the full potential of your coding abilities!
Frequently Asked Questions
What is CUDA?
CUDA stands for Compute Unified Device Architecture. It’s a tool created by NVIDIA that helps programmers use the power of NVIDIA graphics cards to speed up their calculations.
How do I start programming with CUDA?
To begin, you need to install the CUDA Toolkit on your computer. This toolkit has everything you need to write and run CUDA programs.
What are threads in CUDA?
In CUDA, threads are the smallest units of work. They run tasks in parallel, which means many threads can work at the same time to finish a job faster.
Can I run CUDA programs on any computer?
No, you need a computer with an NVIDIA GPU that supports CUDA. Not all graphics cards can run CUDA programs.
What is the difference between a kernel and a thread?
A kernel is a function that runs on the GPU, while a thread is a single instance of that function. Many threads can run the same kernel at once.
How can I check if CUDA is installed correctly?
You can check your CUDA installation by running a command in your terminal: `nvcc –version`. This will show you the version of the CUDA compiler.
What are some common mistakes when starting with CUDA?
Some common mistakes include not managing memory properly, not understanding the thread hierarchy, and not checking for errors in your code.
Where can I find help for learning CUDA?
You can find help on NVIDIA’s official documentation, online forums, and various coding tutorials that focus on CUDA programming.