Unlocking Efficiency: How Greedy Algorithms Can Optimize Problem Solving
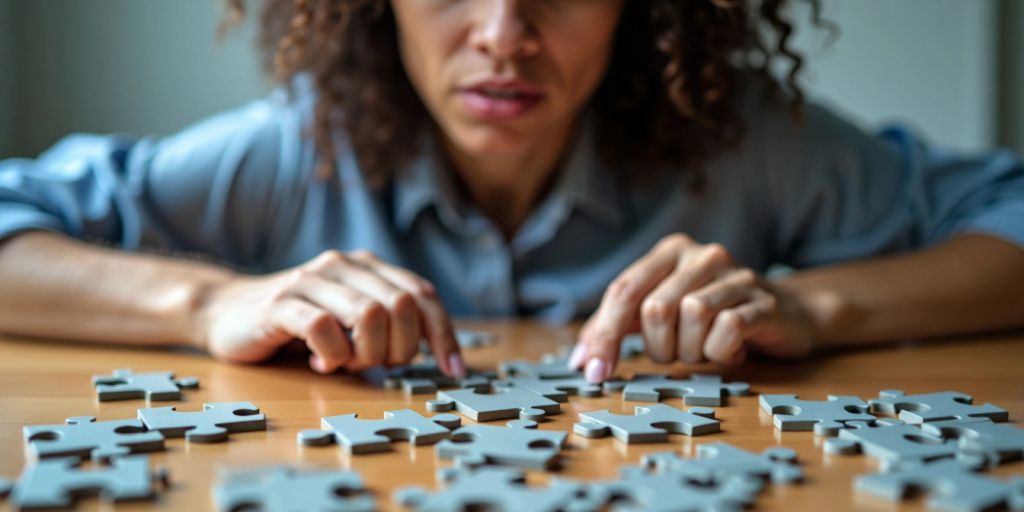
Greedy algorithms are a special type of problem-solving method that makes the best choice at each step. They are often used because they are easy to understand and can solve certain problems quickly. However, they may not always lead to the best overall solution. In this article, we will explore what greedy algorithms are, their advantages and disadvantages, and how they can be applied to various problems.
Key Takeaways
- Greedy algorithms make the best choice at each step without looking ahead.
- They are simple to implement and can be very efficient for specific problems.
- Not all problems can be solved optimally with greedy algorithms.
- Greedy methods are useful in real-life situations like scheduling and resource allocation.
- Understanding the strengths and weaknesses of greedy algorithms is crucial for problem-solving.
Understanding the Basics of Greedy Algorithms
Definition and Key Concepts
A greedy algorithm is a method for solving problems by making the best choice at each step. It focuses on making the most immediate, or local, optimal choice with the hope that these choices will lead to a global optimum. This approach is often used in optimization problems where the goal is to find the best solution among many possible options.
How Greedy Algorithms Work
Greedy algorithms work by following a simple process:
- Define the problem clearly.
- Identify the greedy choice that seems best at the moment.
- Make the choice and update the current state.
- Repeat until a solution is found.
Examples of Greedy Algorithms
Here are some common examples of greedy algorithms:
- Activity Selection Problem: Choosing the maximum number of activities that don’t overlap.
- Job Sequencing Problem: Scheduling jobs to maximize profit.
- Huffman Coding: A method for data compression that assigns shorter codes to more frequent symbols.
Greedy algorithms are often simple to implement and can be very efficient for certain types of problems. However, they may not always yield the best solution.
Example Problem | Description |
---|---|
Activity Selection | Maximize non-overlapping activities |
Job Sequencing | Maximize profit from jobs |
Huffman Coding | Efficient data compression |
Advantages of Using Greedy Algorithms
Simplicity and Ease of Implementation
Greedy algorithms are known for their simplicity. They are straightforward to understand and implement, making them a popular choice for many programmers. Here are some key points:
- Quick to code: You can write them in a short amount of time.
- Easy to debug: Fewer lines of code mean fewer chances for errors.
- Clear logic: The decision-making process is easy to follow.
Efficiency in Certain Scenarios
In many cases, greedy algorithms can provide efficient solutions. They often have a lower time complexity compared to other methods. For example:
- Fast execution: They can solve problems quickly, especially when the problem structure allows it.
- Optimal for specific problems: Some problems are perfectly suited for greedy approaches, leading to optimal solutions.
- Low resource usage: They typically require less memory than more complex algorithms.
Real-World Applications
Greedy algorithms are widely used in various fields. Here are some common applications:
- Network routing: Algorithms like Dijkstra’s help find the shortest path in networks.
- Resource allocation: They can efficiently assign resources to tasks.
- Data compression: Huffman coding is a classic example of using greedy methods to compress data.
Greedy algorithms are often the go-to choice for quick solutions, especially when the problem allows for local optimization.
Application | Description |
---|---|
Network Routing | Finding shortest paths in graphs |
Resource Allocation | Assigning tasks to minimize waiting time |
Data Compression | Compressing data using optimal coding techniques |
Common Problems Solved by Greedy Algorithms
Activity Selection Problem
The Activity Selection Problem is a classic example where the goal is to select the maximum number of activities that don’t overlap. By always choosing the next activity that finishes the earliest, we can ensure the most efficient use of time. Here’s how it works:
- Sort the activities by their finish times.
- Select the first activity and then continue selecting the next activity that starts after the last selected one finishes.
Job Sequencing Problem
In the Job Sequencing Problem, the aim is to schedule jobs to maximize profit. Each job has a deadline and a profit. The greedy approach involves:
- Sorting jobs by profit in descending order.
- Scheduling each job in the latest available time slot before its deadline.
- This ensures that we maximize profit while meeting deadlines.
Huffman Coding
Huffman Coding is used for data compression. It assigns variable-length codes to input characters based on their frequencies. The greedy method works as follows:
- Create a priority queue of all characters based on their frequencies.
- Combine the two least frequent characters to form a new node.
- Repeat until only one node remains, which represents the optimal prefix code.
Greedy algorithms are powerful tools for solving optimization problems, but they may not always yield the best solution. Understanding their application is key to effective problem-solving.
Limitations and Disadvantages of Greedy Algorithms
Suboptimal Solutions
Greedy algorithms do not always guarantee the best solution. They make decisions based on immediate benefits, which can lead to suboptimal outcomes. This means that while they can be fast, they might not always be right.
Dependency on Problem Structure
The effectiveness of a greedy algorithm heavily relies on the structure of the problem. If the problem does not have the properties that allow for a greedy approach, the algorithm may fail to find a good solution. For example:
- Problems that require a global view of the data.
- Situations where future consequences of current choices matter.
- Problems with multiple optimal solutions.
Comparison with Other Algorithms
When compared to other algorithms like dynamic programming, greedy algorithms can be less effective. Here’s a quick comparison:
Feature | Greedy Algorithms | Dynamic Programming |
---|---|---|
Optimal Solution | Not guaranteed | Guaranteed |
Time Complexity | Generally faster | Generally slower |
Problem Types | Specific cases | Broader range |
Greedy algorithms are useful for quick solutions, but they can miss the best answer. Always consider the problem type before choosing this method.
Greedy Algorithms in Graph Theory
Kruskal’s Minimum Spanning Tree
Kruskal’s algorithm is a popular greedy method used to find the minimum spanning tree of a graph. It works by sorting all the edges and adding them one by one, ensuring no cycles are formed. The steps are:
- Sort all edges in non-decreasing order of their weight.
- Pick the smallest edge. Check if it forms a cycle with the spanning tree formed so far. If not, include it.
- Repeat until there are enough edges in the spanning tree.
Prim’s Minimum Spanning Tree
Prim’s algorithm also finds the minimum spanning tree but starts from a single vertex. It grows the spanning tree by adding the smallest edge from the tree to a vertex not yet in the tree. The process is:
- Start with any vertex and mark it.
- Find the smallest edge connecting the marked vertex to an unmarked vertex.
- Add this edge and mark the new vertex.
- Repeat until all vertices are marked.
Dijkstra’s Shortest Path Algorithm
Dijkstra’s algorithm is used to find the shortest path from a source vertex to all other vertices in a graph. It works as follows:
- Set the distance to the source to zero and all others to infinity.
- Mark the source vertex as current.
- For the current vertex, consider all its unvisited neighbors and calculate their tentative distances.
- Once all neighbors are considered, mark the current vertex as visited.
- Repeat until all vertices are visited.
Greedy algorithms are powerful tools in graph theory, but they have limited applicability: some problems may not be suited for a greedy approach, requiring other algorithms like dynamic programming or backtracking to find optimal solutions.
Greedy Algorithms for Array Problems
Minimum Product Subset
Greedy algorithms can be used to find the minimum product subset of an array. The idea is to select the smallest elements to minimize the product. Here’s how it works:
- Identify negative and positive numbers in the array.
- If there are an even number of negative numbers, include all of them.
- If there are an odd number of negative numbers, exclude the one with the largest absolute value.
Maximize Array Sum
To maximize the array sum after K negations, we can use a greedy approach:
- Sort the array in ascending order.
- Negate the smallest K elements.
- Sum the array to get the maximum possible value.
Partitioning Arrays
Partitioning an array into two subarrays can also be solved using a greedy algorithm. The goal is to maximize the difference between the sums of the two subarrays. The steps include:
- Sort the array in descending order.
- Alternate adding elements to each subarray.
- Calculate the sums and find the difference.
Greedy algorithms are often effective for array problems, but they may not always yield the best solution. Understanding the problem structure is key to applying the right approach.
Problem Type | Description |
---|---|
Minimum Product Subset | Find the subset with the smallest product. |
Maximize Array Sum | Maximize sum after K negations. |
Partitioning Arrays | Maximize the difference between two subarray sums. |
Steps to Develop a Greedy Algorithm
Defining the Problem
To create a greedy algorithm, the first step is to clearly define the problem you want to solve. This includes:
- Stating the objective you want to optimize.
- Understanding the constraints of the problem.
- Identifying the input and expected output.
Identifying the Greedy Choice
Next, you need to determine the greedy choice. This means:
- Finding the best option available at each step.
- Ensuring that this choice is based on the current state of the problem.
- Recognizing that this choice should lead towards a solution, even if it’s not the best overall.
Iterative Process
Finally, you will implement an iterative process:
- Make the greedy choice: Select the best option based on your previous step.
- Update the current state: Adjust your problem’s state based on the choice made.
- Repeat: Continue this process until you reach a solution.
Greedy algorithms are often more efficient than dynamic programming because they generally involve a simple decision-making process at each step.
By following these steps, you can effectively develop a greedy algorithm that addresses various optimization problems.
Greedy Algorithms in Operating Systems
Memory Management Algorithms
Greedy algorithms play a significant role in memory management within operating systems. They help in efficiently allocating memory to processes. Here are some common greedy approaches:
- First Fit: Allocates the first available memory block that is large enough.
- Best Fit: Chooses the smallest available block that meets the requirement, aiming to leave larger blocks free for future use.
- Worst Fit: Allocates the largest available block, hoping to leave enough space for larger processes later.
Job Scheduling Algorithms
In job scheduling, greedy algorithms help in optimizing the order of job execution. Some popular methods include:
- Shortest Job First (SJF): Prioritizes jobs with the shortest execution time, reducing average waiting time.
- Round Robin: Allocates a fixed time slice to each job, cycling through them to ensure fairness.
- Priority Scheduling: Assigns jobs based on priority levels, allowing more critical tasks to be executed first.
Page Replacement Algorithms
Greedy algorithms are also used in page replacement strategies to manage memory efficiently. Key algorithms include:
- Least Recently Used (LRU): Replaces the page that has not been used for the longest time.
- Optimal Page Replacement: Replaces the page that will not be used for the longest period in the future.
- First-In-First-Out (FIFO): Replaces the oldest page in memory.
Greedy algorithms are a class of algorithms that make locally optimal choices at each step with the hope of finding a global optimum solution. They are essential in operating systems for efficient resource management and scheduling.
Comparing Greedy Algorithms with Other Techniques
Dynamic Programming vs. Greedy Algorithms
Dynamic programming and greedy algorithms are both used for optimization problems, but they differ significantly in their approach:
- Dynamic Programming:
- Greedy Algorithms:
Divide and Conquer vs. Greedy Algorithms
Divide and conquer is another strategy that contrasts with greedy algorithms:
- Divide and Conquer:
- Greedy Algorithms:
Hybrid Approaches
Combining different techniques can sometimes yield better results:
- Hybrid Approaches:
- Use greedy methods for initial solutions and refine them with dynamic programming.
- Can balance speed and accuracy.
- Examples include algorithms that start with a greedy choice and then optimize further.
Greedy algorithms are effective for many problems, but understanding their limitations is crucial for choosing the right approach. They work well for problems where a local optimum leads to a global optimum.
Advanced Applications of Greedy Algorithms
Approximate Solutions for NP-Complete Problems
Greedy algorithms can be used to find approximate solutions for complex problems that are hard to solve exactly. Some examples include:
- Set Cover Problem: Selecting the smallest number of sets to cover all elements.
- Bin Packing Problem: Minimizing the number of bins used to pack items of different sizes.
- Graph Coloring: Assigning colors to vertices so that no two adjacent vertices share the same color.
Special Cases in Dynamic Programming
In some scenarios, greedy algorithms can provide solutions that are also applicable in dynamic programming. For instance:
- Fractional Knapsack Problem: Allows breaking items into smaller parts to maximize value.
- Minimum Number of Coins: Finding the least number of coins needed to make a specific amount.
- Job Sequencing with Deadlines: Scheduling jobs to maximize profit while meeting deadlines.
Complex Real-World Scenarios
Greedy algorithms are often used in real-world applications where quick decisions are necessary. Examples include:
- Network Routing: Finding the shortest path in communication networks.
- Resource Allocation: Distributing limited resources efficiently.
- Data Compression: Using Huffman coding to reduce file sizes.
Greedy algorithms are ideal for optimization problems. They are especially useful when the problem has the following properties: greedy choice property; optimal substructure.
Problem Type | Greedy Algorithm Used | Resulting Benefit |
---|---|---|
NP-Complete | Set Cover Problem | Approximate solution |
Dynamic Programming | Fractional Knapsack | Efficient resource use |
Real-World Application | Network Routing | Faster data transmission |
Evaluating the Efficiency of Greedy Algorithms
Time Complexity Analysis
When assessing the efficiency of greedy algorithms, one of the first aspects to consider is their time complexity. Greedy algorithms often have a straightforward structure, which can lead to faster execution times compared to other methods. Here are some common time complexities:
- O(n log n): Often seen in sorting-based greedy algorithms.
- O(n): For simpler greedy algorithms that make a single pass through the data.
- O(1): In cases where the solution can be derived directly without iteration.
Space Complexity Considerations
Space complexity is another important factor. Greedy algorithms typically require less memory than other approaches, such as dynamic programming. Here are some points to note:
- Constant Space: Many greedy algorithms only need a few variables to keep track of the current state.
- Linear Space: Some may require additional space proportional to the input size, especially if they store intermediate results.
- Minimal Overhead: Greedy algorithms usually have low overhead, making them efficient in terms of memory usage.
Performance Metrics
To evaluate the performance of greedy algorithms, consider the following metrics:
- Execution Time: How long the algorithm takes to run.
- Memory Usage: The amount of memory consumed during execution.
- Optimality: Whether the algorithm produces the best possible solution.
Greedy algorithms are often more efficient than dynamic programming because they generally involve a simple decision-making process at each step.
In summary, while greedy algorithms can be very efficient, their effectiveness depends on the specific problem structure. Understanding their time and space complexities, along with performance metrics, is crucial for determining their suitability for a given task.
Future Trends in Greedy Algorithms
Innovations in Algorithm Design
The field of greedy algorithms is constantly evolving. New techniques are being developed to enhance their efficiency and applicability. Some notable trends include:
- Hybrid approaches that combine greedy methods with other algorithms.
- Adaptive algorithms that adjust their strategies based on problem characteristics.
- Parallel processing to speed up greedy solutions in large datasets.
Emerging Applications
Greedy algorithms are finding new uses in various fields. Here are some areas where they are making an impact:
- Data compression techniques, such as Huffman coding, are being refined.
- Network optimization for improving data flow and resource allocation.
- Machine learning applications, where greedy methods help in feature selection.
Integration with Machine Learning
The intersection of greedy algorithms and machine learning is a promising area. As algorithms evolve, they are increasingly being used to:
- Optimize training processes by selecting the most relevant data.
- Enhance model performance through efficient feature selection.
- Address real-time decision-making challenges in dynamic environments.
Greedy algorithms are not just about making quick decisions; they are evolving to become more sophisticated and adaptable to complex problems.
In summary, the future of greedy algorithms looks bright, with ongoing innovations and applications that promise to unlock even greater efficiencies in problem-solving.
As we look ahead, greedy algorithms are set to play a crucial role in solving complex problems efficiently. These algorithms make quick, local choices that lead to a global solution, making them ideal for various applications. If you’re eager to dive deeper into coding and enhance your skills, visit our website today!
Conclusion: Enhancing Problem Solving with Greedy Algorithms
In summary, greedy algorithms play a crucial role in making problem-solving easier and faster. By focusing on the best immediate choice, these algorithms can quickly lead to good solutions for many challenges. However, it’s important to remember that they might not always find the perfect answer. Understanding when to use greedy methods can help you tackle various tasks more effectively. As you continue to learn about algorithms, consider how these strategies can improve your coding skills and problem-solving abilities.
Frequently Asked Questions
What is a greedy algorithm?
A greedy algorithm is a method used to solve problems by making the best choice at each step. It focuses on what seems best at the moment without looking ahead.
When should I use a greedy algorithm?
You should use a greedy algorithm when the problem can be solved by making a series of choices that seem best at the time, leading to a good overall solution.
What are some examples of greedy algorithms?
Some common examples include the Activity Selection Problem, Job Sequencing Problem, and Huffman Coding.
What are the advantages of greedy algorithms?
Greedy algorithms are usually simple to understand and implement. They can be very efficient for certain types of problems.
What are the limitations of greedy algorithms?
Greedy algorithms might not always give the best solution. They can miss better options because they only look at immediate gains.
How do greedy algorithms differ from dynamic programming?
Greedy algorithms make local choices at each step, while dynamic programming considers all possible solutions to find the best one.
Can greedy algorithms be used for all problems?
No, greedy algorithms are not suitable for all problems. They work best for optimization problems where local choices lead to a global solution.
How can I learn more about greedy algorithms?
You can explore online tutorials, coding platforms like AlgoCademy, and practice problems to improve your understanding of greedy algorithms.