Are you ready to dive into the world of Python programming? Whether you’re a beginner or looking to brush up on your skills, this guide will help you unlock the power of Python. Python is one of the most popular and versatile programming languages today, used in web development, data science, machine learning, and more. Let’s get started on this exciting journey!
Key Takeaways
- Python is a versatile language used in various fields like web development, data science, and machine learning.
- Setting up your Python environment is the first step to start coding in Python.
- Understanding Python syntax and writing your first program are fundamental skills.
- Mastering Python basics like variables, data types, and control structures is essential.
- Exploring advanced topics and popular libraries can take your Python skills to the next level.
Getting Started with Python
Setting Up Your Python Environment
Before you can start coding in Python, you need to set up your development environment. Getting started with Python is easy due to its straightforward syntax and extensive documentation. Follow these steps to get your environment ready:
- Download and install Python: Visit the official Python website and download the latest version of Python. Follow the installation instructions for your operating system.
- Install an Integrated Development Environment (IDE): An IDE helps you write and test your code. Popular choices include PyCharm, VS Code, and Jupyter Notebook.
- Verify the installation: Open your terminal or command prompt and type
python --version
to ensure Python is installed correctly.
Setting up your Python environment is the first step towards becoming a proficient Python programmer.
Understanding Python Syntax
Python’s syntax is designed to be readable and straightforward. Here are some key points to understand:
- Indentation: Python uses indentation to define code blocks. This makes the code clean and easy to read.
- Variables: You don’t need to declare the type of a variable. Just assign a value to it, and Python will handle the rest.
- Comments: Use
#
to add comments in your code. Comments are ignored by the interpreter and are useful for explaining your code.
Writing Your First Python Program
Let’s write a simple Python program to print "Hello, World!". Follow these steps:
- Open your IDE and create a new file named
hello.py
. - Type the following code:
print("Hello, World!")
- Save the file and run it. You should see the output
Hello, World!
on your screen.
Congratulations! You’ve written your first Python program. This is just the beginning of your journey into Python programming.
Mastering Python Basics
Variables and Data Types
In Python, variables are used to store data. You can think of them as containers for holding information. Python supports various data types like integers, floats, strings, and booleans. Here’s a quick look at some common data types:
Data Type | Description |
---|---|
Integer | Whole numbers, e.g., 1, 2, 3 |
Float | Decimal numbers, e.g., 1.5, 2.75 |
String | Text, e.g., "Hello, World!" |
Boolean | True or False values |
Operators and Expressions
Operators are symbols that perform operations on variables and values. Python has several types of operators, including arithmetic, comparison, and logical operators. For example:
- Arithmetic Operators: +, -, *, /
- Comparison Operators: ==, !=, >, <
- Logical Operators: and, or, not
Expressions combine variables and operators to produce a new value. For instance, 3 + 4
is an expression that evaluates to 7.
Control Structures: Conditionals and Loops
Control structures help you manage the flow of your program. Conditionals allow you to execute code based on certain conditions, while loops let you repeat code multiple times.
- Conditionals: Use
if
,elif
, andelse
to make decisions in your code. - Loops: Use
for
andwhile
loops to repeat actions.
# Example of a conditional
if x > 10:
print("x is greater than 10")
else:
print("x is 10 or less")
# Example of a loop
for i in range(5):
print(i)
Mastering these basics will set a strong foundation for your Python journey. Once you get comfortable with variables, operators, and control structures, you’ll be well on your way to becoming proficient in Python.
Working with Data Structures
Lists and Tuples
In Python, lists and tuples are fundamental data structures that allow you to store collections of items. Lists are mutable, meaning you can change their content, while tuples are immutable and cannot be altered once created. Here’s a quick comparison:
Feature | Lists | Tuples |
---|---|---|
Mutability | Mutable | Immutable |
Syntax | [1, 2, 3] |
(1, 2, 3) |
Use Cases | Dynamic data | Fixed data |
Dictionaries and Sets
Dictionaries and sets are also crucial in Python. Dictionaries store data in key-value pairs, making data retrieval efficient. Sets, on the other hand, are collections of unique items. They are useful for membership testing and eliminating duplicates.
- Dictionaries: Use curly braces
{}
and store data as key-value pairs. - Sets: Use curly braces
{}
but only store unique items.
Common Data Structure Operations
Python provides a variety of operations to manipulate data structures. Here are some common ones:
- Adding Items: Use
append()
for lists,add()
for sets, and key assignment for dictionaries. - Removing Items: Use
remove()
for lists and sets, andpop()
for dictionaries. - Accessing Items: Use indexing for lists and tuples, and keys for dictionaries.
Understanding these basic data structures is essential for any Python developer. They form the backbone of more complex data manipulation and algorithms.
In this section, we will discuss all the in-built data structures like lists, tuples, dictionaries, etc., as well as some advanced data structures like trees, graphs, etc.
Functions and Modules
Defining and Calling Functions
Functions are blocks of code that perform a specific task. You define a function using the def
keyword, followed by the function name and parentheses. Inside the parentheses, you can include parameters. Here’s a simple example:
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
Function Arguments and Return Values
Functions can take arguments and return values. Arguments are the inputs you pass to the function, and the return value is the output. You can have multiple arguments and return values. For example:
def add(a, b):
return a + b
result = add(3, 5)
print(result) # Output: 8
Creating and Using Modules
A Python module is a file that contains functions, classes, and variables. You can create your own modules and use them in other programs. To use a module, you import it using the import
statement. Here’s how you can create and use a module:
- Create a file named
mymodule.py
with the following content:
def say_hello(name):
return f"Hello, {name}!"
- In another file, import and use the module:
import mymodule
print(mymodule.say_hello("Bob"))
Modules help you organize your code and make it reusable. There are many Python modules, each with its specific work.
Object-Oriented Programming in Python
Classes and Objects
Object-Oriented Programming (OOP) is a way to design your programs using objects. An object is a self-contained piece of code that has properties and methods. Think of a class as a blueprint for creating objects. For example, you can have a class Animal
and create objects like Dog
and Cat
from it.
Attributes and Methods
Attributes are the data stored inside an object, and methods are the functions that belong to an object. For instance, a Dog
object might have an attribute name
and a method speak
that makes the dog bark.
Inheritance and Polymorphism
Inheritance allows one class to inherit the attributes and methods of another class. This helps in reusing code. Polymorphism lets you use a single interface to represent different data types. For example, both Dog
and Cat
can have a speak
method, but they will produce different sounds.
Object-oriented programming is a fundamental concept in Python, empowering developers to build modular, maintainable, and scalable applications.
Encapsulation
Encapsulation is about bundling the data and the methods that operate on the data into a single unit called a class. This helps in keeping the data safe from outside interference and misuse.
Error Handling and Exceptions
Common Python Errors
When you start coding in Python, you’ll encounter various errors. These errors can be syntax errors, runtime errors, or logical errors. Understanding these errors is crucial for debugging your code effectively.
Using try and except
Python provides a way to handle errors using try
and except
blocks. This allows your program to continue running even when an error occurs. Here’s a simple example:
try:
# Code that might cause an error
result = 10 / 0
except ZeroDivisionError:
# Code to run if there is a ZeroDivisionError
print("You can't divide by zero!")
Creating Custom Exceptions
Sometimes, you might need to create your own exceptions to handle specific situations in your code. You can do this by defining a new class that inherits from Python’s built-in Exception
class.
class MyCustomError(Exception):
pass
try:
raise MyCustomError("This is a custom error message")
except MyCustomError as e:
print(e)
Errors detected during execution are called exceptions and are not unconditionally fatal: you will soon learn how to handle them in Python programs.
Writing Clean and Efficient Code
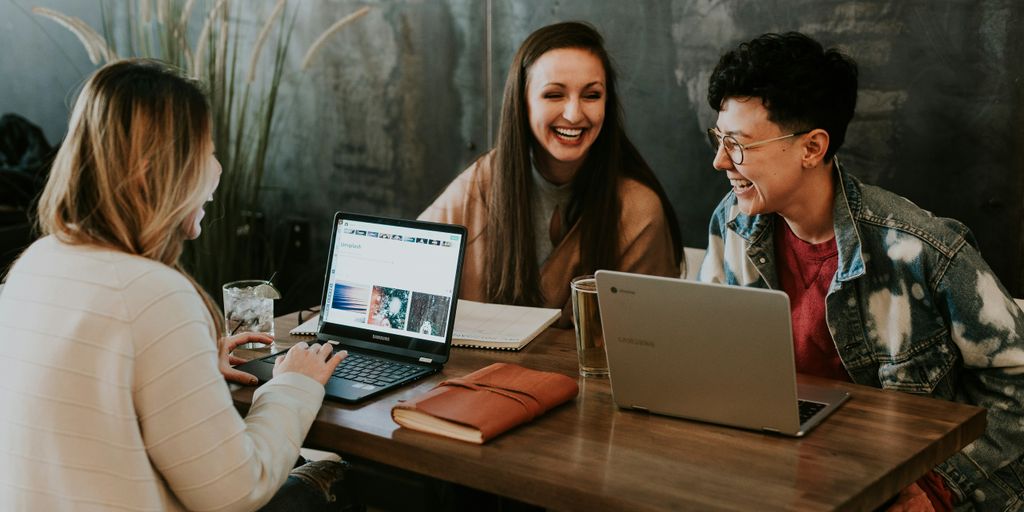
PEP 8 – Style Guide for Python Code
PEP 8 is the official style guide for Python code. It helps you write code that is more readable and consistent. Following PEP 8 makes it easier for others to understand your code. Here are some key points:
- Use 4 spaces per indentation level.
- Limit lines to 79 characters.
- Use blank lines to separate functions and classes.
- Use spaces around operators and after commas.
Code Commenting and Documentation
Good comments and documentation are essential for maintaining code. They help others understand what your code does and why. Here are some tips:
- Write comments to explain why, not what.
- Use docstrings to describe the purpose of functions and classes.
- Keep comments up-to-date with code changes.
Clear comments and documentation can save time and reduce errors when you or others revisit the code later.
Naming Conventions
Using proper naming conventions makes your code more readable. Here are some guidelines:
- Use descriptive names for variables, functions, and classes.
- Follow the naming conventions:
snake_case
for variables and functions,CamelCase
for classes. - Avoid using single-character names except for counters or iterators.
By following these practices, you can write clean and efficient Python code that is easy to read and maintain.
Advanced Python Topics
Decorators and Generators
Decorators are a powerful feature in Python that allows you to modify the behavior of a function or class. They are often used for logging, access control, and instrumentation. Generators, on the other hand, are a special type of iterator that allows you to iterate over a sequence of values. They are memory efficient and can be used to handle large datasets.
Context Managers
Context managers are used to manage resources like file streams or network connections. They allow you to allocate and release resources precisely when you want to. The most common way to implement a context manager is by using the with
statement.
Metaprogramming
Metaprogramming is a technique where you write code that manipulates other code. This can be done using features like decorators, metaclasses, and the exec
function. Metaprogramming allows you to write more flexible and reusable code.
Advanced Python topics like decorators, context managers, and metaprogramming can significantly enhance your coding skills and make your programs more efficient and readable.
Exploring Popular Python Libraries
Python’s extensive library ecosystem makes it a powerful tool for various domains. Let’s dive into some of the most popular libraries that you should know.
NumPy and Pandas for Data Science
In the realm of data science, NumPy and Pandas are indispensable. NumPy provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. Pandas, on the other hand, offers data structures and operations for manipulating numerical tables and time series. These libraries are essential for data manipulation, analysis, and visualization.
Django and Flask for Web Development
Python’s web development frameworks, such as Django and Flask, have gained immense popularity among developers. Django is a high-level framework that encourages rapid development and clean, pragmatic design. Flask is a micro-framework that is easy to learn and simple to use, making it perfect for small to medium-sized applications. Both frameworks provide a solid foundation for building robust and scalable web applications.
TensorFlow and PyTorch for Machine Learning
In the field of machine learning and artificial intelligence, TensorFlow and PyTorch are the go-to libraries. TensorFlow, developed by Google, is an open-source library for numerical computation and large-scale machine learning. PyTorch, developed by Facebook, is known for its flexibility and ease of use, especially in research and development. These libraries have revolutionized the field, enabling developers to create sophisticated neural networks and deep learning models.
Exploring these libraries can open up a world of possibilities in data science, web development, and machine learning. Whether you’re analyzing data, building a website, or creating intelligent systems, Python’s libraries have you covered.
Here’s a quick overview of the popular Python libraries in different domains:
Domain | Popular Libraries/Frameworks |
---|---|
Web Development | Django, Flask |
Data Science | NumPy, Pandas, Matplotlib |
Machine Learning | TensorFlow, PyTorch, Scikit-learn |
Data Visualization | Seaborn, Plotly, Bokeh |
Natural Language Processing | NLTK, spaCy, Gensim |
Building Real-World Projects
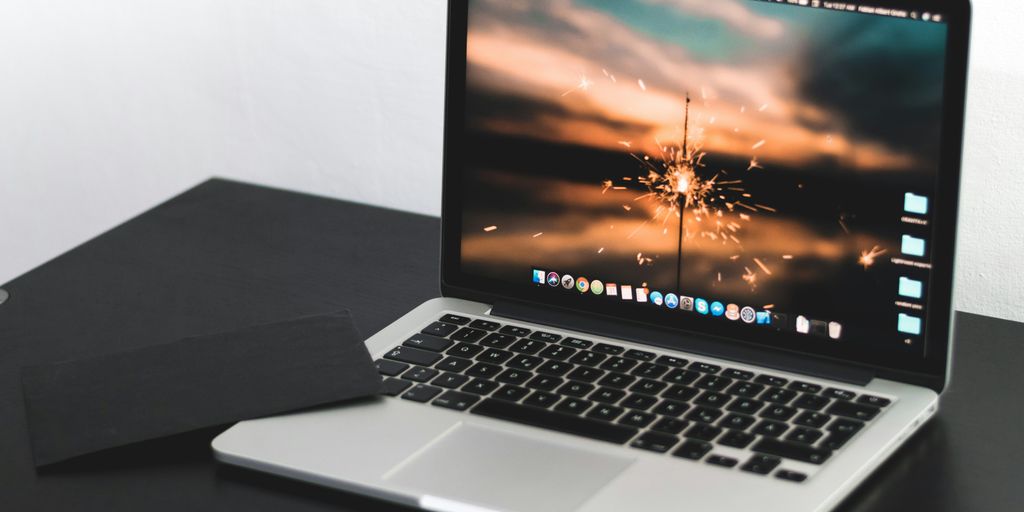
Planning Your Project
Before you start coding, it’s crucial to plan your project. Building Python projects is the ultimate learning tool. Here are some steps to help you get started:
- Define the project goals.
- Identify the main features.
- Sketch a basic design or flowchart.
- Break down the project into smaller tasks.
- Set a timeline for each task.
Implementing Your Project
Once you have a plan, it’s time to start coding. Follow these tips to make the process smoother:
- Write clean and readable code.
- Test each feature as you develop it.
- Use version control systems like Git.
- Keep your code modular and reusable.
Testing and Debugging
Testing and debugging are essential parts of any project. They help ensure your code works as expected. Here are some best practices:
- Write unit tests for individual functions.
- Perform integration tests to check how different parts of the project work together.
- Use debugging tools to find and fix errors.
- Regularly review and refactor your code.
Building real-world projects not only reinforces the concepts you’ve learned but also provides valuable experience in problem-solving and coding best practices.
Deploying and Distributing Python Applications
Packaging Your Python Code
To share your Python projects with others, you need to package your code. Packaging involves bundling your code and its dependencies so that others can easily install and use it. The most common way to package Python code is by creating a setup.py
file or a [pyproject.toml](https://packaging.python.org/en/latest/guides/section-build-and-publish/)
file. These files contain metadata about your project, such as its name, version, and dependencies.
Using Virtual Environments
Virtual environments are essential for managing dependencies in Python projects. They allow you to create isolated environments for different projects, ensuring that dependencies do not conflict. To create a virtual environment, you can use the venv
module:
python -m venv myenv
Activate the virtual environment with:
- Windows:
myenv\Scripts\activate
- macOS/Linux:
source myenv/bin/activate
Distributing Your Application
Once your code is packaged and dependencies are managed, you can distribute your application. There are several ways to do this:
- PyPI (Python Package Index): Upload your package to PyPI so others can install it using
pip
. - Executable Files: Use tools like PyInstaller to convert your Python scripts into standalone executables.
- Docker: Containerize your application using Docker for consistent deployment across different environments.
Properly packaging and distributing your Python code ensures that others can easily use and contribute to your projects.
By following these steps, you can make your Python applications accessible and easy to use for others.
Deploying and distributing Python applications can be a breeze with the right guidance. Whether you’re a beginner or have some experience, our step-by-step tutorials will help you get your project up and running smoothly. Don’t miss out on the chance to enhance your skills and make your coding journey easier. Visit our website to start learning today!
Conclusion
In conclusion, Python is a powerful and versatile programming language that opens up a world of possibilities. Whether you’re just starting out or looking to enhance your skills, mastering Python can be a game-changer. This tutorial has covered the basics and advanced topics, giving you a solid foundation to build upon. Remember, practice is key to becoming proficient. So, keep experimenting, keep coding, and most importantly, have fun with it. The journey of learning Python is filled with endless opportunities, and with dedication, you can unlock its full potential. Happy coding!
Frequently Asked Questions
What is Python?
Python is a popular programming language known for its simplicity and readability, making it great for beginners.
How do I install Python?
You can download Python from the official website, python.org, and follow the installation instructions for your operating system.
What can I do with Python?
Python can be used for web development, data analysis, machine learning, artificial intelligence, and more.
Is Python good for beginners?
Yes, Python’s simple syntax and readability make it an excellent choice for people who are new to programming.
What is a Python library?
A Python library is a collection of modules that provide pre-written code to help you perform common tasks.
How can I learn Python?
You can learn Python through online tutorials, coding bootcamps, and books. Practice by working on small projects.
What is an IDE, and do I need one for Python?
An IDE (Integrated Development Environment) is a software application that provides tools for coding. While not necessary, it can make coding easier.
Can Python be used for web development?
Yes, Python has frameworks like Django and Flask that are widely used for building web applications.