Understanding Time Complexity/ A Crucial Skill for Coding Interviews
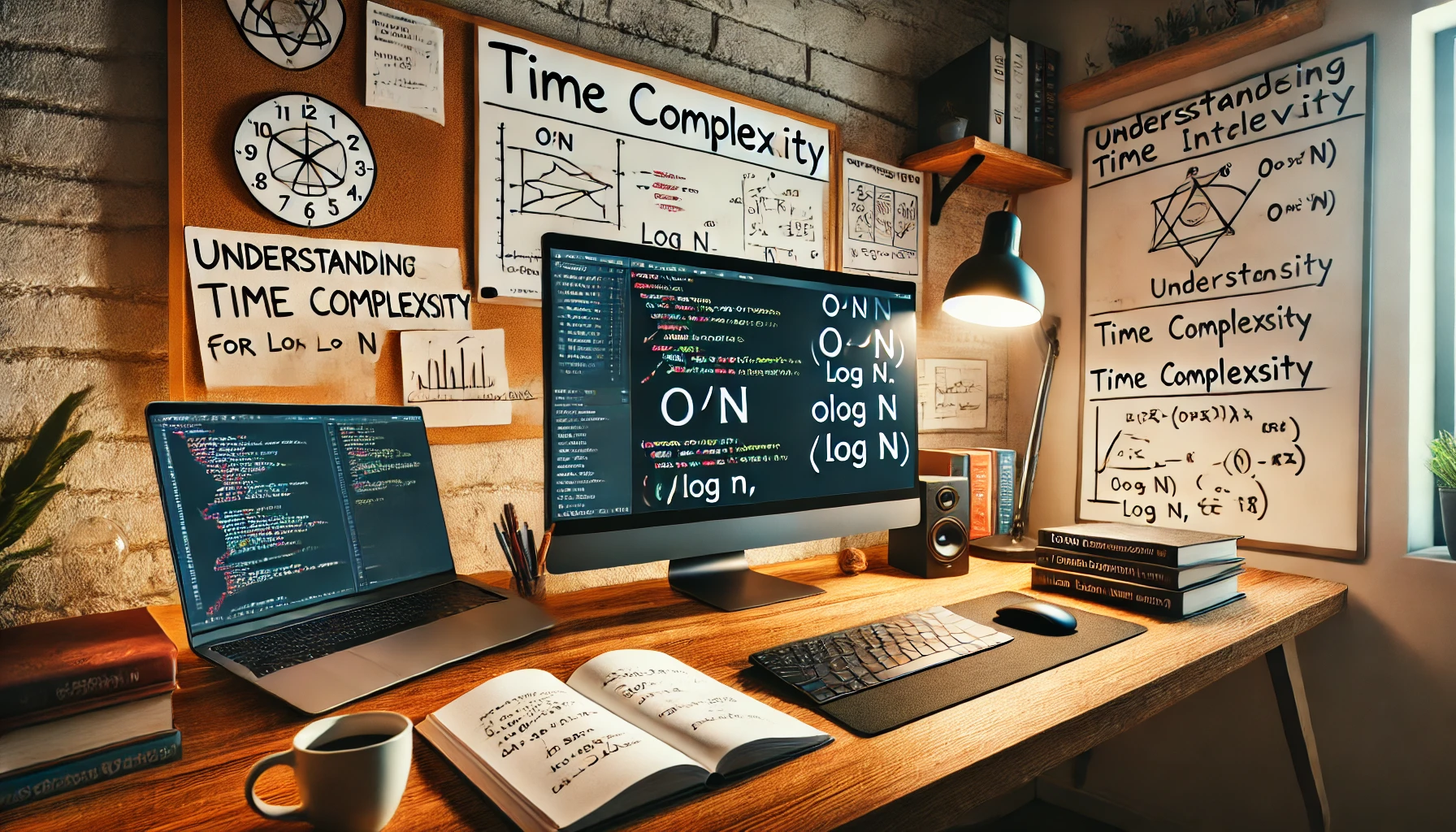
Time complexity is a key concept in coding interviews. It helps gauge how well a candidate can analyze and optimize algorithms, which is crucial for creating efficient software. Understanding time complexity can make a big difference in software performance and scalability, making it an essential skill for programmers.
Key Takeaways
- Time complexity measures how the runtime of an algorithm changes with input size.
- Companies evaluate time complexity to find skilled programmers who can optimize code.
- Big O Notation is a common way to express time complexity.
- Different algorithms have varying time complexities, affecting their efficiency.
- Preparing for time complexity questions is crucial for coding interviews.
The Significance of Time Complexity in Coding Interviews
Why Companies Evaluate Time Complexity
Understanding time complexity is crucial for companies during coding interviews. It helps them identify candidates who can write efficient code. Efficient algorithms can significantly impact software performance and scalability. By evaluating a candidate’s ability to analyze algorithm performance, companies can ensure they hire skilled programmers who can optimize resources and reduce execution time.
Impact on Software Performance and Scalability
Efficient algorithms are essential for good software performance and scalability. When a programmer understands time complexity, they can design algorithms that handle large datasets and complex computations effectively. This knowledge helps in identifying potential bottlenecks and areas for optimization within existing codebases, leading to enhanced system responsiveness.
Identifying Skilled Programmers
Proficiency in time complexity allows programmers to make informed decisions when selecting algorithms for solving real-world problems. By assessing this skill, companies can ensure that their hires have the capacity to handle large datasets and complex computations effectively. This is why understanding time complexity is a crucial skill for coding interviews.
Fundamentals of Time Complexity
Defining Time Complexity
Time complexity is a measure of how long an algorithm takes to run as the input size increases. Rather than focusing on the actual runtime, it looks at how the runtime grows with the input size. Understanding time complexity helps programmers predict how their code will perform as data scales up.
Importance in Algorithm Analysis
Time complexity is crucial in algorithm analysis because it allows developers to compare the efficiency of different algorithms. By evaluating time complexity, programmers can choose the most efficient algorithm for a given problem, saving both time and computational resources.
When you learn time complexity, you can quickly weed out bad ideas and avoid wasting time on suboptimal approaches.
Common Misconceptions
There are several misconceptions about time complexity. One common mistake is thinking that it measures the actual runtime of an algorithm. Instead, it measures how the runtime grows with the input size. Another misconception is ignoring lower-order terms and constants, which can sometimes be significant in practical scenarios.
Big O Notation Explained
Understanding Big O Notation
Big O Notation is a way to describe how an algorithm’s runtime or space requirements grow as the input size increases. It helps us understand the upper-bound performance of an algorithm. For instance, if an algorithm has a time complexity of O(N^2), it means that the runtime increases proportionally to the square of the input size.
Different Types of Big O Notations
There are several common Big O notations used to describe algorithm performance:
- O(1): Constant time complexity, where the runtime does not change with the input size.
- O(N): Linear time complexity, where the runtime grows directly with the input size.
- O(N^2): Quadratic time complexity, where the runtime grows with the square of the input size.
- O(log N): Logarithmic time complexity, where the runtime grows logarithmically as the input size increases.
- O(N log N): Linearithmic time complexity, a combination of linear and logarithmic growth.
Real-World Examples
Let’s look at some real-world examples to understand these notations better:
- O(1): Accessing an element in an array by index.
- O(N): Looping through an array to find a specific element.
- O(N^2): Nested loops, such as checking all pairs of elements in an array.
- O(log N): Binary search in a sorted array.
- O(N log N): Efficient sorting algorithms like Merge Sort and Quick Sort.
Big O Notation in data structure tells us how well an algorithm will perform in a particular situation. It gives an algorithm’s upper-bound performance, helping us predict its efficiency.
Analyzing Algorithm Efficiency
Understanding how to analyze the efficiency of an algorithm is a key skill for any programmer. Algorithmic efficiency is about how well an algorithm uses computational resources, like time and memory. Here, we’ll break down the steps to analyze time complexity, compare different algorithms, and explore tools and techniques to help you along the way.
Steps to Analyze Time Complexity
- Identify the basic operations: Determine the fundamental operations that significantly affect the algorithm’s runtime.
- Count the operations: Calculate how many times these basic operations are executed as the input size grows.
- Express in Big O notation: Use Big O notation to describe the upper limit of the algorithm’s growth rate.
- Simplify the expression: Remove constant factors and lower-order terms to simplify the Big O expression.
Comparing Different Algorithms
When comparing algorithms, consider the following:
- Time complexity: How does the runtime increase with the input size?
- Space complexity: How much memory does the algorithm use?
- Scalability: Can the algorithm handle larger datasets efficiently?
Tools and Techniques
Several tools and techniques can help you analyze and improve algorithm efficiency:
- Profilers: Tools like gprof or Valgrind can help you measure the time complexity of your code.
- Benchmarking: Running your algorithm with different input sizes to observe performance changes.
- Visualization: Graphing the runtime against input size can help you understand the algorithm’s behavior.
Efficient algorithms are crucial for building scalable and high-performance software. By mastering these analysis techniques, you can ensure your code runs optimally, even as data sizes grow.
Common Time Complexity Classes
Understanding different time complexity classes is essential for evaluating the efficiency of algorithms. Here, we will discuss some of the most common time complexity classes.
Constant Time Complexity
Constant time complexity, denoted as O(1), means that the algorithm’s runtime does not change regardless of the input size. For example, accessing an element in an array by its index is an O(1) operation.
Linear Time Complexity
Linear time complexity, represented as O(n), indicates that the runtime of the algorithm increases linearly with the input size. A common example is a for loop that iterates through all elements of an array.
Quadratic and Higher-Order Complexities
Quadratic time complexity, denoted as O(n^2), means that the runtime of the algorithm is proportional to the square of the input size. This is often seen in algorithms with nested loops, such as bubble sort. Higher-order complexities, like O(n^3) or O(2^n), grow even faster and are generally less efficient for large inputs.
Understanding these complexity classes helps in identifying the set of problems that are hard for NP and choosing the most efficient algorithm for a given problem.
Practical Applications of Time Complexity
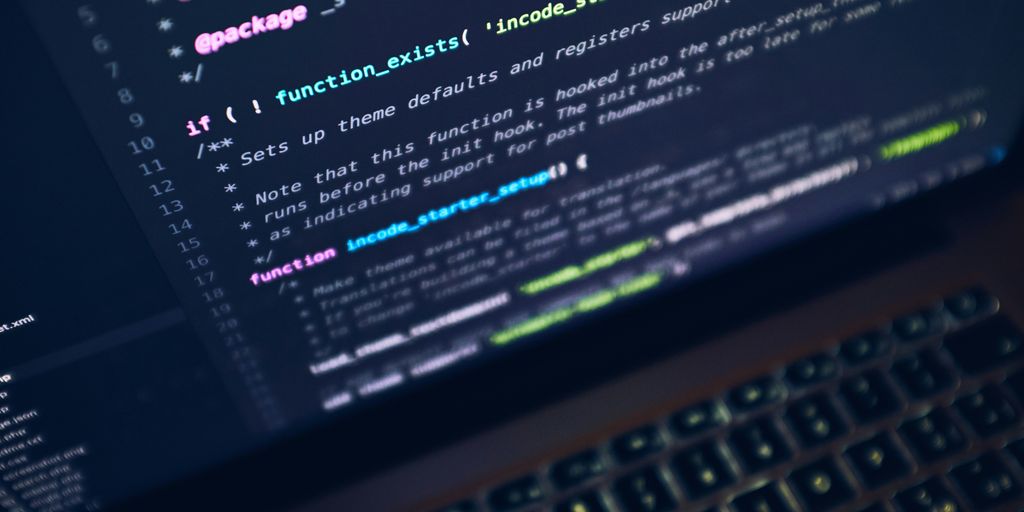
Algorithm Selection and Optimization
Time complexity analysis helps programmers evaluate different algorithms and select the most efficient one for a given problem. By choosing algorithms with lower time complexity, developers can improve performance and reduce computational costs.
Performance Analysis
Time complexity allows for performance analysis of existing code. By analyzing the time complexity of specific algorithms or code snippets, developers can pinpoint potential bottlenecks and optimize critical sections for better overall performance.
Scalability Assessment
Time complexity enables programmers to assess how algorithms or software systems will handle increased input sizes. By understanding the relationship between input size and execution time, developers can predict scalability and ensure that solutions remain efficient as the data volume grows.
Time complexity is a fundamental concept in programming that has practical applications across various domains.
Codebase Optimization
Time complexity analysis helps identify opportunities for codebase optimization. By applying algorithmic techniques to reduce time complexity, such as implementing caching or more efficient data structures, programmers can enhance the overall efficiency of their code.
System Design Considerations
Time complexity plays a crucial role in system design. Architects need to consider the time complexity of individual components and their interactions to ensure that the system as a whole meets performance requirements.
Time Complexity in Popular Algorithms
Understanding the time complexity of popular algorithms is essential for any programmer. It helps in predicting how an algorithm will perform as the size of the input data grows. This section will cover the time complexity of sorting, search, and graph algorithms.
Best Practices for Time Complexity Analysis
Optimizing Code for Efficiency
To write efficient code, always aim to minimize the time complexity. Focus on reducing the number of operations your algorithm performs. This can often be achieved by choosing the right data structures and algorithms. For example, using a hash table can reduce the time complexity of search operations from O(n) to O(1).
Avoiding Common Pitfalls
Be aware of common mistakes that can lead to inefficient code. One such mistake is ignoring the impact of input size on your algorithm’s performance. Always consider the worst-case scenario, as it provides a clear picture of your algorithm’s efficiency. For instance, in a search algorithm, the worst case might occur when the element is at the last index, leading to a time complexity of O(n).
Continuous Learning and Improvement
Time complexity analysis is not a one-time task. Continuously learn and improve your skills by practicing different types of problems. Stay updated with the latest algorithmic techniques and best practices. This will help you identify more efficient solutions and keep your skills sharp.
Regular practice and staying updated with new techniques are crucial for mastering time complexity analysis.
Preparing for Time Complexity Questions in Interviews
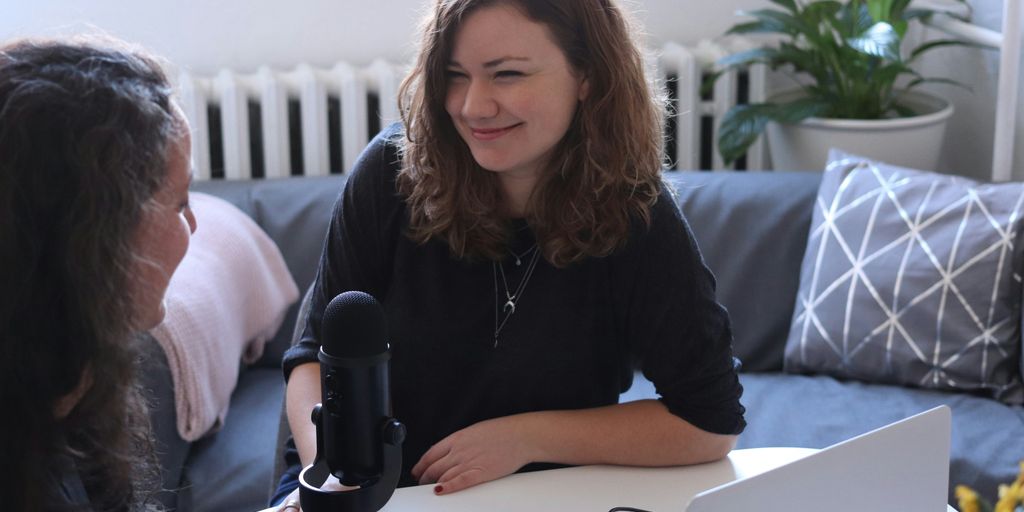
Common Interview Questions
When preparing for coding interviews, it’s crucial to understand the types of questions you might face. Commonly asked questions often revolve around analyzing the time complexity of given algorithms or optimizing existing code. You might be asked to calculate the Big O notation for a specific algorithm or compare the efficiencies of different approaches.
Strategies for Effective Preparation
- Understand the Basics: Make sure you have a solid grasp of fundamental concepts like Big O notation, time complexity vs. space complexity, and common algorithmic patterns.
- Practice Regularly: Use platforms like LeetCode or HackerRank to practice a variety of problems. This will help you get comfortable with different types of questions.
- Mock Interviews: Participate in mock interviews to simulate the real experience. This can help you manage time and stress effectively.
- Review and Reflect: After each practice session or mock interview, review your performance. Identify areas for improvement and focus on them.
Resources for Practice
To effectively prepare, utilize a variety of resources:
- Online Platforms: Websites like LeetCode, HackerRank, and CodeSignal offer a plethora of problems to practice.
- Books: Titles like “Cracking the Coding Interview” provide in-depth insights and practice questions.
- Articles and Tutorials: Read articles such as “Top 30 Big-O Notation Interview Questions & Answers 2023” to get a sense of what to expect.
Even with all the prep work, the bar is set mad high. Studies show only about a quarter of candidates make it past the interviews, proving just how intense these assessments can be.
By following these strategies and utilizing these resources, you’ll be better equipped to tackle time complexity questions in your coding interviews.
Roles Requiring Strong Time Complexity Skills
Data Scientists
Data scientists use time complexity skills to make machine learning algorithms and data processing workflows run faster. They need to handle large datasets and complex computations efficiently.
Software Engineers
Software engineers with strong time complexity skills can create highly optimized algorithms and code. This leads to better performance and responsiveness of software applications.
AI and Machine Learning Engineers
AI and machine learning engineers use time complexity skills to design and implement complex AI models. They ensure these models can process large datasets efficiently.
Understanding time complexity is crucial for anyone looking to write efficient and scalable code. It helps in making informed decisions and optimizing systems for real-world scenarios.
Data Engineers
Data engineers use time complexity skills to design efficient data pipelines and optimize data processing operations. This ensures smooth and fast data flow.
DevOps Engineers
DevOps engineers leverage time complexity skills to optimize software systems. They ensure efficient deployment and scalability, making sure everything runs smoothly.
Data Warehouse Engineers
Data warehouse engineers design and implement efficient data storage and retrieval systems. They use time complexity skills to ensure optimal data access and analysis.
Advanced Topics in Time Complexity
Amortized Analysis
Amortized analysis is a method used to average the time complexity of an algorithm over a sequence of operations. Instead of looking at the worst-case time for a single operation, it considers the average time per operation in the worst-case scenario. This is particularly useful for algorithms where occasional operations are costly, but most are cheap. By understanding amortized analysis, programmers can make more informed decisions about algorithm efficiency.
Probabilistic Analysis
Probabilistic analysis involves evaluating the time complexity of an algorithm based on the probability of different inputs. This type of analysis is useful when the input is random or follows a certain distribution. It helps in predicting the average-case performance of an algorithm, which can be more practical than the worst-case scenario. Probabilistic analysis is often used in algorithms where the input size or pattern is not fixed.
Average-Case vs Worst-Case Analysis
Average-case analysis looks at the expected time complexity for a typical input, while worst-case analysis focuses on the maximum time complexity for any input. Both types of analysis are important for understanding the overall performance of an algorithm. Average-case analysis can provide a more realistic expectation of performance, but worst-case analysis is crucial for ensuring that an algorithm can handle the most demanding situations.
By comprehending these subtopics within time complexity, programmers can make informed decisions, optimize algorithms, and ultimately improve the overall efficiency of their code.
Understanding advanced topics in time complexity can be a game-changer for your coding skills. Dive deep into these concepts and see how they can help you solve problems more efficiently. Want to learn more? Check out our website for interactive tutorials and expert guidance.
Conclusion
Understanding time complexity is a game-changer for anyone preparing for coding interviews. It helps you figure out how well an algorithm performs as the input size grows, which is super important for writing efficient code. By mastering this skill, you can quickly spot bad ideas and focus on the best solutions, saving time and impressing interviewers. Plus, knowing time complexity helps you design systems that can handle big data and complex problems. So, keep practicing and learning about time complexity—it’s a crucial skill that will make you a better programmer and help you ace those coding interviews.
Frequently Asked Questions
What is time complexity?
Time complexity measures how long an algorithm takes to run as the input size grows. It’s a way to understand the efficiency of an algorithm.
Why is time complexity important in coding interviews?
Time complexity helps interviewers see if you can write efficient code. It shows how well you can optimize algorithms to run faster and handle larger data.
What is Big O notation?
Big O notation is a way to describe the upper limit of an algorithm’s running time. It helps in comparing the efficiency of different algorithms.
How can I improve my understanding of time complexity?
Practice analyzing different algorithms, study common time complexity classes, and solve problems on coding platforms like LeetCode or HackerRank.
What are some common time complexity classes?
Some common classes include O(1) for constant time, O(n) for linear time, and O(n^2) for quadratic time. Each describes how the runtime grows with input size.
How do I analyze the time complexity of an algorithm?
Break down the algorithm into individual steps, count the number of operations, and use Big O notation to express the overall time complexity.
What roles require strong time complexity skills?
Roles like software engineers, data scientists, and AI engineers often need strong time complexity skills to optimize code and handle large datasets.
How can I prepare for time complexity questions in interviews?
Study common algorithms, practice coding problems, and understand how to analyze and optimize time complexity. Mock interviews can also help.