Understanding Redux Meaning: A Comprehensive Guide to Its Definition and Usage
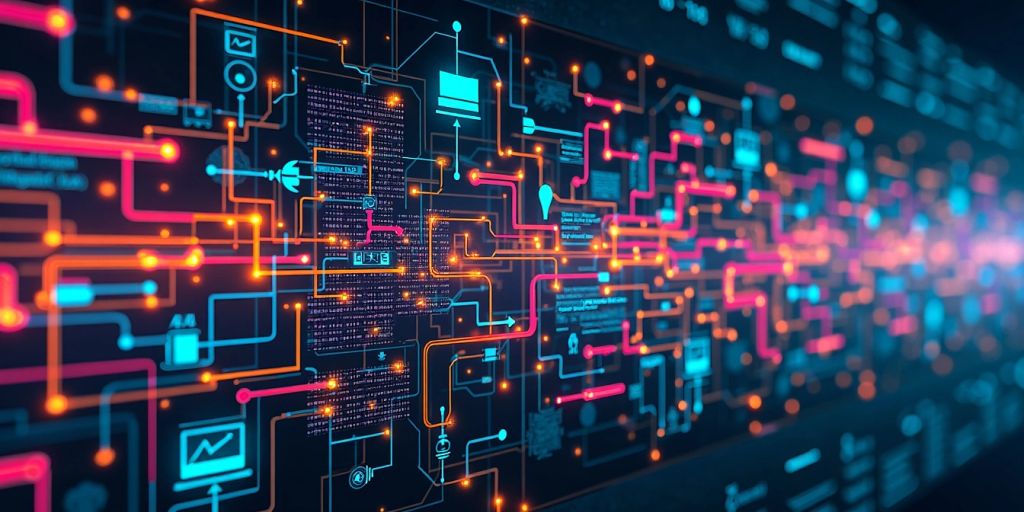
This guide aims to demystify Redux, a popular tool for managing application state. Whether you’re building a small project or a large application, understanding Redux can help you keep your data organized and predictable. In this article, we will explore what Redux is, its benefits, and how it can be implemented effectively. By the end, you’ll have a solid grasp of Redux and its core concepts, making it easier to decide if it’s the right fit for your projects.
Key Takeaways
- Redux helps manage application state in a single place, making it easier to keep track of changes.
- It separates actions (events) from reducers (how state changes), promoting clear and predictable code.
- Using Redux can simplify complex state management, especially in larger applications.
- Redux is often used with React but can work with other frameworks too.
- While Redux offers many advantages, it’s important to assess if it’s necessary for your specific project.
What is Redux?
Definition of Redux
Redux is a library and pattern used for managing and updating global application state. It allows the user interface (UI) to trigger events known as actions that describe what has happened. These actions are then processed by separate functions called reducers, which update the state accordingly. Essentially, Redux acts as a centralized store for state that needs to be accessed throughout the application, ensuring that updates happen in a predictable way.
Core Principles of Redux
- Single Source of Truth: The entire application state is stored in one place, making it easier to manage.
- State is Read-Only: The only way to change the state is by dispatching actions, ensuring that state changes are intentional.
- Changes are Made with Pure Functions: Reducers are pure functions that take the previous state and an action, returning the new state without side effects.
Common Misconceptions About Redux
- Redux is only for large applications: While it shines in larger apps, it can also be beneficial for smaller ones.
- Redux is complex: Although it has a learning curve, it simplifies state management in the long run.
- You must use Redux with React: Redux can be used with any UI framework, not just React.
Redux helps in managing shared state across components, making it easier to build complex applications without losing control over the state.
Why Use Redux?
Benefits of Using Redux
Redux is a powerful tool for managing state in applications. Here are some key benefits:
- Centralized State Management: Redux keeps the state in one place, making it easier to manage and track changes.
- Predictable State Updates: With Redux, state changes are predictable, which helps in debugging and testing.
- Easier Collaboration: When working in teams, having a clear structure for state management helps everyone understand the code better.
Challenges and Trade-offs
While Redux has many advantages, there are also some challenges:
- Learning Curve: It can be complex to learn, especially for beginners.
- Boilerplate Code: Redux often requires more code to set up compared to simpler state management solutions.
- Performance Overhead: In some cases, using Redux can slow down your application if not implemented correctly.
When Redux is Most Useful
Redux is particularly useful in the following scenarios:
- When your application has a lot of shared state.
- If the state changes frequently and needs to be accessed by many components.
- For larger applications where multiple developers are involved.
Redux is a great choice when managing complex state across many components. It helps keep your application organized and predictable.
In summary, understanding the fundamental basics of Redux can greatly enhance your ability to manage state in your applications effectively. It’s essential to weigh the benefits against the challenges to determine if Redux is the right fit for your project.
Key Concepts in Redux
Actions in Redux
Actions are plain objects that describe what happened in your application. They must have a type
property, which is a string that indicates the action’s purpose. Actions are essential for Redux because they help manage state changes. Here are some key points about actions:
- Actions must be plain objects.
- They should have a
type
property. - They can include additional data as needed.
Reducers in Redux
Reducers are functions that take the current state and an action as arguments and return a new state. They are pure functions, meaning they do not modify the existing state but create a new one based on the action received. Here’s what you need to know:
- Reducers must be pure functions.
- They should not mutate the state directly.
- They combine to form the root reducer.
Redux Store
The Redux store is the central hub for managing the application’s state. It holds the entire state tree and allows access to it through methods. Here are some important features of the store:
- It holds the global state of the application.
- You can only change the state by dispatching actions.
- The store notifies subscribers when the state changes.
The Redux store is crucial for maintaining a predictable state across your application. It ensures that all parts of your app can access the same data consistently.
In summary, understanding these key concepts—actions, reducers, and the store—is vital for effectively using Redux in your applications. They form the foundation of how Redux operates and helps manage state efficiently.
Setting Up Redux
Initial Setup Steps
To get started with Redux, follow these simple steps:
- Install Redux and React-Redux: Use npm or yarn to add Redux and React-Redux to your project.
- Create a Store: Set up a Redux store to hold your application’s state.
- Connect Redux to React: Use the
Provider
component to make the store available to your React components.
Configuring the Redux Store
When configuring your Redux store, you can use the Redux Toolkit for a smoother experience. Here’s a quick example:
import { configureStore } from '@reduxjs/toolkit';
import rootReducer from './reducers';
const store = configureStore({
reducer: rootReducer,
});
Integrating Redux with React
To integrate Redux with your React application, follow these steps:
- Wrap your main application component with the
Provider
from React-Redux. - Pass the store as a prop to the
Provider
. - Use the
useSelector
anduseDispatch
hooks to interact with the Redux store in your components.
Tip: Using Redux Toolkit can greatly simplify your setup process and reduce boilerplate code. This tutorial will briefly introduce you to Redux Toolkit and teach you how to start using it correctly.
Redux Data Flow
One-Way Data Flow
In Redux, data flows in a single direction. This means that:
- The UI sends actions to the store.
- The store processes these actions and updates the state.
- The UI then re-renders based on the new state.
This one-way flow helps keep the application predictable and easier to debug.
Dispatching Actions
When a user interacts with the app, actions are dispatched. Here’s how it works:
- A user clicks a button or performs an action.
- An action object is created to describe what happened.
- This action is sent to the store using
store.dispatch(action)
.
State Updates and Re-rendering
After an action is dispatched, the following occurs:
- The store runs the reducer to calculate the new state.
- The UI components that are subscribed to the store check for changes.
- If there are changes, those components re-render to display the updated data.
In summary, Redux ensures that the UI always reflects the current state of the application, making it easier to manage and understand.
Step | Description |
---|---|
1 | User interacts with the UI |
2 | Action is dispatched to the store |
3 | Store updates the state |
4 | UI re-renders with new state |
Advanced Redux Techniques
Using Middleware
Middleware in Redux allows you to extend the store’s capabilities. It can be used for various purposes, such as logging actions, handling asynchronous requests, or even managing side effects. Here are some common middleware options:
- Redux Thunk: Enables action creators to return a function instead of an action.
- Redux Saga: Uses generator functions to handle side effects more elegantly.
- Redux Logger: Logs actions and state changes to the console for easier debugging.
Redux Toolkit
Redux Toolkit is the official, recommended way to write Redux logic. It simplifies the process of setting up a Redux store and includes useful tools like:
- createSlice: Automatically generates action creators and reducers.
- configureStore: Sets up the store with good defaults.
- createAsyncThunk: Simplifies handling asynchronous actions.
Optimizing Performance
To ensure your Redux application runs smoothly, consider these performance tips:
- Use Reselect: Create memoized selectors to prevent unnecessary re-renders.
- Batch Updates: Group multiple state updates into a single render.
- Avoid Deeply Nested State: Keep your state structure flat to simplify updates and reduce complexity.
By mastering these advanced redux patterns, you can tackle complex state management challenges effectively.
These techniques can greatly enhance your Redux experience, making your applications more efficient and easier to manage.
State Management with Redux
State management is a way to handle and share data across different parts of your application. It helps create a clear structure for your app’s data, making it easier to read and update. Using Redux simplifies this process significantly.
Global State Management
- Centralized Data: Redux keeps all your app’s important data in one place, making it easier to manage.
- Predictable State Changes: With Redux, you can always expect the same outcome when the same action is performed.
- Easier Debugging: Since the state is predictable, tracking down issues becomes simpler.
Local vs Global State
- Local State: This is managed within a single component. It’s great for small, simple applications.
- Global State: This is shared across multiple components. Redux is perfect for this, especially in larger applications.
- When to Use Each: Use local state for simple data needs and global state for complex data shared across many components.
State Persistence
- Keeping Data: Redux can help keep your data even when the user refreshes the page.
- Using Middleware: Middleware can be used to save the state to local storage or other storage solutions.
- Restoring State: When the app loads, you can restore the state from where it was saved.
Managing state can get complicated as your app grows. Using Redux helps keep everything organized and predictable.
In summary, Redux is a powerful tool for managing state in your applications, especially when dealing with complex data needs. It allows for a clear structure and makes it easier to maintain and debug your code.
Debugging and Testing Redux Applications
Using Redux DevTools
Redux DevTools is a powerful tool that helps you track changes in your application’s state. By integrating Redux DevTools and enabling source maps in Webpack, you can significantly improve your experience debugging Redux apps. This tool allows you to see a history of state changes, making it easier to identify where things went wrong.
Writing Testable Redux Code
Testing Redux applications is straightforward because they rely on pure functions. Here are some key points to remember:
- Pure Functions: These functions return the same output for the same input, making them easy to test.
- Action Creators: Test these functions to ensure they return the correct action objects.
- Reducers: Test reducers by passing in the current state and an action to check if the new state is correct.
Common Debugging Scenarios
When debugging Redux applications, you might encounter several common issues:
- State Not Updating: Check if the action is dispatched correctly and if the reducer handles it properly.
- Unexpected State Shape: Ensure that the state structure matches what your components expect.
- Performance Issues: Use the Redux DevTools to monitor re-renders and identify performance bottlenecks.
Debugging Redux applications can be made easier with the right tools and practices. By using Redux DevTools and writing testable code, you can catch errors early and maintain a smooth development process.
Best Practices for Using Redux
Code Organization
To keep your Redux code clean and manageable, consider the following tips:
- Group related files: Organize actions, reducers, and components in the same folder.
- Use clear naming conventions: Name your actions and reducers clearly to reflect their purpose.
- Keep your reducers pure: Ensure that reducers do not have side effects and only return new state based on the input.
Performance Optimization
To enhance the performance of your Redux application, follow these practices:
- Use memoization: Utilize libraries like Reselect to avoid unnecessary re-renders.
- Batch updates: Dispatch multiple actions in a single call to minimize re-renders.
- Avoid deep nesting: Keep your state structure flat to simplify updates and improve performance.
Maintaining Readability
To ensure your code remains readable and understandable:
- Comment your code: Provide explanations for complex logic.
- Use TypeScript: Implement TypeScript for better type safety and clarity.
- Follow a consistent style guide: Adhere to a coding style guide to maintain uniformity across your codebase.
Remember: Following these best practices can significantly improve your Redux application’s maintainability and performance.
Summary Table of Best Practices
Practice | Description |
---|---|
Code Organization | Group related files and use clear naming. |
Performance Optimization | Use memoization and batch updates. |
Maintaining Readability | Comment code and use TypeScript. |
Alternatives to Redux
When it comes to managing state in applications, there are several alternatives to Redux that developers can consider. Each option has its own strengths and weaknesses, making it important to choose the right one for your project.
Context API
The Context API is a built-in feature of React that allows you to share state across components without having to pass props down manually. It’s great for smaller applications or when you need to share data between a few components. Here are some key points:
- Simple to use for small apps.
- No extra libraries needed.
- Good for avoiding prop drilling.
MobX
MobX is another popular state management library that focuses on simplicity and reactivity. It allows you to manage state in a more intuitive way. Here are some highlights:
- Less boilerplate code compared to Redux.
- Automatically tracks state changes.
- Works well with React and other frameworks.
Recoil
Recoil is a relatively new state management library developed by Facebook. It provides a way to manage state that is both flexible and easy to use. Some benefits include:
- Fine-grained control over state updates.
- Supports derived state and asynchronous queries.
- Integrates seamlessly with React.
Library | Pros | Cons |
---|---|---|
Context API | Simple, no extra libraries | Not ideal for large apps |
MobX | Less boilerplate, reactive | Learning curve for new users |
Recoil | Flexible, integrates with React | Still evolving, less mature |
Choosing the right state management tool depends on your app’s size and complexity. Consider your specific needs before making a decision.
Real-World Examples of Redux
Case Studies
Redux is used in many popular applications. Here are a few notable examples:
- Facebook: Manages complex user interactions and real-time updates.
- Instagram: Handles image uploads and user feeds efficiently.
- Netflix: Manages user preferences and streaming data seamlessly.
Sample Applications
Many developers create sample applications to showcase Redux. Some common examples include:
- Todo List App: A simple app to manage tasks.
- Shopping Cart: An app to handle product selections and purchases.
- Weather App: Displays weather data using Redux for state management.
Lessons Learned
From these examples, developers have learned:
- Scalability: Redux helps manage large applications with ease.
- Predictability: State changes are easier to track and debug.
- Community Support: A large community means plenty of resources and tools.
Redux has proven to be a powerful tool for managing state in complex applications, making it a popular choice among developers.
In the world of coding, seeing real-life examples of how Redux is used can really help you understand its power. For instance, many popular apps use Redux to manage their state efficiently, making it easier for developers to handle complex data flows. If you want to dive deeper into coding and learn how to tackle challenges like these, visit our website and start coding for free today!
Conclusion
In this guide, we explored what Redux is and how it can help your applications. While Redux offers many useful features, it’s not always necessary for every project. Knowing when to use Redux is key. One of its biggest advantages is the ability to track changes in the state over time, which helps developers see how the state evolves as the app runs. However, it’s crucial to only use Redux if it meets your needs and if your project requires a tool for managing state.
Frequently Asked Questions
What is Redux used for?
Redux is used to manage and update data in applications, making it easy for multiple parts of the app to share information.
Why should I use Redux?
Redux helps keep your app’s state organized, especially when many parts need to access the same data.
What are actions in Redux?
Actions are events that describe what happened in your app, like a button click or a form submission.
How do reducers work in Redux?
Reducers are functions that take the current state and an action, then return a new state based on that action.
What is the Redux store?
The Redux store is a central place where all the state of your application is kept.
Can I use Redux with any JavaScript framework?
Yes, Redux can be used with various frameworks, but it works best with React.
What are the benefits of using Redux?
Redux makes it easier to manage state, debug your app, and keep your code organized.
When should I consider using Redux?
You should think about using Redux when your app has a lot of shared state that needs to be managed.