Understanding Python Range: A Deep Dive into range() and its Applications
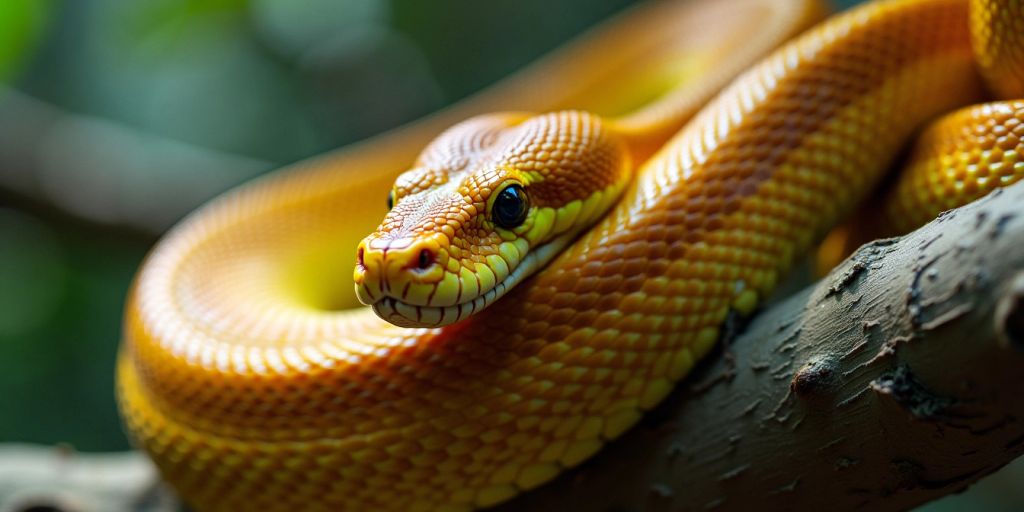
The Python range() function is a handy tool that helps programmers create sequences of numbers. It’s especially useful in loops where you need to repeat actions a certain number of times. This article will break down how range() works, its different parts, and how you can use it effectively in your coding projects. We’ll also look at some common mistakes to avoid and share tips to make the most out of this function.
Key Takeaways
- The range() function generates a sequence of numbers, making it easy to loop through a set of values.
- It has three main parts: start, stop, and step, which control the sequence’s beginning, end, and increments.
- Using range() in loops helps simplify code and makes it more readable.
- The function is memory-efficient because it creates numbers on the fly instead of storing them all at once.
- Understanding the differences between range() in Python 2 and 3 can help avoid compatibility issues.
What is the Python range() Function?
The Python range() function is a built-in tool that generates a sequence of numbers. It is commonly used for iterating over a sequence of numbers in loops. The function can take up to three parameters: start, stop, and step. By default, the start is 0, and the step is 1. The stop parameter defines the upper limit of the sequence, but this limit is not included in the output.
Definition and Purpose
The range() function is essential for creating sequences of numbers. It allows programmers to specify where the sequence begins and ends, as well as the difference between each number. This makes it a powerful tool for various programming tasks.
Basic Syntax and Parameters
The syntax for the range() function is as follows:
range(start, stop, step)
Here’s a quick breakdown of the parameters:
- start: (optional) The starting value of the sequence. Defaults to 0.
- stop: (required) The end value of the sequence, which is not included.
- step: (optional) The difference between each number in the sequence. Defaults to 1.
Common Use Cases
The range() function is widely used in various scenarios, including:
- Looping through a sequence: It is often used in for loops to iterate over a specific range of numbers.
- Creating lists: You can generate lists of numbers easily using range().
- Indexing: It helps in accessing elements in a list by their index.
The Python range() function returns a sequence of numbers, in a given range. The most common use of it is to iterate sequences on a sequence of numbers using loops.
Syntax and Parameters of the range() Function
The range()
function is a built-in function in Python that generates a sequence of numbers. It has three main parameters:
Start Parameter
- Start (optional): This is the first number in the sequence. If you don’t specify it, the default value is 0.
Stop Parameter
- Stop (required): This is the last number in the sequence, but it is not included. For example, if you set it to 5, the sequence will go up to 4.
Step Parameter
- Step (optional): This defines the difference between each number in the sequence. The default is 1. For example, if you set it to 2, the sequence will skip every other number.
Syntax Example
The syntax for using the range()
function is:
range(start, stop, step)
Example Usage
Here’s a simple example of how to use the range()
function:
for i in range(1, 10, 2):
print(i)
This will output:
1
3
5
7
9
Important Note
You can also use a negative step to create a sequence in reverse. For instance:
for i in range(10, 0, -2):
print(i)
This will output:
10
8
6
4
2
The range() function is a powerful tool in Python that helps in generating sequences efficiently. It is one of the built-in functions that are always available for use.
Generating Sequences with the range() Function
Creating Simple Sequences
The range()
function is a handy tool for creating sequences of numbers. It can generate a list of numbers starting from 0 up to a specified limit. For example:
for i in range(5):
print(i)
This code will output:
0
1
2
3
4
Using range() with Steps
You can also specify a step value to skip numbers in the sequence. For instance, using range(0, 10, 2)
will give you:
for i in range(0, 10, 2):
print(i)
This will print:
0
2
4
6
8
Generating Reverse Sequences
To create a sequence in reverse order, you can use a negative step. For example, range(5, 0, -1)
will generate:
for i in range(5, 0, -1):
print(i)
This will output:
5
4
3
2
1
The range() function is versatile and can be used to create various sequences easily.
In summary, the range()
function allows you to:
- Create simple sequences starting from 0.
- Use steps to skip numbers in the sequence.
- Generate sequences in reverse order.
Understanding how to use range()
effectively can enhance your programming skills!
Using the Python range() Function in Loops
The range()
function in Python is a powerful tool for creating sequences of numbers, especially when used in loops. It allows you to iterate over a specific range of values efficiently.
For Loops with range()
In a for
loop, the range()
function is often used to repeat an action a certain number of times. Here’s a simple example:
for i in range(5):
print(i)
Output:
0
1
2
3
4
This code prints numbers from 0 to 4. The range(5)
generates numbers starting from 0 up to, but not including, 5.
While Loops with range()
You can also use range()
in a while
loop to control how many times the loop runs. Here’s an example:
count = 0
while count < 5:
print(count)
count += 1
Output:
0
1
2
3
4
In this case, the loop continues until count
reaches 5, printing each number along the way.
Nested Loops with range()
Using range()
in nested loops can help you create more complex patterns. For example:
for i in range(3):
for j in range(2):
print(f'i: {i}, j: {j}')
Output:
i: 0, j: 0
i: 0, j: 1
i: 1, j: 0
i: 1, j: 1
i: 2, j: 0
i: 2, j: 1
This code creates a grid of values by combining two loops.
Using the range() function in loops is a fundamental skill in Python programming. It helps you control the flow of your code and manage iterations effectively.
Advanced Applications of the range() Function
Creating Lists and Tuples
The range()
function can be very useful for creating lists and tuples. Here’s how you can do it:
- Lists: You can create a list of numbers by wrapping
range()
with thelist()
function. For example:my_list = list(range(0, 10)) # Creates a list from 0 to 9
- Tuples: Similarly, you can create a tuple by using
tuple()
:my_tuple = tuple(range(0, 5)) # Creates a tuple from 0 to 4
Generating Indices for Iteration
Using range()
is a great way to generate indices when you need to iterate over a sequence. For example:
- Accessing elements in a list:
my_list = ['a', 'b', 'c'] for i in range(len(my_list)): print(my_list[i])
- Looping through a string:
my_string = "hello" for i in range(len(my_string)): print(my_string[i])
- Using with
enumerate()
:for index, value in enumerate(my_list): print(index, value)
Implementing Conditional Statements
The range()
function can also be used in conditional statements to control the flow of your program. For example:
- Checking if a number is even:
for num in range(10): if num % 2 == 0: print(num, "is even")
- Counting down:
for num in range(10, 0, -1): print(num)
- Creating a simple countdown timer:
import time for i in range(5, 0, -1): print(i) time.sleep(1) print("Time's up!")
The range() function is a powerful tool that can simplify many tasks in Python programming. By understanding its advanced applications, you can write more efficient and readable code.
Performance Considerations and Optimization Techniques
Memory Efficiency
When using the range()
function, it is important to consider how it uses memory. Unlike lists, which store all elements in memory, range()
generates numbers on-the-fly. This means it is more memory-efficient, especially for large ranges. Using range()
can save a lot of memory compared to creating a list of numbers.
Time Complexity
The time complexity of the range()
function is O(1) for generating the range object. However, the time taken to iterate through the range depends on the number of elements. For example:
Operation | Time Complexity |
---|---|
Creating range | O(1) |
Iterating through | O(n) |
Optimizing range() Usage
To make the most of the range()
function, consider the following tips:
- Use
range()
instead of lists when you only need to iterate through numbers. - Avoid unnecessary conversions to lists unless needed.
- Combine
range()
with other functions for better performance.
Using range() effectively can lead to faster and more efficient code.
By understanding these performance aspects, you can write better Python code that runs smoothly and efficiently.
Comparing range() with Other Iteration Techniques
range() vs. List Comprehension
The range() function is more memory-efficient than list comprehension. This is because it generates numbers on the fly instead of creating a full list of numbers. Here’s a quick comparison:
Feature | range() | List Comprehension |
---|---|---|
Memory Usage | Low | High |
Execution Speed | Faster | Slower |
Output Type | Iterable | List |
range() vs. While Loops
When you need to iterate over a specific range of values, using the range() function is often better than while loops. Here are some reasons:
- Concise Syntax: range() provides a cleaner way to write loops.
- Less Error-Prone: It reduces the chances of mistakes in loop conditions.
- Easier to Read: Code using range() is generally easier to understand.
range() vs. numpy.arange()
The range() function is built into Python, while numpy.arange() is part of the numpy library. Here’s how they compare:
- Lightweight: range() is simpler and uses less memory for basic tasks.
- Powerful: numpy.arange() is better for complex numerical computations.
- Use Case: Choose range() for simple iterations and numpy.arange() for advanced numerical tasks.
In summary, the range() function is a versatile tool that can often outperform other iteration techniques in terms of memory efficiency and readability. Understanding when to use it can greatly enhance your coding skills.
Tips and Tricks for Effective Usage of range()
Utilizing the range() Function in Combination with Other Functions
The range()
function can be paired with other Python functions to enhance its capabilities. Here are some ways to do this:
- Use with
zip()
: Combinerange()
withzip()
to iterate over multiple lists at once. - Pair with
list()
: Convert the output ofrange()
into a list for easier manipulation. - Integrate with
map()
: Apply a function to each number generated byrange()
.
Leveraging the range() Function for Efficient Memory Management
Using range()
is a smart choice for saving memory. Unlike creating a full list of numbers, range()
generates numbers on the fly. This means:
- Less memory usage: Ideal for large sequences.
- Faster execution: Your code runs quicker without the overhead of a full list.
- Simplicity: Keeps your code clean and easy to read.
Exploring Advanced Applications of the Python range() Function
The range()
function is not just for loops. Here are some advanced uses:
- Creating patterns: Use
range()
to generate sequences for graphics or designs. - Simulating data: Generate numbers for testing algorithms or functions.
- Algorithm implementation: Use
range()
in sorting or searching algorithms.
Remember: The range() function is a powerful tool in Python, allowing you to easily create lists and number sequences for a variety of programming applications. By understanding its features, you can optimize your code effectively.
Common Mistakes and How to Avoid Them
Off-by-One Errors
One of the most common mistakes when using the range()
function is the off-by-one error. This happens when you forget that the stop
value is not included in the generated sequence. For example:
for i in range(5):
print(i)
This will print numbers from 0 to 4, not 5. To avoid this mistake, always remember that the stop
value is exclusive.
Incorrect Step Values
Another frequent issue is using incorrect step values. If you set the step to zero, it will raise a ValueError
. Here’s how to use it correctly:
- Positive Step:
range(0, 10, 2)
generates 0, 2, 4, 6, 8. - Negative Step:
range(10, 0, -2)
generates 10, 8, 6, 4, 2.
Make sure your step is not zero and is appropriate for your needs.
Misusing range() in Loops
When using range()
in loops, it’s easy to misuse it, especially in nested loops. For instance, if you want to iterate through a list of items, using range(len(list))
can lead to confusion. Instead, consider using:
- Direct Iteration:
for item in my_list:
- Enumerate:
for index, item in enumerate(my_list):
This way, you avoid potential errors and make your code cleaner.
Remember, understanding how range() works is key to avoiding these common pitfalls. Always test your code with different scenarios to ensure it behaves as expected!
Exploring range() with Practical Examples
Basic Examples
The range()
function is a powerful tool in Python that helps us create sequences of numbers. Here are some simple examples:
- This will print numbers from 0 to 4.
- This will output:
[1, 2, 3, 4, 5]
. - This will give you all even numbers from 0 to 18.
Intermediate Examples
The range()
function can also be used in more complex scenarios:
- This will output:
[1, 4, 9, 16, 25]
. - This will print numbers from 5 to 1.
- This will print each number with a label.
Advanced Examples
For more advanced applications, consider the following:
- This will print combinations of
i
andj
values. - This will output:
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19]
. - This will print each item with its index.
In summary, the range() function in Python is a built-in function we can use to generate a sequence of numbers within a given range. It is versatile and can be applied in various scenarios to simplify coding tasks.
Understanding range() in Python 2 vs. Python 3
Differences Between range() and xrange()
In Python 2, there are two functions for generating sequences of numbers: range() and xrange(). The key difference is that xrange() returns an iterator, which means it generates numbers on the fly, while range() creates a list of all numbers at once. In Python 3, the range() function behaves like xrange() from Python 2, providing better memory efficiency.
Feature | Python 2 (range) | Python 2 (xrange) | Python 3 (range) |
---|---|---|---|
Return Type | List | Iterator | Iterator |
Memory Usage | High | Low | Low |
Speed | Slower | Faster | Faster |
Migration Tips
If you’re moving from Python 2 to Python 3, here are some tips to help you:
- Replace
xrange()
withrange()
: In your loops, simply changexrange()
torange()
. - Test your code: Make sure to run your code to check for any issues after the change.
- Use tools: Consider using tools like
2to3
to automate the conversion process.
Best Practices
To ensure smooth coding in Python 3, keep these practices in mind:
- Always use
range()
for loops to maintain compatibility. - Avoid using
list(range())
unless necessary, as it consumes more memory. - Familiarize yourself with the behavior of
range()
in Python 3 to utilize its full potential.
Understanding the differences between Python 2 and Python 3 is crucial for writing efficient and effective code. By adapting to the changes, you can enhance your programming skills and ensure your code runs smoothly across different versions.
In Python, the range()
function works a bit differently between version 2 and version 3. In Python 2, range()
creates a list of numbers, while in Python 3, it returns a special object that generates numbers on demand. This change helps save memory, especially when dealing with large ranges. If you want to learn more about coding and improve your skills, visit our website and start coding for free!
Conclusion
In summary, the range() function in Python is a handy tool for creating lists of numbers. It’s often used in loops to help us repeat actions a certain number of times. By learning how to use range(), including its start, stop, and step options, we can write better and more efficient code. Understanding these basics can make programming in Python easier and more fun, allowing us to tackle various tasks with confidence.
Frequently Asked Questions
What does the range() function do in Python?
The range() function creates a list of numbers in a specific range. You can use it to repeat actions in loops.
How do you use the range() function?
You call it with one, two, or three numbers. For example, range(5) gives numbers from 0 to 4.
What are the parameters of range()?
The range() function has three parameters: start, stop, and step. Start is where the count begins, stop is where it ends, and step is how much to count by.
Can you give an example of using range() in a loop?
Sure! In a for loop, you can write: for i in range(5): print(i). This will print numbers from 0 to 4.
What happens if I set the step to a negative number?
If you set the step to a negative number, range() will count down. For example, range(5, 0, -1) gives 5, 4, 3, 2, 1.
Is range() available in Python 2 and 3?
Yes, but in Python 2, there’s also a function called xrange(). In Python 3, range() works like xrange() did in Python 2.
Can range() create a list of numbers?
No, range() itself does not create a list. But you can convert it to a list using the list() function, like this: list(range(5)).
Are there any common mistakes when using range()?
Yes, a common mistake is forgetting that the stop value is not included in the output. So, range(5) gives 0 to 4, not 5.