Understanding MVC Architecture: A Comprehensive Guide to Its Principles and Applications
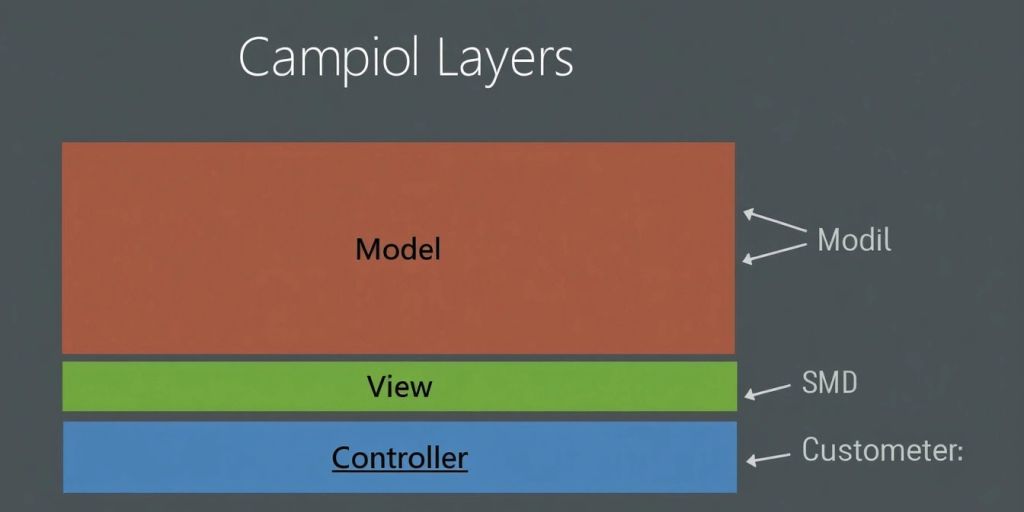
In software development, organizing code properly is key to creating applications that are easy to maintain and expand. One popular method for achieving this is the Model-View-Controller (MVC) architecture. This approach breaks down applications into three main parts: the Model, the View, and the Controller. Each part has its own job, making it easier to manage complex projects. This guide will explore MVC’s principles and its practical uses in the real world.
Key Takeaways
- MVC splits applications into three parts: Model, View, and Controller.
- The Model handles data and rules, the View shows what users see, and the Controller connects the two.
- Using MVC makes it easier to update and test each part separately.
- This architecture helps keep code organized and manageable as projects grow.
- MVC is widely used in web development frameworks like Django and Ruby on Rails.
Core Components of MVC Architecture
Understanding the Model
The Model is the heart of the MVC architecture. It represents the data and the business logic of the application. The Model is responsible for managing data, processing business rules, and responding to requests from the View and Controller. It ensures that the application’s data is accurate and up-to-date.
Role of the View
The View is what the user interacts with. It displays data from the Model and sends user inputs to the Controller. The View is passive, meaning it does not directly interact with the Model. Instead, it receives data from the Model and forwards user actions to the Controller for processing.
Function of the Controller
The Controller acts as a bridge between the Model and the View. It handles user input, updates the Model, and refreshes the View to show any changes. The Controller contains the application logic, such as validating user input and transforming data. This separation of roles helps keep the application organized and easier to manage.
In MVC architecture, each component has a specific role, leading to a more maintainable and scalable application.
Component | Responsibility |
---|---|
Model | Manages data and business logic |
View | Displays data and captures user input |
Controller | Processes input and updates Model and View |
How MVC Architecture Works
User Interaction with the View
When a user interacts with the application, they do so through the View. This is the part of the application that displays information and receives user input. For example, when a user clicks a button or fills out a form, the View captures this action and sends it to the Controller for processing.
Controller Processing
The Controller acts as a middleman between the Model and the View. It takes the input from the View, processes it, and then updates the Model accordingly. This means that the Controller interprets user actions and decides what to do with them. For instance, if a user submits a form, the Controller will validate the data and then update the Model with the new information.
Model Updates and Notifications
Once the Model is updated, it notifies the View about the changes. This is crucial because the View needs to reflect the latest data. The Model holds the application’s data and business logic, ensuring that the information is accurate and up-to-date. This separation of concerns allows for easier maintenance and scalability of the application.
In MVC architecture, each component has a specific role, making it easier to manage complex applications.
Component | Role |
---|---|
Model | Manages data and business logic |
View | Displays data and handles user interface |
Controller | Processes user input and updates Model |
By understanding how these components interact, developers can create applications that are not only functional but also easy to maintain and expand.
Benefits of Using MVC Architecture
Separation of Concerns
MVC architecture promotes a clear separation of concerns. This means that developers can focus on different parts of the application without them getting mixed up. This makes the code easier to read, maintain, and test.
Reusability of Components
By breaking down the application into smaller parts, MVC allows for reusability. Models, Views, and Controllers can be used in different places, which saves time and effort in coding. This modular approach helps in reducing duplication and boosts productivity.
Scalability and Maintainability
As applications grow, MVC makes it easier to scale them. Developers can change or add new features without messing up the whole system. This flexibility is crucial for adapting to new requirements and keeping the application running smoothly.
Enhanced Testability
MVC’s structure allows for better testability. Each part can be tested on its own, ensuring that everything works correctly. This is especially helpful for unit testing, where developers can check the business logic, user interface, and data handling separately.
By using MVC, developers can create applications that are not only efficient but also easy to manage and update.
Benefit | Description |
---|---|
Separation of Concerns | Clear division of responsibilities among components. |
Reusability | Components can be reused across different parts of the application. |
Scalability | Easy to extend and modify without affecting the entire system. |
Enhanced Testability | Simplifies unit testing for each component. |
In summary, the MVC architecture provides a strong foundation for building applications that are organized, flexible, and easy to work with.
Best Practices for Implementing MVC
Keeping Controllers Thin
To ensure a clean architecture, controllers should be kept thin. This means they should only handle the flow of data between the model and the view, without containing complex business logic. By doing this, you make your application easier to manage and understand.
Making Models Independent
Models should be designed to be independent. This means they should handle all the data and business logic on their own. When models are independent, they can be reused in different parts of the application, which saves time and effort.
Using Passive Views
Views should be passive. This means they should not contain any logic. Instead, they should focus on displaying data. Let the controllers handle any data fetching or updates. This keeps your views simple and easy to maintain.
Embracing Dependency Injection
Using dependency injection is a great practice. This means instead of creating objects directly in your controllers or models, you pass them in. This makes your code cleaner and easier to test. It also helps in managing changes in the future.
Summary of Best Practices
Here’s a quick summary of the best practices for implementing MVC:
Best Practice | Description |
---|---|
Keep Controllers Thin | Avoid complex logic in controllers. |
Make Models Independent | Ensure models can be reused across the application. |
Use Passive Views | Keep views simple and focused on displaying data. |
Embrace Dependency Injection | Pass dependencies instead of creating them directly. |
Following these best practices can lead to a more organized and efficient application. By keeping components separate and focused, you can build a system that is easier to maintain and scale over time.
Real-World Applications of MVC
MVC in Web Development Frameworks
MVC is a popular choice in web development. Many frameworks use this architecture to help developers build strong and flexible applications. Some well-known frameworks include:
- Ruby on Rails: A powerful framework for building web applications in Ruby.
- Django: A Python framework that emphasizes rapid development and clean design.
- ASP.NET: A framework for building web apps using .NET technologies.
These frameworks allow developers to create applications that are easy to maintain and scale.
Case Studies of MVC Implementations
Many companies have successfully used MVC in their projects. Here are a few examples:
- E-commerce Websites: Many online stores use MVC to manage product listings, user accounts, and shopping carts.
- Social Media Platforms: These platforms often use MVC to handle user interactions, posts, and notifications.
- Content Management Systems (CMS): Systems like WordPress use MVC to separate content management from presentation.
Challenges and Solutions in MVC
While MVC has many benefits, it also comes with challenges. Here are some common issues and how to solve them:
Challenge | Solution |
---|---|
Overcomplicating small apps | Keep it simple; use MVC only when necessary. |
Poor communication between components | Use clear interfaces and documentation. |
Difficulty in testing | Write unit tests for each component separately. |
MVC architecture is a powerful tool for developers, but it’s important to use it wisely to avoid unnecessary complexity.
By understanding how MVC works in real-world applications, developers can better utilize its strengths and overcome its challenges.
Common Pitfalls and How to Avoid Them
Overcomplicating Small Projects
When working on smaller projects, it’s easy to overcomplicate things by applying MVC principles too rigidly. Instead, keep it simple. Focus on the essential features and avoid unnecessary layers. This will help you save time and effort.
Ignoring Separation of Concerns
One of the main ideas behind MVC is the separation of concerns. If you mix up your Model, View, and Controller, it can lead to confusion and bugs. Always ensure that each component has its own responsibilities. This makes your code cleaner and easier to manage.
Poor Naming Conventions
Using unclear or confusing names for your classes and methods can lead to misunderstandings. Stick to clear and descriptive names. For example, instead of naming a method doStuff
, use calculateTotalPrice
. This helps others (and your future self) understand your code better.
Remember, simplicity is key. Keeping your code straightforward will make it easier to maintain and understand.
Pitfall | Description | How to Avoid |
---|---|---|
Overcomplicating Small Projects | Adding unnecessary complexity to simple applications. | Focus on essential features. |
Ignoring Separation of Concerns | Mixing responsibilities of Model, View, and Controller. | Keep components distinct. |
Poor Naming Conventions | Using unclear names for classes and methods. | Use clear and descriptive names. |
When preparing for coding interviews, many people stumble over common mistakes that can be easily avoided. To ensure you don’t fall into these traps, check out our resources that guide you through effective study techniques and practical coding exercises. Don’t let these pitfalls hold you back—visit our website today and start your journey to success!
Conclusion
In summary, the Model-View-Controller (MVC) architecture is an effective way to design software that is easy to manage and expand. By breaking down an application into three main parts—Model, View, and Controller—MVC helps keep the code organized and makes it easier to work on. This structure not only improves how developers write code but also makes it simpler to test and reuse different parts of the application. Understanding MVC is key for anyone looking to create strong and flexible software. It lays the groundwork for building applications that are clear, adaptable, and efficient.
Frequently Asked Questions
What is the main goal of MVC architecture?
The main goal of MVC architecture is to divide an application into three parts: the Model, the View, and the Controller. This helps keep the code organized and makes it easier to work on.
Can I use MVC with any programming language?
Yes, you can use MVC with almost any programming language. It works well with languages like JavaScript, Python, Ruby, and C#.
How does MVC make testing easier?
MVC makes testing easier because it separates different parts of the application. You can test each part on its own, which helps ensure everything works well.
Are there any downsides to using MVC?
While MVC is helpful, it can make things a bit complicated, especially for smaller projects. Beginners might also find it a little hard to learn.
What does the Controller do in MVC?
The Controller acts like a middleman between the Model and the View. It takes user input, updates the Model, and then changes the View to show the new data.
How does MVC help with organizing code?
MVC helps organize code by clearly separating different functions. This makes it easier for developers to work on specific parts without messing up other areas.