Understanding HTML Inline Styles: A Comprehensive Guide
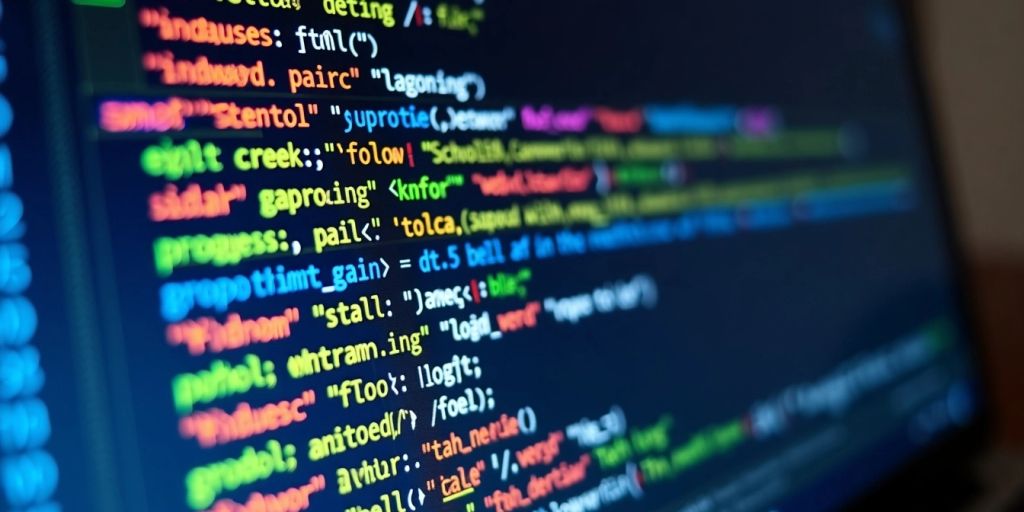
Inline styles in HTML provide a simple way to apply CSS directly to specific elements. This guide will help you understand what inline styles are, their benefits and drawbacks, and when to use them effectively. By the end, you’ll be able to make informed decisions about styling your web pages with inline styles.
Key Takeaways
- Inline styles are applied directly within HTML tags using the ‘style’ attribute.
- They are great for quick changes but can make your code harder to read.
- Using inline styles can increase the size of your HTML files.
- Consider using inline styles for testing, dynamic content, or HTML emails.
- Always aim for balance; use inline styles sparingly and prefer external stylesheets for most cases.
What Are HTML Inline Styles?
Definition and Syntax
Inline styles are a way to add CSS directly to an HTML element using the style attribute. This means you can change how an element looks right in the HTML code. The basic format is:
<tag style="property1: value1; property2: value2;">
For example, to make a paragraph’s text red, you could write:
<p style="color: red;">This is a red paragraph.</p>
Examples of Inline Styles
Here are some common CSS properties you can use with inline styles:
- color
- background-color
- font-size
- margin
- padding
You can combine multiple styles in one line, like this:
<div style="background-color: yellow; padding: 10px;">This div has a yellow background.</div>
Differences from Internal and External Styles
Inline styles are different from internal and external styles because they are written directly in the HTML element. This means they are quick to apply but can make your code harder to read. Inline CSS is a style sheet that includes a CSS property in the content of an element. It’s best to use inline styles only when necessary, as they can clutter your HTML.
Remember: Using inline styles can be helpful for quick changes, but they should be used sparingly to keep your code clean and organized.
Benefits of Using HTML Inline Styles
Quick and Easy Application
Using inline styles is super simple. You just add the style
attribute to an HTML element, and you can apply styles right away. This means you don’t have to create separate stylesheets or navigate through multiple files. It’s a fast way to make changes without much hassle.
Higher Specificity
Inline styles have a special power: they can override other styles. This is because they have higher specificity than styles defined in internal or external stylesheets. So, if you want to make sure a certain style is applied, using inline styles is a reliable choice.
No Additional HTTP Requests
Another great benefit is that inline styles are part of the HTML file itself. This means they don’t require any extra downloads, unlike external stylesheets. This can help your page load faster, especially for critical content that needs to be displayed quickly.
Inline styles can be very useful for quick changes, but remember to use them wisely to keep your code clean.
Benefit | Description |
---|---|
Quick Application | Add styles directly in the HTML tag without extra files. |
Higher Specificity | Inline styles override other styles due to their higher specificity. |
No Extra Downloads | Styles are included in the HTML, reducing load times for critical content. |
In summary, while inline styles can be very handy, it’s important to use them sparingly to maintain clean and manageable code.
Common Use Cases for HTML Inline Styles
Rapid Testing and Experimentation
Using inline styles is a quick way to test how different styles look on a webpage. You can change styles directly in the HTML without needing to switch files or refresh the page. This is especially useful for developers who want to see immediate results.
Dynamically Generated Content
When content is created by JavaScript, inline styles allow you to easily set visual properties on the fly. This means you can change how elements look based on user actions or other events without needing to reload the page.
Styling HTML Emails
Many email clients strip out external styles, making inline styles the most reliable way to style HTML emails. This ensures that your emails look good across different platforms, as inline styles are less likely to be removed.
Summary of Use Cases
Here’s a quick list of when to use inline styles:
- Quick testing of styles
- Dynamic content changes
- HTML emails for consistent styling
- Third-party platforms that only allow inline styles
- Overriding other styles easily
Inline styles can be very handy in specific situations, but they should be used carefully to avoid cluttering your HTML.
In conclusion, while inline styles have their place, it’s best to use them sparingly and consider other styling methods for most cases.
Drawbacks of HTML Inline Styles
Not Easily Reused
Inline styles are applied directly to individual HTML elements, which means they cannot be reused across multiple elements or pages. This leads to redundancy, as you must repeat the same styles for each element, making your code less efficient.
Harder to Maintain
When styles are scattered throughout your HTML, it becomes challenging to keep track of them. If you want to change a style, you have to search through your markup instead of updating a single stylesheet. This can be time-consuming and prone to errors.
Increased File Size
Using many inline styles can bloat your HTML files. Each inline style adds to the overall size of the document, which can slow down loading times, especially on slower internet connections. This is a significant drawback compared to using external stylesheets, which keep your HTML cleaner and smaller.
In summary, while inline styles can be convenient, they often lead to a cluttered structure and maintenance challenges.
Summary of Drawbacks
Drawback | Description |
---|---|
Not Easily Reused | Styles must be repeated for each element, leading to redundancy. |
Harder to Maintain | Changes require searching through HTML instead of updating a single stylesheet. |
Increased File Size | More inline styles lead to larger HTML files, affecting loading times. |
No Support for Pseudo-Classes | Inline styles cannot target pseudo-elements or pseudo-classes, limiting styling options. |
Reduces Readability | Mixing style with structure makes HTML harder to read and understand at a glance. |
Overall, while inline styles have their uses, they come with significant drawbacks that can affect your project’s efficiency and maintainability.
Best Practices for HTML Inline Styles
Use Sparingly and When Necessary
Inline styles should be used sparingly. Reserve them for unique or one-off styling needs that cannot be easily addressed with external or internal styles. This helps keep your code clean and manageable.
Keep Declarations Short
When using inline styles, try to limit the number of properties you include. Keeping declarations short not only reduces clutter but also makes your HTML easier to read. For example:
<p style="color: red; font-size: 16px;">This is a styled paragraph.</p>
Document Inline Styles
It’s important to document any inline styles you use. Adding comments can help others (or yourself in the future) understand why a specific style was applied. For instance:
<p style="color: red;">This is a red paragraph. <!-- Used for emphasis --></p>
Remember, maintainability is key. Keeping your code organized will save you time in the long run.
Avoid Duplication
If you find yourself repeating the same inline styles across multiple elements, consider creating a CSS class instead. This will help reduce redundancy and improve maintainability. For example:
<style>
.red-text { color: red; }
</style>
<p class="red-text">This is a red paragraph.</p>
<p class="red-text">This is another red paragraph.</p>
By following these best practices, you can effectively use inline styles while keeping your HTML clean and efficient.
Advanced Techniques with HTML Inline Styles
Using JavaScript to Apply Inline Styles
You can easily change inline styles using JavaScript. This is helpful for making your web pages interactive. For example, you can set the color of an element like this:
document.getElementById('myElement').style.color = 'green';
This code changes the text color of the element with the ID myElement
to green. This technique allows for dynamic styling based on user actions.
Combining Multiple Styles
When you want to apply several styles at once, you can combine them in a single style
attribute. For example:
<div style="color: red; background-color: yellow; font-size: 20px;">
This text is red on a yellow background with a larger font size.
</div>
This method keeps your HTML clean and makes it easy to see all styles applied to an element at a glance.
Leveraging CSS Variables
CSS variables can also be used with inline styles. This allows you to define a variable and use it in your inline styles. For example:
<style>
--main-color: blue;
</style>
<div style="color: var(--main-color);">
This text is blue.
</div>
Using CSS variables helps maintain consistency and makes it easier to change styles later.
Inline styles can be powerful, but they should be used wisely. Overusing them can lead to messy code that is hard to maintain.
Summary
In summary, advanced techniques like using JavaScript, combining styles, and leveraging CSS variables can enhance your use of inline styles. These methods allow for more flexibility and control over how your elements look and behave on the page. Remember to use these techniques thoughtfully to keep your code organized and efficient.
Common Pitfalls and How to Avoid Them
Overusing Inline Styles
Using inline styles too much can make your code messy and hard to manage. It’s better to use them only when necessary and rely on external or internal CSS for most of your styling needs.
Specificity Issues
Inline styles have a higher specificity than styles defined in external or internal stylesheets. This can lead to unexpected results when trying to apply styles. Always be aware of how styles interact with each other.
Performance Concerns
While inline styles do not require extra HTTP requests, using too many can slow down your website. This is because they increase the size of your HTML file, which can lead to longer loading times, especially on slower connections.
Lack of Consistency
Achieving a consistent look across your website can be tough with inline styles. If you want to ensure a uniform appearance, consider using CSS classes and external stylesheets. This helps avoid slight variations that can confuse users.
Remember: Keeping your HTML clean and organized is key to maintaining a good user experience.
Summary of Common Pitfalls
Pitfall | Description |
---|---|
Overusing Inline Styles | Leads to cluttered code and maintenance issues. |
Specificity Issues | Higher specificity can cause unexpected style results. |
Performance Concerns | Increases HTML file size, slowing down page load times. |
Lack of Consistency | Makes it hard to maintain a uniform look across the site. |
By being mindful of these pitfalls, you can use inline styles effectively without compromising your code’s quality.
When preparing for coding interviews, many people stumble over common mistakes that can be easily avoided. To ensure you’re on the right track, check out our resources that guide you through the process step-by-step. Don’t let these pitfalls hold you back—visit our website today and start your journey to coding success!
Conclusion
In this guide, we explored the world of HTML inline styles! While they aren’t the main way to style a webpage, they can be useful in certain situations. Inline styles are great for quick changes, dynamic effects, and when you need to override other styles. However, it’s important to use them wisely. Most of your styling should come from external CSS files to keep your code clean and easy to manage. Remember, inline styles can be handy, but they should be used carefully. With this knowledge, you can now use inline styles effectively in your HTML projects!
Frequently Asked Questions
What are HTML inline styles?
HTML inline styles are CSS styles that you apply directly to an HTML element using the ‘style’ attribute. This means you can change how a specific element looks right in the HTML code.
When should I use inline styles?
You should use inline styles when you need to quickly change the appearance of a single element, like during testing or when creating dynamic content with JavaScript.
What are the advantages of using inline styles?
Inline styles are easy to apply and don’t require extra files. They can also override other styles, making them useful for quick adjustments.
What are the downsides of inline styles?
The main downsides are that they can make your code harder to read, are not reusable for multiple elements, and can lead to larger file sizes.
How do inline styles compare to external stylesheets?
Inline styles are applied directly to elements, while external stylesheets keep styling separate from HTML. External styles are generally better for maintaining a clean and organized codebase.
Can I use JavaScript with inline styles?
Yes! You can easily change inline styles using JavaScript, allowing for dynamic updates to how elements look based on user actions.