Understanding Binary Tree vs Binary Search Tree: Key Differences Explained
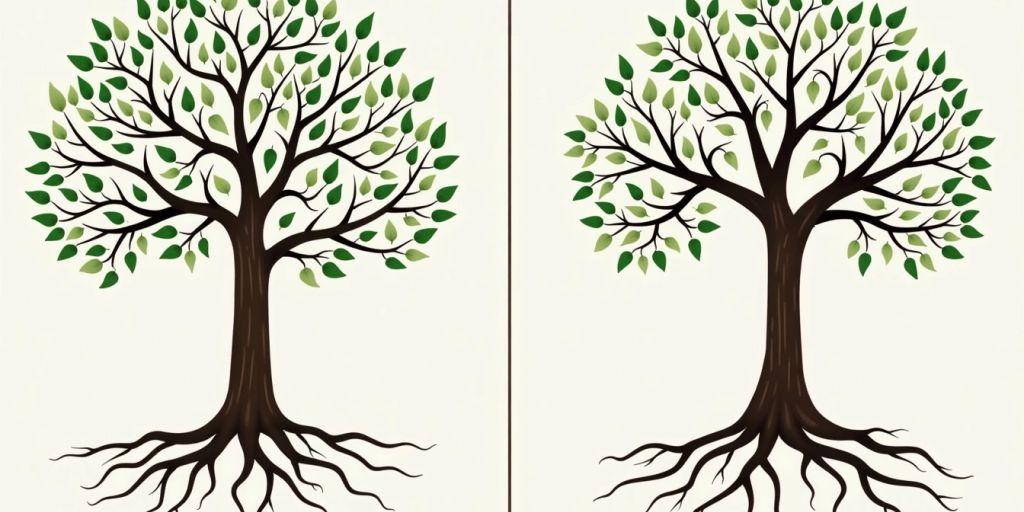
In this article, we will explore the key differences between binary trees and binary search trees. While both structures are essential in computer science, they serve different purposes and have unique characteristics. Understanding these differences will help you choose the right tree structure for your data organization needs.
Key Takeaways
- A binary tree can have up to two children per node, while a binary search tree (BST) organizes nodes in a specific order.
- In a binary tree, nodes can be arranged randomly, but in a BST, left child nodes are always smaller than their parent node.
- Binary trees allow duplicate values, whereas BSTs do not permit duplicates.
- Searching in a binary tree may require checking every node, making it slower than searching in a BST.
- Insertion and deletion operations in a binary tree do not follow any order, while BSTs maintain order during these operations.
- The height of a binary tree can vary widely, affecting its efficiency, while BSTs aim to keep a balanced height for optimal performance.
- Binary trees are used in various applications like expression trees, while BSTs are ideal for fast data retrieval.
- Understanding the differences between these two structures is crucial for effective data management in programming.
Definition and Basic Concepts of Binary Tree vs Binary Search Tree
Understanding Binary Trees
A binary tree is a type of data structure where each node can have up to two children. This means a node can have zero, one, or two children. The top node is called the root, and nodes without children are known as leaf nodes. Binary trees are used in various applications, such as expression trees and decision trees.
Understanding Binary Search Trees
A binary search tree (BST) is a special kind of binary tree. In a BST, the left child of a node contains values that are less than the node’s value, while the right child contains values that are greater than the node’s value. This arrangement allows for efficient searching, insertion, and deletion operations.
Key Characteristics of Binary Trees
- Each node can have 0, 1, or 2 children.
- The height of the tree is the longest path from the root to a leaf node.
- Supports various traversal methods like in-order, pre-order, and post-order.
Key Characteristics of Binary Search Trees
- Nodes are arranged in a specific order, which helps in efficient searching.
- No duplicate nodes are allowed.
- Both left and right subtrees must also be binary search trees.
Common Misconceptions
Many people confuse binary trees with binary search trees. While all binary search trees are binary trees, not all binary trees are binary search trees. The key difference lies in the ordering of the nodes.
Importance in Computer Science
Understanding these structures is crucial in computer science as they are foundational for various algorithms and data management techniques. They help in organizing data efficiently, which is essential for performance in applications like databases and search engines.
Binary trees and binary search trees are fundamental concepts that help in organizing data efficiently. Their unique properties make them suitable for different applications in computer science.
Structural Differences Between Binary Tree and Binary Search Tree
Node Arrangement in Binary Trees
In a binary tree, each node can have up to two children, known as the left and right child. The arrangement of nodes does not follow any specific order. This means that the values of the nodes can be placed randomly.
Node Arrangement in Binary Search Trees
In contrast, a binary search tree (BST) has a specific arrangement. In a BST:
- All nodes in the left subtree have values less than the parent node.
- All nodes in the right subtree have values greater than the parent node.
- This structure allows for efficient searching and sorting of values.
Root Node Characteristics
- The root node in both trees is the topmost node.
- In a binary tree, the root can have any value, while in a BST, the root’s value determines the arrangement of its children.
Child Node Characteristics
- In a binary tree, child nodes can have any values.
- In a BST, child nodes must follow the rule of being less than or greater than the parent node.
Leaf Node Characteristics
- Leaf nodes in both trees are nodes without children.
- In a binary tree, leaf nodes can be anywhere, while in a BST, they are determined by the values of their ancestors.
Impact on Tree Traversal
The structure of these trees affects how we traverse them:
- In a binary tree, traversal can be done in various ways without any specific order.
- In a BST, traversal methods like in-order traversal yield sorted values, making it easier to retrieve data efficiently.
Understanding the structural differences between binary trees and binary search trees is crucial for selecting the right data structure for specific applications.
Feature | Binary Tree | Binary Search Tree |
---|---|---|
Node Arrangement | Random | Ordered |
Search Efficiency | O(n) | O(h) (height) |
Duplicate Values Allowed | Yes | No |
Insertion Operations in Binary Tree vs Binary Search Tree
General Insertion in Binary Trees
In a binary tree, insertion can happen at any position. The new node is typically added as a leaf node. Here are the steps for inserting a node:
- Start at the root node.
- Traverse the tree level by level.
- Insert the new node in the first available position.
General Insertion in Binary Search Trees
In a binary search tree (BST), insertion follows a specific order. A new key is always inserted at the leaf by maintaining the property of the binary search tree. The steps are:
- Start at the root node.
- Compare the new key with the current node’s key.
- If the new key is smaller, move to the left child; if larger, move to the right child.
- Repeat until you find an empty spot to insert the new node.
Insertion Complexity
- Binary Tree: O(n) in the worst case, as you may need to traverse the entire tree.
- Binary Search Tree: O(h), where h is the height of the tree. In a balanced BST, this is O(log n).
Handling Duplicates
- Binary Tree: Duplicates can be inserted without any restrictions.
- Binary Search Tree: Duplicates are generally not allowed, but if allowed, they can be placed in a specific manner (e.g., always to the right).
Balancing the Tree
- Binary Tree: No balancing is required.
- Binary Search Tree: Balancing techniques (like AVL or Red-Black trees) can be applied to maintain efficiency.
Practical Examples
- Binary Tree: Used in applications where the order of nodes is not important.
- Binary Search Tree: Ideal for applications requiring fast search, insert, and delete operations, such as databases.
Insertion in a binary search tree is crucial for maintaining its ordered structure, which allows for efficient searching and retrieval of data.
Deletion Operations in Binary Tree vs Binary Search Tree
General Deletion in Binary Trees
In a binary tree, deleting a node can be straightforward. You can remove a node without worrying about the order of the remaining nodes. Here are the steps:
- Find the node you want to delete.
- If the node has no children, simply remove it.
- If the node has one child, replace it with its child.
- If the node has two children, you can replace it with either its inorder predecessor or inorder successor.
General Deletion in Binary Search Trees
In a binary search tree (BST), deletion is a bit more complex due to the need to maintain the order of nodes. The process involves:
- Locate the node to delete.
- If the node has no children, remove it directly.
- If it has one child, link its parent to its child.
- If it has two children, the trick is to find the inorder successor of the node. Copy the contents of the inorder successor to the node, and delete the inorder successor.
Deletion Complexity
The complexity of deletion operations varies:
- In a binary tree, deletion is generally O(1) if the node is a leaf.
- In a BST, the average deletion complexity is O(h), where h is the height of the tree.
Handling Duplicates
Handling duplicates can differ:
- In a binary tree, duplicates can exist without any specific rules.
- In a BST, duplicates are usually not allowed, but if they are, you can decide to either ignore them or store them in a specific way.
Balancing the Tree
After deletion, it’s important to check if the tree remains balanced:
- In a binary tree, balancing is not a concern.
- In a BST, you may need to implement balancing techniques like rotations to maintain efficiency.
Practical Examples
- Example 1: Deleting a leaf node in a binary tree is simple and quick.
- Example 2: In a BST, deleting a node with two children requires finding the inorder successor, which can be a bit tricky.
Deletion operations are crucial for maintaining the efficiency of both binary trees and binary search trees. Understanding the differences helps in choosing the right approach for your data structure needs.
Search Operations in Binary Tree vs Binary Search Tree
General Search in Binary Trees
Searching in a binary tree is not as efficient as in a binary search tree. In a binary tree, you may need to check every node to find a specific value. This can take a long time, especially if the tree is large. Here are some key points:
- No specific order: Nodes can be arranged in any way.
- Traversal needed: You often have to use traversal methods like in-order or pre-order to find a value.
- Time complexity: The worst-case scenario is O(n), where n is the number of nodes.
General Search in Binary Search Trees
In a binary search tree (BST), searching is much faster due to its organized structure. The properties of a BST allow you to eliminate half of the tree with each comparison. Here’s how it works:
- Ordered nodes: Left child nodes are less than the parent, and right child nodes are greater.
- Efficient searching: You can quickly decide which side of the tree to search next.
- Time complexity: The average time complexity is O(log n), making it much faster than a binary tree.
Search Complexity
Tree Type | Average Time Complexity | Worst-Case Time Complexity |
---|---|---|
Binary Tree | O(n) | O(n) |
Binary Search Tree | O(log n) | O(n) |
Efficiency Comparison
- Binary Tree: Slower due to lack of order.
- Binary Search Tree: Faster because of its sorted nature.
- Use Cases: BSTs are preferred for applications requiring frequent searches.
Handling Duplicates
- Binary Tree: Can store duplicates without any special rules.
- Binary Search Tree: Usually, duplicates are not allowed, but some implementations may allow them by placing duplicates in a specific subtree.
Practical Examples
- Binary Tree: Used in scenarios where data is not frequently searched, like representing hierarchical data.
- Binary Search Tree: Ideal for databases and applications where quick search, insert, and delete operations are necessary.
In summary, searching in a binary search tree is significantly more efficient than in a binary tree due to its structured arrangement of nodes. Understanding these differences is crucial for selecting the right data structure for your needs.
Traversal Methods in Binary Tree and Binary Search Tree
In-Order Traversal
In in-order traversal, the nodes are visited in the following order: left child, parent, and then right child. This method is particularly useful for binary search trees because it retrieves the nodes in sorted order.
Pre-Order Traversal
In pre-order traversal, the nodes are accessed in this order: parent, left child, and then right child. This method is often used to create a copy of the tree or to get a prefix expression of the tree.
Post-Order Traversal
In post-order traversal, the nodes are visited in the order of left child, right child, and then parent. This is useful for deleting trees or evaluating postfix expressions.
Level-Order Traversal
Level-order traversal visits nodes level by level from top to bottom and left to right. This method is implemented using a queue and is useful for finding the shortest path in unweighted trees.
Traversal Complexity
The time complexity for all these traversal methods is O(n), where n is the number of nodes in the tree. This is because each node is visited exactly once.
Practical Examples
- In-Order: For a BST, in-order traversal gives sorted values.
- Pre-Order: Used in serialization of trees.
- Post-Order: Useful in deleting nodes safely.
- Level-Order: Helps in finding the height of the tree.
Tree traversal techniques are essential for accessing each node of the tree in a specific order. Understanding these methods is crucial for effective data management in computer science.
Balancing Techniques in Binary Tree vs Binary Search Tree
Importance of Balancing
Balancing a tree is crucial for maintaining its efficiency. A balanced binary tree ensures that operations like insertion, deletion, and searching can be performed quickly. When a tree is balanced, the height is kept to a minimum, which directly affects performance.
Balancing in Binary Trees
- General Approach: Binary trees do not have strict balancing rules. They can become unbalanced, leading to inefficient operations.
- Common Techniques: While there are no specific balancing techniques for general binary trees, some methods include:
- Rearranging nodes to improve structure.
- Using heuristics to maintain a more balanced shape.
Balancing in Binary Search Trees
- Key Techniques: Binary search trees (BSTs) often use specific balancing techniques to maintain efficiency:
- AVL Trees: These trees maintain a balance factor of -1, 0, or 1 for each node, ensuring the height remains logarithmic.
- Red-Black Trees: These trees use color properties to ensure balance, allowing for efficient insertions and deletions.
AVL Trees
- Definition: An AVL tree is a type of self-balancing binary search tree where the difference in heights between the left and right subtrees is at most one.
- Height Maintenance: A binary tree is balanced if the height of the tree is O(log n) where n is the number of nodes. For example, the AVL tree maintains O(log n) height.
Red-Black Trees
- Properties: Red-black trees maintain balance through color-coding nodes, ensuring that no path from the root to a leaf is more than twice as long as any other such path.
- Efficiency: This balancing technique allows for efficient search, insertion, and deletion operations.
Impact on Performance
- Traversal Efficiency: Balanced trees allow for faster traversal methods, reducing the time complexity of operations.
- Comparison Table:
Tree Type | Balancing Technique | Height Complexity | Operations Complexity |
---|---|---|---|
Binary Tree | None | O(n) | O(n) |
AVL Tree | Height Balancing | O(log n) | O(log n) |
Red-Black Tree | Color Properties | O(log n) | O(log n) |
Balancing techniques are essential for optimizing the performance of binary trees and binary search trees, ensuring efficient data operations.
Applications of Binary Tree vs Binary Search Tree
Use Cases of Binary Trees
- Expression Trees: Used in compilers to represent expressions.
- Decision Trees: Help in making decisions based on certain conditions.
- Hierarchical Data Representation: Useful for representing data with a parent-child relationship.
Use Cases of Binary Search Trees
- Database Indexing: Efficiently organizes data for quick retrieval.
- Sorting Algorithms: Helps in sorting data quickly.
- Dynamic Set Operations: Supports operations like insertions and deletions efficiently.
Real-World Examples
- File Systems: Binary trees can represent directories and files.
- Network Routing: Binary search trees help in routing data efficiently.
- Game Development: Used for managing game states and decisions.
Performance Considerations
- Binary Trees: Generally simpler but can be less efficient for searching.
- Binary Search Trees: More complex but provide faster search times due to their ordered structure.
Scalability
- Binary Trees: Can handle various data sizes but may become inefficient as data grows.
- Binary Search Trees: Scale better with larger datasets due to their search efficiency.
Future Trends
- Emerging Technologies: Both structures are being adapted for new technologies like AI and machine learning.
- Innovations in Tree Structures: New types of trees are being developed to improve performance and efficiency.
In summary, understanding the applications of binary trees and binary search trees is crucial for effective data management in computer science. Their unique properties make them suitable for different tasks, from simple data representation to complex algorithms.
Advantages and Disadvantages of Binary Tree vs Binary Search Tree
Advantages of Binary Trees
- Flexible Structure: Binary trees can have varying shapes, allowing for diverse applications.
- Simple Implementation: They are easier to implement compared to binary search trees.
- Supports Various Traversals: Different traversal methods can be applied, making them versatile.
Disadvantages of Binary Trees
- Inefficient Searching: Searching for a specific value can be slow, especially if the tree is unbalanced.
- No Order: There is no specific order for the nodes, which can complicate certain operations.
- Memory Usage: They may use more memory due to pointers for child nodes.
Advantages of Binary Search Trees
- Faster Search Operations: Searching is quicker due to the sorted nature of the nodes.
- Efficient Insertions and Deletions: Operations can be performed faster compared to binary trees.
- Ordered Structure: The order of nodes allows for easier data management.
Disadvantages of Binary Search Trees
- Not Self-Balancing: Unbalanced BSTs can lead to poor performance, especially in the worst-case scenarios.
- Complex Implementation: They can be more complex to implement and maintain.
- Handling Duplicates: Duplicates can complicate the structure and operations.
Feature | Binary Tree | Binary Search Tree |
---|---|---|
Search Time | O(n) | O(log n) (average case) |
Insertion Time | O(n) | O(log n) (average case) |
Deletion Time | O(n) | O(log n) (average case) |
In summary, while binary trees offer flexibility and simplicity, binary search trees provide efficiency and order, making them suitable for different applications. Understanding these differences is crucial for choosing the right data structure for your needs.
Complexity Analysis of Binary Tree vs Binary Search Tree
Time Complexity
When comparing the time complexity of operations in binary trees and binary search trees, it’s essential to understand how they differ:
Operation | Binary Tree (BT) | Binary Search Tree (BST) |
---|---|---|
Insertion | O(n) | O(h) |
Deletion | O(n) | O(h) |
Search | O(n) | O(h) |
- O(n) indicates that the operation may require traversing all nodes in the worst case.
- O(h) means the operation depends on the height of the tree, which is generally lower in a balanced BST.
Space Complexity
Both binary trees and binary search trees have a space complexity of O(n), as they store the same number of nodes. However, the arrangement of nodes in a BST can lead to more efficient use of space in certain scenarios.
Insertion Complexity
- Binary Tree: Insertion can happen at any position, leading to a time complexity of O(n) in the worst case.
- Binary Search Tree: Insertion follows the BST property, making it faster with a time complexity of O(h).
- Balancing: If the BST is balanced, the height (h) is minimized, improving performance.
Deletion Complexity
- Deleting a node in a binary tree can be straightforward but may require O(n) time.
- In a binary search tree, deletion is more complex due to the need to maintain the BST properties, but it still operates in O(h) time.
Search Complexity
- Searching in a binary tree may require checking every node, resulting in O(n) time.
- In contrast, searching in a binary search tree is efficient, typically O(h), due to its ordered structure.
Understanding the time complexity of key operations is crucial when choosing between a binary search tree and other data structures.
Summary
In summary, binary search trees generally offer better performance for insertion, deletion, and search operations compared to binary trees, especially when balanced. This makes them a preferred choice in many applications where efficiency is key.
Memory Usage in Binary Tree vs Binary Search Tree
Memory Allocation
- Binary Trees: Memory is allocated for each node without any specific order. Each node can have up to two children, leading to potentially wasted space.
- Binary Search Trees: Memory is allocated based on the values of the nodes, ensuring that left child values are smaller and right child values are larger. This can lead to more efficient use of memory.
Memory Efficiency
- Binary Trees: Can be less efficient due to the lack of structure. Nodes can be scattered, leading to higher memory usage.
- Binary Search Trees: More efficient as they maintain a specific order, which can reduce the overall height of the tree and thus the memory used.
Impact of Tree Height
- The height of a tree affects memory usage significantly. A taller tree (more levels) can lead to increased memory usage due to more pointers.
- Balanced Trees: Both types benefit from balancing techniques, which help keep the height low and memory usage efficient.
Feature | Binary Tree | Binary Search Tree |
---|---|---|
Memory Allocation | Unordered | Ordered |
Memory Efficiency | Less efficient | More efficient |
Impact of Tree Height | Higher with more levels | Lower with balancing |
Impact of Balancing
- Balancing techniques can significantly reduce memory usage in both tree types. For example, AVL trees and Red-Black trees are used to keep binary search trees balanced, which helps in maintaining efficient memory usage.
In summary, the structure of a binary search tree allows for more efficient memory usage compared to a binary tree. This is due to its ordered nature, which helps in reducing the height and thus the memory required for pointers.
Common Algorithms Used with Binary Tree and Binary Search Tree
Insertion Algorithms
- Binary Tree Insertion: In a binary tree, insertion can happen at any available position. The new node is added as a child of the first available node.
- Binary Search Tree Insertion: In a BST, the new node is placed in a specific position. If the value is less than the current node, it goes to the left; if greater, it goes to the right.
- Complexity: Insertion in a binary tree is O(1) for adding at the end, while in a BST, it is O(h), where h is the height of the tree.
Deletion Algorithms
- Binary Tree Deletion: To delete a node, find the node and remove it. If it has children, replace it with the last node in the tree.
- Binary Search Tree Deletion: In a BST, deletion is more complex. If the node has two children, find the in-order successor to replace it.
- Complexity: Deletion in a binary tree is O(1) if removing the last node, while in a BST, it is O(h).
Search Algorithms
- Binary Tree Search: Searching in a binary tree is done by traversing each node. It can take O(n) time in the worst case.
- Binary Search Tree Search: Searching in a BST is efficient. You can skip half of the tree based on comparisons, leading to O(h) time complexity.
- Key Point: Binary search trees (BSTs) are data structures that excel in searching, insertion, and deletion operations.
Traversal Algorithms
- In-Order Traversal: Visit left child, then parent, then right child. This gives sorted order in a BST.
- Pre-Order Traversal: Visit parent first, then left and right children. Useful for copying the tree.
- Post-Order Traversal: Visit children first, then parent. This is used for deleting the tree.
Balancing Algorithms
- Balancing in Binary Trees: Generally, binary trees do not require balancing.
- Balancing in Binary Search Trees: Techniques like AVL trees and Red-Black trees are used to keep the tree balanced, ensuring O(log n) operations.
Optimization Techniques
- Caching: Store results of expensive operations to speed up future requests.
- Iterative Methods: Use loops instead of recursion to save memory and avoid stack overflow.
Understanding these algorithms is crucial for efficient data management and retrieval in computer science.
Binary Tree vs Binary Search Tree in Database Indexing
Role in Database Indexing
Binary trees and binary search trees (BSTs) are essential in organizing data for quick access. Binary search trees are particularly effective for indexing because they maintain a sorted order, allowing for faster searches.
Efficiency in Data Retrieval
- Binary Trees:
- Binary Search Trees:
Impact on Query Performance
- Query Speed: BSTs can reduce search time significantly compared to binary trees.
- Complex Queries: BSTs handle complex queries more efficiently due to their structure.
- Scalability: As data grows, BSTs maintain performance better than binary trees.
Feature | Binary Tree | Binary Search Tree |
---|---|---|
Search Complexity | O(n) | O(log n) |
Insertion Complexity | O(n) | O(log n) |
Structure | Unordered | Ordered |
Balancing and Rebalancing
- Importance of Balancing: Keeping a BST balanced is crucial for maintaining efficiency.
- Rebalancing Techniques: Techniques like AVL trees or Red-Black trees can be used to keep the BST balanced.
Case Studies
- Database Systems: Many databases use BSTs for indexing to ensure quick data retrieval.
- Real-World Applications: Examples include search engines and data management systems where speed is critical.
Future Trends
- Emerging Technologies: As data grows, the need for efficient indexing will lead to innovations in tree structures.
- Integration with AI: Future databases may leverage AI to optimize tree structures for even faster access.
Binary Tree vs Binary Search Tree in File Systems
Role in File Systems
Binary trees and binary search trees (BSTs) are essential in organizing data within file systems. They help manage how files are stored and retrieved efficiently. Binary search trees are particularly useful for quick access to files.
Efficiency in File Retrieval
- Binary Trees:
- Binary Search Trees:
Impact on File Organization
- Binary Trees:
- Binary Search Trees:
Feature | Binary Tree | Binary Search Tree |
---|---|---|
Structure | Hierarchical | Ordered |
Search Time | O(n) (worst case) | O(log n) (average case) |
Use Case | General file storage | Quick file retrieval |
Balancing and Rebalancing
- Balancing: Ensures that the tree remains efficient for operations.
- Rebalancing: Necessary when files are added or removed to maintain performance.
In file systems, the choice between a binary tree and a binary search tree can significantly affect performance, especially as the number of files grows.
Binary Tree vs Binary Search Tree in Networking
Role in Networking
In networking, both binary trees and binary search trees (BSTs) can be used to manage data efficiently. Binary search trees are particularly useful for organizing routing tables and managing network connections. They allow for quick lookups and updates, which are essential for maintaining network performance.
Efficiency in Data Routing
- Binary Trees: These can be used for simple hierarchical data representation, such as organizational structures or basic routing paths.
- Binary Search Trees: They excel in scenarios where quick access to data is needed, such as finding the shortest path or managing dynamic routing tables.
- Comparison: BSTs generally provide faster search times compared to binary trees due to their ordered structure.
Impact on Network Performance
- Latency: Using BSTs can reduce latency in data retrieval, which is crucial for real-time applications.
- Scalability: As networks grow, BSTs can handle larger datasets more efficiently than binary trees.
- Load Balancing: BSTs can help in distributing network load evenly, improving overall performance.
In networking, the choice between a binary tree and a binary search tree can significantly affect the efficiency of data handling and routing operations.
Summary Table
Feature | Binary Tree | Binary Search Tree |
---|---|---|
Structure | Non-ordered | Ordered |
Search Efficiency | Slower | Faster |
Use Case | Simple hierarchies | Dynamic routing tables |
Binary Tree vs Binary Search Tree in Artificial Intelligence
Role in AI Algorithms
In artificial intelligence, both binary trees and binary search trees (BSTs) are used to manage data efficiently. Binary search trees are particularly useful for quick data retrieval, which is essential in AI applications like decision-making algorithms.
Efficiency in Data Processing
- Binary Trees: These structures can represent hierarchical data, making them suitable for tasks like parsing expressions or organizing decision trees.
- Binary Search Trees: They allow for faster search operations, which is crucial when dealing with large datasets in AI.
- Comparison: The efficiency of BSTs in searching is significantly higher than that of regular binary trees due to their ordered nature.
Impact on AI Performance
- Speed: BSTs can reduce the time complexity of search operations, which is vital for real-time AI applications.
- Scalability: As data grows, the performance of BSTs remains efficient, making them a preferred choice in AI systems.
- Flexibility: Both structures can be adapted for various AI tasks, but BSTs often provide better performance in search-heavy applications.
In AI, choosing the right data structure can greatly influence the performance and efficiency of algorithms. Understanding the difference between binary tree and binary search tree is essential for optimizing data handling in AI systems.
Binary Tree vs Binary Search Tree in Machine Learning
Role in ML Algorithms
Binary trees and binary search trees (BSTs) are important in machine learning. They help in organizing data efficiently. Binary search trees are particularly useful for quick data retrieval. This is because they allow for faster searching compared to regular binary trees.
Efficiency in Data Processing
- Binary Trees: Used for various tasks like decision trees, where each node represents a decision point.
- Binary Search Trees: Ideal for storing sorted data, making it easier to find and manage information.
- Performance: BSTs can significantly reduce the time needed for searching and sorting data.
Impact on ML Performance
- Speed: Using BSTs can speed up algorithms that require frequent data access.
- Scalability: As data grows, BSTs maintain efficiency better than binary trees.
- Flexibility: Both structures can adapt to different types of data and algorithms.
In machine learning, choosing the right data structure can greatly influence the performance of algorithms and the efficiency of data handling.
Summary
In summary, while both binary trees and binary search trees have their uses in machine learning, BSTs are often preferred for their efficiency in searching and sorting data. Understanding their differences can help in selecting the right structure for specific tasks.
Binary Tree vs Binary Search Tree in Game Development
Role in Game Algorithms
In game development, binary trees and binary search trees (BSTs) are essential for organizing data efficiently. They help manage various game elements, such as characters, items, and levels. Here are some key roles they play:
- Game State Management: Trees can represent different states of a game, allowing for quick transitions.
- AI Decision Making: Binary trees can help AI make decisions based on player actions.
- Scene Graphs: They can structure the game world, making it easier to render and manage objects.
Efficiency in Game Logic
Using binary search trees can significantly improve the efficiency of game logic. Here’s how:
- Fast Searching: BSTs allow for quick lookups of game objects, which is crucial during gameplay.
- Dynamic Updates: They can handle frequent changes in game state, like adding or removing items.
- Sorted Data: BSTs keep data sorted, making it easier to manage inventories or leaderboards.
Impact on Game Performance
The choice between a binary tree and a binary search tree can affect game performance. Here are some considerations:
- Traversal Speed: BSTs generally offer faster traversal times compared to binary trees.
- Memory Usage: Binary trees may use more memory if not balanced, while BSTs can be more efficient.
- Complexity: Implementing a balanced BST can be more complex but offers better performance in the long run.
In game development, choosing the right data structure can greatly influence the game’s performance and user experience. Understanding the differences between binary trees and binary search trees is crucial for developers.
Case Studies
Several games have successfully implemented these structures:
- Strategy Games: Use binary trees for managing game states and AI decisions.
- RPGs: Employ BSTs for inventory management and quick item retrieval.
- Simulation Games: Utilize trees for organizing complex interactions between game elements.
Future Trends
As game development evolves, the use of trees will likely expand. Innovations may include:
- Hybrid Structures: Combining binary trees with other data structures for enhanced performance.
- AI Integration: Using trees to improve AI decision-making processes in real-time.
- Procedural Generation: Leveraging trees for creating dynamic game worlds and levels.
Binary Tree vs Binary Search Tree in Web Development
Role in Web Algorithms
In web development, both binary trees and binary search trees (BSTs) are essential for organizing data efficiently. They help in managing various data structures that are crucial for web applications. Here are some roles they play:
- Data organization: They help in structuring data for quick access.
- Search optimization: BSTs allow for faster search operations compared to regular binary trees.
- Dynamic data handling: Both structures can adapt to changing data needs in web applications.
Efficiency in Data Handling
When it comes to handling data, the efficiency of these trees can significantly impact performance. Here’s a quick comparison:
Feature | Binary Tree | Binary Search Tree |
---|---|---|
Search Time | O(n) | O(log n) |
Insertion Time | O(n) | O(log n) |
Deletion Time | O(n) | O(log n) |
Impact on Web Performance
The choice between a binary tree and a binary search tree can affect the performance of web applications. Here are some considerations:
- Scalability: BSTs are generally more scalable due to their efficient search capabilities.
- Complexity: Implementing a BST can be more complex than a binary tree, but the benefits often outweigh the challenges.
- Use Cases: For applications requiring frequent searches, BSTs are preferred, while binary trees may suffice for simpler tasks.
In web development, choosing the right tree structure can lead to better performance and user experience. Understanding the differences between binary trees and binary search trees is crucial for effective data management.
Balancing and Rebalancing
Maintaining balance in these trees is vital for performance. Here are some techniques:
- AVL Trees: A type of self-balancing BST that maintains height balance.
- Red-Black Trees: Another self-balancing BST that ensures the tree remains approximately balanced.
- Splay Trees: A self-adjusting binary search tree that moves frequently accessed elements closer to the root.
By understanding these concepts, web developers can make informed decisions about data structures, leading to more efficient applications.
Binary Tree vs Binary Search Tree in Cybersecurity
Role in Security Algorithms
Binary trees and binary search trees (BSTs) play important roles in security algorithms. They help in organizing data efficiently, which is crucial for quick access and processing. Here are some key points:
- Data organization: Both structures help in storing data in a way that makes it easy to retrieve.
- Search efficiency: BSTs allow for faster searches, which is vital in security applications where time is critical.
- Data integrity: They help maintain the integrity of data by ensuring that it is organized correctly.
Efficiency in Threat Detection
Using binary trees and BSTs can enhance threat detection systems. Here’s how:
- Quick lookups: BSTs can quickly find potential threats by searching through organized data.
- Efficient updates: Adding or removing data is faster in BSTs, which is important for real-time threat monitoring.
- Scalability: Both structures can handle large amounts of data, making them suitable for growing security needs.
Impact on Security Performance
The choice between binary trees and BSTs can significantly affect security performance. Consider the following:
- Speed: BSTs generally provide faster access times compared to binary trees.
- Complexity: The complexity of operations in BSTs is lower, which can lead to better performance in security applications.
- Resource usage: Efficient use of memory and processing power is crucial in cybersecurity, and BSTs often excel in this area.
In cybersecurity, the choice of data structure can greatly influence the effectiveness of security measures. Binary search trees are often preferred for their efficiency in searching and updating data, which is essential for real-time threat detection and response.
Case Studies
Several case studies highlight the effectiveness of binary trees and BSTs in cybersecurity:
- Intrusion detection systems: Many systems use BSTs to quickly identify and respond to threats.
- Firewall management: Binary trees help in organizing rules and policies for efficient processing.
- Data encryption: Both structures can be used to manage keys and encryption algorithms effectively.
Future Trends
As cybersecurity threats evolve, the use of binary trees and BSTs will likely continue to grow. Future trends may include:
- Integration with AI: Combining these structures with AI for smarter threat detection.
- Enhanced algorithms: Developing new algorithms that leverage the strengths of both data structures.
- Real-time processing: Improving the speed and efficiency of data handling in security applications.
Future Directions for Binary Tree and Binary Search Tree
Emerging Trends
- Increased Use of AI: As artificial intelligence grows, binary trees and binary search trees will be essential for organizing data efficiently.
- Integration with Quantum Computing: New algorithms may emerge that utilize tree structures for faster data processing.
- Enhanced Balancing Techniques: Future research may focus on improving balancing methods to optimize performance.
Innovations in Tree Structures
- Self-Balancing Trees: New types of trees that automatically adjust themselves during insertions and deletions.
- Hybrid Structures: Combining features of binary trees and other data structures for better performance.
- Dynamic Trees: Trees that can change shape based on the data being processed, improving efficiency.
Impact of Quantum Computing
- Faster Search Algorithms: Quantum algorithms could revolutionize how we search through binary search trees.
- New Data Structures: Quantum computing may lead to entirely new types of tree structures that outperform classical ones.
- Complex Problem Solving: Trees may play a crucial role in solving complex problems more efficiently.
Integration with AI
- Machine Learning Applications: Trees will be vital in organizing data for machine learning models.
- Data Processing Efficiency: Improved algorithms will enhance how trees manage and process large datasets.
- AI-Driven Optimization: AI could help in dynamically optimizing tree structures based on usage patterns.
Scalability Challenges
- Handling Large Datasets: Future trees must efficiently manage and scale with increasing data sizes.
- Distributed Systems: Trees may need to adapt for use in distributed computing environments.
- Performance Under Load: Research will focus on maintaining performance as data loads increase.
Future Research Areas
- Algorithm Development: New algorithms for insertion, deletion, and searching in trees.
- Memory Management: Techniques to optimize memory usage in tree structures.
- Real-Time Applications: Exploring how trees can be used in real-time data processing scenarios.
In summary, the future of binary trees and binary search trees is bright, with numerous opportunities for innovation and improvement.
As we look ahead, the future of binary trees and binary search trees is bright and full of possibilities. These data structures will continue to evolve, offering new ways to solve complex problems efficiently. If you’re eager to enhance your coding skills and prepare for your dream job, visit our website today!
Conclusion
In summary, understanding the differences between a binary tree and a binary search tree is essential for anyone studying data structures. A binary tree is a simple structure where each node can have up to two children, without any specific order for the values. On the other hand, a binary search tree organizes its nodes in a way that makes searching for values much faster. In a BST, the left child is always smaller than the parent, and the right child is always larger. This organization allows for quicker insertions, deletions, and searches. Knowing these differences can help you choose the right structure for your coding projects.
Frequently Asked Questions
What is a binary tree?
A binary tree is a type of data structure where each node can have up to two children. These children are called the left and right child.
What is a binary search tree (BST)?
A binary search tree is a special kind of binary tree where the left child has a smaller value than the parent node, and the right child has a larger value.
How do binary trees and binary search trees differ?
The main difference is that binary trees can have any arrangement of values, while binary search trees must follow a specific order.
What are the uses of binary trees?
Binary trees are used in various applications like expression trees, decision trees, and hierarchical data representation.
Why are binary search trees useful?
Binary search trees allow for faster searching, adding, and removing of items because of their ordered structure.
Can binary trees have duplicate values?
Yes, binary trees can have duplicate values, while binary search trees usually do not allow duplicates.
What is tree traversal?
Tree traversal is the process of visiting all the nodes in a tree, and there are several methods, including in-order, pre-order, and post-order.
What is the height of a binary tree?
The height of a binary tree is the number of edges in the longest path from the root to a leaf.
How do you insert a node in a binary search tree?
To insert a node in a binary search tree, you compare the value with the current node and place it in the left or right subtree based on its value.
What are some common types of binary trees?
Common types include full binary trees, complete binary trees, and perfect binary trees.
How do you delete a node from a binary search tree?
To delete a node, you find it first, then adjust the tree to maintain its order, which may involve replacing it with its in-order successor.
What are the advantages of using binary search trees?
Binary search trees offer efficient searching, inserting, and deleting operations, making them ideal for many applications.