Top Instacart Interview Questions: Ace Your Technical Interview
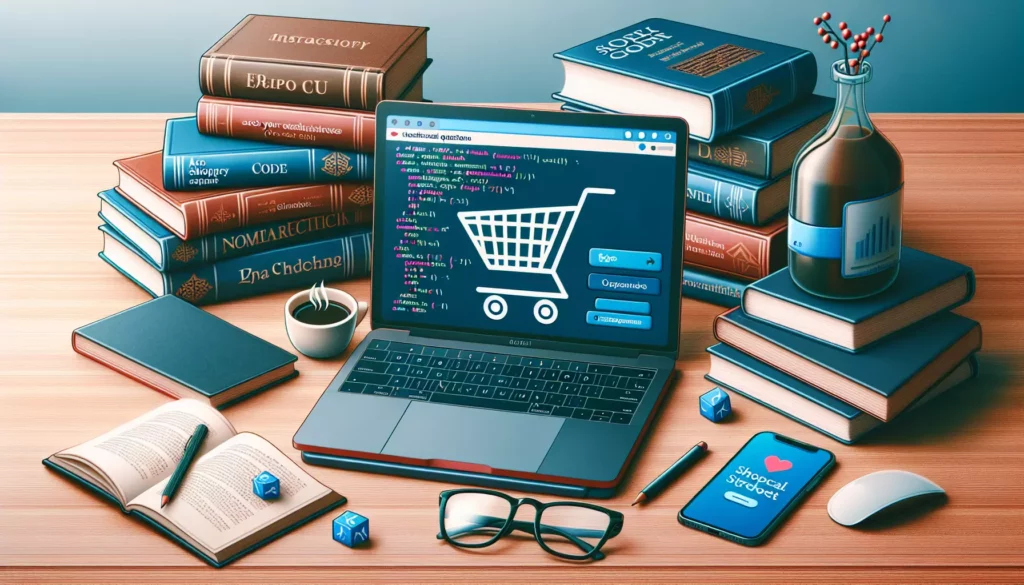
Are you preparing for an interview with Instacart? You’ve come to the right place! In this comprehensive guide, we’ll explore some of the most common Instacart interview questions, provide detailed explanations, and offer tips to help you succeed in your technical interview. Whether you’re a seasoned developer or just starting your journey in the tech world, this article will equip you with the knowledge and confidence you need to tackle Instacart’s interview process.
Table of Contents
- Introduction to Instacart Interviews
- Coding Questions
- System Design Questions
- Behavioral Questions
- Interview Tips and Strategies
- Preparation Resources
- Conclusion
1. Introduction to Instacart Interviews
Instacart, the leading online grocery delivery platform, is known for its rigorous interview process. The company seeks talented individuals who can contribute to its innovative technology stack and help solve complex problems in the e-commerce and logistics space. Instacart’s interview process typically consists of several rounds, including:
- Phone screen with a recruiter
- Technical phone interview
- On-site interviews (or virtual on-site interviews)
The technical interviews at Instacart focus on assessing your problem-solving skills, coding abilities, system design knowledge, and cultural fit. Let’s dive into the types of questions you might encounter during your Instacart interview.
2. Coding Questions
Coding questions are a crucial part of the Instacart interview process. These questions are designed to evaluate your ability to write clean, efficient, and scalable code. Here are some common coding questions you might encounter:
2.1. Two Sum
Question: Given an array of integers and a target sum, return the indices of two numbers in the array that add up to the target sum.
Example:
Input: nums = [2, 7, 11, 15], target = 9
Output: [0, 1]
Explanation: nums[0] + nums[1] = 2 + 7 = 9
Solution:
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
Explanation: This solution uses a hash map to store the numbers and their indices. We iterate through the array once, checking if the complement (target – current number) exists in the hash map. If it does, we’ve found our pair. If not, we add the current number and its index to the hash map. This approach has a time complexity of O(n) and a space complexity of O(n).
2.2. Merge Intervals
Question: Given an array of intervals where intervals[i] = [starti, endi], merge all overlapping intervals, and return an array of the non-overlapping intervals that cover all the intervals in the input.
Example:
Input: intervals = [[1,3],[2,6],[8,10],[15,18]]
Output: [[1,6],[8,10],[15,18]]
Explanation: Since intervals [1,3] and [2,6] overlap, merge them into [1,6].
Solution:
def merge_intervals(intervals):
if not intervals:
return []
# Sort intervals based on start time
intervals.sort(key=lambda x: x[0])
merged = [intervals[0]]
for interval in intervals[1:]:
if interval[0] <= merged[-1][1]:
merged[-1][1] = max(merged[-1][1], interval[1])
else:
merged.append(interval)
return merged
Explanation: This solution first sorts the intervals based on their start times. Then, we iterate through the sorted intervals, merging overlapping intervals as we go. If the current interval’s start time is less than or equal to the end time of the last merged interval, we update the end time of the last merged interval. Otherwise, we add the current interval to the merged list. This approach has a time complexity of O(n log n) due to the sorting step, and a space complexity of O(n) to store the merged intervals.
2.3. LRU Cache
Question: Design and implement a data structure for a Least Recently Used (LRU) cache. It should support the following operations: get and put.
- get(key) – Get the value (will always be positive) of the key if the key exists in the cache, otherwise return -1.
- put(key, value) – Set or insert the value if the key is not already present. When the cache reaches its capacity, it should invalidate the least recently used item before inserting a new item.
The cache is initialized with a positive capacity.
Example:
LRUCache cache = new LRUCache(2);
cache.put(1, 1);
cache.put(2, 2);
cache.get(1); // returns 1
cache.put(3, 3); // evicts key 2
cache.get(2); // returns -1 (not found)
cache.put(4, 4); // evicts key 1
cache.get(1); // returns -1 (not found)
cache.get(3); // returns 3
cache.get(4); // returns 4
Solution:
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity: int):
self.capacity = capacity
self.cache = OrderedDict()
def get(self, key: int) -> int:
if key not in self.cache:
return -1
self.cache.move_to_end(key)
return self.cache[key]
def put(self, key: int, value: int) -> None:
if key in self.cache:
self.cache.move_to_end(key)
self.cache[key] = value
if len(self.cache) > self.capacity:
self.cache.popitem(last=False)
Explanation: This solution uses Python’s OrderedDict, which maintains the order of insertion. When we get or update a key, we move it to the end of the OrderedDict, making it the most recently used item. When we need to evict an item, we remove the first item (least recently used) from the OrderedDict. This implementation provides O(1) time complexity for both get and put operations.
3. System Design Questions
System design questions are an essential part of the Instacart interview process, especially for senior-level positions. These questions assess your ability to design scalable, reliable, and efficient systems. Here are some system design questions you might encounter:
3.1. Design Instacart’s Order Fulfillment System
Question: Design a system that can handle Instacart’s order fulfillment process, from when a customer places an order to when it’s delivered.
Key Considerations:
- How to handle real-time inventory updates across multiple stores
- Efficient assignment of orders to shoppers
- Handling peak load during busy hours
- Ensuring data consistency and fault tolerance
High-level Design:
- Order Placement Service: Handles customer orders and validates them against inventory.
- Inventory Management System: Keeps track of real-time inventory across all partner stores.
- Shopper Assignment Service: Assigns orders to available shoppers based on location, order size, and shopper ratings.
- Routing Service: Provides optimal routes for shoppers to pick items and deliver orders.
- Notification Service: Sends updates to customers about their order status.
- Payment Processing Service: Handles secure payment transactions.
Technologies to Consider:
- Distributed database (e.g., Cassandra) for storing order and inventory data
- Message queue (e.g., Apache Kafka) for handling real-time updates
- Caching layer (e.g., Redis) for frequently accessed data
- Load balancers and auto-scaling for handling traffic spikes
- Microservices architecture for scalability and maintainability
3.2. Design a Real-time Analytics Dashboard
Question: Design a real-time analytics dashboard for Instacart that displays key metrics such as active orders, shopper performance, and customer satisfaction scores.
Key Considerations:
- Handling large volumes of real-time data
- Efficient data aggregation and processing
- Low-latency updates to the dashboard
- Scalability to support a growing number of users and data points
High-level Design:
- Data Ingestion Layer: Collects data from various services (orders, shoppers, customers) in real-time.
- Stream Processing Layer: Processes and aggregates incoming data streams.
- Data Storage Layer: Stores processed data for historical analysis and backup.
- Query Layer: Handles requests for specific metrics and time ranges.
- Visualization Layer: Presents data in an interactive and user-friendly format.
Technologies to Consider:
- Apache Kafka or Amazon Kinesis for data ingestion
- Apache Flink or Apache Spark for stream processing
- InfluxDB or Prometheus for time-series data storage
- Elasticsearch for fast querying of aggregated data
- Grafana or Tableau for data visualization
4. Behavioral Questions
Behavioral questions are an important part of the Instacart interview process. They help the interviewer assess your soft skills, problem-solving abilities, and cultural fit. Here are some common behavioral questions you might encounter:
4.1. Tell me about a time when you had to deal with a difficult team member.
Tips for answering:
- Use the STAR method (Situation, Task, Action, Result) to structure your response
- Focus on how you handled the situation professionally and constructively
- Highlight any lessons learned or personal growth from the experience
4.2. Describe a project where you had to meet a tight deadline. How did you manage it?
Tips for answering:
- Explain the project’s importance and the challenges you faced
- Discuss your time management and prioritization strategies
- Highlight any innovative solutions or processes you implemented
- Mention the outcome and any positive feedback received
4.3. Give an example of a time when you had to make a difficult decision with limited information.
Tips for answering:
- Describe the context and why the decision was important
- Explain your thought process and how you gathered available information
- Discuss any risks you considered and how you mitigated them
- Share the outcome and any lessons learned
4.4. Tell me about a time when you received constructive criticism. How did you respond?
Tips for answering:
- Show that you’re open to feedback and committed to personal growth
- Describe how you implemented the feedback to improve your performance
- Highlight any positive outcomes that resulted from the changes you made
5. Interview Tips and Strategies
To increase your chances of success in your Instacart interview, consider the following tips and strategies:
5.1. Prepare thoroughly
- Review fundamental data structures and algorithms
- Practice coding problems on platforms like LeetCode, HackerRank, or AlgoCademy
- Study system design principles and common architectures
- Research Instacart’s business model, technology stack, and recent news
5.2. Communicate effectively
- Think out loud during coding and system design questions
- Ask clarifying questions when needed
- Explain your thought process and reasoning behind your decisions
- Be open to feedback and suggestions from the interviewer
5.3. Manage your time wisely
- Allocate your time effectively between different parts of the interview
- If you’re stuck on a problem, consider asking for a hint or moving on to the next question
- For system design questions, spend time understanding requirements before diving into the solution
5.4. Show enthusiasm and cultural fit
- Demonstrate your passion for technology and problem-solving
- Show interest in Instacart’s mission and challenges
- Ask thoughtful questions about the company, team, and role
5.5. Practice mock interviews
- Conduct mock interviews with friends, mentors, or online platforms
- Simulate realistic interview conditions, including time constraints
- Seek feedback on your performance and areas for improvement
6. Preparation Resources
To help you prepare for your Instacart interview, consider using the following resources:
6.1. Online Coding Platforms
- LeetCode: Offers a wide range of coding problems, including many that are frequently asked in technical interviews.
- HackerRank: Provides coding challenges and competitions to help you improve your skills.
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance for learning algorithms and data structures.
6.2. System Design Resources
- System Design Primer: A comprehensive GitHub repository covering system design concepts and examples.
- Grokking the System Design Interview: An online course that covers various system design scenarios and best practices.
6.3. Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell: A classic resource for technical interview preparation.
- “Designing Data-Intensive Applications” by Martin Kleppmann: An excellent book for understanding distributed systems and data processing at scale.
6.4. Mock Interview Platforms
- Pramp: Offers free peer-to-peer mock interviews for both coding and system design questions.
- interviewing.io: Provides anonymous technical mock interviews with experienced engineers.
7. Conclusion
Preparing for an Instacart interview can be challenging, but with the right approach and resources, you can significantly increase your chances of success. By focusing on coding skills, system design knowledge, and behavioral competencies, you’ll be well-equipped to tackle the various aspects of the interview process.
Remember that the key to success lies in consistent practice, thorough preparation, and effective communication during the interview. Leverage the resources mentioned in this article, and don’t hesitate to seek additional help or clarification when needed.
As you prepare, keep in mind that Instacart is looking for candidates who are not only technically proficient but also passionate about solving complex problems in the e-commerce and logistics space. Showcase your enthusiasm for the company’s mission and your ability to contribute to its innovative technology stack.
Good luck with your Instacart interview! With dedication and the right preparation, you’ll be well on your way to joining one of the leading companies in the online grocery delivery industry.