The Ultimate Guide to Preparing for Technical Coding Interviews
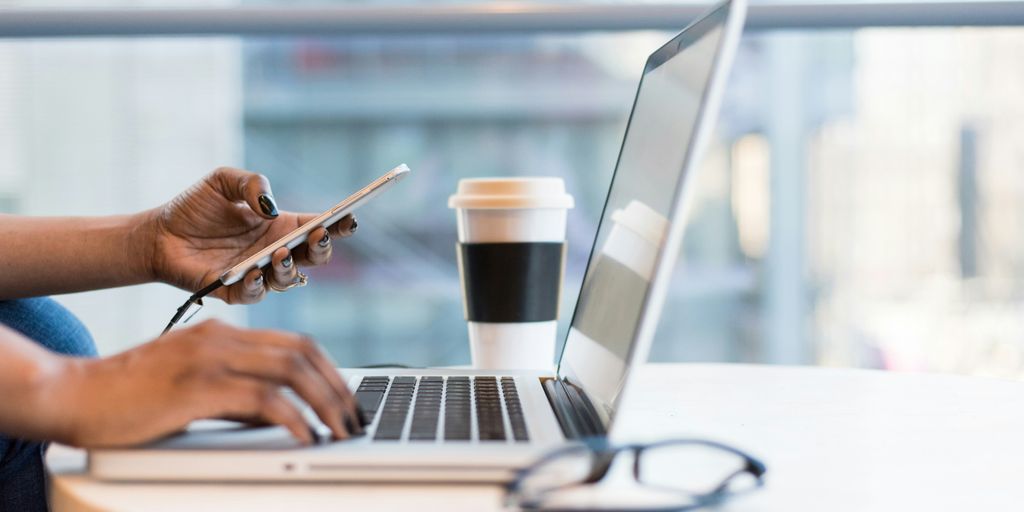
Technical coding interviews can be pretty tough. They test your coding skills, problem-solving abilities, and how you handle pressure. But with the right preparation, you can ace them. This guide will help you get ready for your technical coding interviews, covering everything from understanding the process to mastering key skills.
Key Takeaways
- Understand the stages and formats of technical interviews so you know what to expect.
- Master essential data structures and algorithms to solve coding problems effectively.
- Practice coding problems regularly on top platforms to build confidence and improve speed.
- Develop strong problem-solving skills by breaking down complex problems and thinking aloud during interviews.
- Prepare for behavioral questions and build a strong technical portfolio to showcase your skills and experiences.
Understanding the Technical Interview Process
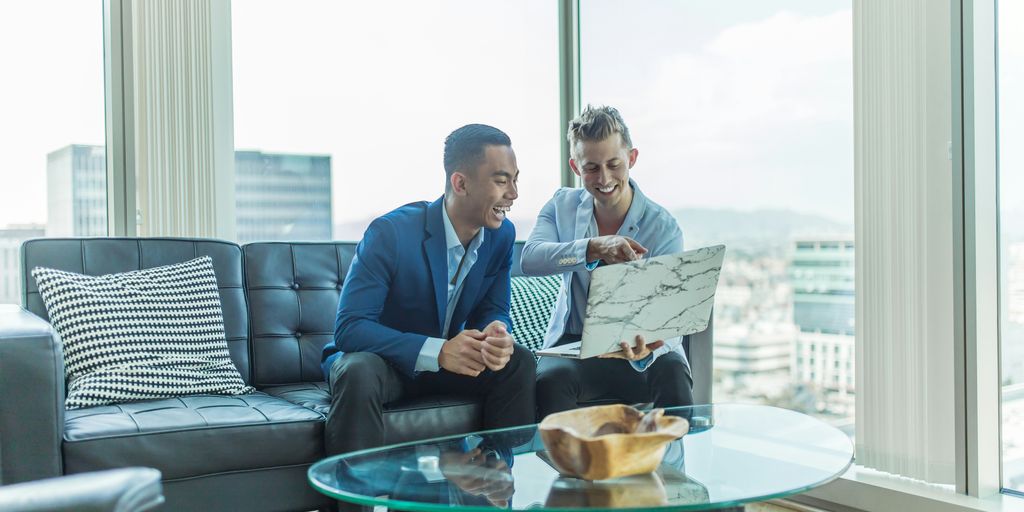
Stages of a Technical Interview
Technical interviews often come in three main stages:
- Technical Screening Interview: This is usually a short phone or video call to see if you are qualified and excited about the job. It typically lasts 15-30 minutes.
- Remote Coding Interview/Assignment: Some companies test your coding skills with a preliminary test. This can be done over the phone, via video call, or as a take-home assignment.
- Onsite Interview/Whiteboarding Challenge: This is the main technical interview. You will solve coding problems on a whiteboard in front of interviewers. This stage can last from a few hours to a full day.
What Interviewers Look For
Interviewers want to see how you tackle real-world problems. They are not trying to trick you with impossible questions. Instead, they want to see your problem-solving skills and how you think through challenges.
Tip: Slow down and ask clarifying questions before you start solving the problem. This shows that you are thoughtful and understand the challenge fully.
Common Interview Formats
There are several types of technical interviews you might face:
- Take-Home Challenge: You will be given requirements and need to code a solution. This could be a small front-end application or a simple REST API.
- Automated Coding Interview: This is a coding test conducted through a web application.
- Live Coding Interview: You will solve coding problems in real-time, often while sharing your screen with the interviewer.
- System Design Interview: You will be asked to design a system or architecture to solve a specific problem.
Each company may have a different process, but these are the common formats you might encounter.
Mastering Data Structures and Algorithms
Essential Data Structures
Understanding data structures is crucial for technical interviews. Data structures are ways to organize information in a program, impacting its performance. Key data structures to know include:
- Arrays
- Linked Lists
- Stacks
- Queues
- Hash Tables
- Trees (Binary Trees, Binary Search Trees)
- Heaps
- Graphs
Key Algorithms to Know
Algorithms are the steps a program takes to solve problems. Knowing these algorithms is essential:
- Sorting (e.g., Merge Sort, Quick Sort)
- Searching (e.g., Binary Search)
- Dynamic Programming
- Backtracking
- Greedy Algorithms
- Graph Algorithms (e.g., Dijkstra’s, A*)
The coding interview is a fight with yourself, and the single way to win it is to practice a lot.
Resources for Learning
There are many resources to help you master data structures and algorithms. Some recommended ones include:
- AlgoMonster: Helps you master coding interview questions with easy-to-understand guides.
- Grokking the Coding Interview: Focuses on question patterns to help you understand and solve new problems.
- JudoCoder: Offers coding challenges and question-answer sessions to strengthen your skills.
Remember, the more you practice, the better you’ll get. Start practicing today and increase your comfort zone with these topics.
Practicing Coding Problems
Top Platforms for Practice
To crack any coding interview, you need to practice regularly. Here are some top platforms to help you:
- LeetCode: Offers a wide range of problems sorted by difficulty.
- HackerRank: Great for beginners and offers a variety of challenges.
- CodeSignal: Provides a mix of coding tasks and interview practice.
- AlgoExpert: Focuses on coding interview questions with detailed solutions.
Types of Coding Problems
Coding problems can be categorized into several types:
- Array and String Manipulation: Common and fundamental problems.
- Dynamic Programming: Often seen as challenging but essential to master.
- Graph Algorithms: Important for understanding complex data structures.
- Sorting and Searching: Basic yet crucial for many coding tasks.
Time Management During Practice
Managing your time effectively is key to success. Here are some tips:
- Set a Schedule: Dedicate specific hours each day to practice.
- Use a Timer: Simulate real interview conditions by timing yourself.
- Review and Reflect: After solving a problem, review your solution and think about improvements.
Consistent practice and time management are crucial for success in coding interviews. Start early and stay disciplined.
Developing Problem-Solving Skills
Breaking Down Complex Problems
When faced with a tough problem, the first step is to break it down into smaller, more manageable parts. This makes it easier to understand and solve. Start by identifying the main components and then tackle each one individually. This method not only simplifies the problem but also helps in finding a solution more efficiently.
Thinking Aloud During Interviews
During an interview, it’s crucial to talk through your thought process. This gives the interviewer insight into how you approach problems. Don’t be afraid to share your initial thoughts, even if they aren’t perfect. The key is to keep the conversation going and show that you are actively working towards a solution. Remember, the more you practice, the faster you will find optimal solutions.
Common Problem-Solving Techniques
Here are some common techniques to help you solve problems effectively:
- Divide and Conquer: Break the problem into smaller parts and solve each one individually.
- Pattern Recognition: Look for patterns or similarities to problems you have solved before.
- Algorithm Design: Think about the steps involved in problem solving, such as analyzing the problem, developing the algorithm, coding, and testing.
- Simplify the Problem: If you’re stuck, try solving a simpler version of the problem first.
Keep in mind to talk aloud and explain what you are thinking.
By using these techniques, you can approach problems in a structured and systematic way, making it easier to find solutions.
Preparing for Behavioral Questions
Behavioral questions are a key part of technical interviews. They help interviewers understand how you think, solve problems, and interact with others. Here’s how to get ready for them.
Common Behavioral Questions
Interviewers often ask questions to gauge your soft skills and how you handle various situations. Some common questions include:
- How do you handle disagreements, for example, in a code review?
- Tell me about a time when you identified a critical bug in the codebase. How did you go about it?
- Give me an example of when you missed an important deadline. What did you do about it, and how did it go?
Crafting Your Responses
To answer behavioral questions effectively, prepare some real-life examples and stories ahead of time. This can help you adapt to nearly any question. For instance:
- Think of an accomplishment you’re proud of. This can be used for questions like, "Describe a time you went above and beyond at work" or "What’s your proudest accomplishment?"
- Have an example of a time you made a mistake at work. This can be used for questions like, "Tell me about a time you made a mistake at work" or "Give me an example of a time you overcame a challenge."
Using the STAR Method
The STAR method is a great way to structure your answers. STAR stands for Situation, Task, Action, and Result. Here’s how to use it:
- Situation: Describe the context within which you performed a task or faced a challenge at work.
- Task: Explain the actual task or challenge that was involved.
- Action: Describe the specific actions you took to address the task or challenge.
- Result: Share the outcomes or results of your actions.
Preparing for behavioral questions can make the interview more comfortable and less stressful. Practice your responses and you’ll be ready for anything.
By following these tips, you’ll be well-prepared to handle any behavioral question that comes your way.
Building a Strong Technical Portfolio
Showcasing Projects
To build a strong technical portfolio, start by showcasing your best projects. Highlight projects that demonstrate your skills and the impact you had. Make sure to include a variety of projects to show your versatility.
- Select diverse projects: Include different types of projects like web apps, mobile apps, and backend services.
- Detail your role: Clearly explain what you did in each project. Were you the lead developer, or did you focus on a specific part?
- Show the impact: Mention any metrics or outcomes that show the success of your project.
Highlighting Relevant Experience
Your portfolio should also highlight your relevant experience. This includes internships, part-time jobs, and any freelance work.
- List your experiences: Include the companies you worked for, your job titles, and the duration of your employment.
- Describe your responsibilities: Be specific about what you did in each role. This helps interviewers understand your skills and expertise.
- Mention technologies used: List the programming languages, frameworks, and tools you used in each job.
Creating an Online Portfolio
An online portfolio is a great way to make your work accessible to potential employers. It should be easy to navigate and visually appealing.
- Choose a platform: Use platforms like GitHub, GitLab, or personal websites to host your portfolio.
- Organize your projects: Make it easy for visitors to find and explore your work. Use categories and tags to organize your projects.
- Proofread and revise: Now that your software engineer portfolio is complete, proofread and revise it. This ensures that everything you wrote is accurate and free of errors.
A well-crafted portfolio can set you apart from other candidates and give you a competitive edge in the job market.
Mock Interviews and Feedback
Mock interviews are a crucial part of preparing for technical coding interviews. They help you get used to the interview format and reduce anxiety. Practicing with mock interviews can significantly boost your confidence and performance.
Technical Interview Etiquette
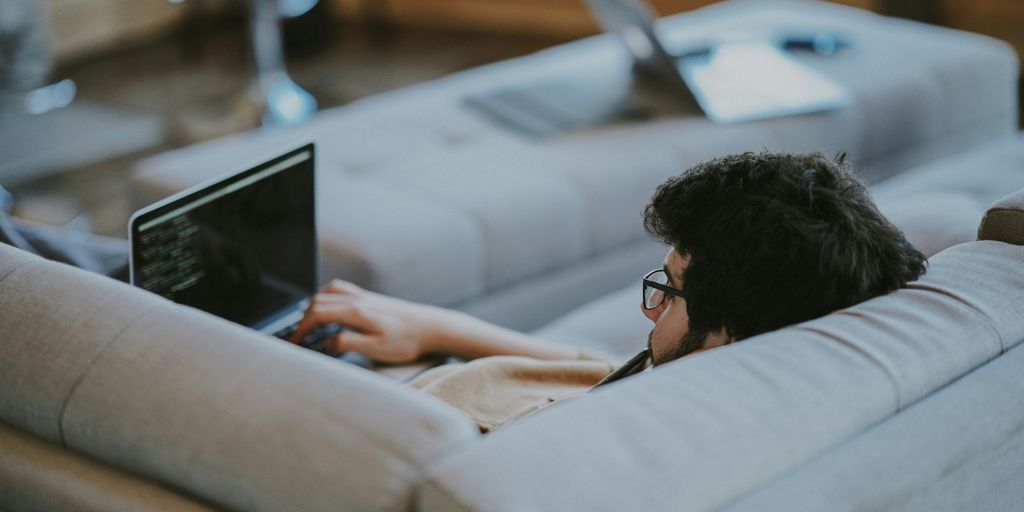
Communication Skills
Effective communication is key during a technical interview. Clearly explain your thought process and reasoning as you work through problems. This not only shows your problem-solving skills but also helps the interviewer understand your approach. Remember, an interview is not just about answering questions correctly but also about having a conversation. Ask good questions, laugh, and make jokes at appropriate times to create a positive impression.
Handling Mistakes Gracefully
Everyone makes mistakes, and interviewers know this. If you make an error, don’t panic. Instead, acknowledge it, correct it, and move on. This shows that you can handle setbacks and learn from them. Jumping into the code without taking a moment to think it through can lead to avoidable mistakes. Take a few minutes to question your assumptions and make a game plan first.
Follow-Up Etiquette
After the interview, it’s important to follow up. Send a short and sweet thank you email to the recruiter or hiring manager within 24 hours. This shows your appreciation and keeps you on their radar. If you haven’t heard back after a week, send a brief follow-up email reiterating your interest in the role and asking about potential next steps.
By considering your “soft” interview skills and choosing your words carefully, you can avoid misunderstandings and make a positive impression.
Utilizing Study Resources
Preparing for technical coding interviews can be daunting, but the right resources can make a significant difference. Here are some essential study resources to help you ace your interview.
Recommended Books
Books are a great way to build a strong foundation in coding and algorithms. Some highly recommended titles include:
- Cracking the Coding Interview by Gayle Laakmann McDowell
- Introduction to Algorithms by Thomas H. Cormen
- Elements of Programming Interviews by Adnan Aziz
These books cover a wide range of topics and provide numerous practice problems to help you prepare.
Online Courses and Tutorials
Online courses offer structured learning paths and interactive content. Fortunately, there are already excellent coding interview preparation resources available. Some top platforms include:
- AlgoMonster
- Grokking the Coding Interview: Patterns for Coding Questions
- Coursera and edX courses on data structures and algorithms
These courses often include video lectures, quizzes, and coding exercises to reinforce your learning.
Cheat Sheets and Study Guides
Cheat sheets are handy for quick revisions and summarizing key concepts. They can help you internalize the must-dos and must-remembers for coding interviews. Some useful cheat sheets include:
- Coding interview techniques: how to find a solution and optimize your approach
- Algorithms study cheatsheets: covers the best learning resources, must-remember tips, and practice questions for every data structure and algorithm
Using these guides ensures that you cultivate good habits and muscle memory with regards to interviews right from the beginning.
By leveraging these resources, you can systematically prepare for your technical coding interviews and increase your chances of success.
Managing Interview Anxiety
Techniques for Staying Calm
Feeling nervous before an interview is normal, but there are ways to manage it. Practice is the key to feeling more confident. The more you practice, the more comfortable you’ll become. Try mock interviews to simulate the real experience. Also, deep breathing exercises can help calm your nerves.
Mental Preparation Strategies
Prepare mentally by visualizing a successful interview. Imagine yourself answering questions confidently and connecting with the interviewer. Reflect on your past achievements to boost your self-esteem. Remember, don’t make assumptions about the outcome; focus on doing your best.
Physical Well-being Tips
Taking care of your body can also help reduce anxiety. Ensure you get enough sleep the night before the interview. Eat a healthy meal to keep your energy levels up. Avoid too much caffeine as it can increase anxiety. A short walk or light exercise can also help clear your mind and reduce stress.
Remember, the goal is to be in the best possible state, both mentally and physically, to handle the interview with confidence.
Understanding Company-Specific Interviews
Researching the Company
Before you go for an interview, it’s important to research the company. Look into their mission, vision, and values. This will help you understand what they are looking for in a candidate. Check out their tech blog or Glassdoor page for insights into their interview process. Knowing the types of questions they ask can give you a big advantage.
Tailoring Your Preparation
Every company has a different interview process. Some might have multiple rounds, including technical and behavioral interviews. Tailor your preparation to match the company’s style. For example, Google has a mix of phone and onsite interviews, while Facebook might have a full-day onsite interview. Adjust your study plan accordingly.
Understanding Company Culture
Understanding the company’s culture is crucial. Companies want to see if you are a good fit for their team. Show your excitement about the company and be professional. Use stories from your past experiences to demonstrate your skills and how you handle conflicts. This will help you stand out from other candidates.
Remember, each company is unique. Tailor your preparation to fit their specific needs and culture. This will increase your chances of success.
Post-Interview Strategies
Analyzing Your Performance
After the technical interview, take some time to reflect on how you did. Think about what went well and what didn’t. This will help you improve for future interviews. Write down any questions you found difficult and research the answers. This way, you’ll be better prepared next time.
Following Up with Interviewers
It’s important to follow up after your interview. Send a thank-you email to the recruiter or hiring manager within 24 hours. Mention something specific from the interview to make it personal. If you haven’t heard back in a week, send a brief follow-up email to show your continued interest in the role.
Continuing Your Preparation
Even after the interview, keep preparing. You never know when the next opportunity will come. Keep practicing coding problems and revising key concepts. This will keep your skills sharp and ready for the next interview.
Remember, every interview is a learning experience. Use each one to get better and more confident.
- Reflect on your performance
- Follow up with a thank-you email
- Keep preparing for future opportunities
After your interview, it’s crucial to follow up and reflect on your performance. This can help you identify areas for improvement and prepare better for future opportunities. For more tips and strategies to ace your next interview, visit our website and explore our resources.
Conclusion
Preparing for a technical coding interview can be challenging, but with the right approach, you can succeed. Remember to practice regularly, understand the key concepts, and get comfortable with the interview format. Use the resources available to you, like online courses, books, and mock interviews, to build your confidence. Stay calm, be yourself, and show your passion for coding. With dedication and preparation, you’ll be ready to ace your technical interview and land your dream job. Good luck!
Frequently Asked Questions
What is a technical interview?
A technical interview is a special kind of job interview that tests your coding skills, problem-solving abilities, and personality. You usually have to solve coding problems, sometimes on a whiteboard or computer, to show that you have the skills needed for the job.
How should I prepare for a technical phone interview?
To prepare for a technical phone interview, practice coding problems, review key data structures and algorithms, and be ready to explain your thought process clearly. Make sure you have a quiet place to take the call and a good internet connection.
What should I do the day before my tech interview?
The day before your tech interview, review your notes, get a good night’s sleep, and make sure you have everything you need, like a notebook, pen, and a charged laptop. Try to relax and stay calm.
What should I wear to a technical interview?
For a technical interview, it’s best to wear something comfortable but professional. A nice shirt or blouse with slacks or a skirt usually works well. If it’s a video interview, make sure your background is neat and you look presentable.
How important are algorithms for interviews?
Algorithms are very important for technical interviews. Many interview questions will test your understanding of algorithms and your ability to use them to solve problems. Make sure to study the most common algorithms before your interview.
How do I study for a technical interview?
To study for a technical interview, practice coding problems, review data structures and algorithms, and do mock interviews. Use online platforms like LeetCode, HackerRank, and Interview Cake to find practice problems and resources.
How do I answer technical interview questions I don’t know?
If you don’t know the answer to a technical interview question, be honest and say so. Then, try to talk through how you would approach finding a solution. Interviewers appreciate seeing your problem-solving process, even if you don’t have the exact answer.
What should I bring to a technical interview?
Bring a notebook, pen, and a copy of your resume to a technical interview. If it’s an in-person interview, bring a charged laptop if you expect to do any coding. For a video interview, make sure your computer and internet connection are reliable.