The Art of Writing Efficient Code: Mastering Performance and Readability
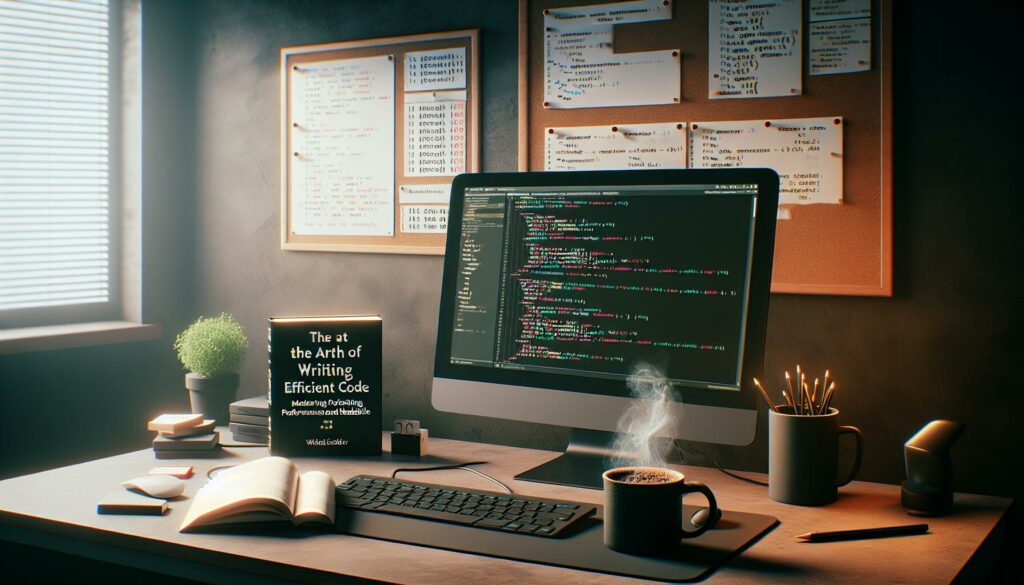
In the world of programming, writing code that simply works is often not enough. As software systems grow in complexity and scale, the need for efficient, performant, and maintainable code becomes increasingly crucial. This is where the art of writing efficient code comes into play. In this comprehensive guide, we’ll explore the principles, techniques, and best practices that can help you elevate your coding skills and create software that not only functions correctly but also runs smoothly and can be easily understood and maintained by others.
Understanding the Importance of Efficient Code
Before we dive into the specifics of writing efficient code, it’s essential to understand why it matters. Efficient code offers several benefits:
- Improved Performance: Efficient code runs faster and uses fewer resources, leading to better user experiences and lower operational costs.
- Scalability: Well-written code can handle increased loads and growing datasets more effectively.
- Maintainability: Clean, efficient code is easier for other developers (including your future self) to understand and modify.
- Cost-Effectiveness: Efficient code can reduce hardware requirements and energy consumption, leading to cost savings.
- Better User Experience: Faster, more responsive applications result in higher user satisfaction and engagement.
Principles of Writing Efficient Code
To master the art of writing efficient code, it’s crucial to internalize certain core principles:
1. Keep It Simple (KISS)
The KISS principle (Keep It Simple, Stupid) is a fundamental concept in software development. Simple code is often more efficient, easier to understand, and less prone to bugs. When writing code, always ask yourself if there’s a simpler way to achieve the same result.
2. Don’t Repeat Yourself (DRY)
The DRY principle advocates for reducing repetition in code. When you find yourself writing similar code in multiple places, it’s time to abstract that functionality into a reusable function or module. This not only makes your code more efficient but also easier to maintain and update.
3. Write Readable Code
Efficient code isn’t just about performance; it’s also about readability. Code that is easy to read and understand is less likely to contain bugs and is easier to maintain. Use meaningful variable names, add comments where necessary, and structure your code logically.
4. Optimize Judiciously
While optimization is important, it’s crucial to remember Donald Knuth’s famous quote: “Premature optimization is the root of all evil.” Focus on writing clear, correct code first, and optimize only when necessary and after profiling to identify bottlenecks.
5. Consider Time and Space Complexity
Understanding the time and space complexity of your algorithms is crucial for writing efficient code. Always consider how your code will perform with larger inputs and choose algorithms and data structures accordingly.
Techniques for Writing Efficient Code
Now that we’ve covered the principles, let’s explore some specific techniques for writing efficient code:
1. Choose the Right Data Structures
Selecting the appropriate data structure for your task can significantly impact the efficiency of your code. For example:
- Use arrays for fast, indexed access to elements
- Use hash tables (dictionaries in Python, objects in JavaScript) for quick key-based lookups
- Use linked lists for efficient insertions and deletions
- Use trees for hierarchical data and efficient searching
Here’s a simple example in Python demonstrating the performance difference between a list and a set for checking membership:
import time
# Using a list
large_list = list(range(1000000))
start_time = time.time()
999999 in large_list
end_time = time.time()
print(f"List lookup time: {end_time - start_time} seconds")
# Using a set
large_set = set(range(1000000))
start_time = time.time()
999999 in large_set
end_time = time.time()
print(f"Set lookup time: {end_time - start_time} seconds")
You’ll notice that the set lookup is significantly faster, especially for large datasets.
2. Optimize Loops
Loops are often a source of inefficiency in code. Here are some tips for optimizing loops:
- Minimize work inside loops
- Use break and continue statements wisely
- Consider using list comprehensions or map() in Python for simple loops
- Avoid nested loops when possible
Here’s an example of optimizing a loop in Python:
# Inefficient
result = []
for i in range(1000000):
if i % 2 == 0:
result.append(i ** 2)
# More efficient
result = [i ** 2 for i in range(1000000) if i % 2 == 0]
3. Use Built-in Functions and Libraries
Most programming languages come with a wealth of built-in functions and libraries that are often more efficient than custom implementations. Familiarize yourself with these and use them when appropriate.
For example, in Python:
# Less efficient
def find_max(numbers):
max_num = numbers[0]
for num in numbers[1:]:
if num > max_num:
max_num = num
return max_num
# More efficient
max_num = max(numbers)
4. Avoid Unnecessary Computations
Look for opportunities to reduce unnecessary computations. This could involve:
- Caching results of expensive operations
- Using lazy evaluation when appropriate
- Avoiding redundant calculations
Here’s an example of caching in Python using the functools.lru_cache
decorator:
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
# Now, repeated calls with the same argument will be much faster
print(fibonacci(100))
5. Use Appropriate Algorithms
Choosing the right algorithm for a task can make a huge difference in efficiency. For example, when sorting data:
- Use quicksort or mergesort for large datasets
- Use insertion sort for small datasets or nearly sorted data
- Use counting sort or radix sort for integers within a specific range
Here’s a Python example comparing bubble sort with the built-in sorted() function (which uses Timsort, a hybrid of mergesort and insertion sort):
import time
import random
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Generate a large list of random numbers
numbers = [random.randint(1, 1000) for _ in range(10000)]
# Time bubble sort
start_time = time.time()
bubble_sort(numbers.copy())
end_time = time.time()
print(f"Bubble sort time: {end_time - start_time} seconds")
# Time Python's built-in sort
start_time = time.time()
sorted(numbers)
end_time = time.time()
print(f"Built-in sort time: {end_time - start_time} seconds")
You’ll see that the built-in sort function is significantly faster for large datasets.
Best Practices for Writing Efficient Code
In addition to the principles and techniques we’ve discussed, here are some best practices to keep in mind:
1. Write Clean, Modular Code
Organize your code into logical, reusable modules. This not only makes your code more maintainable but can also lead to performance improvements through better code organization and reuse.
2. Use Appropriate Variable Scoping
Understanding and properly using variable scoping can lead to more efficient code. In many languages, accessing local variables is faster than accessing global variables.
3. Leverage Asynchronous Programming
For I/O-bound operations, using asynchronous programming can significantly improve the efficiency of your code by allowing other operations to proceed while waiting for I/O to complete.
4. Profile Your Code
Use profiling tools to identify performance bottlenecks in your code. Don’t rely on intuition alone; let data guide your optimization efforts.
5. Write Tests
Having a comprehensive test suite allows you to refactor and optimize your code with confidence, ensuring that improvements in efficiency don’t come at the cost of correctness.
6. Keep Learning and Staying Updated
Programming languages and best practices evolve. Stay updated with the latest features and optimizations in your chosen language and framework.
Common Pitfalls to Avoid
While striving for efficiency, it’s important to be aware of common pitfalls:
1. Premature Optimization
As mentioned earlier, don’t optimize prematurely. Write clear, correct code first, then optimize based on profiling results.
2. Sacrificing Readability for Performance
While performance is important, don’t sacrifice code readability unless absolutely necessary. Maintainable code is often more valuable than slightly faster but obscure code.
3. Reinventing the Wheel
Before implementing a complex algorithm or data structure from scratch, check if there’s already a well-tested, efficient implementation available in standard libraries or popular third-party packages.
4. Ignoring the Bigger Picture
Sometimes, the most significant performance gains come from architectural changes rather than code-level optimizations. Don’t lose sight of the overall system design.
Tools for Writing and Analyzing Efficient Code
Several tools can assist you in writing and analyzing efficient code:
1. Profilers
Profilers help identify performance bottlenecks in your code. Examples include:
- cProfile for Python
- Chrome DevTools for JavaScript
- Visual Studio Profiler for C#
2. Linters
Linters can help identify potential issues and inefficiencies in your code. Examples include:
- Pylint for Python
- ESLint for JavaScript
- RuboCop for Ruby
3. Code Analyzers
Static code analyzers can provide insights into code quality and potential optimizations. Examples include:
- SonarQube
- CodeClimate
- Checkmarx
4. Version Control Systems
While not directly related to efficiency, version control systems like Git allow you to track changes, experiment with optimizations, and collaborate effectively.
Conclusion
Writing efficient code is indeed an art that combines technical knowledge, experience, and creativity. It’s a skill that develops over time through practice, learning, and a constant drive for improvement. By following the principles, techniques, and best practices outlined in this guide, you’ll be well on your way to mastering the art of writing efficient code.
Remember, the journey to becoming an efficient coder is ongoing. Each project presents new challenges and opportunities to refine your skills. Embrace these challenges, stay curious, and never stop learning. With dedication and practice, you’ll not only write code that works but code that works efficiently, elegantly, and stands the test of time.
As you continue your coding journey, keep exploring new languages, paradigms, and tools. Engage with the developer community, contribute to open-source projects, and share your knowledge with others. The art of writing efficient code is not just about personal growth; it’s about contributing to the broader goal of creating better, more sustainable software systems that can tackle the complex challenges of our digital world.