Python for Beginners/ Free Lessons to Learn Coding from Scratch
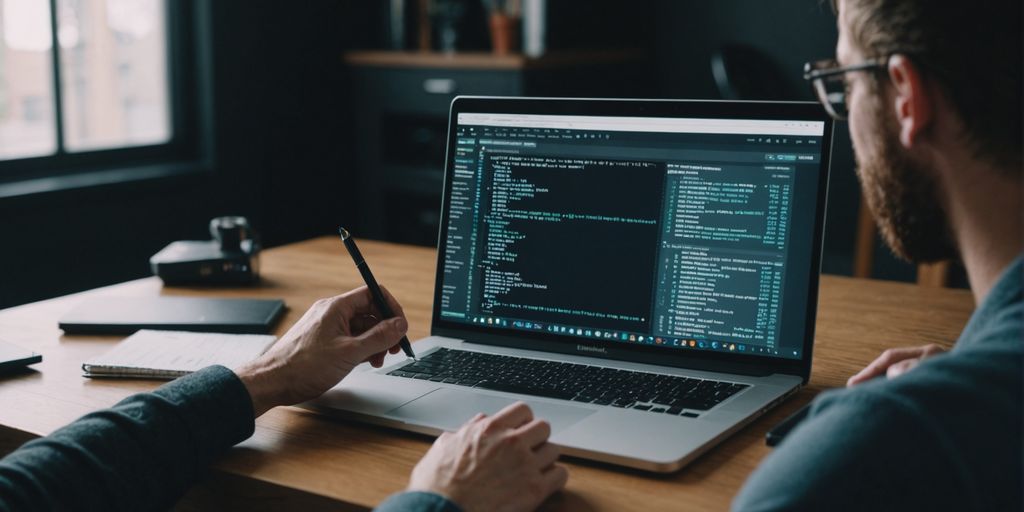
Python is a fantastic language for beginners who want to dive into the world of coding. Its simple syntax and readability make it an ideal choice for those just starting out. This article will guide you through the basics of Python, from installation to building your first projects, and even exploring advanced topics.
Key Takeaways
- Python is beginner-friendly with its easy-to-understand syntax.
- It is a versatile language used in web development, data analysis, and more.
- Python has extensive libraries that simplify coding tasks.
- A large, active community supports Python learners and developers.
- Many free resources are available to help you learn Python.
Why Choose Python for Beginners
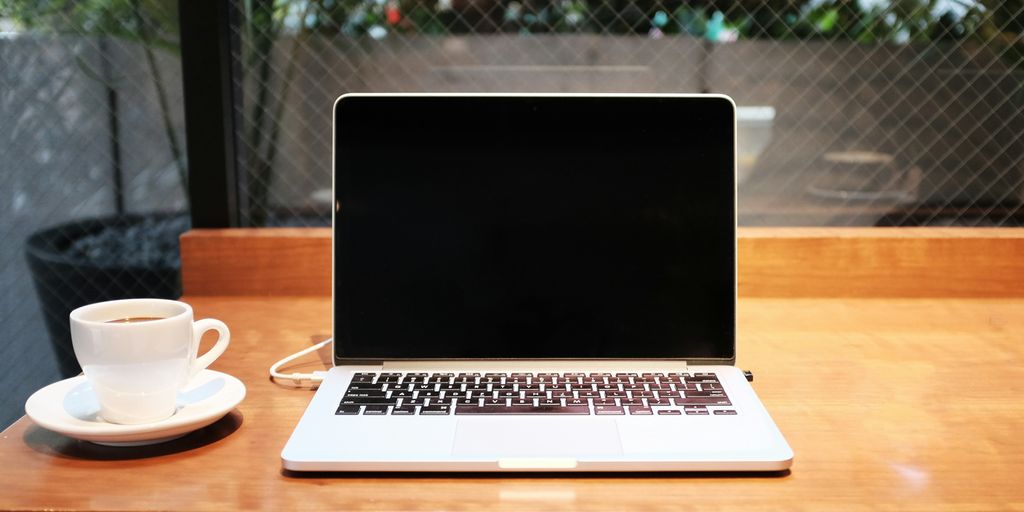
Beginner-Friendly Syntax
Python is known for its simple and concise syntax, making it an excellent choice for those new to coding. The language is interpreted, which means you can run your code and see results immediately, simplifying the debugging process. This instant feedback helps beginners learn and correct mistakes quickly.
Versatility Across Applications
One of Python’s standout features is its versatility. It can be used in various fields such as web development, machine learning, data analysis, and automation. This multipurpose nature allows you to apply Python skills to many different projects and industries.
Extensive Libraries and Modules
Python offers a vast array of libraries and modules that streamline development. Instead of writing code from scratch, you can use pre-existing libraries to save time and effort. This is particularly helpful for beginners who may find it challenging to write complex code from the ground up.
Active Developer Community
Python has a large, active community of developers and learners. This community is a valuable resource for beginners, offering a wealth of tutorials, documentation, and forums where you can ask questions and get help. The community’s support makes it easier to overcome challenges and continue learning.
Getting Started with Python
Installing Python and PyCharm
To begin your Python journey, you need to install Python on your computer. Visit the official Python website and download the latest version. Once installed, you can use IDLE, the default Python IDE, or opt for a more advanced IDE like PyCharm. PyCharm offers many features that make coding easier, such as code completion and debugging tools.
Understanding Python Syntax
Python’s syntax is known for being clear and easy to read. This makes it a great choice for beginners. The language uses indentation to define code blocks, which helps keep the code clean and organized. For example, a simple if
statement in Python looks like this:
if x > 0:
print("x is positive")
First Steps: Writing Your First Python Program
Writing your first Python program is a significant milestone. Start with a simple program that prints a message to the screen. Open your IDE and type the following code:
print("Hello, World!")
Run the program, and you should see the message "Hello, World!" displayed. This simple exercise helps you get comfortable with the basics of Python programming.
Core Python Concepts
Variables and Data Types
In Python, variables are used to store data. You can think of them as containers for holding information. Python supports various data types like integers, floats, strings, and booleans. For example, you can store a number in a variable like this:
age = 25
Control Flow: Conditionals and Loops
Control flow statements allow you to control the execution of your code. Conditionals like if
, elif
, and else
help you make decisions in your code. Loops, such as for
and while
, let you repeat actions multiple times. Here’s a simple example of a loop:
for i in range(5):
print(i)
Functions and Modules
Functions are blocks of code that perform a specific task. They help you organize your code and make it reusable. You can define a function using the def
keyword. Modules are files containing Python code that can be imported into your program. This allows you to use functions and variables defined in those modules. For instance:
def greet(name):
return f"Hello, {name}!"
import math
print(math.sqrt(16))
Once you learn the basics, you can continue on through the rest of the specialization and take the Python Data Structures course, the Using Python to Access Web Data course, and the Using Databases with Python course.
Working with Data in Python
Lists, Tuples, and Dictionaries
Python offers several ways to store and manage data. Lists, tuples, and dictionaries are among the most common data structures. Lists are ordered collections that can be changed, while tuples are ordered but cannot be changed. Dictionaries store data in key-value pairs, making it easy to look up values.
File Handling
File handling is a crucial skill in Python. You can read from and write to files, which is essential for many applications. Here are the basic steps:
- Open the file using
open()
function. - Read or write to the file using methods like
read()
,write()
, orreadlines()
. - Close the file using
close()
method.
Data Manipulation with Pandas
Pandas is a powerful library for data manipulation and analysis. It provides data structures like DataFrames, which make it easy to handle large datasets. With Pandas, you can perform operations like filtering, grouping, and merging data.
Pandas is especially useful for those looking to learn data science with Python. It simplifies many tasks that would otherwise be complex and time-consuming.
Building Projects with Python
Creating a Basic Calculator
One of the first projects you can try is building a basic calculator. This project will help you understand how to take user input, perform arithmetic operations, and display results. You can start with simple operations like addition, subtraction, multiplication, and division. As you get more comfortable, you can add more complex features like handling errors and performing advanced calculations.
Developing a Mad Libs Game
Creating a Mad Libs game is a fun way to practice string manipulation and user input in Python. In this project, you will ask the user for different types of words (nouns, verbs, adjectives, etc.) and then insert those words into a pre-defined story template. The result is a hilarious and often nonsensical story that can be different every time you play.
Building a Simple Web Scraper
A web scraper is a tool that extracts data from websites. This project will introduce you to libraries like BeautifulSoup and requests, which are essential for web scraping in Python. You can start by scraping simple data like headlines from a news website or product prices from an e-commerce site. This project is a great way to gain practical experience with Python programming while building real-world applications.
Exploring Python Libraries
Introduction to NumPy
NumPy is a powerful library for numerical computing in Python. It provides support for arrays, matrices, and many mathematical functions to operate on these data structures. NumPy is essential for scientific computing and data analysis.
Data Visualization with Matplotlib
Matplotlib is a plotting library for creating static, animated, and interactive visualizations in Python. It is highly customizable and can produce publication-quality figures. You can create a wide range of plots, including line graphs, bar charts, and scatter plots.
Web Development with Django
Django is a high-level web framework that encourages rapid development and clean, pragmatic design. It is known for its simplicity and ease of use, making it a popular choice for web developers. With Django, you can build robust and scalable web applications quickly.
Python libraries are collections of related modules that contain bundles of code you can use repeatedly in different programs.
Learning Resources for Python
Free Online Courses
There are many free online courses to help you learn Python. Google’s Python Class is a great place to start. The class includes written materials, lecture videos, and lots of code exercises to practice Python coding. Another excellent resource is FreeCodeCamp’s Learn Python, which offers a comprehensive introduction to Python’s core concepts. You can also check out Educative’s Learn Python 3 from Scratch, which provides a hands-on learning experience in live coding environments.
YouTube Tutorials
YouTube is full of helpful Python tutorials. CS Dojo’s Python Tutorials for Absolute Beginners is a popular series that covers dictionaries, loops, functions, and more. Programming with Mosh offers a Python Tutorial – Python for Beginners, where you can learn programming basics and build projects like a website with Django. Brad Traversy’s Python Crash Course for Beginners is another great option, covering lists, tuples, dictionaries, and more.
Books and Documentation
Books and official documentation are also valuable resources. The Python Handbook by Flavio Copes is a great book to learn about strings, lists, tuples, recursion, and more. Automate the Boring Stuff with Python is another excellent book that teaches you about dictionaries, strings, debugging, and regular expressions. For official documentation, the Python Software Foundation’s website is the best place to find detailed information on Python’s features and libraries.
Interactive Python Learning
Coding Challenges and Quizzes
Engaging in coding challenges and quizzes is a fantastic way to test your Python skills. These activities not only help you practice but also make learning fun. Platforms like CodeCombat are designed to introduce beginners to the world of coding in a fun and interactive manner. You can find various websites offering Python coding games and challenges to keep you motivated.
Interactive Coding Platforms
Interactive coding platforms provide a hands-on approach to learning Python. Websites like Codecademy and DataCamp offer interactive tutorials where you can write and run Python code directly in your browser. These platforms often include instant feedback, which is crucial for beginners to understand their mistakes and learn from them.
Community Forums and Support
Joining community forums and support groups can be incredibly beneficial. These communities are filled with experienced developers who can offer advice, answer questions, and provide support. Platforms like Stack Overflow, Reddit, and specialized Python forums are excellent places to seek help and share knowledge.
Tip: Don’t hesitate to ask questions in forums. The Python community is known for being welcoming and helpful to beginners.
Advanced Python Topics
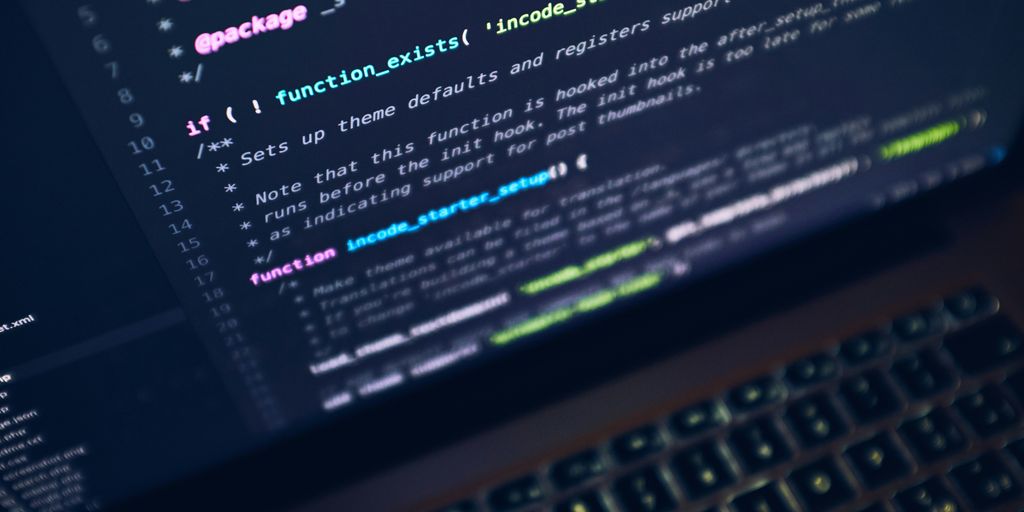
Object-Oriented Programming
Object-Oriented Programming (OOP) is a programming paradigm that uses objects and classes. OOP helps organize code into reusable and modular pieces. Key concepts include:
- Classes and Objects
- Inheritance
- Polymorphism
- Encapsulation
Working with APIs
APIs (Application Programming Interfaces) allow different software systems to communicate. In Python, you can use libraries like requests
to interact with APIs. This is useful for tasks like fetching data from web services or integrating different applications.
Introduction to Machine Learning
Machine Learning (ML) is a field of artificial intelligence that enables computers to learn from data. Python is popular in ML due to its extensive libraries like scikit-learn
, TensorFlow
, and Keras
. Machine learning can be applied to various tasks such as classification, regression, and clustering.
Exploring advanced Python topics can significantly enhance your coding skills and open up new career opportunities.
Career Opportunities with Python
Job Roles for Python Developers
Python opens doors to various job roles. You can become a software developer who excels in Python or a data scientist who uses this language to make data more valuable for businesses. Here are some common job roles:
- Python Developer: Typically works on the server side of project development, using Python to code, design, deploy, and debug. The average annual salary is $81,098, with top earners making $116,000.
- Software Engineer: Involves programming and coding in Python and other languages like C#, Java, and JavaScript. The average annual salary is $92,378, with the top 10% earning $135,000.
- Data Scientist: Designs and develops processes for modeling data, creating algorithms, and performing custom analyses. The average annual salary is $99,586, with top earners making $136,000.
- Machine Learning Engineer: Researches, designs, and builds AI systems using Python. The average annual salary is $115,498, with the top 10% earning $157,000.
Building a Python Portfolio
Creating a strong portfolio is essential for showcasing your Python skills. Include projects that highlight your ability to solve real-world problems. Here are some tips:
- Start with simple projects: Build a basic calculator or a to-do list app.
- Show diversity: Include projects from different domains like web development, data analysis, and automation.
- Use version control: Host your projects on platforms like GitHub to show your coding history and collaboration skills.
Networking and Professional Development
Networking is crucial for career growth. Join Python communities, attend meetups, and participate in online forums. Here are some ways to network:
- Attend conferences: Events like PyCon offer opportunities to meet industry experts.
- Join online communities: Platforms like Reddit and Stack Overflow have active Python communities.
- Participate in hackathons: These events provide hands-on experience and networking opportunities.
Staying connected with the Python community can open doors to new job opportunities and collaborations.
Maintaining Your Python Skills
Continuous Learning and Practice
To keep your Python skills sharp, continuous learning and practice are essential. Here are some ways to stay on top of your game:
- Online Courses: Enroll in advanced Python courses to learn new concepts and techniques.
- Coding Challenges: Participate in coding challenges on platforms like LeetCode or HackerRank.
- Projects: Work on personal or open-source projects to apply your skills in real-world scenarios.
Contributing to Open Source
Contributing to open-source projects is a great way to improve your skills and give back to the community. You can:
- Find Projects: Look for projects on GitHub that interest you and need help.
- Collaborate: Work with other developers to solve issues and add new features.
- Learn: Gain insights from experienced developers and improve your coding practices.
Staying Updated with Python Trends
The tech world is always evolving, and Python is no exception. Stay updated with the latest trends by:
- Reading Blogs: Follow popular Python blogs and websites.
- Attending Conferences: Participate in Python conferences and webinars.
- Joining Communities: Be active in Python communities on forums and social media.
Keeping your Python skills up-to-date requires dedication and a proactive approach. By continuously learning, contributing to open-source projects, and staying informed about the latest trends, you can ensure that your skills remain relevant and sharp.
Keeping your Python skills sharp is essential in today’s tech world. Whether you’re a beginner or an experienced coder, continuous learning is key. Our platform offers interactive tutorials and a supportive community to help you stay ahead. Don’t miss out on the chance to improve your coding abilities. Visit our website to start your journey today!
Conclusion
Learning Python can be a rewarding journey, especially for beginners. Its simple syntax and vast community support make it an ideal first programming language. Whether you want to build websites, analyze data, or automate tasks, Python has the tools you need. With numerous free resources available, there’s no better time to start coding. Dive into the world of Python and unlock endless possibilities. Happy coding!
Frequently Asked Questions
What is Python?
Python is a popular programming language known for its simple and easy-to-read syntax. It’s used in web development, data analysis, artificial intelligence, and more.
Why should beginners learn Python?
Python is beginner-friendly due to its straightforward syntax. It also has a large community and many resources, making it easier for newcomers to find help and tutorials.
How do I install Python?
You can download Python from its official website, python.org. Follow the instructions for your operating system to install it. You can also use an Integrated Development Environment (IDE) like PyCharm.
What are Python libraries?
Python libraries are collections of pre-written code that you can use to add functionality to your projects without writing code from scratch. Examples include NumPy for numerical operations and Pandas for data manipulation.
Can I use Python for web development?
Yes, Python can be used for web development. Frameworks like Django and Flask make it easier to build web applications using Python.
What is the best way to practice Python?
The best way to practice Python is by working on projects, solving coding challenges, and participating in coding platforms like LeetCode or HackerRank. Consistent practice helps improve your skills.
Are there free resources to learn Python?
Yes, there are many free resources to learn Python, including online courses, YouTube tutorials, and documentation. Websites like Coursera, freeCodeCamp, and Codecademy offer free Python courses.
What career opportunities are available for Python developers?
Python developers can work in various fields such as web development, data analysis, machine learning, and automation. Job roles include software developer, data scientist, and machine learning engineer.