Perfect Squares: Mastering a Fundamental Concept in Mathematics and Programming
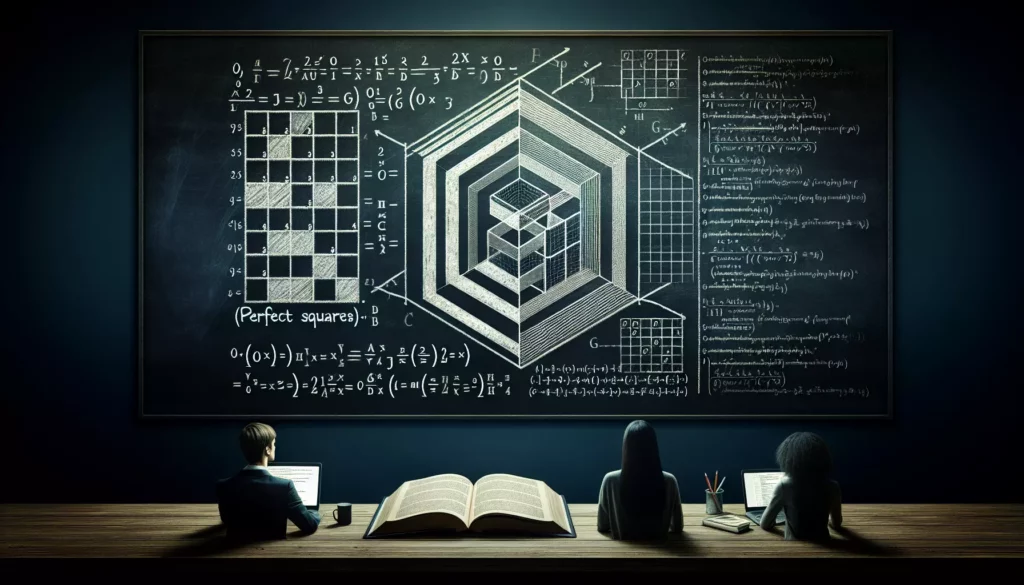
In the realm of mathematics and computer science, certain concepts serve as building blocks for more complex problem-solving. One such fundamental concept is that of perfect squares. Understanding perfect squares is crucial for developing strong algorithmic thinking and coding skills, especially when preparing for technical interviews at major tech companies. In this comprehensive guide, we’ll dive deep into the world of perfect squares, exploring their properties, applications, and how they relate to various programming challenges.
What Are Perfect Squares?
Perfect squares, also known as square numbers, are integers that result from multiplying an integer by itself. In mathematical terms, a perfect square is the product of an integer with itself. For example:
- 1 is a perfect square (1 × 1 = 1)
- 4 is a perfect square (2 × 2 = 4)
- 9 is a perfect square (3 × 3 = 9)
- 16 is a perfect square (4 × 4 = 16)
- 25 is a perfect square (5 × 5 = 25)
The sequence of perfect squares continues infinitely: 36, 49, 64, 81, 100, and so on. Each of these numbers is the result of an integer multiplied by itself.
Properties of Perfect Squares
Perfect squares possess several interesting properties that make them valuable in various mathematical and programming contexts:
- Positive Nature: All perfect squares are non-negative. The smallest perfect square is 0 (0 × 0 = 0), and all others are positive integers.
- Digital Root: The digital root (sum of digits repeatedly until a single digit is obtained) of a perfect square is always 1, 4, 7, or 9.
- Parity: The parity (oddness or evenness) of a perfect square is determined by its root. If the root is even, the square is even; if the root is odd, the square is odd.
- Divisibility: Every perfect square is divisible by 3 or leaves a remainder of 1 when divided by 3.
- Prime Factors: The prime factorization of a perfect square always contains even exponents for each prime factor.
Identifying Perfect Squares
Recognizing perfect squares is a valuable skill in both mathematics and programming. Here are some methods to identify perfect squares:
1. Square Root Method
The most straightforward method is to calculate the square root of a number. If the result is an integer, the original number is a perfect square.
def is_perfect_square(n):
root = int(n ** 0.5)
return root * root == n
# Example usage
print(is_perfect_square(16)) # Output: True
print(is_perfect_square(18)) # Output: False
2. Binary Search Method
For larger numbers, a binary search approach can be more efficient:
def is_perfect_square(n):
if n < 2:
return True
left, right = 2, n // 2
while left <= right:
mid = left + (right - left) // 2
square = mid * mid
if square == n:
return True
elif square < n:
left = mid + 1
else:
right = mid - 1
return False
# Example usage
print(is_perfect_square(1000000)) # Output: True
print(is_perfect_square(1000001)) # Output: False
3. Digital Root Method
While not foolproof, checking the digital root can quickly eliminate many non-perfect squares:
def digital_root(n):
return 1 + (n - 1) % 9 if n else 0
def could_be_perfect_square(n):
root = digital_root(n)
return root in [1, 4, 7, 9]
# Example usage
print(could_be_perfect_square(16)) # Output: True
print(could_be_perfect_square(18)) # Output: False
Applications of Perfect Squares in Programming
Perfect squares find applications in various programming scenarios, particularly in algorithm design and problem-solving. Let’s explore some common applications:
1. Geometric Calculations
Perfect squares are fundamental in geometric calculations, especially those involving areas and distances.
Example: Calculating Euclidean Distance
import math
def euclidean_distance(x1, y1, x2, y2):
dx = x2 - x1
dy = y2 - y1
return math.sqrt(dx*dx + dy*dy)
# Example usage
print(euclidean_distance(0, 0, 3, 4)) # Output: 5.0
2. Number Theory Problems
Many number theory problems involve perfect squares, such as finding the smallest perfect square divisible by a given number.
Example: Smallest Perfect Square Divisible by N
def smallest_square_divisible_by(n):
i = 1
while True:
if (i * i) % n == 0:
return i * i
i += 1
# Example usage
print(smallest_square_divisible_by(72)) # Output: 144
3. Cryptography
Perfect squares play a role in various cryptographic algorithms, particularly those based on modular arithmetic.
Example: Simple Modular Square Root
def modular_square_root(n, p):
for i in range(p):
if (i * i) % p == n:
return i
return None # No square root exists
# Example usage
print(modular_square_root(2, 7)) # Output: 3 (since 3^2 ≡ 2 (mod 7))
4. Game Development
Perfect squares are often used in game development, particularly for grid-based games or for calculating distances and movements.
Example: Checking if a Move is Diagonal on a Chessboard
def is_diagonal_move(x1, y1, x2, y2):
dx = abs(x2 - x1)
dy = abs(y2 - y1)
return dx == dy and dx > 0
# Example usage
print(is_diagonal_move(1, 1, 3, 3)) # Output: True
print(is_diagonal_move(1, 1, 2, 3)) # Output: False
Advanced Topics Related to Perfect Squares
As we delve deeper into the world of perfect squares, we encounter more advanced concepts and problems that are often featured in coding interviews and competitive programming.
1. Sum of Perfect Squares Problem
One classic problem involving perfect squares is determining the minimum number of perfect squares that sum to a given number. This problem has applications in dynamic programming and number theory.
Example: Lagrange’s Four-Square Theorem Implementation
def min_perfect_squares(n):
if int(n**0.5)**2 == n:
return 1
# Check if n can be represented as sum of two squares
for i in range(1, int(n**0.5) + 1):
if int((n - i*i)**0.5)**2 == n - i*i:
return 2
# Check if n is of the form 4^a(8b + 7)
while n % 4 == 0:
n //= 4
if n % 8 == 7:
return 4
return 3
# Example usage
print(min_perfect_squares(12)) # Output: 3 (4 + 4 + 4)
print(min_perfect_squares(13)) # Output: 2 (4 + 9)
2. Perfect Square Subsequences
Finding subsequences of numbers that form perfect squares is another interesting problem that combines sequence manipulation and perfect square properties.
Example: Longest Perfect Square Subsequence
def longest_perfect_square_subsequence(arr):
n = len(arr)
dp = [1] * n
for i in range(1, n):
for j in range(i):
if int((arr[i] + arr[j])**0.5)**2 == arr[i] + arr[j]:
dp[i] = max(dp[i], dp[j] + 1)
return max(dp)
# Example usage
sequence = [1, 2, 3, 4, 9, 8, 7, 16]
print(longest_perfect_square_subsequence(sequence)) # Output: 4
3. Perfect Square Factors
Analyzing the perfect square factors of a number can lead to interesting mathematical insights and is often used in number theory problems.
Example: Count Perfect Square Factors
def count_perfect_square_factors(n):
count = 0
for i in range(1, int(n**0.5) + 1):
if n % i == 0:
if i * i == n:
count += 1
elif (n // i) * (n // i) == n:
count += 1
return count
# Example usage
print(count_perfect_square_factors(72)) # Output: 2 (1 and 36)
Perfect Squares in Competitive Programming
Perfect squares often appear in competitive programming problems, especially those related to number theory and mathematical optimization. Here are some tips for handling perfect square-related problems in coding competitions:
- Precomputation: For problems involving multiple queries about perfect squares, consider precomputing a list of perfect squares up to a certain limit.
- Efficient Checking: Use efficient methods to check if a number is a perfect square, such as the binary search method mentioned earlier.
- Mathematical Properties: Leverage the unique properties of perfect squares, such as their digital roots or divisibility rules, to optimize your solutions.
- Dynamic Programming: For problems involving sums or sequences of perfect squares, dynamic programming approaches can often lead to efficient solutions.
Example: Precomputing Perfect Squares
MAX_N = 10**6
perfect_squares = set(i*i for i in range(int(MAX_N**0.5) + 1))
def is_perfect_square(n):
return n in perfect_squares
# Example usage
print(is_perfect_square(16)) # Output: True
print(is_perfect_square(17)) # Output: False
Perfect Squares in Technical Interviews
Understanding perfect squares and their properties can be valuable in technical interviews, especially when dealing with problems related to mathematics, optimization, or geometric algorithms. Here are some ways perfect squares might be relevant in interview scenarios:
- Optimization Problems: Problems that involve minimizing or maximizing certain values often have solutions related to perfect squares.
- Grid-based Problems: In problems involving 2D grids or matrices, perfect squares can be useful for calculating distances or determining relationships between points.
- Number Theory Questions: Interviewers might ask questions that require understanding the properties of perfect squares as part of a larger number theory problem.
- Algorithm Design: Knowledge of perfect squares can lead to more efficient algorithms for certain types of problems, showcasing your ability to optimize solutions.
Interview Question Example: Closest Perfect Square
Consider this potential interview question: “Given a positive integer n, find the closest perfect square to n. If there are two equally close perfect squares, return the smaller one.”
Here’s a solution to this problem:
def closest_perfect_square(n):
root = int(n ** 0.5)
lower_square = root * root
upper_square = (root + 1) * (root + 1)
if n - lower_square <= upper_square - n:
return lower_square
else:
return upper_square
# Example usage
print(closest_perfect_square(15)) # Output: 16
print(closest_perfect_square(18)) # Output: 16
This solution demonstrates understanding of perfect squares, efficient computation, and careful handling of edge cases.
Conclusion
Perfect squares are a fundamental concept in mathematics and computer science, with wide-ranging applications in algorithm design, problem-solving, and technical interviews. By mastering the properties and applications of perfect squares, you’ll be better equipped to tackle a variety of coding challenges and optimize your solutions in competitive programming scenarios.
As you continue your journey in coding education and skills development, remember that concepts like perfect squares are just one piece of the puzzle. The key to success in technical interviews and competitive programming lies in building a strong foundation in algorithmic thinking, practicing regularly with diverse problems, and continuously expanding your knowledge of mathematical and computational concepts.
Keep exploring, keep practicing, and don’t hesitate to dive deep into seemingly simple concepts like perfect squares – you never know when that knowledge might be the key to cracking a complex problem or acing a technical interview at a major tech company. Happy coding!