Mastering the Art of Spinning Up a Local Server: A Comprehensive Guide for Beginners
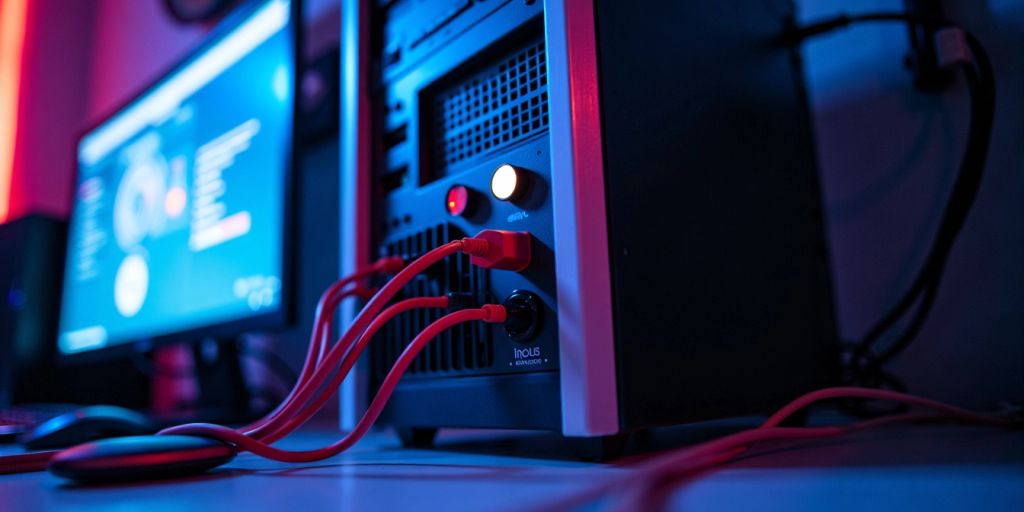
Setting up a local server can seem tough, but it’s really just a series of steps that anyone can follow. This guide is designed to help beginners understand the basics and walk through the process of spinning up a local server. By the end, you’ll have the skills to create your own server and run applications on it.
Key Takeaways
- A local server lets you test and develop applications on your own machine before going online.
- Node.js is a popular tool for building server applications and is easy to set up.
- Using PM2 helps manage your applications, keeping them running smoothly even if they crash.
- Nginx is a helpful tool for directing traffic to your applications, acting like a traffic cop for the web.
- Securing your server is crucial; always set up firewalls and use HTTPS to protect your data.
Understanding the Basics of Spinning Up a Local Server
What is a Local Server?
A local server is a server that runs on your own computer. It allows you to test and develop websites or applications without needing to be connected to the internet. This is useful for learning and experimenting with code. When you run a local server, your computer acts like a web server, serving files to your web browser.
Why Use a Local Server?
Using a local server has several advantages:
- Speed: You can load your projects quickly without internet delays.
- Privacy: Your work is only visible to you until you decide to share it.
- Control: You can easily modify and test your code without affecting a live site.
Common Use Cases for Local Servers
Local servers are commonly used for:
- Web Development: Testing websites before going live.
- Learning: Practicing coding skills in a safe environment.
- Game Development: Creating and testing game servers locally.
Running a local server is a great way to learn how to build and manage web applications. It gives you the freedom to experiment without the fear of breaking something important.
Use Case | Description |
---|---|
Web Development | Test websites before launching them online. |
Learning | Practice coding skills in a controlled setting. |
Game Development | Create and test game servers locally. |
Setting Up Your Development Environment
Choosing the Right Tools
To start your journey in web development, selecting the right tools is crucial. Here are some essential tools you should consider:
- Code Editor: Use a specialized code editor like Visual Studio Code (VS Code) for better coding experience.
- Version Control: Git helps you manage changes in your code.
- Web Browser: A modern browser like Chrome or Firefox is necessary for testing your applications.
Installing Necessary Software
Once you have your tools, it’s time to install the necessary software. Follow these steps:
- Download and install Node.js for running JavaScript on your server.
- Install Git for version control.
- Set up your code editor (like VS Code) to start coding.
Configuring Your System
After installation, you need to configure your system for development. Here’s how:
- Set up your environment variables for Node.js and Git.
- Create a project folder where you will store your code.
- Ensure your code editor is set to recognize the programming languages you will use.
Setting up your development environment is the first step towards becoming a successful developer. It lays the foundation for your coding journey.
By following these steps, you will have a solid development environment ready for building your applications. Remember, this guide will walk you through the steps of getting a basic development environment set up for building WooCommerce extensions!
Installing and Configuring Node.js
Downloading Node.js
To get started with Node.js, you first need to download the installer. Here’s how:
- Go to the official Node.js website.
- Choose the version suitable for your operating system (Windows, macOS, or Linux).
- Click on the download link to start the installation.
Setting Up Node.js
After downloading, follow these steps to set up Node.js:
- Run the installer you downloaded.
- Follow the prompts in the installation wizard.
- Make sure to check the box that says "Add to PATH" during installation.
Verifying the Installation
Once the installation is complete, you can verify it:
- Open your command line terminal (Command Prompt on Windows, Terminal on macOS/Linux).
- Type
node -v
and press Enter. This command will show you the installed version of Node.js. - If you see a version number, congratulations! Node.js is successfully installed.
Remember: Node.js allows you to run JavaScript outside of a web browser, making it a powerful tool for developers.
Summary Table
Here’s a quick summary of the steps:
Step | Action |
---|---|
1. Download | Get the installer from the Node.js site |
2. Install | Run the installer and follow prompts |
3. Verify Installation | Use node -v in the terminal |
Creating Your First Node.js Application
Setting Up Your Project Directory
To start your Node.js journey, you need to create a project directory. Here’s how:
- Open your terminal.
- Navigate to the location where you want to create your project.
- Run the command:
mkdir my-node-app
to create a new folder. - Change into that directory with
cd my-node-app
.
Writing Your First Script
Now, let’s write a simple script. Follow these steps:
- Create a new file named
app.js
in your project directory. - Open
app.js
in your favorite text editor. - Add the following code:
console.log('Hello, Node.js!');
- Save the file.
This is your first Node.js application! It simply prints a message to the console.
Running Your Application
To see your application in action, you need to run it:
- Go back to your terminal.
- Make sure you are in the project directory.
- Type
node app.js
and hit Enter. - You should see
Hello, Node.js!
printed in the terminal.
Remember, this is just the beginning! As you learn more, you can create more complex applications.
Now you have successfully created and run your first Node.js application! This is a crucial step in the ultimate tutorial to getting started with Node.js. Keep exploring and building!
Using PM2 for Process Management
What is PM2?
PM2 is a powerful process manager for Node.js applications. It helps you manage your app’s state, allowing you to start, stop, restart, and delete processes easily. With PM2, you can ensure that your application runs smoothly, even if it crashes unexpectedly.
Installing PM2
To install PM2, you can use npm, which is included with Node.js. Here’s how to do it:
- Open your terminal.
- Run the command:
npm install pm2 -g
- Wait for the installation to complete.
Managing Your Application with PM2
Once PM2 is installed, you can manage your applications with simple commands:
- Start your app:
pm2 start app.js
- Stop your app:
pm2 stop app.js
- Restart your app:
pm2 restart app.js
- Delete your app:
pm2 delete app.js
Command | Description |
---|---|
pm2 start |
Starts your application |
pm2 stop |
Stops your application |
pm2 restart |
Restarts your application |
pm2 delete |
Deletes your application from PM2 |
PM2 not only keeps your app running but also provides monitoring features to help you track performance.
By using PM2, you can focus on building your application while it takes care of managing your processes efficiently. Managing your bot process with PM2 ensures that your application is always available and running as expected.
Setting Up Nginx as a Reverse Proxy
Introduction to Nginx
Nginx is a powerful web server that can also act as a reverse proxy. This means it can take requests from users and send them to your application running on a different port. It helps manage traffic and improves performance.
Installing Nginx
To get started, you need to install Nginx on your local server. Here’s how:
- Open your terminal.
- Run the command:
sudo apt install nginx
. - Once installed, start Nginx with:
sudo systemctl start nginx
.
Configuring Nginx for Your Application
To configure Nginx as a reverse proxy, you need to create a new configuration file. This file will contain the server blocks and directives needed for routing your application. Here’s a simple example:
server {
listen 80;
server_name yourdomain.com;
location / {
proxy_pass http://localhost:3000;
}
}
This configuration tells Nginx to listen on port 80 and forward requests to your application running on port 3000. Make sure to replace yourdomain.com
with your actual domain.
Important Notes
- Always test your configuration with
nginx -t
before restarting Nginx. - Restart Nginx using
sudo systemctl restart nginx
after making changes.
Setting up Nginx correctly can greatly enhance your application’s performance and security.
By following these steps, you can successfully set up Nginx as a reverse proxy for your application, allowing for better traffic management and improved user experience.
Securing Your Local Server
Understanding Security Basics
Securing your local server is crucial to protect your data and applications. A strong security setup can prevent unauthorized access and data breaches. Here are some key points to consider:
- Create strong passwords: Use passwords that are at least 12 characters long, combining letters, numbers, and symbols.
- Keep software updated: Regularly update your server software to patch vulnerabilities.
- Limit user access: Only give access to those who need it.
Setting Up Firewalls
Firewalls act as a barrier between your server and potential threats. Here’s how to set one up:
- Choose a firewall solution (like UFW for Linux).
- Configure rules to allow only necessary traffic.
- Regularly review and update your firewall settings.
Firewall Type | Description |
---|---|
Software | Installed on the server, controls incoming/outgoing traffic |
Hardware | A physical device that protects the network |
Using HTTPS and SSL
Using HTTPS is essential for secure data transmission. Here’s how to implement it:
- Obtain an SSL certificate: This can often be done for free through services like Let’s Encrypt.
- Configure your server to use HTTPS: Update your server settings to redirect HTTP traffic to HTTPS.
- Test your setup: Use online tools to ensure your SSL is correctly installed.
Remember, securing your server is an ongoing process. Regularly review your security measures to adapt to new threats.
Testing and Debugging Your Server
Local Testing Tools
Testing your local server is crucial to ensure everything works as expected. Here are some tools you can use:
- Browser Developer Tools: Use the Network and Console tabs to check requests and responses.
- HTTP Testing Tools: Applications like Postman can simulate various requests to your server.
- Log Aggregators: Services like Loggly can help capture logs from your local environment.
Common Debugging Techniques
Even the best developers face issues. Here are some tips to troubleshoot common problems:
- Check the Console: Look for error messages or logs that can help identify issues.
- Use a Built-In Inspector: Some tools offer a GUI-based inspector for easier debugging.
- Review Documentation: Familiarize yourself with the documentation of the tools you are using for additional help.
Automated Testing
Automated testing can save you time and effort. Here are some benefits:
- Consistency: Automated tests run the same way every time, reducing human error.
- Speed: Tests can be executed quickly, allowing for faster feedback.
- Coverage: You can cover more scenarios than manual testing.
Remember: Testing is an essential part of development. It helps you catch issues early and ensures your application runs smoothly.
By using these tools and techniques, you can effectively test and debug your local server, ensuring a smoother development process. Testing your server locally is a vital step before deployment, helping you catch issues early on.
Deploying Your Local Server to Production
Preparing for Deployment
Before you can share your application with the world, you need to get it ready for deployment. This involves several key steps:
- Choose a Hosting Provider: Select a reliable hosting service that fits your needs. Popular options include DigitalOcean, AWS, and Heroku.
- Set Up Your Server: Create a new server instance and configure it according to your application requirements.
- Transfer Your Files: Use tools like FTP or Git to upload your application files to the server.
Choosing a Hosting Provider
When selecting a hosting provider, consider the following factors:
- Cost: Make sure it fits your budget.
- Performance: Look for good uptime and speed.
- Support: Check if they offer reliable customer service.
Provider | Cost (Monthly) | Uptime Guarantee | Support Type |
---|---|---|---|
DigitalOcean | $5 | 99.99% | 24/7 Chat |
AWS | Pay-as-you-go | 99.99% | Email/Chat |
Heroku | Free/Paid | 99.95% |
Deploying Your Application
Once your server is set up, follow these steps to deploy your application:
- Install Necessary Software: Make sure your server has Node.js and any other dependencies installed.
- Run Your Application: Start your application using a process manager like PM2 to keep it running.
- Test Your Application: Access your application through the server’s IP address or domain name to ensure everything works.
Remember: Testing is crucial! Always check for errors before announcing your application to the public.
Conclusion
Deploying your local server to production is an exciting step. With the right preparation and tools, you can successfully share your application with users around the world. This tutorial provides some guidance on your options for choosing a hosting site and what you need to do to get your app ready for the big stage!
Maintaining and Updating Your Server
Keeping your server in good shape is essential for smooth operation. Regular maintenance helps prevent issues and ensures everything runs efficiently. Here are some key areas to focus on:
Regular Maintenance Tasks
- Check for Updates: Always install the latest software updates to keep your server secure.
- Backup Data: Regularly back up your data to avoid loss in case of a failure.
- Monitor Performance: Use tools to keep an eye on server performance and identify any potential problems early.
Updating Software and Dependencies
Updating your software and dependencies is crucial. Here’s how to do it:
- Identify Outdated Software: Use a tool to scan for outdated applications.
- Schedule Updates: Plan updates during off-peak hours to minimize disruption.
- Test Updates: Before applying updates, test them in a safe environment to ensure they won’t cause issues.
Monitoring Server Performance
Monitoring is key to maintaining a healthy server. Consider these methods:
- Use Monitoring Tools: Tools like Nagios or Zabbix can help track server health.
- Set Alerts: Configure alerts for unusual activity or performance drops.
- Review Logs: Regularly check server logs for any errors or warnings.
Keeping your server updated and maintained is not just about fixing problems; it’s about ensuring reliability and performance for your users.
By following these practices, you can ensure your server remains robust and ready to handle any tasks you throw at it. Remember, ensuring regular maintenance and an appropriate lifecycle is the first thing to put in place before looking at resilience. The server hardware should be monitored closely to avoid unexpected failures.
Exploring Advanced Topics
Using Docker for Containerization
Docker is a tool that helps you create, deploy, and run applications in containers. Containers are lightweight and portable, making it easier to manage your applications. Here are some key benefits of using Docker:
- Isolation: Each container runs independently, so changes in one do not affect others.
- Consistency: You can run the same container on any machine, ensuring your app behaves the same everywhere.
- Efficiency: Containers use fewer resources than traditional virtual machines.
Scaling Your Application
Scaling your application means adjusting its capacity to handle more users or data. Here are some common methods:
- Vertical Scaling: Adding more resources (CPU, RAM) to your existing server.
- Horizontal Scaling: Adding more servers to distribute the load.
- Load Balancing: Distributing incoming traffic across multiple servers to ensure no single server is overwhelmed.
Integrating with Cloud Services
Cloud services can enhance your local server’s capabilities. Here are some popular options:
- Amazon Web Services (AWS): Offers a wide range of services, including storage and computing power.
- Google Cloud Platform (GCP): Provides tools for machine learning and data analytics.
- Microsoft Azure: Integrates well with Windows-based applications and services.
Exploring these advanced topics can help you kickstart your web journey with a local server. They provide a foundation for building robust applications that can grow with your needs.
By understanding these concepts, you can take your local server skills to the next level!
Dive into the world of coding with us! At AlgoCademy, we make learning to code fun and easy. Whether you’re just starting or looking to sharpen your skills, our interactive lessons are designed for everyone. Don’t wait any longer—visit our website and start coding for free today!
Conclusion
In conclusion, setting up a local server might seem tough at first, but with the right steps, it can be a fun learning experience. By following this guide, you’ve learned how to install necessary tools, create applications, and manage your server effectively. Remember, practice is key! The more you work with your server, the more comfortable you’ll become. Don’t hesitate to explore further resources and keep experimenting. Soon, you’ll be able to create and run your own projects with ease. Happy coding!
Frequently Asked Questions
What is a local server?
A local server is a server that runs on your own computer. It helps you test websites or applications without needing the internet.
Why should I use a local server?
Using a local server allows you to develop and test your projects quickly and safely before sharing them online.
What tools do I need to set up a local server?
You will need software like Node.js, a code editor, and possibly a web server like Nginx.
How do I install Node.js?
You can download Node.js from its official website and follow the installation instructions for your operating system.
What is PM2 and why is it useful?
PM2 is a tool that helps manage Node.js applications. It can restart your app if it crashes and helps keep it running smoothly.
How do I set up Nginx as a reverse proxy?
To set up Nginx, you need to install it and create a configuration file that directs traffic to your Node.js application.
How can I secure my local server?
You can secure your server by setting up a firewall, using HTTPS, and keeping your software up to date.
What should I do if my server has problems?
You can use debugging tools to find issues, check logs for errors, and test your application locally to troubleshoot.