Mastering the Art of Building Apps with Vite: A Comprehensive Guide
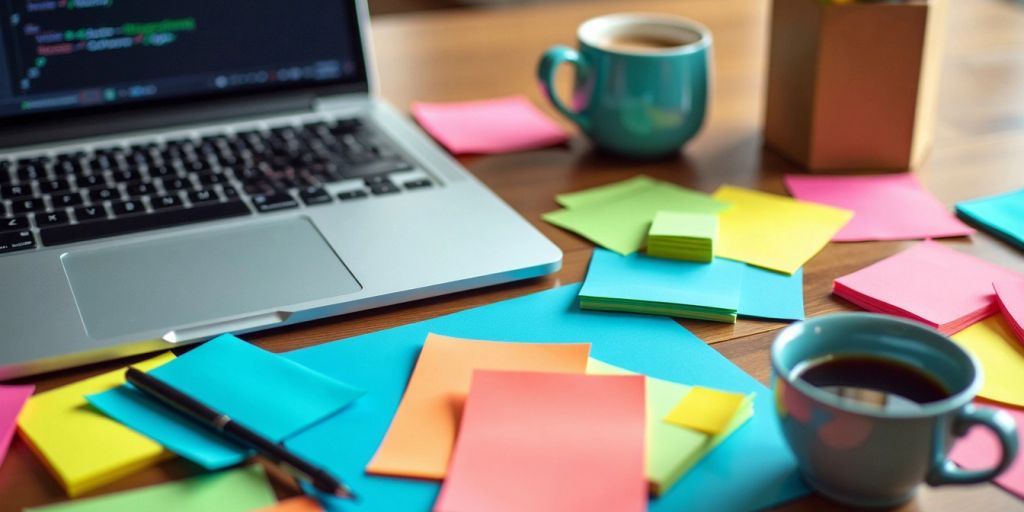
In this guide, we will explore how to effectively use Vite to build applications. Vite is a modern tool that makes developing web apps easier and faster. Whether you are a beginner or have some experience, this guide will help you understand Vite’s features and how to use them in your projects.
Key Takeaways
- Vite is a fast and efficient tool for building web applications.
- It simplifies the setup process for various frameworks like React and Vue.
- Vite supports plugins that can enhance your development experience.
- Understanding Vite’s core concepts can help you troubleshoot issues easily.
- Following best practices can improve your app’s performance and maintainability.
Getting Started with Vite
Introduction to Vite
Vite is a modern build tool that makes developing applications easier and faster. It provides a smooth experience with features like hot module replacement and fast builds. Vite is designed to work with various frameworks, making it a versatile choice for developers.
Setting Up Your Development Environment
To get started with Vite, you need to set up your development environment. Here are the steps:
- Install Node.js: Make sure you have Node.js installed on your computer. You can download it from the official website.
- Open your terminal: This is where you will run commands to create your Vite project.
- Install Vite: You can do this by running the command:
npm create vite@latest
This command will guide you through the setup process.
Creating Your First Vite Project
Now that your environment is ready, let’s create your first Vite project. Here’s how:
- Run the command: In your terminal, type the following command:
npx create-vite your-project-name
Replace
your-project-name
with the name you want for your project. - Follow the prompts: The setup will ask you some questions to configure your project.
- Navigate to your project folder: Use the command:
cd your-project-name
- Install dependencies: Run:
npm install
- Start the development server: Finally, run:
npm run dev
This will start your Vite project, and you can view it in your browser at
http://localhost:5173
.
Vite makes it easy to get started with modern web development. Just follow the steps above, and you’ll be up and running in no time!
Understanding Vite’s Core Concepts
How Vite Works
Vite is a modern build tool that makes developing applications easier and faster. It uses a unique approach by serving files over native ESM (ECMAScript Modules). This means that instead of bundling everything upfront, Vite only processes files when they are requested. This results in much quicker startup times.
Key Features of Vite
Vite comes with several important features that enhance the development experience:
- Fast Hot Module Replacement (HMR): Changes are reflected instantly in the browser without a full reload.
- Optimized Build: Vite uses Rollup under the hood for production builds, ensuring efficient code splitting and tree-shaking.
- Rich Plugin Ecosystem: Vite supports a variety of plugins to extend its functionality.
Comparing Vite with Other Build Tools
When comparing Vite to other build tools like Webpack or Parcel, several differences stand out:
Feature | Vite | Webpack | Parcel |
---|---|---|---|
Development Speed | Very Fast | Moderate | Fast |
Configuration | Minimal | Complex | Simple |
Hot Module Replacement | Yes | Yes | Yes |
Build Performance | Excellent | Good | Good |
Vite’s design focuses on speed and simplicity, making it a great choice for modern web development.
In summary, understanding Vite’s core concepts is essential for leveraging its full potential in your projects. By grasping how it works, its key features, and how it compares to other tools, you can make informed decisions when building applications.
Building a React Application with Vite
Installing React with Vite
To start building a React app with Vite, you need to follow a few simple steps. Vite makes it easy to set up a React project quickly. Here’s how:
- Install Node.js: If you haven’t installed Node.js, download it from the official website.
- Replace
your-project-name
with your desired project name. - Navigate to the Project Directory: Change to your project folder:
- After running these commands, your React app should be live on a local server, usually at
http://localhost:5173
.
Configuring React in Vite
Once your project is set up, you can start customizing it. Here are some key points to consider:
- Folder Structure: Organize your components in a way that makes sense for your project.
- State Management: Decide how you will manage state in your app (e.g., using Context API, Redux, etc.).
- Routing: If your app has multiple pages, consider using React Router for navigation.
Running Your React App
To see your app in action, simply run the development server. You can make changes to your code, and Vite will automatically refresh the page to reflect those changes. This feature is called Hot Module Replacement (HMR), and it makes development much smoother.
Vite provides a fast and efficient way to build React applications, allowing developers to focus on writing code rather than configuration.
By following these steps, you can easily set up and run a React application using Vite, making your development process faster and more enjoyable.
Advanced Vite Configuration
Customizing Vite Configurations
To make your Vite project truly yours, you can customize its configurations. Here are some key areas to focus on:
- Entry Points: Define where your application starts.
- Output Settings: Control how and where your files are generated.
- Plugins: Enhance functionality with various plugins.
Using Vite Plugins
Vite supports a variety of plugins to extend its capabilities. Some popular plugins include:
- Vite Plugin React: For React applications.
- Vite Plugin Vue: For Vue.js projects.
- Vite Plugin PWA: To add Progressive Web App features.
Optimizing Build Performance
To ensure your Vite application runs smoothly, consider these optimization techniques:
- Code Splitting: Break your code into smaller chunks to load only what’s needed.
- Lazy Loading: Load components only when they are required.
- Minification: Reduce file sizes for faster loading times.
Remember, optimizing your build can significantly improve your app’s performance and user experience.
For a more advanced setup, you might want to look into configuring Vitest. To create a vitest configuration file, follow the guide. Make sure you understand how vitest config resolution works before proceeding.
Using Vite with Other Frameworks
Building Vue.js Apps with Vite
Vite is a fantastic tool for building Vue.js applications. It offers a smooth setup and fast development experience. To get started:
- Install Vite with Vue template:
npm create vite@latest my-vue-app --template vue
- Navigate to your project directory:
cd my-vue-app
- Install dependencies and run the app:
npm install npm run dev
Integrating Svelte with Vite
Svelte is another popular framework that works well with Vite. Here’s how to set it up:
- Create a new Svelte project using Vite:
npm create vite@latest my-svelte-app --template svelte
- Move into your project folder:
cd my-svelte-app
- Install the necessary packages and start the server:
npm install npm run dev
Using Vite with Preact
Preact is a lightweight alternative to React, and it can also be used with Vite. Follow these steps:
- Set up a new Preact project:
npm create vite@latest my-preact-app --template preact
- Change to the project directory:
cd my-preact-app
- Install dependencies and launch the app:
npm install npm run dev
Vite makes it easy to work with different frameworks, allowing developers to choose the best tools for their projects. This flexibility is one of Vite’s key strengths.
Managing State in Vite Projects
State Management with Redux
Managing state in a Vite project can be done effectively using Redux. Redux is a predictable state container for JavaScript apps. Here are some key steps to get started:
- Install Redux: Use npm to add Redux to your project.
- Create a Store: Set up a store to hold your application’s state.
- Connect Components: Use the
connect
function to link your components to the Redux store.
Using Zustand for State Management
Zustand is another great option for state management in Vite projects. It is simpler and more lightweight than Redux. Here’s how to use it:
- Install Zustand: Add Zustand to your project using npm.
- Create a Store: Define a store with your state and actions.
- Use the Store: Access the store in your components easily.
Integrating MobX with Vite
MobX is a powerful library for state management that makes it easy to manage state in your applications. To integrate MobX:
- Install MobX: Add MobX to your project.
- Create Observables: Define observable state that your components can react to.
- Use MobX in Components: Use the
observer
function to make your components reactive.
Managing state effectively is crucial for building responsive applications. Choose the right tool based on your project needs and complexity.
State Management Tool | Pros | Cons |
---|---|---|
Redux | Predictable, scalable | Boilerplate code |
Zustand | Simple, minimal setup | Less community support |
MobX | Reactive, easy to use | Can be less predictable |
Testing and Debugging Vite Applications
Setting Up Testing Frameworks
To effectively test your Vite applications, you need to set up a testing framework. Here are some popular options:
- Jest: A widely-used testing framework that works well with Vite.
- Vitest: A Vite-native testing framework that offers fast performance.
- Mocha: A flexible testing framework that can be configured to work with Vite.
Writing Unit Tests
Writing unit tests is crucial for ensuring your application works as expected. Here’s how to get started:
- Create a test file: Place your test files in a
__tests__
directory. - Write test cases: Use your chosen framework’s syntax to write tests.
- Run tests: Execute your tests using the command line.
Debugging Vite Projects
Debugging is an essential part of development. Here are some tips:
- Use console.log statements to track variable values.
- Leverage the JavaScript Debug Terminal in VS Code. A quick way to debug tests in VS Code is via the JavaScript debug terminal. Open a new JavaScript debug terminal and run
npm run test
or Vitest directly. - Utilize browser developer tools to inspect elements and monitor network requests.
Debugging can be challenging, but with the right tools and techniques, you can quickly identify and fix issues in your Vite applications.
Deploying Vite Applications
Preparing for Deployment
Before you can deploy your Vite application, you need to prepare it for production. This involves building your project so that it can run efficiently on a server. Here are the steps to follow:
- Build your project: Run the command
npm run build
in your terminal. This will create adist
folder containing your production-ready files. - Check the output: Ensure that the
dist
folder has all the necessary files, includingindex.html
and any JavaScript or CSS files. - Test locally: You can use a simple server to test your built application locally before deploying it.
Deploying to Vercel
Vercel is a popular platform for deploying frontend applications. To deploy your Vite project on Vercel, follow these steps:
- Install Vercel CLI: If you haven’t already, install the Vercel CLI by running
npm i -g vercel
. - Deploy your project: Run
vercel
in your terminal. Follow the prompts to create your site, select a team if necessary, and optionally create a site name. - Visit your site: After deployment, Vercel will provide a URL where you can view your application.
Deploying to Netlify
Netlify is another great option for deploying Vite applications. Here’s how to do it:
- Create a Netlify account: Sign up for a free account on Netlify.
- Connect your repository: Link your GitHub or GitLab repository to Netlify.
- Set build settings: In the build settings, set the build command to
npm run build
and the publish directory todist
. - Deploy your site: Click on the deploy button, and your site will be live shortly.
Remember: Always test your application after deployment to ensure everything works as expected!
Best Practices for Building Apps with Vite
Code Splitting and Lazy Loading
To improve your app’s performance, consider using code splitting and lazy loading. This means loading only the parts of your app that are needed at the moment. Here are some tips:
- Use dynamic imports to load components only when they are needed.
- Split your code into smaller chunks to reduce the initial load time.
- Monitor your app’s performance using tools like Lighthouse.
Ensuring Code Quality
Maintaining high code quality is essential for any project. Here are some practices to follow:
- Use a linter to catch errors early.
- Write unit tests to ensure your code works as expected.
- Regularly review your code with peers to catch potential issues.
Maintaining Performance
Performance is key in app development. Here are some strategies:
- Optimize images and other assets to reduce load times.
- Use caching strategies to speed up repeated requests.
- Regularly analyze your app’s performance and make adjustments as needed.
Remember, performance matters. A fast app leads to happier users and better engagement.
Summary Table of Best Practices
Practice | Description |
---|---|
Code Splitting | Load only necessary parts of the app. |
Lazy Loading | Load components on demand. |
Code Quality | Use linters and write tests. |
Performance Optimization | Optimize assets and use caching. |
Troubleshooting Common Issues in Vite
When working with Vite, you might run into some common problems. Here’s how to tackle them effectively.
Resolving Dependency Conflicts
- Check your package versions: Make sure that you’re using a supported version of React (17.x or later).
- Update your dependencies: Run
npm update
to ensure all packages are up to date. - Clear cache: Sometimes, clearing the npm cache can resolve conflicts. Use
npm cache clean --force
.
Fixing Build Errors
- Read the error messages carefully: They often provide clues about what went wrong.
- Check your configuration: Ensure that your
vite.config.js
file is set up correctly. - Look for missing files: Make sure all necessary files are included in your project.
Handling Environment Variables
- Define your variables: Use a
.env
file to set environment variables. - Access them correctly: Remember to prefix your variables with
VITE_
to make them accessible in your code. - Restart your server: After making changes to the
.env
file, restart the Vite server to apply the updates.
Tip: Always keep your dependencies updated to avoid compatibility issues.
By following these steps, you can effectively troubleshoot and resolve common issues in your Vite projects. Happy coding!
Exploring the Vite Ecosystem
Popular Vite Plugins
Vite has a rich ecosystem of plugins that enhance its functionality. Here are some popular ones:
- Vite Plugin React: This plugin allows you to use React with Vite seamlessly.
- Vite Plugin Vue: Perfect for Vue.js applications, making integration smooth.
- Vite Plugin PWA: Helps in building Progressive Web Apps easily.
Community Resources
The Vite community is vibrant and supportive. Here are some resources to help you:
- Official Documentation: A great starting point for understanding Vite.
- GitHub Discussions: Engage with other developers and get your questions answered.
- YouTube Tutorials: Visual learners can find many helpful video guides.
Contributing to Vite
If you’re interested in contributing to Vite, here are some ways to get involved:
- Report Issues: Help improve Vite by reporting bugs you encounter.
- Submit Pull Requests: If you have a feature or fix, consider submitting it.
- Join the Community: Participate in discussions and share your knowledge.
Vite is a powerful alternative to create-react-app for React development. Explore Vite’s features to optimize your projects and enhance your development experience!
Dive into the exciting world of Vite! This powerful tool can help you build fast and efficient web applications. If you’re eager to learn more about how Vite can transform your coding experience, visit our website today and start your journey!
Conclusion
In conclusion, mastering app development with Vite can open up many doors for you as a developer. This guide has shown you the basics, from setting up your project to running your app. Vite makes it easy to create fast and efficient applications, allowing you to focus on writing great code. Remember, practice is key! Keep experimenting and building projects to improve your skills. With Vite, you have a powerful tool at your fingertips, so go ahead and create something amazing!
Frequently Asked Questions
What is Vite?
Vite is a modern tool that helps developers build web applications quickly. It makes development faster by using a special method to bundle code.
How do I start a project with Vite?
To start a project, you can use a command in your terminal. Just type ‘npx create-vite my-project –template react’ to create a new React project.
Can I use Vite with React?
Yes, Vite works great with React! It has templates that make it easy to set up React projects.
What are the benefits of using Vite?
Vite is known for its speed and efficiency. It allows for quick updates while you code, which makes it easier to see changes instantly.
How do I install Vite?
You can install Vite by running ‘npm install vite’ in your project folder. This will add Vite to your project.
What makes Vite different from other tools?
Vite is different because it uses a new way to bundle code that is faster than traditional methods. This makes it more efficient for developers.
Is Vite suitable for beginners?
Yes, Vite is beginner-friendly! It has clear instructions and templates that help new developers get started easily.
Where can I find help if I have issues with Vite?
If you run into problems, you can check the Vite documentation online or ask for help in community forums.