Mastering Swift Coding Interview Questions: Essential Tips and Resources for Success
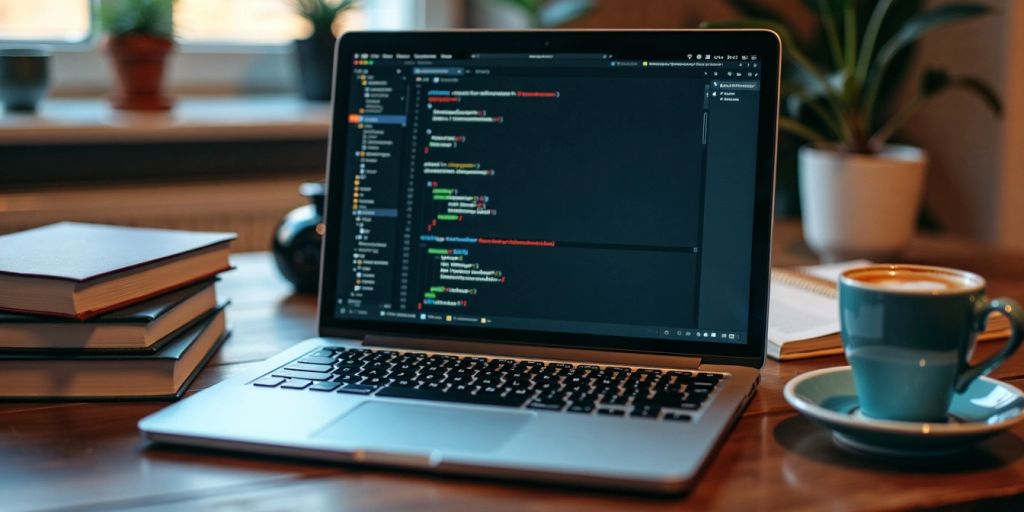
Preparing for Swift coding interviews can be a daunting task, but with the right strategies and resources, you can boost your confidence and skills. This article focuses on key areas that every aspiring Swift developer should master to excel in interviews. By understanding the basics, practicing essential features, and utilizing helpful tools, you can set yourself up for success in the competitive world of iOS development.
Key Takeaways
- Master the core concepts of Swift programming to build a solid foundation for interviews.
- Practice common interview questions to familiarize yourself with what employers are looking for.
- Understand how to navigate UI and Auto Layout, as these are critical for iOS app development.
- Learn about memory management and design patterns to enhance your coding skills and efficiency.
- Utilize various tools and resources to stay updated and improve your coding abilities.
Understanding the Basics of Swift Coding Interview Questions
Key Concepts in Swift Programming
To excel in Swift coding interviews, it’s crucial to grasp the key concepts of the language. Here are some fundamental ideas:
- Syntax and Semantics: Understand how Swift code is structured.
- Data Types: Familiarize yourself with common data types like Int, String, and Array.
- Control Flow: Learn how to use if statements, loops, and switch cases effectively.
Commonly Asked Swift Interview Questions
When preparing for interviews, you might encounter questions like:
- What is Swift?
- How do you manage memory in Swift?
- Can you explain Optionals?
- What are closures in Swift?
- How do you ensure thread safety?
These questions often focus on your understanding of the language and its features. For example, one common question is: "How familiar are you with the Swift programming language and how do you use it in your projects?" This helps interviewers gauge your practical experience.
Importance of Swift in iOS Development
Swift is not just a programming language; it’s the backbone of iOS development. Here’s why it matters:
- Performance: Swift is designed for speed and efficiency.
- Safety: The language helps prevent common programming errors.
- Community: A vibrant community supports developers with resources and tools.
Swift is essential for anyone looking to build applications in the Apple ecosystem. Understanding its core principles can significantly boost your confidence in interviews.
Essential Swift Language Features to Master
Control Flow and Access Modifiers
In Swift, control flow is essential for directing the execution of code. You can use:
- If statements to make decisions.
- Switch statements for multiple conditions.
- Loops like
for
,while
, andrepeat-while
to repeat actions.
Access modifiers help you control the visibility of your code components. The main types are:
- Public: Accessible from anywhere.
- Internal: Accessible within the same module.
- Private: Accessible only within the defining scope.
Understanding Type Safety and Optionals
Swift is a type-safe language, meaning it helps you catch errors at compile time. This reduces bugs and improves code quality. One of the key features is optionals, which allow variables to have a value or be nil
. This is crucial for avoiding crashes due to unexpected nil
values. Here’s a quick overview:
Type | Description |
---|---|
Optional | Can hold a value or nil |
Implicitly Unwrapped Optional | Assumed to have a value after being set once |
Non-Optional | Must always have a value |
Working with Structs and Classes
In Swift, you can create your own data types using structs and classes. Here are some differences:
- Structs are value types, meaning they are copied when assigned or passed.
- Classes are reference types, meaning they share a single instance.
Key Features of Swift include a modern and user-friendly syntax, strong type safety, and automatic memory management through Automatic Reference Counting (ARC). This makes Swift a powerful choice for iOS development.
Swift’s features not only enhance productivity but also ensure safer and more reliable code. Mastering these elements is crucial for any aspiring Swift developer.
Navigating UI and Auto Layout in Swift
Introduction to Auto Layout
Auto Layout is a powerful system that helps you create responsive user interfaces in iOS apps. It allows you to define rules for how your UI elements should be arranged and resized. This means your app can look great on any device, whether it’s an iPhone or an iPad.
UIKit Essentials for Swift Developers
UIKit is the framework that provides the necessary tools to build user interfaces in iOS. Here are some key components:
- UIView: The basic building block for all UI elements.
- UIViewController: Manages a view and its interactions.
- Auto Layout Constraints: Define how views relate to each other.
Creating Custom Views in Swift
Creating custom views can enhance your app’s user experience. Here’s how to get started:
- Subclass UIView: Create a new class that inherits from UIView.
- Override draw(): Customize the appearance of your view.
- Add Subviews: Use the addSubview method to include other UI elements.
Custom views can significantly improve the look and feel of your app, making it more engaging for users.
In summary, mastering Auto Layout and UIKit is essential for any Swift developer. By understanding these concepts, you can create beautiful and functional iOS applications. Remember, the key to great iOS user interfaces with Swift UI is practice and experimentation!
Concurrency and Threading in Swift
Basics of Threading in Swift
Concurrency in Swift allows multiple tasks to run at the same time. This is important for creating responsive applications. However, managing these tasks can introduce certain issues. Here are some key points to understand:
- Synchronous vs. Asynchronous: Synchronous tasks wait for one to finish before starting another, while asynchronous tasks can start and return control immediately.
- Thread Safety: Ensuring that shared resources are accessed safely to avoid problems like race conditions.
- Grand Central Dispatch (GCD): A powerful tool for managing concurrent tasks in Swift.
Concurrency Models and Best Practices
When working with concurrency, it’s essential to follow best practices to avoid common pitfalls. Here are some strategies:
- Use Serial Queues: This ensures that tasks are executed one at a time, preventing conflicts.
- Implement Barriers: In concurrent queues, barriers can help manage access to shared resources.
- Utilize Synchronization Tools: Tools like locks and semaphores can help control access to shared data.
Handling Asynchronous Code
Handling asynchronous code effectively is crucial for maintaining a smooth user experience. Here are some tips:
- Use Completion Handlers: These allow you to execute code after an asynchronous task finishes.
- Leverage Async/Await: This modern approach simplifies writing asynchronous code, making it easier to read and maintain.
- Test Thoroughly: Always test your asynchronous code to ensure it behaves as expected under different conditions.
In summary, mastering concurrency in Swift is vital for building efficient applications. Understanding how to manage tasks and resources will lead to better performance and user experience.
Memory Management in Swift
Memory management is crucial for building efficient applications in Swift. Automatic Reference Counting (ARC) is the primary method used to manage memory in Swift. It helps track how much memory your app is using and automatically frees up memory when it’s no longer needed.
Automatic Reference Counting (ARC)
ARC works by counting the number of references to each class instance. When the count drops to zero, meaning there are no more references to that instance, it is deallocated, and the memory can be reclaimed. Here are some key points about ARC:
- Strong References: These are the default type of reference. An instance will not be deallocated as long as there is at least one strong reference to it.
- Weak References: These references do not keep a strong hold on the instance, allowing ARC to deallocate it if there are no strong references. This is useful to avoid retain cycles.
- Unowned References: Similar to weak references, but they are expected to always have a value. Use these when you are sure the reference will not become nil.
Avoiding Memory Leaks
To prevent memory leaks, consider the following:
- Use weak references in parent-child relationships to avoid retain cycles.
- Be cautious with closures that capture references; use weak or unowned references where necessary.
- Regularly check for memory leaks using tools like Instruments.
Managing memory effectively is essential for app performance and stability.
Best Practices for Efficient Memory Management
- Always prefer weak references when dealing with delegate patterns.
- Use unowned references when you are certain the instance will not be nil.
- Regularly profile your app to identify and fix memory issues.
By understanding and applying these concepts, you can navigate the challenges of iOS memory management effectively, ensuring your applications run smoothly and efficiently.
Design Patterns Every Swift Developer Should Know
Model-View-Controller (MVC)
The Model-View-Controller (MVC) pattern is one of the most recognized design patterns in Swift. It helps separate the application into three main components:
- Model: This part handles the data and business logic.
- View: This is what the user sees and interacts with.
- Controller: It connects the Model and View, managing the flow of data.
Model-View-ViewModel (MVVM)
The Model-View-ViewModel (MVVM) pattern is another popular choice. It enhances the separation of concerns by introducing a ViewModel that:
- Holds the data for the View.
- Handles the logic to prepare data for display.
- Allows for easier testing and maintenance.
Protocol-Oriented Programming
Swift encourages Protocol-Oriented Programming, which focuses on using protocols to define methods and properties. This approach:
- Promotes code reusability.
- Enhances flexibility in your code.
- Makes it easier to manage changes.
Understanding these design patterns is crucial for building robust iOS applications. They help in organizing code, making it easier to maintain and scale.
Summary of Key Design Patterns
Design Pattern | Description |
---|---|
MVC | Separates data, UI, and logic. |
MVVM | Enhances MVC with a ViewModel. |
Protocol-Oriented | Focuses on protocols for flexibility. |
By mastering these patterns, you can improve your coding skills and prepare for Swift coding interviews effectively.
Testing Swift Applications
Unit Testing with XCTest
Testing is a crucial part of software development. XCTest is the main framework used for unit testing in Swift. It helps developers ensure that their code works as expected. Here’s a simple example of a unit test:
import XCTest
@testable import MyApp
class MyAppTests: XCTestCase {
func testExample() {
let calculator = Calculator()
let result = calculator.add(2, 3)
XCTAssertEqual(result, 5, "The calculator should return 5 for 2 + 3")
}
}
Behavior-Driven Development with Quick/Nimble
Another popular approach is using Quick and Nimble. These frameworks allow for a more readable and expressive way to write tests. They help in writing tests that are easy to understand and maintain.
Performance Profiling and Memory Leak Detection
To keep your app running smoothly, it’s important to check for performance issues and memory leaks. Tools like Instruments can help you find and fix these problems. Here are some common tools used for testing Swift applications:
Tool/Framework | Purpose |
---|---|
XCTest | Unit and UI testing |
Quick/Nimble | Behavior-driven development |
TestFlight | Beta testing with real users |
SwiftLint | Enforcing coding style |
Instruments | Performance profiling |
Testing your Swift applications is essential for maintaining quality and ensuring that your code meets the required standards.
Summary
In summary, testing is vital for any Swift developer. Here are some key points to remember:
- Use XCTest for unit tests.
- Explore Quick and Nimble for behavior-driven tests.
- Regularly check for performance issues using Instruments.
- Always aim for best practices in your coding to avoid common pitfalls like memory leaks and poor performance.
Performance Optimization in Swift
Identifying Common Performance Issues
Performance issues can slow down your Swift applications. Here are some common problems:
- Memory Leaks: These occur when your app uses more memory than it should, which can lead to crashes.
- Synchronous Operations: Running heavy tasks on the main thread can make your app feel sluggish.
- Poor Concurrency Management: This can cause race conditions or deadlocks, making your app unresponsive.
Optimizing Code for Speed
To improve performance, consider these strategies:
- Use Instruments: Track down memory leaks and fix retain cycles by using weak references.
- Offload Tasks: Move long-running tasks off the main thread using Grand Central Dispatch (GCD).
- Profile Your Code: Identify bottlenecks and refactor your code to use more efficient algorithms.
Efficient Use of Resources
Managing resources wisely is key to performance:
- Optimize UI Updates: Only redraw views when necessary to avoid unnecessary processing.
- Compress Assets: Use smaller images and load resources only when needed.
- Asynchronous Loading: Load large files in the background to keep the UI responsive.
Remember: Regularly profiling and optimizing your code can lead to significant performance improvements.
Summary Table of Performance Tips
Issue | Solution |
---|---|
Memory Leaks | Use weak references |
Main Thread Blockage | Use GCD for heavy tasks |
Inefficient Algorithms | Profile and refactor code |
Preparing for a Swift Coding Interview
Reviewing Key Swift Concepts
To get ready for your Swift coding interview, it’s important to review key concepts. Focus on:
- Swift syntax and language features
- Memory management techniques
- Common design patterns like MVC and MVVM
Practicing Coding Challenges
Practicing coding challenges is essential. Here are some platforms to consider:
- LeetCode
- HackerRank
- CodeSignal
These platforms offer a variety of problems that can help you sharpen your skills.
Mock Interviews and Real-World Scenarios
Mock interviews can help you feel more comfortable. Try to:
- Simulate real interview conditions
- Get feedback from peers or mentors
- Discuss your past projects to showcase your experience
Preparing well can make a big difference in your confidence and performance during the interview.
By focusing on these areas, you can enhance your chances of success in your upcoming interview. Remember, essential iOS interview questions often cover a range of topics, so be ready to demonstrate your knowledge and skills!
Advanced Swift Topics for Experienced Developers
Generics and Higher-Order Functions
Generics allow you to write flexible and reusable code. They enable you to create functions and types that can work with any data type. Higher-order functions like map
, filter
, and reduce
are essential for functional programming in Swift. Here are some key points:
- Generics help avoid code duplication.
- Higher-order functions can simplify complex operations.
- They enhance code readability and maintainability.
Advanced Error Handling
Swift provides powerful error handling capabilities. You can use do-catch
blocks to manage errors effectively. Here’s how to handle errors:
- Use
throws
to indicate a function can throw an error. - Use
do
to call the function andcatch
to handle errors. - Always provide meaningful error messages.
Swift Package Manager and Modular Code
The Swift Package Manager (SPM) is a tool for managing the distribution of Swift code. It helps in creating modular code, making it easier to manage dependencies. Here are some benefits of using SPM:
- Simplifies dependency management.
- Encourages code reuse across projects.
- Supports versioning for better stability.
Mastering these advanced topics can significantly improve your coding skills and prepare you for top interview questions for senior iOS developers in 2024. Understanding concepts like protocol-oriented programming and generics is crucial for success in the Swift ecosystem.
Utilizing Tools and Resources for Swift Development
Essential Tools like Xcode and Swift Playgrounds
When developing in Swift, Xcode is the primary tool used by developers. It provides a complete environment for coding, debugging, and testing your applications. Another great tool is Swift Playgrounds, which is perfect for learning and experimenting with Swift code in a fun way. Here are some key tools:
- Xcode: Integrated development environment (IDE) for macOS.
- Swift Playgrounds: An app for learning Swift through interactive puzzles.
- TestFlight: For beta testing your apps with real users.
Leveraging Online Coding Platforms
Practicing coding is crucial for mastering Swift. Here are some popular platforms:
- LeetCode: Great for coding challenges and interview prep.
- HackerRank: Offers a variety of coding problems to solve.
- Codewars: A fun way to improve your coding skills through challenges.
Staying Updated with Swift Communities
Being part of the Swift community can help you stay informed about the latest trends and updates. Here are some ways to engage:
- Join forums like Swift Forums to discuss topics with other developers.
- Follow Swift-related blogs and podcasts for insights.
- Participate in local meetups or online webinars to network and learn.
Staying connected with the community can provide you with valuable insights and support as you grow your skills in Swift development.
In summary, utilizing the right tools and resources is essential for success in Swift development. By mastering these tools, you can enhance your coding skills and prepare effectively for interviews. Find the best Swift IDEs and code editors for iOS app development to streamline your workflow and improve your coding experience.
If you’re eager to enhance your coding skills and land your dream job, visit our website today! We offer free coding lessons that can help you get started on your journey. Don’t wait—take the first step towards success now!
Final Thoughts on Swift Interview Success
In conclusion, preparing for Swift coding interviews can be a rewarding journey. By focusing on the key areas like language features, UI design, and memory management, you can build a strong foundation. Remember to practice common interview questions and work on your problem-solving skills. Utilize resources like the Swift Interview Questions app to enhance your learning. With dedication and the right tools, you can approach your interviews with confidence and increase your chances of landing that dream job in iOS development.
Frequently Asked Questions
What is Swift and why is it important?
Swift is a programming language made by Apple for building apps on iOS and other Apple devices. It’s important because it’s fast and easy to use, helping developers create better apps.
What are some basic concepts I should know in Swift?
You should understand variables, constants, functions, and control flow. These are the building blocks of writing code in Swift.
How can I prepare for a Swift coding interview?
Practice common interview questions, work on coding challenges, and review key Swift concepts to boost your confidence.
What is Auto Layout in Swift?
Auto Layout is a system used to design the layout of user interfaces in apps. It helps ensure that your app looks good on all screen sizes.
What is ARC in Swift?
ARC stands for Automatic Reference Counting. It’s a way Swift manages memory automatically, helping to prevent memory leaks.
What design patterns should I know for Swift development?
You should be familiar with Model-View-Controller (MVC) and Model-View-ViewModel (MVVM). These patterns help organize your code.
How do I test my Swift applications?
You can use XCTest, which is a framework provided by Apple, to write tests for your code and make sure everything works as expected.
What tools are essential for Swift development?
Some important tools are Xcode for coding and Swift Playgrounds for learning. These help you write and test your Swift code easily.