Mastering Recursion Problems for Coding Interviews: A Comprehensive Guide to Ace Your Next Interview
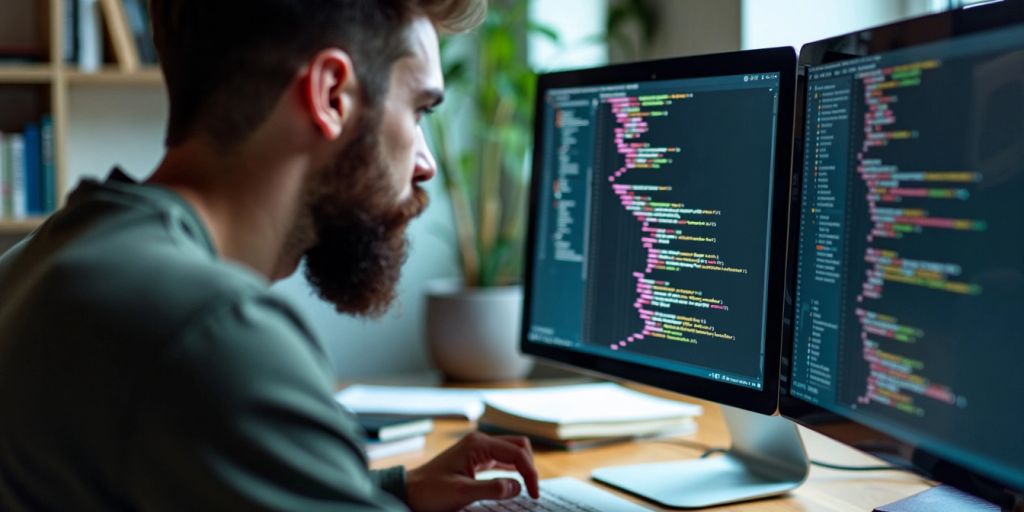
Recursion can seem tricky, but it’s a powerful tool in programming. This guide will help you understand recursion, why it’s important for coding interviews, and how to tackle common problems. Whether you’re just starting out or looking to sharpen your skills, this comprehensive guide will prepare you for success in your next coding interview.
Key Takeaways
- Recursion is a method where a function calls itself to solve smaller parts of a problem.
- It’s crucial for coding interviews as many problems can be efficiently solved using recursion.
- Understanding the call stack is essential for grasping how recursion works.
- Common recursive problems include the Fibonacci sequence and tree traversals.
- Practicing recursion can boost your problem-solving skills and prepare you for real interview scenarios.
Understanding the Basics of Recursion
What is Recursion?
Recursion is a method where a function calls itself to solve a problem. It’s a powerful tool that can simplify complex tasks by breaking them down into smaller, more manageable parts. For example, when calculating the factorial of a number, the function can call itself with a smaller number until it reaches the base case.
How Recursion Works in Programming
In programming, recursion works through a call stack. Each time a function calls itself, a new layer is added to the stack. This continues until the base case is reached, at which point the stack starts to unwind, returning values back through the layers. Here’s a simple breakdown of how it operates:
- Function Call: The function is called with an initial value.
- Base Case: The function checks if it has reached the base case.
- Recursive Call: If not, it calls itself with a modified value.
- Return: Once the base case is reached, values are returned back through the stack.
Common Misconceptions About Recursion
Many people think recursion is only for advanced programmers, but that’s not true. Here are some common misconceptions:
- Recursion is always better: Sometimes, iterative solutions are more efficient.
- It’s too complex: With practice, recursion can be understood easily.
- You need to use it for every problem: Not every problem requires recursion; sometimes, simpler solutions exist.
Recursion can seem tricky at first, but with practice, it becomes a valuable skill in your programming toolkit. Understanding the basics is the first step to mastering it.
Summary
In summary, recursion is a method of solving problems by having functions call themselves. It’s essential to understand how it works and to recognize when to use it. By grasping these concepts, you’ll be better prepared for coding interviews and real-world programming challenges. Remember, recursion is a tool that can simplify your coding tasks when used appropriately.
Why Recursion is Important for Coding Interviews
The Role of Recursion in Problem-Solving
Recursion is a powerful tool in programming that helps solve complex problems by breaking them down into smaller, more manageable parts. Many coding challenges require a solid understanding of recursion. Here are some key points about its role in problem-solving:
- It simplifies code by reducing the need for loops.
- It allows for elegant solutions to problems like tree traversal.
- It can be more intuitive for certain algorithms, making them easier to understand.
Recursion vs. Iteration in Interviews
In coding interviews, candidates often face questions that can be solved using either recursion or iteration. Here’s a quick comparison:
Aspect | Recursion | Iteration |
---|---|---|
Readability | Often clearer for complex tasks | Can be more straightforward |
Performance | May use more memory | Generally more efficient |
Use Cases | Best for tree and graph problems | Suitable for simple loops |
When to Use Recursion in Coding Challenges
Knowing when to apply recursion is crucial. Here are some scenarios:
- When the problem can be divided into similar subproblems.
- When the solution involves exploring multiple paths, like in backtracking.
- When working with data structures like trees or graphs.
Recursion can seem daunting, but with practice, it becomes a valuable skill in your coding toolkit. Understanding its principles can help you tackle many coding challenges effectively.
In summary, mastering recursion is essential for coding interviews. It not only enhances your problem-solving skills but also prepares you for a variety of technical challenges. Remember, the first reason why a lot of people find coding interviews hard is because they lack the fundamental knowledge in data structures and algorithms.
Classic Recursion Problems and Solutions
The Tower of Hanoi
The Tower of Hanoi is a classic problem that involves moving disks from one peg to another, following specific rules. This problem is a must-do coding question for companies like Amazon. Here’s how it works:
- Move the top n-1 disks from the source peg to an auxiliary peg.
- Move the nth disk directly to the destination peg.
- Finally, move the n-1 disks from the auxiliary peg to the destination peg.
The Fibonacci Sequence
The Fibonacci sequence is another well-known recursion problem. It generates a series where each number is the sum of the two preceding ones. The sequence starts with 0 and 1. Here’s a simple way to calculate it:
- Base Case: If n is 0, return 0. If n is 1, return 1.
- Recursive Case: Return Fibonacci(n-1) + Fibonacci(n-2).
The Flood Fill Algorithm
The Flood Fill algorithm is used in graphics applications, like paint bucket tools. It fills a connected area with a specific color. Here’s a brief overview of how it works:
- Check if the current pixel is the target color.
- Change the color of the current pixel.
- Recursively call the function for adjacent pixels (up, down, left, right).
Recursion can seem tricky at first, but with practice, it becomes a powerful tool for solving complex problems.
By mastering these classic recursion problems, you’ll be well-prepared for coding interviews and can tackle a variety of challenges with confidence.
Advanced Recursion Techniques
Backtracking Algorithms
Backtracking is a powerful technique used to solve problems incrementally. It builds candidates for solutions and abandons them if they fail to satisfy the conditions of the problem. Here are some key points about backtracking:
- Explores all possibilities: It tries every option until it finds a solution.
- Prunes unnecessary paths: If a path doesn’t lead to a solution, it stops exploring that path.
- Commonly used in puzzles: Problems like Sudoku and the N-Queens problem are classic examples.
Divide-and-Conquer Strategies
Divide-and-conquer is another important technique that breaks a problem into smaller subproblems, solves each one, and combines the results. Here’s how it works:
- Divide: Split the problem into smaller parts.
- Conquer: Solve each part recursively.
- Combine: Merge the solutions to get the final answer.
This method is often used in algorithms like Merge Sort and Quick Sort.
Dynamic Programming with Recursion
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It is particularly useful when the same subproblems are solved multiple times. Here are some techniques:
- Memoization: Store results of expensive function calls and reuse them when the same inputs occur again.
- Tabulation: Build a table in a bottom-up manner to store results of subproblems.
- Optimal substructure: Ensure that the optimal solution of the problem can be constructed from optimal solutions of its subproblems.
Recursion can be a powerful tool, but it’s essential to know when to use it. Understanding these advanced techniques can help you tackle complex problems more effectively.
In summary, mastering these advanced recursion techniques can significantly enhance your problem-solving skills. Whether you’re tackling coding interviews or real-world programming challenges, these strategies will be invaluable. Familiarity with these methods can set you apart in interviews, especially when discussing the top 50 array coding problems for interviews.
Optimizing Recursive Algorithms
Memoization Techniques
Memoization is a powerful technique that helps speed up recursive algorithms by storing the results of expensive function calls and reusing them when the same inputs occur again. This can significantly reduce the time complexity of algorithms that have overlapping subproblems. Here are some key points about memoization:
- Store results: Keep a record of previously computed results.
- Check before computing: Before performing a calculation, check if the result is already stored.
- Use data structures: Commonly, dictionaries or arrays are used to store results.
Tail Call Optimization
Tail call optimization is a method that allows some recursive functions to be executed without adding a new stack frame for each call. This can prevent stack overflow errors and improve performance. Here’s how it works:
- Identify tail calls: A tail call is the last operation in a function.
- Reuse stack frame: Instead of creating a new stack frame, reuse the current one.
- Language support: Not all programming languages support this optimization, so check your language’s documentation.
Avoiding Stack Overflow
Stack overflow can occur when a recursive function calls itself too many times without reaching a base case. To avoid this:
- Set a base case: Always define a clear base case to stop recursion.
- Limit recursion depth: Use iterative solutions when possible for deep recursions.
- Monitor stack size: Be aware of the maximum stack size in your programming environment.
Optimizing recursive algorithms is crucial for efficient programming. By using techniques like memoization and tail call optimization, you can make your recursive solutions faster and safer. Understanding these methods will help you tackle complex problems more effectively.
Recursion in Data Structures
Tree Traversal Algorithms
Recursion is a powerful tool for navigating tree structures. Here are some common traversal methods:
- Pre-order Traversal: Visit the root, then the left subtree, followed by the right subtree.
- In-order Traversal: Visit the left subtree first, then the root, and finally the right subtree.
- Post-order Traversal: Visit the left subtree, then the right subtree, and finally the root.
These methods help in processing each node in a structured way. Understanding these algorithms is crucial for solving many coding problems.
Graph Traversal with Recursion
Graphs can also be traversed using recursion. The two main methods are:
- Depth-First Search (DFS): Explore as far as possible along each branch before backtracking.
- Breadth-First Search (BFS): Visit all the neighbors of a node before moving to the next level.
Recursion simplifies the implementation of DFS, making it easier to manage the call stack.
Using Recursion in Linked Lists
Recursion can be applied to linked lists for various operations:
- Reversing a Linked List: Swap the pointers recursively.
- Finding the Middle Element: Use two pointers, one moving fast and the other slow.
- Detecting Cycles: Use a recursive approach to check if a node points back to a previous node.
Recursion can make complex problems easier to solve by breaking them down into smaller parts. It’s essential to define a base case to prevent infinite loops.
In summary, recursion is a vital technique in data structures, allowing for elegant solutions to complex problems. By mastering these concepts, you can enhance your problem-solving skills for coding interviews.
Practical Applications of Recursion
File System Navigation
Recursion is often used to navigate through file systems. When you want to list all files in a directory and its subdirectories, a recursive function can help. Here’s how it works:
- Start at the main directory.
- List all files in that directory.
- For each subdirectory, repeat the process.
This method is efficient for exploring complex directory structures.
Generating Permutations and Combinations
Recursion is a powerful tool for generating permutations and combinations. Here’s a simple breakdown:
- Permutations: Arranging items in different orders.
- Combinations: Selecting items without regard to order.
- Recursive functions can easily handle these tasks by breaking them down into smaller problems.
Drawing Fractals with Recursion
Fractals are complex patterns that can be created using recursive algorithms. For example:
- Start with a simple shape.
- Repeat the process of adding smaller shapes to create a complex design.
- Each iteration adds more detail, resulting in beautiful patterns.
Recursion allows us to solve problems by breaking them down into smaller, manageable parts, making it a valuable skill in programming.
Summary Table of Applications
Application | Description |
---|---|
File System Navigation | Explore directories and subdirectories recursively |
Generating Permutations | Create different arrangements of items |
Drawing Fractals | Build complex patterns through repeated shapes |
Common Pitfalls and How to Avoid Them
Overcomplicating Solutions
One of the biggest mistakes in coding interviews is overcomplicating solutions. When faced with a problem, it’s easy to think of complex ways to solve it. Instead, try to keep your approach simple. Here are some tips to avoid this pitfall:
- Break the problem down into smaller parts.
- Use straightforward algorithms first.
- Always ask yourself if there’s a simpler way to achieve the same result.
Performance Issues
Another common issue is not considering performance. Your solution might work, but it could be slow. To avoid this:
- Analyze the time and space complexity of your solution.
- Test your code with large inputs to see how it performs.
- Optimize your code by removing unnecessary calculations.
Debugging Recursive Functions
Debugging can be tricky, especially with recursive functions. Here are some strategies to help:
- Use print statements to track the flow of your recursion.
- Check for base cases to avoid infinite loops.
- Test your function with simple inputs before using complex ones.
Remember, coding interviews are not just about finding the right answer; they are also about showing your thought process and problem-solving skills.
By being aware of these common pitfalls, you can improve your chances of success in coding interviews. Always practice and refine your approach to become a better coder!
Preparing for Recursion Questions in Interviews
Studying Common Recursion Problems
To get ready for recursion questions in coding interviews, it’s essential to study common problems. Here are some key areas to focus on:
- Understand the basics: Make sure you know what recursion is and how it works.
- Practice classic problems: Work on problems like the Fibonacci sequence, Tower of Hanoi, and more.
- Review sample questions: Look at 6 recursion interview questions with sample answers to learn how to tackle similar questions.
Practicing with Online Judges
Using online platforms can help you sharpen your skills. Here are some popular ones:
- LeetCode: Offers a wide range of recursion problems.
- HackerRank: Great for practicing coding challenges.
- CodeSignal: Provides a fun way to practice coding skills.
Mock Interviews and Feedback
Mock interviews can be a game-changer. Here’s how to make the most of them:
- Partner up: Find a friend to practice with.
- Simulate real interviews: Time yourself and answer questions as if you were in an actual interview.
- Seek feedback: After each mock interview, discuss what went well and what could be improved.
Practicing regularly will help you feel more confident and prepared for your coding interviews. Don’t underestimate the power of practice!
Comparing Recursion Across Programming Languages
Recursion in Python
Python is known for its simplicity and readability, making it a great language for learning recursion. Here are some key points about recursion in Python:
- Easy to understand: Python’s syntax is straightforward, which helps beginners grasp recursive concepts quickly.
- Limitations: Python has a default recursion limit (usually 1000), which can lead to a
RecursionError
if exceeded. - Use cases: Commonly used for problems like tree traversals and combinatorial problems.
Recursion in JavaScript
JavaScript also supports recursion, and it is widely used in web development. Here are some highlights:
- Flexible: JavaScript allows for both functional and object-oriented programming styles, making recursion versatile.
- Tail call optimization: Some JavaScript engines support tail call optimization, which can help prevent stack overflow in certain cases.
- Common applications: Often used in algorithms for manipulating arrays and objects.
Recursion in Other Popular Languages
Many programming languages support recursion, but they may have different characteristics. Here’s a quick comparison:
Language | Recursion Support | Tail Call Optimization | Common Use Cases |
---|---|---|---|
C | Yes | No | Algorithms, data structures |
Java | Yes | No | Tree structures, sorting |
Ruby | Yes | Yes | Web applications, data processing |
Recursion is a powerful tool, but it’s important to know when to use it. Understanding the differences in how various languages handle recursion can help you choose the right approach for your coding challenges.
In summary, while recursion is a common concept across programming languages, each language has its own quirks and limitations. Knowing these can help you become a better programmer and ace your coding interviews!
Real-World Examples of Recursion
Recursion in Web Development
Recursion is often used in web development for tasks like navigating through nested structures. For example, when dealing with a complex menu system, recursion can help traverse through multiple levels of menus. Here are some common uses:
- Dynamic Menu Generation: Creating menus that can expand and collapse.
- Nested Comments: Displaying comments in a threaded format.
- Data Fetching: Recursively fetching data from APIs that return nested objects.
Recursion in Game Development
In game development, recursion can simplify complex tasks. Some examples include:
- Pathfinding Algorithms: Finding the best route in a game map.
- Game State Management: Managing different states of a game using recursive functions.
- AI Behavior Trees: Implementing decision-making processes for non-player characters (NPCs).
Recursion in Data Analysis
Data analysis often involves recursive techniques to process large datasets. Key applications include:
- Hierarchical Data Processing: Analyzing data that has a parent-child relationship, like organizational charts.
- Fractal Analysis: Studying patterns that repeat at different scales.
- Recursive Functions for Aggregation: Summarizing data through recursive calculations.
Recursion is a powerful tool that can simplify complex problems, making it easier to manage and understand data structures.
By understanding these real-world applications, you can see how recursion is not just a theoretical concept but a practical tool in various fields.
Recursion is a powerful tool in programming that can solve complex problems by breaking them down into smaller, more manageable parts. For instance, think about how a family tree is built: each person can have parents, and those parents can have their own parents, creating a recursive structure. If you want to dive deeper into coding and learn how to tackle challenges like this, visit our website and start coding for free today!
Final Thoughts on Recursion
In conclusion, mastering recursion is a valuable skill for anyone preparing for coding interviews. While it may seem tough at first, with practice and the right resources, you can become confident in using recursive techniques. Remember, recursion is not just about solving problems; it’s about understanding when to use it effectively. By practicing different recursion problems and learning from examples, you’ll be better equipped to tackle any coding challenge that comes your way. So keep practicing, stay curious, and you’ll ace your next interview!
Frequently Asked Questions
What does recursion mean in programming?
Recursion is when a function calls itself to solve a problem. It breaks a big problem into smaller, similar problems until it reaches a simple case.
Why is recursion important in coding interviews?
Many coding challenges require you to think in a recursive way. Understanding recursion helps you solve complex problems more easily.
What are some common recursion problems?
Classic examples include the Tower of Hanoi, calculating Fibonacci numbers, and flood fill algorithms.
How can I improve my recursive algorithms?
You can use techniques like memoization to save results of expensive function calls and avoid repeating the same work.
What is the difference between recursion and iteration?
Recursion involves a function calling itself, while iteration uses loops to repeat actions. Each has its own advantages depending on the problem.
When should I use recursion?
Use recursion when a problem can be broken down into smaller, similar subproblems, especially in tree or graph traversal.
What are some pitfalls to avoid with recursion?
Common mistakes include creating overly complex solutions, running into performance issues, and not handling base cases properly.
How can I prepare for recursion questions in interviews?
Practice common recursion problems, use online coding platforms, and participate in mock interviews to build your confidence.