Mastering React for Beginners: A Step-by-Step Guide to Your First App
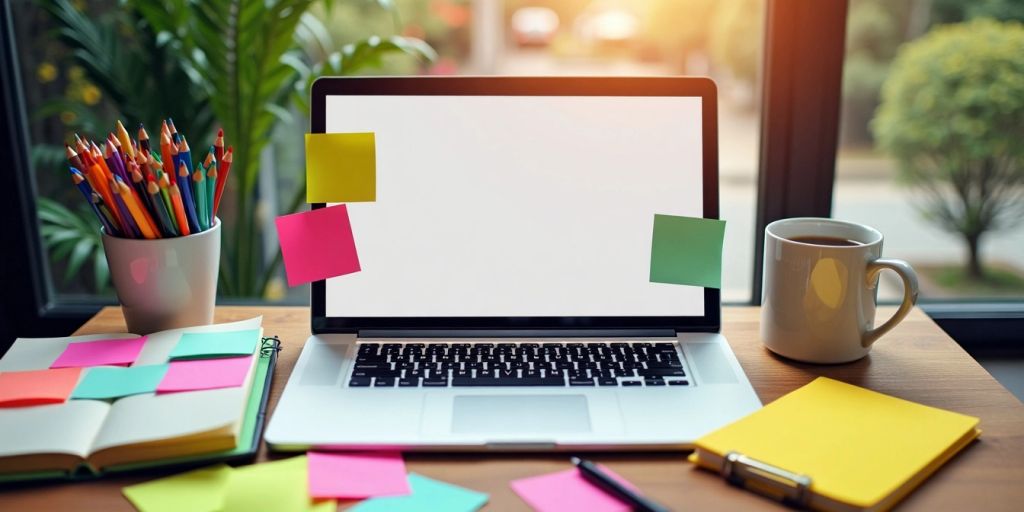
React is a powerful tool for building interactive web apps, and this guide will help you learn the basics step by step. Whether you’re just starting or looking to improve your skills, you’ll find valuable insights here. Let’s dive into the world of React and start creating amazing applications!
Key Takeaways
- React is a JavaScript library for building user interfaces.
- Components are the main building blocks of React applications.
- State and props are essential for managing data in React.
- React makes it easy to handle user interactions with events.
- You can style your components using various methods, including CSS and styled-components.
Getting Started with React
Setting Up Your Development Environment
To begin your journey with React, you need to set up your development environment. Here are the steps:
- Install Node.js: Make sure you have Node.js installed on your computer. This is essential for running React.
- Create a New React App: Use the command
npx create-react-app my-app
to create a new project. This command sets up everything you need to start coding. - Navigate to Your Project: Change into your project directory with
cd my-app
.
Understanding the Basics of React
React is a powerful library for building user interfaces. Here are some key points:
- Component-Based: React applications are built using components, which are reusable pieces of code.
- Virtual DOM: React uses a virtual representation of the DOM to improve performance.
- Declarative Syntax: You describe what your UI should look like based on the current state.
Creating Your First React Component
Now, let’s create your first component. Open the src/App.js
file and replace its content with:
function App() {
return <h1>Hello, React!</h1>;
}
export default App;
This simple component displays a greeting. Congratulations! You’ve just created your first React component!
Remember, this brings us to the end of our initial look at React, including how to install it locally, creating a starter app, and how the basics work.
By following these steps, you are well on your way to mastering React!
Understanding React Components
Functional Components vs Class Components
In React, there are two main types of components: functional components and class components. Functional components are simpler and are written as functions that return JSX. Class components, on the other hand, are more complex and use ES6 classes. They can manage their own state and lifecycle methods. Here’s a quick comparison:
Feature | Functional Components | Class Components |
---|---|---|
Syntax | Function | Class |
State Management | Hooks | Lifecycle Methods |
Simplicity | Easier to read | More complex |
Creating Reusable Components
One of the best things about React is the ability to create reusable components. This means you can build a component once and use it in different parts of your app. Here are some tips for creating reusable components:
- Keep components small and focused on one task.
- Use props to pass data to your components.
- Avoid hardcoding values; instead, use props to make your components flexible.
Component Lifecycle Methods
Class components have special methods called lifecycle methods that allow you to run code at specific points in a component’s life. Here are some important lifecycle methods:
componentDidMount
: Runs after the component is added to the DOM.componentDidUpdate
: Runs after the component updates.componentWillUnmount
: Runs just before the component is removed from the DOM.
Understanding these lifecycle methods is crucial for managing side effects and optimizing performance in your React applications.
React separates the user interface into numerous components, making debugging more accessible, and each component has its own set of properties and functions. This modular approach helps in building complex UIs efficiently.
State and Props in React
Understanding State in React
State is a special object in React that allows components to manage their own data. State is used to keep track of information that can change over time. For example, if you have a button that counts how many times it has been clicked, you would use state to store that count.
- State is local to the component.
- It can be updated using the
setState
method. - Changes in state trigger a re-render of the component.
Using Props to Pass Data
Props, short for properties, are used to transfer data from a parent component to a child component. In simple terms, props are used to send information down the component tree. Here’s how it works:
- Define props in the parent component.
- Pass props to the child component like HTML attributes.
- Access props in the child component using
this.props
.
Parent Component | Child Component |
---|---|
Passes data | Receives data |
Managing State with Hooks
React Hooks, like useState
, allow you to use state in functional components. This makes it easier to manage state without needing to convert your component to a class. Here’s a quick overview:
useState
initializes state and returns the current state and a function to update it.- You can call the update function to change the state, which will re-render the component.
- Hooks can be used to create custom logic that can be reused across components.
Note: Lifting state up is a common practice in React. This means moving the state to a common parent component so that multiple child components can share it. This keeps your components in sync and makes your app easier to manage.
By understanding state and props, you can build dynamic and interactive applications in React!
Handling Events in React
In any interactive web application, handling events is essential for a smooth user experience. React makes this easy with built-in methods that allow developers to create listeners for dynamic interfaces and responses. In this section, we’ll look at how to manage user interactions using event handling in React.
Adding Event Handlers
In React, adding event handlers is similar to traditional DOM event handling, but with some differences due to JSX. Here’s a simple example of a button component that responds to a click event:
import React, { useState } from 'react';
function ButtonComponent() {
const [count, setCount] = useState(0);
const handleClick = () => {
setCount(count + 1);
};
return (
<div>
<button onClick={handleClick}>Click Me</button>
<p>Clicked {count} times</p>
</div>
);
}
export default ButtonComponent;
In this code, we have a functional component called ButtonComponent
. We use the useState
hook to keep track of how many times the button has been clicked. Each time the button is clicked, the count increases.
Synthetic Events in React
React uses a system called Synthetic Events to handle events. This means that React creates a wrapper around the native event to ensure consistent behavior across different browsers. Here’s how you can access the event object:
const handleClick = (event) => {
alert(`Button text: ${event.target.textContent}`);
};
This code shows how to get the text of the button that was clicked. When you click the button, an alert will pop up displaying the button’s text.
Conditional Rendering Based on Events
Event handling is crucial for creating interactive user interfaces. Here are some common scenarios where you might use event handling:
- Updating state based on user actions.
- Displaying alerts or messages when certain events occur.
- Changing styles or classes based on user interactions.
Event handling allows developers to respond to user actions, update the application’s state, and re-render components as needed. This interaction is what makes React a powerful tool for web development.
By mastering event handling in React, you can create dynamic and engaging applications that respond to user input effectively.
Styling React Components
When it comes to styling React components, there are several methods you can use. Here are the most common approaches:
Using CSS in React
- Inline Styling: You can apply styles directly to elements using the
style
attribute. The styles must be in camelCase format. For example:<h1 style={{ color: 'blue', backgroundColor: 'lightgray' }}>Hello World!</h1>
- JavaScript Objects: You can create a JavaScript object to hold your styles and then apply it to your component. For instance:
const myStyle = { color: 'red', padding: '10px' }; <h1 style={myStyle}>Hello World!</h1>
- CSS Stylesheets: You can write your styles in a separate
.css
file and import it into your component. This keeps your styles organized and reusable.
CSS Modules and Styled Components
- CSS Modules: This approach allows you to write CSS that is scoped locally to the component, preventing style conflicts.
- Styled Components: A library that lets you write CSS in your JavaScript files, allowing for dynamic styling based on props.
Responsive Design with React
To ensure your app looks good on all devices, consider using:
- Media queries in your CSS.
- Flexbox or Grid layout for responsive designs.
- Libraries like Bootstrap or Material-UI for pre-built responsive components.
Remember, the way you style your components can greatly affect the user experience. Choose the method that best fits your project needs.
By understanding these different styling methods, you can create visually appealing and functional React applications.
Managing State with Hooks
Introduction to React Hooks
React Hooks were introduced in version 16.8, allowing developers to use state and other React features in functional components. Hooks make it easier to manage state without needing to convert components into class components. The most commonly used hook is useState
, which helps you add state to your components.
Using useState and useEffect
The useState
hook accepts an initial state and returns two values: the current state and a function to update that state. Here’s a simple example:
import { useState } from 'react';
function App() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<button onClick={increment}>Click me</button>
<h2>{`Button clicked ${count} times`}</h2>
</div>
);
}
In this example, clicking the button increases the count. The useState
hook is essential for managing state in functional components.
Custom Hooks for Reusable Logic
Custom hooks allow you to extract component logic into reusable functions. This can help keep your components clean and organized. Here’s how you can create a custom hook:
- Define a function that starts with
use
. - Use built-in hooks like
useState
oruseEffect
inside it. - Return any values or functions you want to share.
For example:
function useCounter(initialValue) {
const [count, setCount] = useState(initialValue);
const increment = () => setCount(count + 1);
return [count, increment];
}
Conclusion
Using hooks like useState
and creating custom hooks can greatly simplify your React code. Hooks provide a powerful way to manage state and share logic across components, making your applications more efficient and easier to maintain.
Remember, hooks can only be called at the top level of a component and not inside loops or conditions. This ensures they work correctly every time your component renders.
Working with Forms in React
Forms are essential for gathering user input in web applications. In React, you can create a form using React JS to handle user data efficiently. Here’s how to work with forms in React:
Controlled vs Uncontrolled Components
- Controlled Components: These components have their form data controlled by React state. This means that the form data is stored in the component’s state and updated via event handlers.
- Uncontrolled Components: These components store their form data in the DOM itself. You can access the data using refs.
- Choosing the Right Approach: Controlled components are generally preferred for their predictability and ease of testing.
Handling Form Submissions
- Prevent Default Behavior: Use
event.preventDefault()
to stop the page from refreshing when the form is submitted. - Access Form Data: Use state to capture the input values.
- Submit the Data: Handle the submission logic, such as sending the data to an API.
Form Validation Techniques
- Client-Side Validation: Check user input before submission to ensure it meets certain criteria (e.g., required fields, valid email format).
- Using Libraries: Consider using libraries like Formik or Yup for more complex validation needs.
- User Feedback: Provide immediate feedback to users about their input, such as error messages or success notifications.
Working with forms in React allows for a smooth user experience and efficient data handling. Understanding how to manage form state is crucial for building interactive applications.
By mastering these techniques, you can create forms that are both functional and user-friendly.
Routing in React Applications
Setting Up React Router
To manage navigation in your React app, you need to set up React Router. This library helps you create a complete guide to routing in React. Here’s how to get started:
- Install React Router:
npm install react-router-dom
- Import BrowserRouter in your main file:
import { BrowserRouter } from 'react-router-dom';
- Wrap your application with BrowserRouter:
<BrowserRouter> <App /> </BrowserRouter>
Creating Navigable Links
Once you have React Router set up, you can create links to navigate between different components. Use the Link
component to do this:
- Example:
import { Link } from 'react-router-dom'; function Navigation() { return ( <nav> <Link to="/home">Home</Link> <Link to="/about">About</Link> </nav> ); }
Nested Routes and Dynamic Routing
React Router also allows you to create nested routes and dynamic routing. Here’s how:
- Nested Routes: You can define routes inside other routes to create a hierarchy.
- Dynamic Routing: Use URL parameters to pass data to your components. For example:
<Route path="/user/:id" component={User} />
Remember: Proper routing is essential for a smooth user experience in your app. It helps users navigate easily and find what they need without confusion.
By following these steps, you can effectively manage routing in your React applications, making them more user-friendly and organized.
Connecting to APIs
Fetching Data with Fetch API
To get data from an API, you can use the Fetch API. This is a built-in JavaScript function that allows you to make network requests. Here’s how to use it:
- Call the
fetch()
function with the API URL. - Use
.then()
to handle the response. - Convert the response to JSON format.
Example:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data));
Using Axios for HTTP Requests
Another popular way to connect to APIs is by using Axios. It’s a promise-based HTTP client that makes it easier to send requests. Here’s how to use it:
- Install Axios using npm:
npm install axios
- Import Axios in your component.
- Use
axios.get()
to fetch data.
Example:
import axios from 'axios';
axios.get('https://api.example.com/data')
.then(response => console.log(response.data));
Handling API Responses and Errors
When working with APIs, it’s important to handle responses and errors properly. Here are some tips:
- Always check the response status.
- Use
.catch()
to handle errors. - Display user-friendly messages if something goes wrong.
Example:
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.catch(error => console.error('There was a problem with the fetch operation:', error));
Connecting to APIs is a crucial skill for building modern web applications. React Native provides the Fetch API for your networking needs. It’s simple and effective for getting data from various sources.
Optimizing React Performance
Using React.memo for Performance Optimization
To improve the performance of your React app, you can use React.memo. This higher-order component helps prevent unnecessary re-renders of functional components. Here are some key points:
- Wrap your component with React.memo to optimize rendering.
- It only re-renders when the props change.
- This is especially useful for components that receive the same props frequently.
Code Splitting with React.lazy
Code splitting allows you to load parts of your application only when needed. You can achieve this using React.lazy. Here’s how:
- Use
React.lazy()
to dynamically import components. - Wrap the lazy-loaded component in a
Suspense
component. - Provide a fallback UI while the component is loading.
Example:
const LazyComponent = React.lazy(() => import('./LazyComponent'));
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
Optimizing Rendering with useCallback and useMemo
Using useCallback and useMemo can help you avoid unnecessary calculations and re-renders:
- useCallback: Returns a memoized version of the callback function that only changes if one of the dependencies has changed.
- useMemo: Returns a memoized value that only recalculates when dependencies change.
Example:
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
const memoizedCallback = useCallback(() => { doSomething(a, b); }, [a, b]);
Remember: Optimizing performance is crucial for a smooth user experience. By implementing these techniques, you can significantly enhance your app’s efficiency.
Tips for Optimizing Your React App’s Performance
- Always provide a unique key for list items.
- Use the map method for rendering lists.
- Consider virtualization for large lists to improve rendering speed.
By following these strategies, you can ensure that your React application runs smoothly and efficiently, providing a better experience for your users.
Deploying Your React Application
Preparing Your App for Deployment
Before you can deploy your app, you need to prepare it. This involves creating a production build. You can do this by running:
npm run build
This command creates a build
folder containing optimized files for deployment. Make sure to test your app locally before moving on.
Deploying to Vercel or Netlify
Both Vercel and Netlify are popular platforms for hosting React applications. Here’s how to deploy your app on each:
- Vercel:
- Netlify:
Continuous Deployment with GitHub Actions
To automate your deployment process, you can use GitHub Actions. Here’s a simple setup:
- Create a
.github/workflows/deploy.yml
file in your repository. - Add the following code:
name: Deploy to Vercel on: push: branches: - main jobs: deploy: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 - name: Deploy to Vercel uses: amondnet/vercel-action@v20 with: vercel-token: ${{ secrets.VERCEL_TOKEN }} vercel-args: '--prod'
- Make sure to set your Vercel token in the GitHub secrets.
Deploying your React app can be quick and easy! With the right tools, you can have your application live in just a few minutes.
By following these steps, you can successfully deploy your React application and share it with the world!
Ready to launch your React app? It’s time to take the next step! Visit our website to discover how our coding tutorials can help you build and deploy your application with confidence. Don’t wait—start coding for free today!
Wrapping Up Your React Journey
Congratulations on reaching the end of this guide! You’ve taken important steps in learning React, a powerful tool for building web apps. Remember, the more you practice, the better you’ll get. Don’t hesitate to create your own projects and explore more resources. Keep experimenting and have fun with your coding journey!
Frequently Asked Questions
What is React and why should I learn it?
React is a JavaScript library that helps you build user interfaces. Learning it is great because it makes creating interactive websites easier.
Do I need to know JavaScript before learning React?
Yes, it’s best to have a basic understanding of JavaScript. This will help you understand React better.
Can I use React for mobile app development?
Yes! You can use React Native, which is based on React, to build mobile apps.
What are components in React?
Components are like building blocks for your app. They let you break your UI into smaller parts that you can manage easily.
What are props and state in React?
Props are how you pass data to components, while state is used to manage data that can change in your app.
How do I handle events in React?
You can handle events in React using event handlers. This lets you respond to user actions like clicks.
What is JSX in React?
JSX is a special syntax that allows you to write HTML-like code in your JavaScript. It makes it easier to create React components.
Is React hard to learn?
Not really! If you have some basic coding skills, you can learn React with practice and patience.