Mastering JavaScript Interview Questions: Your Ultimate Guide to Success in 2024
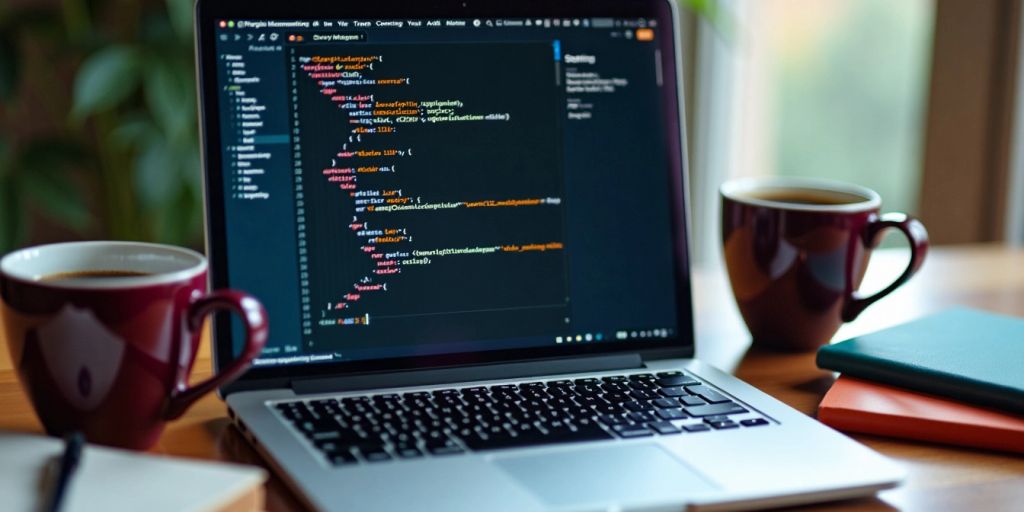
Are you gearing up for a JavaScript interview and feeling lost with all the topics? This guide is here to help! It covers essential JavaScript concepts, from the basics to advanced ideas, making sure you’re ready to impress in your next interview. JavaScript is a key language in web development, so understanding it well is crucial for both new and experienced developers. Let’s dive into the key takeaways that will set you on the path to success!
Key Takeaways
- Understand the main data types and how to use variables correctly.
- Learn different ways to write functions and what closures are.
- Get familiar with handling asynchronous code using promises and async/await.
- Practice manipulating the DOM and handling events effectively.
- Know common interview questions and how to answer them confidently.
Understanding JavaScript Basics
JavaScript is a programming language that adds interactivity to your website. This happens in games, in the behavior of responses when buttons are pressed, and much more. Understanding the basics is crucial for anyone looking to master JavaScript.
Key Data Types and Variables
JavaScript has several key data types:
- Number: Represents numeric values.
- String: Represents text.
- Boolean: Represents true or false values.
- Object: A collection of properties.
- Null: Represents an empty value.
- Undefined: A variable that has been declared but not assigned a value.
Basic Syntax and Operators
JavaScript syntax is the set of rules that define a correctly structured JavaScript program. Here are some basic operators:
- Arithmetic Operators: +, -, *, /, %
- Comparison Operators: ==, ===, !=, !==, >, <
- Logical Operators: &&, ||, !
Control Structures and Loops
Control structures help manage the flow of a program. Common types include:
- If Statements: Execute code based on a condition.
- Switch Statements: Choose between multiple options.
- Loops: Repeat code, such as
for
,while
, anddo...while
loops.
Understanding these basic concepts is essential for building a strong foundation in JavaScript programming. They will help you tackle more complex topics later on.
Deep Dive into Functions and Closures
Function Declarations and Expressions
In JavaScript, functions can be defined in two main ways: function declarations and function expressions. Here’s a quick overview:
Type | Description |
---|---|
Function Declaration | A named function defined using the function keyword. |
Function Expression | A function defined within an expression, often assigned to a variable. |
Understanding Closures
Closures are a powerful feature in JavaScript. A closure is a function that has access to variables from an outer function, even after the outer function has finished executing. This is possible because of closure scope chains. Here’s a simple example:
function outerFunction() {
let outerVariable = "I am from outer!";
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
const myInnerFunction = outerFunction();
myInnerFunction(); // Outputs: I am from outer!
Higher-Order Functions
Higher-order functions are functions that can take other functions as arguments or return them. They are essential for functional programming in JavaScript. Here are some common examples:
- map: Transforms an array by applying a function to each element.
- filter: Creates a new array with elements that pass a test.
- reduce: Reduces an array to a single value by applying a function.
Closures allow for data hiding and encapsulation, making them invaluable in JavaScript programming. They help maintain state and create private variables, enhancing code organization and security.
Mastering Asynchronous JavaScript
Asynchronous JavaScript is essential for creating responsive web applications. In this section, we will explore how JavaScript handles asynchronous tasks by starting with callbacks, moving through promises, and finally reaching the modern async/await syntax.
Event Loop and Concurrency
The event loop is a core concept in JavaScript that allows it to perform non-blocking operations. Here’s how it works:
- Call Stack: Executes synchronous code.
- Event Queue: Holds asynchronous callbacks.
- Event Loop: Checks if the call stack is empty and processes the next event from the queue.
Promises and Async/Await
Promises are a way to handle asynchronous operations more effectively than callbacks. They can be in one of three states: pending, fulfilled, or rejected. Here’s a quick comparison:
Feature | Callbacks | Promises | Async/Await |
---|---|---|---|
Readability | Low | Medium | High |
Error Handling | Difficult | Easier | Simplified |
Execution Flow | Nested (Callback Hell) | Chained | Sequential |
Handling Asynchronous Operations
To manage asynchronous tasks effectively, consider these strategies:
- Use Promises to avoid callback hell.
- Implement async/await for cleaner syntax.
- Always handle errors using
.catch()
or try/catch blocks.
Asynchronous programming is a powerful tool that allows developers to create efficient and responsive applications. Mastering it is key to success in modern web development.
Working with the DOM
DOM Manipulation Techniques
The Document Object Model (DOM) is a way for JavaScript to interact with HTML documents. It represents the structure of a webpage as a tree of objects. Here are some common techniques for manipulating the DOM:
- Selecting Elements: Use methods like
getElementById
,querySelector
, andgetElementsByClassName
to find elements. - Changing Content: Modify the text or HTML of elements using properties like
textContent
andinnerHTML
. - Adding and Removing Elements: Use methods like
appendChild
,removeChild
, andcreateElement
to manage elements dynamically.
Event Handling and Delegation
Event handling is crucial for making web pages interactive. Here are some key points:
- Event Listeners: Use
addEventListener
to respond to user actions like clicks or key presses. - Event Delegation: Instead of adding listeners to each element, attach one to a parent. This is efficient for dynamic content.
- Event Propagation: Understand how events bubble up or capture down the DOM tree to manage event flow effectively.
Understanding the Document Object Model
The DOM is a powerful tool for web developers. It allows for:
- Dynamic Updates: Change the content and structure of a webpage without reloading.
- Cross-Platform Compatibility: Works across different browsers and devices.
- Event Handling: Respond to user interactions in real-time.
The DOM is essential for creating interactive web applications. Mastering it can significantly improve your coding skills.
In summary, mastering the DOM is vital for any JavaScript developer. It allows for dynamic and interactive web experiences, making it a key topic in interviews.
Highlighted Content
Through this article, we’ve explored some of the top interview questions that test your understanding of the DOM, including techniques for element removal, and more.
Advanced JavaScript Concepts
Prototypal Inheritance
Prototypal inheritance is a unique feature of JavaScript that allows objects to inherit properties from other objects. This means that you can create a new object based on an existing one, sharing its properties and methods. Understanding this concept is crucial for mastering JavaScript.
Understanding ‘this’ Keyword
The this
keyword can be tricky in JavaScript. It refers to the context in which a function is called. Here are some key points to remember:
- In a regular function,
this
refers to the global object (orundefined
in strict mode). - In an object method,
this
refers to the object itself. - In an arrow function,
this
retains the value from the enclosing lexical context.
JavaScript Design Patterns
Design patterns are standard solutions to common problems in software design. Here are a few popular JavaScript design patterns:
- Module Pattern: Encapsulates private variables and functions.
- Singleton Pattern: Ensures a class has only one instance.
- Observer Pattern: Allows an object to notify other objects about changes in its state.
Mastering these advanced concepts will help you tackle complex JavaScript problems and improve your coding skills.
Conclusion
By diving deep into advanced JavaScript concepts, you can enhance your understanding of closures, prototypes, and asynchronous programming. This knowledge is essential for modern JavaScript development and will prepare you for challenging interview questions.
JavaScript in Web Development
JavaScript is a vital part of web development, allowing developers to create interactive and dynamic websites. In 2024, JavaScript continues to be essential for shaping the front-end and web development landscape.
Integrating JavaScript with HTML and CSS
- JavaScript works alongside HTML and CSS to enhance web pages.
- It can manipulate HTML elements and apply styles dynamically.
- This integration allows for responsive designs and interactive features.
Using JavaScript Frameworks
- Frameworks like React, Angular, and Vue simplify the development process.
- They promote a component-based architecture, making code reusable and easier to manage.
- These frameworks help in building scalable applications efficiently.
Building Interactive Web Applications
- User Interaction: JavaScript enables real-time updates and user feedback.
- Data Handling: It can fetch and display data from servers without reloading the page.
- Enhanced User Experience: Features like animations and transitions improve engagement.
JavaScript’s role in web development is not just about functionality; it also enhances the overall user experience, making websites more enjoyable to use.
Framework | Key Feature | Use Case |
---|---|---|
React | Component-based architecture | Single-page applications |
Angular | Full MVC framework | Enterprise-level applications |
Vue | Progressive framework | Small to medium projects |
Common JavaScript Interview Questions
Basic to Intermediate Questions
When preparing for JavaScript interviews, you will often encounter questions that test your understanding of the fundamentals. Here are some common topics:
- Data Types: What are the different data types in JavaScript?
- Variable Declarations: What is the difference between
var
,let
, andconst
? - Control Structures: How do loops and conditionals work?
Advanced Questions
As you progress, expect to face more challenging questions that delve into complex concepts. Some examples include:
- Closures: Can you explain what a closure is and provide an example?
- Promises: How do promises work in JavaScript?
- Prototypal Inheritance: What is prototypal inheritance, and how does it differ from classical inheritance?
Tricky and Edge Case Questions
These questions often focus on the quirks of JavaScript. Be prepared for:
- Type Coercion: How does JavaScript handle type coercion in comparisons?
- Hoisting: What is hoisting, and how does it affect variable declarations?
- Floating-Point Precision: Why does
0.1 + 0.2
not equal0.3
in JavaScript?
Understanding these concepts is crucial for demonstrating your knowledge during interviews. Practice is key to mastering these topics!
Best Practices for JavaScript Coding
Writing Clean and Maintainable Code
To write clean and maintainable code, it’s essential to adhere to a JavaScript code style standard. This helps in keeping your code consistent and easy to read. Here are some tips:
- Use meaningful names for variables and functions.
- Keep your functions small and focused on a single task.
- Avoid using global variables whenever possible.
Performance Optimization
Optimizing performance is crucial for a smooth user experience. Here are some strategies:
- Minimize DOM manipulations as they can be slow.
- Use event delegation to handle events efficiently.
- Avoid memory leaks by cleaning up unused objects and event listeners.
Security Considerations
Security is vital in coding. Here are some practices to keep in mind:
- Always validate user input to prevent injection attacks.
- Use HTTPS to secure data transmission.
- Regularly update libraries and frameworks to patch vulnerabilities.
Following these best practices will not only improve your code quality but also enhance your skills as a developer. Remember, making use of shorthands can be helpful, but exercise caution with them to avoid confusion.
Preparing for JavaScript Interviews
Effective Study Techniques
To prepare well for your JavaScript interview, consider these strategies:
- Practice coding regularly to improve your skills.
- Review key concepts like data types, functions, and control flow.
- Work on sample problems to get familiar with common interview questions.
Resources for Practice
Here are some great resources to help you practice:
- LeetCode – Offers a variety of coding challenges.
- HackerRank – Focuses on coding skills and interview prep.
- Books – "Eloquent JavaScript" is a great read for understanding the language.
Mock Interview Strategies
Mock interviews can be very helpful. Here’s how to make the most of them:
- Simulate real interview conditions to get comfortable.
- Ask a friend or mentor to conduct the interview.
- Record your sessions to review your performance.
Preparing for a JavaScript interview is crucial. With the right techniques and resources, you can boost your confidence and skills.
Remember, mastering the top 80+ JavaScript interview questions will give you a solid foundation to tackle any interview successfully!
Understanding JavaScript Debugging
Debugging in JavaScript is a crucial skill for any developer. It refers to the process of identifying and fixing errors or bugs in code. Here are some key aspects to consider:
Common Debugging Tools
- Browser Console: A built-in tool in browsers that allows you to run JavaScript code and view errors.
- Debugger: A feature that lets you pause code execution to inspect variables and flow.
- Linting Tools: These help catch errors before running the code.
Debugging Techniques
- Console Logging: Use
console.log()
to print variable values and track code execution. - Breakpoints: Set breakpoints in your code to pause execution and inspect the current state.
- Step Through Code: Execute your code line by line to see where it goes wrong.
Handling Errors and Exceptions
- Try-Catch Blocks: Use these to handle errors gracefully without crashing your program.
- Error Messages: Pay attention to error messages in the console; they often provide clues about what went wrong.
Debugging is not just about fixing errors; it’s about understanding your code better and improving your skills.
By mastering these tools and techniques, you can become more efficient at debugging and enhance your overall coding abilities.
JavaScript in Full-Stack Development
In today’s tech world, being a full-stack developer means you can work on both the front-end and back-end of web applications. JavaScript plays a crucial role in this journey. Here’s a closer look at how JavaScript fits into full-stack development.
Client-Side vs Server-Side JavaScript
- Client-Side JavaScript: This is the code that runs in the user’s browser. It makes websites interactive and dynamic. Common tasks include:
- Server-Side JavaScript: This runs on the server and handles requests from the client. Node.js is a popular choice for this. Key features include:
Using Node.js
Node.js allows developers to use JavaScript on the server side. This means you can write both the front-end and back-end in the same language, which simplifies the development process. Here are some benefits of using Node.js:
- Fast Performance: It uses an event-driven model, making it efficient for handling multiple requests.
- Large Ecosystem: With npm (Node Package Manager), developers have access to thousands of libraries and tools.
- Real-Time Applications: Great for building chat applications or live updates.
Integrating with Databases
Full-stack developers often need to connect their applications to databases. JavaScript can work with various databases:
- NoSQL Databases: Such as MongoDB, which stores data in flexible, JSON-like documents.
- SQL Databases: Like MySQL or PostgreSQL, which use structured query language for data management.
In summary, mastering JavaScript for both client-side and server-side development is essential for any aspiring full-stack developer. The full stack developer roadmap will guide you on how to become a full stack developer in 2024. Learn about both front-end and back-end development!
JavaScript plays a key role in full-stack development, making it easier to create dynamic web applications. If you’re eager to learn how to use JavaScript effectively and boost your coding skills, visit our website today! Start your journey towards mastering coding with us!
Conclusion
Getting a good grasp of JavaScript interview questions is key to doing well in the fast-paced world of web development. By exploring topics like closures, event handling, and asynchronous coding, you can boost your skills and feel more confident for your interviews. Don’t forget to practice often, keep up with the latest trends, and use helpful resources. With hard work and smart preparation, you’ll be ready to show off your skills and succeed in your next JavaScript interview.
Frequently Asked Questions
What should beginners focus on when preparing for a JavaScript interview?
Beginners should start by learning the basics like data types, variables, and how to write simple code. It’s also important to understand loops and conditionals.
Where can I find good resources to practice JavaScript interview questions?
You can use websites like LeetCode and HackerRank for practice. Books like ‘Eloquent JavaScript’ are also helpful.
Why is it important to know about asynchronous JavaScript for interviews?
Understanding asynchronous JavaScript is crucial, especially for web development jobs. Interviewers often ask about callbacks, promises, and async/await.
What types of questions can I expect for basic JavaScript interviews?
You might be asked about the differences between var, let, and const, or basic concepts like loops and conditionals.
How can I prepare for tricky JavaScript interview questions?
Focus on JavaScript’s unique features, like type coercion and hoisting. Practice these concepts to be ready for tricky questions.
What are some common JavaScript libraries I should know about?
Familiarize yourself with libraries like React and Angular, especially if the job involves front-end development.
What advanced topics should I study for senior JavaScript roles?
For advanced roles, study closures, promises, and design patterns. These topics often come up in interviews.
How can I improve my JavaScript coding skills?
Regular practice is key. Work on coding challenges and build small projects to apply what you’ve learned.