Mastering HTML Comment: Tips and Best Practices for Clean Code
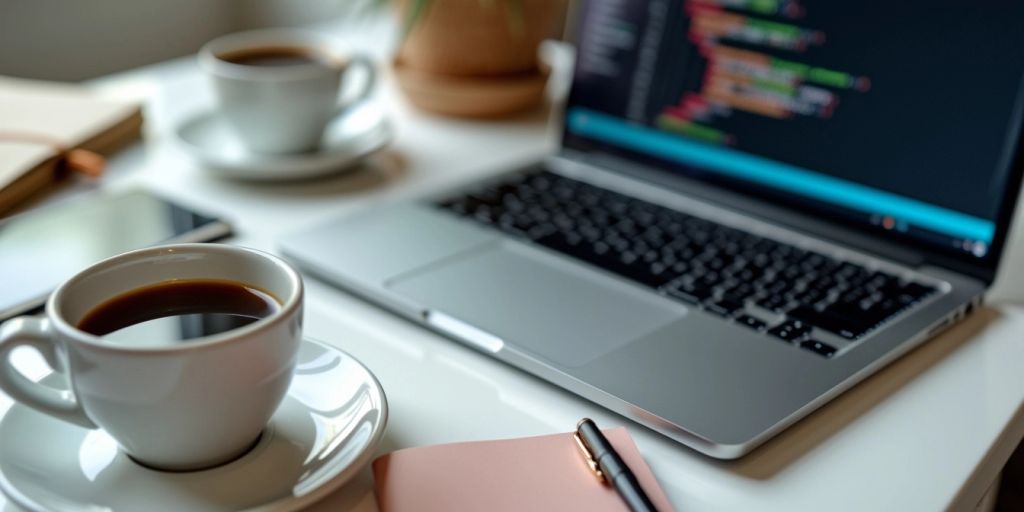
HTML comments are an essential part of coding that help make your code easier to read and maintain. They provide explanations, mark important notes, and can even assist in debugging. However, knowing how to use them effectively is crucial. This article will guide you through the importance of HTML comments and share best practices for writing them.
Key Takeaways
- HTML comments help others understand your code better.
- Avoid writing comments that simply repeat what the code already says.
- Use comments to clarify complex parts of your code.
- Keep comments updated to reflect any changes in the code.
- Be careful not to overuse comments, as they can clutter your code.
Understanding the Importance of HTML Comments
Why HTML Comments Matter
HTML comments are essential for developers and designers. They allow you to include notes or reminders in your code that are not visible to visitors. This helps keep your code organized and understandable. Here are some reasons why comments are important:
- They clarify the purpose of code sections.
- They help others (or yourself) understand the code later.
- They can prevent confusion about complex logic.
Common Misconceptions About HTML Comments
Many people think that comments are unnecessary or just clutter. However, comments can be very valuable. They can explain why certain decisions were made in the code, which can save time and effort later. For example, a comment might explain why a non-obvious solution was chosen, helping others understand the reasoning behind it.
The Role of Comments in Code Maintenance
Comments play a crucial role in maintaining code. They help:
- Identify areas that need updates or fixes.
- Provide context for future developers.
- Mark incomplete implementations or known issues.
Comments are not just for explaining how code works; they also explain why it was written that way. This can save hours of work in the long run.
Best Practices for Writing HTML Comments
Avoiding Redundant Comments
When writing HTML comments, it’s crucial to avoid redundancy. Comments should add value and not simply repeat what the code already states. For example, instead of writing:
<div> <!-- This is a div --> </div>
A better comment would explain the purpose of the div:
<div> <!-- This div contains the main content of the page --> </div>
Using Comments to Explain Complex Code
Sometimes, code can be tricky to understand. In such cases, comments can help clarify the logic. Here are some tips:
- Use comments to break down complex functions.
- Explain why certain decisions were made in the code.
- Provide context for future developers who may work on the code.
Keeping Comments Up-to-Date
It’s essential to keep comments current. Outdated comments can lead to confusion. Here are some practices to ensure your comments remain relevant:
- Review comments during code updates.
- Remove comments that no longer apply.
- Add new comments when making significant changes.
Keeping your comments updated is as important as writing them in the first place. They should reflect the current state of the code.
By following these best practices, you can ensure that your HTML comments are effective and helpful, making your code cleaner and easier to maintain. Remember, the best guide to HTML comments is to write them with clarity and purpose!
Different Types of HTML Comments
Single-Line Comments
Single-line comments in HTML are straightforward and begin with <!--
and end with -->
. They are useful for brief notes or explanations. These comments are not displayed in the browser. Here’s how you can use them:
<!-- This is a single-line comment -->
Multi-Line Comments
Multi-line comments allow you to write longer explanations or notes. They also start with <!--
and end with -->
, but you can include multiple lines of text. This is helpful for providing detailed information about a section of code. For example:
<!--
This is a multi-line comment.
It can span multiple lines.
-->
Conditional Comments
Conditional comments are a special type of comment used mainly in older versions of Internet Explorer. They allow you to include code that only certain browsers will read. This can be useful for ensuring compatibility. Here’s an example:
<!--[if IE]>
<p>This is only for Internet Explorer.</p>
<![endif]-->
Summary
Using the right type of comment can help keep your code organized and understandable. Here’s a quick comparison:
Type of Comment | Purpose |
---|---|
Single-Line Comments | Brief notes or explanations |
Multi-Line Comments | Detailed information |
Conditional Comments | Browser-specific code |
Remember, comments should enhance your code, not clutter it. Use them wisely to improve readability and maintainability!
When to Use HTML Comments
Commenting Out Code for Debugging
When you’re working on a project, you might need to test different parts of your code. Commenting out code can help you do this without deleting anything. Here are some reasons to comment out code:
- It allows you to test changes without losing the original code.
- You can easily restore the code if needed.
- It helps in isolating issues during debugging.
Using Comments for Documentation
Comments can serve as a guide for anyone reading your code later. They can explain what certain sections do or why specific choices were made. Here are some tips for effective documentation:
- Use clear and simple language.
- Explain the purpose of complex functions.
- Reference any related issues or tasks.
Marking Incomplete Implementations
Sometimes, you might have code that isn’t finished yet. Using comments to mark these areas is crucial. For example, you can use a TODO
comment to indicate that something still needs work. This helps keep track of what needs to be done and prevents confusion later on.
Comments are not just for explaining code; they also help in managing tasks and keeping track of what needs to be completed.
In summary, using HTML comments wisely can enhance your code’s readability and maintainability. They are a valuable tool for both debugging and documentation, ensuring that your code remains clear and organized.
Common Mistakes to Avoid with HTML Comments
Over-Commenting Your Code
One of the biggest mistakes is adding too many comments. This can clutter your code and make it harder to read. Here are some points to consider:
- Comments should not repeat what the code does.
- Avoid commenting on every single line; it can be overwhelming.
- Focus on explaining the why behind complex logic instead.
Using Comments to Justify Bad Code
Sometimes, developers use comments to cover up unclear or poorly written code. Instead of relying on comments, strive to write clean and understandable code. Remember:
- Good comments do not excuse unclear code.
- If you find yourself needing to explain something extensively, it might be time to refactor.
- Always aim for clarity in your code first.
Failing to Update Comments
Comments can become outdated quickly. If you change your code, make sure to update the comments as well. Here’s why:
- Outdated comments can lead to confusion.
- They can mislead other developers who read your code later.
- Regularly review comments to ensure they reflect the current state of the code.
Keeping your comments relevant is just as important as writing them in the first place.
By avoiding these common mistakes, you can ensure that your HTML comments enhance your code rather than detract from it.
Tools and Techniques for Managing HTML Comments
Using Linters to Check Comments
Linters are tools that help you find errors in your code, including issues with comments. They can check for:
- Redundant comments that don’t add value.
- Comments that are outdated or no longer relevant.
- Formatting issues that make comments hard to read.
Automating Comment Updates
Keeping comments up-to-date can be a challenge. Here are some ways to automate this process:
- Use scripts to scan for comments that reference outdated code.
- Set reminders to review comments during code reviews.
- Integrate comments with your version control system to track changes.
Integrating Comments with Issue Trackers
Linking comments to issue trackers can enhance collaboration. This allows team members to:
- Reference specific issues directly in comments.
- Track the status of tasks related to the code.
- Ensure that comments are relevant to ongoing work.
Effective management of HTML comments can greatly improve code readability and maintainability.
By using these tools and techniques, you can ensure that your comments are helpful and relevant, making your code easier to understand for everyone involved. These comments aid collaboration and enhance code readability, offering insights into the code structure and purpose.
HTML Comments and SEO
How Comments Affect Page Load Time
HTML comments can impact page load time, but the effect is usually minimal. Comments are ignored by browsers, meaning they don’t affect rendering speed directly. However, excessive comments can increase file size, which may slow down loading times slightly. Here are some points to consider:
- Keep comments concise.
- Avoid unnecessary comments that add bulk.
- Use comments wisely to maintain a balance between clarity and performance.
Using Comments to Improve SEO
While HTML comments themselves are not indexed by search engines, they can still play a role in SEO indirectly. Here’s how:
- Organize your code: Well-structured comments can help developers maintain clean code, which can lead to better site performance.
- Document important sections: Use comments to highlight critical areas of your code that may need optimization.
- Facilitate collaboration: Clear comments help teams work together effectively, ensuring that SEO best practices are followed consistently.
Avoiding SEO Pitfalls with Comments
To ensure that comments do not negatively impact your SEO efforts, follow these guidelines:
- Don’t overuse comments: Too many comments can clutter your code and make it harder to read.
- Avoid misleading comments: Ensure that comments accurately reflect the code they describe.
- Regularly update comments: Outdated comments can confuse developers and lead to mistakes.
Keeping your code clean and well-commented is essential for both developers and search engines. A well-maintained codebase can lead to better performance and improved SEO.
Advanced HTML Commenting Strategies
Using Comments for Team Collaboration
Effective collaboration among team members is crucial in coding projects. Comments can serve as a bridge between developers, ensuring everyone is on the same page. Here are some ways to use comments for better teamwork:
- Clarify intentions: Explain why certain decisions were made in the code.
- Assign tasks: Use comments to indicate who is responsible for specific sections.
- Share knowledge: Document any unique solutions or workarounds that others might need to know.
Embedding Metadata in Comments
Adding metadata in comments can enhance the understanding of the code. This can include:
- Author information: Who wrote the code and when.
- Version history: Changes made over time.
- Related issues: Reference to issue trackers for context.
Metadata Type | Example |
---|---|
Author | // Author: Jane Doe |
Date | // Date: 2023-10-01 |
Issue Reference | // Issue #1234 |
Creating Custom Comment Tags
Custom tags can help organize comments and make them more useful. Consider using:
TODO
: For tasks that need to be completed.FIXME
: For known issues that need fixing.NOTE
: For important information that should not be overlooked.
Using structured comments can help future developers understand the code better and reduce confusion.
By implementing these strategies, you can make your HTML comments more effective and beneficial for everyone involved in the project.
HTML Comments in Different Environments
Comments in Static Websites
Static websites are simple and often consist of HTML files that do not change. Here, comments can be used to:
- Explain the structure of the HTML.
- Provide notes for future updates.
- Mark sections for easier navigation.
Using comments effectively can enhance your code. For example, you might write a comment like this:
<!-- Header Section -->
<header>
<h1>Welcome to My Site</h1>
</header>
Comments in Dynamic Web Applications
Dynamic web applications often use server-side languages. In these cases, comments can help:
- Clarify complex logic.
- Document API calls.
- Indicate where to add new features.
For instance, a comment might look like:
<!-- Fetching user data from API -->
<script>
fetch('/api/user')
.then(response => response.json())
.then(data => console.log(data));
</script>
Comments in Content Management Systems
Content Management Systems (CMS) allow users to create and manage content easily. Here, comments can be used to:
- Provide guidance for editors.
- Explain template structures.
- Note areas needing updates.
In a CMS, you might see comments like:
<!-- This section is for the footer links -->
<footer>
<a href="#">Privacy Policy</a>
</footer>
Comments are essential for maintaining clarity in your code, especially in different environments. They help both current and future developers understand the purpose and function of various code sections.
By following these practices, you can ensure that your HTML comments are useful and relevant across different environments, making your code cleaner and easier to manage.
Case Studies: Effective Use of HTML Comments
Real-World Examples of Good Comments
In many coding projects, effective comments can make a significant difference. Here are some examples:
- Clear Purpose: Comments that explain the purpose of a function or a block of code help others understand its role.
- TODO Notes: Using comments to mark incomplete tasks, like
// TODO: Add error handling
, keeps track of what still needs to be done. - Reference Issues: Linking comments to issue trackers, such as
// Issue #1425: Fix this bug
, provides context for future developers.
Lessons Learned from Bad Comments
Not all comments are helpful. Here are some common pitfalls:
- Vague Comments: Comments like
// This is important
do not provide useful information. - Obscure References: Comments that reference outdated issues or use jargon can confuse readers.
- Over-Commenting: Writing too many comments can clutter the code and make it harder to read.
Industry Standards for Commenting
To ensure comments are effective, consider these standards:
- Be Concise: Ensure your comments are clear and easy to understand. Avoid jargon unless it’s necessary.
- Stay Relevant: Update comments as the code changes to keep them accurate.
- Use Consistent Formatting: Follow a standard format for comments to maintain clarity across the codebase.
Effective comments complement good code by providing context and clarity. They help others understand not just how the code works, but why it was written that way. Good comments can save time and reduce confusion.
In our section on case studies, we explore how HTML comments can enhance your coding projects. These comments not only help you keep your code organized but also make it easier for others to understand your work. Want to learn more about coding and improve your skills? Visit our website and start coding for free today!
Conclusion
In closing, it’s clear that comments are not a fix for poor code; instead, they enhance well-written code by adding important context. As Jeff Atwood, co-founder of Stack Overflow, wisely said, "Code shows how to do something, while comments explain why it’s done that way." By following the guidelines shared in this article, you can save yourself and your team from confusion and wasted time. Remember, these tips are just a starting point, and I encourage you to share any additional insights in the comments below.
Frequently Asked Questions
What are HTML comments used for?
HTML comments help explain the code and make it easier for others to understand. They can also mark sections of code that might need attention later.
Can comments slow down a website?
No, HTML comments don’t affect the speed of your website. They are ignored by browsers, so they won’t slow down page loading.
Should I comment every line of code?
No, commenting every line can make code messy. Only comment when it adds value or helps explain complex parts.
What’s the difference between single-line and multi-line comments?
Single-line comments use ‘’ for short notes, while multi-line comments can span several lines and are also written as ‘’.
How often should I update comments in my code?
You should update comments whenever you change the code they refer to. Keeping comments current helps avoid confusion.
Are there any mistakes to avoid when writing comments?
Yes, avoid over-commenting, using comments to cover bad code, and forgetting to update them when code changes.
What are conditional comments?
Conditional comments are used to target specific browsers or versions. They help ensure that certain code runs only in those cases.
Can comments be used for SEO purposes?
While comments themselves don’t affect SEO directly, they can help organize your code better, which might improve overall site management.