Mastering Graph Theory for Coding Interviews: Key Concepts and Strategies
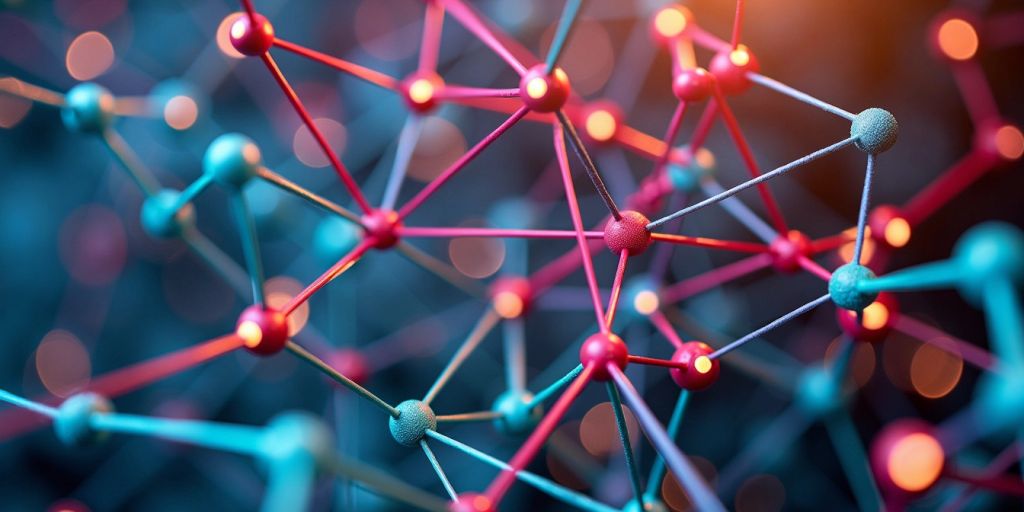
Graph theory is a vital area of study in computer science, especially for those preparing for coding interviews. Understanding the fundamental concepts and algorithms can significantly enhance your problem-solving skills. This article will guide you through essential graph topics, techniques, and strategies to help you excel in interviews.
Key Takeaways
- Graph theory is essential for coding interviews, focusing on key algorithms and concepts.
- Common graph types include directed, undirected, weighted, and unweighted graphs.
- Familiarity with graph representations like adjacency lists and matrices is crucial.
- Mastering traversal techniques such as DFS and BFS is fundamental for solving graph problems.
- Understanding shortest path algorithms like Dijkstra’s is vital for many interview questions.
- Cycle detection is important for both directed and undirected graphs.
- Topological sorting is a key concept for processing tasks in order.
- Practice with real interview questions is necessary to solidify your understanding.
Understanding Graph Theory for Coding Interviews
Definition and Importance
Graph theory is a branch of mathematics that studies graphs, which are structures made up of vertices (or nodes) and edges (connections between nodes). Understanding graph theory is crucial for coding interviews because many problems can be modeled as graphs. Mastering these concepts can significantly improve your problem-solving skills.
Common Graph Terminologies
Here are some key terms you should know:
- Vertex: A point in the graph.
- Edge: A connection between two vertices.
- Path: A sequence of edges connecting vertices.
- Cycle: A path that starts and ends at the same vertex.
Types of Graphs
Graphs can be classified into several types:
- Directed Graphs: Edges have a direction.
- Undirected Graphs: Edges do not have a direction.
- Weighted Graphs: Edges have weights (costs).
- Unweighted Graphs: Edges do not have weights.
Applications in Coding Interviews
Graph theory is often used in various coding problems, such as:
- Finding the shortest path between two nodes.
- Detecting cycles in a graph.
- Implementing network flow algorithms.
Challenges in Graph Theory Problems
Graph problems can be tricky due to:
- The presence of cycles.
- The need for efficient traversal methods.
- Handling large datasets.
Resources for Learning Graph Theory
To master graph theory, consider the following resources:
- Books: Look for titles that cover graph algorithms.
- Online Courses: Platforms like Coursera and Udacity offer courses on graph theory.
- Practice Platforms: Websites like LeetCode and HackerRank have graph-related problems to solve.
Understanding graph theory is not just about memorizing algorithms; it’s about developing a mindset to approach complex problems.
In summary, grasping the fundamentals of graph theory is essential for success in coding interviews. By familiarizing yourself with key concepts and practicing regularly, you can enhance your problem-solving abilities and stand out in interviews.
Highlighted Concept
This article covered essential graph theory concepts and demonstrated how to implement key graph algorithms in Python.
Graph Representations and Their Uses
Adjacency Matrix
An adjacency matrix is a 2D array used to represent a graph. Each cell in the matrix indicates whether pairs of vertices are connected. For example, if there are 4 vertices, the matrix will be 4×4. Here’s a simple representation:
Vertex | 0 | 1 | 2 | 3 |
---|---|---|---|---|
0 | 0 | 1 | 0 | 1 |
1 | 1 | 0 | 1 | 0 |
2 | 0 | 1 | 0 | 1 |
3 | 1 | 0 | 1 | 0 |
Adjacency List
An adjacency list is another way to represent a graph. It uses an array of lists. Each index in the array represents a vertex, and the list at that index contains all the vertices connected to it. This method is more space-efficient for sparse graphs.
Edge List
An edge list is a simple representation that lists all the edges in the graph. Each edge connects two vertices. For example, an edge list for a graph with edges (0, 1), (1, 2), and (2, 3) would look like this:
Edge |
---|
(0, 1) |
(1, 2) |
(2, 3) |
Comparison of Representations
Each representation has its pros and cons:
- Adjacency Matrix: Easy to check if an edge exists but uses more space.
- Adjacency List: More space-efficient but harder to check for edges.
- Edge List: Simple but not efficient for checking connections.
Choosing the Right Representation
Choosing the right representation depends on the problem. If you need to check for edges frequently, an adjacency matrix might be best. For sparse graphs, an adjacency list is often more efficient.
Practice Problems
To get better at graph representations, try these problems:
- Build a graph from an edge list.
- Convert an adjacency matrix to an adjacency list.
- Find the degree of each vertex in a graph.
Understanding how to represent a graph is crucial for solving graph problems effectively. A graph is a non-linear data structure consisting of vertices and edges.
Mastering Graph Traversal Techniques
Depth First Search (DFS)
Depth First Search (DFS) is a fundamental algorithm used to explore graphs. It starts at a selected node and explores as far as possible along each branch before backtracking. Mastering DFS is crucial for technical interviews, as it helps in solving various graph problems efficiently. Here’s a simple way to implement DFS:
- Start at the root (or any arbitrary node).
- Mark the node as visited.
- Recursively visit all the adjacent nodes that haven’t been visited yet.
Breadth First Search (BFS)
Breadth First Search (BFS) is another essential graph traversal technique. Unlike DFS, BFS explores all neighbors at the present depth prior to moving on to nodes at the next depth level. This is particularly useful for finding the shortest path in unweighted graphs. The steps to implement BFS are:
- Start at the root node.
- Use a queue to keep track of nodes to visit next.
- Mark nodes as visited and enqueue their unvisited neighbors.
Comparing DFS and BFS
Both DFS and BFS have their unique advantages:
- DFS is memory efficient and can be implemented using recursion.
- BFS guarantees the shortest path in unweighted graphs but uses more memory.
Feature | DFS | BFS |
---|---|---|
Memory Usage | Lower (stack) | Higher (queue) |
Shortest Path | No | Yes |
Implementation | Recursive | Iterative |
Implementing DFS and BFS
To implement these algorithms, you can use either an adjacency list or an adjacency matrix. Here’s a quick example of how to implement both:
# DFS Implementation
def dfs(node, visited):
if node not in visited:
visited.add(node)
for neighbor in graph[node]:
dfs(neighbor, visited)
# BFS Implementation
def bfs(start):
visited = set()
queue = [start]
while queue:
node = queue.pop(0)
if node not in visited:
visited.add(node)
queue.extend(neighbor for neighbor in graph[node] if neighbor not in visited)
Applications of Traversal Techniques
Graph traversal techniques are widely used in various applications, including:
- Finding connected components in a graph.
- Solving puzzles like mazes.
- Analyzing social networks.
Common Mistakes to Avoid
When working with graph traversal, be cautious of the following:
- Forgetting to mark nodes as visited, leading to infinite loops.
- Using the wrong algorithm for the problem type.
- Not considering edge cases, such as disconnected graphs.
Understanding these traversal techniques is essential for tackling graph-related problems in coding interviews. With practice, you can become proficient in identifying and applying the right algorithms effectively.
Advanced Graph Algorithms for Interviews
Dijkstra’s Algorithm
Dijkstra’s Algorithm is a popular method used to find the shortest path from a starting node to all other nodes in a weighted graph. It works by maintaining a priority queue of nodes to explore, ensuring that the closest node is always processed next. This algorithm is efficient and widely used in various applications, including GPS navigation.
A* Search Algorithm
The A* Search Algorithm is an extension of Dijkstra’s Algorithm that uses heuristics to improve efficiency. It combines the cost to reach a node and an estimated cost to reach the goal, making it particularly useful in pathfinding scenarios. Understanding heuristics is crucial for optimizing this algorithm.
Bellman-Ford Algorithm
The Bellman-Ford Algorithm is another method for finding the shortest paths in graphs, especially when negative weights are involved. It works by relaxing edges repeatedly and can detect negative cycles, making it a versatile choice for certain problems.
Floyd-Warshall Algorithm
The Floyd-Warshall Algorithm is used to find shortest paths between all pairs of nodes in a graph. It uses a dynamic programming approach and is particularly useful for dense graphs. The algorithm has a time complexity of O(V^3), where V is the number of vertices.
Prim’s Algorithm
Prim’s Algorithm is a greedy algorithm that finds the minimum spanning tree of a graph. It starts with a single node and adds edges with the smallest weights until all nodes are connected. This algorithm is efficient for dense graphs and is often used in network design.
Kruskal’s Algorithm
Kruskal’s Algorithm is another method for finding the minimum spanning tree. It sorts all edges and adds them one by one, ensuring no cycles are formed. This algorithm is particularly effective for sparse graphs.
Summary Table of Algorithms
Algorithm | Purpose | Complexity |
---|---|---|
Dijkstra’s Algorithm | Shortest path in weighted graphs | O(E + V log V) |
A* Search Algorithm | Pathfinding with heuristics | O(E) |
Bellman-Ford Algorithm | Shortest paths with negative weights | O(VE) |
Floyd-Warshall Algorithm | All pairs shortest paths | O(V^3) |
Prim’s Algorithm | Minimum spanning tree | O(E log V) |
Kruskal’s Algorithm | Minimum spanning tree | O(E log E) |
Understanding these algorithms is essential for coding interviews, especially for positions at top-tier companies like FAANG. Mastering them will give you a strong advantage in solving complex graph problems effectively.
Shortest Path Problems in Graphs
Understanding Shortest Path
In graph theory, the shortest path problem is about finding the quickest route between two points in a graph. This involves calculating the path that has the least total weight, which can represent distance, time, or cost.
Unweighted Graphs Solutions
For graphs without weights, the best method to find the shortest path is Breadth First Search (BFS). This algorithm explores all neighboring nodes at the present depth before moving on to nodes at the next depth level.
Weighted Graphs Solutions
When dealing with weighted graphs, Dijkstra’s Algorithm is commonly used. It efficiently finds the shortest path from a starting node to all other nodes in the graph. Another option is the A Search Algorithm*, which is often faster due to its heuristic approach.
Implementing Dijkstra’s Algorithm
To implement Dijkstra’s Algorithm, follow these steps:
- Initialize distances from the source to all vertices as infinite, except for the source itself, which is zero.
- Use a priority queue to explore the vertex with the smallest distance.
- Update the distances of neighboring vertices.
- Repeat until all vertices are processed.
Common Pitfalls
- Forgetting to mark nodes as visited can lead to infinite loops.
- Not considering negative weights can cause incorrect results. Dijkstra’s Algorithm does not work with negative weights; use the Bellman-Ford Algorithm instead.
Practice Questions
- Find the shortest path in a grid.
- Implement Dijkstra’s Algorithm for a given graph.
- Solve problems involving weighted edges and negative weights using Bellman-Ford.
Cycle Detection in Graphs
Importance of Cycle Detection
Cycle detection is crucial in graph theory because cycles can lead to infinite loops in algorithms. Detecting cycles helps ensure the correctness of algorithms that rely on traversing graphs.
Detecting Cycles in Directed Graphs
To find a cycle in a directed graph, we can use the depth-first traversal (DFS) technique. It is based on the idea that there is a cycle in a graph only if we revisit a node that is currently in the recursion stack. Here’s a simple approach:
- Mark the current node as visited.
- Add the node to the recursion stack.
- For each adjacent node, check:
- If it is not visited, recursively call DFS.
- If it is in the recursion stack, a cycle is detected.
- Remove the node from the recursion stack after exploring all adjacent nodes.
Detecting Cycles in Undirected Graphs
In undirected graphs, we can also use DFS, but we need to keep track of the parent node to avoid false positives. The steps are:
- Mark the current node as visited.
- For each adjacent node, check:
- If it is not visited, recursively call DFS with the current node as the parent.
- If it is visited and not the parent, a cycle is detected.
Using DFS for Cycle Detection
Using DFS is a common method for cycle detection. It is efficient and straightforward. Here’s a quick summary:
- Time Complexity: O(V + E) where V is vertices and E is edges.
- Space Complexity: O(V) for the recursion stack.
Using Union-Find for Cycle Detection
Another method is the Union-Find algorithm, which is particularly useful for undirected graphs. It works by:
- Initializing a parent array for each node.
- For each edge, check if the two nodes belong to the same set:
- If yes, a cycle is detected.
- If no, union the two sets.
Example Problems
- Detect Cycle in a Directed Graph
- Detect Cycle in an Undirected Graph
- Course Schedule Problem (Topological Sort)
Cycle detection is a fundamental concept in graph theory that helps prevent errors in algorithms and ensures efficient processing of data structures.
Topological Sorting and Its Applications
Understanding Topological Sort
Topological sorting is a way to arrange the vertices of a directed graph. In topological sorting, we need to print a vertex before its adjacent vertices. For example, in a graph, the vertex ‘5’ should be printed before any vertex that points to it. This is especially useful in scenarios like scheduling tasks where some tasks depend on others.
Kahn’s Algorithm
Kahn’s Algorithm is one method to achieve topological sorting. Here’s how it works:
- Calculate the in-degree (number of incoming edges) for each vertex.
- Add all vertices with an in-degree of zero to a queue.
- While the queue is not empty:
- Remove a vertex from the queue and add it to the topological order.
- Decrease the in-degree of its neighbors. If any neighbor’s in-degree becomes zero, add it to the queue.
DFS-Based Topological Sort
Another way to perform topological sorting is using Depth First Search (DFS). The steps are:
- Mark all vertices as unvisited.
- For each unvisited vertex, perform a DFS.
- After visiting all neighbors, add the vertex to the topological order.
Applications in Coding Interviews
Topological sorting is commonly used in:
- Task scheduling
- Course prerequisite management
- Build systems
Common Challenges
Some challenges you might face include:
- Detecting cycles in the graph, as topological sorting is only possible for Directed Acyclic Graphs (DAGs).
- Implementing the algorithms efficiently.
Practice Problems
To master topological sorting, try these problems:
- Course Schedule
- Alien Dictionary
- Task Scheduler
Topological sorting is a powerful tool in graph theory, especially for problems involving dependencies. Understanding it can greatly enhance your problem-solving skills in coding interviews.
Network Flow Algorithms
Introduction to Network Flow
Network flow is a way to model how things move through a network. It helps us understand how to send the most items from one point to another without exceeding limits. Mastering network flow is crucial for solving many coding problems.
Ford-Fulkerson Method
The Ford-Fulkerson method is a popular way to find the maximum flow in a network. It uses paths to push flow from the source to the sink until no more flow can be sent. This method is efficient and forms the basis for other algorithms.
Edmonds-Karp Algorithm
The Edmonds-Karp algorithm is an implementation of the Ford-Fulkerson method for computing a maximal flow in a flow network. It uses BFS to find the shortest augmenting paths, making it easier to understand and implement.
Applications of Network Flow
Network flow algorithms are used in various real-world scenarios, such as:
- Transportation: Optimizing routes for delivery trucks.
- Telecommunications: Managing data flow in networks.
- Project Management: Scheduling tasks with dependencies.
Common Interview Questions
When preparing for interviews, you might encounter questions like:
- Explain the Ford-Fulkerson method.
- How does the Edmonds-Karp algorithm work?
- Can you give an example of a network flow problem?
Practice Problems
To get better at network flow algorithms, try these practice problems:
- Maximum Flow Problem
- Minimum Cut Problem
- Flow Network Challenges
Conclusion
Understanding network flow algorithms is essential for coding interviews. They help solve complex problems efficiently and are widely applicable in many fields.
"Mastering these algorithms can significantly boost your problem-solving skills in coding interviews."
Minimum Spanning Tree Algorithms
Understanding MST
A minimum spanning tree (MST) is defined as a spanning tree that has the minimum weight among all the possible spanning trees. This means it connects all the vertices in a graph with the least total edge weight.
Kruskal’s Algorithm
- Sort all the edges in non-decreasing order of their weight.
- Pick the smallest edge. Check if it forms a cycle with the spanning tree formed so far. If it doesn’t, include it in the MST.
- Repeat until there are (V-1) edges in the MST, where V is the number of vertices.
Prim’s Algorithm
- Start with a single vertex and add edges to the MST.
- Choose the smallest edge that connects a vertex in the MST to a vertex outside it.
- Repeat until all vertices are included in the MST.
Comparison of Kruskal’s and Prim’s
Feature | Kruskal’s Algorithm | Prim’s Algorithm |
---|---|---|
Edge Selection | Global | Local |
Data Structure | Edge List | Adjacency List |
Complexity | O(E log E) | O(E + V log V) |
Applications of MST
- Network Design: Minimizing the cost of connecting different points.
- Cluster Analysis: Grouping data points based on similarity.
- Approximation Algorithms: For NP-hard problems.
Practice Problems
- Find the MST of a given graph.
- Compare the performance of Kruskal’s and Prim’s algorithms on different graphs.
Common Mistakes to Avoid
- Forgetting to check for cycles in Kruskal’s algorithm.
- Not updating the edge list correctly in Prim’s algorithm.
Understanding MST algorithms is crucial for optimizing network designs and solving various real-world problems efficiently.
Graph Coloring Techniques
Introduction to Graph Coloring
Graph coloring is a method where we assign colors to the vertices of a graph so that no two adjacent vertices have the same color. This is important in many applications, such as scheduling and resource allocation.
Greedy Coloring Algorithm
One common approach to graph coloring is the Greedy Coloring Algorithm. This algorithm works by assigning colors to vertices one by one, ensuring that no two adjacent vertices share the same color. Here’s a simple step-by-step process:
- Start with the first vertex and assign it the first color.
- Move to the next vertex and assign the lowest numbered color that hasn’t been used by its neighbors.
- Repeat until all vertices are colored.
Backtracking for Coloring
For more complex graphs, a backtracking approach can be used. This method tries to color the graph and backtracks if it finds a conflict. It’s more thorough but can be slower.
Applications in Coding Interviews
Graph coloring techniques are often used in coding interviews to solve problems like:
- Scheduling tasks
- Register allocation in compilers
- Map coloring problems
Common Mistakes
When working with graph coloring, avoid these common pitfalls:
- Forgetting to check adjacent vertices before assigning a color.
- Using too many colors when fewer would suffice.
Practice Questions
To master graph coloring, try these practice questions:
- Color a graph with a given number of colors.
- Find the minimum number of colors needed to color a graph.
Conclusion
Understanding graph coloring is essential for solving various problems in coding interviews. Mastering these techniques can give you an edge in your preparation.
Handling Special Graph Structures
Trees as Graphs
Trees are a special type of graph that have no cycles and are connected. They are often used in coding interviews because they simplify many problems. Understanding trees is crucial as they can be represented as graphs, but with specific properties.
Graphs with Cycles
Graphs can have cycles, which means you can start at one node and return to it by following edges. This can complicate traversal algorithms. When working with graphs that have cycles, it’s important to keep track of visited nodes to avoid infinite loops.
Disjoint Graphs
Disjoint graphs consist of two or more separate components that are not connected. When solving problems involving disjoint graphs, you may need to use algorithms that can handle multiple components, such as Depth First Search (DFS) or Breadth First Search (BFS).
Graphs Represented as Matrices
Graphs can also be represented as matrices, where each cell corresponds to a node. This representation is useful for certain algorithms, especially when dealing with grid-based problems. Here’s a simple example of how a graph can be represented:
Node | Connections |
---|---|
0 | 1, 2 |
1 | 0, 3 |
2 | 0, 3 |
3 | 1, 2 |
Handling Large Graphs
When working with large graphs, memory management becomes crucial. You may not be able to store the entire graph in memory. Instead, consider using techniques like adjacency lists or only storing necessary edges to optimize space.
Practice Problems
- Clone Graph
- Number of Islands
- Course Schedule
- Graph Valid Tree
In coding interviews, being familiar with different graph structures can help you quickly identify the right approach to solve problems. Understanding how to handle special cases will give you an edge in your interviews.
Graph Theory in Real-World Applications
Social Network Analysis
Graphs are essential in understanding social networks. Each user can be a node, and connections between them are edges. This helps in analyzing relationships, finding influencers, and understanding community structures.
Web Page Ranking
Search engines use graphs to rank web pages. Each page is a node, and links between them are edges. Algorithms like PageRank utilize this structure to determine the importance of a page based on its connections.
Network Routing
In communication networks, graphs help in routing data efficiently. Nodes represent routers, and edges represent connections. This allows for optimal pathfinding, ensuring data reaches its destination quickly.
Biological Network Analysis
Graphs are used to model biological systems, such as protein interactions. Each protein is a node, and interactions are edges. This helps in understanding complex biological processes and disease mechanisms.
Recommendation Systems
Many recommendation systems use graphs to suggest products or content. Users and items are nodes, and interactions (like purchases or views) are edges. This structure helps in providing personalized recommendations.
Application | Description |
---|---|
Social Network Analysis | Analyzing relationships and community structures. |
Web Page Ranking | Ranking pages based on their connections. |
Network Routing | Efficient data routing in communication networks. |
Biological Network Analysis | Modeling interactions in biological systems. |
Recommendation Systems | Suggesting products based on user interactions. |
Graphs can be used to model a wide variety of real-world problems, including social networks, transportation networks, and communication networks.
Understanding these applications can greatly enhance your problem-solving skills in coding interviews. By recognizing how graph theory applies to real-world scenarios, you can approach problems with a more informed perspective.
Dynamic Programming on Graphs
Introduction to DP on Graphs
Dynamic programming, or DP, is a method used in mathematics and computer science to solve complex problems by breaking them down into simpler subproblems. This technique is especially useful in graph-related problems where optimal solutions are needed.
Shortest Path with DP
When dealing with graphs, dynamic programming can help find the shortest path efficiently. For example, in a weighted graph, you can use DP to keep track of the shortest distance to each node as you explore the graph.
Longest Path in a DAG
In a Directed Acyclic Graph (DAG), you can also use DP to find the longest path. This involves calculating the longest distance from the starting node to each node, ensuring you only consider paths that follow the direction of the edges.
DP for Counting Paths
Dynamic programming can also be applied to count the number of distinct paths between two nodes in a graph. By storing the number of ways to reach each node, you can efficiently calculate the total paths.
Common Challenges
Here are some common challenges you might face when using DP on graphs:
- Identifying overlapping subproblems: Recognizing when the same subproblem is solved multiple times.
- State representation: Deciding how to represent the state in your DP table.
- Transition relations: Figuring out how to transition from one state to another.
Practice Problems
To master dynamic programming on graphs, consider practicing the following problems:
- 0/1 Knapsack
- Climbing Stairs
- Coin Change
- Longest Increasing Subsequence
- Longest Common Subsequence
- Word Break Problem
- Combination Sum
- House Robber
- Unique Paths
- Jump Game
Mastering dynamic programming on graphs can significantly improve your problem-solving skills in coding interviews. Focus on understanding the core concepts and practicing various problems to build your confidence.
Graph Theory Problem-Solving Strategies
Identifying Graph Problems
When faced with a problem, the first step is to identify if it can be represented as a graph. Look for relationships between items, such as connections or paths. Here are some common indicators:
- Pairs of items that are connected.
- Hierarchical structures like trees.
- Networks where items interact.
Choosing the Right Algorithm
Once you identify a graph problem, the next step is to select the appropriate algorithm. Here’s a quick guide:
Problem Type | Recommended Algorithm |
---|---|
Shortest Path (Unweighted) | BFS |
Shortest Path (Weighted) | Dijkstra’s or A* |
Cycle Detection | DFS or Union-Find |
Topological Sorting | DFS |
Breaking Down Complex Problems
For complex problems, break them down into smaller parts. This can make it easier to solve. Here’s how:
- Identify subproblems that can be solved independently.
- Solve each subproblem using the appropriate algorithm.
- Combine the solutions to form the final answer.
Optimizing Solutions
After finding a solution, think about how to make it better. Consider:
- Reducing time complexity by using more efficient algorithms.
- Minimizing space usage by avoiding unnecessary data storage.
Remember, the goal is to find a short fundamental list of questions to ask yourself that will always lead to the solution, not just categorizing by algorithms or techniques.
Common Pitfalls
Be aware of common mistakes:
- Ignoring edge cases that could affect your solution.
- Overcomplicating the problem instead of simplifying it.
- Not testing your solution with different inputs.
Practice Questions
To improve your skills, practice with these questions:
- Detecting cycles in a graph.
- Finding the shortest path in a maze.
- Implementing a topological sort.
By following these strategies, you can enhance your problem-solving skills in graph theory and perform better in coding interviews.
Graph Theory Resources and Tools
Books and Tutorials
When diving into graph theory, several resources can help you grasp the concepts effectively:
- "Introduction to Graph Theory" by Douglas B. West
- "Graph Theory" by Reinhard Diestel
- Online tutorials like graph theory tutorial that cover various topics such as characteristics, Eulerian graphs, and trees.
Online Courses
Many platforms offer courses specifically focused on graph theory:
- Coursera: Offers courses on algorithms that include graph theory.
- edX: Provides comprehensive courses on data structures and algorithms.
Coding Practice Platforms
Practicing coding problems is crucial for mastering graph theory. Here are some platforms:
- LeetCode: Features a variety of graph-related problems.
- HackerRank: Offers challenges that focus on graph algorithms.
Graph Visualization Tools
Visualizing graphs can enhance understanding. Some useful tools include:
- Graphviz: A tool for creating visual representations of graphs.
- Gephi: An open-source software for network visualization.
Community and Forums
Engaging with others can provide insights and support:
- Stack Overflow: A great place to ask questions and share knowledge.
- Reddit: Subreddits like r/algorithms can be helpful for discussions.
Additional Reading
For those looking to deepen their knowledge, consider:
- Research papers on advanced graph algorithms.
- Blogs and articles that discuss real-world applications of graph theory.
Understanding graph theory is essential for coding interviews, as it helps in solving complex problems efficiently. Mastering these resources will give you a solid foundation.
Common Graph Theory Interview Questions
Types of Questions Asked
In coding interviews, you may encounter various types of graph-related questions. Here are some common categories:
- Traversal Questions: Implementing BFS or DFS.
- Shortest Path Problems: Finding the shortest path using Dijkstra’s or Bellman-Ford algorithms.
- Cycle Detection: Identifying cycles in directed or undirected graphs.
- Minimum Spanning Tree: Using Prim’s or Kruskal’s algorithms.
How to Approach Graph Problems
When faced with a graph problem, follow these steps:
- Understand the Problem: Read the question carefully and clarify any doubts.
- Identify the Graph Type: Determine if it’s directed, undirected, weighted, or unweighted.
- Choose the Right Algorithm: Based on the problem type, select an appropriate algorithm.
- Implement and Test: Write your code and test it with different cases.
Time and Space Complexity
Understanding the efficiency of your solution is crucial. Here’s a quick reference:
Algorithm | Time Complexity | Space Complexity |
---|---|---|
BFS | O(V + E) | O(V) |
DFS | O(V + E) | O(V) |
Dijkstra’s | O((V + E) log V) | O(V) |
Bellman-Ford | O(V * E) | O(V) |
Prim’s | O(E log V) | O(V) |
Kruskal’s | O(E log E) | O(V) |
Common Mistakes to Avoid
- Ignoring Edge Cases: Always consider empty graphs or graphs with one node.
- Misunderstanding Graph Types: Clarify if the graph is directed or undirected.
- Not Testing Thoroughly: Test your solution with various inputs to ensure accuracy.
Example Questions
- Clone a Graph: Given a graph, create a deep copy of it.
- Course Schedule: Determine if you can finish all courses given prerequisites.
- Number of Islands: Count the number of islands in a 2D grid.
Mock Interview Tips
- Practice Regularly: Use platforms like LeetCode or HackerRank to practice.
- Explain Your Thought Process: During mock interviews, articulate your reasoning clearly.
- Review Mistakes: After practice, analyze any errors to improve your understanding.
Remember: Mastering graph data structure and algorithms is essential for coding interviews. Focus on practicing various problems to build confidence and skill.
Preparing for Graph Theory Interviews
Study Plan
To effectively prepare for your graph theory interviews, create a structured study plan. Focus on key concepts and practice regularly. Here’s a simple plan to follow:
- Understand the Basics: Start with definitions and basic terminologies.
- Learn Graph Representations: Familiarize yourself with adjacency lists, matrices, and edge lists.
- Master Traversal Techniques: Practice Depth First Search (DFS) and Breadth First Search (BFS).
- Explore Advanced Algorithms: Study algorithms like Dijkstra’s and Prim’s.
- Practice Problems: Solve various graph-related problems to reinforce your learning.
Key Topics to Cover
Here are the essential topics you should focus on:
- Graph representations
- Traversal techniques (DFS, BFS)
- Shortest path algorithms
- Cycle detection methods
- Minimum spanning trees
- Graph coloring techniques
Practice Strategies
To enhance your skills, consider these strategies:
- Daily Practice: Dedicate time each day to solve graph problems.
- Mock Interviews: Simulate interview conditions with a friend or mentor.
- Review Mistakes: Analyze your errors to avoid repeating them.
Mock Interviews
Participate in mock interviews to build confidence. This will help you get used to the pressure of real interviews and improve your problem-solving speed.
Reviewing Mistakes
After each practice session, take time to review your mistakes. Understanding where you went wrong is crucial for improvement.
Final Tips
- Stay calm and think clearly during the interview.
- Don’t hesitate to ask clarifying questions if you don’t understand the problem.
- Practice makes perfect; the more you practice, the better you will become!
Graph Theory Challenges and Competitions
Popular Coding Competitions
Many coding competitions focus on graph theory, providing a platform to test your skills. Here are some notable ones:
- International Olympiad in Informatics (IOI): A prestigious competition where exploring graph theory through IOI challenges can significantly boost your chances of success.
- Google Code Jam: Features various graph-related problems that require innovative solutions.
- Facebook Hacker Cup: Includes challenges that often involve graph algorithms.
Graph Theory Challenges
Graph theory challenges can vary in complexity. Here are some common types:
- Cycle Detection: Identifying cycles in directed and undirected graphs.
- Shortest Path Problems: Finding the shortest path using algorithms like Dijkstra’s.
- Minimum Spanning Tree: Problems that require constructing a minimum spanning tree using Prim’s or Kruskal’s algorithms.
How to Prepare
To excel in graph theory competitions, consider the following strategies:
- Practice Regularly: Solve problems on platforms like LeetCode and Codeforces.
- Study Algorithms: Focus on key algorithms such as BFS, DFS, and Dijkstra’s.
- Join Online Communities: Engage with peers in forums to discuss strategies and solutions.
Benefits of Participation
Participating in graph theory competitions can:
- Enhance your problem-solving skills.
- Provide exposure to real-world applications of graph theory.
- Improve your coding interview readiness.
Success Stories
Many successful programmers attribute their skills to participation in coding competitions. They often highlight how these experiences helped them in their careers and interviews.
Resources for Competitions
To prepare effectively, utilize the following resources:
- Books: "Introduction to Algorithms" by Cormen et al.
- Online Courses: Platforms like Coursera and edX offer courses on algorithms and data structures.
- Practice Platforms: Websites like HackerRank and Codewars provide a variety of graph problems to solve.
Graph Theory in Advanced Topics
Computational Geometry
Computational geometry deals with the study of geometric objects and their relationships. Understanding this field is crucial for solving problems related to shapes, sizes, and spatial relationships. Here are some key concepts:
- Euclidean Distance: When comparing distances between points, you can use the formula
dx² + dy²
without needing to square root the value. - Circle Overlap: To check if two circles overlap, ensure the distance between their centers is less than the sum of their radii.
String Algorithms
String algorithms are essential for processing and analyzing sequences of characters. They often intersect with graph theory in various applications, such as pattern matching and text processing. Key algorithms include:
- Knuth-Morris-Pratt (KMP): Efficient substring search.
- Rabin-Karp: Uses hashing for quick searches.
Advanced Data Structures
Advanced data structures enhance the efficiency of graph algorithms. Some important ones are:
- Segment Trees: Useful for range queries.
- Fenwick Trees: Efficient for cumulative frequency tables.
System Design Fundamentals
Understanding graph theory is vital in system design, especially for:
- Network Routing: Optimizing paths for data transmission.
- Load Balancing: Distributing workloads across multiple resources.
Real-World Problem Solving
Graph theory is applied in various real-world scenarios, such as:
- Social Networks: Analyzing connections between users.
- Transportation Networks: Optimizing routes for delivery services.
Further Reading
For those interested in diving deeper, consider exploring:
- Books on Graph Theory: Comprehensive texts that cover both theory and applications.
- Online Courses: Platforms offering specialized courses in graph algorithms and their applications.
Graph theory is not just a theoretical concept; it has practical applications in many fields, making it essential for aspiring developers and engineers.
Graph Theory for Different Domains
Artificial Intelligence
Graph theory plays a crucial role in artificial intelligence. It helps in modeling relationships between data points, which is essential for algorithms like recommendation systems and social network analysis. For instance, in AI, graphs can represent connections between users and items, allowing for personalized suggestions.
Network Security
In network security, graphs are used to model networks and detect vulnerabilities. By analyzing the connections between different nodes (like computers), security experts can identify potential threats and improve defenses. This is particularly important in understanding how attacks spread through networks.
Data Science
Graph theory is also significant in data science. It helps in visualizing complex data relationships and can be used in clustering algorithms. For example, social network analysis often employs graph structures to understand user interactions and behaviors.
Software Engineering
In software engineering, graphs are used to represent dependencies between modules or components. This is vital for tasks like build systems and project management, where understanding the relationships between different parts of a project can lead to better organization and efficiency.
Operations Research
In operations research, graphs help in optimizing routes and resources. For example, transportation problems can be modeled using graphs to find the most efficient paths for delivery trucks, minimizing costs and time.
Case Studies
Here are some real-world applications of graph theory across different domains:
Domain | Application Example |
---|---|
Artificial Intelligence | Recommendation Systems |
Network Security | Vulnerability Detection |
Data Science | Social Network Analysis |
Software Engineering | Dependency Management |
Operations Research | Route Optimization |
Graphs and networks find applications across various domains. In healthcare, they help model the spread of diseases. In finance, they assist in analyzing market trends and relationships between assets.
Understanding how graph theory applies in these areas can enhance your problem-solving skills and prepare you for various challenges in coding interviews.
Graph Theory and Algorithm Design
Principles of Algorithm Design
When designing algorithms for graphs, it’s essential to understand the basic principles that guide their creation. These principles include:
- Efficiency: Aim for algorithms that run quickly and use minimal resources.
- Correctness: Ensure that the algorithm produces the right output for all possible inputs.
- Simplicity: Strive for clear and understandable code, which is easier to maintain.
Graph Theory in Algorithm Design
Graph theory plays a crucial role in algorithm design. It helps in solving problems related to network connectivity and optimization. Here are some key points:
- Graphs can represent various real-world problems, such as social networks and transportation systems.
- Understanding graph properties can lead to more efficient algorithms.
Optimizing Graph Algorithms
To make graph algorithms faster and more efficient, consider the following strategies:
- Use appropriate data structures: Choose between adjacency lists, matrices, or edge lists based on the problem.
- Limit memory usage: Sometimes, you don’t need to store the entire graph. For example, only keeping the last two rows of a DP table can save space.
- Avoid unnecessary calculations: For instance, when calculating distances, you can skip square roots if you only need comparisons.
Case Studies
Analyzing real-world examples can provide insights into effective graph algorithm design. Here are a few notable cases:
- Social Network Analysis: Understanding connections between users can help in recommending friends.
- Network Routing: Algorithms like Dijkstra’s help find the shortest path in communication networks.
Common Challenges
While working with graph algorithms, you may face challenges such as:
- Handling large graphs efficiently.
- Detecting cycles in directed and undirected graphs.
- Ensuring algorithms work under various constraints.
Further Reading
To deepen your understanding of graph theory and algorithm design, consider exploring:
- Books on algorithm design and graph theory.
- Online courses that focus on practical applications of graph algorithms.
In summary, mastering graph theory is essential for designing effective algorithms. Understanding the principles and challenges can significantly enhance your problem-solving skills in coding interviews.
Graph theory is a fascinating area of math that helps us understand how different things connect. It plays a big role in designing algorithms, which are step-by-step instructions for solving problems. If you’re curious about how to improve your coding skills and get ready for interviews, check out our website! We offer free coding lessons that can help you learn the algorithms you need to succeed.
Conclusion
In summary, mastering graph theory is essential for success in coding interviews. Understanding key concepts like BFS and DFS can make a big difference in solving problems effectively. Remember, practice is key! Work on various graph problems to build your skills and confidence. Don’t forget to explore different graph representations and algorithms, as they can help you tackle a wide range of questions. With dedication and the right strategies, you’ll be well-prepared to impress your interviewers and excel in your coding journey.
Frequently Asked Questions
What is graph theory?
Graph theory is a branch of mathematics that studies graphs, which are structures made up of nodes (or vertices) connected by edges. It’s important for solving problems in computer science.
Why is graph theory important for coding interviews?
Many coding interviews include questions on graph theory because it helps evaluate problem-solving and algorithm skills, which are key for software development.
What are some common types of graphs?
Common types include directed graphs, undirected graphs, weighted graphs, and unweighted graphs. Each type has its own characteristics and uses.
What is the difference between BFS and DFS?
BFS (Breadth-First Search) explores all neighbors at the present depth before moving on to nodes at the next depth level, while DFS (Depth-First Search) explores as far as possible along one branch before backtracking.
How do you represent a graph in code?
Graphs can be represented using various methods, including adjacency matrices, adjacency lists, and edge lists, depending on the problem requirements.
What are some common graph algorithms?
Some common algorithms include Dijkstra’s Algorithm for shortest paths, Prim’s and Kruskal’s for minimum spanning trees, and BFS/DFS for traversal.
How can I practice graph theory problems?
You can practice graph theory problems on platforms like LeetCode, HackerRank, or CodeSignal, which offer various challenges to improve your skills.
What are some real-world applications of graph theory?
Graph theory is used in social networks, transportation systems, recommendation systems, and many other fields to model relationships and optimize solutions.
What mistakes should I avoid in graph problems?
Common mistakes include not considering edge cases, misunderstanding the graph structure, and not optimizing the algorithm for time or space complexity.
How do I prepare for graph theory questions in interviews?
Focus on understanding key concepts, practicing common algorithms, and solving a variety of problems to build confidence.
What resources can I use to learn more about graph theory?
There are many resources, including online courses, textbooks, and coding practice platforms that provide tutorials and exercises on graph theory.
How can I improve my problem-solving skills for graph theory?
To improve, practice regularly, study different algorithms, and work on problems that require you to apply graph theory concepts creatively.