Mastering Graph Algorithms for Coding: A Comprehensive Guide
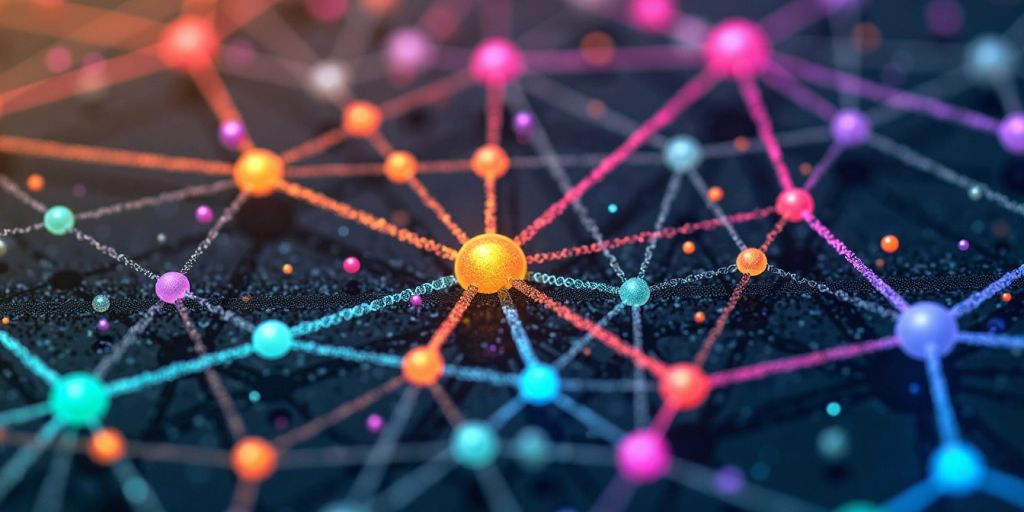
Graph algorithms are essential tools in computer science that help us solve complex problems involving networks. Whether you’re working on social media connections, transportation systems, or game maps, mastering these algorithms can significantly improve your coding skills. This guide will break down the core concepts, techniques, and applications of graph algorithms in a way that’s easy to understand, making it perfect for beginners and those looking to enhance their knowledge.
Key Takeaways
- Graph algorithms are crucial for solving problems related to networks and connections.
- Understanding the basic concepts like nodes and edges is essential for working with graphs.
- Traversal techniques like DFS and BFS help in exploring graphs effectively.
- Shortest path algorithms, including Dijkstra’s and Bellman-Ford, are vital for finding the best routes in networks.
- Learning about minimum spanning trees and network flow can optimize resource allocation in various applications.
Understanding Graph Algorithms for Coding
What Are Graph Algorithms?
Graph algorithms are a set of procedures designed to solve problems related to graph data structures. A graph consists of nodes (or vertices) connected by edges. These algorithms help in navigating and analyzing the relationships between different nodes. Understanding the fundamentals of graph data structure is crucial for effective coding.
Importance of Graph Algorithms in Coding
Graph algorithms are essential for several reasons:
- Enhanced Coding Skills: They allow you to write more efficient and maintainable code.
- Better Career Opportunities: Knowledge of these algorithms is highly valued in the tech industry.
- Foundational Knowledge: They provide a solid base for learning advanced topics like machine learning.
Common Applications of Graph Algorithms
Graph algorithms are used in various real-world scenarios, including:
- Social Networks: Analyzing connections between users.
- Navigation Systems: Finding the shortest path between locations.
- Network Design: Optimizing the layout of computer networks.
Graph algorithms are the backbone of many applications, enabling efficient data processing and decision-making.
Fundamental Concepts in Graph Theory
Nodes and Edges
In graph theory, nodes (or vertices) are the fundamental units that represent entities, while edges are the connections between these nodes. Each edge can connect two nodes, showing a relationship or pathway between them. For example, in a social network, each person can be a node, and friendships can be the edges connecting them.
Types of Graphs
Graphs can be categorized into several types:
- Directed Graphs: Edges have a direction, indicating a one-way relationship.
- Undirected Graphs: Edges do not have a direction, showing a two-way relationship.
- Weighted Graphs: Edges have weights, representing costs or distances.
- Unweighted Graphs: Edges do not have weights.
Graph Representations
Graphs can be represented in various ways:
- Adjacency Matrix: A 2D array where each cell indicates if an edge exists between nodes.
- Adjacency List: A list where each node has a list of its connected nodes.
- Edge List: A list of all edges in the graph, showing pairs of connected nodes.
Representation Type | Description | Pros | Cons |
---|---|---|---|
Adjacency Matrix | 2D array of edges | Fast lookups | Space inefficient for sparse graphs |
Adjacency List | List of edges per node | Space efficient | Slower lookups |
Edge List | List of edges | Simple to implement | Not efficient for lookups |
Understanding the fundamentals of graph theory is crucial for solving complex problems in computer science. This knowledge lays the groundwork for mastering various graph algorithms and their applications.
Graph Traversal Techniques
Depth-First Search (DFS)
Depth-First Search, or DFS, is a method for exploring a graph. The algorithm starts from a given source and explores all reachable vertices from the given source. It is similar to preorder tree traversal where we visit the node first and then its children. Here’s how it works:
- Start at the root (or any arbitrary node).
- Mark the node as visited.
- Explore each adjacent node that hasn’t been visited yet.
- Repeat until all nodes are visited.
Breadth-First Search (BFS)
Breadth-First Search, or BFS, is another way to traverse a graph. It explores all the neighbors of a node before moving on to the next level. The steps are:
- Start at the root node.
- Mark it as visited and add it to a queue.
- While the queue is not empty:
- Dequeue a node.
- Visit all its unvisited neighbors, mark them, and enqueue them.
Applications of Graph Traversal
Graph traversal techniques have many practical uses, including:
- Finding the shortest path in a maze.
- Web crawling to index pages.
- Social network analysis to find connections.
Graph traversal techniques are essential for solving many real-world problems, making them a fundamental part of graph algorithms.
Shortest Path Algorithms
Dijkstra’s Algorithm
Dijkstra’s Algorithm is a popular method used to find the shortest path from a starting node to all other nodes in a graph. It works well with graphs that have non-negative weights. The algorithm uses a priority queue to explore the nearest unvisited node and update the shortest path to its neighbors.
Bellman-Ford Algorithm
The Bellman-Ford Algorithm can handle graphs with negative weights. It repeatedly relaxes the edges, ensuring that the shortest path is found even if some edges have negative weights. This algorithm is slower than Dijkstra’s but is more versatile.
Floyd-Warshall Algorithm
The Floyd-Warshall Algorithm finds the shortest paths between all pairs of nodes in a graph. It uses a dynamic programming approach to update the shortest paths iteratively. This algorithm is useful for dense graphs and can handle negative weights as well.
Algorithm | Time Complexity | Space Complexity |
---|---|---|
Dijkstra’s Algorithm | O(E + V log V) | O(V) |
Bellman-Ford Algorithm | O(VE) | O(V) |
Floyd-Warshall Algorithm | O(V^3) | O(V^2) |
The shortest path problem is crucial in many applications, such as navigation systems and network routing. Understanding these algorithms can greatly enhance your coding skills.
In summary, mastering these shortest path algorithms is essential for solving various problems in graph theory. Each algorithm has its strengths and weaknesses, making them suitable for different scenarios. Choose the right one based on your specific needs!
Minimum Spanning Tree Algorithms
Kruskal’s Algorithm
Kruskal’s algorithm is a popular method for finding the minimum spanning tree (MST) of a graph. It works by sorting all the edges in the graph by their weight and adding them one by one to the MST, as long as they don’t form a cycle. Here’s how it works:
- Sort all edges in non-decreasing order of their weight.
- Pick the smallest edge. Check if it forms a cycle with the spanning tree formed so far. If it doesn’t, include it in the MST.
- Repeat until there are enough edges in the MST.
Prim’s Algorithm
Prim’s algorithm is another way to find the MST. It starts with a single vertex and grows the MST by adding the smallest edge that connects a vertex in the MST to a vertex outside it. The steps are:
- Start with any vertex as the initial MST.
- Find the smallest edge connecting the MST to a vertex outside it.
- Add that edge and the new vertex to the MST.
- Repeat until all vertices are included.
Applications of Minimum Spanning Trees
Minimum spanning trees have several important applications, including:
- Network Design: Used in designing least-cost networks, such as telecommunications and computer networks.
- Cluster Analysis: Helps in clustering data points in machine learning.
- Approximation Algorithms: Used in algorithms for solving NP-hard problems.
A minimum spanning tree (MST) is defined as a spanning tree that has the minimum weight among all the possible spanning trees. This property makes MSTs essential in various fields, from computer networking to operations research.
Network Flow Algorithms
Ford-Fulkerson Method
The Ford-Fulkerson method is a popular algorithm used to find the maximum flow in a flow network. It works by repeatedly finding augmenting paths from the source to the sink and increasing the flow along these paths until no more augmenting paths can be found. This method is efficient and can handle various types of networks.
Edmonds-Karp Algorithm
The Edmonds-Karp algorithm is an implementation of the Ford-Fulkerson method that uses Breadth-First Search (BFS) to find the shortest augmenting path. This approach ensures that the algorithm runs in polynomial time, making it suitable for larger networks. The key steps include:
- Initialize the flow to zero.
- While there is an augmenting path, increase the flow.
- Update the capacities of the edges accordingly.
Applications in Real-World Networks
Network flow algorithms have numerous real-world applications. Here are some examples:
- Transportation: Optimizing routes for delivery trucks to minimize costs and time.
- Telecommunications: Managing data flow in networks to ensure efficient communication.
- Supply Chain Management: Ensuring that resources are allocated efficiently from suppliers to consumers.
Network flow algorithms are essential for solving complex problems in various fields, making them a vital part of algorithm studies.
Application Area | Example Use Case |
---|---|
Transportation | Route optimization for delivery services |
Telecommunications | Data packet routing in networks |
Supply Chain Management | Efficient resource allocation |
Advanced Graph Algorithms
A* Search Algorithm
The A* search algorithm is a popular pathfinding and graph traversal method. It combines the benefits of both Dijkstra’s algorithm and Greedy Best-First Search. A* uses a heuristic to estimate the cost from the current node to the goal, allowing it to find the shortest path efficiently. This makes it particularly useful in applications like game development and robotics.
Johnson’s Algorithm
Johnson’s algorithm is designed to find the shortest paths between all pairs of nodes in a graph. It works well for sparse graphs and uses both Dijkstra’s and Bellman-Ford algorithms. The process involves reweighting the edges to ensure all weights are non-negative, which allows Dijkstra’s algorithm to be applied effectively.
Graph Coloring Algorithms
Graph coloring is a method of assigning colors to the vertices of a graph such that no two adjacent vertices share the same color. This concept is widely used in scheduling problems, register allocation in compilers, and frequency assignment in mobile networks. The most common algorithms for graph coloring include:
- Greedy Coloring
- Backtracking
- DSATUR Algorithm
Understanding these advanced algorithms is crucial for solving complex graph problems effectively. They provide powerful tools for optimizing various applications in computer science and beyond.
Dynamic Programming in Graph Algorithms
Memoization Techniques
Dynamic programming (DP) is a powerful method used to solve complex problems by breaking them down into simpler sub-problems. Memoization is a key technique in DP that involves storing the results of expensive function calls and reusing them when the same inputs occur again. This can significantly reduce the time complexity of algorithms.
Dynamic Programming on Trees
When applying dynamic programming to trees, we often use a bottom-up approach. This means we solve the problem starting from the leaves of the tree and work our way up to the root. This method is particularly useful for problems like calculating the height of a tree or finding the longest path.
Applications of Dynamic Programming in Graphs
Dynamic programming can be applied to various graph problems, such as:
- Finding the shortest path in weighted graphs.
- Calculating the maximum flow in a network.
- Solving the Traveling Salesman Problem (TSP).
Problem Type | Dynamic Programming Approach |
---|---|
Shortest Path | Dijkstra’s Algorithm |
Maximum Flow | Ford-Fulkerson Method |
Traveling Salesman Problem | Held-Karp Algorithm |
Dynamic programming is essential for optimizing solutions in graph algorithms, making it easier to tackle complex problems efficiently.
By mastering these techniques, you can enhance your problem-solving skills and tackle a wide range of challenges in coding competitions and real-world applications.
Greedy Algorithms in Graph Theory
Characteristics of Greedy Algorithms
Greedy algorithms are a type of algorithm that makes the best choice at each step, hoping to find the overall best solution. They are often simpler and faster than other methods. Here are some key features:
- Local Optimization: Greedy algorithms focus on making the best choice at the moment.
- No Backtracking: Once a choice is made, it is not reconsidered.
- Efficiency: They usually run faster than other algorithms, making them suitable for many problems.
Greedy Techniques for Graph Problems
Greedy techniques are widely used in graph problems. Some common applications include:
- Minimum Spanning Trees: Algorithms like Kruskal’s and Prim’s help find the least costly way to connect all nodes in a graph.
- Shortest Path Problems: Dijkstra’s algorithm finds the shortest path from a starting node to all other nodes.
- Activity Selection: This problem involves selecting the maximum number of activities that don’t overlap.
Limitations of Greedy Algorithms
While greedy algorithms are useful, they have limitations:
- Not Always Optimal: They may not always lead to the best solution. For example, the greedy choice might miss a better overall solution.
- Complex Problems: For some complex problems, other methods like dynamic programming may be more effective.
Greedy algorithms are powerful tools, but understanding their limitations is crucial for effective problem-solving.
Algorithm Type | Example Problem | Optimal Solution? |
---|---|---|
Minimum Spanning Tree | Connecting cities | Yes |
Shortest Path | Finding the quickest route | Yes |
Activity Selection | Scheduling tasks | Yes |
Knapsack Problem | Maximizing value with weight | No |
Graph Algorithms for Competitive Programming
Common Graph Problems in Competitions
Graph algorithms are essential in competitive programming. They help solve various problems efficiently. Here are some common types of graph problems you might encounter:
- Finding the shortest path between two nodes.
- Detecting cycles in a graph.
- Finding connected components in an undirected graph.
- Calculating the minimum spanning tree.
Optimizing Graph Algorithms for Speed
To excel in competitions, you need to optimize your graph algorithms. Here are some tips:
- Choose the right algorithm based on the problem type.
- Use efficient data structures like adjacency lists or matrices.
- Analyze time and space complexity to ensure your solution is optimal.
Practice Problems and Solutions
Practicing is key to mastering graph algorithms. Here’s a structured approach:
Problem Type | Example Problem | Suggested Platform |
---|---|---|
Shortest Path | Dijkstra’s Algorithm | LeetCode |
Cycle Detection | Detect Cycle in a Directed Graph | HackerRank |
Minimum Spanning Tree | Prim’s Algorithm | Codeforces |
Graph Traversal | BFS and DFS | GeeksforGeeks |
Remember: Consistent practice is crucial for success in competitive programming. Focus on understanding the algorithms and their applications.
By mastering these concepts, you can tackle graph-related challenges with confidence and skill. Graph algorithms are a vital part of the top 10 algorithms and data structures for competitive coding.
Tools and Resources for Learning Graph Algorithms
Recommended Books and Courses
To master graph algorithms, consider starting with these resources:
- Books:
- Introduction to Algorithms by Cormen, Leiserson, Rivest, and Stein
- Algorithms Unlocked by Thomas H. Cormen
- Online Courses:
- Coursera: Data Structures and Algorithms Specialization
- Udacity: Data Structures and Algorithms Nanodegree
Online Platforms for Practice
Practicing is key to mastering graph algorithms. Here are some platforms:
- LeetCode: Offers a variety of graph problems to solve.
- HackerRank: Provides challenges specifically focused on graph algorithms.
- GeeksforGeeks: A comprehensive resource for learning and practicing graph algorithms.
Community and Peer Learning
Engaging with others can enhance your learning experience. Join these communities:
- Reddit: Subreddits like r/algorithms and r/learnprogramming.
- Stack Overflow: A great place to ask questions and share knowledge.
Remember: The graph data structure is a collection of nodes connected by edges. It’s used to represent relationships between different entities.
By utilizing these tools and resources, you can effectively enhance your understanding of graph algorithms and improve your coding skills.
If you’re eager to dive into the world of graph algorithms, there are plenty of tools and resources available to help you learn. From interactive tutorials to comprehensive study plans, you can find everything you need to boost your coding skills. Don’t wait any longer—visit our website to start your journey in mastering graph algorithms today!
Final Thoughts on Graph Algorithms
In conclusion, mastering graph algorithms is a vital step for anyone looking to excel in coding. These algorithms help us understand how to navigate complex networks and solve real-world problems. By practicing regularly and using the right resources, you can build a strong foundation in graph algorithms. Remember, the key to success is consistent practice and a willingness to learn. So keep coding, stay curious, and don’t hesitate to seek help when needed. Your journey in mastering graph algorithms will open many doors in your coding career!
Frequently Asked Questions
What are graph algorithms?
Graph algorithms are step-by-step methods used to solve problems involving graphs, which are made up of nodes and edges.
Why are graph algorithms important?
Graph algorithms are crucial because they help us solve many real-world problems, like finding the shortest route on a map.
What are some common uses for graph algorithms?
Graph algorithms are often used in navigation systems, social networks, and network flow analysis.
What is the difference between DFS and BFS?
DFS, or Depth-First Search, explores as far as possible down one path before backtracking, while BFS, or Breadth-First Search, explores all neighbors at the present depth before moving on.
What is Dijkstra’s Algorithm?
Dijkstra’s Algorithm is a way to find the shortest path from one node to all other nodes in a weighted graph.
What is a minimum spanning tree?
A minimum spanning tree is a subset of a graph that connects all the vertices together without any cycles and with the minimum possible total edge weight.
How do greedy algorithms work in graphs?
Greedy algorithms make the best choice at each step, hoping that these choices will lead to a globally optimal solution.
How can I practice graph algorithms?
You can practice graph algorithms on coding platforms like LeetCode or HackerRank, where you can find various problems to solve.