Mastering Git Cherry Pick: A Comprehensive Guide to Selective Commit Management
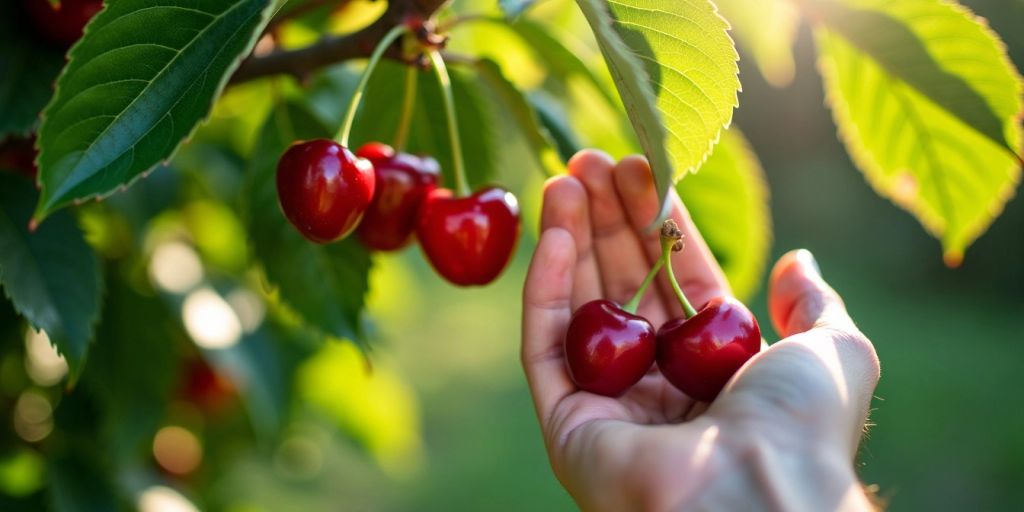
Git is a powerful tool that helps developers keep track of changes in their code. One of its handy features is cherry-picking, which lets you select specific changes from one branch and apply them to another. This guide will help you understand how to use cherry-picking effectively, when to use it, and what to watch out for.
Key Takeaways
- Cherry-picking allows you to choose specific commits from one branch to apply to another.
- It’s useful for fixing bugs, adding features, or moving changes without merging an entire branch.
- You can cherry-pick single commits or ranges of commits easily with Git commands.
- Be aware of conflicts that may arise and know how to resolve them manually.
- Using cherry-picking wisely helps maintain a clean project history.
Understanding Git Cherry Pick
Definition and Purpose
Git cherry-pick is a command that allows you to select specific commits from one branch and apply them to another. This means you can take changes from one branch and add them to another without merging the entire branch. It’s a handy tool for developers who want to manage their code more effectively.
How It Differs from Merge and Rebase
Cherry-picking is different from merging and rebasing in a few key ways:
- Merging combines entire branches, bringing all changes together.
- Rebasing rewrites commit history, moving commits to a new base.
- Cherry-picking allows you to pick and choose specific commits, making it more selective.
Common Use Cases
Here are some common situations where cherry-picking is useful:
- Bug Fixes: If a bug is fixed in one branch, you can cherry-pick that fix to another branch.
- Feature Backporting: You can take new features from a development branch and apply them to an older version.
- Selective Changes: If you want to move specific changes from one branch to another without affecting other commits.
Cherry-picking is a powerful way to manage your code, allowing for flexibility in how changes are applied across branches.
In summary, git cherry-pick is a valuable tool for developers, enabling them to manage their codebase with precision and control. It’s especially useful when you want to apply specific changes without merging everything from one branch to another.
When to Use Git Cherry Pick
Bug Fixes
Cherry-picking is a great way to apply bug fixes from one branch to another. If a bug is fixed in a different branch, you can easily bring that fix over without merging everything else. This saves time and keeps your code clean.
Feature Backporting
Sometimes, you might develop a new feature in a newer version of your project. If you want to use that feature in an older version, cherry-picking allows you to do just that. This is known as feature backporting.
Selective Changes
If you accidentally made some changes in the wrong branch, cherry-picking lets you move those specific changes to the right branch. This is useful for keeping your project organized and ensuring that only the right changes are applied.
Cherry-picking is especially useful when you need to apply hotfixes to several branches or selectively incorporate features without merging entire branches.
Summary of Use Cases
Use Case | Description |
---|---|
Bug Fixes | Apply fixes from one branch to another without merging all changes. |
Feature Backporting | Bring new features from a newer version to an older version. |
Selective Changes | Move specific changes made in the wrong branch to the correct one. |
Preparing for a Cherry Pick
Before you start cherry-picking commits, it’s important to prepare properly. This ensures a smooth process and helps avoid potential issues. Here are the key steps to follow:
Identifying Commits to Cherry Pick
- Find the commit hashes: Use the
git log
command to see the history of commits. Look for the specific commits you want to cherry-pick. - Make a note of the hashes: Write down the hashes of the commits you wish to apply to your target branch.
- Check the changes: Review the changes in each commit to ensure they are what you need.
Checking Out the Target Branch
- Switch to the target branch: Use the command
git checkout <target-branch>
to move to the branch where you want to apply the changes. - Confirm your branch: Run
git branch
to make sure you are on the correct branch before proceeding.
Ensuring a Clean Working Directory
- Check for uncommitted changes: Use
git status
to see if there are any changes that need to be committed or stashed. - Commit or stash changes: If there are changes, either commit them or stash them using
git stash
to keep your working directory clean.
Remember: A clean working directory helps prevent conflicts and makes the cherry-pick process smoother.
By following these steps, you can set yourself up for a successful cherry-pick operation. This preparation is crucial for maintaining a clean history and avoiding complications during the cherry-pick process.
Executing a Basic Cherry Pick
Using the Git Cherry Pick Command
To start cherry-picking, you need to use the command git cherry-pick <commit-hash>
. This command allows you to apply specific changes from one branch to another. Cherry-picking is a powerful way to manage your commits. Here’s how to do it step by step:
- Identify the Commit: First, find the commit hash you want to cherry-pick. You can use
git log
to see the list of commits. - Checkout the Target Branch: Switch to the branch where you want to apply the changes using
git checkout <target-branch>
. - Execute the Cherry Pick: Run the command
git cherry-pick <commit-hash>
to apply the changes from the selected commit.
Cherry Picking Multiple Commits
If you want to cherry-pick several commits at once, you can do so by specifying a range. The command looks like this:
git cherry-pick <start-commit-hash>^..<end-commit-hash>
This command will apply all commits from the start to the end, excluding the start commit itself.
Handling Simple Conflicts
Sometimes, conflicts may arise during cherry-picking. If this happens, Git will notify you. Here’s how to handle it:
- Open the files with conflicts.
- Manually resolve the conflicts by editing the files.
- After resolving, use
git add <file>
to stage the changes. - Finally, run
git cherry-pick --continue
to finish the process.
Remember, cherry-picking is a useful tool, but it’s important to resolve conflicts carefully to maintain a clean project history.
Advanced Cherry Pick Techniques
Cherry Picking a Range of Commits
You can cherry-pick multiple commits at once by specifying a range. This is useful when you want to apply several changes without picking them one by one. The command looks like this:
git cherry-pick <start-commit-hash>^..<end-commit-hash>
Note: The ^
symbol excludes the starting commit from the range, meaning only the commits after it will be cherry-picked.
Interactive Cherry Picking
Interactive cherry-picking allows you to choose which commits to apply from a list. This is helpful when you want to pick specific changes from a series of commits. You can use the following command:
git cherry-pick -n <commit-hash>
This command applies the changes without committing them immediately, giving you a chance to review and modify them before finalizing.
Cherry Picking with Merge Conflicts
Sometimes, cherry-picking can lead to conflicts if the changes clash with existing code. Here’s how to handle it:
- Identify Conflicts: Git will notify you of any conflicts during the cherry-pick process.
- Resolve Conflicts: Open the files with conflicts and manually fix the issues.
- Continue Cherry Picking: After resolving, use:
git cherry-pick –continue
4. **Abort if Necessary:** If you want to stop the cherry-pick process, use:
```bash
git cherry-pick --abort
Cherry-picking is a powerful tool, but it should be used carefully to avoid creating a messy commit history. Mastering these techniques can greatly enhance your version control skills.
Resolving Conflicts During Cherry Pick
Understanding Conflict Messages
When you cherry-pick a commit, you might run into conflicts. This happens when the changes in the commit clash with the existing code. Git will mark these conflicts in the files, making it clear where the issues are. Some Git commands will show you that there are conflicts like git cherry-pick
, git rebase
, and git pull --rebase
.
Manual Conflict Resolution
To fix conflicts, follow these steps:
- Open the files with conflicts. Look for the conflict markers (like
<<<<<<<
,=======
, and>>>>>>>
). - Decide which changes to keep. You can choose one side, both, or make a new change.
- Remove the conflict markers and save the file.
- After resolving all conflicts, run
git cherry-pick --continue
to finish the cherry-pick.
Using Git Tools for Conflict Resolution
There are tools that can help you resolve conflicts more easily:
- Git GUI Tools: These provide a visual interface to see and resolve conflicts.
- Command Line Tools: Commands like
git mergetool
can help you manage conflicts. - Text Editors: Many text editors have built-in features to highlight conflicts and assist in resolution.
Resolving conflicts can be tricky, but with practice, you’ll get better at it. Always double-check your changes before finalizing them!
Best Practices for Cherry Picking
Maintaining a Clean History
- Keep your commit history tidy: Avoid clutter by only cherry-picking necessary commits.
- Use descriptive commit messages to clarify the purpose of each cherry-picked change.
- Regularly review your branches to ensure they reflect the intended state of your project.
Avoiding Duplicate Commits
- Be cautious when cherry-picking: duplicate commits can confuse your team.
- Before cherry-picking, check if the commit already exists in the target branch.
- Use
git log
to verify the commit history and prevent unnecessary duplication.
Testing Cherry Picked Changes
- Always test your changes after cherry-picking to ensure they work as expected.
- Create a separate branch for testing cherry-picked commits before merging them into the main branch.
- Use automated tests to catch any issues that may arise from the cherry-picked changes.
Cherry-picking is a powerful tool, but it should be used wisely to maintain a clear and effective workflow. By following these best practices, you can enhance your Git experience and ensure smooth collaboration.
Common Pitfalls and How to Avoid Them
Duplicate Commits
One of the main issues with cherry-picking is creating duplicate commits. This happens when you cherry-pick a commit that has already been applied to the target branch. To avoid this:
- Always check the commit history before cherry-picking.
- Use
git log
to see if the commit already exists. - Consider using
git cherry
to identify which commits are not in the target branch.
Lost Changes
Another common problem is lost changes. If you cherry-pick a commit and then make changes to the same code, you might lose those changes if you don’t handle them properly. To prevent this:
- Ensure your working directory is clean before cherry-picking.
- Always test your changes after cherry-picking to confirm everything works as expected.
- Use branches to isolate your changes until you are sure they are stable.
Complex Conflict Resolution
Cherry-picking can lead to complex conflict resolution if the changes you are applying conflict with existing code. Here are some tips to manage conflicts:
- Read the conflict messages carefully to understand what went wrong.
- Use tools like
git mergetool
to help resolve conflicts visually. - If conflicts are too complex, consider merging instead of cherry-picking.
Remember, cherry-picking is a powerful tool, but it should be used with caution. Always double-check your commits and ensure you understand the changes being applied.
Real-World Examples of Cherry Picking
Bug Fix Scenario
In a situation where a critical bug is fixed in a development branch, you might want to apply that fix to your main branch without merging all changes. For example:
- Identify the commit hash of the bug fix.
- Switch to the main branch using
git checkout main
. - Execute
git cherry-pick <commit-hash>
to apply the fix.
This allows you to keep your main branch stable while still addressing important issues.
Feature Backport Scenario
Sometimes, a new feature is developed in a newer version of your code, but you need it in an older version. Here’s how you can handle it:
- Identify the commit hash of the new feature.
- Checkout the older version branch.
- Use
git cherry-pick <commit-hash>
to bring the feature into the older version.
This method helps maintain consistency across versions without merging unnecessary changes.
Selective Change Scenario
If you accidentally made changes in the wrong branch, cherry-picking can help you move those changes to the correct branch. For instance:
- Find the commit hashes of the changes you want to move.
- Checkout the correct branch.
- Run
git cherry-pick <commit-hash>
for each change.
This way, you can keep your branches organized and relevant.
Cherry-picking is a powerful tool that allows developers to manage their code effectively, ensuring that only the necessary changes are applied to the right branches.
By understanding these scenarios, you can effectively utilize cherry-picking in your workflow, making your development process smoother and more efficient.
Tools and Resources for Effective Cherry Picking
Git GUI Tools
Using graphical user interface (GUI) tools can make cherry-picking easier. Here are some popular options:
- GitHub Desktop: A user-friendly client designed for developers who primarily work with GitHub repositories. It simplifies handling Git commands.
- Sourcetree: A free Git client that provides a visual interface for managing repositories, making cherry-picking straightforward.
- GitKraken: A powerful Git GUI that offers a visually appealing way to manage branches and commits, including cherry-picking.
Command Line Tips
When using the command line, here are some helpful tips:
- Use
git log
: This command helps you find commit hashes easily. - Cherry-pick ranges: You can cherry-pick multiple commits at once using the syntax
git cherry-pick <start-commit-hash>^..<end-commit-hash>
. - Resolve conflicts: If conflicts arise, use
git status
to see which files need attention.
Further Reading and Tutorials
To deepen your understanding of cherry-picking, consider these resources:
- Official Git Documentation: A comprehensive guide on all Git commands, including cherry-picking.
- Online Tutorials: Websites like Codecademy and freeCodeCamp offer interactive lessons on Git.
- YouTube Videos: Many creators provide visual guides on using Git effectively, including cherry-picking.
Mastering cherry-picking can greatly enhance your Git workflow. It allows you to apply specific changes without merging entire branches, making your development process more efficient.
Integrating Cherry Pick into Your Workflow
Combining Cherry Pick with Other Git Commands
Integrating cherry-pick into your workflow can enhance your Git experience. Here are some ways to combine it with other commands:
- Branch Management: Use
git checkout
to switch branches before cherry-picking. - Conflict Resolution: If conflicts arise, use
git status
to identify issues andgit add
to stage resolved files. - Commit History: After cherry-picking, use
git log
to review your commit history and ensure everything looks correct.
Automating Cherry Pick Processes
To make cherry-picking easier, consider automating some steps:
- Scripts: Write scripts to automate repetitive cherry-picking tasks.
- Aliases: Create Git aliases for common cherry-pick commands to save time.
- Hooks: Use Git hooks to trigger actions after cherry-picking, like running tests.
Team Collaboration Tips
When working in a team, effective communication is key:
- Document Changes: Always document cherry-picked commits in your project management tool.
- Notify Team Members: Let your team know when you cherry-pick important changes.
- Review Process: Implement a review process for cherry-picked commits to maintain code quality.
Integrating cherry-pick into your workflow can lead to better code management and collaboration. By mastering this tool, you can streamline your development process.
If you want to make coding easier and more effective, try adding Cherry Pick to your routine. This tool can help you manage your code better and make your workflow smoother. Ready to take your coding skills to the next level? Visit our website to start coding for free!
Conclusion
In summary, cherry-picking in Git is a handy tool that lets developers choose specific changes from one branch and apply them to another. This method is especially useful when you want to add important updates without merging everything from the source branch. While cherry-picking can be very effective, it’s important to be aware that it might cause conflicts that you’ll need to fix manually. With the knowledge from this guide, you can confidently use cherry-picking to manage your code changes more precisely and efficiently.
Frequently Asked Questions
What is Git cherry-pick?
Git cherry-pick is a command that lets you take specific changes (commits) from one branch and apply them to another. This is useful when you only want certain changes, not everything from the branch.
When should I use cherry-pick?
You should use cherry-pick when you need to apply a bug fix from another branch, backport features to an older version, or move specific changes to the right branch.
How do I cherry-pick a commit?
To cherry-pick a commit, first switch to the branch where you want to apply the changes. Then use the command ‘git cherry-pick ‘ to apply the specific commit.
What if there are conflicts while cherry-picking?
If there are conflicts, Git will let you know. You will need to manually fix the conflicts in the files, then use ‘git add’ to mark them as resolved before continuing with ‘git cherry-pick –continue’.
Can I cherry-pick multiple commits at once?
Yes, you can cherry-pick multiple commits by using a range. You can do this with the command ‘git cherry-pick ^..’.
What are the risks of using cherry-pick?
Using cherry-pick can lead to duplicate commits in your history if not managed carefully. It can also create complex conflicts that need to be resolved manually.
How can I avoid issues when cherry-picking?
To avoid problems, always ensure your working directory is clean before starting, and carefully check for conflicts during the cherry-pick process.
Is there a way to undo a cherry-pick?
Yes, if you need to undo a cherry-pick, you can use ‘git cherry-pick –abort’ to stop the process before it finishes.