Mastering Git Checkout: A Comprehensive Guide to Branch Management and Version Control
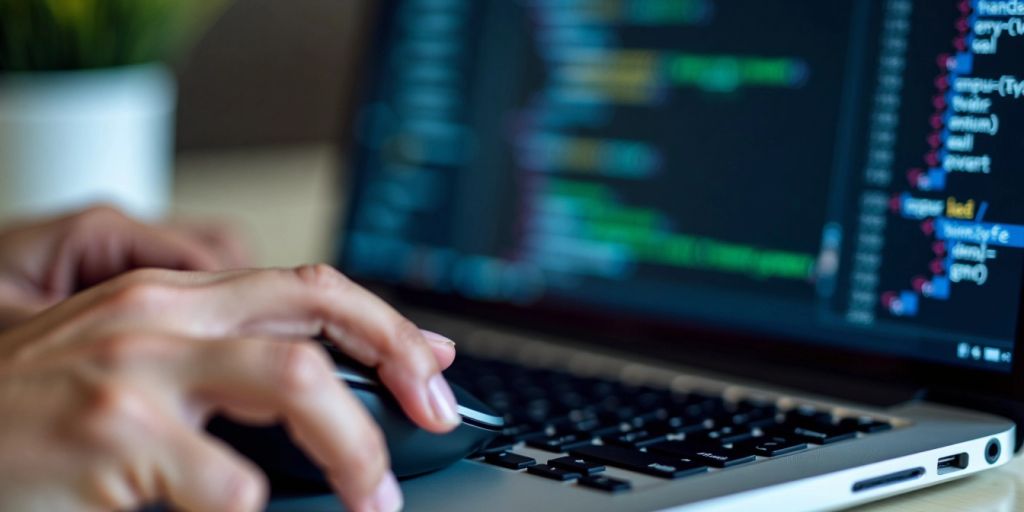
In this guide, we’ll explore how to effectively use Git’s checkout command for managing branches and controlling versions. Git is a powerful tool that helps developers keep track of changes in their projects, making it easier to collaborate and maintain a clean codebase. Whether you’re just starting or looking to sharpen your skills, understanding Git checkout is essential for any developer.
Key Takeaways
- Git checkout is crucial for switching between branches and managing versions.
- Creating branches allows developers to work on features without affecting the main code.
- Merging branches integrates changes, ensuring everyone is on the same page.
- Using Git stash helps save uncommitted changes temporarily when switching tasks.
- Always write clear commit messages to keep a clean project history.
Understanding Git Checkout
What is Git Checkout?
The git checkout command is a key tool in Git that allows you to switch between different branches or check out specific versions of files. This command is essential for managing your project effectively. Here are some important points about it:
- Switching Branches: You can easily move from one branch to another.
- Checking Out Files: Retrieve specific versions of files from your history.
- Creating New Branches: You can create and switch to a new branch in one command.
Basic Git Checkout Commands
Here are some basic commands you should know:
Command | Description |
---|---|
git checkout <branch_name> |
Switch to an existing branch. |
git checkout -b <new_branch> |
Create and switch to a new branch. |
git checkout <file_name> |
Restore a file to its last committed state. |
Common Use Cases for Git Checkout
Git checkout is used in various scenarios, including:
- Feature Development: Working on new features in separate branches.
- Bug Fixes: Quickly switching to a branch dedicated to fixing bugs.
- Experimentation: Trying out new ideas without affecting the main codebase.
Git checkout is a powerful command that helps you manage your project’s history and branches effectively. Mastering it will enhance your workflow significantly.
Setting Up Your Git Environment
Installing Git on Different Operating Systems
To start using Git, you need to install it on your computer. Here’s how to do it for various operating systems:
- Windows: Download the installer from the official Git website and follow the instructions.
- macOS: Git usually comes pre-installed. If not, you can install it using Homebrew by running
brew install git
in the terminal. - Linux: Use your package manager. For example, on Ubuntu, run
sudo apt-get install git
.
Configuring Git for the First Time
After installing Git, you need to set it up. This includes adding your name and email, which will be linked to your commits. Use these commands:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Make sure to replace the placeholders with your actual information.
Verifying Your Git Installation
To confirm that Git is installed correctly, open your terminal or command prompt and type:
git --version
If Git is installed, you will see the version number. This means you are ready to start using Git!
Branch Management with Git Checkout
Creating and Switching Branches
Managing branches is a key part of using Git effectively. Creating and monitoring a new git branch allows you to work on features or fixes without affecting the main code. To create a new branch, you can use the following command:
git checkout -b <branch_name>
This command creates a new branch and switches to it immediately. After making your changes, you can push the new branch to the remote repository with:
git push -u origin <branch_name>
Merging Branches Using Git Checkout
When you’re ready to combine changes from one branch into another, you can merge branches. To do this, first switch to the branch you want to merge into:
git checkout <target_branch>
Then, use the merge command:
git merge <source_branch>
This will integrate the changes from the source branch into the target branch. If there are conflicts, Git will notify you, and you will need to resolve them before completing the merge.
Deleting Branches Safely
Once a branch is no longer needed, it’s good practice to delete it. You can delete a branch using:
git branch -d <branch_name>
This command deletes the branch only if it has been fully merged. If you want to forcefully delete a branch, use:
git branch -D <branch_name>
Deleting branches that are no longer needed helps keep your repository clean and organized.
By mastering these branch management techniques, you can enhance your workflow and maintain a tidy project structure.
Advanced Git Checkout Techniques
Rebasing with Git Checkout
Rebasing is a powerful way to keep your project history clean. It allows you to move or combine commits from one branch to another. This helps maintain a clear and linear commit history. Here’s how to do it:
- Switch to the branch you want to rebase onto:
git checkout <branch_to_rebase_onto>
- Then, rebase the branch you want to move:
git rebase <branch_to_be_rebased>
Cherry-Picking Commits
Cherry-picking lets you select specific commits from one branch and apply them to another. This is useful when you want to take a bug fix or feature without merging the entire branch. To cherry-pick a commit, use:
git cherry-pick <commit_SHA>
Using Git Stash with Checkout
Sometimes, you might need to switch branches but have uncommitted changes. Git stash allows you to temporarily save your changes. Here’s how:
- Stash your changes:
git stash
- Switch to the desired branch:
git checkout <branch_name>
- Reapply your stashed changes:
git stash pop
By mastering these advanced techniques, you can enhance your version control workflow and tackle complex development scenarios with confidence. Experiment with these commands to see how they can optimize your development process.
Technique | Description |
---|---|
Rebasing | Keeps commit history clean and linear. |
Cherry-Picking | Selects specific commits to apply elsewhere. |
Stashing | Temporarily saves changes for later use. |
Handling Merge Conflicts
Identifying Merge Conflicts
When working with Git, merge conflicts happen when two branches have changes in the same part of a file. This can occur during a git merge
or git pull
. Here are some common reasons for conflicts:
- Two branches modify the same line in a file.
- Changes are made in parallel on different branches.
- A merge attempt involves overlapping changes.
Resolving Conflicts Manually
To resolve conflicts, follow these steps:
- Check for conflict markers in the affected files. They look like this:
<<<<<<<
(your changes)=======
(the changes from the other branch)>>>>>>>
(end of the conflict)
- Edit the file to keep the desired changes and remove the conflict markers.
- Stage the resolved files using
git add
. - Commit the changes with a message like "Merge conflict resolved".
Using Git Tools to Resolve Conflicts
Git provides tools to help with conflict resolution. Here are some options:
- Use a text editor to manually resolve conflicts.
- Utilize GUI tools like GitKraken or SourceTree for a visual approach.
- Leverage IDEs like Visual Studio Code, which have built-in merge conflict resolution features.
Resolving merge conflicts is a normal part of collaborative development. Understanding how to handle them effectively is key to maintaining a smooth workflow.
By mastering these techniques, you can confidently manage conflicts and keep your project on track. Remember, this article explains the basics of git merge conflicts and one of the advanced operations of git: resolving a git merge conflict.
Working with Remote Repositories
In today’s software development world, working with remote repositories is essential for teamwork. These repositories allow developers to share their code and keep everything in sync. Here, we will look at the key commands and steps to effectively manage remote repositories.
Cloning Remote Repositories
To start working with a remote repository, you first need to clone it to your local machine. Here’s how:
- Open your terminal or command prompt.
- Use the following command:
git clone <repository_URL>
Replace
<repository_URL>
with the link to the remote repository. - This command creates a local copy of the repository on your machine.
Pushing and Pulling Changes
Once you have your local repository set up, you can share your changes and get updates from others:
- Pushing Changes: After making changes, you can share them with others by using:
git push origin <branch_name>
Replace
<branch_name>
with the name of your branch. - Pulling Changes: To get the latest updates from the remote repository, use:
git pull origin <branch_name>
This command fetches the latest changes and merges them into your local branch.
Handling Remote Branches
When working with remote branches, it’s important to keep track of them:
- Use
git branch -r
to list all remote branches. - To check out a remote branch, first fetch the latest changes:
git fetch
- Then, you can check out the branch:
git checkout <remote_branch_name>
This allows you to work on the latest version of the branch.
Tip: To checkout a remote branch, you first need to fetch the latest changes from the remote repository, then you can checkout the remote branch locally.
By mastering these commands, you can effectively collaborate with your team and manage your projects with ease.
Optimizing Your Workflow with Git Checkout
Using Aliases for Git Commands
Creating aliases for your most-used Git commands can save you time and keystrokes. Here are some common aliases you might consider:
git co
forgit checkout
git br
forgit branch
git ci
forgit commit
Automating Tasks with Git Hooks
Git hooks are scripts that run automatically at certain points in the Git workflow. They can help automate repetitive tasks. Here are a few examples:
- Pre-commit hook: Check for code style issues before committing.
- Post-commit hook: Send notifications after a commit.
- Pre-push hook: Run tests before pushing changes.
Integrating Git with CI/CD Pipelines
Continuous Integration and Continuous Deployment (CI/CD) can streamline your development process. Here’s how to integrate Git:
- Use Git to trigger builds automatically.
- Deploy code to production after successful tests.
- Monitor changes and roll back if necessary.
By using Git stash, you can break down large commits and save your work when switching branches. This helps keep your workflow smooth and organized.
In summary, optimizing your workflow with Git Checkout involves using aliases, automating tasks, and integrating with CI/CD pipelines. These practices can significantly enhance your productivity and collaboration in software development.
Best Practices for Git Checkout
Writing Clear Commit Messages
When you make changes to your project, it’s important to write clear commit messages. This helps everyone understand what changes were made and why. Here are some tips:
- Use simple language.
- Be specific about what you changed.
- Keep it short but informative.
Maintaining a Clean Commit History
A clean commit history makes it easier to track changes. To keep your history tidy:
- Regularly commit and push changes to avoid losing work.
- Use a branching strategy to separate features and fixes.
- Pull before pushing to ensure you have the latest updates.
Branching Strategies for Teams
Using a good branching strategy can help your team work better together. Here are some common strategies:
- Feature branching: Create a new branch for each feature.
- Bug fix branches: Use separate branches for bug fixes.
- Release branches: Prepare a branch for upcoming releases.
By following these best practices, you can avoid conflicts and data loss, making your Git experience smoother and more efficient.
Troubleshooting Common Issues
Undoing Changes with Git Checkout
When you want to undo changes in your working directory, you can use the git checkout
command. This command allows you to revert files back to their last committed state. Be careful, as this will discard any uncommitted changes.
- To undo changes in a specific file:
git checkout -- <file-name>
- To undo changes in all files:
git checkout -- .
- Always check your status before using this command:
git status
Recovering Lost Commits
If you accidentally lose commits, you can often recover them using the reflog. The reflog records updates to the tip of branches and allows you to find lost commits.
- To view the reflog:
git reflog
- Identify the commit hash you want to recover.
- Use the following command to reset to that commit:
git reset --hard <commit-hash>
Dealing with Detached HEAD State
A detached HEAD state occurs when you check out a specific commit instead of a branch. In this state, any new commits you make won’t be saved to a branch. Here’s how to handle it:
- Create a new branch to save your changes:
git checkout -b new-branch-name
- If you want to return to a branch, use:
git switch existing-branch-name
- Always remember to commit your changes before switching branches to avoid losing work.
In Git, solving git issues often involves understanding the commands and their effects. Always back up your work before making significant changes!
Exploring Git Checkout in Real-World Scenarios
Case Study: Feature Development
When working on a new feature, developers often create a separate branch to keep the main codebase stable. This allows for focused development without affecting the main project. Here’s how it typically goes:
- Create a new branch for the feature:
git checkout -b feature-branch
- Develop the feature and commit changes.
- Once completed, merge the feature branch back into the main branch.
Case Study: Bug Fixes
Fixing bugs is a common task in software development. Using Git Checkout, developers can quickly switch to a bug-fix branch:
- Identify the bug and create a branch:
git checkout -b bugfix-branch
- Make necessary changes and commit.
- Merge the bug-fix branch back into the main branch after testing.
Case Study: Code Reviews
Code reviews are essential for maintaining code quality. Here’s how Git Checkout plays a role:
- Create a branch for the review:
git checkout -b review-branch
- Make changes based on feedback.
- Once approved, merge the review branch into the main branch.
In real-world scenarios, mastering Git branching is crucial for effective collaboration and project management. By using branches wisely, teams can work on multiple features or fixes simultaneously without conflicts.
Scenario | Key Commands | Outcome |
---|---|---|
Feature Development | git checkout -b feature-branch |
Isolated feature development |
Bug Fixes | git checkout -b bugfix-branch |
Quick bug resolution |
Code Reviews | git checkout -b review-branch |
Streamlined feedback process |
Security and Compliance with Git
Managing Access Controls
To ensure that only authorized users can access your Git repositories, it’s crucial to implement strong access controls. Here are some key strategies:
- Use SSH keys for secure connections.
- Set up user roles to define permissions clearly.
- Regularly review access logs to monitor activity.
Auditing Changes
Regular audits help maintain the integrity of your codebase. Consider the following practices:
- Track all changes with commit messages.
- Use tools to analyze commit history for unusual patterns.
- Implement automated alerts for unauthorized changes.
Ensuring Compliance with Git Policies
To meet regulatory standards, it’s essential to have clear policies in place. Here are some steps to follow:
- Document your Git workflow to ensure everyone understands the process.
- Train your team on compliance requirements.
- Regularly update policies to reflect changes in regulations.
Maintaining security and compliance is not just a best practice; it’s essential for protecting your project and meeting regulatory standards.
Compliance Aspect | Description |
---|---|
Data Protection | Ensure sensitive data is encrypted and access is restricted. |
Change Management | Document all changes and maintain a clear history. |
User Training | Regularly train users on security practices and compliance requirements. |
By following these guidelines, you can effectively manage security and compliance within your Git environment, ensuring that your projects remain safe and meet necessary standards.
When it comes to using Git, keeping your code safe and following the rules is super important. Make sure you understand how to protect your work and follow the guidelines. If you want to learn more about coding and get ready for your dream job, visit our website today!
Conclusion
In summary, mastering Git checkout is essential for anyone looking to manage their code effectively. By understanding how to create, switch, and merge branches, you can work on multiple features or fixes without getting confused. This guide has covered the basics and some advanced techniques, making it easier for you to collaborate with others. Remember, practice is key! The more you use Git, the more comfortable you’ll become. So dive in, experiment, and enjoy the benefits of version control!
Frequently Asked Questions
What is Git Checkout?
Git Checkout is a command that lets you switch between different branches or versions of your project in Git.
How do I create a new branch?
You can create a new branch by using the command `git checkout -b branch_name`.
What does merging branches mean?
Merging branches means combining the changes from one branch into another, so both branches share the same updates.
How can I delete a branch safely?
To delete a branch safely, make sure you are not on that branch, then use `git branch -d branch_name`.
What should I do if I encounter merge conflicts?
If you face merge conflicts, you need to manually edit the files to resolve the differences and then commit the changes.
Can I undo changes made with Git Checkout?
Yes, you can undo changes by using `git checkout — file_name` to revert a file to its last committed state.
What is the purpose of Git Stash?
Git Stash allows you to temporarily save your changes without committing them, so you can switch branches or work on something else.
How do I push changes to a remote repository?
You can push changes to a remote repository using the command `git push origin branch_name`.