Mastering Frontend Development Tutorials: A Comprehensive Guide for Beginners
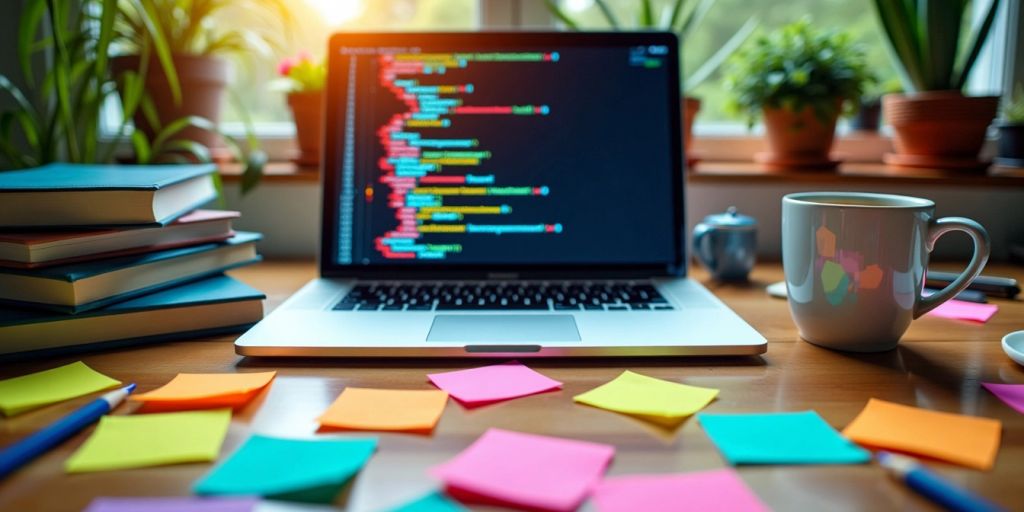
In our digital world, front-end development is key to creating the websites and apps we use every day. This guide is made for beginners who want to learn the basics of front-end development, including how to use HTML, CSS, and JavaScript. By the end, you’ll have a good understanding of what it takes to build user-friendly and interactive web pages.
Key Takeaways
- Front-end development focuses on what users see and interact with on websites.
- HTML, CSS, and JavaScript are the main tools for building web pages.
- Responsive design ensures websites work well on all devices, from phones to desktops.
- Learning frameworks like React or Vue.js can speed up your development process.
- Using version control systems like Git helps track changes and collaborate with others.
Understanding Frontend Development
Frontend development is all about creating the parts of websites that users see and interact with. It involves three main technologies: HTML, CSS, and JavaScript. Each of these plays a unique role in making web pages functional and appealing.
The Role of HTML, CSS, and JavaScript
HTML, or Hypertext Markup Language, is the backbone of any website. It provides the basic structure, like headings and paragraphs. CSS, or Cascading Style Sheets, is used to style this structure, adding colors, fonts, and layouts. Finally, JavaScript makes the website interactive, allowing for features like animations and form validation.
Frontend vs. Backend Development
While frontend development focuses on what users see, backend development deals with the server-side, managing databases and server logic. Both are essential for a fully functional website, but they require different skill sets.
Importance of User Experience
User experience (UX) is crucial in frontend development. A well-designed interface can make a website easy to use and enjoyable. Here are some key points to consider:
- Intuitive Navigation: Users should easily find what they need.
- Responsive Design: Websites must work well on all devices, from phones to desktops.
- Fast Loading Times: Users expect quick access to information.
Frontend development is not just about coding; it’s about creating a seamless experience for users.
In summary, mastering frontend development means understanding how to combine HTML, CSS, and JavaScript to create engaging and user-friendly websites. This knowledge is essential for anyone looking to enter the field of web development.
Essential Frontend Development Skills
In this section, we will explore the key skills that every frontend developer should have. Mastering these skills will help you create engaging and effective web experiences.
HTML and CSS Fundamentals
Understanding the basics of HTML and CSS is crucial. These are the building blocks of web development. Here are some important points:
- HTML structures the content of web pages.
- CSS styles the appearance of that content.
- Learning how to use semantic HTML elements helps in organizing your content better.
JavaScript Mastery
JavaScript is essential for making websites interactive. Here’s what you need to know:
- Variables store data.
- Functions perform actions.
- Conditionals help in decision-making.
- Loops repeat actions.
- DOM manipulation allows you to change the content and structure of a webpage.
Responsive Design and Mobile Optimization
Creating websites that look good on all devices is important. Here are some techniques:
- Use media queries to adjust styles based on screen size.
- Implement flexible layouts that adapt to different screens.
- Optimize images for faster loading on mobile devices.
Version Control with Git
Using Git is vital for tracking changes in your code. It allows you to:
- Collaborate with others easily.
- Keep a history of your project.
- Roll back to previous versions if needed.
Mastering these skills will set a strong foundation for your journey as a frontend developer. Start with the basics of web technologies and build your way up!
Exploring Frontend Frameworks and Libraries
Frontend frameworks and libraries are essential tools that help developers create modern web applications more efficiently. These tools provide pre-built components and reusable code, making it easier to build complex user interfaces.
Introduction to React
React is a popular JavaScript library developed by Facebook. It allows developers to create user interfaces using components, which are reusable pieces of code. React is known for its virtual DOM, which improves performance by minimizing direct interactions with the actual DOM.
Getting Started with Angular
Angular is a full-fledged framework maintained by Google. It provides a comprehensive solution for building dynamic web applications. Angular uses TypeScript, which adds static typing to JavaScript, making it easier to catch errors early in the development process.
Building with Vue.js
Vue.js is a progressive framework that is easy to integrate with other projects. It focuses on the view layer and is great for building single-page applications. Vue.js is known for its simplicity and flexibility, allowing developers to adopt it gradually.
Framework/Library | Key Features | Use Cases |
---|---|---|
React | Component-based, Virtual DOM | Single-page applications, Dynamic UIs |
Angular | Full framework, TypeScript | Large-scale applications, Enterprise solutions |
Vue.js | Progressive, Easy integration | Small to medium projects, Prototyping |
Frontend frameworks and libraries are crucial for modern web development, as they help streamline the process and enhance user experience.
By understanding these frameworks and libraries, you can choose the right tools for your projects and improve your development skills.
Embracing Responsive Design and Cross-Browser Compatibility
In today’s world, where people use many different devices, responsive design is crucial. It helps ensure that websites look good and work well on all screen sizes. Here are some key points to consider:
Fluid Layouts and Flexible Images
- Flexible grids: Use grids that can change based on the screen size. This allows your website to adapt to different devices.
- Flexible images: Make sure images can resize without losing quality. This helps them fit well on any screen.
Media Queries for Responsive Design
- Breakpoints: Set specific points in your design where the layout changes. This is important for making sure your site looks good on phones, tablets, and desktops.
- Responsive media queries: Use coding commands to adjust the size of images and other media based on the device.
Ensuring Cross-Browser Compatibility
To make sure your website works well on all browsers, consider the following:
- Test your site on different browsers to catch any issues.
- Use tools to check for compatibility problems.
Remember, ensuring your web apps run smoothly on all browsers is essential for user satisfaction.
By focusing on these areas, you can create a website that is not only visually appealing but also functional across various platforms. This will enhance the overall user experience and keep visitors engaged.
Harnessing the Power of Frontend Development Tools
Frontend development is made easier with a variety of tools that help developers work more efficiently. These tools are essential for writing, debugging, and collaborating on code.
Code Editors and IDEs
Popular code editors and integrated development environments (IDEs) are crucial for frontend developers. Here are some widely used options:
- Visual Studio Code: Highly customizable with many extensions.
- Sublime Text: Known for its speed and simplicity.
- WebStorm: A powerful IDE specifically for JavaScript.
Browser Developer Tools
Every major web browser comes with built-in developer tools. These tools allow you to:
- Inspect and modify HTML and CSS.
- Debug JavaScript code.
- Analyze website performance.
Build Tools and Task Runners
Build tools and task runners automate repetitive tasks, making development smoother. Here are some examples:
- Webpack: Bundles JavaScript files for usage in a browser.
- Gulp: Automates tasks like minifying code and optimizing images.
- Grunt: A task runner that helps automate tasks in your workflow.
Using the right tools can significantly improve your productivity and the quality of your code.
In summary, mastering these tools is vital for any aspiring frontend developer. They not only enhance your coding experience but also help you create better web applications.
Building Your First Frontend Project
Starting your journey in frontend development can be exciting and a bit overwhelming. Building your first project is a great way to apply what you’ve learned and gain confidence.
Planning Your Project
- Choose a simple idea: Start with something manageable, like a personal portfolio or a basic to-do list app.
- Outline your features: Decide what functionalities you want. For example, if you’re building a to-do list, you might want to add, delete, and mark tasks as complete.
- Sketch your layout: Draw a rough design of how you want your project to look. This will help you visualize the end product.
Choosing the Right Technologies
- HTML: Use it for the structure of your project.
- CSS: Style your project to make it visually appealing.
- JavaScript: Add interactivity and dynamic features.
Implementing Features and Functionalities
- Start coding your project step by step.
- Test each feature as you go to ensure everything works correctly.
- Don’t hesitate to look for help online if you get stuck.
Remember, every expert was once a beginner. Take your time and enjoy the process of learning and creating.
By following these steps, you can successfully build your first frontend project. This experience will not only enhance your skills but also prepare you for more complex projects in the future. Happy coding!
Frontend Development Best Practices
Writing Clean and Maintainable Code
Writing clean code is essential for any developer. It makes your code easier to read and understand. Here are some tips to help you:
- Use clear and descriptive names for variables and functions.
- Keep your code organized by using comments and proper indentation.
- Break your code into smaller, reusable functions.
Following these practices will help you and others understand your code better.
Performance Optimization Techniques
Performance is key in frontend development. Here are some techniques to improve your website’s speed:
- Minimize HTTP requests by combining files.
- Use image optimization tools to reduce file sizes.
- Implement lazy loading for images and videos.
Technique | Description |
---|---|
Minimize HTTP requests | Combine CSS and JavaScript files |
Image optimization | Use tools like TinyPNG to reduce sizes |
Lazy loading | Load images only when they are in view |
Accessibility and Inclusive Design
Making your website accessible is important. Here are some ways to ensure everyone can use your site:
- Use alt text for images.
- Ensure good color contrast for readability.
- Make sure your site is navigable with a keyboard.
Accessibility is not just a feature; it’s a necessity for a better web experience.
Testing and Debugging
Testing your code helps catch errors before they reach users. Here are some methods:
- Use browser developer tools to inspect elements and debug JavaScript.
- Write unit tests to check individual parts of your code.
- Regularly test your site on different devices and browsers to ensure compatibility.
Collaboration and Workflow Efficiency
Working with others can be challenging. Here are some tips to improve teamwork:
- Use version control systems like Git to track changes.
- Hold regular meetings to discuss progress and challenges.
- Use project management tools to keep everyone on the same page.
By following these best practices, you can create better, more efficient, and user-friendly web applications. Remember, React is the ideal front-end framework for building a web application that expects high interaction and frequently-changing states.
Testing and Debugging in Frontend Development
Unit Testing and Integration Testing
Testing is a crucial part of frontend development. It helps ensure that your code works as expected. Unit testing focuses on individual components, while integration testing checks how different parts of your application work together. Here’s a simple breakdown:
Type of Testing | Purpose |
---|---|
Unit Testing | Tests individual components |
Integration Testing | Tests how components work together |
Debugging with Browser Developer Tools
Debugging is the process of finding and fixing errors in your code. You can use browser developer tools to inspect elements, view console messages, and track down issues. Here are some common features:
- Inspect HTML and CSS
- Debug JavaScript
- Monitor network requests
Using Linters and Code Formatters
Linters and code formatters help keep your code clean and error-free. They automatically check your code for mistakes and enforce coding standards. This makes it easier to read and maintain. Testing is composed of the validation and verification of software, while debugging seeks to match symptoms with cause, leading to error correction.
Regular testing and debugging can save you time and effort in the long run. It helps catch issues early before they become bigger problems.
Conclusion
Mastering testing and debugging techniques is essential for any frontend developer. By using the right tools and practices, you can create more reliable and user-friendly applications.
Keeping Up with Frontend Trends and Resources
Frontend development is always changing, so it’s important to stay updated with the latest trends and resources. Here are some key areas to focus on:
Online Communities and Forums
- Join active communities like Stack Overflow, Reddit, or dev.to. These platforms allow developers to ask questions, share knowledge, and engage in discussions.
Blogs and Newsletters
- Follow popular blogs and newsletters that provide insights and tutorials. Some notable mentions include CSS-Tricks, Smashing Magazine, and A List Apart. These resources keep you informed about the latest trends and technologies.
Online Courses and Tutorials
- Consider platforms like Udemy, Coursera, or freeCodeCamp for comprehensive courses. These can help you enhance your skills and knowledge in frontend development.
Conferences and Events
- Attend frontend development conferences and events, both in-person and virtual. These gatherings offer opportunities to network with industry experts, attend workshops, and learn about emerging trends.
Staying updated is essential for growth in frontend development. Engaging with the community and utilizing available resources can significantly enhance your skills.
Resource Type | Examples |
---|---|
Online Communities | Stack Overflow, Reddit, dev.to |
Blogs and Newsletters | CSS-Tricks, Smashing Magazine |
Online Courses | Udemy, Coursera, freeCodeCamp |
Conferences and Events | Various local and international events |
Collaboration and Workflow Efficiency
Utilizing Version Control Systems
Using version control systems like Git is essential for teamwork in frontend development. It allows multiple developers to work on the same project without overwriting each other’s changes. Version control helps track changes, making it easier to revert to previous versions if needed.
Adopting Agile Methodologies
Agile methodologies promote flexibility and collaboration. Teams can adapt to changes quickly, ensuring that the project meets user needs. Regular meetings and feedback loops help keep everyone on the same page.
Effective Communication Channels
Clear communication is key to successful collaboration. Tools like Slack or Microsoft Teams can facilitate discussions and updates. Establishing regular check-ins can also help address issues promptly.
Project Management Tools
Using project management tools like Trello or Asana can streamline tasks and responsibilities. These tools help teams visualize their progress and prioritize work effectively. Here’s a simple table to illustrate some popular tools:
Tool | Purpose |
---|---|
Trello | Task management |
Asana | Project tracking |
Slack | Team communication |
GitHub | Version control |
Collaboration is not just about working together; it’s about creating a shared vision and achieving goals as a team.
Conclusion
By utilizing these strategies, frontend developers can enhance their collaboration and workflow efficiency, leading to better project outcomes.
Working together can really boost how well we get things done. If you want to learn more about improving your teamwork and workflow, check out our website for helpful tips and resources!
Conclusion
In conclusion, diving into front-end development is an exciting journey filled with opportunities for creativity and growth. By learning the key concepts and tools outlined in this guide, you will be ready to take on real-world projects and make your mark in the web development field. Always remember to stay curious and keep learning. Embrace the challenges that come your way, as they will help you grow and improve as a front-end developer. Your adventure in coding is just beginning, and with dedication, you can achieve great things!
Frequently Asked Questions
What is frontend development?
Frontend development is about creating the part of websites that users see and interact with. It uses languages like HTML, CSS, and JavaScript.
Do I need to know coding to start learning frontend development?
Yes, basic coding skills are important. Start with HTML and CSS, as they are the foundation of web design.
What tools do I need for frontend development?
You will need a code editor like Visual Studio Code, a web browser for testing, and tools for version control like Git.
How long does it take to learn frontend development?
It usually takes a few months to learn the basics, but mastering it can take longer depending on your practice and dedication.
What are some good resources for learning frontend development?
You can find online courses on platforms like Codecademy, freeCodeCamp, and Udemy. Also, look for tutorials on YouTube.
What is responsive design?
Responsive design ensures that websites look good on all devices, from phones to desktops. It adapts the layout based on the screen size.
What are some common frontend frameworks?
Popular frameworks include React, Angular, and Vue.js. They help developers build websites more efficiently.
How can I practice my frontend development skills?
You can practice by working on personal projects, contributing to open source, or taking part in coding challenges.