Mastering Data Structures and Algorithms: A Comprehensive Guide to Efficient Programming
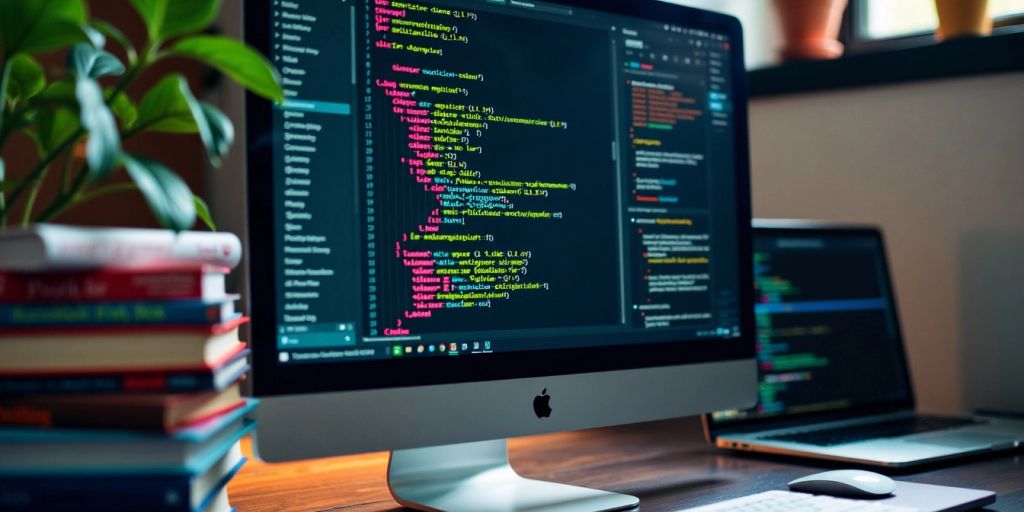
Data structures and algorithms are the backbone of programming, playing a crucial role in how we organize and manipulate data. Understanding these concepts is essential for anyone looking to enhance their coding skills, especially for those preparing for coding interviews. This guide will help you grasp the basics and explore advanced techniques to become a more efficient programmer.
Key Takeaways
- Data structures help organize data for easy access and modification.
- Algorithms are step-by-step instructions to solve problems efficiently.
- Understanding time and space complexity is vital for optimizing code performance.
- Practicing coding problems regularly builds confidence and skill.
- Utilizing the right data structures and algorithms can significantly improve program efficiency.
Understanding the Basics of Data Structures
Definition and Importance
Data structures are the fundamental building blocks of computer programming. They define how data is organized, stored, and manipulated within a program. Understanding data structures is crucial for writing efficient code and solving problems effectively.
Types of Data Structures
There are several types of data structures, each with its own strengths and weaknesses. Here are some common ones:
- Arrays: A collection of elements stored in contiguous memory locations.
- Linked Lists: A series of nodes where each node points to the next.
- Stacks: A collection of elements that follows the Last In First Out (LIFO) principle.
- Queues: A collection that follows the First In First Out (FIFO) principle.
- Trees: A hierarchical structure that represents data in a parent-child relationship.
- Graphs: A collection of nodes connected by edges, useful for representing networks.
Basic Operations on Data Structures
Each data structure supports various operations. Here are some basic operations:
- Insertion: Adding a new element.
- Deletion: Removing an existing element.
- Traversal: Accessing each element in the structure.
Understanding these operations helps in choosing the right data structure for your needs.
By mastering these basics, you can build a strong foundation for more complex programming concepts.
Exploring Fundamental Algorithms
Definition and Importance
Algorithms are step-by-step instructions designed to solve specific problems or perform tasks. Understanding algorithms is crucial for efficient programming, as they help in processing data effectively. Here are some key points about algorithms:
- They can sort data.
- They can search for specific data.
- They can perform mathematical operations.
Types of Algorithms
There are various types of algorithms, each serving different purposes. Here are some common ones:
- Sorting Algorithms: These arrange data in a specific order, such as ascending or descending. Examples include:
- Bubble Sort
- Quick Sort
- Merge Sort
- Searching Algorithms: These help find specific elements in data structures. Notable examples are:
- Linear Search
- Binary Search
- Graph Algorithms: These are used to traverse and manipulate graph data structures.
Basic Operations of Algorithms
Understanding the basic operations of algorithms is essential for effective programming. Here are some common operations:
- Insertion: Adding new data.
- Deletion: Removing existing data.
- Traversal: Accessing each element in a data structure.
Mastering algorithms is a vital skill for any programmer, as it leads to better problem-solving and optimized code performance.
In summary, algorithms are fundamental to programming, and knowing how to use them effectively can greatly enhance your coding skills. By learning about different types of algorithms and their operations, you can tackle a wide range of programming challenges, including the top 10 algorithms and data structures for competitive programming.
Analyzing Time and Space Complexity
Introduction to Asymptotic Notation
Asymptotic notation helps us understand how an algorithm’s performance changes as the input size grows. Big O notation is the most common way to express this. It gives us an upper limit on the time or space an algorithm will need. Here are some key complexities:
Notation | Description |
---|---|
O(1) | Constant time |
O(n) | Linear time |
O(n^2) | Quadratic time |
O(log n) | Logarithmic time |
O(n log n) | Quasi-linear time |
Time Complexity Analysis
When analyzing time complexity, we look at how the execution time of an algorithm increases with the size of the input. Here are some common types of algorithms:
- Linear Search: Checks each element one by one. Time complexity is O(n).
- Binary Search: Efficiently finds an element in a sorted array by dividing the search space in half. Time complexity is O(log n).
- Depth First Search (DFS): Explores as far as possible along each branch before backtracking. Time complexity is O(V + E), where V is vertices and E is edges.
Space Complexity Analysis
Space complexity measures how much memory an algorithm uses relative to the input size. It’s important to consider both the space needed for the input and any additional space used during execution. Here are some points to remember:
- In-place algorithms: Use a small, constant amount of extra space (e.g., O(1)).
- Recursive algorithms: May use additional space for the call stack, which can lead to O(n) space complexity.
- Data structures: The choice of data structure can greatly affect space usage. For example, arrays use less space than linked lists for the same number of elements.
Understanding time and space complexity is crucial for writing efficient code. It helps you choose the right algorithm and data structure for your problem.
Sorting and Searching Techniques
Overview of Sorting Algorithms
Sorting algorithms are essential for organizing data. A sorting algorithm is used to rearrange a given array or list of elements according to a comparison operator on the elements. Here are some common sorting algorithms:
- Bubble Sort: This simple algorithm repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. It continues until the list is sorted. Its time complexity is O(n²).
- Selection Sort: This algorithm finds the minimum element from the unsorted part and places it at the beginning. It also has a time complexity of O(n²).
- Insertion Sort: This method builds the final sorted array one item at a time. It is less efficient on large lists compared to more advanced algorithms like quicksort or mergesort, with a time complexity of O(n²).
- Merge Sort: An efficient, stable sorting algorithm that divides the input array into two halves, sorts them, and then merges them back together. Its time complexity is O(n log n).
- Quick Sort: A highly efficient algorithm that partitions an array into smaller arrays based on a pivot value. Its average-case time complexity is O(n log n), but it can degrade to O(n²) in the worst case.
Overview of Searching Algorithms
Searching algorithms help find specific data within a structure. Here are two common types:
- Linear Search: This method checks each element one by one until the target value is found. Its time complexity is O(n).
- Binary Search: This efficient algorithm works on sorted arrays by repeatedly dividing the search interval in half. Its time complexity is O(log n).
Comparative Analysis of Sorting and Searching
Algorithm Type | Example | Time Complexity |
---|---|---|
Sorting | Quick Sort | O(n log n) |
Searching | Binary Search | O(log n) |
Linear Search | O(n) |
Understanding sorting and searching algorithms is crucial for efficient programming. They help in organizing data and retrieving it quickly, which is vital in many applications.
Mastering Graphs and Trees
Introduction to Graph Theory
Graphs are a way to represent connections between different items. They consist of nodes (also called vertices) and edges that connect these nodes. Understanding graphs is essential because they are used in many real-world applications, such as social networks and transportation systems. Here are some key points about graphs:
- Directed vs. Undirected: In directed graphs, edges have a direction, while in undirected graphs, they do not.
- Weighted vs. Unweighted: Weighted graphs have edges with values (weights), while unweighted graphs do not.
- Applications: Graphs can model relationships, networks, and pathways.
Graph Traversal Algorithms
To work with graphs, we often need to explore them. Two common methods for traversing graphs are:
- Breadth-First Search (BFS): This method explores all neighbors at the present depth before moving on to nodes at the next depth level.
- Depth-First Search (DFS): This method explores as far as possible along each branch before backtracking.
Both algorithms have their own strengths and weaknesses, and choosing the right one depends on the problem at hand.
Tree Data Structures and Traversal
Trees are a special type of graph that has a hierarchical structure. They consist of nodes connected by edges, with one node designated as the root. Here are some important aspects of trees:
- Binary Trees: Each node has at most two children, known as the left and right child.
- Tree Traversal: Common methods include:
- In-order: Visit the left child, then the node, and finally the right child.
- Pre-order: Visit the node first, then the left child, and finally the right child.
- Post-order: Visit the left child, then the right child, and finally the node.
Understanding trees is crucial for coding interviews. A tree cheatsheet for coding interviews can help you prepare effectively, including practice questions, techniques, time complexity, and recommended resources.
By mastering graphs and trees, you will enhance your problem-solving skills and be better prepared for challenges in programming and coding interviews.
Dynamic Programming and Greedy Algorithms
Introduction to Dynamic Programming
Dynamic programming is a method used to solve complex problems by breaking them down into simpler sub-problems. It is especially useful when the same sub-problems are solved multiple times. This technique helps save time and resources. Here are two common approaches:
- Memoization: This technique stores the results of expensive function calls and returns the cached result when the same inputs occur again.
- Tabulation: This approach builds a table in a bottom-up manner, solving all related sub-problems first and using their solutions to construct a solution to the larger problem.
Common Dynamic Programming Algorithms
Some well-known dynamic programming algorithms include:
- Fibonacci series: A classic example where each number is the sum of the two preceding ones.
- Longest common subsequence: This finds the longest sequence that can appear in the same order in both strings.
- Knapsack problem: This problem involves selecting items with given weights and values to maximize value without exceeding weight capacity.
Introduction to Greedy Algorithms
Greedy algorithms make the best choice at each step, hoping to find the global optimum. They are often easier to implement and faster than dynamic programming solutions. Here’s how they work:
- Start with the initial state of the problem.
- Evaluate all possible choices you can make from the current state.
- Choose the option that seems the best at that moment.
Common Greedy Algorithms
Some popular greedy algorithms include:
- Activity selection problem: This selects the maximum number of activities that don’t overlap.
- Huffman coding: This is used for data compression by assigning variable-length codes to input characters.
- Prim’s and Kruskal’s algorithms: These are used for finding the minimum spanning tree in a graph.
Greedy algorithms are not always optimal, but they can be very efficient for certain problems. Understanding when to use them is key to mastering algorithms.
Advanced Algorithmic Techniques
Divide and Conquer Algorithms
Divide and conquer is a powerful strategy used in algorithms. It involves breaking a problem into smaller parts, solving each part, and then combining the results. This method is effective for complex problems. Here are some common examples:
- Merge Sort: A sorting algorithm that divides the array into halves, sorts them, and merges them back together.
- Quick Sort: Another sorting method that selects a pivot and partitions the array around it.
- Binary Search: A searching algorithm that divides the search interval in half repeatedly.
Backtracking Algorithms
Backtracking is a technique for solving problems incrementally. It tries to build a solution step by step and removes those solutions that fail to satisfy the conditions. Here are some key points:
- N-Queens Problem: Placing N queens on a chessboard so that no two queens threaten each other.
- Sudoku Solver: Filling a Sudoku grid while following the game rules.
- Subset Sum Problem: Finding a subset of numbers that add up to a specific target.
Other Advanced Algorithms
There are many other advanced algorithms that can be useful in various scenarios. Here’s a brief overview:
- Dijkstra’s Algorithm: Used for finding the shortest path in a graph.
- A Search Algorithm*: Combines features of Dijkstra’s and greedy algorithms for pathfinding.
- Floyd-Warshall Algorithm: A method for finding shortest paths in a weighted graph with positive or negative edge weights.
Mastering these advanced techniques can significantly enhance your programming skills and problem-solving abilities. Understanding when and how to apply these algorithms is crucial for efficient programming.
In summary, advanced algorithmic techniques like divide and conquer, backtracking, and others are essential for tackling complex problems. By learning and practicing these methods, you can improve your coding efficiency and effectiveness.
Practical Applications of Data Structures and Algorithms
Real-World Use Cases
Data structures and algorithms are not just theoretical concepts; they have real-life applications that impact our daily lives. Here are some key areas where they are used:
- Spam Email Detection: Algorithms help identify and filter out unwanted emails.
- Plagiarism Detection: Data structures are used to compare documents for similarities.
- Search Engines: Efficient algorithms enable quick retrieval of information from vast databases.
Industry Applications
Different industries leverage data structures and algorithms to enhance their operations. Some examples include:
- Finance: Algorithms are used for stock trading and risk assessment.
- Healthcare: Data structures help manage patient records and medical data.
- E-commerce: Algorithms optimize product recommendations and inventory management.
Case Studies
To illustrate the impact of data structures and algorithms, consider the following case studies:
Application | Data Structure Used | Algorithm Used |
---|---|---|
Online Shopping | Hash Tables | Recommendation Systems |
Social Media Platforms | Graphs | Friend Suggestion |
Navigation Systems | Trees | Pathfinding Algorithms |
Understanding how data structures and algorithms work in real-world scenarios can greatly enhance your programming skills and problem-solving abilities.
By mastering these concepts, you can create more efficient and effective software solutions.
Tips for Mastering Data Structures and Algorithms
Mastering data structures and algorithms is essential for becoming a skilled programmer. Here are some helpful tips to guide you:
Starting with the Basics
- Begin with simple structures: Start with basic data structures like arrays and linked lists.
- Understand core concepts: Make sure you grasp the fundamental ideas before moving on to complex topics.
- Use visual aids: Diagrams can help you understand how data structures work.
Practicing Regularly
- Set a schedule: Dedicate specific times each week to practice coding.
- Use online platforms: Websites like LeetCode and HackerRank offer many problems to solve.
- Join coding groups: Collaborate with peers to learn from each other.
Analyzing and Optimizing Code
- Check your code’s efficiency: Look at the time and space complexity of your solutions.
- Refactor when needed: Don’t hesitate to improve your code for better performance.
- Learn from mistakes: Review errors to avoid repeating them in the future.
Mastering data structures and algorithms requires practice, patience, and persistence. Keep pushing yourself to learn and improve!
Resources for Further Learning
Online Courses and Tutorials
There are many great online courses available to help you learn data structures and algorithms. Here are some popular options:
- Coursera’s Data Structures and Algorithms Specialization
- edX’s Data Structures and Algorithms course
- 30DayCoding offers a variety of data structures and algorithms online courses to learn and practice.
Recommended Books
Books can provide in-depth knowledge and examples. Here are some recommended titles:
- "Introduction to Algorithms" by Thomas H. Cormen
- "Data Structures and Algorithms in Python" by Michael T. Goodrich et al.
- "Cracking the Coding Interview" by Gayle Laakmann McDowell
Practice Platforms
Practicing coding problems is essential for mastering these concepts. Here are some platforms to consider:
- LeetCode
- HackerRank
- CodeForces
Regular practice on these platforms can significantly improve your coding skills and prepare you for interviews.
By utilizing these resources, you can enhance your understanding and application of data structures and algorithms effectively.
If you’re eager to dive deeper into coding, check out our website for more resources! We offer a variety of interactive tutorials and helpful guides that can boost your skills and prepare you for coding interviews. Don’t wait—start your journey today!
Conclusion
In summary, getting a good grasp of data structures and algorithms is key to becoming a skilled programmer. This guide has shown you important ideas and methods that will help you write code that is not only efficient but also easy to maintain. Keep in mind that practice is essential. Regularly work on coding challenges, study your own code, and learn from others to improve your skills in data structures and algorithms. With time and effort, you’ll be able to tackle complex programming tasks with confidence.
Frequently Asked Questions
What are data structures?
Data structures are ways to organize and store data on a computer so that it can be easily accessed and changed.
Why are data structures important?
They help programmers write efficient code that runs faster and uses less memory.
What is an algorithm?
An algorithm is a set of steps to solve a problem or complete a task.
Why should I learn algorithms?
Learning algorithms helps you think logically and solve problems more effectively.
What is time complexity?
Time complexity measures how the time to complete an algorithm grows with the size of the input.
What is space complexity?
Space complexity measures how much memory an algorithm uses based on the size of the input.
Can you give an example of a data structure?
Sure! An array is a simple data structure that holds a list of items.
How can I practice data structures and algorithms?
You can practice on websites like LeetCode, HackerRank, and CodeForces, which have many coding challenges.