Mastering Conditional Operations in Python: A Comprehensive Guide to np.where
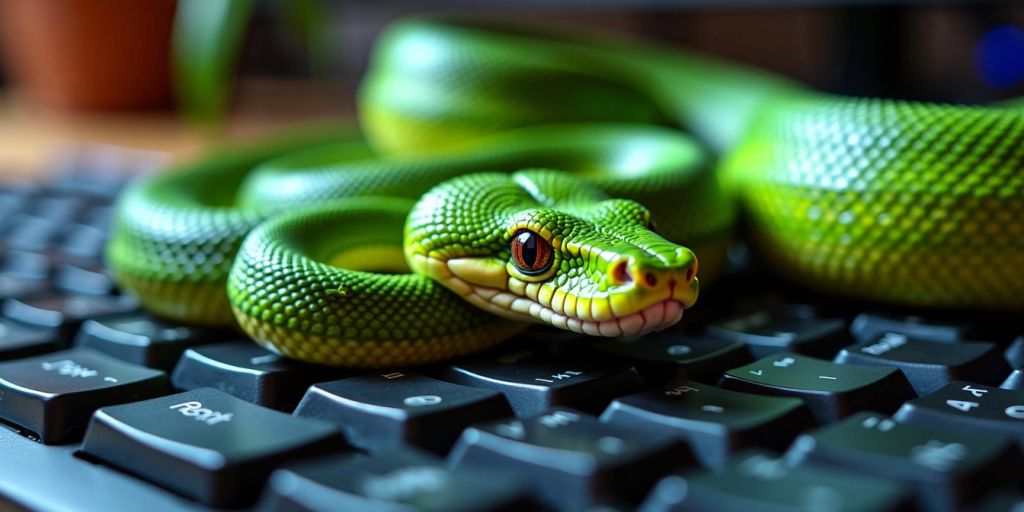
In this guide, we will explore the powerful function `np.where` from the NumPy library. This function allows you to perform conditional operations on arrays, making it easier to manipulate and analyze data. Whether you’re replacing values based on certain conditions or working with multi-dimensional arrays, `np.where` is a versatile tool that can help streamline your data processing tasks. By the end of this article, you’ll have a solid understanding of how to use `np.where` effectively in your Python projects.
Key Takeaways
- `np.where` is great for selecting and changing values in arrays based on conditions.
- You can use `np.where` with multi-dimensional arrays to handle complex data.
- Combining `np.where` with other NumPy functions expands its capabilities.
- Using `np.where` can help clean data by handling missing values and replacing unwanted entries.
- Understanding the syntax and practice with examples is key to mastering `np.where`.
Understanding the Basics of np.where
The np.where
function is a key tool in the NumPy library that allows you to perform conditional operations on arrays. It helps you select or change elements based on specific conditions. The basic syntax is:
import numpy as np
result = np.where(condition, x, y)
In this syntax:
- condition: A boolean array or a condition that results in a boolean array.
- x: The value to use where the condition is True.
- y: The value to use where the condition is False.
Syntax and Parameters
Here’s a breakdown of the parameters:
Parameter | Description |
---|---|
condition | A boolean array or condition |
x | Value to use when condition is True |
y | Value to use when condition is False |
Simple Examples
Let’s look at a simple example:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
result = np.where(arr > 3, "greater than 3", "not greater")
print(result)
In this example, we create an array and use np.where
to replace values greater than 3 with the string "greater than 3" and the rest with "not greater". The output will show the results based on the condition.
Common Use Cases
Here are some common scenarios where np.where
is useful:
- Replacing values based on conditions.
- Filtering data in arrays.
- Creating new arrays based on existing data.
Using np.where can greatly simplify your code when dealing with conditions in arrays. It allows for cleaner and more efficient data manipulation.
By understanding these basics, you can start leveraging np.where
in your own Python projects effectively!
Advanced Applications of np.where
Using np.where with Broadcasting
Broadcasting is a powerful feature in NumPy that allows you to perform operations on arrays of different shapes. With np.where
, you can apply conditions across these arrays. For example:
import numpy as np
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
row_threshold = np.array([2, 5, 8])
result = np.where(matrix > row_threshold[:, np.newaxis], "numpyarray.com", matrix)
print(result)
In this code, each row of the matrix is compared against a different threshold value, showcasing how broadcasting works with np.where
.
Chaining Multiple np.where Calls
You can also chain multiple np.where
calls to handle complex conditions, similar to nested if-else statements. Here’s a simple example:
import numpy as np
arr = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
result = np.where(arr < 4, "numpyarray.com_low",
np.where((arr >= 4) & (arr < 7), "numpyarray.com_medium",
np.where(arr >= 7, "numpyarray.com_high", "numpyarray.com_unknown")))
print(result)
This example categorizes the elements based on their values, demonstrating the flexibility of np.where
.
Complex Conditions
When dealing with more intricate scenarios, np.where
can handle complex conditions effectively. For instance, you can use it to manage NaN values in an array:
import numpy as np
arr = np.array([1, 2, np.nan, 4, 5])
result = np.where(np.isnan(arr), "numpyarray.com", arr)
print(result)
This code replaces NaN values with a specified string, showing how np.where
can be used for data cleaning tasks.
Remember, mastering np.where can greatly enhance your data manipulation skills. Experiment with different conditions and array types to see its full potential!
Leveraging np.where for Data Cleaning
Data cleaning is an essential part of data analysis, and np.where() can be a powerful tool in this process. Here’s how you can use it effectively:
Handling Missing Data
- Use
np.where()
to identify and replace missing values in your dataset. - For example, if you have a dataset where
-999
indicates missing data, you can replace it withnp.nan
:import numpy as np data = np.array([1, 2, -999, 4, 5, -999, 7]) cleaned_data = np.where(data == -999, np.nan, data) print(cleaned_data)
- This will help in standardizing how missing data is represented.
Replacing Values
- You can also use
np.where()
to replace specific values based on conditions. For instance:arr = np.array([1, 2, 3, 4, 5]) result = np.where(arr > 3, "greater than 3", "not greater") print(result)
- This allows for quick adjustments to your data based on defined criteria.
Conditional Data Cleaning
- Combine
np.where()
with other NumPy functions for more complex data cleaning tasks. For example:data = np.array([1, 2, 3, 4, 5, np.nan]) mean_value = np.nanmean(data) imputed_data = np.where(np.isnan(data), mean_value, data) print(imputed_data)
- This replaces
NaN
values with the mean of the dataset, ensuring your data is complete and ready for analysis.
Using np.where() effectively can significantly enhance your data cleaning process, making it more efficient and reliable.
By leveraging these techniques, you can ensure your data is clean and ready for further analysis, which is crucial for accurate results in any data-driven project.
Practical Examples of Data Cleaning Using Pandas and NumPy
- In this article, we’ll explore practical examples of data cleaning using Python’s popular libraries, Pandas and NumPy, with a focus on the provided Olympics dataset.
Using np.where with Multi-Dimensional Arrays
2D Arrays
The np.where() function can be applied to 2D arrays effortlessly. For example, consider a matrix where we want to replace even numbers with a specific string while keeping odd numbers unchanged:
import numpy as np
matrix = np.array([
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
])
result = np.where(matrix % 2 == 0, "even", matrix)
print(result)
This will output:
[[1 'even' 3]
['even' 5 'even']
[7 'even' 9]]
3D Arrays
When working with 3D arrays, np.where() can also be utilized. Here’s a simple example:
import numpy as np
a_3d_array = np.array([[[1, 3], [1, -3]],
[[5, -1], [3, 1]]
])
result = np.where(a_3d_array < 0, "negative", a_3d_array)
print(result)
This will replace negative values with the string "negative" while keeping the rest of the values intact.
Higher-Dimensional Arrays
For higher-dimensional arrays, np.where() continues to function effectively. Here are some key points to remember:
- Broadcasting: np.where() works across all dimensions, applying the condition to each element.
- Performance: Be cautious of performance when dealing with large arrays.
- Type Consistency: Ensure that the types of values being replaced are consistent to avoid unexpected results.
Using np.where() with multi-dimensional arrays allows for flexible data manipulation, making it a powerful tool in data analysis.
By mastering these techniques, you can handle complex data structures with ease!
Applying np.where in Image Processing
Image processing is a fascinating area where numpy shines, especially with the np.where
function. This function allows you to manipulate images easily by applying conditions to pixel values. Here are some key applications:
Thresholding Images
Thresholding is a common technique used to convert grayscale images into binary images. For example, you can set a threshold value, and all pixels above this value will be turned white (255), while those below will be turned black (0). Here’s a simple code snippet:
import numpy as np
# Simulating a grayscale image as a 2D array
image = np.random.randint(0, 256, size=(5, 5))
threshold = 128
binary_image = np.where(image > threshold, 255, 0)
print("Original Image:")
print(image)
print("\nBinary Image:")
print(binary_image)
Conditional Pixel Manipulation
You can also use np.where
to change pixel values based on certain conditions. For instance:
- Change all pixels below a certain brightness to a specific color.
- Adjust the brightness of pixels based on their current value.
- Apply filters conditionally to enhance image quality.
Combining with Other Image Processing Techniques
np.where
can be combined with other techniques for more complex operations:
- Edge Detection: Use
np.where
to highlight edges in an image. - Color Filtering: Isolate specific colors in an image based on RGB values.
- Image Blending: Blend two images based on conditions applied to pixel values.
Using np.where in image processing not only simplifies tasks but also enhances performance, making it a powerful tool for anyone looking to explore the image processing using numpy.
By mastering these techniques, you can unlock the full potential of image manipulation with numpy!
Optimizing Performance with np.where
When using np.where, it’s important to consider how to make it work faster and more efficiently. Here are some key points to keep in mind:
Memory Usage Considerations
- Memory Impact: Using np.where creates a new array for the results, which can be heavy on memory, especially with large datasets.
- Avoid Copies: Try to minimize unnecessary copies of data to save memory.
- Data Type Consistency: Keeping data types consistent can help improve performance.
Performance Testing
- Benchmarking: Always test your code to see if np.where is the best option for your needs.
- Use Vectorization: np.where is faster than using loops for element-wise operations.
- Simplify Conditions: Simple conditions run faster than complex ones.
Best Practices
- Readability: Write clear and understandable code. Break complex conditions into simpler parts.
- Error Handling: Be cautious with operations that might fail, like division by zero.
- Combine Functions: Use np.where with other NumPy functions for better results.
Remember, optimizing performance is key when working with large datasets. Optimizing pandas performance on large datasets can save you a lot of time and resources!
Combining np.where with Other NumPy Functions
Using np.where with np.select
The np.select
function allows you to choose from multiple conditions. Here’s how you can use it with np.where
:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
conditions = [arr < 3, arr > 3]
choices = ["low", "high"]
result = np.select(conditions, choices, default="medium")
print(result)
This will categorize the values in arr
as "low", "high", or "medium" based on the conditions.
Using np.where with np.choose
The np.choose
function can also be combined with np.where
. It allows you to select elements from a list of arrays based on indices:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
choices = [arr, arr**2, arr**3]
result = np.choose(np.where(arr > 3, 1, 0), choices)
print(result)
In this example, it will choose between the original array and its square based on the condition.
Using np.where with np.piecewise
The np.piecewise
function is useful for applying different functions based on conditions. Here’s an example:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
result = np.piecewise(arr, [arr < 3, arr >= 3], [lambda x: x**2, lambda x: x**3])
print(result)
This will square values less than 3 and cube values 3 or greater.
Combining np.where with other NumPy functions can greatly enhance your data manipulation capabilities.
Summary
np.select
: Choose from multiple conditions.np.choose
: Select from a list of arrays based on indices.np.piecewise
: Apply different functions based on conditions.
By mastering these combinations, you can perform more complex operations efficiently!
Using np.where for Conditional Aggregation
Summing Based on Conditions
You can use np.where to sum values based on specific conditions. Here’s a simple example:
import numpy as np
data = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
condition = data % 2 == 0
# Sum of even numbers
sum_even = np.sum(np.where(condition, data, 0))
# Sum of odd numbers
sum_odd = np.sum(np.where(~condition, data, 0))
print(f"Even sum: {sum_even}, Odd sum: {sum_odd}")
In this example, we create a condition to identify even numbers and use np.where to sum them separately from odd numbers.
Calculating Averages
You can also calculate averages conditionally. For instance:
weights = np.where(data % 2 == 0, 2, 1)
weighted_mean = np.average(data, weights=weights)
print(f"Weighted Mean: {weighted_mean}")
This code assigns different weights to even and odd numbers, allowing for a weighted average calculation.
Other Aggregation Functions
Here are some other aggregation functions you can use with np.where:
- np.min: Find the minimum value based on a condition.
- np.max: Find the maximum value based on a condition.
- np.median: Calculate the median of selected values.
Using np.where for conditional aggregation can greatly simplify your data analysis tasks. It allows you to efficiently filter and compute statistics based on specific criteria, making your code cleaner and more effective.
By mastering these techniques, you can leverage np.where to perform powerful data manipulations and analyses in Python.
Working with Custom Data Types in np.where
Structured Arrays
When using np.where()
, you can work with custom data types. This allows you to create more complex data structures in your arrays. For example, you can define a structured array with names and ages:
import numpy as np
dt = np.dtype([('name', 'U10'), ('age', int)])
people = np.array([('Alice', 25), ('Bob', 30), ('Charlie', 35), ('David', 40)], dtype=dt)
result = np.where(people['age'] > 30,
people['name'] + '@numpyarray.com',
people['name'])
print(result)
In this example, we create a structured array with names and ages. We then use np.where()
to append ‘@numpyarray.com’ to the names of people over 30. This demonstrates how np.where()
can be used with custom data types for more complex data manipulations.
Custom Functions
You can also use np.where()
with custom functions. Here’s a simple example:
import numpy as np
def custom_operation(x):
return f"numpyarray.com_{x**2}"
arr = np.array([1, 2, 3, 4, 5])
result = np.where(arr % 2 == 0, custom_operation(arr), arr)
print(result)
In this case, we define a custom function that squares a number and prepends “numpyarray.com_” to it. We then apply this function to even numbers in the array, while leaving odd numbers unchanged.
Masked Arrays
np.where()
can also be effectively used with masked arrays. Here’s how:
import numpy as np
data = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
mask = np.array([True, False, True, False, True, False, True, False, True, False])
masked_array = np.ma.masked_array(data, mask)
result = np.where(masked_array.mask, "numpyarray.com", masked_array)
print(result)
In this example, we create a masked array and use np.where()
to replace masked values with a string. This shows how np.where()
can be used for selective array manipulation based on conditions.
Using np.where() with custom data types opens up many possibilities for data manipulation.
Exploring np.where for Data Binning
Data binning is a technique used to group a set of data points into bins or intervals. This can help in simplifying data analysis and visualization. Using np.where
can make this process easier and more efficient.
Creating Bins
To create bins using np.where
, follow these steps:
- Define your data: Start with a NumPy array containing the data you want to bin.
- Set your bin edges: Create a list of bin edges that define the intervals.
- Use
np.digitize
: This function will categorize your data into the defined bins. - Apply
np.where
: Use this function to label each bin appropriately.
Labeling Bins
Here’s a simple example:
import numpy as np
data = np.array([15, 25, 35, 45, 55, 65, 75, 85, 95])
bins = [0, 30, 60, 90, 120]
binned_data = np.digitize(data, bins)
result = np.where(binned_data == 1, "Low",
np.where(binned_data == 2, "Medium",
np.where(binned_data == 3, "High", "Very High")))
print(result)
This code will categorize the data into four bins: Low, Medium, High, and Very High.
Advanced Binning Techniques
For more complex binning, consider the following:
- Custom bin sizes: Adjust the size of your bins based on data distribution.
- Dynamic binning: Use statistical methods to determine bin edges based on data characteristics.
- Error bars: You can also get the error bars to bins of a dataset by using bootstrapping methods, which can help in understanding the variability within each bin.
Binning can significantly enhance data analysis by reducing noise and highlighting trends. It’s a powerful tool in data preprocessing.
By mastering np.where
for data binning, you can streamline your data analysis process and make your results clearer and more interpretable.
Best Practices for Using np.where
Readability and Maintainability
When using np.where, clarity is key. Here are some tips to keep your code easy to read:
- Break complex conditions into smaller parts.
- Use descriptive variable names.
- Comment on tricky logic to help others (or yourself) understand later.
Error Handling
np.where doesn’t raise errors for invalid operations. To manage this:
- Use
np.errstate()
to control warnings. - Check your conditions to avoid unexpected results.
- Test your code with edge cases to ensure it behaves as expected.
Type Checking
Be mindful of the data types in your arrays. Mixing types can lead to unexpected results. Here are some practices:
- Ensure input arrays have consistent types.
- Use
astype()
to convert types when necessary. - Validate your output to confirm it matches your expectations.
Remember, the key to mastering np.where is practice. Experiment with different conditions and array types to see how it works in various scenarios!
When using np.where
, it’s important to follow some key tips to make your code cleaner and more efficient. First, always ensure your conditions are clear and easy to understand. This will help you avoid confusion later on. Also, try to use vectorized operations instead of loops whenever possible, as they can speed up your code significantly. For more tips and to start your coding journey, visit our website today!
Conclusion
In summary, mastering numpy.where() is essential for anyone working with data in Python. This function is not just powerful; it’s also flexible, allowing you to handle many tasks with ease. Whether you need to change values based on certain conditions or perform complex calculations, numpy.where() can help you do it efficiently. Throughout this guide, we’ve looked at how to use numpy.where() for simple tasks and more advanced operations. By practicing and applying what you’ve learned, you’ll find that numpy.where() becomes a key tool in your data analysis toolkit. Keep experimenting, and you’ll discover even more ways to use this function in your projects.
Frequently Asked Questions
What is np.where in Python?
np.where is a function in Python’s NumPy library that helps you select and change elements in arrays based on certain conditions.
How do I use np.where?
You can use np.where by providing a condition, a value for when that condition is true, and another value for when it’s false.
Can np.where handle multiple conditions?
Yes, you can use np.where with multiple conditions by chaining it or using logical operators.
Is np.where only for one-dimensional arrays?
No, np.where works with multi-dimensional arrays too, making it very flexible.
How does np.where help in data cleaning?
np.where can replace missing or unwanted values in your data, making it great for cleaning datasets.
What are some common use cases for np.where?
Common uses include replacing values, filtering data, and performing conditional calculations.
Does using np.where affect performance?
Using np.where can be memory-intensive for large arrays, so it’s good to test its performance with your data.
Can I combine np.where with other NumPy functions?
Absolutely! np.where works well with other NumPy functions to create more complex operations.