Mastering Clean Code: Essential Techniques for Agile Software Development
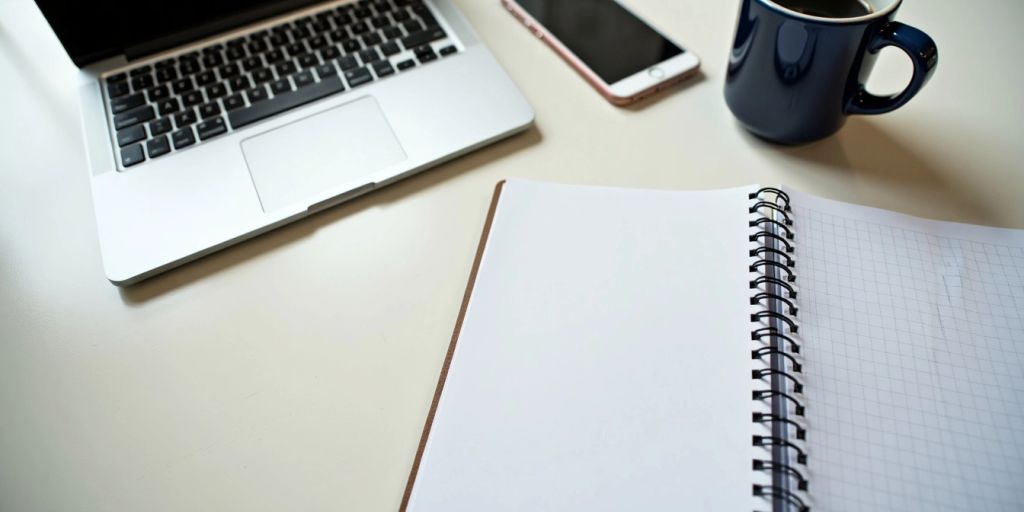
In the fast-paced world of software development, writing clean code is more important than ever. Clean code not only makes it easier for developers to read and understand each other’s work, but it also helps teams work together more effectively. This article explores essential techniques for mastering clean code, especially in Agile environments, where flexibility and collaboration are key. Let’s dive into some of the most important takeaways.
Key Takeaways
- Clean code boosts readability, making it easier for anyone to understand and work with.
- Reducing technical debt is crucial; tidy code prevents future problems and saves time.
- Good naming and organization in code help everyone on the team collaborate better.
- Regular code reviews and refactoring keep the code clean and up-to-date.
- Effective commenting practices clarify the intent behind code, helping future developers.
The Importance of Clean Code in Agile Development
In Agile development, clean code is crucial for maintaining flexibility and responsiveness. It helps teams adapt quickly to changing requirements and enhances overall productivity. Here are some key reasons why clean code matters:
Enhancing Code Readability
- Clean code is easier to read and understand.
- It allows new team members to get up to speed faster.
- Well-structured code reduces confusion and errors.
Reducing Technical Debt
- Clean code minimizes the buildup of technical debt, which can slow down development.
- It prevents the need for costly rewrites in the future.
- Teams can focus on delivering features rather than fixing messy code.
Improving Team Collaboration
- Clean code fosters better communication among team members.
- It allows developers to work on each other’s code without extensive explanations.
- Teams can collaborate more effectively, leading to higher quality software.
Clean code is not just about writing code that works; it’s about writing code that is a joy to work with. By embracing clean coding practices, teams can deliver higher-quality software products and maintain a sustainable pace of development.
In summary, prioritizing clean code in Agile development leads to a more efficient, adaptable, and collaborative environment, ultimately benefiting both the team and the end-users.
Principles of Writing Clean Code
Writing clean code is essential for maintaining a healthy codebase. Here are some key principles to follow:
The Boy Scout Rule
- Always leave the code cleaner than you found it.
- Make small improvements whenever you touch a piece of code.
- This helps in gradually enhancing the overall quality of the codebase.
Meaningful Variable Names
- Use names that clearly describe the purpose of the variable.
- Avoid vague names like
temp
ordata
. - For example, instead of
x
, useuserAge
to indicate what the variable represents.
Proper Code Formatting
- Consistent formatting makes code easier to read.
- Use indentation and spacing to organize code logically.
- Follow a style guide to maintain uniformity across the codebase.
Clean code is not just about writing code that works; it’s about writing code that others can understand and maintain.
By adhering to these principles, developers can create a codebase that is easier to manage and less prone to errors. Clean code leads to better collaboration and reduces the chances of introducing bugs.
Common Code Smells and How to Fix Them
Duplicated Code
Duplicated code is a common issue that can lead to maintenance headaches. When the same code appears in multiple places, any change requires updates in all locations, increasing the risk of errors. To fix this:
- Identify duplicated code blocks.
- Extract them into a single method or function.
- Call this method wherever needed.
Long Methods
Methods that are too long can be hard to understand. They often do too much, making it difficult to follow the logic. To improve this:
- Break long methods into smaller, focused methods.
- Each method should perform a single task.
- Use descriptive names to clarify their purpose.
Misplaced Responsibility
When a class or method takes on too many responsibilities, it can become confusing. This is often referred to as the God Object smell. To address this:
- Identify the responsibilities of your classes.
- Refactor to ensure each class has a single responsibility.
- Use design patterns like Dependency Injection to manage dependencies effectively.
By addressing these code smells early, you can make your code more maintainable and easier to understand. Remember, clean code is a team effort!
Effective Commenting Practices
When to Comment
Comments should be used only when necessary. If your code is clear and understandable, you might not need comments at all. Here are some situations where comments are helpful:
- To explain complex logic that isn’t obvious.
- To clarify the purpose of a method or class.
- To provide context for why a decision was made.
Writing Clear Comments
When you do write comments, make sure they are clear and concise. Here are some tips:
- Explain why something is done, not just what is done. This helps others understand your thought process.
- Avoid stating the obvious. For example, don’t write "This function adds two numbers" if the function name is already
addNumbers
. - Keep comments up to date. Outdated comments can confuse developers.
Avoiding Redundant Comments
It’s important to avoid comments that don’t add value. Here are some examples of comments to avoid:
- Comments that repeat what the code does.
- Commented-out code. Instead of leaving old code in comments, just remove it. Old code can clutter the codebase and confuse others.
- Comments that are too vague or general. Be specific about what you mean.
Comments should complement code: comments should provide additional context, not compensate for poor code. They should explain why something is done, not what is done.
By following these practices, you can ensure that your comments enhance the readability and maintainability of your code, making it easier for everyone to work together effectively.
Refactoring Techniques for Clean Code
Refactoring is a crucial part of maintaining clean code. It helps improve the structure and readability of your code without changing its behavior. Here are some effective techniques:
Extract Method
- Purpose: Break down large methods into smaller, more manageable ones.
- Benefits: Increases readability and makes testing easier.
- Example: If a method does multiple tasks, create separate methods for each task.
Rename Variable
- Purpose: Use clear and meaningful names for variables.
- Benefits: Helps others understand the code quickly.
- Example: Instead of using
x
, usetotalPrice
to indicate what the variable represents.
Simplify Conditionals
- Purpose: Make complex conditional statements easier to read.
- Benefits: Reduces confusion and potential errors.
- Example: Instead of
if (a == true)
, simply useif (a)
.
Refactoring is not just about fixing bugs; it’s about making your code easier to work with.
By applying these techniques, you can ensure that your code remains clean and maintainable over time. Remember, clean code is not just a goal; it’s a continuous journey!
Testing and Clean Code
In the world of software development, testing is crucial for maintaining clean code. It ensures that your code works as intended and helps catch bugs early in the process. Here are some key aspects to consider:
Writing Unit Tests
- Unit tests are small tests that check individual parts of your code. They help ensure that each piece functions correctly.
- Writing unit tests can boost code quality and make it easier to spot issues before they become bigger problems.
- Aim for at least 70% code coverage with your tests to ensure most of your code is being checked.
Test-Driven Development
- Test-Driven Development (TDD) is a practice where you write tests before writing the actual code. This approach helps clarify what your code should do.
- TDD can lead to cleaner, more organized code because it forces you to think about the design before implementation.
- Following TDD can help you catch bugs early and reduce the time spent on debugging later.
Maintaining Clean Tests
- Just like your code, your tests should also be clean and easy to understand. Here are some tips:
- Use meaningful names for your test cases to describe what they do.
- Keep your tests short and focused on one specific behavior.
- Regularly review and refactor your tests to keep them relevant and efficient.
Keeping your tests clean is just as important as keeping your code clean. It makes it easier for others to understand and maintain your work.
By following these practices, you can ensure that your testing process contributes positively to your clean code efforts. Remember, clean code is not just about writing code that works; it’s about writing code that is easy to read, maintain, and test effectively.
Error Handling and Clean Code
Using Exceptions Wisely
When it comes to handling errors, using exceptions is a smart choice. Exceptions help keep error handling separate from the main code, making it easier to read and understand. This way, you can focus on what the code is supposed to do without getting lost in error checks. Here’s a simple example:
- Use exceptions for unexpected issues.
- Keep regular code clean and focused.
- Avoid using return values for error states.
Failing Fast
Failing fast means that your code should stop running as soon as it encounters a problem. This approach helps catch errors early, making it easier to fix them. Here are some tips:
- Validate inputs right away.
- Throw exceptions for invalid states.
- Don’t let errors pile up; address them immediately.
Logging and Monitoring
Logging is essential for understanding what happens in your code. It helps you track down issues when they arise. Here are some best practices for logging:
- Log important events and errors.
- Use clear and descriptive messages.
- Monitor logs regularly to catch issues early.
Keeping your error handling clean is just as important as writing clean code. It helps everyone understand what’s going on and makes fixing problems easier.
By following these practices, you can ensure that your code remains clean and easy to maintain, even when things go wrong. Remember, using exceptions separates error handling from regular processing, resulting in cleaner, more readable code.
Code Reviews and Clean Code
Code reviews are a vital part of maintaining clean code in any software project. They help ensure that the code is not only functional but also easy to read and understand. Here are some key points to consider:
Best Practices for Code Reviews
- Create a standardized process for code reviews, including how reviews are requested, how feedback is provided, and how issues are tracked and resolved.
- Encourage team members to review each other’s code regularly to share knowledge and improve overall code quality.
- Use tools that facilitate code reviews, making it easier to comment and track changes.
Tools for Code Quality
Tool Name | Purpose | Benefits |
---|---|---|
GitHub | Code hosting and review | Easy collaboration and tracking |
SonarQube | Code quality analysis | Identifies code smells and bugs |
ESLint | JavaScript linting | Enforces coding standards |
Collaborative Code Improvement
- Foster a culture of open feedback where team members feel comfortable sharing their thoughts.
- Schedule regular review sessions to discuss code quality and improvements.
- Celebrate improvements and recognize team members who contribute to clean code practices.
Code reviews are not just about finding mistakes; they are an opportunity to learn and grow as a team.
By implementing these practices, teams can significantly enhance the quality of their code and ensure that it remains clean and maintainable over time. Effective code reviews lead to better collaboration and a stronger codebase.
Tools and Practices for Maintaining Clean Code
Maintaining clean code is essential for any software development team. Here are some effective tools and practices that can help:
Static Code Analysis
- Static code analysis tools automatically check your code for potential errors and enforce coding standards. They help catch issues early, making it easier to maintain clean code.
- Examples include:
- SonarQube
- ESLint
- Checkstyle
Automated Code Formatting
- Using automated code formatting tools ensures that your code follows a consistent style. This makes it easier to read and understand.
- Popular tools include:
- Prettier
- Black (for Python)
- ClangFormat
Continuous Integration
- Continuous integration (CI) tools help automate the process of testing and deploying code. They ensure that every change is tested, which helps maintain code quality.
- Some widely used CI tools are:
- Jenkins
- Travis CI
- CircleCI
Keeping your code clean is not just about writing it well; it’s about using the right tools to support your efforts.
By adopting these tools and practices, teams can significantly improve their code quality and reduce technical debt. Remember, clean code principles transform your programming and lead to better software development outcomes.
Design Patterns and Clean Code
Design patterns are essential tools in agile software development that help create clean and maintainable code. They provide proven solutions to common problems, making it easier for developers to communicate and collaborate effectively.
Single Responsibility Principle
- Each class should have one responsibility.
- This makes the code easier to understand and maintain.
- Changes in one part of the code will have minimal impact on others.
Open/Closed Principle
- Software entities should be open for extension but closed for modification.
- This allows developers to add new features without altering existing code, reducing the risk of introducing bugs.
- It promotes a more stable codebase.
Dependency Injection
- This design pattern helps in managing dependencies between classes.
- It makes the code more flexible and easier to test.
- By injecting dependencies, you can swap out implementations without changing the code that uses them.
Using design patterns not only improves code quality but also enhances team collaboration. When everyone understands the patterns being used, it leads to a more cohesive development process.
Incorporating these design patterns into your coding practices can significantly improve the principles of writing clean code. They help in structuring your code in a way that is both efficient and easy to manage, ultimately leading to better software development outcomes.
The Role of Documentation in Clean Code
Documentation is a key part of writing clean code. It helps everyone understand how the code works and why certain choices were made. Good documentation can save time and reduce confusion. Here are some important points to consider:
Creating Useful Documentation
- Explain the purpose of the code: Describe what the code does and why it exists.
- Include examples: Show how to use the code with simple examples.
- Use clear language: Avoid jargon and make it easy for anyone to understand.
Keeping Documentation Updated
- Review regularly: Make sure the documentation matches the current state of the code.
- Update with changes: Whenever you change the code, update the documentation too.
- Involve the team: Encourage team members to contribute to documentation updates.
Integrating Documentation with Code
- Comment wisely: Use comments to explain complex parts of the code.
- Link to documentation: Reference external documentation where necessary.
- Use tools: Consider using tools that generate documentation from the code itself.
Documentation is not just an afterthought; it is an essential part of clean code that helps maintain clarity and understanding.
In summary, effective documentation is crucial for maintaining clean code. It enhances collaboration, reduces errors, and ensures that everyone is on the same page. By following these practices, teams can create a more efficient and enjoyable coding environment.
Documentation plays a key role in writing clean code. It helps you and others understand what your code does, making it easier to maintain and improve. If you want to learn more about coding and how to write better code, visit our website and start coding for free today!
Conclusion
In conclusion, mastering clean code is essential for anyone involved in software development. By following the techniques discussed, you can create code that is not only functional but also easy to read and maintain. This approach helps reduce mistakes and makes it simpler for others to understand your work. Remember, clean code leads to better teamwork and less frustration in the long run. Whether you’re just starting out or have years of experience, adopting these practices will improve your coding skills and make your projects more successful.
Frequently Asked Questions
What is clean code?
Clean code means writing code that is easy to read and understand. It helps other programmers know what the code does without confusion.
Why is clean code important in Agile development?
Clean code is important in Agile development because it makes teamwork easier. When everyone understands the code, it leads to better collaboration and fewer mistakes.
What are some basic rules for writing clean code?
Some basic rules include using clear names for variables, keeping functions short, and organizing your code well.
What are code smells?
Code smells are signs that something might be wrong with your code. For example, if you see repeated code or very long functions, these are code smells.
How can I improve my code commenting?
You should comment on why you wrote the code, not just what it does. Avoid writing comments that repeat what the code already says.
What is refactoring?
Refactoring is changing your code to make it cleaner without changing what it does. This helps in keeping the code easy to manage.
How does testing relate to clean code?
Testing ensures that your code works as expected. Clean code is easier to test, which helps catch errors early.
What tools can help maintain clean code?
Tools like static code analyzers and automated formatting tools can help keep your code clean and organized.