Mastering Bootstrap Menus, Navbar, and Modals: A Comprehensive Guide
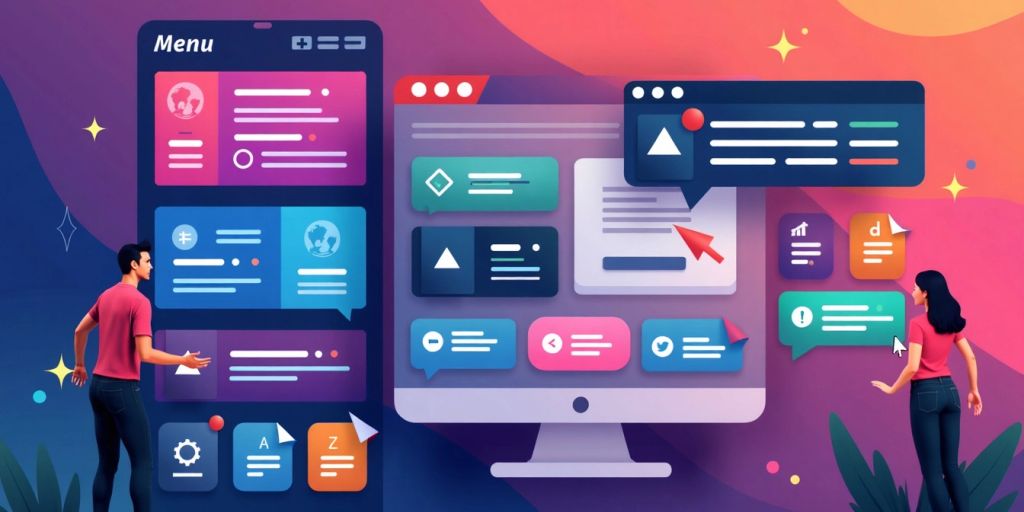
In this guide, we’ll explore how to effectively use Bootstrap’s menus, navbars, and modals to create user-friendly web applications. Whether you’re a beginner or looking to refine your skills, this article will provide you with practical insights and tips to enhance your web development projects.
Key Takeaways
- Bootstrap makes it easy to create dropdown menus that help organize content.
- Responsive navbars adapt to different screen sizes, ensuring a good user experience.
- Modals are useful for displaying important information without leaving the current page.
- Customizing Bootstrap components can give your project a unique look and feel.
- Best practices in accessibility ensure that all users can navigate your site easily.
Understanding Bootstrap Menus
Bootstrap menus are essential for creating user-friendly navigation on websites. They allow users to easily access different sections of a site. With Bootstrap, you can create various types of menus, including dropdowns and mega menus.
Creating Dropdown Menus
Dropdown menus are a popular choice for organizing links. Here’s how to create one:
- Use the
.dropdown
class to set up the menu. - Add a button or link that will trigger the dropdown.
- Include the
.dropdown-menu
class for the list of items.
Here’s a simple example:
<div class="dropdown">
<button class="btn btn-secondary dropdown-toggle" type="button" id="dropdownMenuButton" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false">
Dropdown button
</button>
<div class="dropdown-menu" aria-labelledby="dropdownMenuButton">
<a class="dropdown-item" href="#">Action</a>
<a class="dropdown-item" href="#">Another action</a>
<a class="dropdown-item" href="#">Something else here</a>
</div>
</div>
Styling and Customizing Menus
You can customize the look of your menus using Bootstrap’s utility classes. Here are some tips:
- Change colors with
.bg-*
and.text-*
classes. - Adjust padding and margins using spacing utilities.
- Use custom CSS for more specific styles.
Responsive Menu Design
Creating a responsive menu is crucial for mobile users. Bootstrap’s grid system helps in making menus that adapt to different screen sizes. Here are some key points:
- Use the
.navbar
class for a responsive navigation bar. - Implement media queries for additional customization.
- Test on various devices to ensure usability.
Remember, a well-designed menu enhances user experience and keeps visitors engaged.
Feature | Description |
---|---|
Easy to Use | Simple to implement with basic HTML knowledge. |
Responsive Design | Adapts to different screen sizes automatically. |
Customizable | Easily change styles to fit your project. |
Building a Responsive Navbar with Bootstrap
Creating a responsive navbar is essential for modern web design. Bootstrap makes it easy to create a navigation bar that adjusts to different screen sizes. Here’s how you can build one:
Navbar Basics
To start, you need to set up the basic structure of your navbar. Use the following HTML code:
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<a class="navbar-brand" href="#">Brand</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item active">
<a class="nav-link" href="#">Home</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Features</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Pricing</a>
</li>
</ul>
</div>
</nav>
Customizing the Navbar
You can customize your navbar by changing its color, size, and layout. Here are some tips:
- Use different background colors with classes like
bg-dark
orbg-light
. - Adjust the size using classes like
navbar-lg
ornavbar-sm
. - Add icons or images to enhance the visual appeal.
Adding Dropdowns to the Navbar
Dropdowns can make your navbar more functional. To add a dropdown, use the following code:
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" href="#" id="navbarDropdown" role="button" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false">
Dropdown
</a>
<div class="dropdown-menu" aria-labelledby="navbarDropdown">
<a class="dropdown-item" href="#">Action</a>
<a class="dropdown-item" href="#">Another action</a>
<div class="dropdown-divider"></div>
<a class="dropdown-item" href="#">Something else here</a>
</div>
</li>
A well-designed navbar can significantly improve user navigation and overall experience on your website.
In summary, building a responsive navbar with Bootstrap is straightforward. You can easily create a navbar that adapts to various devices by following these steps. Remember to test your navbar on different screen sizes to ensure it looks great everywhere!
Advanced Navbar Techniques
Sticky and Fixed Navbars
Sticky and fixed navbars are great for keeping navigation visible as users scroll. Here’s how they differ:
- Sticky Navbar: Stays at the top of the viewport when you scroll past it.
- Fixed Navbar: Always stays at the top, even when you scroll up or down.
To implement a sticky navbar, use the .sticky-top
class. For a fixed navbar, use .fixed-top
.
Collapsible Navbars
Collapsible navbars are essential for mobile responsiveness. They allow the navbar to collapse into a toggleable menu on smaller screens. Here’s how to create one:
- Use the
.navbar-expand-*
class to specify when the navbar should be collapsible. - Add a button with the
.navbar-toggler
class to toggle the menu. - Wrap the menu items in a collapsible div with the
.collapse
class.
Using Navbar with Offcanvas
Offcanvas components provide a way to hide the navbar off-screen until needed. This is especially useful for mobile views. To use it:
- Add the
.offcanvas
class to your navbar. - Use JavaScript to toggle the visibility of the offcanvas menu.
Tip: Offcanvas menus can enhance user experience by saving screen space.
Feature | Sticky Navbar | Fixed Navbar | Collapsible Navbar | Offcanvas Navbar |
---|---|---|---|---|
Always Visible | No | Yes | Yes | Yes |
Mobile Friendly | Yes | Yes | Yes | Yes |
Customizable | Yes | Yes | Yes | Yes |
Introduction to Bootstrap Modals
Bootstrap modals are a great way to display content in a dialog box that overlays the main content of your webpage. They are useful for showing important information without navigating away from the current page. Modals can enhance user interaction by providing a focused area for user input or notifications.
Creating Basic Modals
To create a basic modal in Bootstrap, you need to include the following components:
- Modal Structure: Use the appropriate HTML structure to define the modal.
- Trigger Button: A button or link that opens the modal when clicked.
- JavaScript: Ensure Bootstrap’s JavaScript is included to handle the modal’s functionality.
Here’s a simple example:
<!-- Button trigger modal -->
<button type="button" class="btn btn-primary" data-bs-toggle="modal" data-bs-target="#exampleModal">
Launch demo modal
</button>
<!-- Modal -->
<div class="modal fade" id="exampleModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Modal title</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
Your content goes here.
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-bs-dismiss="modal">Close</button>
<button type="button" class="btn btn-primary">Save changes</button>
</div>
</div>
</div>
</div>
Styling Modals
You can customize the appearance of modals using Bootstrap’s built-in classes or your own CSS. Here are some tips:
- Use utility classes to adjust margins and padding.
- Change colors using Bootstrap’s color classes.
- Add animations for a smoother appearance.
Modal Options and Methods
Bootstrap 5 modal options can be used in two ways: using data attributes and using JavaScript. Options are added to append different utilities and properties to enhance the modal’s functionality. Here are some common options:
backdrop
: Controls the modal backdrop behavior.keyboard
: Allows closing the modal with the keyboard.focus
: Automatically focuses on the modal when opened.
Modals are a powerful tool in web design, allowing for a clean and organized way to present information without cluttering the main interface.
By understanding how to create and customize modals, you can significantly improve the user experience on your website.
Enhancing User Experience with Modals
Using Modals for Forms
Modals are a great way to present forms without taking users away from their current page. Here are some benefits of using modals for forms:
- Space-saving: They keep the main page clean and focused.
- User engagement: Users can fill out forms without navigating away.
- Immediate feedback: You can show success or error messages right after submission.
Modals for Notifications
Notifications can be effectively displayed using modals. They can alert users about important updates or actions. Here are some key points:
- Visibility: Modals grab attention immediately.
- Interactivity: Users can take action directly from the notification.
- Customization: You can style notifications to match your website’s theme.
Interactive Modals
Interactive modals can enhance user experience by allowing users to engage with content directly. Consider these features:
- Dynamic content: Load different content based on user actions.
- Multiple actions: Allow users to perform various tasks within the modal.
- User-friendly design: Ensure the modal is easy to navigate and understand.
Modals are not just for showing information; they can be a powerful tool for improving user interaction on your website. By using them wisely, you can create a more engaging experience for your users.
Combining Menus, Navbar, and Modals
Integrating Menus in Navbar
Combining menus with a navbar can enhance navigation on your website. Here are some steps to achieve this:
- Use Bootstrap’s built-in classes to create a responsive navbar.
- Add dropdown menus using the
.dropdown
class within the navbar. - Ensure that the dropdowns are accessible by using proper ARIA attributes.
Triggering Modals from Navbar
You can easily trigger modals from your navbar. Here’s how:
- Use a button or link in the navbar that calls the modal.
- Add the
data-toggle="modal"
attribute to the button or link. - Specify the modal ID in the
data-target
attribute.
Best Practices for Combined Components
To ensure a smooth user experience when combining these components, consider the following:
- Keep the design consistent across menus, navbar, and modals.
- Test the responsiveness on different devices.
- Optimize for accessibility to make sure all users can navigate easily.
Combining these elements effectively can lead to a more user-friendly interface. Aligning two navbars in Bootstrap involves utilizing its built-in navbar classes and custom CSS styles to create and position navigation bars on a web page.
By following these guidelines, you can create a seamless navigation experience that enhances your website’s usability.
Customizing Bootstrap Components
Using Bootstrap’s Utility Classes
Bootstrap provides a variety of utility classes that make it easy to customize components without writing additional CSS. Here are some common utility classes you can use:
- Margin and Padding: Use classes like
.m-3
or.p-2
to adjust spacing. - Text Color: Change text color with classes like
.text-primary
or.text-danger
. - Background Color: Set background colors using
.bg-success
or.bg-warning
.
Overriding Default Styles
If you want to change the default styles of Bootstrap components, you can do so by adding your own CSS. Here’s how:
- Create a custom CSS file.
- Link it in your HTML after the Bootstrap CSS link.
- Use more specific selectors to override Bootstrap styles.
For example:
.custom-button {
background-color: #4CAF50;
color: white;
}
Creating Custom Themes
Creating a custom theme allows you to maintain a consistent look across your project. Here are steps to create one:
- Choose a Color Palette: Select colors that represent your brand.
- Modify Bootstrap Variables: If using SASS, change Bootstrap’s default variables.
- Test Your Theme: Ensure all components look good with your new styles.
Customizing Bootstrap can greatly enhance your project’s design and user experience. By using utility classes and custom styles, you can create a unique look that fits your needs.
Summary
In summary, customizing Bootstrap components is straightforward. You can use utility classes for quick adjustments, override styles with custom CSS, and create themes for a cohesive design. This flexibility makes Bootstrap a powerful tool for web development.
Bootstrap Menus, Navbar, and Modals in Real Projects
In real-world applications, Bootstrap components like menus, navbars, and modals play a crucial role in enhancing user experience. Here’s how they can be effectively utilized:
Case Study: Employee Management System
- Features: This project includes a login page, employee lists, and modals for adding or editing employee details.
- Navbar: A responsive navbar allows easy navigation between different sections.
- Modals: Used for confirming actions like deletions or for displaying detailed employee information.
Case Study: Blog Application
- Navbar: The navbar includes links to home, posts, and categories, making it user-friendly.
- Dropdowns: Dropdown menus can be used for categories or tags, enhancing navigation.
- Modals: Implemented for user comments or notifications, improving interactivity.
Case Study: Educational Website
- Menus: A well-structured menu helps users find courses and resources quickly.
- Navbar: Features a sticky navbar that remains visible while scrolling, ensuring easy access.
- Modals: Used for course details and registration forms, streamlining the user experience.
Project Type | Key Features | Components Used |
---|---|---|
Employee Management System | Login, Employee Lists, Modals | Navbar, Modals |
Blog Application | Posts, Categories, User Comments | Navbar, Dropdowns, Modals |
Educational Website | Course Listings, Registration Forms | Menus, Sticky Navbar, Modals |
Using Bootstrap components effectively can significantly improve the usability and functionality of your web projects.
By integrating these components, developers can create dynamic and responsive web applications that cater to user needs efficiently.
Troubleshooting Common Issues
Debugging Menus
When your menus aren’t working as expected, consider the following steps:
- Check for JavaScript Errors: Open your browser’s console to see if there are any errors.
- Inspect HTML Structure: Ensure your menu’s HTML is correctly structured according to Bootstrap’s guidelines.
- Verify CSS Styles: Make sure your custom styles aren’t conflicting with Bootstrap’s default styles.
Fixing Navbar Problems
If your navbar isn’t displaying correctly, try these solutions:
- Ensure Proper Classes: Check that you are using the correct Bootstrap classes for your navbar.
- Responsive Design: Make sure your navbar is responsive by testing it on different screen sizes.
- JavaScript Functionality: Ensure that the necessary JavaScript files are included and loaded properly.
Resolving Modal Issues
Modals can sometimes be tricky. Here’s how to troubleshoot:
- Check Trigger Elements: Ensure that the elements meant to trigger the modal are correctly set up.
- Inspect Modal Markup: Verify that your modal’s HTML structure is correct.
- JavaScript Errors: Look for any JavaScript errors that might prevent the modal from opening.
Remember: Always test your components in different browsers to catch any cross-browser issues early on.
By following these steps, you can effectively troubleshoot common issues with Bootstrap menus, navbars, and modals. If you encounter a specific problem, refer to the Bootstrap documentation for more detailed guidance.
Highlighted Context
In this tutorial, we will learn how to create a multi-level menu navbar using Bootstrap 5. This kind of navbar is perfect when you need to organize your content efficiently.
Best Practices for Accessibility
Making Menus Accessible
To ensure that your menus are accessible to all users, consider the following tips:
- Use semantic HTML: This helps screen readers understand the structure of your menu.
- Implement keyboard navigation: Users should be able to navigate through the menu using the keyboard alone.
- Add ARIA roles: These attributes help assistive technologies interpret your menu correctly.
Accessible Navbar Design
Creating an accessible navbar involves:
- Clear labeling: Ensure that each link is descriptive enough for users to understand its purpose.
- Focus indicators: Make sure that users can see which item is currently focused when navigating with a keyboard.
- Skip links: Provide a way for users to skip directly to the main content, bypassing the navbar if they choose.
Ensuring Modals are Screen Reader Friendly
When using modals, keep these points in mind:
- Properly manage focus: When a modal opens, focus should shift to it, and when it closes, focus should return to the triggering element.
- Use aria-labelledby and aria-describedby: These attributes help screen readers announce the modal’s title and content.
- Close buttons: Ensure that the close button is easily accessible and clearly labeled.
Remember, accessibility is not just a feature; it’s a necessity. By following these best practices, you can create a more inclusive experience for all users.
By implementing these strategies, you can enhance the accessibility of your Bootstrap components, ensuring that everyone can navigate and interact with your site effectively. Don’t forget about accessibility; Bootstrap has specific attributes and classes that will allow assistive technology to function properly on your app. Test your components regularly to ensure they meet accessibility standards.
Performance Optimization Tips
Minimizing Load Times
To ensure your website loads quickly, consider the following strategies:
- Minify your CSS and JS files: This reduces file size by removing unnecessary spaces and comments.
- Optimize images: Use formats like JPEG or WebP for better compression without losing quality.
- Consider using a Content Delivery Network (CDN): This helps deliver your content faster by using servers closer to your users.
Efficient Use of JavaScript
JavaScript can slow down your site if not used wisely. Here are some tips:
- Load scripts asynchronously to prevent blocking the rendering of your page.
- Use only the necessary libraries and plugins to keep your code lightweight.
- Defer loading non-essential scripts until after the main content has loaded.
Optimizing CSS for Bootstrap Components
To enhance the performance of your Bootstrap components:
- Use Bootstrap’s utility classes instead of writing custom CSS when possible.
- Override default styles only when necessary to keep your CSS clean and efficient.
- Create custom themes that only include the styles you need, reducing the overall CSS file size.
Remember, a fast website improves user experience and can boost your search engine rankings.
If you want to improve your coding skills and get ready for interviews, check out our website! We offer fun and easy lessons that can help you learn at your own pace. Don’t wait—start your journey to success today!
Wrapping Up: Your Bootstrap Journey
In conclusion, mastering Bootstrap menus, navbars, and modals can greatly enhance your web development skills. This guide has walked you through the basics and more advanced features, helping you understand how to create responsive and user-friendly designs. By practicing with real projects, you can apply what you’ve learned and build impressive websites. Remember, the more you practice, the better you’ll get. So, keep experimenting with Bootstrap, and soon you’ll be creating amazing web applications with ease!
Frequently Asked Questions
What is Bootstrap?
Bootstrap is a free tool that helps you build websites quickly. It has ready-made designs and styles for buttons, menus, and more.
How can I create a dropdown menu in Bootstrap?
To make a dropdown menu, use the class ‘dropdown’ in your HTML. You can add items inside it to show when you click.
Is Bootstrap good for mobile websites?
Yes! Bootstrap is designed to make websites look good on phones and tablets. It adjusts automatically to different screen sizes.
Can I customize Bootstrap components?
Absolutely! You can change colors, sizes, and styles using your own CSS, or use Bootstrap’s built-in classes.
What are Bootstrap modals?
Modals are pop-up windows that show information without leaving the page. You can use them for forms or alerts.
How do I make a navbar responsive?
You can make a navbar responsive by using Bootstrap’s classes like ‘navbar-expand’ to adjust how it looks on smaller screens.
What are the advantages of using Bootstrap?
Bootstrap saves time with its ready-made components. It also helps ensure your website works well on all devices.
Can I learn Bootstrap as a beginner?
Yes! Bootstrap is beginner-friendly, and there are many tutorials available to help you understand how to use it.