Mastering Big O for Coding Interviews: A Comprehensive Guide
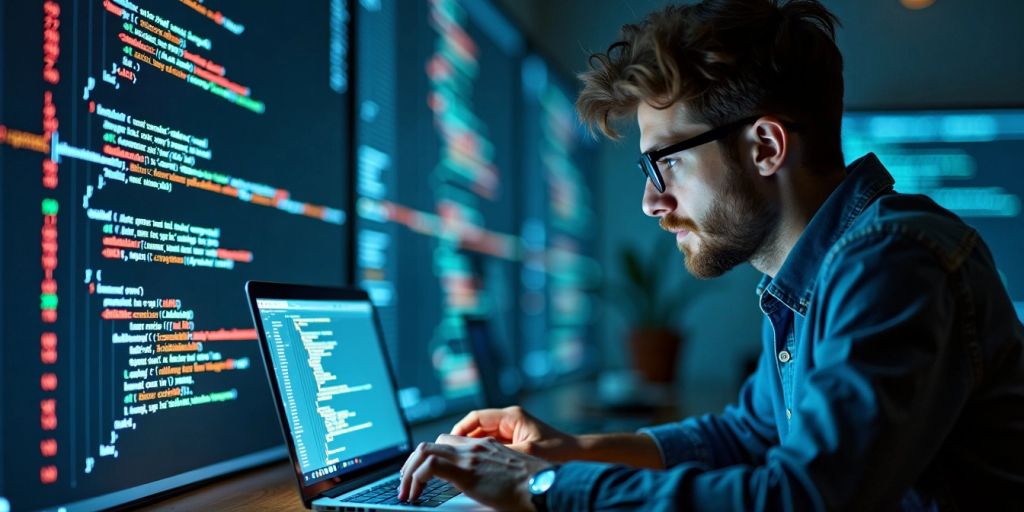
Understanding Big O notation is crucial for anyone interested in coding and algorithms. This guide breaks down the key concepts of Big O, making it easier to grasp its significance in coding interviews and software development. Whether you’re a beginner or looking to sharpen your skills, this overview will help you navigate the world of algorithm efficiency.
Key Takeaways
- Big O notation helps measure how fast an algorithm runs as the input size grows.
- Understanding Big O is essential for coding interviews, especially with top tech companies.
- Different types of time complexities include constant, linear, and exponential.
- Space complexity is just as important as time complexity when analyzing algorithms.
- Big O can help you choose the best data structure for a problem.
- Common pitfalls include neglecting edge cases and miscalculating complexities.
- Visual aids like graphs can make understanding Big O easier.
- Practice is key—use sample problems and mock interviews to prepare.
Understanding Big O Notation
Definition of Big O
Big O notation is a way to describe how the time or space requirements of an algorithm grow as the input size increases. It helps us understand the efficiency of algorithms by focusing on the worst-case scenario. For example, an algorithm that runs in O(n) time means that if the input size doubles, the time taken will also roughly double.
Importance in Algorithm Analysis
Understanding Big O is crucial for analyzing algorithms. It allows developers to compare different algorithms and choose the most efficient one for a given problem. This is especially important in coding interviews, where efficiency can be a deciding factor.
Common Misconceptions
Many people think that Big O notation gives an exact measure of performance. However, it only provides a rough estimate. It ignores constants and lower-order terms, focusing instead on the most significant factors that affect performance as input size grows.
Real-World Applications
Big O notation is used in various fields, including software development, data analysis, and machine learning. It helps in optimizing algorithms, ensuring that applications run efficiently even with large datasets.
Historical Context
Big O notation was introduced by mathematician Paul Bachmann in the late 19th century. It has since become a standard way to analyze algorithm efficiency in computer science.
Big O vs. Other Notations
While Big O is the most commonly used notation, there are others like Big Theta (Θ) and Big Omega (Ω). Big Theta provides a tight bound on the performance, while Big Omega gives a lower bound. Understanding these differences can help in more precise algorithm analysis.
Types of Time Complexity
Constant Time Complexity
An algorithm is said to have constant time complexity when its execution time does not change regardless of the input size. This is denoted as O(1). For example, accessing an element in an array by its index is a constant time operation.
Linear Time Complexity
An algorithm has linear time complexity when its execution time increases linearly with the input size. This is represented as O(n). A common example is a simple loop that goes through each element in an array.
Logarithmic Time Complexity
Logarithmic time complexity is when the execution time grows logarithmically as the input size increases. This is denoted as O(log n). A classic example is the binary search algorithm, which efficiently narrows down the search space by half each time.
Quadratic Time Complexity
An algorithm is said to have quadratic time complexity when its execution time is proportional to the square of the input size, represented as O(n²). This often occurs with nested loops, where each element is compared with every other element.
Exponential Time Complexity
Exponential time complexity is when the execution time doubles with each additional element in the input. This is denoted as O(2^n). Algorithms with this complexity are generally impractical for large inputs, such as the brute force solution to the traveling salesman problem.
Comparative Analysis of Complexities
Here’s a quick comparison of different time complexities:
Time Complexity | Notation | Description |
---|---|---|
Constant | O(1) | Time remains constant regardless of input size |
Linear | O(n) | Time increases linearly with input size |
Logarithmic | O(log n) | Time increases logarithmically with input size |
Quadratic | O(n²) | Time increases with the square of input size |
Exponential | O(2^n) | Time doubles with each additional input |
Understanding these complexities is crucial for selecting the right algorithm for a problem. Different algorithms can have vastly different performance based on their time complexity.
Types of Space Complexity
Understanding Space Complexity
Space complexity measures how much memory an algorithm needs based on the size of its input. It is expressed using Big O notation, just like time complexity. Understanding space complexity is crucial for designing algorithms that are not only fast but also memory efficient.
Memory Usage in Algorithms
Here are some common types of space complexity:
Space Complexity Type | Description | Example |
---|---|---|
Constant Space (O(1)) | Uses a fixed amount of memory regardless of input size. | Swapping two variables. |
Linear Space (O(n)) | Memory grows linearly with input size. | Creating a copy of an array. |
Quadratic Space (O(n²)) | Memory grows quadratically with input size. | Storing a 2D matrix. |
Trade-offs Between Time and Space
When designing algorithms, there are often trade-offs between time and space complexity. For example, an algorithm that uses more memory might run faster, while one that uses less memory might be slower. It’s essential to find a balance based on the specific needs of your application.
Examples of Space Complexity
- Constant Space (O(1)): A function that swaps two numbers uses a fixed amount of space.
- Linear Space (O(n)): A function that duplicates an array requires space proportional to the size of the input array.
- Quadratic Space (O(n²)): A function that creates a 2D array for storing data.
Impact on Performance
The space complexity of an algorithm can significantly impact its performance, especially in environments with limited memory. Choosing the right data structure can help optimize both time and space complexity.
Space Complexity in Data Structures
Understanding space complexity is vital when working with data structures. For instance, a linked list may use more memory than an array due to the overhead of storing pointers.
In summary, space complexity is a way to measure how much memory an algorithm needs to run. It tells us the total amount of space or memory an algorithm will use from start to finish.
By being aware of these complexities, you can make better decisions when designing algorithms and choosing data structures.
Analyzing Algorithms with Big O
Step-by-Step Analysis
To analyze an algorithm using Big O notation, follow these steps:
- Identify the input size (usually denoted as
n
). - Determine the basic operations that the algorithm performs.
- Count how many times these operations are executed as the input size increases.
- Express this count using Big O notation.
Identifying Dominant Terms
When analyzing, focus on the dominant term in your calculations. For example, if an algorithm has a time complexity of 3n^2 + 2n + 1
, the dominant term is n^2
, so we express it as O(n²).
Best, Average, and Worst Cases
Understanding the different cases is crucial:
- Best Case: The minimum time an algorithm takes to complete.
- Average Case: The expected time for a random input.
- Worst Case: The maximum time an algorithm could take, often what we focus on in Big O analysis.
Practical Examples
Here are some common algorithms and their complexities:
Algorithm Type | Time Complexity |
---|---|
Linear Search | O(n) |
Binary Search | O(log n) |
Bubble Sort | O(n²) |
Merge Sort | O(n log n) |
Common Patterns in Analysis
Some common patterns to look for include:
- Nested loops often lead to quadratic time complexity (O(n²)).
- Dividing the problem usually results in logarithmic time complexity (O(log n)).
Using Big O in Real Scenarios
In real-world applications, understanding Big O helps in making decisions about which algorithms to use based on their efficiency. For instance, when sorting data, choosing an algorithm with a lower time complexity can significantly improve performance.
In summary, mastering Big O notation allows you to analyze algorithms effectively, ensuring you choose the right one for your needs. Understanding the worst, average, and best case analysis of algorithms is essential for making informed decisions in programming.
Big O Cheat Sheet
Quick Reference Guide
Big O notation is a way to describe how the time or space requirements of an algorithm grow as the input size increases. Here’s a quick reference:
Complexity Type | Big O Notation | Description |
---|---|---|
Constant Time | O(1) | Time remains the same regardless of input size. |
Linear Time | O(n) | Time grows linearly with input size. |
Logarithmic Time | O(log n) | Time grows logarithmically as input size increases. |
Quadratic Time | O(n²) | Time grows quadratically with input size. |
Exponential Time | O(2ⁿ) | Time doubles with each additional input. |
Common Algorithms and Their Complexities
Here are some common algorithms and their time complexities:
- Searching:
- Linear Search: O(n)
- Binary Search: O(log n)
- Sorting:
- Bubble Sort: O(n²)
- Merge Sort: O(n log n)
Data Structures and Their Complexities
Different data structures have different complexities for common operations:
- Array:
- Access: O(1)
- Search: O(n)
- Linked List:
- Access: O(n)
- Search: O(n)
- Hash Table:
- Access: O(1)
- Search: O(1)
Visual Representation of Complexities
Understanding how these complexities grow can be easier with graphs. For example, a graph comparing O(1), O(n), and O(n²) shows how quickly they diverge as n increases.
Tips for Memorization
- Use Mnemonics: Create phrases to remember the order of complexities.
- Practice Problems: Regularly solve problems to reinforce your understanding.
Printable Cheat Sheet
Consider creating a printable version of this cheat sheet for quick reference during coding interviews or study sessions.
Remember: Mastering Big O notation is essential for analyzing algorithms and improving your coding skills!
Big O in Coding Interviews
Why Interviewers Ask About Big O
Understanding Big O notation is crucial in coding interviews because it helps assess how well you can analyze the efficiency of algorithms. Interviewers want to see if you can evaluate the time and space complexity of your solutions. This knowledge is essential for making informed decisions about which algorithms and data structures to use.
Common Interview Questions
Here are some typical questions you might encounter:
- What is the time complexity of a given algorithm?
- Can you explain the difference between O(n) and O(log n)?
- How does the choice of data structure affect performance?
How to Approach Big O Questions
- Identify the algorithm: Understand what the algorithm is doing.
- Count operations: Look at loops, recursive calls, and other operations.
- Determine the dominant term: Focus on the term that grows the fastest as input size increases.
Demonstrating Your Knowledge
When discussing Big O in interviews, be clear and concise. Use examples to illustrate your points. For instance, you might say, "The time complexity of a binary search is O(log n) because it divides the input in half each time."
Real Interview Experiences
Many candidates find that practicing Big O problems helps them feel more confident. Sharing your thought process during the interview can also impress interviewers.
Tips for Success
- Practice regularly: Use platforms like LeetCode or HackerRank to solve problems.
- Understand common patterns: Familiarize yourself with common algorithms and their complexities.
- Stay calm: If you get stuck, talk through your thought process. Interviewers appreciate candidates who can think aloud.
Understanding Big O notation provides a standardized way to describe the time and space complexity of algorithms, enabling developers to analyze and compare different solutions effectively.
Preparing for Coding Interviews
Refreshing Your Knowledge
Before diving into practice, it’s essential to refresh your knowledge of key concepts. Make sure you understand:
- Data Structures:
- Algorithms:
Practicing with Sample Problems
Once you feel confident, start solving coding problems. Here are some resources:
- 73 Data Structure Interview Questions
- 71 Algorithms Interview Questions
Mock Interviews
Practicing with someone else can be very beneficial. If possible, find a partner to simulate the interview experience. This helps you get used to explaining your thought process out loud.
Utilizing Online Resources
There are many platforms available for coding practice. Some popular ones include:
- LeetCode
- HackerRank
- CodeSignal
Building a Study Plan
Create a structured study plan that includes:
- Daily practice sessions
- Weekly mock interviews
- Review of concepts and mistakes
Tracking Your Progress
Keep a log of your practice sessions and progress. This will help you identify areas that need improvement.
Remember, the key to success in coding interviews is not just solving problems but also communicating your thought process clearly.
Tips for Success
- Stay calm during the interview.
- Think aloud to show your reasoning.
- Ask clarifying questions if needed.
- Practice regularly to build confidence.
By following these steps, you’ll be well-prepared to tackle your coding interview and demonstrate your understanding of Big O notation and other essential concepts.
Common Big O Pitfalls
Overlooking Edge Cases
One common mistake is overlooking edge cases when analyzing algorithms. Edge cases can significantly affect the performance of an algorithm, leading to incorrect complexity assessments. Always consider how your algorithm behaves with minimal or maximal input sizes.
Misinterpreting Complexity
Many people misinterpret what Big O notation represents. It describes the upper limit of an algorithm’s performance, not the exact time it will take. Understanding this distinction is crucial for accurate analysis.
Assuming Worst Case is Always Worst
It’s a common misconception that the worst-case scenario is always the most important. In many real-world applications, average-case performance is more relevant. Always analyze all cases: best, average, and worst.
Neglecting Space Complexity
While time complexity often gets the spotlight, neglecting space complexity can lead to inefficient algorithms. Be sure to evaluate how much memory your algorithm uses, especially in large-scale applications.
Ignoring Constants and Lower Order Terms
Big O notation focuses on the highest order term, but ignoring constants and lower order terms can lead to misunderstandings. For example, an algorithm with a complexity of O(2n) is still linear, but it may perform worse than an O(n) algorithm in practice.
Common Miscalculations
Miscalculations can happen easily, especially when dealing with nested loops or recursive functions. Always double-check your calculations to ensure accuracy.
Pitfall | Description | Impact |
---|---|---|
Overlooking Edge Cases | Ignoring how algorithms behave with extreme inputs. | Can lead to incorrect complexity. |
Misinterpreting Complexity | Confusing upper limits with exact performance. | Results in flawed analysis. |
Assuming Worst Case is Always Worst | Focusing only on worst-case scenarios. | Misses average-case performance. |
Neglecting Space Complexity | Ignoring memory usage in algorithms. | Can lead to inefficient solutions. |
Ignoring Constants and Lower Order Terms | Overlooking smaller terms in complexity. | Misunderstands performance. |
Common Miscalculations | Errors in calculating complexities, especially in loops. | Leads to wrong conclusions. |
Always remember that understanding the nuances of Big O notation is essential for effective algorithm analysis. Linting tools and automated code review software can help catch common mistakes, taking some of the bias out of the equation.
Big O and Data Structures
Choosing the Right Data Structure
When solving problems, selecting the appropriate data structure is crucial. Different data structures have different complexities, which can significantly affect performance. Here are some common data structures and their time complexities:
Data Structure | Access | Search | Insertion | Deletion |
---|---|---|---|---|
Array | O(1) | O(n) | O(n) | O(n) |
Linked List | O(n) | O(n) | O(1) | O(1) |
Stack | O(n) | O(n) | O(1) | O(1) |
Queue | O(n) | O(n) | O(1) | O(1) |
HashMap | O(1) | O(1) | O(1) | O(1) |
Impact of Data Structures on Complexity
The choice of data structure can greatly influence the overall efficiency of an algorithm. For example, using a hashmap for checking unique values is much faster than using a list, as it offers constant time complexity for lookups.
Comparing Data Structures
When comparing data structures, consider:
- Time Complexity: How fast operations are performed.
- Space Complexity: How much memory is used.
- Use Case: What problem you are trying to solve.
Common Operations and Their Complexities
Here’s a quick reference for common operations:
- Array: Fast access but slow insertion.
- Linked List: Fast insertion but slow access.
- HashMap: Fast access and insertion, ideal for unique values.
Real-World Examples
In real-world applications, the right data structure can lead to better performance. For instance, a simulation that requires frequent insertions and deletions would benefit from using a queue, which has constant time complexities for these operations.
Data Structures in Interviews
Understanding data structures and their complexities is essential for coding interviews. Interviewers often ask about the trade-offs of different data structures to assess your problem-solving skills and knowledge of Big O notation.
Visualizing Big O
Graphs and Charts
Visualizing Big O notation through graphs and charts can help you understand how different algorithms perform as the input size increases. Here are some common types of complexities represented graphically:
- O(1): Constant time complexity, represented as a flat line.
- O(n): Linear time complexity, shown as a straight line that rises steadily.
- O(log n): Logarithmic time complexity, which increases quickly at first and then levels off.
- O(n^2): Quadratic time complexity, depicted as a steep curve that rises sharply.
Understanding Growth Rates
Understanding how different complexities grow is crucial. Here’s a quick comparison:
Complexity Type | Growth Rate |
---|---|
O(1) | Constant |
O(log n) | Slow |
O(n) | Linear |
O(n log n) | Moderate |
O(n^2) | Fast |
O(2^n) | Very Fast |
Comparative Graphs of Complexities
When comparing algorithms, it’s helpful to visualize their performance:
- Plot multiple algorithms on the same graph to see which one performs better as the input size increases.
- Use different colors for each algorithm to make the graph easy to read.
Using Visuals in Interviews
In coding interviews, being able to explain your thought process visually can set you apart. Here are some tips:
- Draw graphs to illustrate your points.
- Use simple examples to explain complex concepts.
- Practice explaining your visuals clearly and concisely.
Tools for Visualization
There are several tools available to help you create visual representations of Big O notation:
- Graphing software like Desmos or GeoGebra.
- Online graphing calculators for quick visualizations.
- Programming libraries like Matplotlib in Python for custom graphs.
Creating Your Own Visuals
Creating your own visuals can deepen your understanding. Try:
- Sketching graphs by hand to visualize different complexities.
- Using software to create more polished visuals.
- Experimenting with different data sets to see how performance changes.
Visualizing Big O notation not only aids in understanding but also enhances your ability to communicate complex ideas effectively. By mastering these visual tools, you can better analyze algorithms and present your findings clearly.
Big O and Algorithm Design
Designing Efficient Algorithms
When creating algorithms, it’s crucial to consider their efficiency. Big O notation helps in understanding how an algorithm’s performance changes with input size. Here are some key points to keep in mind:
- Understand the problem: Clearly define what you need to solve.
- Choose the right approach: Consider different algorithms and their complexities.
- Optimize: Look for ways to reduce time and space complexity.
Balancing Time and Space
In algorithm design, you often face a trade-off between time and space. Here’s a simple comparison:
Complexity Type | Time Complexity | Space Complexity |
---|---|---|
Constant | O(1) | O(1) |
Linear | O(n) | O(1) |
Quadratic | O(n²) | O(n) |
Exponential | O(2^n) | O(n) |
Iterative vs. Recursive Approaches
Choosing between iterative and recursive methods can impact performance:
- Iterative: Generally uses less memory and is faster.
- Recursive: Easier to implement for problems like tree traversals but can lead to higher space usage.
Optimization Techniques
To enhance algorithm performance, consider these techniques:
- Memoization: Store results of expensive function calls.
- Divide and Conquer: Break problems into smaller subproblems.
- Greedy Algorithms: Make the best choice at each step.
Real-World Algorithm Design
In real-world applications, efficient algorithms can significantly improve performance. For instance, sorting algorithms like mergesort and quicksort are designed to handle large datasets efficiently.
Case Studies
Analyzing successful algorithms can provide insights into effective design. For example, the Dijkstra algorithm for shortest paths uses a priority queue to optimize performance, demonstrating the importance of choosing the right data structure.
In summary, mastering Big O notation is essential for designing algorithms that are both efficient and scalable. By understanding the complexities involved, you can create solutions that perform well under various conditions.
Big O in Different Programming Languages
Language-Specific Considerations
When working with different programming languages, it’s important to understand how Big O notation applies to each one. Each language has its own characteristics that can affect the performance of algorithms. Here are some key points to consider:
- Python: Known for its simplicity, but can be slower due to dynamic typing.
- Java: Offers strong performance with static typing, but can have overhead from its virtual machine.
- C++: Generally faster due to low-level memory management, but requires careful handling of resources.
- JavaScript: Great for web applications, but performance can vary based on the engine used.
Performance Variations
Different languages can lead to different performance outcomes for the same algorithm. Here’s a quick comparison:
Language | Typical Complexity | Notes |
---|---|---|
Python | O(n) | Slower due to interpreted nature |
Java | O(n) | Faster with JIT compilation |
C++ | O(n) | Fastest due to compiled nature |
JavaScript | O(n) | Performance varies with engine |
Common Libraries and Their Complexities
Many programming languages come with libraries that can simplify tasks. Here are some common libraries and their complexities:
- Python: NumPy (O(n) for many operations)
- Java: Collections Framework (O(1) for HashMap)
- C++: STL (O(log n) for set operations)
- JavaScript: Lodash (O(n) for most array methods)
Cross-Language Comparisons
When comparing algorithms across languages, consider:
- Execution Speed: How fast does the algorithm run in each language?
- Memory Usage: Does one language use more memory than another?
- Ease of Implementation: Is it easier to write in one language over another?
Best Practices Across Languages
To optimize your algorithms regardless of the language:
- Always analyze the time and space complexity.
- Choose the right data structures based on Big O analysis.
- Test your algorithms in different environments to see how they perform.
Language-Specific Examples
Here are a few examples of how Big O notation applies in different languages:
- Python: List comprehensions can be O(n).
- Java: Using ArrayList for dynamic arrays is O(1) for access.
- C++: Using vectors can be O(1) for access but O(n) for insertions.
- JavaScript: Array methods like
map
are O(n).
Understanding how Big O notation varies across programming languages can help you make better choices when designing algorithms. By being aware of these differences, you can optimize your code for performance and efficiency.
Advanced Big O Concepts
Amortized Analysis
Amortized analysis helps us understand the average time complexity of an operation over a sequence of operations. This is useful when a single operation might take a long time, but most operations are quick. For example, if you have a dynamic array that occasionally needs to resize, the average time for adding an element remains low even if resizing takes longer occasionally.
Probabilistic Analysis
Probabilistic analysis looks at the expected time complexity of an algorithm when randomness is involved. This is important for algorithms that use randomization to improve performance. For instance, randomized quicksort can have a better average-case time complexity than its deterministic counterpart.
Randomized Algorithms
Randomized algorithms use random numbers to make decisions during execution. They can be faster and simpler than deterministic algorithms. For example, the randomized selection algorithm can find the k-th smallest element in an array more efficiently than sorting the entire array.
Complexity Classes
Complexity classes categorize problems based on their time and space requirements. Some common classes include:
- P: Problems solvable in polynomial time.
- NP: Problems verifiable in polynomial time.
- NP-Complete: The hardest problems in NP, where if one can be solved quickly, all can.
Understanding NP-Completeness
NP-completeness is a key concept in computer science. It helps us identify problems that are difficult to solve but easy to check. If you can prove a problem is NP-complete, it means no efficient solution is known, and it’s unlikely one exists.
Real-World Applications of Advanced Concepts
Understanding these advanced concepts can help in various fields, such as:
- Algorithm design: Creating efficient algorithms for complex problems.
- Cryptography: Ensuring secure communication through complex algorithms.
- Machine learning: Optimizing algorithms for better performance.
In summary, mastering advanced Big O concepts allows you to tackle complex problems more effectively and design better algorithms. Understanding these principles is crucial for anyone looking to excel in computer science and software development.
Big O and Software Development
Impact on Software Performance
Understanding Big O notation is crucial for software developers. It helps in evaluating how efficient an algorithm is, especially when dealing with large data sets. By knowing the time and space complexities, developers can make better choices about which algorithms to use.
Scalability Considerations
When designing software, scalability is key. Here are some points to consider:
- Choose efficient algorithms: Algorithms with lower time complexity are generally preferred.
- Optimize data structures: Selecting the right data structure can significantly impact performance.
- Plan for growth: Consider how the software will perform as the amount of data increases.
Refactoring for Efficiency
Refactoring code to improve its efficiency can lead to better performance. Here are steps to follow:
- Identify bottlenecks: Use profiling tools to find slow parts of the code.
- Analyze complexity: Check the Big O notation of the current algorithms.
- Implement improvements: Replace inefficient algorithms with more efficient ones.
Best Practices in Development
To ensure your software is efficient, follow these best practices:
- Regularly review and analyze your code for performance.
- Keep learning about new algorithms and data structures.
- Test your software under different loads to see how it performs.
Real-World Software Examples
Many successful software applications utilize Big O analysis to enhance performance. For instance, search engines use efficient algorithms to quickly retrieve data, ensuring a smooth user experience.
Understanding Big O notation is essential for creating efficient software solutions. It allows developers to make informed decisions that lead to better performance and scalability.
Big O and System Design
Importance in System Architecture
Understanding Big O notation is crucial for designing systems that can handle large amounts of data efficiently. It helps in predicting how an algorithm will perform as the input size grows. By knowing the time and space complexities, developers can make informed choices about which algorithms and data structures to use.
Designing for Scalability
When designing a system, scalability is key. Here are some considerations:
- Choose efficient algorithms: Opt for algorithms with lower time complexities.
- Select appropriate data structures: Use data structures that minimize time for common operations.
- Plan for growth: Ensure that the system can handle increased loads without significant performance drops.
Trade-offs in System Design
Designing a system often involves trade-offs. Here are some common ones:
- Time vs. Space: Sometimes, using more memory can speed up processing time.
- Complexity vs. Maintainability: A more complex algorithm might be faster but harder to maintain.
- Performance vs. Readability: Code that is easier to read may not always be the fastest.
Real-World System Examples
- Web Applications: Efficient database queries can significantly improve load times.
- Streaming Services: Algorithms that optimize data delivery can enhance user experience.
Case Studies in System Design
Analyzing successful systems can provide insights into effective design strategies. For instance, many large-scale applications use caching to reduce load times, demonstrating the importance of understanding Big O in real-world scenarios.
Best Practices for System Design
- Regularly review algorithms: Ensure they meet current performance needs.
- Benchmark performance: Test how changes affect system efficiency.
- Stay updated: Keep learning about new algorithms and data structures.
In summary, mastering Big O notation is essential for creating systems that are not only efficient but also scalable and maintainable. By applying these principles, developers can build robust applications that perform well under pressure.
Complexity Type | Description | Example |
---|---|---|
O(1) | Constant time | Accessing an array element |
O(n) | Linear time | Looping through an array |
O(n^2) | Quadratic time | Nested loops |
O(log n) | Logarithmic time | Binary search |
Big O and Machine Learning
Understanding Complexity in ML Algorithms
In machine learning, Big O notation helps us understand how the performance of algorithms changes with different input sizes. This is crucial because as datasets grow, the efficiency of our algorithms can significantly impact training time and resource usage.
Impact on Model Training
When training models, the time complexity can vary based on the algorithm used. Here’s a quick overview of some common algorithms and their complexities:
Algorithm | Time Complexity |
---|---|
Linear Regression | O(n) |
Decision Trees | O(n log n) |
Neural Networks | O(n^2) (varies) |
K-Means Clustering | O(n * k * i) |
Evaluating ML Algorithms
When choosing an algorithm, consider:
- Training Time: How long it takes to train the model.
- Prediction Time: How quickly the model can make predictions.
- Memory Usage: How much memory the algorithm requires.
Real-World ML Examples
In practice, understanding Big O can help in:
- Optimizing Training: Selecting algorithms that train faster on large datasets.
- Scaling Models: Ensuring models can handle increased data without significant slowdowns.
- Resource Management: Allocating computational resources effectively based on complexity.
Trade-offs in ML Complexity
When designing machine learning systems, you often face trade-offs:
- Accuracy vs. Speed: More complex models may yield better accuracy but take longer to train.
- Simplicity vs. Performance: Simpler models are easier to interpret but may not perform as well on complex tasks.
Best Practices for ML
To effectively use Big O in machine learning:
- Always analyze the complexity of algorithms before implementation.
- Use profiling tools to measure actual performance.
- Keep scalability in mind when designing models.
Understanding the complexities involved in machine learning algorithms is essential for building efficient and scalable systems. By mastering Big O, you can make informed decisions that enhance performance and resource utilization.
Big O and Competitive Programming
Importance in Competitive Coding
In competitive programming, understanding Big O notation is crucial. It helps you evaluate how fast your algorithms run as the input size increases. This is important because faster algorithms can lead to better performance in competitions.
Common Challenges
Here are some common challenges you might face:
- Time Limits: Many competitions have strict time limits for each problem.
- Complexity Analysis: You need to analyze the time and space complexity of your solutions quickly.
- Optimization: Finding the most efficient solution is often key to winning.
Strategies for Success
To succeed in competitive programming, consider these strategies:
- Practice Regularly: Solve problems on platforms like Codeforces or LeetCode.
- Learn Different Algorithms: Familiarize yourself with various algorithms and their complexities.
- Analyze Your Solutions: After solving a problem, review your solution’s complexity.
Real-World Competitive Examples
Many competitions, like ACM ICPC or Google Code Jam, require participants to solve problems under time constraints. Understanding Big O notation can help you choose the right algorithm to solve problems efficiently.
Analyzing Competitions
When analyzing competitions, consider:
- The types of problems presented.
- The average time complexity of winning solutions.
- How different algorithms perform under various conditions.
Best Practices for Preparation
To prepare effectively:
- Review Past Problems: Look at previous competition problems and their solutions.
- Join Study Groups: Collaborate with others to learn different approaches.
- Use Cheat Sheets: Keep a cheat sheet of common algorithms and their complexities handy.
Understanding Big O notation is not just about passing interviews; it’s about mastering the art of problem-solving in competitive programming. Big O notation is a mathematical concept used to describe the performance and complexity of an algorithm as its input size grows.
Resources for Mastering Big O
Books and Online Courses
- "Master the Coding Interview: Data Structures + Algorithms": This book is a great resource to help you understand the fundamentals of algorithms and data structures. It provides practical examples and exercises to solidify your knowledge.
- Online platforms like Coursera and Udemy offer courses specifically focused on Big O notation and algorithm analysis.
Interactive Learning Platforms
- Websites like LeetCode and HackerRank provide interactive coding challenges that help you practice your Big O skills in real-time.
- Codewars is another platform where you can solve problems and see how your solutions compare in terms of efficiency.
Practice Websites
- GeeksforGeeks: This site has a wealth of articles and problems related to Big O notation and algorithm complexity.
- Exercism: Offers coding exercises with mentor support, which can help you understand Big O in various programming languages.
Communities and Forums
- Join forums like Stack Overflow or Reddit to ask questions and share knowledge about Big O and algorithm analysis.
- Participate in coding groups on platforms like Discord or Slack to connect with others who are also preparing for coding interviews.
Cheat Sheets and Guides
- Utilize Big O cheat sheets available online for quick reference. These sheets summarize common complexities and can be a handy tool during your study sessions.
- Printable guides can also be found that outline key concepts and examples of Big O notation.
Recommended Reading
- Explore articles and blogs that focus on algorithm efficiency and Big O notation. They often provide insights and tips that can enhance your understanding.
By utilizing these resources, you can build a strong foundation in Big O notation, which is essential for success in coding interviews and software development.
Future Trends in Big O Analysis
Emerging Technologies
As technology evolves, new algorithms are being developed that can change how we think about complexity. For instance, advancements in quantum computing may lead to algorithms that operate in ways we haven’t yet imagined.
Impact of Quantum Computing
Quantum computing has the potential to drastically reduce the time complexity of certain problems. For example, algorithms that currently take exponential time could be solved in polynomial time, making previously impossible tasks feasible.
Trends in Algorithm Development
The focus is shifting towards creating algorithms that are not only efficient but also adaptable. This means algorithms that can optimize themselves based on the data they process.
Future of Coding Interviews
Coding interviews are likely to place more emphasis on understanding the real-world implications of Big O notation. Candidates may be asked to explain how their choices impact performance in practical scenarios.
Big O in New Programming Paradigms
With the rise of functional programming and other paradigms, the way we analyze algorithms may change. Understanding how these paradigms affect complexity will be crucial for developers.
Predictions for the Future
- Increased focus on real-time performance: As applications become more complex, the need for real-time analysis will grow.
- Integration of AI in algorithm design: AI could help in creating more efficient algorithms by learning from data patterns.
- Greater emphasis on space complexity: As data grows, understanding how much memory algorithms use will become more important.
In summary, the future of Big O analysis will be shaped by technological advancements and the need for more efficient algorithms. Understanding these trends will be essential for developers aiming to stay ahead in the field.
As we look ahead, understanding Big O analysis will be crucial for anyone diving into coding. This concept helps you grasp how algorithms perform, especially as data grows. If you’re eager to enhance your coding skills and prepare for interviews, visit our website today!
Wrapping Up Your Big O Journey
In summary, getting a good grasp of Big O notation is key to doing well in coding interviews. With some practice and dedication, you can become skilled at using Big O, which will boost your chances of succeeding in interviews this year and in the future. Don’t let the idea of Big O intimidate you—armed with the right knowledge and preparation, you can tackle it confidently and advance in your coding career. If you’re eager to learn more about algorithms and Big O, consider checking out resources like Grokking the Coding Interview and Grokking Dynamic Programming for Coding Interviews.
Frequently Asked Questions
What is Big O notation?
Big O notation is a way to describe how the time or space needed by an algorithm grows as the input size increases. It helps us understand how efficient an algorithm is.
Why is Big O important for coding interviews?
Interviewers ask about Big O to see if you understand how your code will perform with different amounts of data. It shows that you care about making your code efficient.
What are some common types of time complexity?
Common types include constant time (O(1)), linear time (O(n)), logarithmic time (O(log n)), quadratic time (O(n²)), and exponential time (O(2^n)).
How do you analyze the time complexity of an algorithm?
To analyze time complexity, look at how many steps the algorithm takes based on the size of the input. Focus on the biggest factors that affect performance.
What is the difference between time complexity and space complexity?
Time complexity measures how long an algorithm takes to run, while space complexity measures how much memory it uses. Both are important for efficiency.
Can you give an example of an algorithm with O(1) time complexity?
An example of O(1) time complexity is accessing an element in an array by its index. No matter how big the array is, it takes the same amount of time.
What is the worst-case scenario in Big O analysis?
The worst-case scenario is the maximum time or space an algorithm might take for any input size. It helps to ensure the algorithm performs well even in tough situations.
How can I prepare for Big O questions in interviews?
To prepare, practice problems that involve analyzing algorithms, study different types of time and space complexities, and review common data structures.
What are some common mistakes when calculating Big O?
Common mistakes include ignoring constant factors, not considering edge cases, and misinterpreting the complexity of nested loops.
Why should I care about space complexity?
Space complexity is important because using too much memory can slow down programs or cause them to crash, especially with large data sets.
How does Big O apply to data structures?
Different data structures have different time and space complexities for operations like adding, removing, or searching for items. Choosing the right structure can make a big difference.
What resources can help me learn more about Big O?
You can find helpful resources like online courses, coding practice websites, and books focused on algorithms and data structures.