Master These 15 Algorithms to Crush Your Coding Interviews
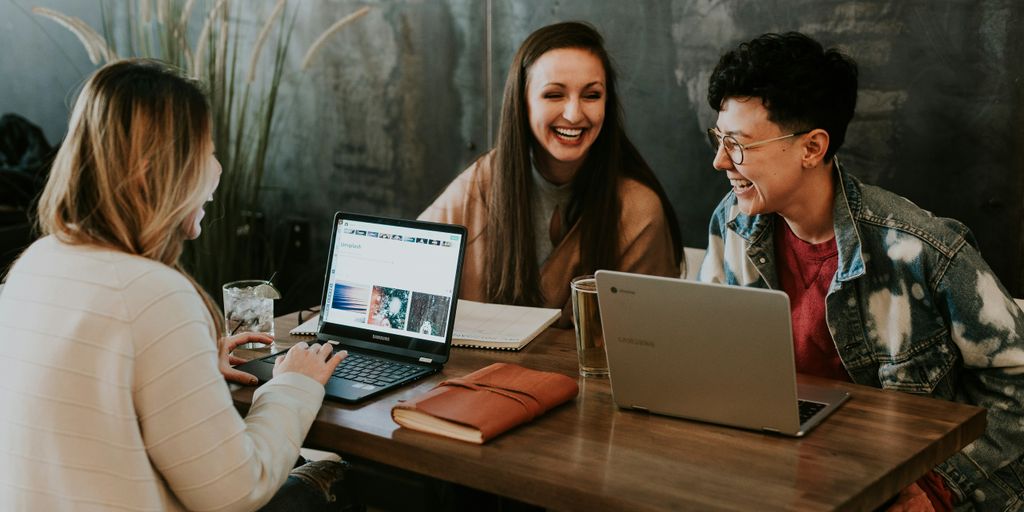
Preparing for coding interviews can be tough, but knowing the right algorithms can make all the difference. This article highlights 15 essential algorithms that will help you ace your coding interviews. From arrays to tries, mastering these will give you the confidence and skills needed to tackle any problem that comes your way.
Key Takeaways
- Understanding arrays is fundamental for solving many coding problems.
- Linked lists are crucial for handling dynamic data and memory management.
- Binary trees and graphs are essential for hierarchical data and network problems.
- Dynamic programming helps in optimizing solutions for complex problems.
- Sorting and searching algorithms are the backbone of efficient data handling.
1. Arrays
Arrays are one of the most fundamental data structures in computer science. They are used to store multiple items of the same type together. Understanding arrays is crucial for solving many coding problems.
Key Operations
- Accessing Elements: You can access any element in an array using its index. This operation is very fast, with a time complexity of O(1).
- Updating Elements: Similar to accessing, updating an element at a specific index is also O(1).
- Inserting Elements: Inserting an element at the end of an array is O(1), but inserting at any other position requires shifting elements, making it O(n).
- Deleting Elements: Deleting the last element is O(1), but deleting from any other position is O(n) due to the need to shift elements.
Common Problems
Arrays are often used in interview questions. Here is a collection of the [top 50 list of frequently asked interview questions on arrays](#e646). Problems in this article are divided into three levels: easy, medium, and hard.
Time and Space Complexity
- Time Complexity: Most operations like accessing and updating are O(1). However, inserting and deleting can be O(n) in the worst case.
- Space Complexity: Arrays are contiguous in memory, which helps in performance. The space needed is proportional to the array’s capacity.
Mastering arrays will give you a strong foundation for tackling more complex data structures and algorithms.
2. Linked Lists
Linked lists are a fundamental data structure that consists of nodes. Each node contains a value and a reference (or link) to the next node in the sequence. This structure allows for efficient insertion and deletion of elements.
Types of Linked Lists
- Singly Linked List: Each node points to the next node, and the last node points to null.
- Doubly Linked List: Each node has two references, one to the next node and another to the previous node.
- Circular Linked List: The last node points back to the first node, forming a circle.
Common Operations
- Insertion: Adding a new node to the list.
- Deletion: Removing a node from the list.
- Traversal: Accessing each node in the list to perform some operation.
- Search: Finding a node with a specific value.
Advantages and Disadvantages
Advantages:
- Dynamic size
- Ease of insertion/deletion
Disadvantages:
- No random access
- Extra memory for storing pointers
Linked lists are often used in scenarios where frequent insertion and deletion of elements are required. However, they are not suitable for scenarios requiring fast access to elements by index.
For those preparing for coding interviews, mastering linked lists is crucial. Here is the collection of the top 50 list of frequently asked interviews question on linked lists. Problems in this article are divided into three levels.
3. Binary Trees
Binary trees are a fundamental data structure in computer science, often used to represent hierarchical data. Mastering binary trees is crucial for coding interviews as they form the basis for many complex data structures and algorithms.
Types of Binary Trees
- Full Binary Tree: Every node has either 0 or 2 children.
- Complete Binary Tree: All levels are fully filled except possibly the last, which is filled from left to right.
- Perfect Binary Tree: All internal nodes have two children, and all leaves are at the same level.
- Balanced Binary Tree: The height of the tree is minimized, ensuring operations like insertion, deletion, and search are efficient.
- Binary Search Tree (BST): A binary tree where each node has a value greater than all nodes in its left subtree and less than all nodes in its right subtree.
Common Operations
- Insertion: Adding a new node to the tree while maintaining its properties.
- Deletion: Removing a node and re-arranging the tree to maintain its properties.
- Traversal: Visiting all nodes in a specific order (Inorder, Preorder, Postorder).
- Searching: Finding a node with a specific value.
Balanced Search Trees
Balanced search trees like AVL and Red-Black trees are essential for maintaining efficient search times. Red-Black trees, for instance, offer worst-case guarantees for insertion, deletion, and search times, making them valuable in time-sensitive applications.
Understanding the different types of binary trees and their operations is key to mastering common binary tree interview questions.
Applications
- Databases: B-trees and their variants are widely used in databases for indexing and quick data retrieval.
- File Systems: Many modern file systems use B-trees to manage files and directories efficiently.
- Networking: Splay trees are used in routers and caches for quick data access.
Binary trees are not just theoretical concepts but have practical applications in various fields, making them a must-know for any aspiring software engineer.
4. Graphs
Graphs are a powerful way to represent many problems in computer science. Understanding graph algorithms is crucial for coding interviews, especially for top-tier companies like FAANG.
Graph Representations
There are four basic ways to represent a graph in memory:
- Objects and pointers
- Adjacency matrix
- Adjacency list
- Adjacency map
Each representation has its own pros and cons, so it’s important to familiarize yourself with them.
Graph Traversal Algorithms
Two fundamental graph traversal algorithms are Breadth-First Search (BFS) and Depth-First Search (DFS). Knowing their computational complexity, trade-offs, and how to implement them is essential.
Advanced Graph Algorithms
For more complex problems, you might need to use advanced algorithms like Dijkstra’s for single-source shortest paths or algorithms for finding minimum spanning trees. These are often covered in courses designed for software engineers preparing for technical interviews.
When faced with a problem, always consider if a graph-based solution might be applicable before moving on to other approaches.
5. Dynamic Programming
Dynamic programming (DP) is a method for solving complex problems by breaking them down into simpler subproblems. It is particularly useful for optimization problems where you need to find the most efficient solution.
Key Concepts
- Memoization: This technique involves storing the results of expensive function calls and reusing them when the same inputs occur again. This can significantly reduce the time complexity of algorithms.
- Tabulation: Unlike memoization, which is top-down, tabulation is a bottom-up approach. It involves solving all subproblems and storing their results in a table, which is then used to solve the overall problem.
Common Problems
Here are some of the most important dynamic programming problems asked in various technical interviews:
- Longest Common Subsequence
- Longest Increasing Subsequence
- 0/1 Knapsack Problem
- Edit Distance
- Fibonacci Sequence
Dynamic programming can be tricky, but understanding the pattern can make it easier. Practice with many examples to get a solid grasp of the concept.
Tips for Mastery
- Recognize problems that can be solved using DP by identifying overlapping subproblems and optimal substructure.
- Start with a basic recursive approach, then optimize it using memoization or tabulation.
- Practice, practice, practice! The more problems you solve, the better you’ll understand the patterns involved.
6. Sorting Algorithms
Sorting algorithms are essential for coding interviews. Understanding these algorithms can help you solve many problems efficiently. Here are some key sorting algorithms you should know:
Bubble Sort
Bubble Sort is a simple comparison-based sorting algorithm. It repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. Although easy to understand, it is not efficient for large datasets.
Selection Sort
Selection Sort divides the input list into two parts: the sorted part and the unsorted part. It repeatedly selects the smallest (or largest) element from the unsorted part and moves it to the sorted part. This algorithm is also not suitable for large datasets due to its O(n^2) time complexity.
Insertion Sort
Insertion Sort builds the final sorted array one item at a time. It is much less efficient on large lists than more advanced algorithms like quicksort, heapsort, or merge sort.
Merge Sort
Merge Sort is a divide-and-conquer algorithm that divides the input array into two halves, sorts them, and then merges them. It has a time complexity of O(n log n) in the average and worst cases.
Quick Sort
Quick Sort is another divide-and-conquer algorithm. It picks an element as a pivot and partitions the array around the pivot. It has an average-case time complexity of O(n log n), but its worst-case is O(n^2).
Heap Sort
Heap Sort involves building a heap from the input data and then repeatedly extracting the maximum element from the heap and reconstructing the heap. It has a time complexity of O(n log n) but is not stable.
Radix Sort
Radix Sort processes the digits of the numbers to sort them. It is not a comparison-based algorithm and can achieve linear time complexity under certain conditions.
Counting Sort
Counting Sort counts the number of objects that have distinct key values and uses arithmetic to determine the positions of each key. It is efficient for sorting integers when the range of the numbers is not significantly greater than the number of numbers to be sorted.
Comparison of Sorting Algorithms
Algorithm | Time Complexity (Average) | Time Complexity (Worst) | Stable |
---|---|---|---|
Bubble Sort | O(n^2) | O(n^2) | Yes |
Selection Sort | O(n^2) | O(n^2) | No |
Insertion Sort | O(n^2) | O(n^2) | Yes |
Merge Sort | O(n log n) | O(n log n) | Yes |
Quick Sort | O(n log n) | O(n^2) | No |
Heap Sort | O(n log n) | O(n log n) | No |
Radix Sort | O(nk) | O(nk) | Yes |
Counting Sort | O(n+k) | O(n+k) | Yes |
Mastering these sorting algorithms will give you a strong foundation for tackling a variety of coding interview problems.
7. Searching Algorithms
Searching algorithms are essential for finding specific elements within data structures. Mastering these algorithms can significantly improve your problem-solving skills in coding interviews.
8. Hash Tables
Hash tables are a fundamental data structure that you will encounter in many coding interviews. They allow for efficient data retrieval using keys. Understanding hash tables is crucial for solving many problems quickly.
Key Operations
- Insert: Add a key-value pair to the table.
- Delete: Remove a key-value pair from the table.
- Search: Find the value associated with a key.
Common Problems
- Find whether an array is a subset of another array.
- Union and intersection of two linked lists.
- Find a pair with a given sum.
- Find an itinerary from a given list of tickets.
Collision Handling
Hash tables need a way to handle collisions, which occur when two keys hash to the same index. Common methods include:
- Chaining: Store multiple elements in a single bucket using a linked list.
- Open Addressing: Find another open slot within the table.
Mastering hash tables can significantly boost your problem-solving speed in coding interviews. Practice problems like finding the longest subarray with distinct entries to get comfortable with this data structure.
9. Recursion
Recursion is a method where the solution to a problem depends on solutions to smaller instances of the same problem. It’s a powerful tool in coding interviews, often used to solve problems that can be broken down into simpler, repetitive tasks.
Key Concepts
- Base Case: The condition under which the recursion ends. Without a base case, the function would call itself indefinitely.
- Recursive Case: The part of the function where the recursion happens. It reduces the problem into smaller instances.
When to Use Recursion
Recursion is particularly useful for problems that can be divided into similar sub-problems, such as:
- Tree Traversal
- Graph Traversal
- Sorting Algorithms like Quick Sort and Merge Sort
- Dynamic Programming problems
Tail Recursion
Tail recursion is a special kind of recursion where the recursive call is the last operation in the function. This can be optimized by the compiler to improve performance.
Steps to Solve Recursive Problems
- Identify the base case.
- Break the problem into smaller sub-problems.
- Ensure that each recursive call moves towards the base case.
- Combine the results of the sub-problems to solve the original problem.
Recursion can be tricky, but mastering it will give you a significant edge in coding interviews. Practice is key to becoming comfortable with this concept.
10. Backtracking
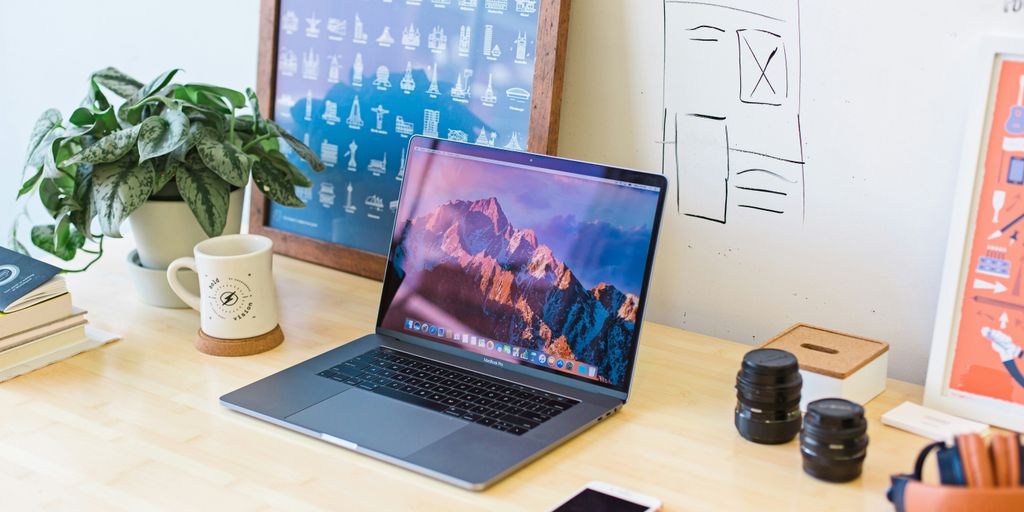
Backtracking is a powerful algorithmic technique used to solve problems incrementally, building candidates for the solutions, and abandoning a candidate as soon as it is determined that the candidate cannot possibly be completed to a valid solution.
Key Concepts
- Incremental Approach: Build the solution step by step, one piece at a time.
- Backtrack: If the current solution is not valid, backtrack to the previous step and try another option.
Common Problems
Here are some common problems that can be solved using backtracking:
- N Queens Problem
- Warnsdorff’s Algorithm
- Word Break Problem
- Remove Invalid Parentheses
- Match a Pattern
Steps to Solve a Problem Using Backtracking
- Choose a starting point.
- Make a move.
- Check if the current move leads to a solution.
- If a solution is found, return the solution.
- If not, backtrack and try the next move.
- Repeat until all moves are exhausted.
Backtracking is like a depth-first search, but with the ability to backtrack and try different paths.
Tips for Mastering Backtracking
- Practice common problems to understand the pattern.
- Use recursion to implement backtracking solutions.
- Keep track of the state to avoid redundant calculations.
Mastering backtracking can help you solve complex problems efficiently and is a valuable skill for coding interviews.
11. Greedy Algorithms
Greedy algorithms are a simple yet powerful technique used to solve optimization problems. They work by making the best choice at each step to ensure the overall solution is optimal. This method doesn’t always guarantee the best solution, but it is often effective for many problems.
Key Concepts
- Local Optimization: At each step, the algorithm makes the best possible choice without considering the global situation.
- Global Solution: The series of local optimal choices leads to a global solution.
Common Problems
Here are some classic problems where greedy algorithms shine:
- Activity Selection Problem: Choose the maximum number of activities that don’t overlap.
- Kruskal’s Minimum Spanning Tree Algorithm: Find the minimum spanning tree for a graph.
- Huffman Coding: Create an efficient prefix code for data compression.
Advantages and Disadvantages
Advantages
- Simple to implement and understand.
- Often faster than other algorithms for the same problem.
Disadvantages
- Doesn’t always produce the optimal solution.
- Requires careful problem analysis to ensure it is applicable.
Greedy algorithms are a go-to method for many optimization problems, but always verify if they are suitable for your specific case.
Conclusion
Greedy algorithms are a valuable tool in your coding arsenal. Practice problems like the activity selection problem and Kruskal’s minimum spanning tree algorithm to get a good grasp of this technique.
12. Divide and Conquer
Divide and conquer is a powerful algorithm design paradigm. It works by breaking a problem into smaller subproblems, solving each subproblem independently, and then combining their solutions to solve the original problem.
Key Steps
- Divide: Break down the original problem into smaller, more manageable subproblems.
- Conquer: Solve each subproblem independently. This step often involves recursion.
- Merge: Combine the solutions of the subproblems to form the solution to the original problem.
Examples
- Merge Sort: This algorithm sorts an array by dividing it into halves, sorting each half, and then merging the sorted halves.
- Quick Sort: This algorithm sorts an array by selecting a pivot element, partitioning the array around the pivot, and then recursively sorting the partitions.
- Binary Search: This algorithm finds an element in a sorted array by repeatedly dividing the search interval in half.
Mastering the divide and conquer algorithm can significantly improve your problem-solving skills and help you tackle complex coding interview questions with ease.
13. Bit Manipulation
Bit manipulation is a powerful technique used in programming to directly manipulate bits. These algorithms manipulate bits directly, typically using bitwise operators such as AND, OR, XOR, shift left, shift right, and complement. Understanding bitwise operations can significantly optimize your code and solve problems efficiently.
Common Bitwise Operations
- AND (&): Used to clear bits.
- OR (|): Used to set bits.
- XOR (^): Used to toggle bits.
- NOT (~): Used to invert bits.
- Shift Left (<<): Used to multiply by powers of two.
- Shift Right (>>): Used to divide by powers of two.
Practical Applications
- Counting Set Bits: Determine the number of 1s in a binary representation.
- Swapping Values: Swap two numbers without using a temporary variable.
- Checking Parity: Check if a number is even or odd.
- Finding Unique Elements: Identify unique elements in an array where every other element appears twice.
Mastering bit manipulation can give you a significant edge in coding interviews, as it allows you to solve problems that are otherwise complex with simple and efficient solutions.
14. Heaps
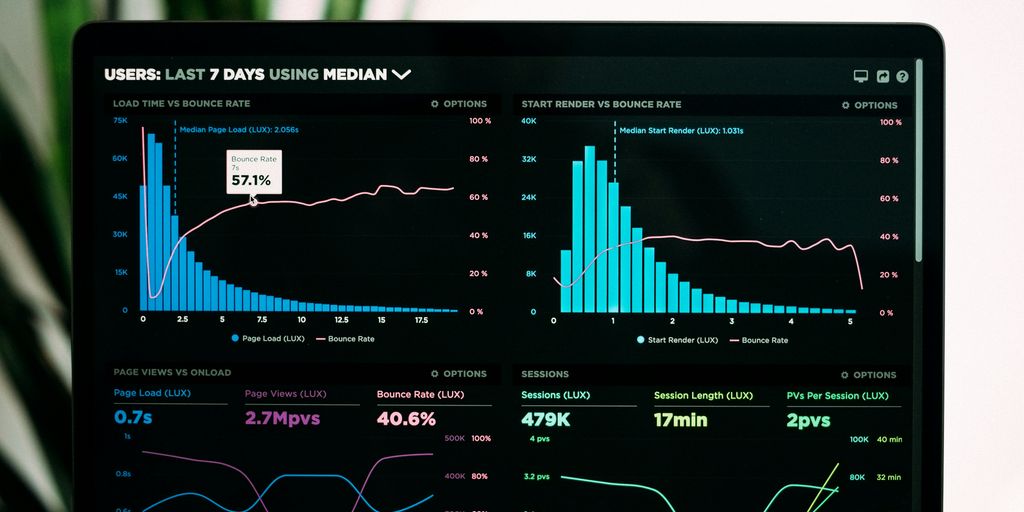
Heaps are a special type of complete binary tree that follow the heap property. This means that for every node, the value of its children is greater than or equal to its own value. Heaps are often used to implement priority queues.
Types of Heaps
- Max-Heap: In a max-heap, the parent node is always greater than or equal to the child nodes. The largest element is at the root.
- Min-Heap: In a min-heap, the parent node is always less than or equal to the child nodes. The smallest element is at the root.
Basic Operations
- Insert: Add a new element to the heap and maintain the heap property.
- Extract-Max/Min: Remove the largest (max-heap) or smallest (min-heap) element from the heap and maintain the heap property.
- Heapify: Convert an arbitrary array into a heap.
- Peek: Get the maximum (max-heap) or minimum (min-heap) element without removing it.
Applications
- Priority Queues: Heaps are commonly used to implement priority queues, where the highest (or lowest) priority element is always at the front.
- Heap Sort: A comparison-based sorting algorithm that uses a heap to sort elements.
Heaps are widely used in real-world applications, so it’s important to understand how to use them effectively. Practice until you can confidently decide when to use a min-heap or a max-heap.
15. Tries
Tries are a special type of tree used to store strings. They are particularly useful for tasks involving dynamic sets of strings. Each node in a trie represents a character of a string, and the path from the root to a node represents a prefix of the stored strings.
Key Operations
- Insert: Adding a string to the trie involves creating nodes for each character that doesn’t already exist in the trie.
- Search: To find a string, traverse the trie following the characters of the string. If you reach the end of the string and are at a terminal node, the string exists in the trie.
- Delete: Removing a string requires careful handling to ensure no other strings are affected.
Advantages
- Efficient for prefix-based searches.
- Can handle dynamic sets of strings.
Disadvantages
- Can consume a lot of memory, especially with a large set of strings.
The trie data structure is a tree-like data structure used for storing a dynamic set of strings. It is commonly used for efficient retrieval and storage of strings.
Tries are a special type of tree used to store associative data structures. They are perfect for tasks like autocomplete and spell checking. If you’re looking to master Tries and other essential algorithms, our interactive tutorials at AlgoCademy can help you get there. Start coding for free today and take the first step towards acing your coding interviews!
Conclusion
Mastering these 15 algorithms is like having a secret weapon for your coding interviews. By dedicating time to practice and understand each one, you’ll not only boost your problem-solving skills but also gain the confidence to tackle any challenge thrown your way. Remember, consistent practice is key. Spend at least an hour a day working on coding problems, and you’ll see improvement in just a few months. Use resources like LeetCode and HackerRank to test your knowledge and simulate real interview conditions. With hard work and determination, you’ll be well on your way to landing your dream job in tech. Keep pushing forward, and don’t forget to enjoy the journey of learning and growth!
Frequently Asked Questions
What is algorithmic complexity?
Algorithmic complexity measures how the performance of an algorithm changes as the size of the input increases. It’s crucial for understanding how efficient your code is.
How can I crack coding interviews easily?
Cracking coding interviews requires practice and understanding what interviewers look for. Focus on mastering data structures and algorithms, practice coding problems, and communicate your thought process clearly during the interview.
What are the most common algorithms asked in coding interviews?
Common algorithms include sorting (like QuickSort and MergeSort), searching (like Binary Search), dynamic programming problems, and data structures like arrays, linked lists, and binary trees.
Why is dynamic programming important?
Dynamic programming is key because it helps solve complex problems by breaking them down into simpler subproblems. It’s often used in optimization problems and is a common topic in coding interviews.
What is the best way to practice coding problems?
The best way to practice is by solving problems on coding platforms like LeetCode and HackerRank. Focus on a variety of problems and try to understand different patterns and techniques.
How important are communication skills in a coding interview?
Communication skills are very important. Interviewers want to see how you approach a problem and if you can explain your thought process clearly. It shows your ability to work well in a team.
What should I do if I get stuck on a problem during an interview?
If you get stuck, try to stay calm and think out loud. Break the problem into smaller parts, and discuss your approach with the interviewer. They might give you hints to guide you in the right direction.
How can I improve my problem-solving skills?
Improving problem-solving skills takes practice and patience. Work on a variety of problems, learn from your mistakes, and try to understand the underlying concepts. Consistent practice is key to improvement.