Java Mastery/ A Detailed Step-by-Step Coding Tutorial
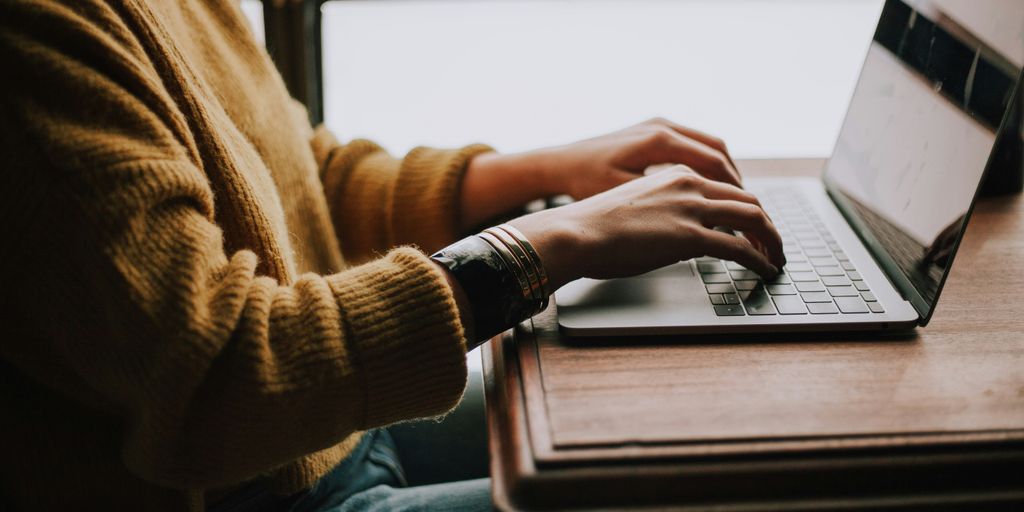
Java is a popular programming language used for building a wide range of applications, from simple desktop programs to complex enterprise systems. This tutorial aims to guide you through mastering Java, starting from the basics and moving on to more advanced topics. Whether you’re a beginner or looking to enhance your Java skills, this step-by-step guide will provide you with the knowledge and tools you need to become proficient in Java programming.
Key Takeaways
- Understand how to set up a Java development environment, including installing JDK and choosing an IDE.
- Learn the basic syntax and structure of Java, including data types, variables, and operators.
- Grasp object-oriented programming concepts such as classes, inheritance, and polymorphism.
- Explore the process of writing, compiling, and executing Java code.
- Build simple to advanced Java applications to enhance your problem-solving skills.
Setting Up Your Java Development Environment
To start your journey in Java programming, you need to set up your development environment. This involves installing the necessary tools and configuring them properly.
Installing the JDK and JRE
First, you need to install the Java Development Kit (JDK) and the Java Runtime Environment (JRE). The JDK provides the compiler (javac) and other tools needed to develop Java applications, while the JRE includes the libraries and JVM required to run Java programs. Download the latest version of the JDK from the official Oracle website and follow the installation instructions for your operating system.
Choosing the Right IDE
An Integrated Development Environment (IDE) can greatly enhance your productivity. Popular choices include Eclipse, IntelliJ IDEA, and NetBeans. These IDEs offer features like code editing, debugging, and project management. Choose one that suits your preferences and install it on your system.
Configuring Your Development Environment
After installing the JDK and your chosen IDE, you need to configure your development environment. Ensure that the JDK is correctly set up in your system’s PATH environment variable. This allows you to compile and run Java programs from the command line. Additionally, configure your IDE to use the installed JDK for compiling and running Java code.
Setting up a Java development environment involves installing the JDK, choosing and configuring an IDE, and writing your first Java program. Whether you choose Eclipse, IntelliJ IDEA, or NetBeans, make sure everything is properly configured to start coding efficiently.
Java Programming Basics: Syntax and Structure
Understanding Basic Syntax
Java’s syntax is similar to other C-based languages like C++ and C#. A Java program consists of one or more classes, each containing methods that define the program’s behavior. Here’s a simple example that prints "Hello, World!" to the console:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Let’s break down the key components of this program:
public class HelloWorld
: Declares a class named HelloWorld.public static void main(String[] args)
: Declares the main method, which is the entry point of the program.System.out.println("Hello, World!");
: Prints "Hello, World!" to the console.
Working with Data Types and Variables
Java offers a wide range of data types, including primitive types like int
, double
, and boolean
, as well as reference types like String
and arrays. Variables are used to store data, and they must be declared before they can be used. Here are some examples:
int age = 30;
double price = 9.99;
boolean isStudent = true;
String name = "John";
Using Operators and Conditional Logic
Operators in Java are used to perform operations on variables and values. Java supports various operators, including arithmetic, logical, and relational operators. Here’s an example of using conditional logic with an if-else
statement:
int x = 10;
if (x > 0) {
System.out.println("Positive");
} else if (x < 0) {
System.out.println("Negative");
} else {
System.out.println("Zero");
}
Mastering the basics of Java syntax is crucial for building a strong foundation in programming. Practice these concepts with simple coding exercises to gain confidence and proficiency.
Object-Oriented Programming Concepts
Defining Classes and Objects
In Java, a class is like a blueprint for creating objects. It defines the properties and behaviors that the objects created from the class will have. For example, consider a Car
class that has attributes like make
, model
, and year
, and a method called drive
. You can create an object of this class and use its properties and methods.
public class Car {
String make;
String model;
int year;
public void drive() {
System.out.println("Driving the " + year + " " + make + " " + model);
}
}
Car myCar = new Car();
myCar.make = "Toyota";
myCar.model = "Camry";
myCar.year = 2022;
myCar.drive();
This will output: Driving the 2022 Toyota Camry
.
Exploring Inheritance and Polymorphism
Inheritance allows a new class to take on the properties and methods of an existing class. This helps in reusing code and creating a hierarchical relationship between classes. For example, if you have a Vehicle
class, you can create a Car
class that inherits from Vehicle
.
Polymorphism allows objects to be treated as instances of their parent class rather than their actual class. This means a Car
object can be treated as a Vehicle
object, allowing for more flexible and reusable code.
Implementing Encapsulation and Abstraction
Encapsulation is about keeping the internal state of an object hidden from the outside world. This is done by making the class attributes private and providing public getter and setter methods to access and update the values. Here’s an example:
class Person {
private String name;
private int age;
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
}
public class Main {
public static void main(String[] args) {
Person person = new Person();
person.setName("John");
person.setAge(30);
System.out.println("Name: " + person.getName());
System.out.println("Age: " + person.getAge());
}
}
Abstraction is about hiding the complex implementation details and showing only the necessary features of an object. This can be achieved using abstract classes and interfaces.
The main principles of OOP in Java are encapsulation, inheritance, polymorphism, and abstraction. Encapsulation ensures that the internal state of an object is hidden from the outside world, while inheritance allows for code reuse and hierarchical relationships. Polymorphism provides flexibility, and abstraction hides complex details, making the code easier to manage and understand.
Java Step by Step Compiler and Execution Process
Writing and Saving Java Code
To start, you write your Java code in a text editor or an Integrated Development Environment (IDE). Save your file with a .java
extension. For example, if your class is named HelloWorld
, save it as HelloWorld.java
.
Compiling Java Code to Bytecode
Next, you need to compile your Java code. Use the Java compiler (javac
) to convert your .java
file into bytecode, which is saved in a .class
file. Run the following command in your terminal:
javac HelloWorld.java
This command generates a HelloWorld.class
file containing the bytecode.
Executing Bytecode in the JVM
Finally, execute the bytecode using the Java Runtime Environment (JRE). The Java Virtual Machine (JVM) reads and runs the bytecode. Use the following command:
java HelloWorld
This process ensures your Java code runs on any platform with a compatible JVM.
Understanding this compilation and execution process is crucial for debugging and optimizing your Java applications.
Building Simple Java Applications
Creating a Basic Calculator
Building a basic calculator is a great way to start with Java. This project will help you understand how to perform arithmetic operations like addition, subtraction, multiplication, and division. Focus on using variables, conditionals, loops, and methods to handle user input and print the results.
Developing a To-Do List Application
A to-do list application is another simple yet effective project. It will teach you how to create, view, edit, and delete tasks in a console-based list. This project will help you practice handling user input and managing data.
Building a Simple Text Editor
Creating a simple text editor will allow you to open, edit, save, and view text files within the application. This project will help you understand how to access files and external resources. It will also give you a chance to apply object-oriented programming principles like classes and objects.
These projects allow you to connect the Java building blocks into practical programs.
By working on these projects, you’ll gain hands-on experience and reinforce your understanding of Java basics.
Advanced Java Projects: Enhancing Problem-Solving Skills
Developing a Web Browser
Creating a web browser in Java is a challenging yet rewarding project. This project will test your understanding of Java’s capabilities and push you to learn new concepts. You’ll need to handle HTTP requests, render HTML, and manage user interactions. Key tasks include:
- Navigating web pages
- Managing bookmarks
- Viewing browsing history
Creating a 2D Game
Building a 2D game is a fun way to enhance your Java skills. This project involves creating game mechanics, graphics, and scoring systems. Focus on:
- Designing game levels
- Implementing player controls
- Managing game state and scores
Building a Mobile Application
Developing a mobile app in Java can significantly boost your problem-solving skills. You’ll learn to build user interfaces, connect to web APIs, and store data. Important aspects include:
- Creating intuitive user interfaces
- Handling data storage
- Connecting to web services
Tackling these projects will challenge you to design robust, scalable software architectures and implement advanced data structures. These projects will bring your Java abilities to an expert level.
Exploring Advanced Frameworks and Libraries
Java has a rich ecosystem of frameworks and libraries that simplify development and solve common problems. Mastering these tools is essential for any serious Java developer.
Leveraging Advanced Tools and Technologies
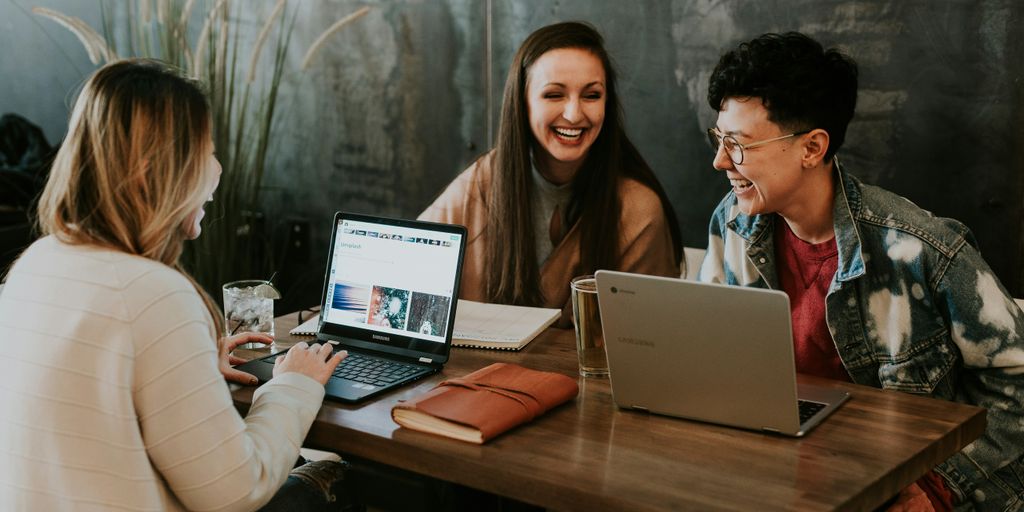
Using IntelliJ IDEA and Eclipse
To be a successful Java developer, it’s important to use the right tools. IntelliJ IDEA and Eclipse are two of the most popular Integrated Development Environments (IDEs) for Java. They offer powerful features for code editing, debugging, and refactoring. These tools can significantly boost your productivity and make coding more enjoyable.
Automating Builds with Maven and Gradle
Managing a Java project can be complex, but tools like Maven and Gradle simplify the process. They automate the build process, manage dependencies, and ensure that your project is always in a consistent state. This makes collaboration easier and helps you focus on writing code rather than managing builds.
Implementing Version Control with Git
Version control is crucial for any development project. Git is a widely-used version control system that helps you track changes, collaborate with others, and manage different versions of your code. By using Git, you can ensure that your codebase is always up-to-date and that you can easily roll back to previous versions if needed.
Embracing Software Design Patterns
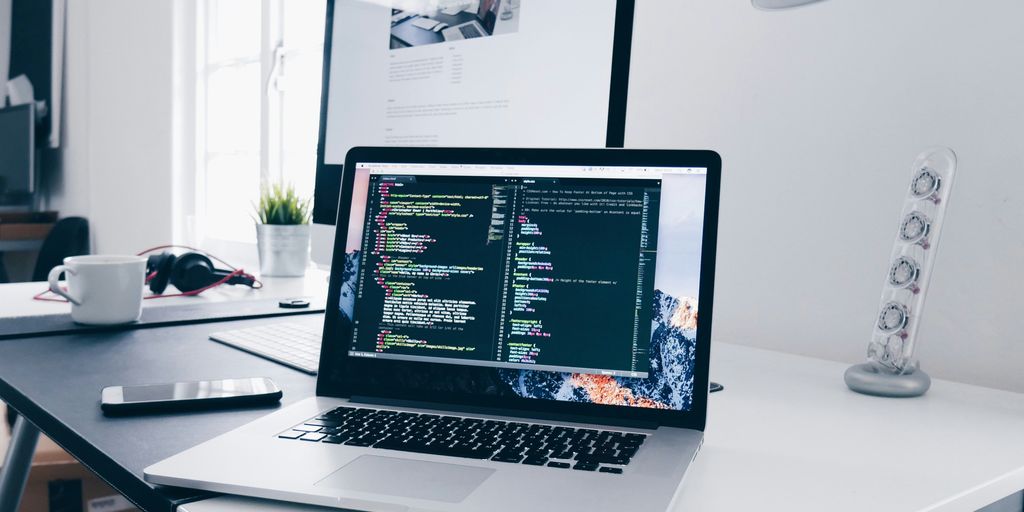
Understanding Creational Patterns
Creational patterns are all about class instantiation. They help make the creation process more adaptable and dynamic. Some common creational patterns include:
- Factory Method: Creates objects without specifying the exact class.
- Singleton: Ensures a class has only one instance and provides a global point of access.
- Builder: Constructs complex objects step by step.
Exploring Structural Patterns
Structural patterns deal with object composition. They help ensure that if one part changes, the entire structure doesn’t need to. Key structural patterns are:
- Adapter: Allows incompatible interfaces to work together.
- Composite: Composes objects into tree structures to represent part-whole hierarchies.
- Decorator: Adds responsibilities to objects dynamically.
Implementing Behavioral Patterns
Behavioral patterns focus on communication between objects. They help make the interaction flexible and reusable. Some important behavioral patterns include:
- Observer: Defines a one-to-many dependency between objects.
- Strategy: Enables selecting an algorithm’s behavior at runtime.
- Command: Encapsulates a request as an object.
Design patterns are like blueprints for solving common problems in software design. They help you create scalable, reliable, and maintainable Java programs.
By understanding and using these patterns, you can design software systems around the business domain, ensuring that the software accurately represents the domain complexities.
Joining the Java Community: Forums and Collaborative Learning
Participating in Online Forums
Engaging in online forums is a great way to exchange Java tips and collaborate with other developers. Some popular forums include:
- /r/Java: A Reddit community where Java developers share knowledge and help each other.
- Java Ranch Forums: Long-running forums with a wealth of existing Java discussions.
- Java Code Geeks: A developer community focused on the JVM ecosystem.
Joining these platforms helps you stay updated with the latest Java trends and get help when you’re stuck while coding.
Contributing to Open Source Projects
Contributing to open source projects is a fantastic way to improve your skills and give back to the community. You can start by:
- Finding a project that interests you on platforms like GitHub or GitLab.
- Reading the project’s contribution guidelines and understanding its codebase.
- Fixing bugs, adding features, or improving documentation.
By contributing, you not only enhance your coding skills but also build a portfolio that can impress potential employers.
Attending Java Meetups and Conferences
Attending meetups and conferences allows you to network with other Java enthusiasts and learn from experts. Some events to consider are:
- JavaOne: An annual conference organized by Oracle, focusing on Java and related technologies.
- Devoxx: A series of community-run conferences for developers interested in Java and other technologies.
- Local Meetups: Check platforms like Meetup.com for Java-related events in your area.
These events provide opportunities to learn about the latest developments in Java and to connect with like-minded individuals.
Engaging with the Java community through forums, open source projects, and events can significantly boost your learning and career prospects.
Joining the Java community can be a game-changer for your coding journey. Dive into forums and collaborative learning to connect with like-minded individuals and enhance your skills. Ready to take the next step? Visit our website to start coding for free and unlock a world of opportunities.
Conclusion
Mastering Java is a journey that takes time, patience, and practice. Throughout this tutorial, we’ve covered everything from setting up your development environment to building complex applications. By following the steps and practicing regularly, you will gain a solid understanding of Java programming. Remember, the key to becoming proficient is continuous learning and application. Keep experimenting with new projects, explore advanced topics, and don’t hesitate to seek help from the Java community. Your dedication and hard work will pay off as you become a confident and skilled Java developer. Happy coding!
Frequently Asked Questions
What do I need to start learning Java?
To start learning Java, you’ll need to install the JDK (Java Development Kit) and JRE (Java Runtime Environment). You also need a text editor or an IDE (Integrated Development Environment) like Eclipse or IntelliJ IDEA.
Is Java difficult to learn for beginners?
Java is considered beginner-friendly because of its clear syntax and readability. However, like any programming language, it requires practice and dedication to master.
What is the difference between JDK, JRE, and JVM?
The JDK (Java Development Kit) includes tools for developing Java applications, the JRE (Java Runtime Environment) is needed to run Java applications, and the JVM (Java Virtual Machine) executes the bytecode produced by the compiler.
Can I build mobile apps with Java?
Yes, Java is widely used for building Android mobile apps. You can use Android Studio, which is an IDE specifically designed for Android development.
What are some basic projects I can start with in Java?
You can start with simple projects like a basic calculator, a to-do list application, or a text editor. These projects will help you understand the basics of Java programming.
How can I improve my Java skills?
You can improve your Java skills by working on projects, reading Java documentation, participating in online forums, and taking advanced courses. Practice is key to mastering Java.
What is object-oriented programming (OOP) in Java?
Object-oriented programming (OOP) in Java is a programming paradigm that uses objects and classes to structure software. Key concepts include encapsulation, inheritance, polymorphism, and abstraction.
Are there any good resources for learning Java online?
Yes, there are many online resources for learning Java, including tutorials on Codecademy, courses on Udemy, and documentation on Oracle’s official website. Joining forums like StackOverflow can also be very helpful.