How to Overlay Dropdown Menu for Enhanced User Experience
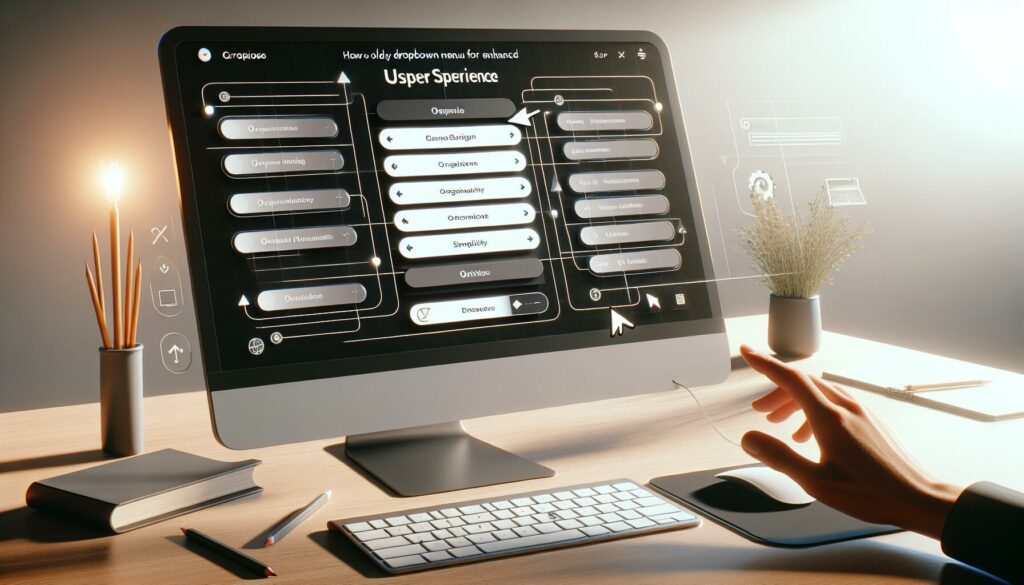
Creating a dropdown menu that enhances user experience is essential for modern web design. This article will guide you through the basics of dropdown menus, design principles, and how to implement them effectively using HTML, CSS, and JavaScript. We’ll also discuss accessibility, mobile considerations, and best practices to ensure that your dropdown menus are user-friendly and efficient.
Key Takeaways
- Dropdown menus provide a simple way to navigate websites.
- Good design principles include readability and consistency.
- CSS can be used to create dropdowns that push content down instead of overlaying it.
- JavaScript enhances dropdown menus by adding interactivity and animations.
- Accessibility features like keyboard navigation are crucial for all users.
Understanding the Basics of Dropdown Menus
What is a Dropdown Menu?
A dropdown menu is a user interface element that lets users pick an option from a list that appears when they click on a button or link. These menus act like helpful assistants when filling out forms, reducing mistakes by offering a set of pre-defined choices.
Common Uses of Dropdown Menus
Dropdown menus are widely used in various applications, including:
- Navigation bars on websites
- Forms for selecting options like country or state
- Filters in e-commerce sites to narrow down product choices
Benefits of Using Dropdown Menus
Dropdown menus offer several advantages:
- Space-saving: They keep the interface clean by hiding options until needed.
- User-friendly: They guide users in making selections, reducing errors.
- Organized: They help categorize information, making it easier to find.
Dropdown menus enhance user experience by providing a clear and organized way to navigate options. They are essential in modern web design, making interactions smoother and more intuitive.
Design Principles for Effective Dropdown Menus
Visual Hierarchy and Readability
Creating a dropdown menu that is easy to read and navigate is essential. Good design helps users find what they need quickly. Here are some tips:
- Use clear labels for each menu item.
- Ensure there is enough contrast between text and background.
- Organize items logically to guide users.
Consistency in Design
Consistency is key in web design. Users should feel familiar with how your dropdown menus work. Here are some points to consider:
- Use the same style for all dropdowns across your site.
- Keep the same color scheme and fonts.
- Ensure similar behavior for dropdowns, like how they open and close.
Responsive Design Considerations
With many users on mobile devices, your dropdown menus must work well on all screen sizes. Here are some strategies:
- Make sure dropdowns are touch-friendly with larger buttons.
- Test how menus look on different devices to ensure usability.
- Use media queries in CSS to adjust styles for various screen sizes.
A well-designed dropdown menu can significantly improve how users interact with your website.
By following these principles, you can create dropdown menus that enhance navigation and improve user experience. Remember, designing dropdown menus that improve navigation is not just about aesthetics; it’s about functionality and ease of use.
How to Overlay Dropdown Menu with CSS
Setting Up the HTML Structure
To create a dropdown menu that overlays content, you first need to set up a simple HTML structure. Here’s a basic example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="styles.css">
<title>Dropdown Menu Example</title>
</head>
<body>
<nav class="navbar">
<div class="dropdown">
<button class="dropbtn">Menu</button>
<div class="dropdown-content">
<a href="#">Link 1</a>
<a href="#">Link 2</a>
<a href="#">Link 3</a>
</div>
</div>
</nav>
<div class="content">
<h1>Welcome to Our Website</h1>
<p>This is some content below the dropdown menu.</p>
</div>
</body>
</html>
Basic CSS Styling Techniques
Now, let’s style the dropdown menu using CSS. The key here is to set the dropdown content to be displayed as a block when the button is clicked. Here’s how you can do it:
* {
box-sizing: border-box;
margin: 0;
padding: 0;
}
body {
font-family: Arial, sans-serif;
}
.navbar {
background-color: #333;
overflow: hidden;
}
.dropdown {
position: relative;
display: inline-block;
}
.dropbtn {
background-color: #4CAF50;
color: white;
padding: 16px;
font-size: 16px;
border: none;
cursor: pointer;
}
.dropdown-content {
display: none; /* Hidden by default */
position: absolute; /* Overlaying content */
background-color: #f9f9f9;
min-width: 160px;
box-shadow: 0px 8px 16px 0px rgba(0,0,0,0.2);
z-index: 1;
}
.dropdown-content a {
color: black;
padding: 12px 16px;
text-decoration: none;
display: block;
}
.dropdown-content a:hover {
background-color: #ddd;
}
.dropdown:hover .dropdown-content {
display: block;
}
Advanced CSS for Overlays
To enhance the dropdown menu, consider these advanced techniques:
- Positioning: Use
position: absolute
for the dropdown content to overlay it on top of other elements. - Display Control: The
display: none
property hides the dropdown content by default. The:hover
pseudo-class shows the dropdown when the user interacts with the button. - Styling: Basic styling ensures the dropdown looks appealing and is easy to navigate.
Remember: Creating a custom <select> dropdown with CSS can make your menus more accessible and user-friendly.
By following these steps, you can create a dropdown menu that overlays content effectively, enhancing the user experience on your website.
Summary
In summary, overlaying dropdown menus can be achieved through a combination of HTML and CSS. Here’s a quick checklist:
- Set up the HTML structure correctly.
- Apply CSS for styling and positioning.
- Use hover effects to display the dropdown content.
With these techniques, you can create a functional and visually appealing dropdown menu that improves navigation on your site.
Enhancing User Experience with JavaScript
Adding Interactivity with JavaScript
To make dropdown menus more engaging, you can use JavaScript to add interactivity. Here are some key features to consider:
- Toggle visibility: Allow users to click on the dropdown button to show or hide the menu.
- Keyboard navigation: Enable users to navigate through the dropdown items using the keyboard.
- Dynamic updates: Change the content of the dropdown based on user actions.
Handling Click Events
Handling click events is crucial for a smooth user experience. Here’s how to do it:
- Select the dropdown button using JavaScript.
- Add an event listener to listen for clicks.
- Toggle the dropdown content visibility when the button is clicked.
Animating Dropdown Transitions
Animations can improve perceived performance, making interfaces feel snappier. Here are some animation techniques:
- Fade in/out: Smoothly transition the dropdown’s visibility.
- Slide down/up: Create a sliding effect for a more dynamic appearance.
- Delay effects: Introduce a slight delay before showing the dropdown to enhance user anticipation.
Using JavaScript effectively can transform a simple dropdown into a more interactive and enjoyable experience for users.
Animation Type | Description | Example |
---|---|---|
Fade | Smoothly changes opacity | opacity: 0 to 1 |
Slide | Moves the dropdown in/out | transform: translateY |
Scale | Changes size during transition | transform: scale(0) to scale(1) |
Accessibility Considerations for Dropdown Menus
Keyboard Navigation
To ensure that everyone can use your dropdown menu, it’s important to allow keyboard navigation. Here are some tips:
- Add
tabindex
attributes to dropdown items so users can navigate using the Tab key. - Use JavaScript to handle keyboard events, allowing users to open and close the menu with the Enter or Space keys.
- Make sure users can close the dropdown with the Escape key.
Using ARIA Roles
Implementing ARIA roles can greatly improve the experience for users with screen readers. Consider the following:
- Use
role="menu"
for the dropdown container. - Assign
role="menuitem"
to each link within the dropdown. - This helps screen readers understand the structure of your menu better.
Ensuring Screen Reader Compatibility
To make your dropdown menu more accessible, keep these points in mind:
- Label your dropdowns clearly so users know what to expect.
- Ensure that the dropdown state (open or closed) is communicated to screen readers.
- Test your dropdown with various screen readers to ensure compatibility.
Accessibility is not just a feature; it’s a necessity for a better user experience.
By following these guidelines, you can create a dropdown menu that is not only functional but also accessible to all users, including those with disabilities. Remember, practical HTML, CSS, ARIA, & JavaScript tips can enhance your dropdown menu’s accessibility significantly!
Best Practices for Mobile Dropdown Menus
Touch-Friendly Design
When designing dropdown menus for mobile, make sure they are easy to tap. Here are some tips:
- Use larger buttons to make tapping easier.
- Ensure there’s enough space between menu items to avoid accidental clicks.
- Consider using icons alongside text for better clarity.
Optimizing for Different Screen Sizes
Mobile devices come in various sizes, so it’s crucial to:
- Test your dropdown on multiple devices to ensure it looks good everywhere.
- Use responsive design techniques to adjust the layout based on screen size.
- Avoid horizontal scrolling; keep the menu items within the screen width.
Testing on Various Devices
Before launching, always test your dropdown menus:
- Check for functionality on different operating systems (iOS, Android).
- Ensure that the dropdown works well in both portrait and landscape modes.
- Gather feedback from real users to identify any issues.
Remember, a well-designed dropdown menu can significantly improve user experience on mobile devices. Focus on simplicity and usability to keep users engaged.
Aspect | Best Practice |
---|---|
Button Size | At least 44px x 44px |
Spacing Between Items | Minimum 10px |
Responsive Design | Use media queries for adjustments |
Common Pitfalls and How to Avoid Them
Overcomplicating the Menu
One major mistake is making the dropdown menu too complex. Deep levels can lead to interaction issues, such as accidentally hovering over the wrong item and causing the menu to close or shift unexpectedly. To avoid this:
- Keep the menu structure simple.
- Limit the number of nested items.
- Use clear labels for each option.
Ignoring Accessibility
Another common pitfall is neglecting accessibility features. This can alienate users who rely on assistive technologies. Here are some tips to enhance accessibility:
- Ensure keyboard navigation is smooth.
- Use ARIA roles to describe the menu.
- Test with screen readers to ensure compatibility.
Poor Performance on Mobile
Many dropdown menus do not perform well on mobile devices. This can frustrate users and lead to a poor experience. To improve mobile usability:
- Design touch-friendly menus.
- Optimize for different screen sizes.
- Test on various devices to ensure functionality.
Remember, a well-designed dropdown menu should enhance user experience, not hinder it. Keeping it simple and accessible is key to success!
Case Studies of Effective Dropdown Menus
E-commerce Websites
E-commerce sites often use dropdown menus to help users find products quickly. A well-designed dropdown can significantly improve navigation. Here are some key features:
- Categorization: Products are grouped into categories for easy access.
- Search Functionality: Some dropdowns include a search bar to find specific items.
- Visual Appeal: Attractive designs that match the website’s theme enhance user experience.
Educational Platforms
Educational websites utilize dropdown menus to organize courses and resources. Here’s what makes them effective:
- Clear Labels: Each dropdown item is clearly labeled for easy understanding.
- Subcategories: Users can drill down into subcategories for more specific content.
- Accessibility: Ensuring that all users can navigate the dropdown easily is crucial.
Corporate Websites
Corporate sites often have complex structures, making dropdown menus essential. Effective features include:
- Multi-level Menus: Allowing users to navigate through various departments.
- Consistent Design: Maintaining a uniform look across all dropdowns helps users feel comfortable.
- Mobile Responsiveness: Ensuring dropdowns work well on mobile devices is vital for user engagement.
In summary, effective dropdown menus are crucial for enhancing user experience across various platforms. They should be designed with clarity, accessibility, and visual appeal in mind.
Website Type | Key Features |
---|---|
E-commerce | Categorization, Search Functionality, Visual Appeal |
Educational | Clear Labels, Subcategories, Accessibility |
Corporate | Multi-level Menus, Consistent Design, Mobile Responsiveness |
Tools and Libraries for Creating Dropdown Menus
Creating dropdown menus can be made easier with the right tools and libraries. Here are some popular options:
Popular CSS Frameworks
- Bootstrap: A widely-used framework that offers pre-designed dropdown components.
- Foundation: Another robust framework that provides customizable dropdown menus.
- Bulma: A modern CSS framework that makes it easy to create responsive dropdowns.
JavaScript Libraries
- jQuery: Simplifies DOM manipulation and event handling, making dropdowns easier to manage.
- React: A library for building user interfaces that can handle dropdowns efficiently.
- Vue.js: Another framework that allows for easy integration of dropdown menus in applications.
UI Design Tools
- Figma: A collaborative interface design tool that helps in designing dropdown menus visually.
- Adobe XD: Useful for prototyping dropdown menus and testing user interactions.
- Sketch: A vector graphics editor that is great for designing UI elements, including dropdowns.
Using the right tools can significantly enhance your workflow and the quality of your dropdown menus.
Summary Table of Tools
Tool/Library | Type | Key Feature |
---|---|---|
Bootstrap | CSS Framework | Pre-designed components |
jQuery | JavaScript Library | Simplifies event handling |
Figma | UI Design Tool | Collaborative design |
React | JavaScript Library | Efficient UI management |
Adobe XD | UI Design Tool | Prototyping and testing |
Testing and Debugging Dropdown Menus
Common Issues and Fixes
When working with dropdown menus, you may encounter several issues. Here are some common problems and their solutions:
- Dropdown not appearing: Ensure that the CSS is correctly linked and that the display property is set to
block
when active. - Items not clickable: Check for overlapping elements that may be blocking clicks.
- Inconsistent behavior across browsers: Test the dropdown in different browsers to identify any discrepancies.
Browser Compatibility
To ensure your dropdown menu works well across various browsers, consider the following:
Browser | Compatibility Status |
---|---|
Chrome | Fully Supported |
Firefox | Fully Supported |
Safari | Minor Issues |
Internet Explorer | Major Issues |
User Testing Methods
Testing with real users can provide valuable insights. Here are some effective methods:
- A/B Testing: Compare two versions of your dropdown to see which performs better.
- Surveys: Ask users for feedback on their experience with the dropdown.
- Observation: Watch users interact with the dropdown to identify any confusion or issues.
Thoroughly test the dropdown menu across different browsers and devices to ensure consistent functionality and appearance. This step is crucial for a smooth user experience.
Future Trends in Dropdown Menu Design
AI and Machine Learning Integration
The future of dropdown menus is likely to be influenced by AI and machine learning. These technologies can help create more personalized user experiences by analyzing user behavior and preferences. For example:
- Dynamic Content: Menus that adapt based on user interactions.
- Predictive Suggestions: Offering options based on previous selections.
- User Behavior Analysis: Understanding how users navigate can lead to better design choices.
Voice-Activated Menus
As voice recognition technology improves, voice-activated menus are becoming more common. This trend allows users to interact with dropdowns using voice commands, making navigation easier, especially for those with disabilities. Key points include:
- Hands-Free Navigation: Users can access menus without using their hands.
- Natural Language Processing: Menus that understand conversational commands.
- Accessibility: Enhancing usability for all users, including those with mobility challenges.
Augmented Reality Interfaces
With the rise of augmented reality (AR), dropdown menus may evolve into immersive experiences. This could involve:
- Interactive Elements: Menus that appear in a 3D space, allowing users to interact with them in a more engaging way.
- Contextual Information: Providing additional details about options in real-time.
- Enhanced User Engagement: Making navigation more fun and intuitive.
The evolution of dropdown menus reflects how menus have evolved over the years with designing, enhancing user experience significantly.
In conclusion, as technology advances, dropdown menus will likely become more interactive and user-friendly, paving the way for a better overall experience.
As we look ahead, dropdown menus are evolving to become more user-friendly and visually appealing. They are not just about functionality anymore; they are about creating a seamless experience for users. If you want to stay updated on the latest trends in web design, visit our website and start your coding journey today!
Conclusion
In summary, making a dropdown menu that pushes content down is a smart way to improve how users interact with your website. By using the HTML and CSS examples shared in this article, you can easily add this feature to your site. Always keep in mind the importance of accessibility and usability, so that everyone can navigate your site without any trouble.
Frequently Asked Questions
What is a dropdown menu?
A dropdown menu is a list that appears when you click on a button or link. It lets you choose an option from the list.
Why should I use dropdown menus?
Dropdown menus help organize content and make it easier for users to find what they need without cluttering the page.
How can I make my dropdown menu look good?
You can style your dropdown with CSS to make it visually appealing and easy to navigate.
Can I make my dropdown menu work on mobile devices?
Yes! You can design dropdown menus to be touch-friendly and adjust for different screen sizes.
What is ARIA and why is it important?
ARIA stands for Accessible Rich Internet Applications. It helps improve accessibility for users with disabilities by providing extra information about elements on the page.
How do I add interactivity to my dropdown menu?
You can use JavaScript to make your dropdown menu open and close when users click on it.
What should I avoid when designing dropdown menus?
Avoid making your dropdown too complicated, ignoring accessibility, or creating a menu that doesn’t work well on mobile.
What tools can I use to create dropdown menus?
You can use CSS frameworks, JavaScript libraries, or UI design tools to help you create effective dropdown menus.