How to Get a Line CString Assigned to a Pointer in C: A Comprehensive Guide
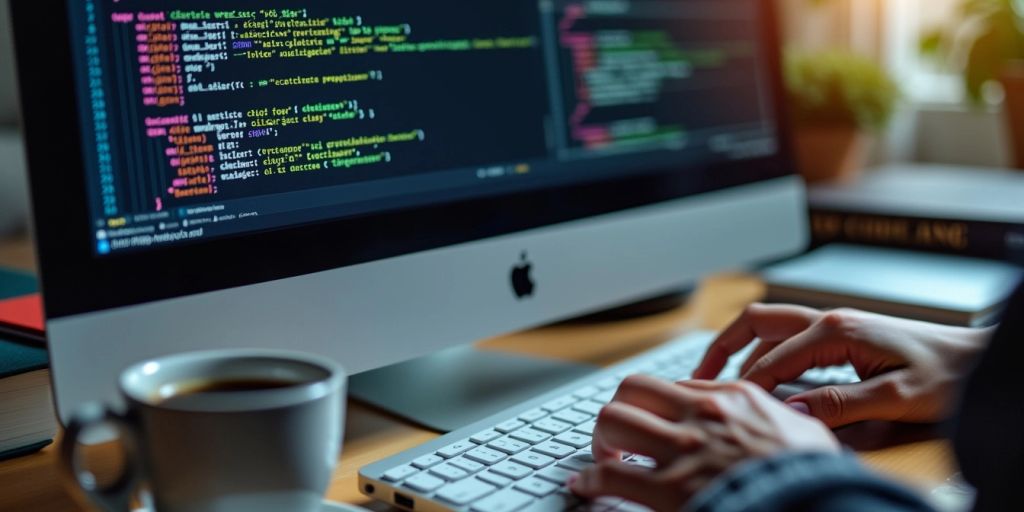
This guide will help you understand how to work with CStrings and pointers in C programming. CStrings are special string objects that simplify string handling, while pointers are essential for managing memory. This article will cover the basics and advanced techniques to effectively use CStrings with pointers, ensuring you avoid common pitfalls and improve your coding skills.
Key Takeaways
- CStrings are special string types in C that make handling text easier.
- Pointers allow you to manage memory and access CString data effectively.
- Using GetBuffer helps in modifying CString without errors.
- Always release the buffer after using GetBuffer to maintain data integrity.
- Follow best practices to avoid common mistakes when using CStrings.
Understanding CString and Pointers in C
What is CString?
A CString is a special type of string used in C++ programming, particularly in the Microsoft Foundation Class (MFC) library. It simplifies string manipulation by managing memory automatically. Unlike regular C strings, which are just arrays of characters, a CString object contains additional information, such as the length of the string and a pointer to the actual character data.
Pointers in C
Pointers are variables that store the memory address of another variable. They are essential in C programming for dynamic memory management and for creating complex data structures. Here are some key points about pointers:
- Memory Address: A pointer holds the address of a variable.
- Dereferencing: You can access the value at the address using the dereference operator (*).
- Null Pointer: A pointer that does not point to any valid memory location is called a null pointer.
Why Use CString with Pointers?
Using CString with pointers can enhance your programming efficiency. Here are some reasons:
- Automatic Memory Management: CString handles memory allocation and deallocation, reducing the risk of memory leaks.
- Ease of Use: CString provides many built-in functions for string manipulation, making it easier to work with strings.
- Unicode Support: CString is Unicode-aware, allowing for better internationalization of applications.
Using CString can greatly simplify string operations in C++, making your code cleaner and easier to maintain.
Feature | CString | C String |
---|---|---|
Memory Management | Automatic | Manual |
Unicode Support | Yes | No |
Built-in Functions | Many | Few |
Basic Syntax for Assigning CString to a Pointer
Declaring a CString
To start using a CString, you first need to declare it. Here’s how you can do it:
CString myString = "Hello, World!";
This line creates a CString named myString
and initializes it with the text "Hello, World!".
Getting a Pointer to CString
Once you have your CString, you can get a pointer to it. This is done using the GetBuffer
method. Here’s an example:
LPTSTR p = myString.GetBuffer(1024);
This line gives you a pointer p
that points to the buffer of myString
. The buffer is guaranteed to be at least 1024 characters long.
Assigning the Pointer
After obtaining the pointer, you can assign it to another variable or use it as needed. Here’s how:
CString anotherString;
anotherString = p;
This assigns the content of the pointer p
to anotherString
. Remember, always ensure that the pointer is valid before using it.
Important Note: When using pointers with CString, be careful not to modify the contents of the buffer directly, as it can lead to unexpected errors.
Summary
- Declare a CString using the
CString
keyword. - Get a pointer to the CString using
GetBuffer
. - Assign the pointer to another CString or use it as needed.
By following these steps, you can effectively manage CStrings and pointers in your C programs!
Using GetBuffer for CString Manipulation
What is GetBuffer?
The GetBuffer
function is a powerful tool in the CString class that allows you to access the internal buffer of a CString object. This function is essential for direct manipulation of the string data. It provides a pointer to the buffer, enabling you to read or modify the string directly.
Syntax and Usage
To use GetBuffer
, you can follow these steps:
- Declare a CString: Start by creating a CString object.
- Call GetBuffer: Use
GetBuffer()
to get a pointer to the internal buffer. You can specify a size if you need more space. - Manipulate the Buffer: Perform your operations on the buffer using the pointer.
- Release the Buffer: Always call
ReleaseBuffer()
after you’re done to ensure the CString object is updated correctly.
Here’s a simple example:
CString s(_T("File.ext"));
LPTSTR p = s.GetBuffer();
// Perform operations on p
s.ReleaseBuffer();
Common Mistakes to Avoid
When using GetBuffer
, keep these points in mind:
- Do not use other CString methods while the buffer is active. This can lead to unexpected behavior.
- Always ensure you call
ReleaseBuffer()
to avoid memory issues. - If you need to extend the string, specify the required size in
GetBuffer(size)
.
Remember, the integrity of the CString is only guaranteed after calling ReleaseBuffer().
By following these guidelines, you can effectively manipulate CString objects using the GetBuffer
method without running into common pitfalls.
Handling CString in Different Scenarios
CString in File Operations
When working with files, CStrings can simplify string handling. Here are some key points to remember:
- Use CString for file paths: It makes managing file names easier.
- Read and write operations: You can directly use CStrings for reading from and writing to files.
- Error handling: Always check if the file operations succeed to avoid crashes.
CString in Network Programming
CStrings are also useful in network programming. Here’s how:
- Storing URLs: Use CStrings to hold URLs for easy manipulation.
- Sending data: Convert CStrings to byte arrays when sending data over the network.
- Receiving data: Store incoming data in CStrings for easy processing.
CString in GUI Applications
In GUI applications, CStrings help manage user input and display text. Consider these tips:
- User input: Capture text from input fields using CStrings.
- Display messages: Use CStrings to format and show messages to users.
- Dynamic updates: Easily update text in controls with CStrings.
CStrings greatly simplify many operations in MFC, making string manipulation much more convenient.
Scenario | Key Benefit |
---|---|
File Operations | Simplifies file path management |
Network Programming | Easy data handling |
GUI Applications | Streamlines user interaction |
Common Errors and Debugging Tips
Memory Allocation Issues
When working with C strings and pointers, memory allocation is crucial. Here are some common issues:
- Not allocating enough memory: Always ensure you allocate enough space for your strings.
- Memory leaks: Remember to free any allocated memory to avoid leaks.
- Using uninitialized pointers: Always initialize your pointers before use.
Pointer Dereferencing Errors
Dereferencing pointers can lead to errors if not done correctly. Here are some tips:
- Check for NULL: Always check if a pointer is NULL before dereferencing it.
- Avoid dangling pointers: Ensure pointers do not point to freed memory.
- Use tools like Valgrind: This can help identify memory issues. For example, you can run your program with Valgrind using the command:
valgrind ./a.out
Debugging Techniques
Debugging can be challenging, but here are some effective strategies:
- Use GDB: The GNU Debugger can help you step through your code and find issues.
- Create small test cases: Isolate the problem by creating minimal examples that replicate the issue.
- Read compiler warnings: They often provide hints about potential problems in your code.
Remember: Debugging is a skill that improves with practice. The more you debug, the better you will become at spotting issues.
Summary Table of Common Errors
Error Type | Description | Solution |
---|---|---|
Memory Leak | Forgetting to free allocated memory | Always free memory after use |
Dereferencing NULL Pointer | Trying to access memory through a NULL pointer | Check for NULL before dereferencing |
Uninitialized Pointer | Using a pointer that hasn’t been initialized | Always initialize pointers |
Advanced Techniques for CString and Pointers
Dynamic Memory Management
Managing memory effectively is crucial when working with CStrings. Here are some key points to consider:
- Always ensure that you allocate enough memory for your CString.
- Use
GetBuffer
to safely access the underlying character array. - Remember to call
ReleaseBuffer
after modifying the CString to maintain its integrity.
Using CString with Arrays
CStrings can be used alongside arrays for more complex data handling. Here’s how:
- Declare an array of CStrings for multiple string storage.
- Use loops to populate or manipulate each CString in the array.
- Access individual CStrings using their index in the array.
Optimizing Performance
To enhance performance when using CStrings, consider the following strategies:
- Minimize unnecessary conversions between CString and other string types.
- Use
Format
instead ofsprintf
to avoid buffer overflows and improve safety. - Avoid frequent memory allocations by reusing CString objects when possible.
Effective memory management is key to preventing errors and crashes.
By mastering these advanced techniques, you can leverage the full power of CStrings in your C programming projects. Understanding how to manage memory, work with arrays, and optimize performance will make your code more efficient and reliable.
Interfacing CString with Other Data Types
CString to char* Conversion
When working with CStrings, you might need to convert them to a char*
. This is common when interfacing with functions that require standard C strings. Here’s how you can do it:
- Use the
GetBuffer
method: This method provides a pointer to the internal buffer of the CString. - Direct assignment: You can assign a CString to a
char*
using theLPCTSTR
type. - Casting: You can cast the CString to
char*
when necessary.
CString to BSTR Conversion
BSTR is a string type used in COM programming. To convert a CString to a BSTR, follow these steps:
- Use the
SysAllocString
function to allocate a BSTR. - Copy the CString content into the BSTR.
- Remember to free the BSTR after use to avoid memory leaks.
Handling Unicode with CString
CStrings can handle Unicode characters, which is essential for modern applications. Here are some tips:
- Always use the
_T()
macro when defining string literals to ensure compatibility. - Be aware of the character set your application is using (ANSI vs. Unicode).
- Use
CStringW
for wide character support if needed.
Remember: Understanding how to convert between different string types is crucial for effective C programming. This knowledge helps avoid common pitfalls in C string handling and ensures your code runs smoothly across different platforms and libraries.
Best Practices for CString and Pointers
Code Readability
- Keep your code clear: Use meaningful variable names for CStrings and pointers.
- Avoid complex expressions that can confuse readers.
- Comment on tricky parts of your code to explain your logic.
Error Handling
- Always check for null pointers before dereferencing.
- Use
CString::Format
instead ofsprintf
to avoid buffer overflows. - Handle exceptions gracefully to prevent crashes.
Performance Considerations
- Minimize unnecessary conversions between CString and other types.
- Use
GetBuffer
wisely to avoid memory issues. - Prefer stack allocation over heap allocation for better performance.
Remember, good practices lead to fewer bugs and easier maintenance. Following these guidelines will help you write better C code with CStrings and pointers.
Real-World Examples and Case Studies
Example 1: File Handling
In file handling, using CString can simplify reading and writing operations. Here’s how you can use it:
- Open a file using
fopen
. - Read data into a
CString
usingfgets
. - Process the data as needed.
- Write back to the file using
fprintf
.
This approach helps manage strings easily, especially when dealing with file paths or content.
Example 2: Network Communication
When working with network programming, CString can be used to handle messages:
- Send messages using
send()
function. - Receive messages with
recv()
function. - Store messages in a
CString
for easy manipulation.
This makes it easier to format and parse data being sent or received over the network.
Example 3: User Interface
In GUI applications, CString is often used for text display:
- Create labels and buttons with
CString
. - Update text dynamically based on user input.
- Handle events that require string manipulation.
Using CString
in this context enhances the user experience by making text management straightforward.
In real-world applications, using CString with pointers can greatly enhance code efficiency and readability.
Scenario | Benefits of Using CString |
---|---|
File Handling | Simplifies file operations |
Network Communication | Eases message handling |
User Interface | Enhances text management |
Tools and Libraries for CString Management
Popular Libraries
- MFC (Microsoft Foundation Classes): This is the primary library for working with CStrings in C++. It provides a rich set of classes for string manipulation.
- ATL (Active Template Library): Offers lightweight string handling and is useful for COM programming.
- Boost Libraries: While not specifically for CStrings, Boost provides many utilities that can enhance string handling in C++.
Useful Tools
- Visual Studio: The integrated development environment (IDE) that supports CString and MFC development.
- CMake: A tool that helps manage the build process of C++ projects, including those using CStrings.
- Valgrind: A tool for memory debugging, which can help identify memory issues when using CStrings.
Community Resources
- Forums and Online Communities: Websites like Stack Overflow and GitHub have many discussions and examples related to CString usage.
- Documentation: The official Microsoft documentation provides detailed information on CString and its methods.
- Tutorials and Blogs: Many developers share their experiences and tips on using CStrings effectively.
Using the right tools and libraries can greatly simplify your CString management.
In summary, understanding the tools and libraries available for CString management is crucial for effective programming in C++. Whether you are using MFC, ATL, or other libraries, having the right resources can make your coding experience smoother and more efficient.
Summary Table of Tools and Libraries
Tool/Library | Purpose | Notes |
---|---|---|
MFC | Primary library for CString | Rich set of classes |
ATL | Lightweight string handling | Useful for COM |
Boost | General C++ utilities | Enhances string handling |
Visual Studio | IDE for C++ development | Supports CString |
CMake | Build management | Helps with project setup |
Valgrind | Memory debugging | Identifies memory issues |
Future Trends in CString and Pointer Management
Evolving Standards
As programming languages evolve, so do their standards. The upcoming new features in C++26 are expected to enhance how we handle strings and pointers. We have a good idea of what features could be adopted for C++26 — although proposals can still be submitted until January 2025.
Impact of New C++ Features
The introduction of new features in C++ can significantly change how developers interact with CStrings. Here are some potential impacts:
- Improved memory management: New features may allow for better handling of memory allocation and deallocation.
- Enhanced safety: Features that promote safer coding practices can reduce errors related to pointers.
- Simplified syntax: New syntax could make it easier to work with CStrings and pointers, making code more readable.
Predictions for the Future
Looking ahead, we can expect:
- Increased integration with modern libraries that simplify string manipulation.
- Greater focus on performance, especially in applications that require high efficiency.
- More educational resources to help new programmers understand CString and pointer management better.
The future of CString and pointer management looks promising, with ongoing developments aimed at making programming easier and more efficient.
As we look ahead, the way we handle C strings and pointers is changing. New tools and methods are making it easier for programmers to manage memory and avoid common mistakes. If you want to stay updated on these exciting trends and improve your coding skills, visit our website today!
Conclusion
In summary, understanding how to work with CStrings in C++ is essential for effective programming. By using methods like GetBuffer and ReleaseBuffer, you can safely manage string data without running into common pitfalls. Remember, always be cautious when handling pointers and ensure you follow the rules to avoid errors. With practice, you’ll find that CStrings can greatly simplify your coding tasks, making string manipulation much easier. Keep experimenting and learning, and you’ll become proficient in using CStrings in no time!
Frequently Asked Questions
What is a CString in C?
A CString is a special type of string in C++ that makes it easier to work with text. It can hold a bunch of characters and knows how many are in it.
Why do we use pointers with CStrings?
Pointers help us access the actual data stored in a CString. They allow us to manipulate the string without copying it.
How do I get a pointer to a CString?
You can get a pointer by using the GetBuffer method. This gives you access to the string’s characters.
What should I avoid when using CStrings?
Don’t try to change the contents of a CString directly through a pointer. Always use methods like Format or ReleaseBuffer.
Can I use CStrings in file operations?
Yes! CStrings are great for handling file names and text when reading from or writing to files.
What are common mistakes when using CStrings?
A common mistake is trying to modify a CString directly through a pointer, which can cause errors.
How can I convert a CString to a regular string?
You can convert a CString to a regular string by using the LPCTSTR operator, which gives you a pointer to the string.
Are there tools to help manage CStrings?
Yes, there are libraries and tools available that can help you work with CStrings more easily.