How to Check if a Param Exists in Vue.js: A Comprehensive Guide
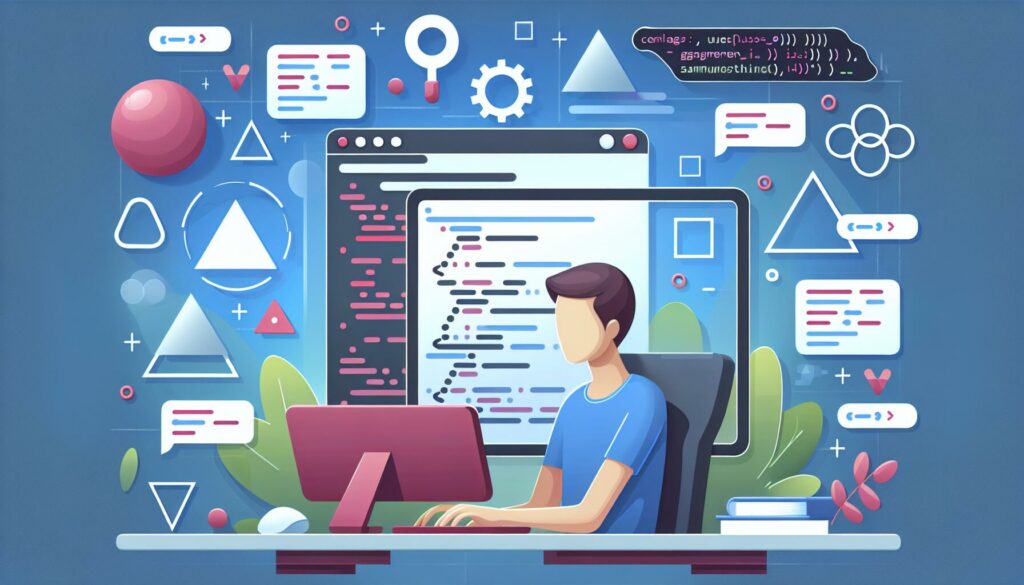
In this guide, we will explore how to check if a parameter exists in Vue.js. Understanding parameters is crucial for building dynamic applications, and this article will walk you through the essential steps to effectively manage them in your Vue projects.
Key Takeaways
- Parameters in Vue.js are essential for passing data and controlling application behavior.
- Checking for the existence of parameters can prevent errors and improve user experience.
- Vue Router offers various methods to access and check parameters easily.
- Using conditional rendering based on parameters can enhance your app’s interactivity.
- Testing for parameter existence is vital for robust application performance.
Understanding Vue.js Parameters
What Are Parameters in Vue.js?
Parameters in Vue.js are special pieces of information that can be passed through the URL. They help in making dynamic routes, allowing different content to be displayed based on the URL. For example, if you have a blog, the URL for each post can include a unique identifier, like /posts/1
, where 1
is the parameter that tells the app which post to show.
Why Are Parameters Important?
Parameters are crucial for several reasons:
- Dynamic Content: They allow your application to display different content without needing separate routes for each item.
- User Experience: Users can navigate easily to specific items, enhancing their experience.
- Data Management: They help in managing data more efficiently by using a single route to handle multiple items.
Common Use Cases for Parameters
Here are some common scenarios where parameters are used in Vue.js:
- Blog Posts: Displaying individual blog posts based on their ID.
- Product Pages: Showing details of products in an e-commerce site using product IDs.
- User Profiles: Accessing user profiles by their unique usernames or IDs.
Parameters in Vue.js are essential for creating dynamic and user-friendly applications. They allow developers to build flexible routes that can adapt to various user needs.
In summary, understanding how to work with parameters in Vue.js is key to building effective applications that can handle dynamic content efficiently. Parameters enable developers to create versatile and engaging user experiences.
Setting Up Your Vue.js Environment
Installing Vue.js
To get started with Vue.js, you need to install it on your computer. The easiest way to do this is by using npm, which is a package manager for JavaScript. Run the following command in your terminal:
npm create vue@latest
This command will prompt you to download the necessary files and guide you through setting up your project. Make sure to select options like adding Vue Router when prompted.
Creating a New Vue Project
Once you have Vue installed, you can create a new project. Here’s how:
- Open your terminal.
- Run the command mentioned above.
- Follow the prompts to name your project and choose features.
After this, a folder structure will be created for you, including files for your views and router configurations.
Setting Up Vue Router
Vue Router is essential for navigating between different pages in your application. To set it up:
- Ensure you select the option to add Vue Router during project creation.
- Check the
router/index.js
file to see the default routes created for you.
Setting up Vue Router correctly is crucial for building a smooth user experience in your app.
By following these steps, you will have a basic Vue.js environment ready for development. This setup is the foundation for building dynamic web applications using Vue.js.
Accessing Route Parameters in Vue.js
Using $route Object
In Vue.js, when you set up routing, you gain access to a special object called $route. This object contains all the information about the current route, including parameters. To access a parameter, you can use the following syntax:
{{ $route.params.username }}
This will display the value of the username
parameter in your template. Remember, the parameters are dynamic and can change based on the URL.
Using Route Props
Another way to access route parameters is through route props. This method allows you to pass parameters directly to your component. Here’s how you can set it up:
- Define your route with props enabled:
{ path: '/profile/:username', component: Profile, props: true }
- In your component, you can access the parameter directly as a prop:
props: ['username']
Dynamic Route Matching
Dynamic routes allow you to create flexible paths that can match various URL patterns. For example, you can define a route like this:
{ path: '/posts/:id', component: Post }
This route will match any URL that follows the pattern /posts/some-id
, where some-id
can be any string. The matched parameter can then be accessed using $route.params.id
.
Route Pattern | Example URL | $route.params |
---|---|---|
/product/:name |
/product/apple-watch |
{ name: "apple-watch" } |
/product/:name/comment/:comment_id |
/product/apple-watch/comment/123 |
{ name: 'apple-watch', comment_id: '123' } |
Note: Using dynamic routes can greatly simplify your code and make it easier to manage multiple paths without creating separate route definitions for each one.
By understanding how to access route parameters, you can create more dynamic and responsive applications in Vue.js.
Checking If a Parameter Exists
Using Vue Router Methods
To determine if a parameter exists in your Vue.js application, you can utilize the Vue Router methods. Here are some common methods:
- $route.params: This object contains all the route parameters. You can check if a specific parameter exists by using
this.$route.params.paramName
. - hasOwnProperty(): This method can be used to check if a parameter exists in the
$route.params
object. For example:if (this.$route.params.hasOwnProperty('id')) { // Parameter exists }
- Using a simple condition: You can also check directly if a parameter is defined:
if (this.$route.params.id) { // Parameter exists }
Conditional Rendering Based on Parameters
You can use parameters to conditionally render components or elements in your Vue.js application. Here’s how:
- v-if Directive: Use the
v-if
directive to render elements based on the existence of a parameter:<div v-if="$route.params.id">This is displayed if the ID exists.</div>
- v-else Directive: You can also use
v-else
to show an alternative when the parameter does not exist:<div v-else>No ID provided.</div>
- Dynamic Components: Render different components based on the parameter value:
<component :is="$route.params.type"></component>
Handling Missing Parameters
When a parameter is missing, it’s essential to handle it gracefully. Here are some strategies:
- Default Values: Set default values for parameters to avoid errors:
const id = this.$route.params.id || 'defaultId';
- Error Handling: Implement error handling to manage cases where parameters are not found:
if (!this.$route.params.id) { this.$router.push('/error'); }
- User Feedback: Provide feedback to users when parameters are missing:
<div v-if="!$route.params.id">Please provide an ID.</div>
Remember: Properly checking for parameters can enhance user experience and prevent unexpected errors in your application.
By following these methods, you can effectively check for the existence of parameters in your Vue.js application, ensuring a smoother user experience and better error management.
Practical Examples of Parameter Checking
Example 1: Basic Parameter Check
In Vue.js, checking if a parameter exists is straightforward. You can use the $route
object to access route parameters. Here’s how you can do it:
- Access the Parameter: Use
this.$route.params
to get the parameters. - Check for Existence: Use a simple conditional statement to check if the parameter exists.
- Handle the Result: Based on the check, you can render different components or messages.
For example:
if (this.$route.params.id) {
// Parameter exists
} else {
// Handle missing parameter
}
Example 2: Nested Routes
When dealing with nested routes, checking parameters can be a bit more complex. Here’s a simple approach:
- Use
this.$route.params
to access parameters from the parent route. - Combine it with child route parameters for a complete check.
- This is useful in applications with multiple layers of routing.
Example 3: Optional Parameters
Optional parameters can be checked similarly. Here’s how:
- Define the Route: In your router configuration, mark parameters as optional.
- Check for Existence: Use the same
$route.params
method to check if the optional parameter is present. - Provide Defaults: If the parameter is missing, you can provide a default value or redirect the user.
const userId = this.$route.params.userId || 'defaultUser';
Remember: Always handle cases where parameters might be missing to improve user experience.
By following these examples, you can effectively manage and check parameters in your Vue.js applications. Understanding how to check parameters is crucial for building dynamic and responsive applications.
Advanced Techniques for Parameter Management
Using Watchers for Parameters
In Vue.js, watchers are a powerful feature that allows you to react to changes in data. They can be particularly useful for monitoring parameters. Here’s how you can use them:
- Define a watcher on the parameter you want to track.
- Execute a function whenever the parameter changes.
- Perform actions based on the new value of the parameter.
For example:
watch: {
'$route.params.id': function(newId) {
this.fetchData(newId);
}
}
Using Computed Properties
Computed properties can also help manage parameters effectively. They allow you to derive values based on existing data. Here’s how:
- Create a computed property that returns a value based on route parameters.
- Use this computed property in your template or methods.
- This keeps your code clean and efficient.
Example:
computed: {
paramId() {
return this.$route.params.id;
}
}
Parameter Validation
Validating parameters is crucial to ensure your application behaves as expected. Here are some steps to validate parameters:
- Check for existence: Ensure the parameter is present.
- Type validation: Confirm the parameter is of the expected type.
- Range checks: If applicable, verify the parameter falls within a specific range.
By implementing these techniques, you can enhance your Vue.js application’s robustness and maintainability.
Remember, managing parameters effectively can lead to a smoother user experience and cleaner code.
Additionally, using vue refs and lifecycle methods can help with focus management, allowing you to directly access the underlying DOM nodes. This is an advanced feature that can significantly improve your application’s interactivity and responsiveness.
Testing Parameter Existence in Vue.js
Unit Testing with Jest
Unit testing is essential for ensuring that your Vue components behave as expected. Using Jest with Vue Test Utils allows you to create tests that check if parameters exist in your components. Here’s how you can set it up:
- Install Jest: Make sure you have Jest and Vue Test Utils installed in your project.
- Create a Test File: Create a new file for your component tests, typically in a
__tests__
folder. - Write Your Tests: Use the
describe
andit
functions to outline your tests. For example:describe('MyComponent.vue', () => { it('checks if parameter exists', () => { // Your test logic here }); });
Mocking Route Parameters
When testing components that rely on route parameters, you can mock these parameters to simulate different scenarios. Here’s a simple way to do it:
- Use
shallowMount
to mount your component. - Pass in mocked route parameters as props.
- Check if your component behaves correctly based on these parameters.
End-to-End Testing
For more comprehensive testing, consider using end-to-end testing tools like Cypress. This allows you to test the entire flow of your application, including how it handles parameters. Here’s a quick guide:
- Set Up Cypress: Install Cypress in your project.
- Write Tests: Create tests that navigate through your app and check if parameters are handled correctly.
- Run Tests: Execute your tests to ensure everything works as expected.
Testing is a crucial part of development. It helps catch errors early and ensures your application runs smoothly.
By following these steps, you can effectively test parameter existence in your Vue.js applications, ensuring a robust and reliable user experience.
Common Pitfalls and How to Avoid Them
Handling Undefined Parameters
When working with parameters in Vue.js, one of the most common mistakes is not checking if a parameter exists before using it. This can lead to unexpected errors in your application. Here are some tips to avoid this pitfall:
- Always validate parameters before accessing them.
- Use default values to prevent errors when parameters are missing.
- Implement error handling to manage cases where parameters are undefined.
Avoiding Over-Complexity
Another issue developers face is creating overly complex logic for parameter handling. This can make your code hard to read and maintain. To keep things simple:
- Break down complex functions into smaller, manageable pieces.
- Use clear naming conventions for your parameters.
- Stick to a consistent structure for your route definitions.
Best Practices
To ensure smooth parameter management, follow these best practices:
- Use Vue Router’s built-in methods for checking parameter existence.
- Keep your components focused on a single responsibility.
- Regularly review and refactor your code to eliminate unnecessary complexity.
Remember, a common pitfall when developing Vue apps is using stateful functions in the methods block, which can lead to hard-to-debug issues. Stay aware of this to maintain clean and efficient code!
Optimizing Performance When Checking Parameters
Lazy Loading Routes
Lazy loading routes can significantly enhance your application’s performance. By loading only the necessary components when needed, you can reduce the initial load time. Here are some benefits of lazy loading:
- Faster initial load: Only essential components are loaded first.
- Reduced bandwidth usage: Users only download what they need.
- Improved user experience: Faster interactions lead to happier users.
Code Splitting
Code splitting allows you to break your application into smaller chunks. This means that only the code required for the current view is loaded. Here’s how it can help:
- Optimized loading: Users don’t have to wait for the entire application to load.
- Better performance: Smaller files load faster.
- Easier maintenance: Smaller codebases are easier to manage.
Caching Strategies
Implementing caching strategies can greatly improve performance when checking parameters. Here are some effective methods:
- Browser caching: Store frequently accessed data in the user’s browser.
- Service workers: Use service workers to cache API responses.
- Local storage: Save data locally to reduce API calls.
By optimizing how you check parameters, you can create a smoother and faster experience for your users. Performance matters!
In summary, focusing on lazy loading, code splitting, and caching can lead to significant performance improvements in your Vue.js applications. These strategies not only enhance speed but also improve user satisfaction.
Real-World Use Cases and Applications
Building a Blog with Dynamic Routes
Creating a blog using Vue.js allows for dynamic content. Each blog post can be accessed through a unique URL, making it easy for users to navigate. Here are some key points:
- Dynamic Routing: Use Vue Router to create routes for each post.
- SEO Optimization: Ensure each post has its own metadata for better search engine visibility.
- User Interaction: Allow users to comment or share posts easily.
E-Commerce Applications
In e-commerce, Vue.js can enhance user experience significantly. Here’s how:
- Product Pages: Each product can have its own route, displaying details and reviews.
- Shopping Cart: Use Vue methods to update the cart dynamically as users add items.
- Checkout Process: Streamline the checkout with clear steps and validation.
User Profile Management
Managing user profiles is crucial for many applications. With Vue.js, you can:
- Dynamic User Profiles: Create routes for each user’s profile page.
- Edit Functionality: Allow users to update their information easily.
- Security: Implement authentication to protect user data.
In the world of web development, understanding how to manage parameters effectively can lead to better user experiences and more robust applications.
By leveraging these real-world applications, developers can create engaging and functional web experiences using Vue.js.
Explore how coding skills can change your life! From landing your dream job to mastering problem-solving, our platform offers the tools you need. Don’t wait any longer—visit our website and start coding for free today!
Conclusion
In summary, this guide has walked you through how to check if a parameter exists in Vue.js. We discussed the importance of understanding parameters and how they can affect your application. Remember, checking for parameters is crucial for ensuring your app behaves as expected. By following the steps outlined in this article, you can confidently manage parameters in your Vue.js projects. If you’re eager to learn more about Vue.js, consider exploring additional resources or courses to deepen your knowledge.
Frequently Asked Questions
What are parameters in Vue.js?
Parameters in Vue.js are pieces of information you can pass to components or routes. They help in customizing the behavior of your application.
Why do parameters matter in Vue.js?
Parameters are important because they allow you to make your app dynamic. They help in displaying different content based on user input or navigation.
How can I check if a parameter exists?
You can check if a parameter exists by using Vue Router methods like checking the $route object or using props.
What is a dynamic route in Vue.js?
A dynamic route is a route that can change based on the parameters passed to it. For example, a user profile URL can change based on the user’s ID.
What are some common mistakes when handling parameters?
Common mistakes include not checking if a parameter exists before using it, which can lead to errors, or making routes too complicated.
How can I improve performance when checking parameters?
You can improve performance by using lazy loading for routes, which means only loading the necessary components when needed.
What tools can I use for testing parameters in Vue.js?
You can use tools like Jest for unit testing and Vitest for checking the functionality of your Vue components.
What are some real-world examples of using parameters?
Real-world examples include building a blog where articles are displayed based on their ID or creating an e-commerce site where products are shown based on their category.