Dynamic Programming for Beginners: A Step-by-Step Journey to Mastery
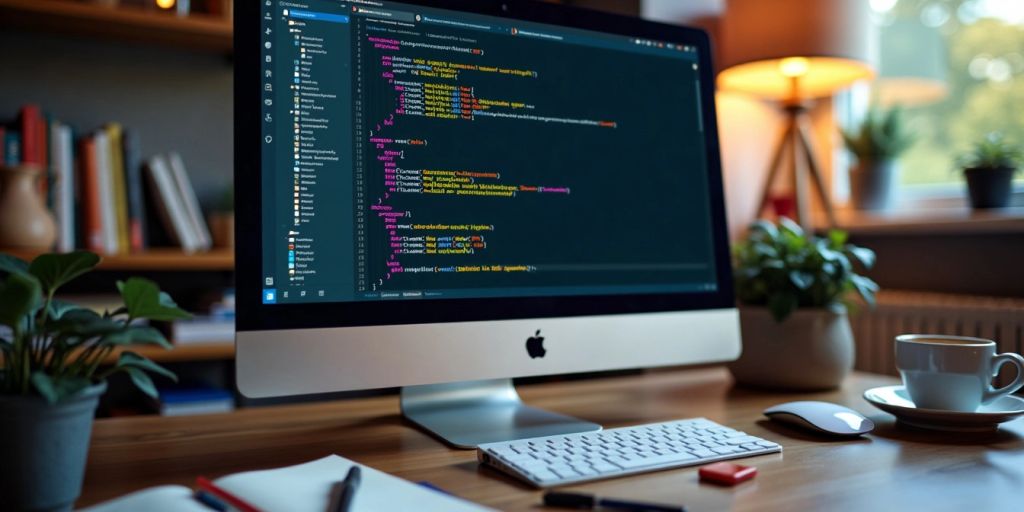
Dynamic Programming (DP) is a key concept in computer science that helps solve complex problems efficiently. It does this by breaking down problems into simpler parts, making it easier to find solutions. This article will guide beginners through the basics of dynamic programming, including its importance, techniques like recursion, memoization, and tabulation, and how to apply these concepts to real-world scenarios. By the end, you’ll have a solid understanding of dynamic programming and be ready to tackle various coding challenges.
Key Takeaways
- Dynamic programming helps solve complex problems by breaking them into smaller, manageable parts.
- Understanding recursion is essential, as it lays the groundwork for dynamic programming techniques.
- Memoization and tabulation are two key strategies for optimizing recursive solutions.
- Classic problems like the Knapsack problem and Longest Common Subsequence are fundamental to learning dynamic programming.
- Practicing with a variety of problems is crucial for mastering dynamic programming techniques.
Understanding the Basics of Dynamic Programming
What is Dynamic Programming?
Dynamic programming is a method used to solve complex problems by breaking them down into smaller, simpler subproblems. It’s particularly useful for optimization problems where the same subproblems are solved multiple times. This technique helps in saving time and resources.
The Importance of Dynamic Programming for Beginners
For beginners, understanding dynamic programming is crucial because:
- It enhances problem-solving skills.
- It provides a foundation for more advanced algorithms.
- It is widely used in competitive programming and technical interviews.
Key Concepts: Overlapping Subproblems and Optimal Substructure
Two key concepts in dynamic programming are:
- Overlapping Subproblems: This means that the problem can be broken down into smaller subproblems that are reused several times.
- Optimal Substructure: This indicates that the optimal solution to a problem can be constructed from optimal solutions of its subproblems.
Concept | Description |
---|---|
Overlapping Subproblems | Problems that can be broken down into smaller, reusable subproblems. |
Optimal Substructure | The optimal solution can be formed from optimal solutions of subproblems. |
Understanding these concepts is essential for mastering dynamic programming. They form the backbone of how we approach and solve problems effectively.
Recursion and Its Role in Dynamic Programming
Introduction to Recursion
Recursion is a method where a function calls itself to solve smaller parts of a problem. It’s like breaking a big task into smaller, easier tasks. This technique is crucial for understanding dynamic programming because it helps in solving complex problems by dividing them into simpler ones.
How Recursion Solves Complex Problems
Recursion can simplify many problems, such as:
- Factorials: Calculating the product of all positive integers up to a number.
- Fibonacci Sequence: Finding numbers in a sequence where each number is the sum of the two preceding ones.
- Tree Traversals: Navigating through tree structures in programming.
Common Recursive Problems: Factorials and Fibonacci
Here’s a quick look at how recursion works for these problems:
Problem | Recursive Formula | Example Calculation |
---|---|---|
Factorial | n! = n * (n-1)! |
5! = 5 * 4 * 3 * 2 * 1 = 120 |
Fibonacci | F(n) = F(n-1) + F(n-2) |
F(5) = F(4) + F(3) = 3 + 2 = 5 |
Recursion is a powerful tool that allows programmers to break down problems into smaller, manageable parts, making it easier to find solutions.
Understanding recursion is essential for mastering dynamic programming, as it lays the groundwork for more advanced techniques like memoization and tabulation.
Memoization: Optimizing Recursive Solutions
What is Memoization?
Memoization is a technique used to improve the performance of recursive functions. It works by storing the results of expensive function calls and reusing them when the same inputs occur again. This can significantly reduce the time complexity of algorithms that involve overlapping subproblems.
Implementing Memoization in Python
To implement memoization in Python, you can use a dictionary to store previously computed results. Here’s a simple example:
memo = {}
def fibonacci(n):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n - 1) + fibonacci(n - 2)
return memo[n]
Examples of Memoization in Real-World Problems
Memoization can be applied to various problems, including:
- Fibonacci Sequence: As shown above, it reduces the time complexity from exponential to linear.
- Dynamic Programming Problems: Such as the Knapsack problem and the Longest Common Subsequence.
- Game Theory: Where optimal strategies can be computed more efficiently.
Memoization is a powerful optimization strategy that enhances a function’s performance by storing results of previous calculations.
By using memoization, you can transform slow recursive solutions into fast ones, making it an essential tool in dynamic programming.
Tabulation: A Bottom-Up Approach
Understanding Tabulation
Tabulation is a method used in dynamic programming that builds solutions from the ground up. Instead of starting with the final solution and breaking it down, tabulation starts with the simplest subproblems and works its way up to the larger problem. This approach is often more efficient because it avoids the overhead of recursive calls.
Differences Between Tabulation and Memoization
While both tabulation and memoization are techniques used to optimize recursive solutions, they differ in their approach:
- Tabulation: Solves all subproblems iteratively and stores their results in a table.
- Memoization: Solves subproblems as needed and stores results to avoid redundant calculations.
Practical Examples of Tabulation
Here are some classic problems that can be solved using tabulation:
- Fibonacci Sequence: Calculate Fibonacci numbers using a table to store previously computed values.
- Knapsack Problem: Use a table to keep track of maximum values for different weights and items.
- Longest Common Subsequence: Build a table to find the longest sequence that appears in both strings.
Tabulation is a powerful technique that allows programmers to efficiently solve complex problems by breaking them down into simpler parts.
In summary, tabulation is a bottom-up approach that systematically solves subproblems, making it a vital tool in the dynamic programming toolkit. By understanding how to implement tabulation, beginners can tackle a variety of problems more effectively.
Classic Dynamic Programming Problems
Dynamic programming is a powerful technique used to solve complex problems by breaking them down into simpler subproblems. Here are some classic dynamic programming problems that are essential for beginners:
The Knapsack Problem
The knapsack problem involves selecting items with given weights and values to maximize the total value without exceeding a weight limit. This problem is often used in resource allocation scenarios. It teaches you how to make optimal choices under constraints.
The Longest Common Subsequence
This problem focuses on finding the longest subsequence that appears in the same order in two sequences. It’s widely applicable in fields like genetics and text comparison. Here’s a quick overview of its approach:
- Identify matching characters.
- Use a table to store results of subproblems.
- Build the solution from the table.
The Rod Cutting Problem
In the rod cutting problem, you need to determine the best way to cut a rod into pieces to maximize profit. This problem can be solved using either memoization or tabulation. Here’s a simple breakdown:
- Define the maximum profit for each length.
- Compare profits from different cuts.
- Store the best profit for each length.
Understanding these classic problems is crucial for mastering dynamic programming. They provide a solid foundation for tackling more complex challenges.
These problems are often asked in coding interviews, especially in tech companies. They help you develop a strong problem-solving mindset and improve your coding skills. Remember, classic dp problems that were asked multiple times in FAANG can be a great way to prepare for interviews!
Advanced Dynamic Programming Techniques
Bitmasking and Dynamic Programming
Bitmasking is a powerful technique used in dynamic programming to represent subsets of a set using binary numbers. This method is particularly useful for problems involving combinations or subsets. Here are some key points about bitmasking:
- Efficient Representation: Each bit in a binary number can represent whether an element is included in a subset.
- Fast Operations: Bitwise operations (AND, OR, NOT) allow for quick calculations and checks.
- Common Applications: Problems like the Traveling Salesman Problem (TSP) and subset sum can be efficiently solved using bitmasking.
K-Dimensional Dynamic Programming
K-dimensional dynamic programming extends the concept of traditional dynamic programming to multiple dimensions. This technique is useful for problems that involve multiple variables. Key aspects include:
- State Representation: Each state is represented by a tuple of dimensions, allowing for complex relationships.
- Transition Functions: These functions define how to move from one state to another, often requiring nested loops.
- Example Problems: Problems like multi-dimensional knapsack and longest common subsequence in multiple strings.
Game Theory and Dynamic Programming
Game theory often intersects with dynamic programming, especially in combinatorial games. Here’s what to know:
- Nim Game: A classic example where players take turns removing objects from piles, and the goal is to avoid being the last player.
- Optimal Strategies: Dynamic programming helps in determining the best moves by evaluating all possible outcomes.
- Complexity: Many game theory problems can be solved using dynamic programming techniques, making them more manageable.
Understanding these advanced techniques can significantly enhance your problem-solving skills in dynamic programming. Mastering these concepts opens doors to tackling complex challenges effectively.
Dynamic Programming in Different Programming Languages
Dynamic programming, often referred to as DP, is a powerful technique used in various programming languages to solve complex problems. This section will explore how dynamic programming can be implemented in three popular languages: Python, Java, and JavaScript.
Dynamic Programming in Python
- Simplicity: Python’s syntax is straightforward, making it easy to implement dynamic programming solutions.
- Libraries: Python has libraries like NumPy that can help with matrix operations, which are often used in dynamic programming.
- Example: A common problem is the Fibonacci sequence, which can be solved using both memoization and tabulation techniques.
Dynamic Programming in Java
- Strong Typing: Java’s strict type system can help catch errors early in the development process.
- Performance: Java is generally faster than Python, which can be beneficial for large-scale problems.
- Example: The Knapsack problem can be efficiently solved using dynamic programming in Java, showcasing its performance advantages.
Dynamic Programming in JavaScript
- Versatility: JavaScript can be used for both front-end and back-end development, making it a flexible choice for dynamic programming.
- Asynchronous Programming: JavaScript’s async features can be useful in certain dynamic programming scenarios.
- Example: The Longest Common Subsequence problem can be implemented in JavaScript, demonstrating its capabilities.
Understanding how to implement dynamic programming in different languages can significantly enhance your problem-solving skills. Each language has its strengths, and choosing the right one can make a big difference in efficiency and ease of use.
Language | Key Features | Example Problem |
---|---|---|
Python | Simple syntax, rich libraries | Fibonacci sequence |
Java | Strong typing, high performance | Knapsack problem |
JavaScript | Versatile, supports async programming | Longest Common Subsequence |
Real-World Applications of Dynamic Programming
Dynamic programming is a powerful tool that can be applied in various fields to solve complex problems efficiently. It helps in optimizing solutions by breaking down problems into smaller, manageable parts. Here are some key areas where dynamic programming shines:
Optimization Problems in Air Traffic Control
- Aircraft Spacing: Dynamic programming can optimize the spacing between aircraft to ensure safety and efficiency.
- Flight Scheduling: It helps in creating optimal flight schedules that minimize delays and maximize resource use.
- Route Planning: Dynamic programming aids in finding the best routes for aircraft, considering various constraints.
Financial Modeling and Stock Market Predictions
- Portfolio Optimization: It assists in determining the best mix of investments to maximize returns while minimizing risk.
- Option Pricing: Dynamic programming techniques are used to calculate the fair value of options in the stock market.
- Risk Assessment: It helps in evaluating the risk associated with different investment strategies.
Game Development and AI
- Pathfinding Algorithms: Dynamic programming is used in AI to find the shortest path in games, enhancing player experience.
- Game Strategy Optimization: It helps in determining the best strategies for game characters, making them more challenging.
- Resource Management: Dynamic programming can optimize resource allocation in games, ensuring balanced gameplay.
Dynamic programming is not just a theoretical concept; it has real-world applications that can significantly improve efficiency and decision-making in various industries.
By understanding these applications, beginners can appreciate the value of dynamic programming in solving practical problems effectively.
Common Pitfalls and How to Avoid Them
Misunderstanding the Problem Requirements
One of the biggest mistakes beginners make is not fully understanding the problem before jumping into coding. Take your time to read the problem statement carefully. Here are some tips to avoid this pitfall:
- Break down the problem into smaller parts.
- Identify the inputs and expected outputs.
- Ask yourself if you can explain the problem in your own words.
Inefficient Use of Memory and Time
Dynamic programming (DP) can be memory-intensive. If you don’t manage your resources well, your program might run slowly or even crash. Here are some strategies:
- Use memoization to store results of subproblems.
- Choose the right data structures to optimize space.
- Analyze the time complexity of your solution to ensure efficiency.
Overcomplicating Simple Problems
Sometimes, beginners try to apply complex DP techniques to problems that can be solved with simpler methods. This can lead to confusion and frustration. To avoid this:
- Start with a brute-force solution and then optimize.
- Look for patterns in simpler problems before applying DP.
- Remember that not every problem requires a DP approach.
Avoiding these common pitfalls will help you become more efficient in your coding journey. Focus on understanding the problem, managing resources wisely, and keeping your solutions simple.
By being aware of these challenges, you can navigate the world of dynamic programming more effectively and build a strong foundation for your coding skills.
Practice Problems to Master Dynamic Programming
Beginner Level Problems
- Fibonacci Sequence: Calculate the nth Fibonacci number using dynamic programming techniques.
- Factorial Calculation: Find the factorial of a number using memoization.
- Climbing Stairs: Determine how many distinct ways you can climb to the top of a staircase with n steps.
Intermediate Level Problems
- Knapsack Problem: Given weights and values of items, find the maximum value that can be carried in a knapsack of a given capacity.
- Longest Common Subsequence: Identify the longest subsequence present in two sequences.
- Rod Cutting Problem: Maximize profit by cutting a rod into pieces of given lengths.
Advanced Level Problems
- Edit Distance: Calculate the minimum number of operations required to convert one string into another.
- Matrix Chain Multiplication: Determine the most efficient way to multiply a given sequence of matrices.
- Word Break Problem: Check if a string can be segmented into a space-separated sequence of one or more dictionary words.
Practice is essential for mastering dynamic programming. The more problems you solve, the better you will understand the concepts and techniques involved.
Level | Problem Type | Example Problem |
---|---|---|
Beginner | Simple Recursion | Fibonacci Sequence |
Intermediate | Optimization | Knapsack Problem |
Advanced | Complex Algorithms | Edit Distance |
Remember, dynamic programming is a powerful tool for solving problems efficiently. By practicing these problems, you will build a strong foundation and improve your problem-solving skills.
Resources for Further Learning
Recommended Books on Dynamic Programming
- Dynamic Programming for Beginners by John Doe
- Introduction to Algorithms by Thomas H. Cormen
- Elements of Programming Interviews by Adnan Aziz
Online Courses and Tutorials
- Udemy: Offers a variety of courses on dynamic programming, including the 10 best data structures and algorithms courses [2024].
- Coursera: Provides courses from top universities that cover dynamic programming concepts.
- edX: Features professional courses that delve into algorithms and data structures.
Coding Practice Platforms
- LeetCode: Great for practicing dynamic programming problems.
- HackerRank: Offers challenges specifically focused on dynamic programming.
- CodeSignal: Provides a platform for coding challenges and competitions.
Learning dynamic programming can be challenging, but with the right resources, you can master it effectively. Practice regularly and seek help when needed!
If you’re eager to dive deeper into coding, check out our website for more resources! We offer a variety of interactive tutorials and helpful guides to boost your skills. Don’t wait—start your coding journey today!
Conclusion
In conclusion, mastering dynamic programming is a rewarding journey that opens up new ways to solve problems. By breaking down complex challenges into smaller parts, you can find efficient solutions that are both effective and elegant. This course has guided you through essential concepts, from recursion to practical applications, helping you build a strong foundation. Remember, practice is key! The more you work on problems, the better you’ll become. So, keep exploring, keep coding, and enjoy the process of learning dynamic programming!
Frequently Asked Questions
What is dynamic programming?
Dynamic programming is a method used to solve complex problems by breaking them down into smaller, easier parts. It focuses on solving each part just once and using those solutions to build up to the final answer.
Why is dynamic programming important for beginners?
Learning dynamic programming helps beginners develop strong problem-solving skills. It also prepares them for coding interviews, where these techniques are often tested.
What are overlapping subproblems?
Overlapping subproblems are smaller parts of a problem that repeat themselves. Dynamic programming takes advantage of these repetitions to save time by storing results.
How does recursion relate to dynamic programming?
Recursion is a way to solve problems by having a function call itself. Dynamic programming often uses recursion but adds a layer of optimization to avoid solving the same problem multiple times.
What is memoization?
Memoization is a technique that stores the results of expensive function calls and returns the cached result when the same inputs occur again. It helps speed up recursive solutions.
What is tabulation in dynamic programming?
Tabulation is a bottom-up approach where you solve smaller problems first and store their results in a table. You then use these results to solve larger problems.
Can you give examples of classic dynamic programming problems?
Sure! Some classic problems include the Knapsack Problem, the Longest Common Subsequence Problem, and the Rod Cutting Problem.
How can I practice dynamic programming?
You can practice dynamic programming by working on problems from online coding platforms, taking courses, or using books that focus on algorithms.