Coding with Obsolete Tools: What Punch Cards Can Teach You About Modern Programming
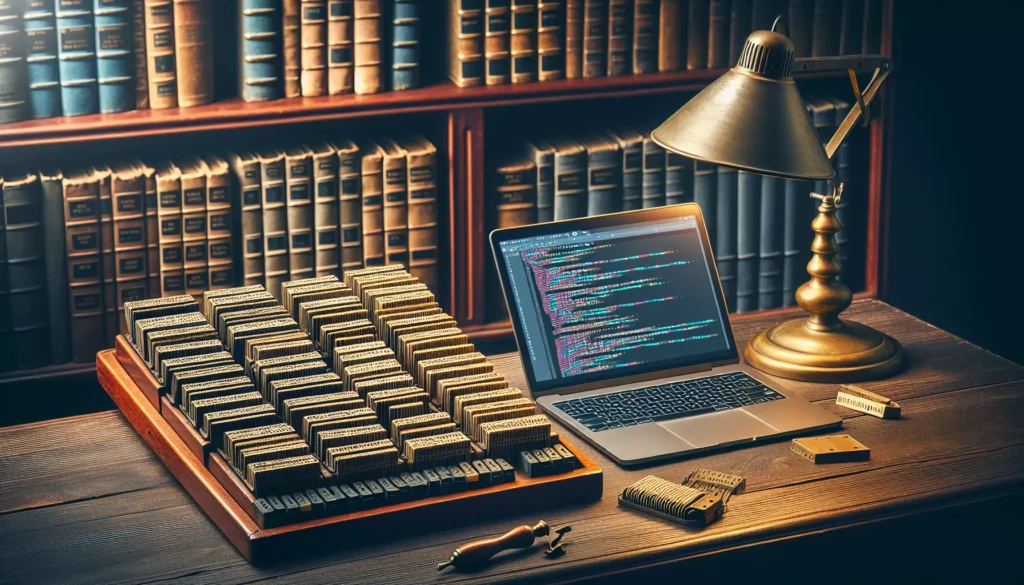
In the fast-paced world of technology, where new programming languages and frameworks seem to emerge every other day, it’s easy to forget about the roots of computer programming. One such relic of the past that played a crucial role in the evolution of coding is the punch card. While these obsolete tools may seem archaic and irrelevant in today’s digital landscape, they hold valuable lessons that can enhance our understanding of modern programming concepts and practices.
In this article, we’ll take a journey through time to explore the world of punch cards and uncover the timeless principles they embody. We’ll see how these principles relate to contemporary coding practices and how understanding them can make you a better programmer. So, let’s dive in and discover what these seemingly outdated tools can teach us about the art and science of programming.
1. The History and Mechanics of Punch Cards
Before we delve into the lessons we can learn from punch cards, it’s essential to understand what they are and how they worked. Punch cards were rectangular pieces of stiff paper used to input instructions and data into early computers. Each card typically contained 80 columns and 12 rows, allowing for a total of 960 punch positions.
The concept of using punch cards for data processing dates back to the early 19th century when Joseph Marie Jacquard invented a loom that used punched cards to control the weaving of complex patterns in textiles. However, it wasn’t until the mid-20th century that punch cards became widely used in computing.
Here’s how punch cards worked in the context of computer programming:
- Programmers would write their code on special coding sheets, which mimicked the layout of punch cards.
- Skilled operators would then use keypunch machines to create the actual punch cards, with each card representing a single line of code or a set of data.
- The cards would be fed into a card reader, which would interpret the punched holes as binary data.
- This data would then be processed by the computer, executing the program or storing the information.
Now that we have a basic understanding of punch cards, let’s explore the valuable lessons they can teach us about modern programming.
2. Precision and Attention to Detail
One of the most striking aspects of programming with punch cards was the level of precision required. A single misplaced punch could render an entire program unusable. This necessity for extreme attention to detail instilled in programmers a discipline that remains valuable in today’s coding environment.
In modern programming, while we have the luxury of integrated development environments (IDEs) with syntax highlighting and error checking, the importance of precision hasn’t diminished. Consider the following examples:
2.1. Syntax Errors
Just as a misplaced punch on a card could cause errors, a misplaced character in your code can lead to syntax errors. For instance, in Python:
def greet(name)
print(f"Hello, {name}!") # Missing colon after function definition
greet("Alice")
This code will result in a syntax error due to the missing colon after the function definition. While modern IDEs might catch this error immediately, the principle of precision remains crucial, especially when working with languages or environments that don’t provide such robust error checking.
2.2. Logic Errors
Even more insidious are logic errors, which don’t cause the program to crash but lead to incorrect results. These errors require the same level of attention to detail that punch card programming demanded. Consider this JavaScript example:
function calculateAverage(numbers) {
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
return sum / numbers.length - 1; // Incorrect logic
}
console.log(calculateAverage([1, 2, 3, 4, 5])); // Output: 2 (incorrect)
The logic error in this function (subtracting 1 from the average) would produce incorrect results, much like a misplaced punch on a card. Catching these errors requires careful review and testing, skills that were honed by punch card programmers.
3. Planning and Forethought
Programming with punch cards required extensive planning before a single card was punched. Programmers had to think through their entire algorithm, consider all possible scenarios, and plan for error handling before they could start creating their program. This need for thorough planning and forethought is a valuable lesson for modern programmers.
3.1. Algorithm Design
In today’s fast-paced development environment, it’s tempting to jump straight into coding without proper planning. However, taking the time to design your algorithm and consider edge cases can save you significant time and effort in the long run. This approach is particularly crucial when solving complex problems or optimizing for performance.
For example, when implementing a sorting algorithm, planning ahead allows you to choose the most appropriate method based on the specific requirements of your project:
def sort_data(data, size):
if size < 1000:
# Use insertion sort for small datasets
return insertion_sort(data)
elif size < 1000000:
# Use quicksort for medium-sized datasets
return quick_sort(data)
else:
# Use an external merge sort for very large datasets
return external_merge_sort(data)
This kind of strategic thinking, reminiscent of the planning required for punch card programming, can lead to more efficient and maintainable code.
3.2. Error Handling
Punch card programmers had to anticipate and plan for errors meticulously, as debugging was a time-consuming process. This principle of comprehensive error handling remains crucial in modern programming. Consider this Python example:
def divide_numbers(a, b):
try:
result = a / b
except ZeroDivisionError:
print("Error: Division by zero is not allowed.")
return None
except TypeError:
print("Error: Both inputs must be numbers.")
return None
else:
return result
print(divide_numbers(10, 2)) # Output: 5.0
print(divide_numbers(10, 0)) # Output: Error: Division by zero is not allowed.
print(divide_numbers(10, "2")) # Output: Error: Both inputs must be numbers.
This function anticipates potential errors and handles them gracefully, much like how punch card programmers had to plan for various scenarios in their code.
4. Efficiency and Resource Management
In the era of punch cards, computing resources were scarce and expensive. Programmers had to make the most of limited memory and processing power, which fostered a culture of efficiency and optimization. This mindset is still valuable today, especially when dealing with large-scale applications or resource-constrained environments.
4.1. Memory Management
While modern languages often handle memory management automatically, understanding how to use memory efficiently can lead to better-performing applications. For instance, in C++, manual memory management is still a crucial skill:
#include <iostream>
class DynamicArray {
private:
int* arr;
int size;
public:
DynamicArray(int s) : size(s) {
arr = new int[size];
}
~DynamicArray() {
delete[] arr; // Properly free allocated memory
}
// Other methods...
};
int main() {
DynamicArray* myArray = new DynamicArray(100);
// Use myArray...
delete myArray; // Properly free the object
return 0;
}
This example demonstrates proper memory allocation and deallocation, a concept that was second nature to punch card programmers who had to manage resources meticulously.
4.2. Algorithmic Efficiency
The limited resources of punch card era computers necessitated highly efficient algorithms. This focus on efficiency is still crucial today, especially when dealing with large datasets or time-critical operations. Consider this Python example of two different approaches to finding prime numbers:
import time
def is_prime_inefficient(n):
if n < 2:
return False
for i in range(2, n):
if n % i == 0:
return False
return True
def is_prime_efficient(n):
if n < 2:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
# Test the efficiency
n = 1000000
start = time.time()
result1 = is_prime_inefficient(n)
end = time.time()
print(f"Inefficient method: {end - start} seconds")
start = time.time()
result2 = is_prime_efficient(n)
end = time.time()
print(f"Efficient method: {end - start} seconds")
The efficient method, which only checks up to the square root of the number, is significantly faster, especially for large numbers. This optimization mindset, ingrained in punch card programmers, remains valuable in modern programming.
5. Documentation and Code Organization
Punch card programming required meticulous documentation and organization. Each card had to be numbered and arranged in the correct order, and programmers had to keep detailed notes about their code. This practice of thorough documentation and organization is still crucial in modern programming, especially for large-scale projects and collaborative development.
5.1. Code Comments and Documentation
While we no longer need to number our code lines like punch cards, providing clear comments and documentation is essential for maintaining and sharing code. Here’s an example of well-documented Python code:
def calculate_fibonacci(n):
"""
Calculate the nth Fibonacci number using dynamic programming.
Args:
n (int): The position of the Fibonacci number to calculate (0-indexed).
Returns:
int: The nth Fibonacci number.
Raises:
ValueError: If n is negative.
"""
if n < 0:
raise ValueError("n must be non-negative")
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
# Example usage
print(calculate_fibonacci(10)) # Output: 55
This code includes a detailed docstring explaining the function’s purpose, arguments, return value, and potential exceptions, making it easier for other developers (or your future self) to understand and use the function.
5.2. Code Structure and Modularity
The organization required for punch card programming translates well into the concept of modularity in modern programming. Breaking down complex programs into smaller, manageable modules improves readability, maintainability, and reusability. Here’s an example of how you might structure a simple program in Python:
# file: main.py
from data_processor import process_data
from report_generator import generate_report
def main():
data = [1, 2, 3, 4, 5]
processed_data = process_data(data)
report = generate_report(processed_data)
print(report)
if __name__ == "__main__":
main()
# file: data_processor.py
def process_data(data):
return [x * 2 for x in data]
# file: report_generator.py
def generate_report(data):
return f"Report: Processed data sum is {sum(data)}"
This structure, with separate files for different functionalities, reflects the organized approach that punch card programming demanded.
6. Debugging and Problem-Solving Skills
Debugging punch card programs was a challenging and time-consuming process. Programmers had to carefully review their code, often by hand, to identify and fix errors. This painstaking process honed their problem-solving skills and attention to detail. While modern debugging tools have made the process easier, the fundamental skills developed during the punch card era remain invaluable.
6.1. Systematic Debugging
The methodical approach required for debugging punch card programs translates well to modern debugging practices. Here’s an example of how you might approach debugging a Python function:
def calculate_average(numbers):
total = sum(numbers)
count = len(numbers)
average = total / count
return average
# Test the function
test_data = [1, 2, 3, 4, 5]
result = calculate_average(test_data)
print(f"Average: {result}")
# Debugging steps:
# 1. Verify input: print(f"Input: {test_data}")
# 2. Check intermediate results:
# print(f"Sum: {sum(test_data)}")
# print(f"Count: {len(test_data)}")
# 3. Validate the calculation:
# expected = sum(test_data) / len(test_data)
# print(f"Expected: {expected}, Actual: {result}")
# 4. If results don't match, examine each step of the calculation
This systematic approach to debugging, reminiscent of the careful review required for punch card programs, can help identify and fix errors efficiently.
6.2. Rubber Duck Debugging
One technique that emerged from the era of punch card programming is “rubber duck debugging.” This method involves explaining your code line by line to an inanimate object (like a rubber duck), which often helps programmers identify issues in their logic. This technique is still widely used today and can be particularly helpful when dealing with complex algorithms or stubborn bugs.
Here’s an example of how you might apply rubber duck debugging to a Python function:
def find_largest_pair_sum(numbers):
"""Find the largest sum of any two numbers in the list."""
if len(numbers) < 2:
return None
largest = max(numbers)
numbers.remove(largest)
second_largest = max(numbers)
return largest + second_largest
# Rubber duck explanation:
# 1. We define a function that takes a list of numbers.
# 2. We check if the list has at least two numbers. If not, we return None.
# 3. We find the largest number in the list.
# 4. We remove the largest number from the list.
# 5. We find the largest number in the remaining list (second largest overall).
# 6. We return the sum of the largest and second largest numbers.
# Test the function
test_data = [1, 4, 3, 2, 5]
result = find_largest_pair_sum(test_data)
print(f"Largest pair sum: {result}")
# Potential issue identified through explanation:
# The function modifies the input list, which might not be intended.
# We should consider creating a copy of the list before modifying it.
By explaining the code step by step, we’ve identified a potential issue with the function modifying the input list. This demonstrates how the rubber duck debugging technique, born from the meticulous review required in punch card programming, can help catch subtle bugs in modern code.
7. Appreciation for Abstraction and High-Level Languages
Programming with punch cards was a low-level, tedious process. Each instruction had to be explicitly defined, and even simple operations required multiple cards. This experience gives us a deep appreciation for the power of abstraction and high-level programming languages that we use today.
7.1. From Machine Code to High-Level Languages
To illustrate the evolution from punch card programming to modern high-level languages, let’s consider a simple task: adding two numbers and printing the result. Here’s how it might look in machine code (simplified for illustration):
0001 1010 0000 0001 # Load value from memory address 1 into register A
0001 1011 0000 0010 # Load value from memory address 2 into register B
0010 1100 1010 1011 # Add values in registers A and B, store result in register C
0011 1100 0000 0011 # Store value from register C into memory address 3
0100 0000 0000 0011 # Print value from memory address 3
Now, let’s see how the same operation would be performed in a high-level language like Python:
a = 5
b = 3
c = a + b
print(c)
This comparison highlights the immense power of abstraction in modern programming languages. The Python code is not only more concise but also more readable and easier to maintain.
7.2. The Power of Libraries and Frameworks
Modern programming also benefits from vast libraries and frameworks that abstract away complex operations. For example, consider the task of sorting a list of numbers. In the punch card era, programmers would have to implement the entire sorting algorithm from scratch. Today, we can use built-in functions or libraries:
import numpy as np
# Create a random array of numbers
numbers = np.random.randint(0, 100, 1000)
# Sort the array
sorted_numbers = np.sort(numbers)
print(sorted_numbers[:10]) # Print the first 10 sorted numbers
This code uses the NumPy library to generate a large array of random numbers and sort them efficiently. The sorting algorithm, which would have required hundreds of punch cards to implement, is abstracted into a single function call.
8. The Importance of Version Control
In the punch card era, version control was a physical process. Programmers had to carefully manage different versions of their program by keeping separate stacks of cards. This physical representation of code versions highlights the importance of version control, a concept that has evolved into sophisticated systems like Git in modern programming.
8.1. Basic Git Workflow
Here’s a basic Git workflow that reflects the careful version management required in punch card programming:
# Initialize a new Git repository
git init
# Create a new file and add some code
echo "print('Hello, World!')" > hello.py
# Stage the new file
git add hello.py
# Commit the changes
git commit -m "Initial commit: Add hello world program"
# Make changes to the file
echo "name = input('Enter your name: ')" >> hello.py
echo "print(f'Hello, {name}!')" >> hello.py
# Stage and commit the changes
git add hello.py
git commit -m "Update: Add user input for name"
# View the commit history
git log
This workflow demonstrates how modern version control systems allow us to track changes, revert to previous versions, and collaborate with others, much like how punch card programmers had to manage different versions of their card decks.
8.2. Branching and Merging
Git’s branching and merging capabilities take version control a step further, allowing for parallel development and experimentation:
# Create and switch to a new branch
git checkout -b feature/add-greeting
# Make changes in the new branch
echo "def greet(name):" > greet.py
echo " return f'Hello, {name}!'" >> greet.py
# Stage and commit the changes
git add greet.py
git commit -m "Add greeting function"
# Switch back to the main branch
git checkout main
# Merge the feature branch into main
git merge feature/add-greeting
# Delete the feature branch
git branch -d feature/add-greeting
This branching and merging workflow allows for more flexible and collaborative development, addressing the limitations of the linear, physical version control used in punch card programming.
Conclusion
While punch cards may seem like relics of a bygone era, the principles and practices they embody continue to shape modern programming. The lessons we can learn from this obsolete technology – precision, planning, efficiency, organization, problem-solving, and appreciation for abstraction – are timeless and valuable for programmers at all levels.
As we’ve seen through various examples, these principles manifest in different ways in modern programming languages and practices. From writing efficient algorithms to organizing code, from debugging systematically to appreciating the power of high-level abstractions, the legacy of punch card programming lives on.
By understanding and applying these lessons, we can become more effective, efficient, and thoughtful programmers. We can write cleaner, more maintainable code, solve problems more systematically, and appreciate the powerful tools and abstractions at our disposal.
So the next time you’re writing code, take a moment to reflect on the journey from punch cards to modern programming. Remember the precision required to punch those cards, the planning needed to organize them, and the problem-solving skills honed by debugging them. Let these reflections inspire you to write better code, to plan more thoroughly, and to approach problem-solving with renewed vigor.
In the end, while our tools have changed dramatically, the fundamental challenges of programming remain the same. By learning from the past, we can better navigate the present and future of programming. The lessons from punch cards, seemingly obsolete, continue to punch above their weight in shaping the art and science of coding.