A Comprehensive Guide to Learn Coding for Beginners: Your First Steps in Programming
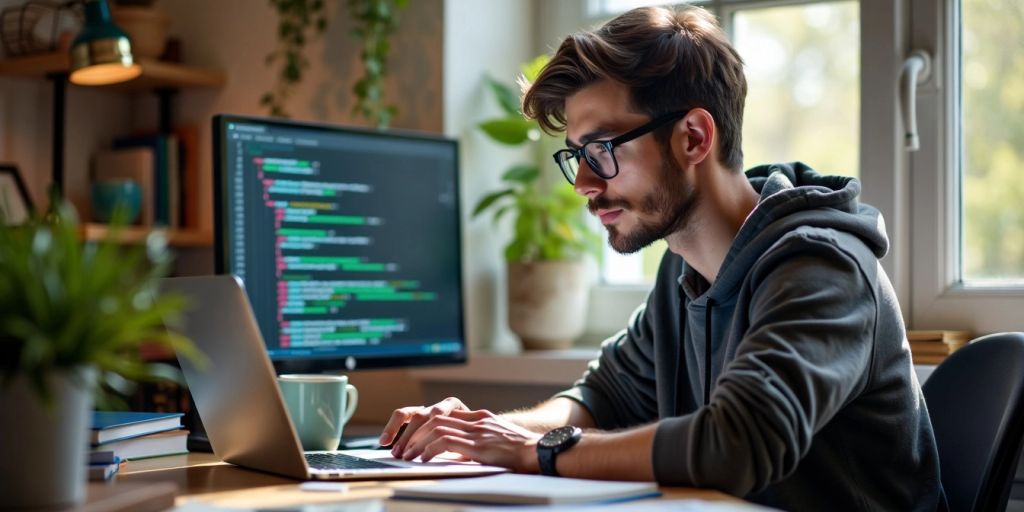
Learning to code can seem tough at first, but with the right guidance, anyone can do it! This guide is designed for beginners and will help you take your first steps in programming. You’ll discover the basics, how to choose a programming language, set up your tools, and find effective ways to learn. Let’s dive in and start your coding adventure!
Key Takeaways
- Start with the basics of programming to build a strong foundation.
- Choose a beginner-friendly programming language like Python or JavaScript.
- Set up your development environment with the right tools and software.
- Practice coding regularly and work on small projects to improve your skills.
- Join coding communities to connect with others and get support on your learning journey.
Understanding the Basics of Programming
When you begin your journey in coding, it’s crucial to grasp the fundamental concepts of programming. Programming is essentially about giving instructions to a computer to perform tasks, which helps in solving various problems. Here are some key ideas to understand:
What is Programming?
Programming refers to a technological process for telling a computer which tasks to perform in order to solve problems. It’s like writing a recipe that the computer follows to achieve a specific outcome.
Key Concepts to Grasp
- Data Types: Data can come in various forms, such as numbers, text, and true/false values. Knowing how to work with these types is essential.
- Variables: Think of variables as containers that hold data. They allow you to store and manipulate information in your programs.
- Control Structures: These help you manage the flow of your program, enabling you to make decisions or repeat tasks.
- Loops: Loops allow a set of instructions to be repeated based on a specific condition.
- Functions: Functions are like mini-programs within your program. They help break down your code into smaller, reusable parts.
- Error Handling: Learning how to handle errors is vital. It allows your program to deal with unexpected situations gracefully.
Importance of Logical Thinking
Programming requires a lot of logical thinking. You need to approach problems methodically and break them down into smaller, manageable parts. This skill is not only useful in coding but also in everyday life.
Remember: Mastering these core concepts is essential for anyone looking to become proficient in coding.
By understanding these basics, you will build a strong foundation for your programming journey.
Choosing the Right Programming Language
When starting your coding journey, one of the first choices you’ll face is what’s the best programming language to learn first? With so many options available, it’s essential to pick a language that suits your goals and interests. Here are some popular languages for beginners:
Popular Languages for Beginners
Language | Key Features | Best For |
---|---|---|
Python | Easy syntax, great for data science and web dev | Beginners, data analysis |
JavaScript | Essential for web development | Front-end development |
Java | Portable, used in Android apps | Mobile and enterprise applications |
C++ | Control over system resources | Game development, system software |
Ruby | Simple syntax, great for web development | Web applications |
Factors to Consider
- Your Goals: Think about what you want to create. Are you interested in web development, mobile apps, or data science?
- Community Support: A strong community can help you learn faster. Look for languages with active forums and resources.
- Job Opportunities: Some languages are more in demand than others. Research which languages are popular in the job market.
Pros and Cons of Each Language
- Python:
- Pros: Easy to learn, versatile.
- Cons: Slower than some other languages.
- JavaScript:
- Pros: Essential for web development, runs in all browsers.
- Cons: Can be tricky for beginners due to its asynchronous nature.
- Java:
- Pros: Strongly typed, good for large applications.
- Cons: More complex syntax compared to Python.
- C++:
- Pros: High performance, great for system-level programming.
- Cons: Steeper learning curve.
- Ruby:
- Pros: Elegant syntax, great for web apps.
- Cons: Slower performance compared to others.
Choosing the right programming language is crucial for your success in coding. Take your time to explore and find the one that resonates with you!
Setting Up Your Development Environment
Setting up your development environment is a crucial step in your coding journey. It means getting the right tools and software ready so you can start writing and running your code. Here’s how to do it:
Essential Tools and Software
- Choose a Text Editor or IDE: You need a place to write your code. Some popular options are Visual Studio Code, Sublime Text, and Atom.
- Install Necessary Software: Depending on the programming language you choose, you might need specific software. For instance, if you’re learning Python, you’ll need to install Python and a package manager like pip.
- Set Up Version Control: Using version control systems like Git is important for tracking changes in your code. You can create an account on platforms like GitHub or GitLab.
- Configure Your Environment: Customize your text editor or IDE with themes and extensions that make coding easier for you.
- Test Your Setup: After everything is installed, write a simple “Hello, World!” program to check if your environment is working correctly.
Step-by-Step Setup Guide
Here’s a quick guide to help you set up your environment:
- Confirm you have a connection to the internet.
- Make sure you have access to a command line interface (CLI) on your local machine.
- Follow the installation instructions for your chosen tools.
Common Issues and Fixes
Sometimes, you might run into problems. Here are a few common issues and how to fix them:
- Installation Errors: Double-check that you downloaded the correct version for your operating system.
- Configuration Issues: Ensure that your settings in the IDE match the requirements of the programming language you are using.
- Running Code: If your code doesn’t run, check for syntax errors or missing files.
Setting up your development environment is the first step to becoming a successful programmer. Take your time to get it right!
Learning Coding Fundamentals
Variables and Data Types
Understanding variables and data types is crucial in programming. Variables are like containers that hold information, and data types define what kind of information can be stored. Here are some common data types:
- Integer: Whole numbers (e.g., 1, 2, 3)
- Float: Decimal numbers (e.g., 1.5, 2.3)
- String: Text (e.g., "Hello, World!")
- Boolean: True or false values
Control Structures
Control structures help you manage the flow of your program. They allow you to make decisions and repeat actions. The main types include:
- If statements: Execute code based on conditions.
- Loops: Repeat code multiple times (e.g., for loops, while loops).
- Switch statements: Choose between multiple options.
Functions and Methods
Functions are blocks of code that perform specific tasks. They help keep your code organized and reusable. Here’s how to define a function:
def my_function():
print("Hello, World!")
Debugging Techniques
Debugging is the process of finding and fixing errors in your code. Here are some tips:
- Read error messages: They often tell you what went wrong.
- Use print statements: Check the values of variables at different points.
- Break down your code: Test small parts to isolate issues.
Learning coding fundamentals is essential for building a strong foundation. This knowledge will guide you as you progress in your coding journey.
Summary
Grasping these coding fundamentals is vital. They form the basis of all programming languages and will help you as you learn more complex topics. Remember, patience is key as you navigate through these concepts. Stay curious and keep practicing!
Effective Learning Strategies
Setting Realistic Goals
Setting clear and achievable goals is essential for your coding journey. Here’s how to break it down:
- Short-term goals: Focus on mastering basic syntax and writing simple programs. Aim to understand variables, loops, and conditionals within a month.
- Long-term goals: Work on complex projects and contribute to open-source communities. For example, aim to develop a web application or contribute to a GitHub repository within six months.
Consistent Practice
Practice is key to becoming a better coder. Here are some benefits of regular coding practice:
- Solving Problems: It helps you tackle different types of challenges, improving your problem-solving skills.
- Building Confidence: The more you code, the more confident you become in your abilities.
- Exploring New Concepts: Regular practice allows you to experiment with new programming ideas and techniques.
Utilizing Online Resources
There are many online platforms that can help you learn coding effectively. Here are some popular ones:
- Codecademy: Offers interactive coding lessons.
- Coursera: Provides courses from universities and colleges.
- HackerRank: Features coding challenges to sharpen your skills.
Joining Coding Communities
Engaging with others can greatly enhance your learning experience. Consider:
- Online forums: Join platforms like Stack Overflow or GitHub to ask questions and share knowledge.
- Local meetups: Attend coding events to connect with fellow learners and experienced developers.
- Open-source projects: Contributing to these projects can provide hands-on experience and help you build a portfolio.
Remember, the journey to learn coding is ongoing. Stay curious and keep exploring new technologies to improve your skills!
Working on Real-World Projects
Choosing Your First Project
Working on real-world projects is essential for learning programming. It’s where you can apply your skills to solve real problems. Start by identifying a simple issue in your daily life that you can tackle with a program. Here are some ideas:
- A to-do list app to help organize tasks.
- A basic calculator for quick math.
- A simple game to entertain yourself and others.
Project-Based Learning
Once you have a project idea, break it down into smaller tasks. Think about what features you want and how the program should function. Sketching your ideas can help you visualize the project. Here’s a simple plan:
- Identify the problem you want to solve.
- Plan the features your project will have.
- Start coding using the programming language you’re learning.
- Test your project to see if it works as expected.
- Seek feedback from friends or online communities to improve your work.
Showcasing Your Work
After completing your project, it’s important to showcase it. You can add it to your portfolio or share it on platforms like GitHub. This not only demonstrates your skills but also shows that you can solve real-world problems through programming.
Remember, every project you complete adds to your experience and confidence in coding!
Overcoming Common Challenges
Dealing with Frustration
Learning to code can be tough, and it’s normal to feel frustrated at times. Many beginners face challenges that can make them want to give up. Here are some tips to help you through:
- Break down complex problems into smaller parts.
- Take regular breaks to clear your mind.
- Remember that everyone struggles at some point.
Finding Help and Mentorship
When you hit a wall, seeking help can make a big difference. Here are some ways to find support:
- Join online forums like Stack Overflow or Reddit.
- Look for local coding meetups or workshops.
- Consider finding a mentor who can guide you through tough spots.
Staying Motivated
Staying motivated is key to learning coding. Here are some strategies:
- Set small, achievable goals to track your progress.
- Celebrate your successes, no matter how small.
- Surround yourself with supportive peers who share your interests.
Remember, every coder started as a beginner. Keep pushing through the challenges, and you’ll see improvement!
Exploring Advanced Topics
Introduction to Algorithms
Understanding algorithms is crucial for solving problems efficiently. Algorithms are step-by-step procedures for calculations, data processing, and automated reasoning tasks. They help in optimizing solutions and improving performance in coding.
Data Structures
Data structures are ways to organize and store data. Here are some common types:
- Arrays: A collection of items stored at contiguous memory locations.
- Linked Lists: A sequence of elements where each element points to the next.
- Trees: A hierarchical structure with nodes connected by edges.
- Graphs: A collection of nodes connected by edges, useful for representing networks.
Data Structure | Description | Use Cases |
---|---|---|
Array | Fixed-size collection of elements | Storing lists of items |
Linked List | Dynamic size, easy to insert/delete | Implementing stacks/queues |
Tree | Hierarchical data representation | File systems, databases |
Graph | Network of nodes | Social networks, routing |
Object-Oriented Programming
Object-oriented programming (OOP) is a programming paradigm based on the concept of "objects". Objects can contain data and code. Key concepts include:
- Classes: Blueprints for creating objects.
- Inheritance: Mechanism to create a new class from an existing class.
- Encapsulation: Hiding the internal state of an object.
- Polymorphism: Ability to present the same interface for different data types.
Conclusion
Exploring these advanced topics will deepen your understanding of programming. Mastering these concepts can significantly enhance your coding skills and prepare you for more complex challenges in the future.
Leveraging Free Online Resources
Top Websites and Platforms
There are many great free online platforms that offer interactive coding lessons for beginners. Here are some of the best options:
- Codecademy: Offers free courses in languages like Python, Java, and JavaScript. The interactive format makes it easy to practice.
- Khan Academy: Focuses on web development basics using HTML, CSS, and JavaScript through video tutorials and challenges.
- freeCodeCamp: An open-source community with self-paced courses and projects, allowing you to earn certifications as you learn.
- Scrimba: Provides interactive screencast tutorials where you can code alongside the instructor.
Interactive Tutorials
One of the best ways to learn coding is through hands-on practice. Websites like W3Schools offer beginner-friendly documentation and tutorials for languages such as:
Language | Description |
---|---|
HTML | Structure of web pages |
CSS | Styling web pages |
JavaScript | Adding interactivity to web pages |
SQL | Managing databases |
Python | General-purpose programming |
Coding Challenges and Competitions
Participating in coding challenges can enhance your skills. Websites like HackerRank and LeetCode provide a platform for practicing coding problems. These challenges help you develop problem-solving skills and apply what you’ve learned.
With so many free resources available, you can start learning coding without spending any money. Explore the top 100+ websites to learn to code for free and find the ones that suit you best!
Joining Programming Communities
Benefits of Community Engagement
Joining programming communities can be a game-changer for beginners. These groups provide support and resources that can help you grow as a coder. Here are some key benefits:
- Learning from Others: You can gain insights into different programming techniques and best practices by participating in discussions.
- Getting Help: If you’re stuck, community members are often willing to offer guidance and solutions.
- Networking Opportunities: Connecting with other programmers can lead to collaboration, mentorship, and job prospects.
- Staying Motivated: Being part of a community keeps you accountable and motivated in your learning journey.
Popular Coding Forums
Here are some popular coding communities to consider:
Community Name | Description |
---|---|
Stack Overflow | A forum for asking programming questions. |
GitHub | A platform for open-source projects and collaboration. |
Women Who Code | A nonprofit supporting women in tech careers. |
r/learnprogramming | A subreddit for beginner coders. |
Engaging in these communities can help you stay updated with the latest programming trends and resources.
Tips for Engaging in Communities
To make the most of your experience, consider these tips:
- Respect Others: Always treat community members with respect.
- Contribute Positively: Share your knowledge and help others when you can.
- Follow Community Guidelines: Familiarize yourself with the rules of each community.
- Be Open to Feedback: Embrace constructive criticism to improve your skills.
Setting and Achieving Your Coding Goals
Short-Term vs Long-Term Goals
Setting goals is crucial in your coding journey. Start with small, achievable goals that can lead to bigger ones. Here’s how you can break it down:
- Short-Term Goals: These can be daily or weekly tasks, like completing a tutorial or coding a simple project.
- Long-Term Goals: Think about what you want to achieve in a year or more, such as building a full application or getting a job in tech.
Tracking Your Progress
Keeping track of your progress helps you stay motivated. You can use a simple table to monitor your achievements:
Date | Goal | Status |
---|---|---|
2023-10-01 | Complete Python Basics | ✅ Completed |
2023-10-15 | Build a Simple App | ⏳ In Progress |
2023-11-01 | Join a Coding Community | ❌ Not Started |
Celebrating Milestones
Don’t forget to celebrate your achievements! Recognizing your progress can boost your motivation. Here are some ways to celebrate:
- Share your success with friends or family.
- Treat yourself to something nice.
- Reflect on how far you’ve come.
Remember, every small step counts. As you work through your coding journey, this roadmap will give you a complete guideline to build a strong coding habit and to achieve your goal as a software developer.
By setting clear goals and tracking your progress, you’ll find it easier to stay focused and motivated on your coding adventure!
Setting goals for your coding journey is the first step to success. Whether you want to ace your coding interviews or simply learn a new programming language, having clear objectives can guide your progress. Don’t wait any longer—visit our website to start coding for free and take the first step toward achieving your dreams!
Conclusion: Your Coding Journey Begins Here
Learning to code is an exciting adventure that can lead to many new opportunities in our tech-focused world. By following the steps in this guide, you’ve made great progress toward becoming a skilled programmer. Remember, practice and patience are key. Don’t rush; take your time to understand each concept. As you move forward, keep trying new technologies, work on projects, and connect with others in the coding community. Setting small, realistic goals will help you stay motivated and celebrate your achievements, no matter how small they seem. With hard work and determination, you can reach your coding dreams. So, embrace the journey, stay curious, and keep learning. Good luck, and happy coding!
Frequently Asked Questions
What is the best way to start learning to code?
To begin learning coding, focus on understanding the basics first. Pick a beginner-friendly language like Python or JavaScript, and use online resources or coding platforms to guide you.
How long does it usually take to learn coding?
The time it takes to learn coding varies. With regular practice, you can start creating simple projects in a few months.
Can I learn coding without any prior knowledge?
Absolutely! Many people start from scratch. Just be patient and stick to learning the fundamentals.
What programming language should I choose as a beginner?
Languages like Python, JavaScript, and Ruby are great for beginners because they are easier to understand and widely used.
How can I stay motivated while learning to code?
Set small, achievable goals and celebrate your progress. Joining a coding community can also help keep you motivated.
What tools do I need to start coding?
You’ll need a computer and some software like a code editor. Visual Studio Code is a popular choice for beginners.
Are there free resources to learn coding?
Yes! Websites like Codecademy, freeCodeCamp, and W3Schools offer free coding lessons and tutorials.
Is it necessary to join a coding community?
While it’s not required, joining a coding community can provide support, feedback, and help you learn faster.