Ensuring Password Security: How to Confirm at Least One Capital Letter in JS
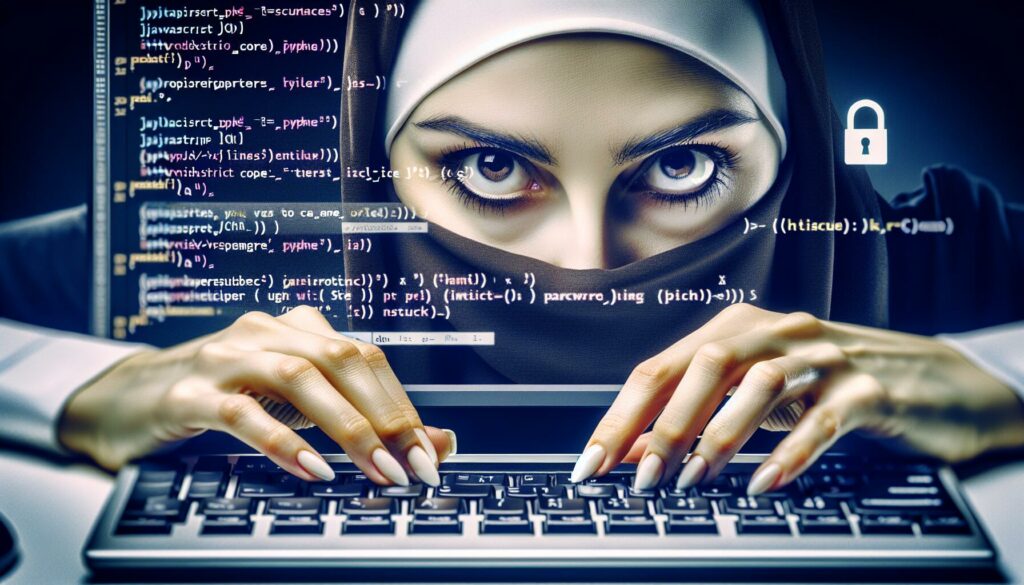
In today’s digital world, ensuring password security is more important than ever. One key aspect of creating a strong password is the use of capital letters. This article will explore how to confirm at least one capital letter in passwords using JavaScript. We’ll cover the basics of password security, the role of capital letters, and how to implement effective validation techniques in your code.
Key Takeaways
- Strong passwords are essential for online security.
- Using at least one capital letter in passwords helps enhance security.
- JavaScript can effectively validate passwords with regular expressions.
- User-friendly error messages improve the password creation experience.
- Regularly updating password policies is crucial for maintaining security.
Understanding Password Security Requirements
Importance of Strong Passwords
Strong passwords are essential for protecting personal and sensitive information. A weak password can lead to unauthorized access to accounts, which can result in identity theft or data breaches. Here are some key points to consider:
- Passwords should be unique for each account.
- They should be at least 8 characters long.
- A mix of letters, numbers, and symbols is recommended.
Common Password Vulnerabilities
Many users fall into the trap of using easily guessable passwords. Some common vulnerabilities include:
- Using personal information (like birthdays or names).
- Reusing passwords across multiple sites.
- Choosing simple passwords like "123456" or "password".
Vulnerability Type | Description |
---|---|
Personal Information | Using names, birthdays, or addresses. |
Reused Passwords | Using the same password for multiple accounts. |
Simple Passwords | Choosing easily guessable passwords. |
Role of Capital Letters in Passwords
Capital letters play a crucial role in enhancing password strength. They increase the complexity and make it harder for attackers to guess passwords. Including at least one capital letter is a simple yet effective way to improve security.
A strong password is not just about length; it’s about complexity and unpredictability.
By understanding these requirements, users can create stronger passwords that better protect their accounts.
JavaScript Basics for Password Validation
Introduction to JavaScript
JavaScript is a powerful programming language that helps make websites interactive. It allows developers to create dynamic content and validate user inputs, such as passwords. Understanding JavaScript is crucial for effective password validation.
Setting Up Your Development Environment
To start coding in JavaScript, you need a few basic tools:
- A text editor (like Visual Studio Code or Notepad++)
- A web browser (like Chrome or Firefox)
- Basic knowledge of HTML to create forms
Basic JavaScript Syntax
JavaScript has a simple syntax that is easy to learn. Here are some key points:
- Variables are used to store data. You can declare them using
var
,let
, orconst
. - Functions are blocks of code designed to perform a specific task. You can create a function to validate passwords.
- Control structures like
if
statements help you make decisions in your code.
In password validation, using JavaScript is essential since it allows the user to create a secure password and prevents password cracking.
By mastering these basics, you can effectively implement password validation in your web applications.
Regular Expressions in JavaScript
What Are Regular Expressions?
Regular expressions, often called regex, are patterns used to match character combinations in strings. In JavaScript, they are also treated as objects, which allows for powerful text processing capabilities. Understanding regex is essential for tasks like validating user input, searching text, and replacing strings.
Using Regular Expressions for Validation
To use regular expressions effectively, you need to know how to create them and apply them in your code. Here are some key points:
- Syntax: Regular expressions can be defined using literal notation or the
RegExp
constructor. - Flags: You can modify the behavior of regex with flags like
g
(global) andi
(case-insensitive). - Methods: Common methods include
test()
, which checks for a match, andexec()
, which retrieves the matched results.
Common Regular Expression Patterns
Here are some common patterns you might use:
Pattern | Description |
---|---|
^abc |
Starts with "abc" |
abc$ |
Ends with "abc" |
a.b |
Contains "a", any character, then "b" |
[a-z] |
Any lowercase letter |
word |
Matches the whole word "word" |
Regular expressions are a powerful tool for text processing, but they can be complex. Practice is key to mastering them!
Creating a Password Validation Function
Defining the Validation Criteria
To create a password validation function, we need to set clear rules. Here are some important criteria:
- Minimum length: Passwords should be at least 8 characters long.
- Maximum length: Passwords should not exceed 15 characters.
- Capital letters: At least one uppercase letter is required.
- Numbers and symbols: Including at least one number and one special character is recommended.
Writing the Validation Function
In JavaScript, we can use a function to check if a password meets these criteria. Here’s a simple example:
function validatePassword(password) {
const minLength = 8;
const maxLength = 15;
const hasUpperCase = /[A-Z]/.test(password);
const hasNumber = /[0-9]/.test(password);
const hasSpecialChar = /[!@#$%^&*]/.test(password);
if (password.length < minLength || password.length > maxLength) {
return false; // Length issue
}
if (!hasUpperCase) {
return false; // No capital letter
}
if (!hasNumber) {
return false; // No number
}
if (!hasSpecialChar) {
return false; // No special character
}
return true; // Valid password
}
This function checks the length and ensures that the password contains at least one capital letter, number, and special character.
Testing the Validation Function
To ensure our function works correctly, we should test it with various passwords. Here are some examples:
Password | Valid? |
---|---|
Password123! |
Yes |
password |
No |
PASSWORD123! |
No |
Pass123 |
No |
Pass123! |
Yes |
Remember: Testing is crucial to ensure that your password validation works as expected. Always try different combinations to cover all cases.
By following these steps, you can create a reliable password validation function in JavaScript. This will help ensure that users create strong passwords that are harder to guess or crack. Password validation in JavaScript is essential for maintaining security in your applications!
Ensuring at Least One Capital Letter in Passwords
Why Capital Letters Matter
Capital letters are essential in passwords because they increase complexity and make it harder for attackers to guess. Using at least one uppercase letter can significantly enhance password strength. Here are some reasons why capital letters are important:
- They add variety to the password.
- They help meet security requirements.
- They reduce the risk of common password vulnerabilities.
Regular Expression for Capital Letters
To check if a password contains at least one capital letter, we can use a regular expression. The pattern /(?=.*[A-Z])/
ensures that there is at least one uppercase letter in the password. This regex works by looking for any character followed by an uppercase letter.
Implementing the Check in JavaScript
Here’s how you can implement this check in JavaScript:
function validatePassword(password) {
const hasUppercase = /[A-Z]/.test(password);
if (!hasUppercase) {
alert("Your password must contain at least one uppercase letter.");
return false;
}
return true;
}
This function checks if the password meets the criteria and alerts the user if it does not. You can easily integrate this into a password validation form using JavaScript to ensure that users create strong passwords.
Advanced Password Validation Techniques
Combining Multiple Criteria
To ensure a strong password, it’s essential to combine various criteria. Here are some key points to consider:
- Length: Passwords should be at least 8 characters long.
- Character Variety: Include uppercase letters, lowercase letters, numbers, and special characters.
- Avoid Common Passwords: Don’t use easily guessable passwords like "password123".
Providing User Feedback
User feedback is crucial for a good experience. Here are some ways to provide it:
- Real-Time Validation: Check the password strength as the user types.
- Error Messages: Clearly indicate what is wrong with the password.
- Strength Meter: Show a visual representation of password strength.
Handling Edge Cases
When validating passwords, consider these edge cases:
- Spaces: Ensure that leading or trailing spaces are not allowed.
- Similar Characters: Avoid passwords that are too similar to the username or email.
- Common Patterns: Check for common patterns like "123456" or "abcdef".
Remember, mastering JavaScript form validation can greatly enhance user experience and ensure data accuracy. By implementing these techniques, you can create a more secure environment for users.
Common Mistakes in Password Validation
Case Sensitivity Issues
When validating passwords, many developers overlook case sensitivity. This can lead to situations where passwords that should be considered different are treated as the same. For example:
Password123
password123
Both of these should be treated as unique passwords, but if the validation is not case-sensitive, they will be considered identical.
Improper Regular Expressions
Using regular expressions incorrectly can cause major issues in password validation. Some common errors include:
- Missing or misplaced characters
- Incorrect use of anchors
- Overly complex patterns
To overcome these issues, it’s essential to test your regex thoroughly.
User Experience Considerations
A poor user experience can lead to frustration. Here are some common mistakes:
- Not providing clear error messages.
- Failing to indicate password requirements.
- Not allowing users to see their password while typing.
A good validation process should not only check for security but also ensure that users can easily understand what is required of them.
By avoiding these common mistakes, you can create a more secure and user-friendly password validation process.
Improving User Experience in Password Validation
Real-Time Feedback
Providing real-time feedback during password entry can significantly enhance user experience. Here are some effective strategies:
- Instant validation: Check password strength as the user types.
- Visual indicators: Use color codes (green for strong, red for weak) to show password strength.
- Guidance: Offer tips on creating a strong password, such as including numbers and symbols.
User-Friendly Error Messages
When users make mistakes, clear and helpful error messages can guide them. Consider these points:
- Specificity: Instead of saying "Invalid password," specify what’s wrong (e.g., "Must include at least one capital letter").
- Tone: Use a friendly tone to avoid frustrating users.
- Examples: Provide examples of strong passwords to help users understand.
Guidelines for Strong Passwords
To help users create secure passwords, provide clear guidelines:
- Length: Passwords should be at least 8 characters long.
- Complexity: Include uppercase letters, lowercase letters, numbers, and symbols.
- Avoid common words: Warn against using easily guessable information like names or birthdays.
Remember, credential management is crucial for improving security protocols. By following these guidelines, you can streamline the process and enhance user satisfaction.
Security Best Practices for Password Management
Storing Passwords Securely
- Use strong encryption to protect passwords in your database.
- Implement hashing techniques like bcrypt to store passwords securely.
- Regularly update your encryption methods to keep up with security standards.
Using Multi-Factor Authentication
- Add an extra layer of security by requiring a second form of verification.
- This could be a text message, email, or an authentication app.
- Multi-factor authentication significantly reduces the risk of unauthorized access.
Regularly Updating Password Policies
- Review and update your password policies at least once a year.
- Encourage users to change their passwords regularly, ideally every 3-6 months.
- Provide guidelines on creating strong passwords, such as using a mix of letters, numbers, and symbols.
Remember, strong password management is essential for protecting sensitive information. Regularly educating users about best practices can help prevent security breaches.
Best Practice | Description |
---|---|
Strong Encryption | Protects passwords in storage |
Multi-Factor Authentication | Adds an extra layer of security |
Regular Policy Updates | Keeps security measures current and effective |
Case Studies and Examples
Example 1: Simple Password Validator
In this example, we create a basic password validator using JavaScript. The goal is to ensure that the password meets certain criteria, including having at least one capital letter. Here’s a simple function:
function validatePassword(password) {
const hasUpperCase = /[A-Z]/.test(password);
return hasUpperCase;
}
This function checks if the password contains at least one uppercase letter.
Example 2: Comprehensive Password Validator
For a more robust solution, we can expand our validator to include additional criteria:
- At least one uppercase letter
- At least one lowercase letter
- At least one number
- Minimum length of 8 characters
Here’s how that might look:
function validatePassword(password) {
const hasUpperCase = /[A-Z]/.test(password);
const hasLowerCase = /[a-z]/.test(password);
const hasNumber = /[0-9]/.test(password);
const isValidLength = password.length >= 8;
return hasUpperCase && hasLowerCase && hasNumber && isValidLength;
}
Real-World Applications
These password validators can be used in various applications, such as:
- User registration forms
- Password change features
- Account recovery processes
Implementing strong password validation is crucial for protecting user data and maintaining security in applications.
Example 3: Testing the Validation Function
To ensure our validation function works correctly, we can test it with different passwords:
Password | Valid? |
---|---|
Password1 |
Yes |
password |
No |
PASSWORD |
No |
Pass1 |
No |
Pass1234 |
Yes |
This table shows how different passwords meet or fail the validation criteria. By using these examples, developers can better understand how to implement effective password validation in their applications.
Tools and Libraries for Password Validation
When it comes to ensuring password security, using the right tools and libraries can make a big difference. Here are some popular options:
Popular JavaScript Libraries
- Joi: A powerful schema description language and data validator for JavaScript.
- Yup: A JavaScript schema builder for value parsing and validation.
- Validator.js: A library for string validation and sanitization.
- Class-validator: A validation library for TypeScript and JavaScript.
- Zod: A TypeScript-first schema declaration and validation library.
These libraries help developers create secure password validation easily.
Integrating Third-Party Tools
- Password Managers: Tools like LastPass or 1Password can help users create and store strong passwords.
- Security APIs: Services like Auth0 provide built-in password validation features.
- Custom Solutions: Developers can create tailored validation functions using libraries mentioned above.
Custom vs. Pre-Built Solutions
- Custom Solutions: Allow for specific requirements but require more development time.
- Pre-Built Solutions: Quick to implement but may not fit all needs.
Using established libraries can save time and ensure better security practices.
By leveraging these tools, developers can enhance their password validation processes and improve overall security.
When it comes to ensuring your passwords are strong and secure, using the right tools and libraries is essential. These resources can help you create passwords that are hard to guess and protect your personal information. Don’t wait any longer—visit our website to explore how you can enhance your coding skills and prepare for your future!
Conclusion
In conclusion, making sure that passwords are secure is very important. By using JavaScript, you can easily check if a password has at least one capital letter, along with other requirements like lowercase letters and numbers. This helps keep accounts safe from hackers. Always remember to create strong passwords and encourage others to do the same. With the right tools and knowledge, we can all contribute to a safer online environment.
Frequently Asked Questions
Why is it important to have at least one capital letter in my password?
Having at least one capital letter makes your password stronger and harder for others to guess.
How can I check if my password meets the requirements?
You can use a password validation function in JavaScript to check if your password contains a capital letter, lowercase letter, number, and special character.
What happens if my password doesn’t have a capital letter?
If your password lacks a capital letter, it may not be accepted by many websites, which can prevent you from accessing your account.
Can I use more than one capital letter in my password?
Yes, using multiple capital letters can further enhance the strength of your password.
What is a regular expression, and how is it used for password validation?
A regular expression is a pattern that helps search and match strings. It’s used in JavaScript to check if a password meets specific criteria, like having a capital letter.
Are there any tools to help me create secure passwords?
Yes, there are many password managers and generators available that can help you create and store secure passwords.
What are some common mistakes people make with passwords?
Common mistakes include using easily guessable passwords, not using a mix of characters, and reusing passwords across multiple sites.
How can I improve my password security?
You can improve your password security by using longer passwords, including a mix of letters, numbers, and symbols, and enabling two-factor authentication.