Mastering How to Make Directed Graph in Python: A Comprehensive Guide for LeetCode Challenges
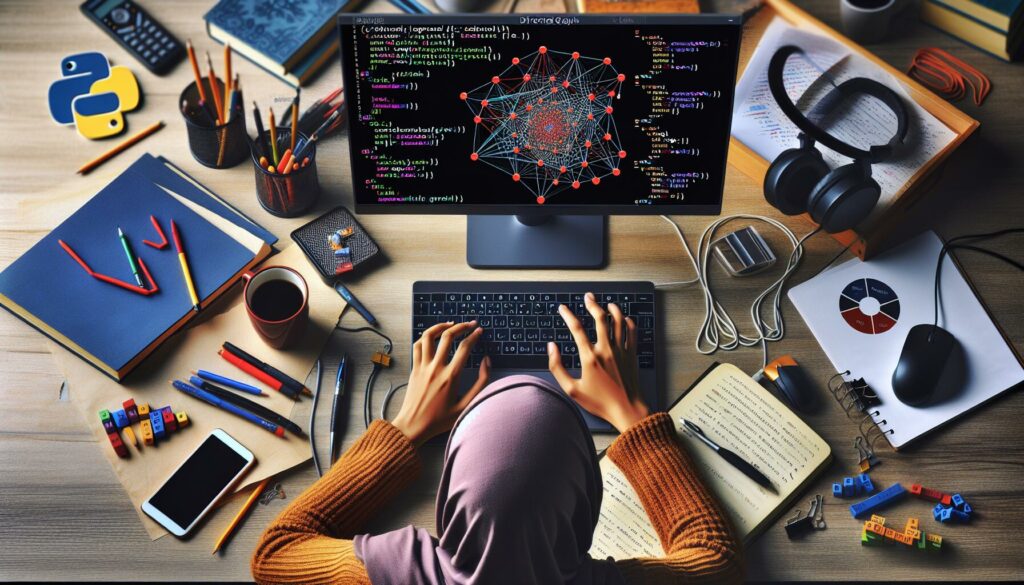
In this guide, we will explore the world of directed graphs and how they are essential for solving coding problems, especially on platforms like LeetCode. We will break down complex concepts into simple, easy-to-understand sections, making it accessible for beginners. From setting up your Python environment to advanced algorithms, this article aims to equip you with the knowledge you need to tackle graph-related challenges with confidence.
Key Takeaways
- Directed graphs have edges that show a specific direction, making them different from regular graphs.
- Understanding how to represent graphs in Python is key for solving coding problems effectively.
- Graph traversal techniques like DFS and BFS are essential for exploring nodes in a graph.
- Mastering algorithms such as topological sorting and cycle detection can help you solve many LeetCode problems.
- Practicing with real-world problems can solidify your understanding and improve your coding skills.
Understanding Directed Graphs and Their Importance in LeetCode
Definition and Characteristics of Directed Graphs
A directed graph is a collection of nodes connected by edges, where each edge has a direction. This means that if there is an edge from node A to node B, you can go from A to B, but not necessarily from B to A. Here are some key characteristics:
- Nodes: The points in the graph.
- Edges: The connections between nodes, which have a direction.
- Directed: The edges point from one node to another.
Why Directed Graphs Are Crucial for Coding Interviews
Understanding directed graphs is essential for coding interviews because:
- They are commonly used in various problems.
- They help in modeling real-world scenarios like web pages and social networks.
- Many algorithms, such as DFS and BFS, are based on directed graphs.
Common Problems Involving Directed Graphs on LeetCode
When tackling directed graph problems on LeetCode, you might encounter:
- Cycle Detection: Checking if a directed graph has cycles.
- Topological Sorting: Ordering nodes in a way that for every directed edge from node A to node B, A comes before B.
- Shortest Path: Finding the shortest route from one node to another.
Understanding these concepts will help you tackle a variety of graph-related challenges effectively. Mastering these patterns can significantly improve your problem-solving skills in coding interviews.
Setting Up Your Python Environment for Graph Implementation
Installing Necessary Libraries
To work with directed graphs in Python, you need to install some libraries. Here are the essential ones:
- NetworkX: A library for creating and manipulating complex networks.
- Matplotlib: Useful for visualizing graphs.
- NumPy: Helps with numerical operations.
You can install these libraries using pip:
pip install networkx matplotlib numpy
Setting Up a Python Project
When you start a new project, it’s important to organize your files properly. Here’s how to do that:
- Create a new folder for your project.
- Open VS Code and start a new PowerShell terminal (Terminal > New Terminal).
- Navigate to your project folder using the terminal.
- Create a virtual environment to keep your dependencies organized:
python -m venv venv
- Activate the virtual environment:
- On Windows:
. vemin\activate
- On macOS/Linux:
source venv/bin/activate
- On Windows:
Basic Python Syntax for Graphs
Understanding the basic syntax is crucial for implementing graphs. Here are some key points:
- Defining a class for your graph:
class Graph: def __init__(self): self.graph = {}
- Adding edges to the graph:
def add_edge(self, u, v): if u not in self.graph: self.graph[u] = [] self.graph[u].append(v)
- Printing the graph:
def print_graph(self): for node in self.graph: print(node, "->", self.graph[node])
Remember: Setting up your environment correctly is the first step to successfully implementing directed graphs in Python!
Representing Directed Graphs in Python
Using Adjacency Lists
An adjacency list is a popular way to represent a directed graph. In this method, each node has a list of nodes it points to. This representation is efficient in terms of space, especially for sparse graphs. Here’s how you can create an adjacency list:
- Initialize a dictionary where each key is a node.
- Add edges by appending the destination node to the list of the source node.
- Example:
graph = {1: [2, 3], 2: [4], 3: [], 4: []}
Using Adjacency Matrices
An adjacency matrix is another way to represent a directed graph. It uses a 2D array where the cell at row i
and column j
indicates whether there is an edge from node i
to node j
. The representation of directed graph as adjacency matrix is shown below:
0 | 1 | 2 | 3 | |
---|---|---|---|---|
0 | 0 | 1 | 0 | 0 |
1 | 0 | 0 | 0 | 0 |
2 | 0 | 1 | 0 | 0 |
3 | 1 | 0 | 0 | 0 |
In this matrix, there is an edge from (0) to (1), (2) to (1), and (3) to (0). Initially, the entire matrix is initialized to 0.
Choosing the Right Representation
When deciding between an adjacency list and an adjacency matrix, consider the following:
- Space Efficiency: Adjacency lists are more space-efficient for sparse graphs.
- Time Complexity: Adjacency matrices allow for faster edge lookups.
- Graph Density: Use an adjacency matrix for dense graphs and an adjacency list for sparse ones.
Choosing the right representation can significantly impact the performance of your graph algorithms.
Understanding these representations is crucial for solving graph problems effectively on platforms like LeetCode.
Building a Directed Graph from Scratch
Creating Nodes and Edges
To build a directed graph, you first need to create nodes and edges. Here’s how you can do it:
- Define a Node: Each node can be represented as a simple class.
- Create Edges: An edge connects two nodes, showing the direction.
- Store the Graph: Use a dictionary to keep track of nodes and their connections.
Implementing Graph Classes
Creating a graph class helps organize your code. Here’s a basic structure:
class Node:
def __init__(self, value):
self.value = value
self.edges = []
class Graph:
def __init__(self):
self.nodes = {}
def add_node(self, value):
new_node = Node(value)
self.nodes[value] = new_node
def add_edge(self, from_value, to_value):
if from_value in self.nodes and to_value in self.nodes:
self.nodes[from_value].edges.append(self.nodes[to_value])
Adding and Removing Edges Dynamically
Managing edges is crucial for a directed graph. Here are some steps:
- Add an Edge: Use the
add_edge
method to connect nodes. - Remove an Edge: Create a method to remove an edge by finding the target node in the source node’s edges.
- Check for Edge Existence: Implement a method to verify if an edge exists between two nodes.
Building a directed graph from scratch allows you to understand the underlying structure and relationships between nodes. This foundational knowledge is essential for tackling complex graph problems.
Graph Traversal Techniques
Depth-First Search (DFS)
Depth-First Search (DFS) is a method for exploring a graph by starting at a root node and moving as far as possible along each branch before backtracking. This technique is useful for traversing or searching tree or graph data structures. Here’s how it works:
- Start at the root node.
- Explore each branch before moving to the next.
- Use a stack (either explicitly or via recursion) to keep track of nodes.
Breadth-First Search (BFS)
Breadth-First Search (BFS) explores the graph level by level. It starts at the root node and visits all its neighbors before moving on to the next level. This method is particularly effective for finding the shortest path in unweighted graphs. Here’s a simple breakdown:
- Start at the root node.
- Visit all neighboring nodes.
- Move to the next level of neighbors.
When to Use DFS vs. BFS
Choosing between DFS and BFS depends on the problem at hand:
- Use DFS when you want to explore all possibilities, such as in puzzles or games.
- Use BFS when you need the shortest path or to explore all nodes at the present depth before moving on.
Understanding these traversal techniques is essential for solving graph-related problems effectively. They form the foundation for many advanced algorithms and are frequently tested in coding interviews.
Technique | Best Use Case | Complexity |
---|---|---|
DFS | Exploring all paths | O(V + E) |
BFS | Shortest path in unweighted graphs | O(V + E) |
Advanced Graph Algorithms
Topological Sorting
Topological sorting is a way to arrange the nodes of a directed graph in a linear order. This is especially useful when you need to schedule tasks that depend on each other. In a topological sort, each directed edge (u, v) means that node u comes before node v. Here are some key points:
- It can only be applied to Directed Acyclic Graphs (DAGs).
- There can be multiple valid topological sorts for a graph.
- Common algorithms include Kahn’s algorithm and Depth-First Search (DFS).
Detecting Cycles in Directed Graphs
Detecting cycles is crucial in many applications, such as ensuring that a task can be completed without getting stuck in a loop. Here’s how you can detect cycles:
- Use Depth-First Search (DFS) and keep track of visited nodes.
- Maintain a recursion stack to track the path of the current DFS.
- If you revisit a node that is already in the recursion stack, a cycle exists.
Shortest Path Algorithms
Finding the shortest path in a directed graph can be done using various algorithms:
- Dijkstra’s Algorithm: Best for graphs with non-negative weights.
- Bellman-Ford Algorithm: Can handle graphs with negative weights but no negative cycles.
- Floyd-Warshall Algorithm: Useful for finding shortest paths between all pairs of nodes.
Understanding these advanced algorithms is essential for solving complex problems on platforms like LeetCode. They help you tackle challenges that require efficient solutions and a deep understanding of graph theory.
By mastering these techniques, you can significantly improve your problem-solving skills in coding interviews and competitive programming.
Practical LeetCode Problems Involving Directed Graphs
Problem-Solving Strategies
When tackling directed graph problems on LeetCode, consider these strategies:
- Understand the problem: Read the problem statement carefully to grasp what is being asked.
- Identify the graph representation: Determine whether to use an adjacency list or matrix based on the problem’s requirements.
- Choose the right algorithm: Depending on the problem, select between BFS, DFS, or other graph algorithms.
Common Pitfalls and How to Avoid Them
Many beginners face challenges when solving graph problems. Here are some common mistakes:
- Ignoring edge cases: Always consider special cases, like empty graphs or single-node graphs.
- Misunderstanding graph traversal: Ensure you know when to use BFS versus DFS.
- Not optimizing for performance: Be aware of time and space complexity to avoid inefficient solutions.
Examples of LeetCode Problems and Solutions
Here are some notable problems involving directed graphs:
Problem Title | Description |
---|---|
LeetCode 785: Is Graph Bipartite? | Check if a graph can be colored using two colors without adjacent nodes having the same color. |
LeetCode 207: Course Schedule | Determine if you can finish all courses given prerequisites, which involves cycle detection in a directed graph. |
LeetCode 261: Graph Valid Tree | Verify if a given graph is a valid tree structure. |
Mastering these problems is essential for success in coding interviews. Understanding the underlying concepts will help you tackle similar challenges with confidence.
Optimizing Graph Algorithms for Performance
Time Complexity Analysis
When working with directed graphs, understanding time complexity is essential. Here are some common complexities:
- O(V + E): For traversals like BFS and DFS, where V is the number of vertices and E is the number of edges.
- O(V^2): For algorithms using adjacency matrices, which can be inefficient for large graphs.
- O(E log V): For algorithms like Dijkstra’s when using a priority queue.
Space Complexity Considerations
Space complexity is just as important as time complexity. Here are some points to consider:
- Adjacency List: Uses less space, especially for sparse graphs.
- Adjacency Matrix: Takes up more space, but can be faster for dense graphs.
- Visited Set: Keep track of visited nodes to avoid cycles, which adds to space usage.
Optimizing Python Code for Graphs
To make your graph algorithms run faster, consider these tips:
- Use built-in data structures like
set
anddict
for faster lookups. - Avoid deep recursion; use iterative methods instead to prevent stack overflow.
- Profile your code to find bottlenecks and optimize them.
Optimization in Python: Python offers a variety of powerful techniques for solving optimization problems. This ranges from simple gradient-based methods to more complex algorithms.
By focusing on these aspects, you can significantly improve the performance of your graph algorithms, making them more efficient for LeetCode challenges.
Testing and Debugging Graph Implementations
Writing Unit Tests for Graph Functions
Testing your graph functions is essential to ensure they work correctly. Here are some steps to follow:
- Identify Key Functions: Focus on functions that add, remove, or traverse nodes and edges.
- Create Test Cases: Write test cases for various scenarios, including edge cases like empty graphs or graphs with one node.
- Use Assertions: Implement assertions to check if the output matches the expected results.
Common Bugs in Graph Algorithms
When working with graphs, you might encounter several common bugs:
- Infinite Loops: Often caused by not marking nodes as visited during traversal.
- Incorrect Edge Cases: Failing to handle graphs with no edges or nodes can lead to errors.
- Memory Leaks: Not properly deleting nodes can cause memory issues.
Debugging Tips and Tools
Debugging can be tricky, but here are some helpful tips:
- Use Print Statements: Print the state of your graph at various points to understand its structure.
- Leverage Debugging Tools: Tools like VSCode can help you step through your code. This article will guide you through the process of setting up and using VSCode to debug a Python module, from initial setup to advanced debugging techniques.
- Check for Cycles: Ensure your algorithms correctly identify cycles, as this is a common source of errors.
Debugging is not just about fixing errors; it’s about understanding your code better. Reflecting on mistakes can lead to improved coding skills.
Real-World Applications of Directed Graphs
Directed graphs are used in many real-life situations. From social networks to transportation systems, directed graphs provide a structured way to represent and analyze complex systems.
Use Cases in Software Engineering
- Version Control Systems: Directed graphs help track changes in code, showing how different versions relate to each other.
- Dependency Management: In software projects, directed graphs can represent dependencies between different modules or packages.
- Workflow Management: They can model workflows, showing the order of tasks and their dependencies.
Applications in Data Science and Machine Learning
- Recommendation Systems: Directed graphs can represent user-item interactions, helping to suggest products or content.
- Neural Networks: The architecture of neural networks can be viewed as a directed graph, where nodes represent neurons and edges represent connections.
- Data Flow Analysis: Directed graphs help analyze how data moves through systems, identifying bottlenecks and optimizing performance.
Directed Graphs in Network Analysis
- Traffic Flow: They model traffic systems, showing how vehicles move from one point to another.
- Social Network Analysis: Directed graphs represent relationships between users, helping to analyze influence and connectivity.
- Supply Chain Management: They can illustrate the flow of goods from suppliers to consumers, optimizing logistics.
Directed graphs are essential tools in various fields, helping to simplify and solve complex problems efficiently.
Resources for Further Learning
Books and Online Courses
- Books: Look for titles that focus on algorithms and data structures. Some popular choices include:
- Introduction to Algorithms by Cormen et al.
- Grokking Algorithms by Bhargava.
- Data Structures and Algorithms Made Easy by Narasimha Karumanchi.
- Online Courses: Websites like Coursera and Udemy offer courses on graph algorithms and Python programming. Consider:
- "Data Structures and Algorithms Specialization" on Coursera.
- "Python for Everybody" on Coursera.
- "Algorithms and Data Structures in Python" on Udemy.
Interactive Coding Platforms
- LeetCode: A great platform for practicing coding problems, especially those related to directed graphs.
- HackerRank: Offers challenges that can help you improve your graph skills.
- CodeSignal: Provides a variety of coding tasks, including graph-related problems.
Communities and Forums for Graph Enthusiasts
- Stack Overflow: A place to ask questions and share knowledge about graph algorithms.
- Reddit: Subreddits like r/learnprogramming and r/coding can be helpful.
- Discord Servers: Join programming communities to discuss graph algorithms and get help from peers.
Remember, learning is a journey. Engaging with others and practicing regularly will help you master directed graphs and their applications in coding challenges.
If you’re eager to dive deeper into coding, check out our website for more resources! We offer a variety of interactive tutorials and helpful guides that can boost your skills and prepare you for coding interviews. Don’t wait—start your journey today!
Conclusion
In wrapping up, it’s clear that understanding directed graphs is essential for tackling coding challenges, especially on platforms like LeetCode. The main goal of this guide was to help you see the different patterns in graph problems. Recognizing these patterns can make a big difference in how you approach each challenge. It took me a while to grasp this concept, and I often mixed up different types of problems. By learning to identify and solve these patterns separately, you’ll feel more confident during interviews. Remember, mastering these skills will not only help you on LeetCode but also in real-world coding situations.
Frequently Asked Questions
What is a directed graph?
A directed graph is a type of graph where the edges have a direction. This means that if there is a connection from point A to point B, it doesn’t go the other way unless there’s another connection.
Why are directed graphs important for coding interviews?
Directed graphs often show up in coding interviews because they help solve many real-world problems, like finding the best route or organizing tasks. Knowing how to work with them can help you impress interviewers.
What are some common problems involving directed graphs on LeetCode?
Some common problems include finding the shortest path, checking if there is a cycle, and topological sorting. These problems test your understanding of graph concepts.
How do I set up my Python environment for graph programming?
You can set up your Python environment by installing libraries like NetworkX and making sure you have a good code editor. This will help you write and test your graph code easily.
What are adjacency lists and matrices?
An adjacency list is a way to represent a graph where each node has a list of its neighbors. An adjacency matrix is a table that shows whether pairs of nodes are connected.
What are DFS and BFS?
DFS (Depth-First Search) explores as far as possible along a branch before backtracking, while BFS (Breadth-First Search) explores all neighbors at the present depth before moving on to nodes at the next depth level.
How can I optimize graph algorithms for better performance?
You can optimize graph algorithms by analyzing their time and space complexity and using efficient data structures to store your graph.
What are some real-world applications of directed graphs?
Directed graphs are used in various fields like computer networks, social media analysis, and project scheduling. They help in organizing and analyzing relationships.