Get Multiple Smallest Elements in a List Without Sorting in Python: A Step-by-Step Guide
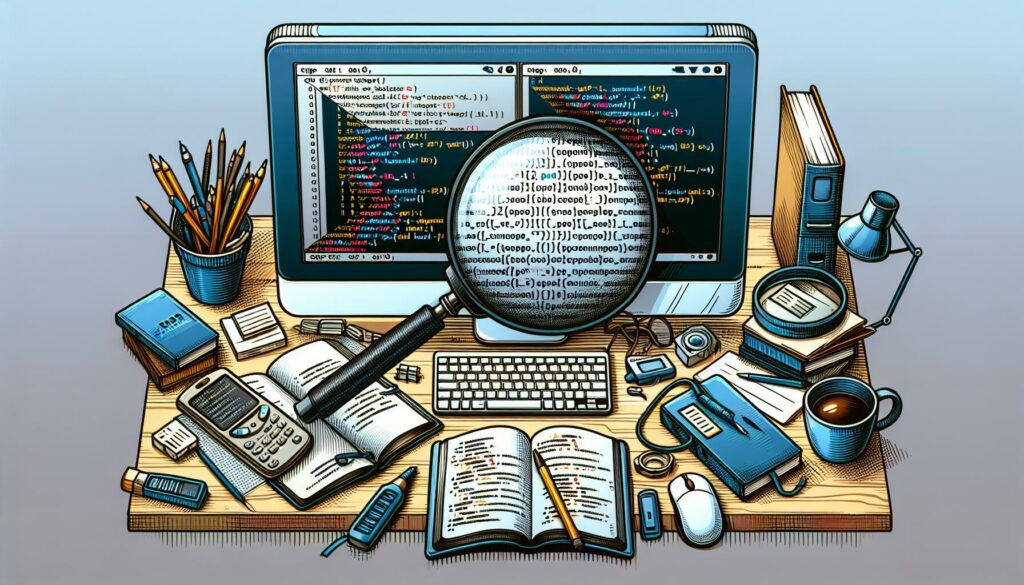
In this guide, we will explore various methods to find multiple smallest elements in a list without resorting to sorting. Sorting can be inefficient, especially for large lists. Instead, we will delve into alternative techniques that are both effective and efficient. Each method will be explained step-by-step, making it easy for you to understand and apply in your own coding projects.
Key Takeaways
- Finding the smallest elements without sorting can save time and resources.
- Using built-in functions like min() can simplify the process but may have limitations.
- Heaps are an efficient way to retrieve the smallest elements in a list.
- The enumerate function allows you to track indices while finding elements.
- Numpy can speed up calculations, especially with large datasets.
Understanding the Problem: Finding Multiple Smallest Elements
Defining the Problem Statement
Finding the smallest elements in a list is a common task in programming. The challenge arises when you need to find multiple smallest elements without sorting the entire list. Sorting can be slow and unnecessary if you only need a few smallest values.
Why Not Use Sorting?
Sorting a list takes time, especially for large lists. Here are some reasons to avoid sorting:
- Time Complexity: Sorting usually takes O(n log n) time, while finding the smallest elements can often be done in O(n).
- Unnecessary Work: If you only need a few smallest elements, sorting the whole list is overkill.
- Memory Usage: Sorting may require additional memory, which can be a concern in large datasets.
Importance of Efficient Solutions
Efficient solutions are crucial for performance, especially in applications that handle large datasets. Here are some benefits of finding multiple smallest elements efficiently:
- Faster Execution: Reduces the time taken to process data.
- Lower Resource Consumption: Saves memory and processing power.
- Scalability: Makes your code more adaptable to larger datasets.
Efficiently finding the smallest elements can significantly improve the performance of your applications, especially when dealing with large lists.
Using the Min Function in Python
Basic Usage of Min Function
The min()
function in Python is a built-in tool that helps you find the smallest item in a list or any iterable. It can take multiple arguments or a single iterable. Here’s how you can use it:
- Single Iterable:
min([3, 1, 4, 1, 5])
returns1
. - Multiple Arguments:
min(3, 1, 4, 1, 5)
also returns1
.
Finding Multiple Smallest Elements
To find multiple smallest elements, you can use the min()
function in a loop or with list comprehensions. Here’s a simple way to do it:
- Create a list of numbers.
- Use a loop to call
min()
multiple times. - Remove the found minimum from the list each time.
For example:
numbers = [5, 3, 1, 4, 2]
smallest = []
for _ in range(3):
smallest.append(min(numbers))
numbers.remove(min(numbers))
This will give you the three smallest numbers.
Limitations of Min Function
While min()
is useful, it has some limitations:
- It only finds one minimum at a time.
- It can be inefficient for large lists if used repeatedly.
- It does not handle duplicates well if you want unique smallest elements.
The min() function is great for quick tasks, but for more complex needs, consider other methods.
In summary, the min()
function is a handy tool in Python for finding the smallest values, but it may not always be the best choice for finding multiple smallest elements efficiently.
Leveraging the Heap Data Structure
Introduction to Heaps
Heaps are special tree-based data structures that satisfy the heap property. In a max heap, for any given node, the value of the node is greater than or equal to the values of its children. Conversely, in a min heap, the value of the node is less than or equal to the values of its children. Heaps are particularly useful for efficiently finding the smallest or largest elements in a list.
Using heapq to Find Smallest Elements
Python provides a built-in module called heapq
that allows us to work with heaps easily. Here’s how you can use it to find the smallest elements in a list:
- Import the module: Start by importing
heapq
. - Create a heap: Use
heapq.heapify()
to transform your list into a heap. - Extract smallest elements: Use
heapq.nsmallest(n, iterable)
to get then
smallest elements.
Here’s a simple example:
import heapq
numbers = [5, 1, 8, 3, 2]
smallest = heapq.nsmallest(3, numbers)
print(smallest) # Output: [1, 2, 3]
Time Complexity Analysis
The time complexity for creating a heap is O(n), and finding the smallest elements using heapq.nsmallest()
is O(n log k), where k
is the number of smallest elements you want to find. This makes heaps a very efficient choice for this task, especially when dealing with large lists.
Using heaps can significantly reduce the time needed to find multiple smallest elements compared to sorting the entire list.
Utilizing the Enumerate Function
How Enumerate Works
The enumerate()
function in Python allows you to loop over a list while keeping track of the index of each element. This is useful when you need both the value and its position in the list. Using enumerate()
can make your code cleaner and easier to read.
Finding Smallest Elements with Enumerate
To find the smallest elements in a list using enumerate()
, you can follow these steps:
- Use
enumerate()
to get both the index and the value of each element. - Compare the values to find the smallest one.
- Store the smallest values in a new list if you want to find multiple smallest elements.
Here’s a simple example:
lst = [20, 10, 20, 1, 100]
a, i = min((a, i) for (i, a) in enumerate(lst))
print(a) # Output: 1
Advantages and Disadvantages
- Advantages:
- Cleaner code with less complexity.
- Easy to understand and maintain.
- Disadvantages:
- May not be the most efficient for very large lists.
- Requires additional logic to handle multiple smallest elements.
Using enumerate() can simplify your code, but always consider the size of your data and the efficiency of your approach.
Applying the Reduce Function
Understanding Reduce
The reduce
function is a powerful tool in Python that allows you to apply a function cumulatively to the items of an iterable. This means you can combine elements in a list to produce a single result. It’s especially useful for finding the smallest element in a list without sorting it.
Using Reduce to Find Smallest Elements
To find the smallest element in a list using reduce
, you can follow these steps:
- Import the
reduce
function from thefunctools
module. - Define a list of numbers.
- Use
reduce
with themin
function to get the smallest element.
Here’s a simple example:
from functools import reduce
lst = [20, 10, 20, 15, 100]
smallest = reduce(min, lst)
print(smallest) # Output: 10
Comparing Reduce with Other Methods
While reduce
is effective, it has some limitations:
- Readability: Code using
reduce
can be harder to read for beginners. - Performance: It may not be as efficient as other methods for very large lists.
- Complexity: Understanding how
reduce
works can be challenging for those new to programming.
Using reduce can simplify your code, but always consider if it’s the best choice for your specific problem.
Implementing Recursion for Smallest Elements
Basics of Recursion
Recursion is a method where a function calls itself to solve smaller instances of the same problem. In Python, this can be a powerful way to find the smallest elements in a list. Using recursion can simplify complex problems by breaking them down into smaller, manageable parts.
Recursive Approach to Find Smallest Elements
To find the smallest element in a list using recursion, we can create a function that compares each element with the current smallest value. Here’s a simple way to do it:
def find_smallest(index, current_min, numbers):
if index == len(numbers): # Base case
return current_min
if numbers[index] < current_min: # Check if current element is smaller
current_min = numbers[index]
return find_smallest(index + 1, current_min, numbers) # Recursive call
# Example usage:
numbers = [5, 3, 8, 1, 4]
smallest = find_smallest(0, numbers[0], numbers)
print(f'The smallest number is {smallest}') # Output: 1
Pros and Cons of Recursion
- Pros:
- Simplifies code for complex problems.
- Easy to understand and implement for small lists.
- Cons:
- Can lead to high memory usage due to function call stack.
- May hit the recursion limit for large lists; this limit can typically be adjusted using the
sys.setrecursionlimit()
function, but it’s important to manage recursion depth wisely to avoid memory issues.
Recursion can be a great tool, but it’s essential to use it wisely to prevent performance issues.
In summary, recursion is a useful technique for finding the smallest elements in a list, but it’s important to be aware of its limitations and potential pitfalls.
Using Numpy for Efficient Computation
Introduction to Numpy
NumPy is a powerful library in Python that helps with numerical calculations. It is especially useful for working with large datasets and performing complex mathematical operations quickly. Using NumPy can significantly speed up your computations compared to regular Python lists.
Finding Smallest Elements with Numpy
To find the smallest elements in a list using NumPy, you can follow these steps:
- Import the NumPy library.
- Convert your list into a NumPy array.
- Use the
np.partition()
function to find the smallest elements efficiently.
Here’s a simple example:
import numpy as np
# Sample list
data = [5, 1, 9, 3, 7]
# Convert to NumPy array
array_data = np.array(data)
# Find the 3 smallest elements
smallest_elements = np.partition(array_data, 2)[:3]
print(smallest_elements) # Output: [1 3 5]
Performance Considerations
Using NumPy can lead to better performance, especially with large datasets. Here’s a quick comparison of methods:
Method | Time Complexity | Suitable for Large Lists |
---|---|---|
Sorting | O(n log n) | No |
Min Function | O(n) | Yes |
NumPy Partition | O(n) | Yes |
NumPy is not just faster; it also provides a wide range of functions that can simplify your code.
In summary, leveraging NumPy for finding the smallest elements in a list can lead to more efficient and cleaner code.
Handling Lists with Duplicate Elements
Challenges with Duplicates
When working with lists that contain duplicate elements, several challenges can arise:
- Identifying unique values: It can be hard to find distinct elements when duplicates are present.
- Maintaining order: Preserving the original order of elements while removing duplicates can be tricky.
- Performance issues: Some methods may be inefficient, especially with large lists.
Strategies to Handle Duplicates
Here are some effective strategies to manage duplicates in lists:
- Using a set: Convert the list to a set to remove duplicates, but this won’t maintain order.
- List comprehension: Create a new list while checking for duplicates, which helps in preserving order.
- Dictionary method: To remove duplicates while keeping the order, create a dictionary from the list and then extract its keys as a new list. This method is efficient and straightforward.
Examples and Code Snippets
Here’s a simple example of how to remove duplicates while preserving order:
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = list(dict.fromkeys(my_list)) # [1, 2, 3, 4, 5]
In this example, we use the dictionary method to remove duplicates efficiently. This approach is particularly useful when you want to keep the original order of elements intact.
Remember: Handling duplicates effectively can lead to cleaner and more efficient code.
By understanding these challenges and strategies, you can better manage lists with duplicate elements in Python.
Finding Indices of Smallest Elements
Why Indices Matter
Finding the indices of the smallest elements in a list can be very useful in various applications, such as data analysis and algorithm optimization. Knowing where the smallest elements are located allows for more efficient data manipulation. Here are some reasons why indices are important:
- Helps in tracking the position of elements for further processing.
- Useful in algorithms that require element positions, like sorting or searching.
- Can assist in visualizing data trends based on element locations.
Methods to Find Indices
There are several ways to find the indices of the smallest elements in a list. Here are a few common methods:
- Using a Loop: Iterate through the list and keep track of the smallest value and its index.
- Using List Comprehension: Create a list of indices where the elements are equal to the smallest value.
- Using the
enumerate
Function: This function allows you to loop through the list while keeping track of the index.
Practical Applications
Finding the indices of the smallest elements can be applied in various scenarios:
- Data Analysis: Identifying outliers or minimum values in datasets.
- Machine Learning: Selecting features based on their importance.
- Game Development: Tracking the lowest scores or best performances.
In programming, understanding how to efficiently find indices can greatly enhance the performance of your algorithms and data handling.
Comparing Different Methods
When it comes to finding the smallest elements in a list, there are several methods to consider. Each method has its own strengths and weaknesses, making it important to choose the right one for your needs.
Overview of Methods
- Min Function: A straightforward way to find the smallest element, but limited to one element at a time.
- Heap Data Structure: Efficient for finding multiple smallest elements without sorting the entire list.
- Enumerate Function: Useful for tracking indices while searching for smallest elements.
- Reduce Function: A functional approach that can be handy but may not be the most efficient.
- Recursion: Offers a unique way to solve the problem but can be complex and less efficient for large lists.
- Numpy: Great for performance, especially with large datasets, but requires additional libraries.
Performance Comparison
Method | Time Complexity | Pros | Cons |
---|---|---|---|
Min Function | O(n) | Simple to use | Only finds one element |
Heap | O(n log k) | Efficient for multiple elements | More complex implementation |
Enumerate | O(n) | Easy to understand | Less efficient for large lists |
Reduce | O(n) | Functional programming style | Can be less intuitive |
Recursion | O(n) | Elegant solution | Risk of stack overflow |
Numpy | O(n) | Fast for large datasets | Requires installation |
Choosing the Right Method
Choosing the right method depends on your specific needs. If you need to find just one smallest element, the min function is quick and easy. For multiple smallest elements, consider using a heap. If you’re working with large datasets, Numpy might be the best choice. Always weigh the pros and cons based on your situation.
In programming, understanding the strengths and weaknesses of different methods can save you time and improve your code’s efficiency.
By comparing these methods, you can make an informed decision on how to tackle the problem of finding the smallest elements in a list effectively. Remember, the goal is to find a balance between simplicity and performance, especially when dealing with larger datasets.
In summary, each method has its unique advantages, and the best choice often depends on the specific requirements of your task.
Common Pitfalls and How to Avoid Them
Typical Mistakes
When working with lists to find the smallest elements, there are several common mistakes that can lead to incorrect results or inefficient code. Here are a few to watch out for:
- Assuming all elements are unique: If your list has duplicates, you might miss some smallest elements.
- Not considering edge cases: Always check how your code behaves with empty lists or lists with one element.
- Using inefficient methods: Relying on sorting can be slow, especially for large lists.
Debugging Tips
Debugging is crucial for ensuring your code works as intended. Here are some tips:
- Print intermediate results: This helps you see what your code is doing at each step.
- Use assertions: Check that your assumptions about the data hold true.
- Test with various inputs: Make sure to try different scenarios, including edge cases.
Best Practices
To write efficient and effective code, follow these best practices:
- Choose the right method: Depending on your needs, select the most efficient way to find the smallest elements.
- Keep your code clean: Write clear and understandable code to make debugging easier.
- Document your code: Use comments to explain complex parts of your code.
Remember: Avoiding these pitfalls can save you time and effort in the long run. Always test your code thoroughly before finalizing it.
Summary Table of Common Pitfalls
Pitfall | Description | Solution |
---|---|---|
Assuming uniqueness | Overlooking duplicates can lead to errors. | Check for duplicates. |
Ignoring edge cases | Failing to handle empty or single-element lists. | Test with various inputs. |
Inefficient methods | Using sorting instead of more efficient methods. | Use heaps or other techniques. |
Advanced Techniques and Optimizations
Beyond Basic Methods
When it comes to finding the smallest elements in a list, there are several advanced techniques that can help improve efficiency. Here are some methods:
- Using Heaps: Heaps are a special tree-based data structure that can help efficiently find the smallest elements without sorting the entire list.
- Numpy Arrays: If you’re working with large datasets, using Numpy can speed up the process significantly due to its optimized functions.
- Custom Algorithms: Sometimes, creating a tailored algorithm can yield better performance for specific types of data.
Optimizing for Large Lists
For larger lists, consider the following strategies:
- Chunk Processing: Break the list into smaller chunks and process each chunk separately.
- Parallel Processing: Utilize multiple cores of your CPU to handle different parts of the list simultaneously.
- Memory Management: Ensure that your program uses memory efficiently to avoid slowdowns.
Future Trends in List Processing
As technology evolves, so do the methods for processing lists. Here are some trends to watch:
- Machine Learning: Algorithms that learn from data can optimize the search for smallest elements.
- Distributed Computing: Using cloud resources to handle large datasets can improve performance.
- New Libraries: Keep an eye on emerging Python libraries that may offer better performance or new features.
In the world of programming, optimization is key. Always look for ways to improve your code’s efficiency and performance.
If you’re ready to take your coding skills to the next level, visit our website today! We offer interactive tutorials that make learning fun and effective. Don’t miss out on the chance to boost your problem-solving abilities and ace your coding interviews!
Conclusion
In conclusion, finding the smallest elements in a list without sorting is quite doable in Python. We explored several methods, including using built-in functions like min()
, the reduce()
function, and even the heapq
module. Each method has its own advantages, depending on the situation. By understanding these techniques, you can efficiently tackle problems involving lists and enhance your coding skills. Remember, practice makes perfect, so try these methods out in your own projects!
Frequently Asked Questions
What is the main goal of this guide?
The guide aims to teach you how to find multiple smallest numbers in a list using Python without sorting the list.
Why shouldn’t I just sort the list?
Sorting can take more time, especially with large lists. There are faster methods to find the smallest elements.
What Python features can I use to find the smallest elements?
You can use functions like min(), heapq, enumerate(), and even recursion to find the smallest elements.
How does the heap data structure help?
Heaps allow you to efficiently find the smallest element without sorting the entire list.
What is the reduce function?
The reduce function helps apply a function cumulatively to the items in a list, allowing you to find the smallest element.
Can I find the indices of the smallest elements?
Yes! There are methods to find the positions of the smallest elements in the list as well.
How do I handle duplicates in the list?
You can use sets or other strategies to manage duplicates when finding the smallest elements.
What are some common mistakes to avoid?
Avoid assuming that the smallest element is unique; also, be careful with how you handle lists with duplicates.