How to Save Width of a Div in Variable CSS: A Comprehensive Guide
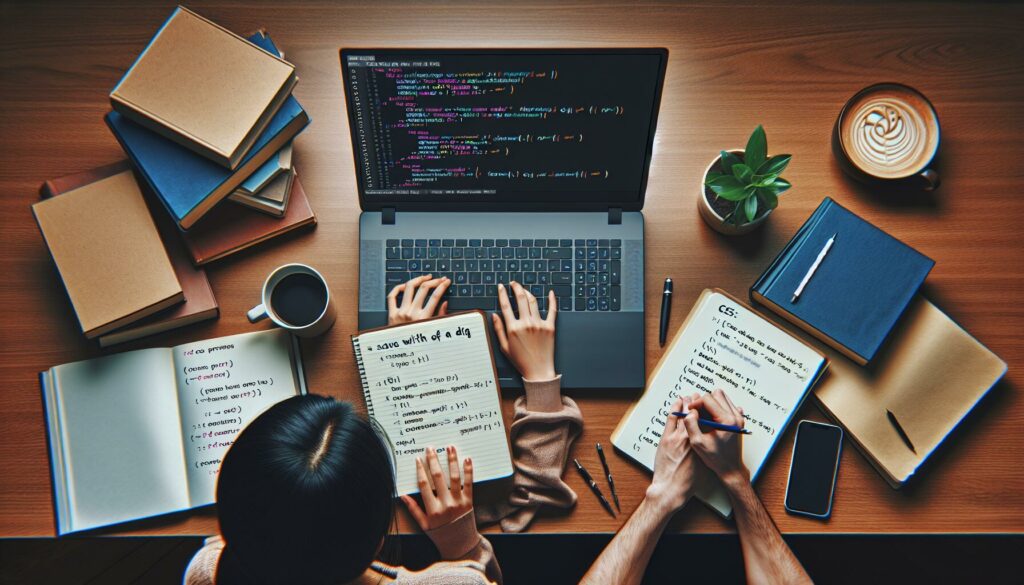
In this guide, we will explore how to effectively save the width of a div using CSS variables. CSS variables allow for more flexible and dynamic styling, making it easier to manage and adjust widths across different screen sizes and layouts. Whether you’re a beginner or looking to refine your skills, this guide will provide you with essential knowledge and practical examples to enhance your web design projects.
Key Takeaways
- CSS variables are defined using a specific syntax, making it easy to reuse values throughout your styles.
- The calc() function allows for dynamic calculations, combining different units for more responsive designs.
- Using media queries with CSS variables helps create responsive designs that adapt to different screen sizes.
- Fallback values can be set for CSS variables, ensuring styles work even if a variable is not defined.
- Browser support for CSS variables is strong, but older browsers may require polyfills for compatibility.
Understanding CSS Variables
What Are CSS Variables?
CSS variables, also known as custom properties, allow you to define a value in one place and use it in multiple locations. This makes it easier to manage your styles and ensures consistency across your design. For example, you can set a primary color once and use it throughout your CSS.
How to Declare CSS Variables
To create a CSS variable, you start with two dashes followed by the variable name. Here’s how you can declare a variable:
:root {
--main-color: blue;
}
You can then use this variable in your styles like this:
h1 {
color: var(--main-color);
}
Scope of CSS Variables
CSS variables have a specific scope. If you declare a variable inside a selector, it will only be available to that selector and its children. For example:
.container {
--padding: 20px;
}
In this case, --padding
is only available to elements inside .container
. If you want a variable to be available everywhere, declare it in the :root
.
CSS variables help reduce repetition and make your code cleaner.
Summary
- CSS variables help maintain consistency.
- They are declared with a special syntax.
- Their scope can be limited to specific elements.
By understanding these basics, you can effectively use CSS variables to enhance your web design.
Using CSS Variables for Width
Setting Width with CSS Variables
CSS variables, also known as custom properties, allow you to define a width that can be reused throughout your styles. To set a width using a CSS variable, you can declare it like this:
:root {
--my-width: 100px;
}
.container {
width: var(--my-width);
}
This way, if you need to change the width later, you only have to update it in one place.
Combining Width and Other Properties
You can also combine width with other CSS properties. For example:
.container {
width: var(--my-width);
padding: 10px;
margin: auto;
}
This allows for a more flexible layout. Here are some benefits of using CSS variables for width:
- Easier maintenance: Change the width in one spot.
- Consistency: Use the same width across multiple elements.
- Dynamic updates: Change widths with JavaScript easily.
Fallback Values for Width
Sometimes, a CSS variable might not be set. In such cases, you can provide a fallback value. For example:
.container {
width: var(--my-width, 50px);
}
This means if --my-width
is not defined, the width will default to 50px
.
Using CSS variables can greatly simplify your styles and make them more adaptable. They are a powerful tool for modern web design.
The Role of calc() in CSS
Introduction to calc() Function
The calc() function in CSS allows you to perform calculations when setting property values. This means you can mix different units like percentages and pixels in a single value. For example, you can set a width like this: width: calc(100% - 20px);
. This is useful for creating flexible layouts that adapt to different screen sizes.
Using calc() for Width Calculations
When you want to set the width of an element, you can use the calc() function to combine different units. Here are some key points to remember:
- You can use operators like
+
,-
,*
, and/
. - Always include units for addition and subtraction.
- Multiplication and division should have unitless numbers.
Here’s a simple example:
.box {
width: calc(50% - 10px);
}
This sets the width to half of the parent element minus 10 pixels.
Common Mistakes with calc()
While using calc(), there are some common mistakes to avoid:
- Forgetting to include units when required.
- Not using spaces around
+
and-
operators. - Trying to divide by zero, which will cause an error.
Remember, the calc() function is a powerful tool that can help you create responsive designs. It allows for more flexibility in your CSS, making it easier to adjust layouts without hardcoding values.
By understanding how to use the calc() function effectively, you can enhance your web designs and make them more adaptable to various screen sizes and resolutions.
Implementing Responsive Design
Media Queries with CSS Variables
Media queries are essential for creating responsive designs. They allow you to apply different styles based on the screen size. Here are some key points:
- Use
@media
rules to define styles for various screen sizes. - Combine CSS variables with media queries for flexible designs.
- Test your design on multiple devices to ensure usability.
Viewport Units and Width
Viewport units like vw
and vh
are useful for setting widths that adapt to the screen size. For example:
1vw
equals 1% of the viewport width.- This allows elements to resize dynamically as the viewport changes.
- Using viewport units can help maintain proportions across devices.
Responsive Width Adjustments
To adjust widths responsively, consider the following:
- Use CSS variables to define base widths.
- Implement
calc()
to combine fixed and flexible units. - Regularly check your layout on different screen sizes to ensure it looks good everywhere.
Responsive web design (RWD) is a web design approach to make web pages render well on all screen sizes and resolutions while ensuring good usability.
By following these guidelines, you can create a layout that looks great on any device!
Advanced Width Calculations
Nested calc() Functions
Using nested calc() functions can help you create more complex width calculations. For example, you can combine multiple calculations to achieve a desired layout:
width: calc(calc(100% / 3) - 20px);
This allows for more flexibility in your designs, especially when working with responsive layouts.
Combining calc() with Other Functions
You can also combine calc()
with other CSS functions to enhance your styles. Here are some examples:
- Using CSS Variables:
width: calc(var(--base-width) + 10px);
- Viewport Units:
width: calc(50vw - 20px);
- Percentage and Fixed Values:
width: calc(100% - 50px);
Dynamic Width Adjustments
Dynamic width adjustments can be achieved by using CSS variables and the calc()
function together. This is particularly useful for creating layouts that adapt to different screen sizes. Here’s how:
- Declare a CSS variable for your base width:
:root { --base-width: 300px; }
- Use calc() to adjust the width dynamically:
.box { width: calc(var(--base-width) + 20px); }
- Combine with media queries to change the variable based on screen size.
Using calc() effectively can greatly enhance your CSS capabilities, allowing for more flexible designs that adapt to various conditions.
By mastering these advanced techniques, you can create layouts that are not only visually appealing but also functional across different devices and screen sizes.
Browser Compatibility
Supported Browsers for CSS Variables
CSS variables, also known as custom properties, are widely supported across modern browsers. Here’s a quick overview of their compatibility:
Browser | Version Supported |
---|---|
Chrome | 49+ |
Firefox | 31+ |
Edge | 16+ |
Safari | 9+ |
Opera | 36+ |
Chrome (Android) | 49+ |
Firefox (Android) | 31+ |
Opera (Android) | 36+ |
Safari (iOS) | 9+ |
Handling Incompatible Browsers
While most modern browsers support CSS variables, some older versions do not. Here are a few strategies to handle this:
- Use fallback values: Always provide a fallback for browsers that do not support CSS variables.
- Conditional CSS: Use the
@supports
rule to apply styles only if the browser supports custom properties. - Polyfills: Consider using polyfills to add support for older browsers.
Polyfills and Workarounds
If you need to support older browsers, you can use polyfills. Here are some options:
- CSS Custom Properties Polyfill: This script allows you to use CSS variables in browsers that do not support them.
- Graceful Degradation: Design your site to work without CSS variables, ensuring it still looks good in older browsers.
- Progressive Enhancement: Start with a basic design and enhance it with CSS variables for modern browsers.
Remember: Always test your designs in multiple browsers to ensure compatibility and a good user experience.
By understanding browser compatibility, you can effectively use CSS variables in your projects, ensuring a consistent look across different platforms.
Practical Examples
Basic Width Example
Using CSS variables can simplify how you manage widths in your designs. For instance, you can set a variable for the width of a <div>
:
:root {
--div-width: 300px;
}
.my-div {
width: var(--div-width);
}
This makes it easy to change the width later by just updating the variable.
Complex Layouts with Width Variables
When creating complex layouts, CSS variables can help maintain consistency. Here’s how you can use them:
- Define multiple width variables:
- Apply these variables in your layout:
- Adjust the variables as needed for different screen sizes.
Interactive Demos
Interactive demos can show how CSS variables work in real-time. Here’s a simple example:
- Create a button that changes the width of a
<div>
when clicked. - Use JavaScript to modify the CSS variable:
document.querySelector('button').addEventListener('click', function() { document.documentElement.style.setProperty('--div-width', '500px'); });
Using CSS variables allows for dynamic adjustments in your designs, making them more flexible and easier to manage.
Summary Table
Here’s a quick summary of the benefits of using CSS variables for width management:
Benefit | Description |
---|---|
Easy to Update | Change width in one place, affects all elements. |
Consistency | Maintain uniformity across different components. |
Responsive Design | Adjust widths based on media queries easily. |
Debugging Width Issues
Common Width Problems
When working with CSS, you might face several issues related to width. Here are some common problems:
- Unexpected Width: Sometimes, a div may not appear as wide as you expect.
- Overflow Issues: Content might overflow its container, causing layout problems.
- Box Model Confusion: Understanding how padding and borders affect width can be tricky.
Tools for Debugging CSS
To effectively debug CSS width issues, you can use various tools:
- Browser DevTools: Most modern browsers have built-in tools to inspect elements and see their computed styles.
- CSS Reset: Using a CSS reset can help eliminate default browser styles that may interfere with your layout.
- Box Model Visualization: Tools that visualize the box model can help you understand how width, padding, and borders interact.
Best Practices for Troubleshooting
To avoid width issues in your designs, consider these best practices:
- Always set the box-sizing property to
border-box
to include padding and borders in the width calculation. - Use flexbox or grid layouts for more predictable behavior.
- Test your designs in multiple browsers to ensure compatibility.
Remember, debugging CSS can be challenging, but with the right tools and practices, you can resolve width issues effectively.
By following these guidelines, you can minimize width-related problems and create more reliable layouts.
Best Practices for CSS Variables
Organizing Your CSS Variables
When using CSS variables, it’s important to keep them organized. Here are some tips:
- Use clear naming conventions: This helps in understanding what each variable represents.
- Group related variables: For example, keep all color variables together.
- Comment your variables: Adding comments can clarify the purpose of each variable.
Maintaining Consistency
Consistency is key when working with CSS variables. Here are some practices:
- Stick to a naming pattern: This could be based on colors, sizes, or components.
- Use the same units: For example, if you use
rem
for font sizes, use it for spacing too. - Regularly review your variables: This helps in removing unused variables and keeping your code clean.
Performance Considerations
While CSS variables are powerful, consider the following for better performance:
- Limit the number of variables: Too many can slow down rendering.
- Avoid complex calculations: Use simple values when possible.
- Test across browsers: Ensure your variables work well in all supported browsers.
Remember: CSS variables can greatly enhance your stylesheets, but they need to be used wisely to avoid confusion and performance issues.
Highlighted Tip
When naming your CSS variables, consider using semantic naming. This makes it easier to understand their purpose at a glance. For example, instead of --color1
, use --primary-color
to clearly indicate its use.
Case Studies
Real-World Examples
In this section, we will explore how different projects have successfully implemented CSS variables to manage widths effectively. Here are some notable examples:
- E-commerce Websites: Many online stores use CSS variables to adjust the width of product cards based on screen size, ensuring a smooth shopping experience.
- Portfolio Sites: Designers often utilize CSS variables to create flexible layouts that adapt to various devices, showcasing their work beautifully.
- Web Applications: Applications like dashboards use CSS variables to maintain consistent widths across different components, enhancing usability.
Lessons Learned
From these case studies, we can draw several important lessons:
- Flexibility: CSS variables allow for easy adjustments without rewriting code.
- Maintainability: Using variables makes it simpler to manage styles across large projects.
- Performance: Reducing redundancy in CSS can lead to faster load times.
Future Trends in CSS Variables
As web design continues to evolve, CSS variables are expected to play a crucial role in future developments. Here are some trends to watch:
- Increased Adoption: More developers will embrace CSS variables for their flexibility.
- Integration with Frameworks: Frameworks like Tailwind CSS will likely enhance their support for CSS variables, making them even more accessible.
- Dynamic Styling: The combination of CSS variables with JavaScript will lead to more interactive and responsive designs.
CSS variables are not just a trend; they are becoming a standard in modern web design. Their ability to simplify and enhance styles is invaluable.
Integrating JavaScript with CSS Variables
Accessing CSS Variables in JavaScript
Integrating JavaScript allows for dynamic adjustments of CSS variables at runtime. This is particularly powerful for creating interactive and responsive user experiences. Here’s how you can access and modify CSS variables:
- Set a Variable Value: You can change a CSS variable using JavaScript like this:
const element = document.getElementById('my-element'); element.style.setProperty('--variable-name', 'new-value');
- Get a Variable Value: To retrieve the value of a CSS variable, use:
const styles = getComputedStyle(document.documentElement); const value = styles.getPropertyValue('--variable-name').trim();
- Scope Matters: Remember that the scope of the variable affects where it can be accessed. If defined in a specific element, it won’t be available outside that element.
Dynamic Width Adjustments with JavaScript
You can also use JavaScript to make dynamic width adjustments. For example, if you want to change the width of a div based on user input or screen size, you can do it like this:
- Listen for Events: Use event listeners to detect changes (like window resizing or input changes).
- Update CSS Variables: Change the width variable based on the event.
- Repaint the Element: The browser will automatically repaint the element with the new width.
Using JavaScript with CSS variables opens up a world of possibilities for creating responsive designs that adapt to user interactions.
Interactive Applications
Integrating CSS variables with JavaScript can lead to interactive applications. Here are some ideas:
- Theme Switchers: Change colors or layouts based on user preferences.
- Dynamic Layouts: Adjust widths and heights based on user actions.
- Real-Time Updates: Reflect changes instantly without needing to reload the page.
By combining CSS variables and JavaScript, you can create a more engaging and responsive user experience.
If you’re looking to enhance your coding skills, integrating JavaScript with CSS variables is a great way to start! This combination allows you to create dynamic and responsive designs effortlessly. Ready to take your coding journey to the next level? Visit our website to start coding for free today!
Conclusion
In summary, using CSS variables to manage the width of a div can make your web design much easier and more flexible. By defining a variable for the width, you can quickly change it in one place and see the effects throughout your site. This not only saves time but also keeps your code cleaner and more organized. Remember to use the calc()
function when you need to do math with your values, and always check for browser support to ensure your designs work everywhere. With these tips, you’ll be well on your way to mastering CSS variables and creating responsive layouts.
Frequently Asked Questions
What are CSS variables and why should I use them?
CSS variables are special values that you can define and reuse throughout your styles. They help make your CSS cleaner and easier to manage.
How do I create a CSS variable?
You can create a CSS variable by using two dashes before the name, like this: `–my-variable: value;`.
Can I use CSS variables in media queries?
Yes! CSS variables can be used inside media queries, which means you can change values based on the size of the screen.
What is the `calc()` function in CSS?
The `calc()` function lets you do math with your CSS values. You can add, subtract, multiply, or divide values to create dynamic sizes.
Are CSS variables supported in all browsers?
Most modern browsers support CSS variables. However, older browsers like Internet Explorer do not.
How do I set a fallback value for a CSS variable?
You can set a fallback value by using the `var()` function with a second argument, like this: `width: var(–my-variable, fallback-value);`.
Can I change the value of a CSS variable with JavaScript?
Yes! You can easily change the value of CSS variables using JavaScript, making your styles dynamic.
What are some best practices for using CSS variables?
It’s best to organize your CSS variables clearly, use meaningful names, and keep them consistent to make your styles easy to read.