Understanding appendTo: Self vs Body in Web Development
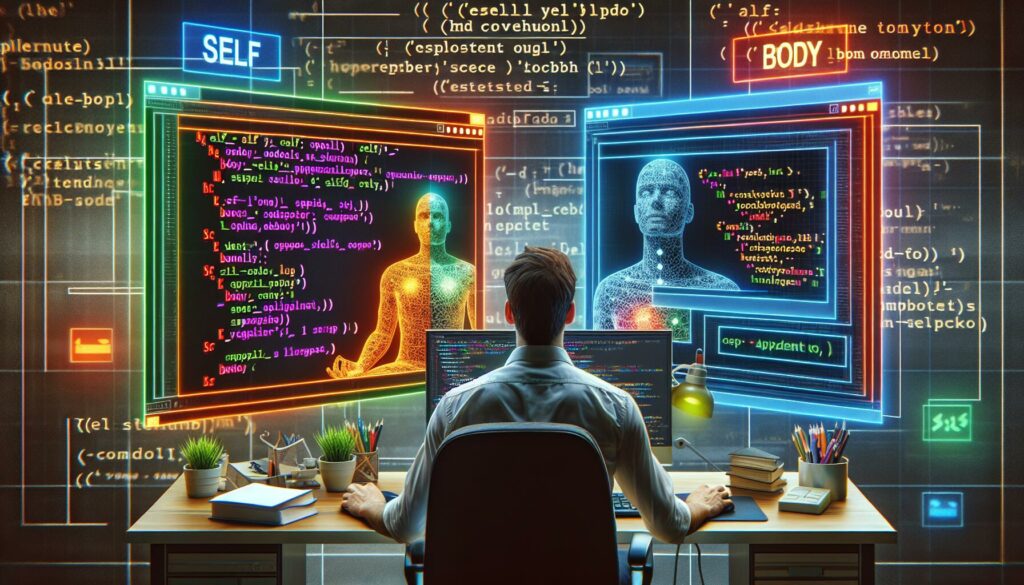
In the world of web development, understanding how to effectively manipulate the Document Object Model (DOM) is crucial. One key method that developers often encounter is `appendTo`. This article will explore the differences between using `appendTo` with ‘self’ and ‘body’, helping you make informed choices in your coding practices.
Key Takeaways
- `appendTo` allows you to add elements to the DOM easily.
- Using `self` means appending to the current element, while `body` adds to the main page body.
- Choosing the right method can improve your site’s performance.
- Security is important; always sanitize inputs when using `appendTo`.
- Avoid common mistakes to ensure smooth DOM manipulation.
Introduction to appendTo in Web Development
What is appendTo?
The appendTo method is a powerful tool in web development, especially when working with jQuery. It allows developers to insert HTML elements at the end of selected elements in the Document Object Model (DOM). This method is particularly useful for dynamically adding content to a webpage without needing to reload it.
Common Use Cases for appendTo
Here are some common scenarios where appendTo is beneficial:
- Adding new items to a list or gallery.
- Inserting user-generated content, like comments or reviews.
- Dynamically updating sections of a webpage based on user interactions.
Benefits of Using appendTo
Using appendTo has several advantages:
- Simplicity: It simplifies the process of adding elements to the DOM.
- Flexibility: You can easily append multiple elements at once.
- Efficiency: It reduces the amount of code needed for DOM manipulation.
The appendTo method is essential for creating interactive web applications that respond to user actions in real-time.
In summary, understanding how to use appendTo effectively can greatly enhance your web development skills, making it easier to manage and manipulate the DOM. This method is a key part of modern web development practices, allowing for more dynamic and engaging user experiences.
Highlighted Context
The insertAdjacentElement() method is another useful tool for inserting elements in relation to existing ones, providing even more flexibility in DOM manipulation.
Understanding the Basics of DOM Manipulation
What is the DOM?
The Document Object Model (DOM) is a programming interface that allows scripts to update the content, structure, and style of a document. It represents the document as a tree of objects, where each node corresponds to a part of the document. This makes it easier to manipulate web pages dynamically.
How JavaScript Interacts with the DOM
JavaScript can interact with the DOM in various ways, such as:
- Selecting elements using methods like
getElementById
orquerySelector
. - Modifying elements by changing their attributes, styles, or content.
- Creating new elements and adding them to the document.
Common Methods for DOM Manipulation
Here are some common methods used for manipulating the DOM:
Method | Description |
---|---|
appendChild() |
Adds a new child node to a specified parent node. |
removeChild() |
Removes a specified child node from a parent node. |
innerHTML |
Gets or sets the HTML content of an element. |
The DOM is essential for creating interactive web pages. Understanding how to manipulate it is crucial for web development. In this article, we’ll look at how to use the DOM in detail and explore various APIs that can enhance your web applications.
appendTo Self vs Body: Key Differences
Definition of Self and Body in appendTo
In web development, appendTo can target two main areas: Self and Body.
- Self refers to the element that is currently being manipulated.
- Body refers to the
<body>
tag, which contains all the visible content of an HTML document, such as headings, images, hyperlinks, tables, lists, paragraphs, etc.
When to Use Self
Using appendTo Self is beneficial when:
- You want to add elements directly to a specific component.
- You need to maintain the structure of a particular section without affecting the entire page.
- You are working with dynamic content that should only appear in a specific area.
When to Use Body
On the other hand, appendTo Body is useful when:
- You want to add elements that should be visible on the entire page.
- You are creating global notifications or alerts that need to be seen regardless of the current view.
- You need to ensure that the elements are part of the main document flow.
Feature | appendTo Self | appendTo Body |
---|---|---|
Scope | Limited to the specific element | Affects the entire document |
Use Case | Localized updates | Global notifications |
Performance | Generally faster for small updates | Can be slower with many elements |
Understanding when to use appendTo Self versus Body can greatly enhance your web development skills. It allows for better control over where elements are placed in the DOM, leading to cleaner and more efficient code.
Practical Examples of appendTo Self
Appending Elements to Self
When using appendTo
with self, you are adding elements to the element itself rather than to the body of the document. This can be useful for creating dynamic interfaces. Here’s how you can do it:
- Select the Element: Choose the element you want to append to.
- Create New Elements: Use JavaScript to create new elements.
- Append to Self: Use the
appendTo
method to add the new elements.
For example:
$('#myDiv').append('<p>New Paragraph</p>');
This code adds a new paragraph inside the myDiv
element.
Common Scenarios for appendTo Self
Using appendTo
with self is great for:
- Dynamic Content: Adding content based on user actions.
- Interactive Elements: Creating elements that respond to events.
- Modular Design: Keeping related elements together.
Code Snippets for appendTo Self
Here are some code snippets to illustrate how to use appendTo
with self:
// Appending a list to a div
$('#myList').append('<li>Item 1</li>');
// Appending an image to a section
$('#mySection').append('<img src="image.jpg" alt="My Image">');
// Appending a button to a form
$('#myForm').append('<button type="submit">Submit</button>');
In these examples, elements are added directly to their respective parents, enhancing the structure of your web page. Unlock the power of Laravel Eloquent by using methods like appendTo
to streamline your code and improve user experience.
Practical Examples of appendTo Body
Appending Elements to Body
Appending elements to the body of a webpage is a common practice in web development. This method allows developers to dynamically add content to the page. Using the body for appending is essential for proper layout and functionality.
Common Scenarios for appendTo Body
Here are some situations where appending to the body is useful:
- Loading new content: When you want to display new information without refreshing the page.
- Creating interactive elements: Such as buttons or forms that users can interact with.
- Displaying notifications: For alerts or messages that need to be visible to the user.
Code Snippets for appendTo Body
Here’s a simple example of how to append a new element to the body:
function append() {
const box = document.createElement('div');
box.style.backgroundColor = '#F00';
box.style.width = '50px';
box.style.height = '50px';
document.body.appendChild(box);
}
In this code, a red box is created and added to the body when the function is called. This demonstrates how easy it is to manipulate the DOM using JavaScript.
Appending elements directly to the body can enhance user experience by making the page more dynamic and interactive.
Summary
Using appendTo
with the body is a powerful tool in web development. It allows for flexible and dynamic content management, making it easier to create engaging user experiences. Remember, the document can only have one body, so appending to it is a straightforward choice for many applications.
Performance Considerations for appendTo
Speed of appendTo Self vs Body
When using appendTo
, the speed can vary based on whether you are appending to self or body. Appending to self is generally faster because it modifies the existing element directly, while appending to the body may involve more reflows and repaints in the browser.
Memory Usage
Memory usage is another important factor. Appending elements to the body can lead to higher memory consumption, especially if many elements are added at once. Here’s a quick comparison:
Method | Memory Usage | Speed |
---|---|---|
appendTo Self | Low | Fast |
appendTo Body | High | Slower |
Best Practices for Optimal Performance
To ensure optimal performance when using appendTo
, consider the following best practices:
- Batch DOM updates: Instead of appending elements one by one, gather them and append them all at once.
- Use Document Fragments: This allows you to build a DOM structure in memory before adding it to the document.
- Minimize reflows: Try to limit the number of times the browser has to re-calculate styles and layouts.
Efficient memory usage and faster performance are crucial when dealing with large string operations.
By following these guidelines, you can enhance the performance of your web applications significantly.
Security Implications of Using appendTo
Potential Security Risks
When using appendTo
, there are some serious security risks to consider. One major concern is Cross-Site Scripting (XSS), where attackers can inject malicious scripts into your web application. This can happen if you append untrusted content directly to the DOM. Here are some common risks:
- XSS Attacks: Malicious scripts can be executed in the user’s browser.
- Data Leakage: Sensitive information can be exposed if not handled properly.
- User Manipulation: Attackers can manipulate user sessions or data.
How to Mitigate Security Issues
To protect your application, consider these strategies:
- Sanitize Input: Always sanitize any user input before appending it to the DOM.
- Use Safe Methods: Prefer methods like
textContent
overinnerHTML
to avoid parsing HTML. - Content Security Policy (CSP): Implement CSP to restrict the sources of content that can be loaded.
Best Practices for Secure Code
Following best practices can help you avoid security pitfalls:
- Validate Input: Ensure that all input is validated and sanitized.
- Limit Permissions: Restrict what scripts can do in your application.
- Regular Security Audits: Conduct regular audits to identify and fix vulnerabilities.
Remember: Always be cautious when appending content to the DOM. Using innerHTML can lead to vulnerabilities that may compromise your application.
Common Pitfalls and How to Avoid Them
Mistakes to Avoid with appendTo
When using appendTo
, developers often encounter several common mistakes. Here are some pitfalls to watch out for:
- Overusing appendTo: Using
appendTo
excessively can lead to performance issues. Instead, consider using it only when necessary. - Ignoring the context: Not understanding the difference between appending to
self
andbody
can cause unexpected results. Always clarify your target. - Neglecting event listeners: When you append elements, existing event listeners may be lost. Ensure you reattach them if needed.
Debugging Tips
If you run into issues while using appendTo
, here are some helpful debugging tips:
- Check the console: Look for any errors in the browser’s console that might indicate what went wrong.
- Use breakpoints: Set breakpoints in your code to see where it might be failing.
- Log outputs: Use
console.log
to track the flow of your code and the state of your variables.
Tools for Testing and Validation
To ensure your code is working correctly, consider using the following tools:
- Browser Developer Tools: Inspect elements and monitor network requests.
- Linting Tools: Use tools like ESLint to catch errors in your JavaScript code.
- Unit Testing Frameworks: Implement frameworks like Jest to test your functions and methods.
Remember: Avoiding these common pitfalls can save you time and frustration in your web development projects. Understanding how to use appendTo effectively is key to building efficient and reliable applications.
In summary, being aware of these mistakes and employing good debugging practices will help you use appendTo
more effectively in your projects. Always validate your code to ensure it behaves as expected!
Advanced Techniques for Using appendTo
Combining appendTo with Other Methods
Using appendTo
can be enhanced by combining it with other methods. Here are some techniques:
- Chaining Methods: You can chain
appendTo
with other jQuery methods for more complex operations. - Event Handling: Attach event listeners right after appending elements to ensure they work as expected.
- Dynamic Content: Use
appendTo
to add elements that are generated based on user input or other dynamic data.
Dynamic Content Loading
Dynamic content loading is a powerful feature in web development. Here’s how you can implement it:
- Fetch Data: Use AJAX to get data from a server.
- Create Elements: Generate HTML elements based on the fetched data.
- Append to DOM: Use
appendTo
to add these elements to the desired location in the DOM.
Real-World Use Cases
Here are some practical scenarios where appendTo
shines:
- User Comments: Dynamically add user comments to a post without refreshing the page.
- Image Galleries: Load images into a gallery as users scroll down.
- Shopping Carts: Add items to a shopping cart interface seamlessly.
Remember: Using appendTo effectively can greatly enhance user experience by making your web applications more interactive and responsive.
In summary, mastering appendTo
and its advanced techniques can lead to more efficient and dynamic web applications. Learn three essential methods—innerHTML, insertAdjacentHTML(), and appendChild()—to seamlessly append and manipulate HTML content using JavaScript.
Comparing appendTo with Other Methods
appendTo vs appendChild
The appendTo
method is often compared to the appendChild
method. Here are some key differences:
- Usage:
appendTo
is a jQuery method, whileappendChild
is a native JavaScript method. - Functionality:
appendTo
can insert HTML strings, whereasappendChild
only works with node objects. - Return Value:
appendTo
returns the jQuery object, allowing for method chaining, whileappendChild
returns the appended node.
appendTo vs innerHTML
When comparing appendTo
with innerHTML
, consider the following:
- Performance: Using
innerHTML
can be slower because it re-parses the entire HTML content of an element. - Security:
innerHTML
can expose your application to XSS attacks if not used carefully. In contrast,appendTo
is generally safer. - Flexibility:
appendTo
allows for more complex DOM manipulations, whileinnerHTML
is limited to string replacements.
When to Use Each Method
Here’s a quick guide on when to use each method:
- Use
appendTo
when you need to insert HTML elements dynamically and want to maintain jQuery’s chaining capabilities. - Use
appendChild
for performance-sensitive applications where you are only working with node objects. - Use
innerHTML
for simple cases where you need to replace the entire content of an element, but be cautious of security risks.
In summary, choosing the right method depends on your specific needs and the context of your application. Understanding the differences can help you make better decisions in your web development projects.
Summary Table
Method | Type | Security Risk | Performance | Flexibility |
---|---|---|---|---|
appendTo | jQuery | Low | Moderate | High |
appendChild | Native JS | Low | High | Moderate |
innerHTML | Native JS | High | Low | Low |
Future Trends in DOM Manipulation
Emerging Technologies
The landscape of web development is constantly evolving. Here are some key trends to watch:
- Web Components: These allow developers to create reusable custom elements, enhancing modularity.
- Frameworks: Libraries like React and Vue are changing how we think about the DOM.
- Server-Side Rendering: This improves performance and SEO by rendering pages on the server before sending them to the client.
Predictions for the Future
As we look ahead, several predictions can be made:
- Increased Use of Web Components: With their modular nature, they will likely become a standard in web development.
- More Focus on Performance: Developers will prioritize speed and efficiency in DOM manipulation.
- Enhanced Security Measures: As vulnerabilities are discovered, better practices will emerge to protect against threats.
How appendTo Fits into Future Trends
appendTo will continue to play a vital role in DOM manipulation. It offers a straightforward way to add elements dynamically. As web components gain traction, the use of appendTo will likely evolve to support these new technologies.
Web components represent a significant advancement in web development, offering a modular, reusable, and encapsulated approach to building web applications.
As we look ahead, the world of DOM manipulation is evolving rapidly. New tools and techniques are emerging, making it easier for developers to create dynamic and interactive web experiences. If you’re eager to stay ahead in this fast-paced field, visit our website to start your coding journey today!
Conclusion
In summary, understanding the difference between using appendTo
with self
and body
is crucial for web developers. When you append to the body
, you are directly adding elements to the main part of your webpage, which is often what you want. On the other hand, appending to self
can be useful for more specific tasks, like attaching elements to a certain part of your layout. Always remember that choosing the right method can make your code cleaner and your website more efficient. As you continue to learn and grow in web development, keep experimenting with these methods to see how they can best serve your projects.
Frequently Asked Questions
What does appendTo do in web development?
appendTo is a method used to add new elements to a specific part of a webpage, either to the body or another element.
When should I use appendTo ‘self’?
You should use appendTo ‘self’ when you want to add content to an element itself, rather than to the body of the document.
What are some common uses for appendTo?
Common uses include adding menus, pop-ups, or any dynamic content to your webpage.
How does appendTo differ from appendChild?
appendTo can add elements to any selected part of the DOM, while appendChild specifically adds elements to the body or a parent node.
Are there any performance issues with using appendTo?
Using appendTo can affect performance, especially if you append many elements at once. It’s best to minimize DOM updates.
What security risks come with using appendTo?
If you add content from untrusted sources, it could lead to security issues like XSS (Cross-Site Scripting) attacks.
What mistakes should I avoid with appendTo?
Avoid appending too many elements at once, and always sanitize content from external sources.
How can I test my appendTo code?
You can use browser developer tools to debug and validate your appendTo implementations.