Mastering Python Replace: A Comprehensive Guide to String Manipulation
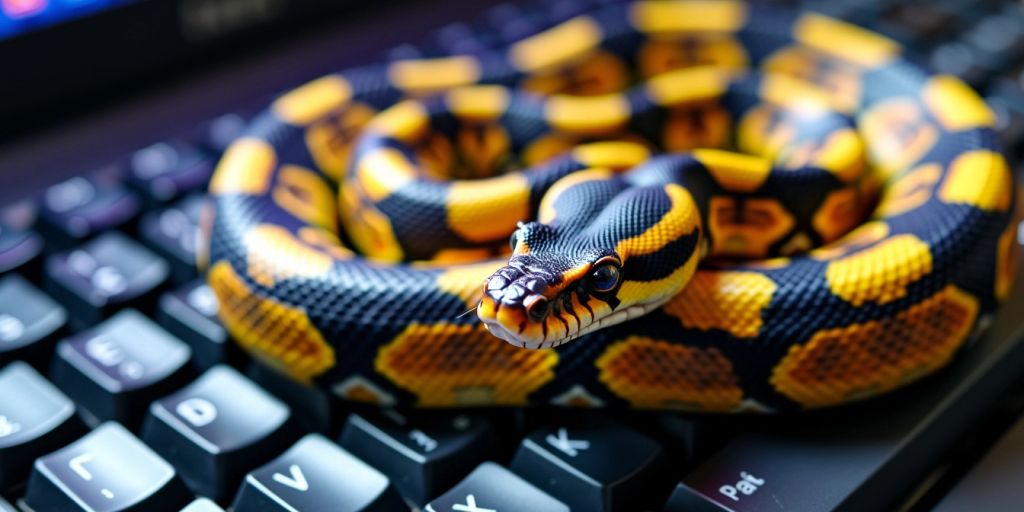
In this guide, we will explore how to effectively use the Python replace method for string manipulation. Whether you’re cleaning up data, formatting text, or performing complex replacements, understanding this method is key. We will break down the basics and dive into advanced techniques, all while providing practical examples to help you grasp these concepts easily.
Key Takeaways
- Python’s replace method allows you to change specific parts of a string easily.
- You can replace multiple substrings at once by chaining replace calls.
- Using regular expressions can enhance your ability to perform complex replacements.
- It’s important to consider performance when dealing with large strings or many replacements.
- Combining replace with other string methods can lead to more efficient code.
Understanding the Basics of Python Replace
What is Python Replace?
The replace() method in Python is a powerful tool for changing parts of a string. It allows you to substitute one substring with another, making it essential for text manipulation. For example, if you have a sentence and want to change a word, you can easily do that using this method.
Syntax and Parameters
The syntax for the replace method is:
string.replace(old, new, count)
- old: The substring you want to replace.
- new: The substring you want to use as a replacement.
- count (optional): The number of times you want to replace the substring. If not specified, all occurrences will be replaced.
Basic Examples
Here are some simple examples to illustrate how the replace method works:
- Basic Replacement:
- Replacing Multiple Occurrences:
- Using Count Parameter:
In summary, the replace method is a straightforward yet powerful way to manipulate strings in Python. It can be used in various scenarios, such as data cleaning and text normalization.
Remember, when using the replace method, it’s important to consider the context of your text to avoid unintended changes.
Additionally, the pandas dataframe.replace() function is used to replace strings, regex, lists, dictionaries, series, numbers, etc., from a pandas dataframe in Python. This makes it a versatile tool for data manipulation in data science.
Advanced Techniques with Python Replace
Replacing Multiple Substrings
When you need to replace several different substrings in a single operation, you can use a loop or a dictionary. Here’s how:
- Using a loop:
- Using a dictionary:
Using Regular Expressions
Regular expressions (regex) provide a powerful way to perform complex replacements. The re.sub()
function allows you to:
- Match patterns in strings.
- Replace matched patterns with new text.
- Use special characters to define your search criteria.
Note: The differences between .replace()
and .re.sub()
methods are significant. The .replace()
method is straightforward, while .re.sub()
offers more flexibility for complex patterns.
Case-Insensitive Replacements
To perform replacements without worrying about letter case, you can convert both the original string and the substring to the same case:
- Use
lower()
orupper()
methods to standardize the case before replacing. - This ensures that all instances, regardless of their case, are replaced.
text = "Hello World"
new_text = text.replace("hello", "Hi").lower()
In summary, mastering these advanced techniques will enhance your string manipulation skills in Python, making your code more efficient and versatile.
Common Use Cases for Python Replace
Data Cleaning and Preparation
Data cleaning is essential in programming. The replace() function in Python helps in removing unwanted characters or correcting typos. Here are some common tasks:
- Removing special characters: Use
replace()
to eliminate unwanted symbols. - Correcting common typos: Quickly fix frequent mistakes in text data.
- Standardizing formats: Ensure consistency in data entries.
Text Normalization
Text normalization makes data uniform. This is crucial for analysis. Here are some techniques:
- Lowercasing: Convert all text to lowercase for uniformity.
- Whitespace removal: Use
replace()
to eliminate extra spaces. - Punctuation removal: Clean up text by removing unnecessary punctuation.
Template Processing
In template processing, the replace()
function is invaluable. It allows for dynamic content generation. Here’s how:
- Dynamic text replacement: Insert user-specific data into templates.
- Generating reports: Create customized reports by replacing placeholders with actual data.
- Email templates: Personalize emails by replacing names and other details.
Mastering the use of the replace() function in Python can significantly enhance your data manipulation skills. It allows for efficient and effective text handling, making your programming tasks easier and more productive.
Use Case | Description |
---|---|
Data Cleaning | Remove unwanted characters and correct typos. |
Text Normalization | Standardize text for analysis. |
Template Processing | Generate dynamic content in templates. |
Performance Considerations for Python Replace
When using the replace()
method in Python, it’s important to think about how it affects your program’s performance. Here are some key points to consider:
Efficiency of Replace Operations
- Speed: The
replace()
method is generally fast for small strings but can slow down with larger texts. - Complexity: The time complexity is O(n), where n is the length of the string. This means it processes each character in the string.
- Best Use Cases: Use
replace()
for straightforward replacements, especially when dealing with short strings.
Memory Usage
- Temporary Strings: Each call to
replace()
creates a new string, which can lead to high memory usage if done repeatedly. - Garbage Collection: Python’s garbage collector will clean up unused strings, but it’s still wise to minimize unnecessary replacements.
- Example: Replacing values in a list can lead to multiple temporary strings being created, which can be inefficient.
Optimizing Replace for Large Texts
- Batch Processing: If you need to replace multiple substrings, consider processing them in batches to reduce the number of calls to
replace()
. - Regular Expressions: For complex patterns, using the
re
module can be more efficient than multiplereplace()
calls. - Profile Your Code: Use profiling tools to identify bottlenecks in your string manipulation code.
In summary, understanding the performance implications of the replace() method can help you write more efficient Python code. Always consider the size of your data and the frequency of replacements to optimize your approach.
By keeping these performance considerations in mind, you can ensure that your string manipulation tasks are both effective and efficient.
Handling Special Characters in Python Replace
When working with strings in Python, you may encounter special characters that need to be replaced. Understanding how to handle these characters is crucial for effective string manipulation.
Replacing Newlines and Tabs
Special characters like newlines (\n
) and tabs (\t
) can be replaced using the replace()
method. Here are some examples:
- To replace newlines:
text = "Hello\nWorld" new_text = text.replace("\n", " ") # Replaces newline with a space
- To replace tabs:
text = "Hello\tWorld" new_text = text.replace("\t", " ") # Replaces tab with a space
Dealing with Unicode Characters
Python supports Unicode, allowing you to work with characters from different languages. To replace Unicode characters, simply use the replace()
method:
- Example:
text = "Café" new_text = text.replace("é", "e") # Replaces é with e
Escape Sequences
Escape sequences are used to represent special characters in strings. Here are some common escape sequences:
\n
– Newline\t
– Tab\"
– Double quote\\
– Backslash
Using these escape sequences correctly is essential when replacing characters in strings.
Remember, handling special characters properly can prevent unexpected behavior in your programs!
Combining Python Replace with Other String Methods
Using Replace with Split and Join
Combining the replace() method with other string methods can enhance your text manipulation skills. Here’s how you can do it:
- Split: Use the
split()
method to break a string into a list of substrings. - Replace: Apply the
replace()
method to each substring as needed. - Join: Finally, use the
join()
method to combine the modified substrings back into a single string.
For example:
text = "I like apples and apples are tasty."
modified_text = " and ".join([word.replace("apples", "bananas") for word in text.split(" and ")])
Chaining Multiple String Methods
You can also chain multiple string methods together for more complex operations. This allows for a more concise and readable code. Here’s a simple example:
result = text.strip().replace("apples", "bananas").upper()
This code first removes any extra spaces, replaces "apples" with "bananas", and then converts the entire string to uppercase.
Integrating Replace with String Formatting
String formatting can be combined with the replace()
method to create dynamic strings. For instance:
name = "Alice"
age = 30
message = f"My name is {name} and I like {"bananas".replace("bananas", "apples")}."
This allows you to replace parts of the string while also including variables.
By mastering these combinations, you can create more efficient and readable code. Python’s replace() is a built-in string method that allows you to replace all occurrences of a specified substring with another substring.
Error Handling in Python Replace
Common Errors and Exceptions
When using the replace()
method in Python, you might encounter some common errors. Here are a few:
- TypeError: This occurs if you try to replace a non-string type.
- ValueError: This happens if the arguments provided are not valid.
- AttributeError: This can occur if you try to call
replace()
on a non-string object.
Debugging Replace Operations
To effectively debug your replace operations, consider these steps:
- Check Data Types: Ensure that the variables you are using are strings.
- Print Statements: Use print statements to display the values before and after replacement.
- Try-Except Blocks: Implement try-except blocks to catch and handle exceptions gracefully.
Best Practices for Error Handling
To make your code more resilient, follow these best practices:
- Use Assertions: Assert that your inputs are of the expected type.
- Log Errors: Keep a log of errors for future reference.
- Custom Exceptions: Create custom exceptions to handle specific error cases effectively. In this blog post, we’ll explore how to create and use custom exceptions in Python to enhance error handling and make your code more resilient.
Remember, effective error handling not only makes your code more robust but also improves the user experience by providing clear feedback on what went wrong.
Testing and Debugging Python Replace Code
Writing Unit Tests for Replace
To ensure your replace operations work correctly, writing unit tests is essential. Here are some steps to follow:
- Create test cases for various scenarios, including edge cases.
- Use the
unittest
module to organize your tests. - Run your tests regularly to catch any issues early.
Using Print Statements for Debugging
When things go wrong, print statements can help you understand what’s happening. Here’s how to use them effectively:
- Print the original string before and after the replace operation.
- Show the expected output alongside the actual output.
- Use descriptive messages to clarify what each print statement is showing.
Leveraging Debugging Tools
Using debugging tools can make your life easier. Here are some popular options:
- PDB: Python’s built-in debugger allows you to step through your code.
- IDE Debuggers: Many Integrated Development Environments (IDEs) have built-in debugging tools.
- Logging: Instead of print statements, consider using the
logging
module for more control over your output.
Debugging is a crucial skill for any programmer. It helps you find and fix issues quickly, making your code more reliable.
By following these practices, you can effectively test and debug your replace operations, ensuring your string manipulations are accurate and efficient. Remember, debugging configurations for python apps can vary, so adapt your approach based on your specific needs.
Real-World Examples of Python Replace
Replacing URLs in Text
In many applications, you might need to replace URLs in a string. For instance, if you have a list of URLs that need to be updated, you can use the replace()
method to change them all at once. Here’s a simple example:
text = "Visit us at http://old-url.com"
new_text = text.replace("http://old-url.com", "http://new-url.com")
print(new_text) # Output: Visit us at http://new-url.com
Censoring Sensitive Information
Another common use case is censoring sensitive information like email addresses or phone numbers. You can replace parts of the string with asterisks or other characters to hide the sensitive data:
text = "Contact me at john.doe@example.com"
censored_text = text.replace("john.doe", "*****")
print(censored_text) # Output: Contact me at *****@example.com
Dynamic Content Generation
In web development, you often need to generate dynamic content. Using replace()
, you can easily insert user-specific data into templates:
template = "Hello, {name}! Welcome to {site}."
user_name = "Alice"
site_name = "Python World"
message = template.replace("{name}", user_name).replace("{site}", site_name)
print(message) # Output: Hello, Alice! Welcome to Python World.
In summary, the replace() method is a powerful tool for various real-world applications, from updating URLs to censoring sensitive information and generating dynamic content. By mastering this method, you can enhance your string manipulation skills significantly.
Summary of Use Cases
Here’s a quick summary of the examples discussed:
- Replacing URLs in text
- Censoring sensitive information
- Dynamic content generation
These examples illustrate how versatile the replace()
method can be in practical scenarios, making it an essential tool for any Python developer.
Comparing Python Replace with Other String Manipulation Methods
When working with strings in Python, there are several methods available for manipulating text. Understanding how Python Replace compares to other string methods can help you choose the right tool for your task.
Replace vs. Sub in Regex
- Replace: The
replace()
method is straightforward and replaces all occurrences of a substring with another substring. For example:text = "I like apples" new_text = text.replace("apples", "bananas")
- Sub: The
sub()
function from there
module allows for more complex replacements using regular expressions. This is useful for pattern matching.
Replace vs. Translate
- Replace: This method is great for simple substring replacements.
- Translate: The
translate()
method is more efficient for replacing multiple characters at once. It uses a translation table to map characters to their replacements.
When to Use Replace
- Simple Substitutions: Use
replace()
for straightforward text changes. - Performance: For large texts, consider
translate()
for multiple character replacements. - Pattern Matching: Use
sub()
when you need to match patterns rather than fixed substrings.
In summary, while the replace() method is effective for basic tasks, other methods like sub() and translate() offer more flexibility and efficiency for complex string manipulations. Understanding these differences can enhance your string manipulation skills in Python.
Best Practices for Using Python Replace
Writing Readable Replace Code
When using the replace() method, clarity is key. Here are some tips:
- Use clear variable names to indicate what you are replacing.
- Keep your code organized by breaking complex replacements into smaller steps.
- Add comments to explain why certain replacements are made.
Maintaining Code Consistency
Consistency in your code helps others (and yourself) understand it better. Consider the following:
- Stick to a single style for naming variables and functions.
- Use the same method for replacements throughout your project.
- Regularly review your code to ensure it follows the same patterns.
Documenting Replace Operations
Documentation is essential for future reference. Make sure to:
- Write clear documentation for each function that uses the replace method.
- Include examples of input and output to illustrate how the replace works.
- Keep a changelog if you modify any replace operations.
Remember: Good documentation can save you time and confusion later on.
By following these best practices, you can ensure that your use of the replace() method is effective and easy to understand. This will not only improve your own coding experience but also make it easier for others to work with your code.
When using Python’s replace function, it’s important to follow some key tips to make your code cleaner and more efficient. Always remember to check if the string you want to change exists before trying to replace it. This can save you from errors and make your program run smoother. For more helpful coding tips and to start your journey in programming, visit our website today!
Conclusion
In summary, getting good at string manipulation is really important for anyone who codes in Python. From simple tasks like putting strings together to more complex ones like formatting, Python gives you many tools to work with text. By learning the basic operations and exploring the different string methods, you’ll be ready to handle various programming challenges with ease. So, keep practicing and experimenting with Python strings, and you’ll discover just how powerful they can be in your coding journey!
Frequently Asked Questions
What does the Python replace function do?
The replace function in Python changes certain words or parts of a string into something else.
How do you use the replace function?
You use it by writing the string you want to change followed by .replace(old, new). Here, ‘old’ is what you want to replace, and ‘new’ is what you want to replace it with.
Can you replace more than one word at a time?
No, the replace function can only change one word or part of a string at a time. However, you can call it multiple times.
What happens if the word you want to replace isn’t in the string?
If the word isn’t found, the original string stays the same, and nothing changes.
Is the replace function case-sensitive?
Yes, it is. This means that ‘apple’ and ‘Apple’ are seen as different words.
Can you use the replace function with special characters?
Yes, you can use it with special characters like punctuation marks or spaces.
How do you replace a word in a string without changing the original string?
You can save the result of the replace function to a new variable. The original string will remain unchanged.
Is it possible to replace text in a case-insensitive way?
You can make it case-insensitive by converting the string to all lower or upper case first, but you’ll need to handle the original case separately.